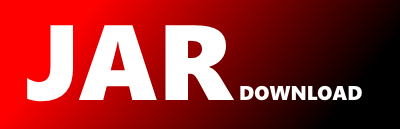
com.amazonaws.services.gamelift.model.CreateMatchmakingConfigurationRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-gamelift Show documentation
/*
* Copyright 2013-2018 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.gamelift.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* Represents the input for a request action.
*
*
* @see AWS API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class CreateMatchmakingConfigurationRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* Unique identifier for a matchmaking configuration. This name is used to identify the configuration associated
* with a matchmaking request or ticket.
*
*/
private String name;
/**
*
* Meaningful description of the matchmaking configuration.
*
*/
private String description;
/**
*
* Amazon Resource Name (ARN) that
* is assigned to a game session queue and uniquely identifies it. Format is
* arn:aws:gamelift:<region>::fleet/fleet-a1234567-b8c9-0d1e-2fa3-b45c6d7e8912
. These queues are
* used when placing game sessions for matches that are created with this matchmaking configuration. Queues can be
* located in any region.
*
*/
private java.util.List gameSessionQueueArns;
/**
*
* Maximum duration, in seconds, that a matchmaking ticket can remain in process before timing out. Requests that
* time out can be resubmitted as needed.
*
*/
private Integer requestTimeoutSeconds;
/**
*
* Length of time (in seconds) to wait for players to accept a proposed match. If any player rejects the match or
* fails to accept before the timeout, the ticket continues to look for an acceptable match.
*
*/
private Integer acceptanceTimeoutSeconds;
/**
*
* Flag that determines whether or not a match that was created with this configuration must be accepted by the
* matched players. To require acceptance, set to TRUE.
*
*/
private Boolean acceptanceRequired;
/**
*
* Unique identifier for a matchmaking rule set to use with this configuration. A matchmaking configuration can only
* use rule sets that are defined in the same region.
*
*/
private String ruleSetName;
/**
*
* SNS topic ARN that is set up to receive matchmaking notifications.
*
*/
private String notificationTarget;
/**
*
* Number of player slots in a match to keep open for future players. For example, if the configuration's rule set
* specifies a match for a single 12-person team, and the additional player count is set to 2, only 10 players are
* selected for the match.
*
*/
private Integer additionalPlayerCount;
/**
*
* Information to attached to all events related to the matchmaking configuration.
*
*/
private String customEventData;
/**
*
* Set of custom properties for a game session, formatted as key:value pairs. These properties are passed to a game
* server process in the GameSession object with a request to start a new game session (see Start a Game Session). This information is added to the new GameSession object that is created for a
* successful match.
*
*/
private java.util.List gameProperties;
/**
*
* Set of custom game session properties, formatted as a single string value. This data is passed to a game server
* process in the GameSession object with a request to start a new game session (see Start a Game Session). This information is added to the new GameSession object that is created for a
* successful match.
*
*/
private String gameSessionData;
/**
*
* Unique identifier for a matchmaking configuration. This name is used to identify the configuration associated
* with a matchmaking request or ticket.
*
*
* @param name
* Unique identifier for a matchmaking configuration. This name is used to identify the configuration
* associated with a matchmaking request or ticket.
*/
public void setName(String name) {
this.name = name;
}
/**
*
* Unique identifier for a matchmaking configuration. This name is used to identify the configuration associated
* with a matchmaking request or ticket.
*
*
* @return Unique identifier for a matchmaking configuration. This name is used to identify the configuration
* associated with a matchmaking request or ticket.
*/
public String getName() {
return this.name;
}
/**
*
* Unique identifier for a matchmaking configuration. This name is used to identify the configuration associated
* with a matchmaking request or ticket.
*
*
* @param name
* Unique identifier for a matchmaking configuration. This name is used to identify the configuration
* associated with a matchmaking request or ticket.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateMatchmakingConfigurationRequest withName(String name) {
setName(name);
return this;
}
/**
*
* Meaningful description of the matchmaking configuration.
*
*
* @param description
* Meaningful description of the matchmaking configuration.
*/
public void setDescription(String description) {
this.description = description;
}
/**
*
* Meaningful description of the matchmaking configuration.
*
*
* @return Meaningful description of the matchmaking configuration.
*/
public String getDescription() {
return this.description;
}
/**
*
* Meaningful description of the matchmaking configuration.
*
*
* @param description
* Meaningful description of the matchmaking configuration.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateMatchmakingConfigurationRequest withDescription(String description) {
setDescription(description);
return this;
}
/**
*
* Amazon Resource Name (ARN) that
* is assigned to a game session queue and uniquely identifies it. Format is
* arn:aws:gamelift:<region>::fleet/fleet-a1234567-b8c9-0d1e-2fa3-b45c6d7e8912
. These queues are
* used when placing game sessions for matches that are created with this matchmaking configuration. Queues can be
* located in any region.
*
*
* @return Amazon Resource Name (ARN) that is assigned to a
* game session queue and uniquely identifies it. Format is
* arn:aws:gamelift:<region>::fleet/fleet-a1234567-b8c9-0d1e-2fa3-b45c6d7e8912
. These
* queues are used when placing game sessions for matches that are created with this matchmaking
* configuration. Queues can be located in any region.
*/
public java.util.List getGameSessionQueueArns() {
return gameSessionQueueArns;
}
/**
*
* Amazon Resource Name (ARN) that
* is assigned to a game session queue and uniquely identifies it. Format is
* arn:aws:gamelift:<region>::fleet/fleet-a1234567-b8c9-0d1e-2fa3-b45c6d7e8912
. These queues are
* used when placing game sessions for matches that are created with this matchmaking configuration. Queues can be
* located in any region.
*
*
* @param gameSessionQueueArns
* Amazon Resource Name (ARN)
* that is assigned to a game session queue and uniquely identifies it. Format is
* arn:aws:gamelift:<region>::fleet/fleet-a1234567-b8c9-0d1e-2fa3-b45c6d7e8912
. These
* queues are used when placing game sessions for matches that are created with this matchmaking
* configuration. Queues can be located in any region.
*/
public void setGameSessionQueueArns(java.util.Collection gameSessionQueueArns) {
if (gameSessionQueueArns == null) {
this.gameSessionQueueArns = null;
return;
}
this.gameSessionQueueArns = new java.util.ArrayList(gameSessionQueueArns);
}
/**
*
* Amazon Resource Name (ARN) that
* is assigned to a game session queue and uniquely identifies it. Format is
* arn:aws:gamelift:<region>::fleet/fleet-a1234567-b8c9-0d1e-2fa3-b45c6d7e8912
. These queues are
* used when placing game sessions for matches that are created with this matchmaking configuration. Queues can be
* located in any region.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setGameSessionQueueArns(java.util.Collection)} or {@link #withGameSessionQueueArns(java.util.Collection)}
* if you want to override the existing values.
*
*
* @param gameSessionQueueArns
* Amazon Resource Name (ARN)
* that is assigned to a game session queue and uniquely identifies it. Format is
* arn:aws:gamelift:<region>::fleet/fleet-a1234567-b8c9-0d1e-2fa3-b45c6d7e8912
. These
* queues are used when placing game sessions for matches that are created with this matchmaking
* configuration. Queues can be located in any region.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateMatchmakingConfigurationRequest withGameSessionQueueArns(String... gameSessionQueueArns) {
if (this.gameSessionQueueArns == null) {
setGameSessionQueueArns(new java.util.ArrayList(gameSessionQueueArns.length));
}
for (String ele : gameSessionQueueArns) {
this.gameSessionQueueArns.add(ele);
}
return this;
}
/**
*
* Amazon Resource Name (ARN) that
* is assigned to a game session queue and uniquely identifies it. Format is
* arn:aws:gamelift:<region>::fleet/fleet-a1234567-b8c9-0d1e-2fa3-b45c6d7e8912
. These queues are
* used when placing game sessions for matches that are created with this matchmaking configuration. Queues can be
* located in any region.
*
*
* @param gameSessionQueueArns
* Amazon Resource Name (ARN)
* that is assigned to a game session queue and uniquely identifies it. Format is
* arn:aws:gamelift:<region>::fleet/fleet-a1234567-b8c9-0d1e-2fa3-b45c6d7e8912
. These
* queues are used when placing game sessions for matches that are created with this matchmaking
* configuration. Queues can be located in any region.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateMatchmakingConfigurationRequest withGameSessionQueueArns(java.util.Collection gameSessionQueueArns) {
setGameSessionQueueArns(gameSessionQueueArns);
return this;
}
/**
*
* Maximum duration, in seconds, that a matchmaking ticket can remain in process before timing out. Requests that
* time out can be resubmitted as needed.
*
*
* @param requestTimeoutSeconds
* Maximum duration, in seconds, that a matchmaking ticket can remain in process before timing out. Requests
* that time out can be resubmitted as needed.
*/
public void setRequestTimeoutSeconds(Integer requestTimeoutSeconds) {
this.requestTimeoutSeconds = requestTimeoutSeconds;
}
/**
*
* Maximum duration, in seconds, that a matchmaking ticket can remain in process before timing out. Requests that
* time out can be resubmitted as needed.
*
*
* @return Maximum duration, in seconds, that a matchmaking ticket can remain in process before timing out. Requests
* that time out can be resubmitted as needed.
*/
public Integer getRequestTimeoutSeconds() {
return this.requestTimeoutSeconds;
}
/**
*
* Maximum duration, in seconds, that a matchmaking ticket can remain in process before timing out. Requests that
* time out can be resubmitted as needed.
*
*
* @param requestTimeoutSeconds
* Maximum duration, in seconds, that a matchmaking ticket can remain in process before timing out. Requests
* that time out can be resubmitted as needed.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateMatchmakingConfigurationRequest withRequestTimeoutSeconds(Integer requestTimeoutSeconds) {
setRequestTimeoutSeconds(requestTimeoutSeconds);
return this;
}
/**
*
* Length of time (in seconds) to wait for players to accept a proposed match. If any player rejects the match or
* fails to accept before the timeout, the ticket continues to look for an acceptable match.
*
*
* @param acceptanceTimeoutSeconds
* Length of time (in seconds) to wait for players to accept a proposed match. If any player rejects the
* match or fails to accept before the timeout, the ticket continues to look for an acceptable match.
*/
public void setAcceptanceTimeoutSeconds(Integer acceptanceTimeoutSeconds) {
this.acceptanceTimeoutSeconds = acceptanceTimeoutSeconds;
}
/**
*
* Length of time (in seconds) to wait for players to accept a proposed match. If any player rejects the match or
* fails to accept before the timeout, the ticket continues to look for an acceptable match.
*
*
* @return Length of time (in seconds) to wait for players to accept a proposed match. If any player rejects the
* match or fails to accept before the timeout, the ticket continues to look for an acceptable match.
*/
public Integer getAcceptanceTimeoutSeconds() {
return this.acceptanceTimeoutSeconds;
}
/**
*
* Length of time (in seconds) to wait for players to accept a proposed match. If any player rejects the match or
* fails to accept before the timeout, the ticket continues to look for an acceptable match.
*
*
* @param acceptanceTimeoutSeconds
* Length of time (in seconds) to wait for players to accept a proposed match. If any player rejects the
* match or fails to accept before the timeout, the ticket continues to look for an acceptable match.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateMatchmakingConfigurationRequest withAcceptanceTimeoutSeconds(Integer acceptanceTimeoutSeconds) {
setAcceptanceTimeoutSeconds(acceptanceTimeoutSeconds);
return this;
}
/**
*
* Flag that determines whether or not a match that was created with this configuration must be accepted by the
* matched players. To require acceptance, set to TRUE.
*
*
* @param acceptanceRequired
* Flag that determines whether or not a match that was created with this configuration must be accepted by
* the matched players. To require acceptance, set to TRUE.
*/
public void setAcceptanceRequired(Boolean acceptanceRequired) {
this.acceptanceRequired = acceptanceRequired;
}
/**
*
* Flag that determines whether or not a match that was created with this configuration must be accepted by the
* matched players. To require acceptance, set to TRUE.
*
*
* @return Flag that determines whether or not a match that was created with this configuration must be accepted by
* the matched players. To require acceptance, set to TRUE.
*/
public Boolean getAcceptanceRequired() {
return this.acceptanceRequired;
}
/**
*
* Flag that determines whether or not a match that was created with this configuration must be accepted by the
* matched players. To require acceptance, set to TRUE.
*
*
* @param acceptanceRequired
* Flag that determines whether or not a match that was created with this configuration must be accepted by
* the matched players. To require acceptance, set to TRUE.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateMatchmakingConfigurationRequest withAcceptanceRequired(Boolean acceptanceRequired) {
setAcceptanceRequired(acceptanceRequired);
return this;
}
/**
*
* Flag that determines whether or not a match that was created with this configuration must be accepted by the
* matched players. To require acceptance, set to TRUE.
*
*
* @return Flag that determines whether or not a match that was created with this configuration must be accepted by
* the matched players. To require acceptance, set to TRUE.
*/
public Boolean isAcceptanceRequired() {
return this.acceptanceRequired;
}
/**
*
* Unique identifier for a matchmaking rule set to use with this configuration. A matchmaking configuration can only
* use rule sets that are defined in the same region.
*
*
* @param ruleSetName
* Unique identifier for a matchmaking rule set to use with this configuration. A matchmaking configuration
* can only use rule sets that are defined in the same region.
*/
public void setRuleSetName(String ruleSetName) {
this.ruleSetName = ruleSetName;
}
/**
*
* Unique identifier for a matchmaking rule set to use with this configuration. A matchmaking configuration can only
* use rule sets that are defined in the same region.
*
*
* @return Unique identifier for a matchmaking rule set to use with this configuration. A matchmaking configuration
* can only use rule sets that are defined in the same region.
*/
public String getRuleSetName() {
return this.ruleSetName;
}
/**
*
* Unique identifier for a matchmaking rule set to use with this configuration. A matchmaking configuration can only
* use rule sets that are defined in the same region.
*
*
* @param ruleSetName
* Unique identifier for a matchmaking rule set to use with this configuration. A matchmaking configuration
* can only use rule sets that are defined in the same region.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateMatchmakingConfigurationRequest withRuleSetName(String ruleSetName) {
setRuleSetName(ruleSetName);
return this;
}
/**
*
* SNS topic ARN that is set up to receive matchmaking notifications.
*
*
* @param notificationTarget
* SNS topic ARN that is set up to receive matchmaking notifications.
*/
public void setNotificationTarget(String notificationTarget) {
this.notificationTarget = notificationTarget;
}
/**
*
* SNS topic ARN that is set up to receive matchmaking notifications.
*
*
* @return SNS topic ARN that is set up to receive matchmaking notifications.
*/
public String getNotificationTarget() {
return this.notificationTarget;
}
/**
*
* SNS topic ARN that is set up to receive matchmaking notifications.
*
*
* @param notificationTarget
* SNS topic ARN that is set up to receive matchmaking notifications.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateMatchmakingConfigurationRequest withNotificationTarget(String notificationTarget) {
setNotificationTarget(notificationTarget);
return this;
}
/**
*
* Number of player slots in a match to keep open for future players. For example, if the configuration's rule set
* specifies a match for a single 12-person team, and the additional player count is set to 2, only 10 players are
* selected for the match.
*
*
* @param additionalPlayerCount
* Number of player slots in a match to keep open for future players. For example, if the configuration's
* rule set specifies a match for a single 12-person team, and the additional player count is set to 2, only
* 10 players are selected for the match.
*/
public void setAdditionalPlayerCount(Integer additionalPlayerCount) {
this.additionalPlayerCount = additionalPlayerCount;
}
/**
*
* Number of player slots in a match to keep open for future players. For example, if the configuration's rule set
* specifies a match for a single 12-person team, and the additional player count is set to 2, only 10 players are
* selected for the match.
*
*
* @return Number of player slots in a match to keep open for future players. For example, if the configuration's
* rule set specifies a match for a single 12-person team, and the additional player count is set to 2, only
* 10 players are selected for the match.
*/
public Integer getAdditionalPlayerCount() {
return this.additionalPlayerCount;
}
/**
*
* Number of player slots in a match to keep open for future players. For example, if the configuration's rule set
* specifies a match for a single 12-person team, and the additional player count is set to 2, only 10 players are
* selected for the match.
*
*
* @param additionalPlayerCount
* Number of player slots in a match to keep open for future players. For example, if the configuration's
* rule set specifies a match for a single 12-person team, and the additional player count is set to 2, only
* 10 players are selected for the match.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateMatchmakingConfigurationRequest withAdditionalPlayerCount(Integer additionalPlayerCount) {
setAdditionalPlayerCount(additionalPlayerCount);
return this;
}
/**
*
* Information to attached to all events related to the matchmaking configuration.
*
*
* @param customEventData
* Information to attached to all events related to the matchmaking configuration.
*/
public void setCustomEventData(String customEventData) {
this.customEventData = customEventData;
}
/**
*
* Information to attached to all events related to the matchmaking configuration.
*
*
* @return Information to attached to all events related to the matchmaking configuration.
*/
public String getCustomEventData() {
return this.customEventData;
}
/**
*
* Information to attached to all events related to the matchmaking configuration.
*
*
* @param customEventData
* Information to attached to all events related to the matchmaking configuration.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateMatchmakingConfigurationRequest withCustomEventData(String customEventData) {
setCustomEventData(customEventData);
return this;
}
/**
*
* Set of custom properties for a game session, formatted as key:value pairs. These properties are passed to a game
* server process in the GameSession object with a request to start a new game session (see Start a Game Session). This information is added to the new GameSession object that is created for a
* successful match.
*
*
* @return Set of custom properties for a game session, formatted as key:value pairs. These properties are passed to
* a game server process in the GameSession object with a request to start a new game session (see Start a Game Session). This information is added to the new GameSession object that is
* created for a successful match.
*/
public java.util.List getGameProperties() {
return gameProperties;
}
/**
*
* Set of custom properties for a game session, formatted as key:value pairs. These properties are passed to a game
* server process in the GameSession object with a request to start a new game session (see Start a Game Session). This information is added to the new GameSession object that is created for a
* successful match.
*
*
* @param gameProperties
* Set of custom properties for a game session, formatted as key:value pairs. These properties are passed to
* a game server process in the GameSession object with a request to start a new game session (see Start a Game Session). This information is added to the new GameSession object that is created
* for a successful match.
*/
public void setGameProperties(java.util.Collection gameProperties) {
if (gameProperties == null) {
this.gameProperties = null;
return;
}
this.gameProperties = new java.util.ArrayList(gameProperties);
}
/**
*
* Set of custom properties for a game session, formatted as key:value pairs. These properties are passed to a game
* server process in the GameSession object with a request to start a new game session (see Start a Game Session). This information is added to the new GameSession object that is created for a
* successful match.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setGameProperties(java.util.Collection)} or {@link #withGameProperties(java.util.Collection)} if you want
* to override the existing values.
*
*
* @param gameProperties
* Set of custom properties for a game session, formatted as key:value pairs. These properties are passed to
* a game server process in the GameSession object with a request to start a new game session (see Start a Game Session). This information is added to the new GameSession object that is created
* for a successful match.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateMatchmakingConfigurationRequest withGameProperties(GameProperty... gameProperties) {
if (this.gameProperties == null) {
setGameProperties(new java.util.ArrayList(gameProperties.length));
}
for (GameProperty ele : gameProperties) {
this.gameProperties.add(ele);
}
return this;
}
/**
*
* Set of custom properties for a game session, formatted as key:value pairs. These properties are passed to a game
* server process in the GameSession object with a request to start a new game session (see Start a Game Session). This information is added to the new GameSession object that is created for a
* successful match.
*
*
* @param gameProperties
* Set of custom properties for a game session, formatted as key:value pairs. These properties are passed to
* a game server process in the GameSession object with a request to start a new game session (see Start a Game Session). This information is added to the new GameSession object that is created
* for a successful match.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateMatchmakingConfigurationRequest withGameProperties(java.util.Collection gameProperties) {
setGameProperties(gameProperties);
return this;
}
/**
*
* Set of custom game session properties, formatted as a single string value. This data is passed to a game server
* process in the GameSession object with a request to start a new game session (see Start a Game Session). This information is added to the new GameSession object that is created for a
* successful match.
*
*
* @param gameSessionData
* Set of custom game session properties, formatted as a single string value. This data is passed to a game
* server process in the GameSession object with a request to start a new game session (see Start a Game Session). This information is added to the new GameSession object that is created
* for a successful match.
*/
public void setGameSessionData(String gameSessionData) {
this.gameSessionData = gameSessionData;
}
/**
*
* Set of custom game session properties, formatted as a single string value. This data is passed to a game server
* process in the GameSession object with a request to start a new game session (see Start a Game Session). This information is added to the new GameSession object that is created for a
* successful match.
*
*
* @return Set of custom game session properties, formatted as a single string value. This data is passed to a game
* server process in the GameSession object with a request to start a new game session (see Start a Game Session). This information is added to the new GameSession object that is
* created for a successful match.
*/
public String getGameSessionData() {
return this.gameSessionData;
}
/**
*
* Set of custom game session properties, formatted as a single string value. This data is passed to a game server
* process in the GameSession object with a request to start a new game session (see Start a Game Session). This information is added to the new GameSession object that is created for a
* successful match.
*
*
* @param gameSessionData
* Set of custom game session properties, formatted as a single string value. This data is passed to a game
* server process in the GameSession object with a request to start a new game session (see Start a Game Session). This information is added to the new GameSession object that is created
* for a successful match.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateMatchmakingConfigurationRequest withGameSessionData(String gameSessionData) {
setGameSessionData(gameSessionData);
return this;
}
/**
* Returns a string representation of this object; useful for testing and debugging.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getName() != null)
sb.append("Name: ").append(getName()).append(",");
if (getDescription() != null)
sb.append("Description: ").append(getDescription()).append(",");
if (getGameSessionQueueArns() != null)
sb.append("GameSessionQueueArns: ").append(getGameSessionQueueArns()).append(",");
if (getRequestTimeoutSeconds() != null)
sb.append("RequestTimeoutSeconds: ").append(getRequestTimeoutSeconds()).append(",");
if (getAcceptanceTimeoutSeconds() != null)
sb.append("AcceptanceTimeoutSeconds: ").append(getAcceptanceTimeoutSeconds()).append(",");
if (getAcceptanceRequired() != null)
sb.append("AcceptanceRequired: ").append(getAcceptanceRequired()).append(",");
if (getRuleSetName() != null)
sb.append("RuleSetName: ").append(getRuleSetName()).append(",");
if (getNotificationTarget() != null)
sb.append("NotificationTarget: ").append(getNotificationTarget()).append(",");
if (getAdditionalPlayerCount() != null)
sb.append("AdditionalPlayerCount: ").append(getAdditionalPlayerCount()).append(",");
if (getCustomEventData() != null)
sb.append("CustomEventData: ").append(getCustomEventData()).append(",");
if (getGameProperties() != null)
sb.append("GameProperties: ").append(getGameProperties()).append(",");
if (getGameSessionData() != null)
sb.append("GameSessionData: ").append(getGameSessionData());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof CreateMatchmakingConfigurationRequest == false)
return false;
CreateMatchmakingConfigurationRequest other = (CreateMatchmakingConfigurationRequest) obj;
if (other.getName() == null ^ this.getName() == null)
return false;
if (other.getName() != null && other.getName().equals(this.getName()) == false)
return false;
if (other.getDescription() == null ^ this.getDescription() == null)
return false;
if (other.getDescription() != null && other.getDescription().equals(this.getDescription()) == false)
return false;
if (other.getGameSessionQueueArns() == null ^ this.getGameSessionQueueArns() == null)
return false;
if (other.getGameSessionQueueArns() != null && other.getGameSessionQueueArns().equals(this.getGameSessionQueueArns()) == false)
return false;
if (other.getRequestTimeoutSeconds() == null ^ this.getRequestTimeoutSeconds() == null)
return false;
if (other.getRequestTimeoutSeconds() != null && other.getRequestTimeoutSeconds().equals(this.getRequestTimeoutSeconds()) == false)
return false;
if (other.getAcceptanceTimeoutSeconds() == null ^ this.getAcceptanceTimeoutSeconds() == null)
return false;
if (other.getAcceptanceTimeoutSeconds() != null && other.getAcceptanceTimeoutSeconds().equals(this.getAcceptanceTimeoutSeconds()) == false)
return false;
if (other.getAcceptanceRequired() == null ^ this.getAcceptanceRequired() == null)
return false;
if (other.getAcceptanceRequired() != null && other.getAcceptanceRequired().equals(this.getAcceptanceRequired()) == false)
return false;
if (other.getRuleSetName() == null ^ this.getRuleSetName() == null)
return false;
if (other.getRuleSetName() != null && other.getRuleSetName().equals(this.getRuleSetName()) == false)
return false;
if (other.getNotificationTarget() == null ^ this.getNotificationTarget() == null)
return false;
if (other.getNotificationTarget() != null && other.getNotificationTarget().equals(this.getNotificationTarget()) == false)
return false;
if (other.getAdditionalPlayerCount() == null ^ this.getAdditionalPlayerCount() == null)
return false;
if (other.getAdditionalPlayerCount() != null && other.getAdditionalPlayerCount().equals(this.getAdditionalPlayerCount()) == false)
return false;
if (other.getCustomEventData() == null ^ this.getCustomEventData() == null)
return false;
if (other.getCustomEventData() != null && other.getCustomEventData().equals(this.getCustomEventData()) == false)
return false;
if (other.getGameProperties() == null ^ this.getGameProperties() == null)
return false;
if (other.getGameProperties() != null && other.getGameProperties().equals(this.getGameProperties()) == false)
return false;
if (other.getGameSessionData() == null ^ this.getGameSessionData() == null)
return false;
if (other.getGameSessionData() != null && other.getGameSessionData().equals(this.getGameSessionData()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getName() == null) ? 0 : getName().hashCode());
hashCode = prime * hashCode + ((getDescription() == null) ? 0 : getDescription().hashCode());
hashCode = prime * hashCode + ((getGameSessionQueueArns() == null) ? 0 : getGameSessionQueueArns().hashCode());
hashCode = prime * hashCode + ((getRequestTimeoutSeconds() == null) ? 0 : getRequestTimeoutSeconds().hashCode());
hashCode = prime * hashCode + ((getAcceptanceTimeoutSeconds() == null) ? 0 : getAcceptanceTimeoutSeconds().hashCode());
hashCode = prime * hashCode + ((getAcceptanceRequired() == null) ? 0 : getAcceptanceRequired().hashCode());
hashCode = prime * hashCode + ((getRuleSetName() == null) ? 0 : getRuleSetName().hashCode());
hashCode = prime * hashCode + ((getNotificationTarget() == null) ? 0 : getNotificationTarget().hashCode());
hashCode = prime * hashCode + ((getAdditionalPlayerCount() == null) ? 0 : getAdditionalPlayerCount().hashCode());
hashCode = prime * hashCode + ((getCustomEventData() == null) ? 0 : getCustomEventData().hashCode());
hashCode = prime * hashCode + ((getGameProperties() == null) ? 0 : getGameProperties().hashCode());
hashCode = prime * hashCode + ((getGameSessionData() == null) ? 0 : getGameSessionData().hashCode());
return hashCode;
}
@Override
public CreateMatchmakingConfigurationRequest clone() {
return (CreateMatchmakingConfigurationRequest) super.clone();
}
}