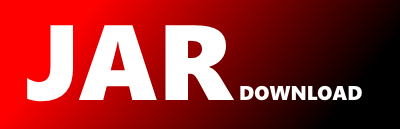
com.amazonaws.services.gamelift.model.CreateFleetRequest Maven / Gradle / Ivy
/*
* Copyright 2013-2018 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.gamelift.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* Represents the input for a request action.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class CreateFleetRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* Descriptive label that is associated with a fleet. Fleet names do not need to be unique.
*
*/
private String name;
/**
*
* Human-readable description of a fleet.
*
*/
private String description;
/**
*
* Unique identifier for a build to be deployed on the new fleet. The build must have been successfully uploaded to
* Amazon GameLift and be in a READY
status. This fleet setting cannot be changed once the fleet is
* created.
*
*/
private String buildId;
/**
*
* This parameter is no longer used. Instead, specify a server launch path using the
* RuntimeConfiguration
parameter. (Requests that specify a server launch path and launch parameters
* instead of a run-time configuration will continue to work.)
*
*/
private String serverLaunchPath;
/**
*
* This parameter is no longer used. Instead, specify server launch parameters in the
* RuntimeConfiguration
parameter. (Requests that specify a server launch path and launch parameters
* instead of a run-time configuration will continue to work.)
*
*/
private String serverLaunchParameters;
/**
*
* This parameter is no longer used. Instead, to specify where Amazon GameLift should store log files once a server
* process shuts down, use the Amazon GameLift server API ProcessReady()
and specify one or more
* directory paths in logParameters
. See more information in the Server API Reference.
*
*/
private java.util.List logPaths;
/**
*
* Name of an EC2 instance type that is supported in Amazon GameLift. A fleet instance type determines the computing
* resources of each instance in the fleet, including CPU, memory, storage, and networking capacity. Amazon GameLift
* supports the following EC2 instance types. See Amazon EC2
* Instance Types for detailed descriptions.
*
*/
private String eC2InstanceType;
/**
*
* Range of IP addresses and port settings that permit inbound traffic to access server processes running on the
* fleet. If no inbound permissions are set, including both IP address range and port range, the server processes in
* the fleet cannot accept connections. You can specify one or more sets of permissions for a fleet.
*
*/
private java.util.List eC2InboundPermissions;
/**
*
* Game session protection policy to apply to all instances in this fleet. If this parameter is not set, instances
* in this fleet default to no protection. You can change a fleet's protection policy using
* UpdateFleetAttributes, but this change will only affect sessions created after the policy change. You can
* also set protection for individual instances using UpdateGameSession.
*
*
* -
*
* NoProtection -- The game session can be terminated during a scale-down event.
*
*
* -
*
* FullProtection -- If the game session is in an ACTIVE
status, it cannot be terminated during
* a scale-down event.
*
*
*
*/
private String newGameSessionProtectionPolicy;
/**
*
* Instructions for launching server processes on each instance in the fleet. The run-time configuration for a fleet
* has a collection of server process configurations, one for each type of server process to run on an instance. A
* server process configuration specifies the location of the server executable, launch parameters, and the number
* of concurrent processes with that configuration to maintain on each instance. A CreateFleet request must include
* a run-time configuration with at least one server process configuration; otherwise the request fails with an
* invalid request exception. (This parameter replaces the parameters ServerLaunchPath
and
* ServerLaunchParameters
; requests that contain values for these parameters instead of a run-time
* configuration will continue to work.)
*
*/
private RuntimeConfiguration runtimeConfiguration;
/**
*
* Policy that limits the number of game sessions an individual player can create over a span of time for this
* fleet.
*
*/
private ResourceCreationLimitPolicy resourceCreationLimitPolicy;
/**
*
* Name of a metric group to add this fleet to. A metric group tracks metrics across all fleets in the group. Use an
* existing metric group name to add this fleet to the group, or use a new name to create a new metric group. A
* fleet can only be included in one metric group at a time.
*
*/
private java.util.List metricGroups;
/**
*
* Unique identifier for the AWS account with the VPC that you want to peer your Amazon GameLift fleet with. You can
* find your Account ID in the AWS Management Console under account settings.
*
*/
private String peerVpcAwsAccountId;
/**
*
* Unique identifier for a VPC with resources to be accessed by your Amazon GameLift fleet. The VPC must be in the
* same region where your fleet is deployed. To get VPC information, including IDs, use the Virtual Private Cloud
* service tools, including the VPC Dashboard in the AWS Management Console.
*
*/
private String peerVpcId;
/**
*
* Indicates whether to use on-demand instances or spot instances for this fleet. If empty, the default is
* ON_DEMAND. Both categories of instances use identical hardware and configurations, based on the instance type
* selected for this fleet. You can acquire on-demand instances at any time for a fixed price and keep them as long
* as you need them. Spot instances have lower prices, but spot pricing is variable, and while in use they can be
* interrupted (with a two-minute notification). Learn more about Amazon GameLift spot instances with at Choose Computing
* Resources.
*
*/
private String fleetType;
/**
*
* Descriptive label that is associated with a fleet. Fleet names do not need to be unique.
*
*
* @param name
* Descriptive label that is associated with a fleet. Fleet names do not need to be unique.
*/
public void setName(String name) {
this.name = name;
}
/**
*
* Descriptive label that is associated with a fleet. Fleet names do not need to be unique.
*
*
* @return Descriptive label that is associated with a fleet. Fleet names do not need to be unique.
*/
public String getName() {
return this.name;
}
/**
*
* Descriptive label that is associated with a fleet. Fleet names do not need to be unique.
*
*
* @param name
* Descriptive label that is associated with a fleet. Fleet names do not need to be unique.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateFleetRequest withName(String name) {
setName(name);
return this;
}
/**
*
* Human-readable description of a fleet.
*
*
* @param description
* Human-readable description of a fleet.
*/
public void setDescription(String description) {
this.description = description;
}
/**
*
* Human-readable description of a fleet.
*
*
* @return Human-readable description of a fleet.
*/
public String getDescription() {
return this.description;
}
/**
*
* Human-readable description of a fleet.
*
*
* @param description
* Human-readable description of a fleet.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateFleetRequest withDescription(String description) {
setDescription(description);
return this;
}
/**
*
* Unique identifier for a build to be deployed on the new fleet. The build must have been successfully uploaded to
* Amazon GameLift and be in a READY
status. This fleet setting cannot be changed once the fleet is
* created.
*
*
* @param buildId
* Unique identifier for a build to be deployed on the new fleet. The build must have been successfully
* uploaded to Amazon GameLift and be in a READY
status. This fleet setting cannot be changed
* once the fleet is created.
*/
public void setBuildId(String buildId) {
this.buildId = buildId;
}
/**
*
* Unique identifier for a build to be deployed on the new fleet. The build must have been successfully uploaded to
* Amazon GameLift and be in a READY
status. This fleet setting cannot be changed once the fleet is
* created.
*
*
* @return Unique identifier for a build to be deployed on the new fleet. The build must have been successfully
* uploaded to Amazon GameLift and be in a READY
status. This fleet setting cannot be changed
* once the fleet is created.
*/
public String getBuildId() {
return this.buildId;
}
/**
*
* Unique identifier for a build to be deployed on the new fleet. The build must have been successfully uploaded to
* Amazon GameLift and be in a READY
status. This fleet setting cannot be changed once the fleet is
* created.
*
*
* @param buildId
* Unique identifier for a build to be deployed on the new fleet. The build must have been successfully
* uploaded to Amazon GameLift and be in a READY
status. This fleet setting cannot be changed
* once the fleet is created.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateFleetRequest withBuildId(String buildId) {
setBuildId(buildId);
return this;
}
/**
*
* This parameter is no longer used. Instead, specify a server launch path using the
* RuntimeConfiguration
parameter. (Requests that specify a server launch path and launch parameters
* instead of a run-time configuration will continue to work.)
*
*
* @param serverLaunchPath
* This parameter is no longer used. Instead, specify a server launch path using the
* RuntimeConfiguration
parameter. (Requests that specify a server launch path and launch
* parameters instead of a run-time configuration will continue to work.)
*/
public void setServerLaunchPath(String serverLaunchPath) {
this.serverLaunchPath = serverLaunchPath;
}
/**
*
* This parameter is no longer used. Instead, specify a server launch path using the
* RuntimeConfiguration
parameter. (Requests that specify a server launch path and launch parameters
* instead of a run-time configuration will continue to work.)
*
*
* @return This parameter is no longer used. Instead, specify a server launch path using the
* RuntimeConfiguration
parameter. (Requests that specify a server launch path and launch
* parameters instead of a run-time configuration will continue to work.)
*/
public String getServerLaunchPath() {
return this.serverLaunchPath;
}
/**
*
* This parameter is no longer used. Instead, specify a server launch path using the
* RuntimeConfiguration
parameter. (Requests that specify a server launch path and launch parameters
* instead of a run-time configuration will continue to work.)
*
*
* @param serverLaunchPath
* This parameter is no longer used. Instead, specify a server launch path using the
* RuntimeConfiguration
parameter. (Requests that specify a server launch path and launch
* parameters instead of a run-time configuration will continue to work.)
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateFleetRequest withServerLaunchPath(String serverLaunchPath) {
setServerLaunchPath(serverLaunchPath);
return this;
}
/**
*
* This parameter is no longer used. Instead, specify server launch parameters in the
* RuntimeConfiguration
parameter. (Requests that specify a server launch path and launch parameters
* instead of a run-time configuration will continue to work.)
*
*
* @param serverLaunchParameters
* This parameter is no longer used. Instead, specify server launch parameters in the
* RuntimeConfiguration
parameter. (Requests that specify a server launch path and launch
* parameters instead of a run-time configuration will continue to work.)
*/
public void setServerLaunchParameters(String serverLaunchParameters) {
this.serverLaunchParameters = serverLaunchParameters;
}
/**
*
* This parameter is no longer used. Instead, specify server launch parameters in the
* RuntimeConfiguration
parameter. (Requests that specify a server launch path and launch parameters
* instead of a run-time configuration will continue to work.)
*
*
* @return This parameter is no longer used. Instead, specify server launch parameters in the
* RuntimeConfiguration
parameter. (Requests that specify a server launch path and launch
* parameters instead of a run-time configuration will continue to work.)
*/
public String getServerLaunchParameters() {
return this.serverLaunchParameters;
}
/**
*
* This parameter is no longer used. Instead, specify server launch parameters in the
* RuntimeConfiguration
parameter. (Requests that specify a server launch path and launch parameters
* instead of a run-time configuration will continue to work.)
*
*
* @param serverLaunchParameters
* This parameter is no longer used. Instead, specify server launch parameters in the
* RuntimeConfiguration
parameter. (Requests that specify a server launch path and launch
* parameters instead of a run-time configuration will continue to work.)
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateFleetRequest withServerLaunchParameters(String serverLaunchParameters) {
setServerLaunchParameters(serverLaunchParameters);
return this;
}
/**
*
* This parameter is no longer used. Instead, to specify where Amazon GameLift should store log files once a server
* process shuts down, use the Amazon GameLift server API ProcessReady()
and specify one or more
* directory paths in logParameters
. See more information in the Server API Reference.
*
*
* @return This parameter is no longer used. Instead, to specify where Amazon GameLift should store log files once a
* server process shuts down, use the Amazon GameLift server API ProcessReady()
and specify one
* or more directory paths in logParameters
. See more information in the Server API Reference.
*/
public java.util.List getLogPaths() {
return logPaths;
}
/**
*
* This parameter is no longer used. Instead, to specify where Amazon GameLift should store log files once a server
* process shuts down, use the Amazon GameLift server API ProcessReady()
and specify one or more
* directory paths in logParameters
. See more information in the Server API Reference.
*
*
* @param logPaths
* This parameter is no longer used. Instead, to specify where Amazon GameLift should store log files once a
* server process shuts down, use the Amazon GameLift server API ProcessReady()
and specify one
* or more directory paths in logParameters
. See more information in the Server API Reference.
*/
public void setLogPaths(java.util.Collection logPaths) {
if (logPaths == null) {
this.logPaths = null;
return;
}
this.logPaths = new java.util.ArrayList(logPaths);
}
/**
*
* This parameter is no longer used. Instead, to specify where Amazon GameLift should store log files once a server
* process shuts down, use the Amazon GameLift server API ProcessReady()
and specify one or more
* directory paths in logParameters
. See more information in the Server API Reference.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setLogPaths(java.util.Collection)} or {@link #withLogPaths(java.util.Collection)} if you want to override
* the existing values.
*
*
* @param logPaths
* This parameter is no longer used. Instead, to specify where Amazon GameLift should store log files once a
* server process shuts down, use the Amazon GameLift server API ProcessReady()
and specify one
* or more directory paths in logParameters
. See more information in the Server API Reference.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateFleetRequest withLogPaths(String... logPaths) {
if (this.logPaths == null) {
setLogPaths(new java.util.ArrayList(logPaths.length));
}
for (String ele : logPaths) {
this.logPaths.add(ele);
}
return this;
}
/**
*
* This parameter is no longer used. Instead, to specify where Amazon GameLift should store log files once a server
* process shuts down, use the Amazon GameLift server API ProcessReady()
and specify one or more
* directory paths in logParameters
. See more information in the Server API Reference.
*
*
* @param logPaths
* This parameter is no longer used. Instead, to specify where Amazon GameLift should store log files once a
* server process shuts down, use the Amazon GameLift server API ProcessReady()
and specify one
* or more directory paths in logParameters
. See more information in the Server API Reference.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateFleetRequest withLogPaths(java.util.Collection logPaths) {
setLogPaths(logPaths);
return this;
}
/**
*
* Name of an EC2 instance type that is supported in Amazon GameLift. A fleet instance type determines the computing
* resources of each instance in the fleet, including CPU, memory, storage, and networking capacity. Amazon GameLift
* supports the following EC2 instance types. See Amazon EC2
* Instance Types for detailed descriptions.
*
*
* @param eC2InstanceType
* Name of an EC2 instance type that is supported in Amazon GameLift. A fleet instance type determines the
* computing resources of each instance in the fleet, including CPU, memory, storage, and networking
* capacity. Amazon GameLift supports the following EC2 instance types. See Amazon EC2 Instance Types for detailed descriptions.
* @see EC2InstanceType
*/
public void setEC2InstanceType(String eC2InstanceType) {
this.eC2InstanceType = eC2InstanceType;
}
/**
*
* Name of an EC2 instance type that is supported in Amazon GameLift. A fleet instance type determines the computing
* resources of each instance in the fleet, including CPU, memory, storage, and networking capacity. Amazon GameLift
* supports the following EC2 instance types. See Amazon EC2
* Instance Types for detailed descriptions.
*
*
* @return Name of an EC2 instance type that is supported in Amazon GameLift. A fleet instance type determines the
* computing resources of each instance in the fleet, including CPU, memory, storage, and networking
* capacity. Amazon GameLift supports the following EC2 instance types. See Amazon EC2 Instance Types for detailed descriptions.
* @see EC2InstanceType
*/
public String getEC2InstanceType() {
return this.eC2InstanceType;
}
/**
*
* Name of an EC2 instance type that is supported in Amazon GameLift. A fleet instance type determines the computing
* resources of each instance in the fleet, including CPU, memory, storage, and networking capacity. Amazon GameLift
* supports the following EC2 instance types. See Amazon EC2
* Instance Types for detailed descriptions.
*
*
* @param eC2InstanceType
* Name of an EC2 instance type that is supported in Amazon GameLift. A fleet instance type determines the
* computing resources of each instance in the fleet, including CPU, memory, storage, and networking
* capacity. Amazon GameLift supports the following EC2 instance types. See Amazon EC2 Instance Types for detailed descriptions.
* @return Returns a reference to this object so that method calls can be chained together.
* @see EC2InstanceType
*/
public CreateFleetRequest withEC2InstanceType(String eC2InstanceType) {
setEC2InstanceType(eC2InstanceType);
return this;
}
/**
*
* Name of an EC2 instance type that is supported in Amazon GameLift. A fleet instance type determines the computing
* resources of each instance in the fleet, including CPU, memory, storage, and networking capacity. Amazon GameLift
* supports the following EC2 instance types. See Amazon EC2
* Instance Types for detailed descriptions.
*
*
* @param eC2InstanceType
* Name of an EC2 instance type that is supported in Amazon GameLift. A fleet instance type determines the
* computing resources of each instance in the fleet, including CPU, memory, storage, and networking
* capacity. Amazon GameLift supports the following EC2 instance types. See Amazon EC2 Instance Types for detailed descriptions.
* @see EC2InstanceType
*/
public void setEC2InstanceType(EC2InstanceType eC2InstanceType) {
withEC2InstanceType(eC2InstanceType);
}
/**
*
* Name of an EC2 instance type that is supported in Amazon GameLift. A fleet instance type determines the computing
* resources of each instance in the fleet, including CPU, memory, storage, and networking capacity. Amazon GameLift
* supports the following EC2 instance types. See Amazon EC2
* Instance Types for detailed descriptions.
*
*
* @param eC2InstanceType
* Name of an EC2 instance type that is supported in Amazon GameLift. A fleet instance type determines the
* computing resources of each instance in the fleet, including CPU, memory, storage, and networking
* capacity. Amazon GameLift supports the following EC2 instance types. See Amazon EC2 Instance Types for detailed descriptions.
* @return Returns a reference to this object so that method calls can be chained together.
* @see EC2InstanceType
*/
public CreateFleetRequest withEC2InstanceType(EC2InstanceType eC2InstanceType) {
this.eC2InstanceType = eC2InstanceType.toString();
return this;
}
/**
*
* Range of IP addresses and port settings that permit inbound traffic to access server processes running on the
* fleet. If no inbound permissions are set, including both IP address range and port range, the server processes in
* the fleet cannot accept connections. You can specify one or more sets of permissions for a fleet.
*
*
* @return Range of IP addresses and port settings that permit inbound traffic to access server processes running on
* the fleet. If no inbound permissions are set, including both IP address range and port range, the server
* processes in the fleet cannot accept connections. You can specify one or more sets of permissions for a
* fleet.
*/
public java.util.List getEC2InboundPermissions() {
return eC2InboundPermissions;
}
/**
*
* Range of IP addresses and port settings that permit inbound traffic to access server processes running on the
* fleet. If no inbound permissions are set, including both IP address range and port range, the server processes in
* the fleet cannot accept connections. You can specify one or more sets of permissions for a fleet.
*
*
* @param eC2InboundPermissions
* Range of IP addresses and port settings that permit inbound traffic to access server processes running on
* the fleet. If no inbound permissions are set, including both IP address range and port range, the server
* processes in the fleet cannot accept connections. You can specify one or more sets of permissions for a
* fleet.
*/
public void setEC2InboundPermissions(java.util.Collection eC2InboundPermissions) {
if (eC2InboundPermissions == null) {
this.eC2InboundPermissions = null;
return;
}
this.eC2InboundPermissions = new java.util.ArrayList(eC2InboundPermissions);
}
/**
*
* Range of IP addresses and port settings that permit inbound traffic to access server processes running on the
* fleet. If no inbound permissions are set, including both IP address range and port range, the server processes in
* the fleet cannot accept connections. You can specify one or more sets of permissions for a fleet.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setEC2InboundPermissions(java.util.Collection)} or
* {@link #withEC2InboundPermissions(java.util.Collection)} if you want to override the existing values.
*
*
* @param eC2InboundPermissions
* Range of IP addresses and port settings that permit inbound traffic to access server processes running on
* the fleet. If no inbound permissions are set, including both IP address range and port range, the server
* processes in the fleet cannot accept connections. You can specify one or more sets of permissions for a
* fleet.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateFleetRequest withEC2InboundPermissions(IpPermission... eC2InboundPermissions) {
if (this.eC2InboundPermissions == null) {
setEC2InboundPermissions(new java.util.ArrayList(eC2InboundPermissions.length));
}
for (IpPermission ele : eC2InboundPermissions) {
this.eC2InboundPermissions.add(ele);
}
return this;
}
/**
*
* Range of IP addresses and port settings that permit inbound traffic to access server processes running on the
* fleet. If no inbound permissions are set, including both IP address range and port range, the server processes in
* the fleet cannot accept connections. You can specify one or more sets of permissions for a fleet.
*
*
* @param eC2InboundPermissions
* Range of IP addresses and port settings that permit inbound traffic to access server processes running on
* the fleet. If no inbound permissions are set, including both IP address range and port range, the server
* processes in the fleet cannot accept connections. You can specify one or more sets of permissions for a
* fleet.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateFleetRequest withEC2InboundPermissions(java.util.Collection eC2InboundPermissions) {
setEC2InboundPermissions(eC2InboundPermissions);
return this;
}
/**
*
* Game session protection policy to apply to all instances in this fleet. If this parameter is not set, instances
* in this fleet default to no protection. You can change a fleet's protection policy using
* UpdateFleetAttributes, but this change will only affect sessions created after the policy change. You can
* also set protection for individual instances using UpdateGameSession.
*
*
* -
*
* NoProtection -- The game session can be terminated during a scale-down event.
*
*
* -
*
* FullProtection -- If the game session is in an ACTIVE
status, it cannot be terminated during
* a scale-down event.
*
*
*
*
* @param newGameSessionProtectionPolicy
* Game session protection policy to apply to all instances in this fleet. If this parameter is not set,
* instances in this fleet default to no protection. You can change a fleet's protection policy using
* UpdateFleetAttributes, but this change will only affect sessions created after the policy change.
* You can also set protection for individual instances using UpdateGameSession.
*
* -
*
* NoProtection -- The game session can be terminated during a scale-down event.
*
*
* -
*
* FullProtection -- If the game session is in an ACTIVE
status, it cannot be terminated
* during a scale-down event.
*
*
* @see ProtectionPolicy
*/
public void setNewGameSessionProtectionPolicy(String newGameSessionProtectionPolicy) {
this.newGameSessionProtectionPolicy = newGameSessionProtectionPolicy;
}
/**
*
* Game session protection policy to apply to all instances in this fleet. If this parameter is not set, instances
* in this fleet default to no protection. You can change a fleet's protection policy using
* UpdateFleetAttributes, but this change will only affect sessions created after the policy change. You can
* also set protection for individual instances using UpdateGameSession.
*
*
* -
*
* NoProtection -- The game session can be terminated during a scale-down event.
*
*
* -
*
* FullProtection -- If the game session is in an ACTIVE
status, it cannot be terminated during
* a scale-down event.
*
*
*
*
* @return Game session protection policy to apply to all instances in this fleet. If this parameter is not set,
* instances in this fleet default to no protection. You can change a fleet's protection policy using
* UpdateFleetAttributes, but this change will only affect sessions created after the policy change.
* You can also set protection for individual instances using UpdateGameSession.
*
* -
*
* NoProtection -- The game session can be terminated during a scale-down event.
*
*
* -
*
* FullProtection -- If the game session is in an ACTIVE
status, it cannot be terminated
* during a scale-down event.
*
*
* @see ProtectionPolicy
*/
public String getNewGameSessionProtectionPolicy() {
return this.newGameSessionProtectionPolicy;
}
/**
*
* Game session protection policy to apply to all instances in this fleet. If this parameter is not set, instances
* in this fleet default to no protection. You can change a fleet's protection policy using
* UpdateFleetAttributes, but this change will only affect sessions created after the policy change. You can
* also set protection for individual instances using UpdateGameSession.
*
*
* -
*
* NoProtection -- The game session can be terminated during a scale-down event.
*
*
* -
*
* FullProtection -- If the game session is in an ACTIVE
status, it cannot be terminated during
* a scale-down event.
*
*
*
*
* @param newGameSessionProtectionPolicy
* Game session protection policy to apply to all instances in this fleet. If this parameter is not set,
* instances in this fleet default to no protection. You can change a fleet's protection policy using
* UpdateFleetAttributes, but this change will only affect sessions created after the policy change.
* You can also set protection for individual instances using UpdateGameSession.
*
* -
*
* NoProtection -- The game session can be terminated during a scale-down event.
*
*
* -
*
* FullProtection -- If the game session is in an ACTIVE
status, it cannot be terminated
* during a scale-down event.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see ProtectionPolicy
*/
public CreateFleetRequest withNewGameSessionProtectionPolicy(String newGameSessionProtectionPolicy) {
setNewGameSessionProtectionPolicy(newGameSessionProtectionPolicy);
return this;
}
/**
*
* Game session protection policy to apply to all instances in this fleet. If this parameter is not set, instances
* in this fleet default to no protection. You can change a fleet's protection policy using
* UpdateFleetAttributes, but this change will only affect sessions created after the policy change. You can
* also set protection for individual instances using UpdateGameSession.
*
*
* -
*
* NoProtection -- The game session can be terminated during a scale-down event.
*
*
* -
*
* FullProtection -- If the game session is in an ACTIVE
status, it cannot be terminated during
* a scale-down event.
*
*
*
*
* @param newGameSessionProtectionPolicy
* Game session protection policy to apply to all instances in this fleet. If this parameter is not set,
* instances in this fleet default to no protection. You can change a fleet's protection policy using
* UpdateFleetAttributes, but this change will only affect sessions created after the policy change.
* You can also set protection for individual instances using UpdateGameSession.
*
* -
*
* NoProtection -- The game session can be terminated during a scale-down event.
*
*
* -
*
* FullProtection -- If the game session is in an ACTIVE
status, it cannot be terminated
* during a scale-down event.
*
*
* @see ProtectionPolicy
*/
public void setNewGameSessionProtectionPolicy(ProtectionPolicy newGameSessionProtectionPolicy) {
withNewGameSessionProtectionPolicy(newGameSessionProtectionPolicy);
}
/**
*
* Game session protection policy to apply to all instances in this fleet. If this parameter is not set, instances
* in this fleet default to no protection. You can change a fleet's protection policy using
* UpdateFleetAttributes, but this change will only affect sessions created after the policy change. You can
* also set protection for individual instances using UpdateGameSession.
*
*
* -
*
* NoProtection -- The game session can be terminated during a scale-down event.
*
*
* -
*
* FullProtection -- If the game session is in an ACTIVE
status, it cannot be terminated during
* a scale-down event.
*
*
*
*
* @param newGameSessionProtectionPolicy
* Game session protection policy to apply to all instances in this fleet. If this parameter is not set,
* instances in this fleet default to no protection. You can change a fleet's protection policy using
* UpdateFleetAttributes, but this change will only affect sessions created after the policy change.
* You can also set protection for individual instances using UpdateGameSession.
*
* -
*
* NoProtection -- The game session can be terminated during a scale-down event.
*
*
* -
*
* FullProtection -- If the game session is in an ACTIVE
status, it cannot be terminated
* during a scale-down event.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see ProtectionPolicy
*/
public CreateFleetRequest withNewGameSessionProtectionPolicy(ProtectionPolicy newGameSessionProtectionPolicy) {
this.newGameSessionProtectionPolicy = newGameSessionProtectionPolicy.toString();
return this;
}
/**
*
* Instructions for launching server processes on each instance in the fleet. The run-time configuration for a fleet
* has a collection of server process configurations, one for each type of server process to run on an instance. A
* server process configuration specifies the location of the server executable, launch parameters, and the number
* of concurrent processes with that configuration to maintain on each instance. A CreateFleet request must include
* a run-time configuration with at least one server process configuration; otherwise the request fails with an
* invalid request exception. (This parameter replaces the parameters ServerLaunchPath
and
* ServerLaunchParameters
; requests that contain values for these parameters instead of a run-time
* configuration will continue to work.)
*
*
* @param runtimeConfiguration
* Instructions for launching server processes on each instance in the fleet. The run-time configuration for
* a fleet has a collection of server process configurations, one for each type of server process to run on
* an instance. A server process configuration specifies the location of the server executable, launch
* parameters, and the number of concurrent processes with that configuration to maintain on each instance. A
* CreateFleet request must include a run-time configuration with at least one server process configuration;
* otherwise the request fails with an invalid request exception. (This parameter replaces the parameters
* ServerLaunchPath
and ServerLaunchParameters
; requests that contain values for
* these parameters instead of a run-time configuration will continue to work.)
*/
public void setRuntimeConfiguration(RuntimeConfiguration runtimeConfiguration) {
this.runtimeConfiguration = runtimeConfiguration;
}
/**
*
* Instructions for launching server processes on each instance in the fleet. The run-time configuration for a fleet
* has a collection of server process configurations, one for each type of server process to run on an instance. A
* server process configuration specifies the location of the server executable, launch parameters, and the number
* of concurrent processes with that configuration to maintain on each instance. A CreateFleet request must include
* a run-time configuration with at least one server process configuration; otherwise the request fails with an
* invalid request exception. (This parameter replaces the parameters ServerLaunchPath
and
* ServerLaunchParameters
; requests that contain values for these parameters instead of a run-time
* configuration will continue to work.)
*
*
* @return Instructions for launching server processes on each instance in the fleet. The run-time configuration for
* a fleet has a collection of server process configurations, one for each type of server process to run on
* an instance. A server process configuration specifies the location of the server executable, launch
* parameters, and the number of concurrent processes with that configuration to maintain on each instance.
* A CreateFleet request must include a run-time configuration with at least one server process
* configuration; otherwise the request fails with an invalid request exception. (This parameter replaces
* the parameters ServerLaunchPath
and ServerLaunchParameters
; requests that
* contain values for these parameters instead of a run-time configuration will continue to work.)
*/
public RuntimeConfiguration getRuntimeConfiguration() {
return this.runtimeConfiguration;
}
/**
*
* Instructions for launching server processes on each instance in the fleet. The run-time configuration for a fleet
* has a collection of server process configurations, one for each type of server process to run on an instance. A
* server process configuration specifies the location of the server executable, launch parameters, and the number
* of concurrent processes with that configuration to maintain on each instance. A CreateFleet request must include
* a run-time configuration with at least one server process configuration; otherwise the request fails with an
* invalid request exception. (This parameter replaces the parameters ServerLaunchPath
and
* ServerLaunchParameters
; requests that contain values for these parameters instead of a run-time
* configuration will continue to work.)
*
*
* @param runtimeConfiguration
* Instructions for launching server processes on each instance in the fleet. The run-time configuration for
* a fleet has a collection of server process configurations, one for each type of server process to run on
* an instance. A server process configuration specifies the location of the server executable, launch
* parameters, and the number of concurrent processes with that configuration to maintain on each instance. A
* CreateFleet request must include a run-time configuration with at least one server process configuration;
* otherwise the request fails with an invalid request exception. (This parameter replaces the parameters
* ServerLaunchPath
and ServerLaunchParameters
; requests that contain values for
* these parameters instead of a run-time configuration will continue to work.)
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateFleetRequest withRuntimeConfiguration(RuntimeConfiguration runtimeConfiguration) {
setRuntimeConfiguration(runtimeConfiguration);
return this;
}
/**
*
* Policy that limits the number of game sessions an individual player can create over a span of time for this
* fleet.
*
*
* @param resourceCreationLimitPolicy
* Policy that limits the number of game sessions an individual player can create over a span of time for
* this fleet.
*/
public void setResourceCreationLimitPolicy(ResourceCreationLimitPolicy resourceCreationLimitPolicy) {
this.resourceCreationLimitPolicy = resourceCreationLimitPolicy;
}
/**
*
* Policy that limits the number of game sessions an individual player can create over a span of time for this
* fleet.
*
*
* @return Policy that limits the number of game sessions an individual player can create over a span of time for
* this fleet.
*/
public ResourceCreationLimitPolicy getResourceCreationLimitPolicy() {
return this.resourceCreationLimitPolicy;
}
/**
*
* Policy that limits the number of game sessions an individual player can create over a span of time for this
* fleet.
*
*
* @param resourceCreationLimitPolicy
* Policy that limits the number of game sessions an individual player can create over a span of time for
* this fleet.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateFleetRequest withResourceCreationLimitPolicy(ResourceCreationLimitPolicy resourceCreationLimitPolicy) {
setResourceCreationLimitPolicy(resourceCreationLimitPolicy);
return this;
}
/**
*
* Name of a metric group to add this fleet to. A metric group tracks metrics across all fleets in the group. Use an
* existing metric group name to add this fleet to the group, or use a new name to create a new metric group. A
* fleet can only be included in one metric group at a time.
*
*
* @return Name of a metric group to add this fleet to. A metric group tracks metrics across all fleets in the
* group. Use an existing metric group name to add this fleet to the group, or use a new name to create a
* new metric group. A fleet can only be included in one metric group at a time.
*/
public java.util.List getMetricGroups() {
return metricGroups;
}
/**
*
* Name of a metric group to add this fleet to. A metric group tracks metrics across all fleets in the group. Use an
* existing metric group name to add this fleet to the group, or use a new name to create a new metric group. A
* fleet can only be included in one metric group at a time.
*
*
* @param metricGroups
* Name of a metric group to add this fleet to. A metric group tracks metrics across all fleets in the group.
* Use an existing metric group name to add this fleet to the group, or use a new name to create a new metric
* group. A fleet can only be included in one metric group at a time.
*/
public void setMetricGroups(java.util.Collection metricGroups) {
if (metricGroups == null) {
this.metricGroups = null;
return;
}
this.metricGroups = new java.util.ArrayList(metricGroups);
}
/**
*
* Name of a metric group to add this fleet to. A metric group tracks metrics across all fleets in the group. Use an
* existing metric group name to add this fleet to the group, or use a new name to create a new metric group. A
* fleet can only be included in one metric group at a time.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setMetricGroups(java.util.Collection)} or {@link #withMetricGroups(java.util.Collection)} if you want to
* override the existing values.
*
*
* @param metricGroups
* Name of a metric group to add this fleet to. A metric group tracks metrics across all fleets in the group.
* Use an existing metric group name to add this fleet to the group, or use a new name to create a new metric
* group. A fleet can only be included in one metric group at a time.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateFleetRequest withMetricGroups(String... metricGroups) {
if (this.metricGroups == null) {
setMetricGroups(new java.util.ArrayList(metricGroups.length));
}
for (String ele : metricGroups) {
this.metricGroups.add(ele);
}
return this;
}
/**
*
* Name of a metric group to add this fleet to. A metric group tracks metrics across all fleets in the group. Use an
* existing metric group name to add this fleet to the group, or use a new name to create a new metric group. A
* fleet can only be included in one metric group at a time.
*
*
* @param metricGroups
* Name of a metric group to add this fleet to. A metric group tracks metrics across all fleets in the group.
* Use an existing metric group name to add this fleet to the group, or use a new name to create a new metric
* group. A fleet can only be included in one metric group at a time.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateFleetRequest withMetricGroups(java.util.Collection metricGroups) {
setMetricGroups(metricGroups);
return this;
}
/**
*
* Unique identifier for the AWS account with the VPC that you want to peer your Amazon GameLift fleet with. You can
* find your Account ID in the AWS Management Console under account settings.
*
*
* @param peerVpcAwsAccountId
* Unique identifier for the AWS account with the VPC that you want to peer your Amazon GameLift fleet with.
* You can find your Account ID in the AWS Management Console under account settings.
*/
public void setPeerVpcAwsAccountId(String peerVpcAwsAccountId) {
this.peerVpcAwsAccountId = peerVpcAwsAccountId;
}
/**
*
* Unique identifier for the AWS account with the VPC that you want to peer your Amazon GameLift fleet with. You can
* find your Account ID in the AWS Management Console under account settings.
*
*
* @return Unique identifier for the AWS account with the VPC that you want to peer your Amazon GameLift fleet with.
* You can find your Account ID in the AWS Management Console under account settings.
*/
public String getPeerVpcAwsAccountId() {
return this.peerVpcAwsAccountId;
}
/**
*
* Unique identifier for the AWS account with the VPC that you want to peer your Amazon GameLift fleet with. You can
* find your Account ID in the AWS Management Console under account settings.
*
*
* @param peerVpcAwsAccountId
* Unique identifier for the AWS account with the VPC that you want to peer your Amazon GameLift fleet with.
* You can find your Account ID in the AWS Management Console under account settings.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateFleetRequest withPeerVpcAwsAccountId(String peerVpcAwsAccountId) {
setPeerVpcAwsAccountId(peerVpcAwsAccountId);
return this;
}
/**
*
* Unique identifier for a VPC with resources to be accessed by your Amazon GameLift fleet. The VPC must be in the
* same region where your fleet is deployed. To get VPC information, including IDs, use the Virtual Private Cloud
* service tools, including the VPC Dashboard in the AWS Management Console.
*
*
* @param peerVpcId
* Unique identifier for a VPC with resources to be accessed by your Amazon GameLift fleet. The VPC must be
* in the same region where your fleet is deployed. To get VPC information, including IDs, use the Virtual
* Private Cloud service tools, including the VPC Dashboard in the AWS Management Console.
*/
public void setPeerVpcId(String peerVpcId) {
this.peerVpcId = peerVpcId;
}
/**
*
* Unique identifier for a VPC with resources to be accessed by your Amazon GameLift fleet. The VPC must be in the
* same region where your fleet is deployed. To get VPC information, including IDs, use the Virtual Private Cloud
* service tools, including the VPC Dashboard in the AWS Management Console.
*
*
* @return Unique identifier for a VPC with resources to be accessed by your Amazon GameLift fleet. The VPC must be
* in the same region where your fleet is deployed. To get VPC information, including IDs, use the Virtual
* Private Cloud service tools, including the VPC Dashboard in the AWS Management Console.
*/
public String getPeerVpcId() {
return this.peerVpcId;
}
/**
*
* Unique identifier for a VPC with resources to be accessed by your Amazon GameLift fleet. The VPC must be in the
* same region where your fleet is deployed. To get VPC information, including IDs, use the Virtual Private Cloud
* service tools, including the VPC Dashboard in the AWS Management Console.
*
*
* @param peerVpcId
* Unique identifier for a VPC with resources to be accessed by your Amazon GameLift fleet. The VPC must be
* in the same region where your fleet is deployed. To get VPC information, including IDs, use the Virtual
* Private Cloud service tools, including the VPC Dashboard in the AWS Management Console.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateFleetRequest withPeerVpcId(String peerVpcId) {
setPeerVpcId(peerVpcId);
return this;
}
/**
*
* Indicates whether to use on-demand instances or spot instances for this fleet. If empty, the default is
* ON_DEMAND. Both categories of instances use identical hardware and configurations, based on the instance type
* selected for this fleet. You can acquire on-demand instances at any time for a fixed price and keep them as long
* as you need them. Spot instances have lower prices, but spot pricing is variable, and while in use they can be
* interrupted (with a two-minute notification). Learn more about Amazon GameLift spot instances with at Choose Computing
* Resources.
*
*
* @param fleetType
* Indicates whether to use on-demand instances or spot instances for this fleet. If empty, the default is
* ON_DEMAND. Both categories of instances use identical hardware and configurations, based on the instance
* type selected for this fleet. You can acquire on-demand instances at any time for a fixed price and keep
* them as long as you need them. Spot instances have lower prices, but spot pricing is variable, and while
* in use they can be interrupted (with a two-minute notification). Learn more about Amazon GameLift spot
* instances with at Choose
* Computing Resources.
* @see FleetType
*/
public void setFleetType(String fleetType) {
this.fleetType = fleetType;
}
/**
*
* Indicates whether to use on-demand instances or spot instances for this fleet. If empty, the default is
* ON_DEMAND. Both categories of instances use identical hardware and configurations, based on the instance type
* selected for this fleet. You can acquire on-demand instances at any time for a fixed price and keep them as long
* as you need them. Spot instances have lower prices, but spot pricing is variable, and while in use they can be
* interrupted (with a two-minute notification). Learn more about Amazon GameLift spot instances with at Choose Computing
* Resources.
*
*
* @return Indicates whether to use on-demand instances or spot instances for this fleet. If empty, the default is
* ON_DEMAND. Both categories of instances use identical hardware and configurations, based on the instance
* type selected for this fleet. You can acquire on-demand instances at any time for a fixed price and keep
* them as long as you need them. Spot instances have lower prices, but spot pricing is variable, and while
* in use they can be interrupted (with a two-minute notification). Learn more about Amazon GameLift spot
* instances with at Choose
* Computing Resources.
* @see FleetType
*/
public String getFleetType() {
return this.fleetType;
}
/**
*
* Indicates whether to use on-demand instances or spot instances for this fleet. If empty, the default is
* ON_DEMAND. Both categories of instances use identical hardware and configurations, based on the instance type
* selected for this fleet. You can acquire on-demand instances at any time for a fixed price and keep them as long
* as you need them. Spot instances have lower prices, but spot pricing is variable, and while in use they can be
* interrupted (with a two-minute notification). Learn more about Amazon GameLift spot instances with at Choose Computing
* Resources.
*
*
* @param fleetType
* Indicates whether to use on-demand instances or spot instances for this fleet. If empty, the default is
* ON_DEMAND. Both categories of instances use identical hardware and configurations, based on the instance
* type selected for this fleet. You can acquire on-demand instances at any time for a fixed price and keep
* them as long as you need them. Spot instances have lower prices, but spot pricing is variable, and while
* in use they can be interrupted (with a two-minute notification). Learn more about Amazon GameLift spot
* instances with at Choose
* Computing Resources.
* @return Returns a reference to this object so that method calls can be chained together.
* @see FleetType
*/
public CreateFleetRequest withFleetType(String fleetType) {
setFleetType(fleetType);
return this;
}
/**
*
* Indicates whether to use on-demand instances or spot instances for this fleet. If empty, the default is
* ON_DEMAND. Both categories of instances use identical hardware and configurations, based on the instance type
* selected for this fleet. You can acquire on-demand instances at any time for a fixed price and keep them as long
* as you need them. Spot instances have lower prices, but spot pricing is variable, and while in use they can be
* interrupted (with a two-minute notification). Learn more about Amazon GameLift spot instances with at Choose Computing
* Resources.
*
*
* @param fleetType
* Indicates whether to use on-demand instances or spot instances for this fleet. If empty, the default is
* ON_DEMAND. Both categories of instances use identical hardware and configurations, based on the instance
* type selected for this fleet. You can acquire on-demand instances at any time for a fixed price and keep
* them as long as you need them. Spot instances have lower prices, but spot pricing is variable, and while
* in use they can be interrupted (with a two-minute notification). Learn more about Amazon GameLift spot
* instances with at Choose
* Computing Resources.
* @see FleetType
*/
public void setFleetType(FleetType fleetType) {
withFleetType(fleetType);
}
/**
*
* Indicates whether to use on-demand instances or spot instances for this fleet. If empty, the default is
* ON_DEMAND. Both categories of instances use identical hardware and configurations, based on the instance type
* selected for this fleet. You can acquire on-demand instances at any time for a fixed price and keep them as long
* as you need them. Spot instances have lower prices, but spot pricing is variable, and while in use they can be
* interrupted (with a two-minute notification). Learn more about Amazon GameLift spot instances with at Choose Computing
* Resources.
*
*
* @param fleetType
* Indicates whether to use on-demand instances or spot instances for this fleet. If empty, the default is
* ON_DEMAND. Both categories of instances use identical hardware and configurations, based on the instance
* type selected for this fleet. You can acquire on-demand instances at any time for a fixed price and keep
* them as long as you need them. Spot instances have lower prices, but spot pricing is variable, and while
* in use they can be interrupted (with a two-minute notification). Learn more about Amazon GameLift spot
* instances with at Choose
* Computing Resources.
* @return Returns a reference to this object so that method calls can be chained together.
* @see FleetType
*/
public CreateFleetRequest withFleetType(FleetType fleetType) {
this.fleetType = fleetType.toString();
return this;
}
/**
* Returns a string representation of this object; useful for testing and debugging.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getName() != null)
sb.append("Name: ").append(getName()).append(",");
if (getDescription() != null)
sb.append("Description: ").append(getDescription()).append(",");
if (getBuildId() != null)
sb.append("BuildId: ").append(getBuildId()).append(",");
if (getServerLaunchPath() != null)
sb.append("ServerLaunchPath: ").append(getServerLaunchPath()).append(",");
if (getServerLaunchParameters() != null)
sb.append("ServerLaunchParameters: ").append(getServerLaunchParameters()).append(",");
if (getLogPaths() != null)
sb.append("LogPaths: ").append(getLogPaths()).append(",");
if (getEC2InstanceType() != null)
sb.append("EC2InstanceType: ").append(getEC2InstanceType()).append(",");
if (getEC2InboundPermissions() != null)
sb.append("EC2InboundPermissions: ").append(getEC2InboundPermissions()).append(",");
if (getNewGameSessionProtectionPolicy() != null)
sb.append("NewGameSessionProtectionPolicy: ").append(getNewGameSessionProtectionPolicy()).append(",");
if (getRuntimeConfiguration() != null)
sb.append("RuntimeConfiguration: ").append(getRuntimeConfiguration()).append(",");
if (getResourceCreationLimitPolicy() != null)
sb.append("ResourceCreationLimitPolicy: ").append(getResourceCreationLimitPolicy()).append(",");
if (getMetricGroups() != null)
sb.append("MetricGroups: ").append(getMetricGroups()).append(",");
if (getPeerVpcAwsAccountId() != null)
sb.append("PeerVpcAwsAccountId: ").append(getPeerVpcAwsAccountId()).append(",");
if (getPeerVpcId() != null)
sb.append("PeerVpcId: ").append(getPeerVpcId()).append(",");
if (getFleetType() != null)
sb.append("FleetType: ").append(getFleetType());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof CreateFleetRequest == false)
return false;
CreateFleetRequest other = (CreateFleetRequest) obj;
if (other.getName() == null ^ this.getName() == null)
return false;
if (other.getName() != null && other.getName().equals(this.getName()) == false)
return false;
if (other.getDescription() == null ^ this.getDescription() == null)
return false;
if (other.getDescription() != null && other.getDescription().equals(this.getDescription()) == false)
return false;
if (other.getBuildId() == null ^ this.getBuildId() == null)
return false;
if (other.getBuildId() != null && other.getBuildId().equals(this.getBuildId()) == false)
return false;
if (other.getServerLaunchPath() == null ^ this.getServerLaunchPath() == null)
return false;
if (other.getServerLaunchPath() != null && other.getServerLaunchPath().equals(this.getServerLaunchPath()) == false)
return false;
if (other.getServerLaunchParameters() == null ^ this.getServerLaunchParameters() == null)
return false;
if (other.getServerLaunchParameters() != null && other.getServerLaunchParameters().equals(this.getServerLaunchParameters()) == false)
return false;
if (other.getLogPaths() == null ^ this.getLogPaths() == null)
return false;
if (other.getLogPaths() != null && other.getLogPaths().equals(this.getLogPaths()) == false)
return false;
if (other.getEC2InstanceType() == null ^ this.getEC2InstanceType() == null)
return false;
if (other.getEC2InstanceType() != null && other.getEC2InstanceType().equals(this.getEC2InstanceType()) == false)
return false;
if (other.getEC2InboundPermissions() == null ^ this.getEC2InboundPermissions() == null)
return false;
if (other.getEC2InboundPermissions() != null && other.getEC2InboundPermissions().equals(this.getEC2InboundPermissions()) == false)
return false;
if (other.getNewGameSessionProtectionPolicy() == null ^ this.getNewGameSessionProtectionPolicy() == null)
return false;
if (other.getNewGameSessionProtectionPolicy() != null
&& other.getNewGameSessionProtectionPolicy().equals(this.getNewGameSessionProtectionPolicy()) == false)
return false;
if (other.getRuntimeConfiguration() == null ^ this.getRuntimeConfiguration() == null)
return false;
if (other.getRuntimeConfiguration() != null && other.getRuntimeConfiguration().equals(this.getRuntimeConfiguration()) == false)
return false;
if (other.getResourceCreationLimitPolicy() == null ^ this.getResourceCreationLimitPolicy() == null)
return false;
if (other.getResourceCreationLimitPolicy() != null && other.getResourceCreationLimitPolicy().equals(this.getResourceCreationLimitPolicy()) == false)
return false;
if (other.getMetricGroups() == null ^ this.getMetricGroups() == null)
return false;
if (other.getMetricGroups() != null && other.getMetricGroups().equals(this.getMetricGroups()) == false)
return false;
if (other.getPeerVpcAwsAccountId() == null ^ this.getPeerVpcAwsAccountId() == null)
return false;
if (other.getPeerVpcAwsAccountId() != null && other.getPeerVpcAwsAccountId().equals(this.getPeerVpcAwsAccountId()) == false)
return false;
if (other.getPeerVpcId() == null ^ this.getPeerVpcId() == null)
return false;
if (other.getPeerVpcId() != null && other.getPeerVpcId().equals(this.getPeerVpcId()) == false)
return false;
if (other.getFleetType() == null ^ this.getFleetType() == null)
return false;
if (other.getFleetType() != null && other.getFleetType().equals(this.getFleetType()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getName() == null) ? 0 : getName().hashCode());
hashCode = prime * hashCode + ((getDescription() == null) ? 0 : getDescription().hashCode());
hashCode = prime * hashCode + ((getBuildId() == null) ? 0 : getBuildId().hashCode());
hashCode = prime * hashCode + ((getServerLaunchPath() == null) ? 0 : getServerLaunchPath().hashCode());
hashCode = prime * hashCode + ((getServerLaunchParameters() == null) ? 0 : getServerLaunchParameters().hashCode());
hashCode = prime * hashCode + ((getLogPaths() == null) ? 0 : getLogPaths().hashCode());
hashCode = prime * hashCode + ((getEC2InstanceType() == null) ? 0 : getEC2InstanceType().hashCode());
hashCode = prime * hashCode + ((getEC2InboundPermissions() == null) ? 0 : getEC2InboundPermissions().hashCode());
hashCode = prime * hashCode + ((getNewGameSessionProtectionPolicy() == null) ? 0 : getNewGameSessionProtectionPolicy().hashCode());
hashCode = prime * hashCode + ((getRuntimeConfiguration() == null) ? 0 : getRuntimeConfiguration().hashCode());
hashCode = prime * hashCode + ((getResourceCreationLimitPolicy() == null) ? 0 : getResourceCreationLimitPolicy().hashCode());
hashCode = prime * hashCode + ((getMetricGroups() == null) ? 0 : getMetricGroups().hashCode());
hashCode = prime * hashCode + ((getPeerVpcAwsAccountId() == null) ? 0 : getPeerVpcAwsAccountId().hashCode());
hashCode = prime * hashCode + ((getPeerVpcId() == null) ? 0 : getPeerVpcId().hashCode());
hashCode = prime * hashCode + ((getFleetType() == null) ? 0 : getFleetType().hashCode());
return hashCode;
}
@Override
public CreateFleetRequest clone() {
return (CreateFleetRequest) super.clone();
}
}