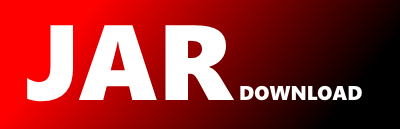
com.amazonaws.services.gamelift.model.Player Maven / Gradle / Ivy
Show all versions of aws-java-sdk-gamelift Show documentation
/*
* Copyright 2013-2018 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.gamelift.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* Represents a player in matchmaking. When starting a matchmaking request, a player has a player ID, attributes, and
* may have latency data. Team information is added after a match has been successfully completed.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class Player implements Serializable, Cloneable, StructuredPojo {
/**
*
* Unique identifier for a player
*
*/
private String playerId;
/**
*
* Collection of key:value pairs containing player information for use in matchmaking. Player attribute keys must
* match the playerAttributes used in a matchmaking rule set. Example:
* "PlayerAttributes": {"skill": {"N": "23"}, "gameMode": {"S": "deathmatch"}}
.
*
*/
private java.util.Map playerAttributes;
/**
*
* Name of the team that the player is assigned to in a match. Team names are defined in a matchmaking rule set.
*
*/
private String team;
/**
*
* Set of values, expressed in milliseconds, indicating the amount of latency that a player experiences when
* connected to AWS regions. If this property is present, FlexMatch considers placing the match only in regions for
* which latency is reported.
*
*
* If a matchmaker has a rule that evaluates player latency, players must report latency in order to be matched. If
* no latency is reported in this scenario, FlexMatch assumes that no regions are available to the player and the
* ticket is not matchable.
*
*/
private java.util.Map latencyInMs;
/**
*
* Unique identifier for a player
*
*
* @param playerId
* Unique identifier for a player
*/
public void setPlayerId(String playerId) {
this.playerId = playerId;
}
/**
*
* Unique identifier for a player
*
*
* @return Unique identifier for a player
*/
public String getPlayerId() {
return this.playerId;
}
/**
*
* Unique identifier for a player
*
*
* @param playerId
* Unique identifier for a player
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Player withPlayerId(String playerId) {
setPlayerId(playerId);
return this;
}
/**
*
* Collection of key:value pairs containing player information for use in matchmaking. Player attribute keys must
* match the playerAttributes used in a matchmaking rule set. Example:
* "PlayerAttributes": {"skill": {"N": "23"}, "gameMode": {"S": "deathmatch"}}
.
*
*
* @return Collection of key:value pairs containing player information for use in matchmaking. Player attribute keys
* must match the playerAttributes used in a matchmaking rule set. Example:
* "PlayerAttributes": {"skill": {"N": "23"}, "gameMode": {"S": "deathmatch"}}
.
*/
public java.util.Map getPlayerAttributes() {
return playerAttributes;
}
/**
*
* Collection of key:value pairs containing player information for use in matchmaking. Player attribute keys must
* match the playerAttributes used in a matchmaking rule set. Example:
* "PlayerAttributes": {"skill": {"N": "23"}, "gameMode": {"S": "deathmatch"}}
.
*
*
* @param playerAttributes
* Collection of key:value pairs containing player information for use in matchmaking. Player attribute keys
* must match the playerAttributes used in a matchmaking rule set. Example:
* "PlayerAttributes": {"skill": {"N": "23"}, "gameMode": {"S": "deathmatch"}}
.
*/
public void setPlayerAttributes(java.util.Map playerAttributes) {
this.playerAttributes = playerAttributes;
}
/**
*
* Collection of key:value pairs containing player information for use in matchmaking. Player attribute keys must
* match the playerAttributes used in a matchmaking rule set. Example:
* "PlayerAttributes": {"skill": {"N": "23"}, "gameMode": {"S": "deathmatch"}}
.
*
*
* @param playerAttributes
* Collection of key:value pairs containing player information for use in matchmaking. Player attribute keys
* must match the playerAttributes used in a matchmaking rule set. Example:
* "PlayerAttributes": {"skill": {"N": "23"}, "gameMode": {"S": "deathmatch"}}
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Player withPlayerAttributes(java.util.Map playerAttributes) {
setPlayerAttributes(playerAttributes);
return this;
}
public Player addPlayerAttributesEntry(String key, AttributeValue value) {
if (null == this.playerAttributes) {
this.playerAttributes = new java.util.HashMap();
}
if (this.playerAttributes.containsKey(key))
throw new IllegalArgumentException("Duplicated keys (" + key.toString() + ") are provided.");
this.playerAttributes.put(key, value);
return this;
}
/**
* Removes all the entries added into PlayerAttributes.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Player clearPlayerAttributesEntries() {
this.playerAttributes = null;
return this;
}
/**
*
* Name of the team that the player is assigned to in a match. Team names are defined in a matchmaking rule set.
*
*
* @param team
* Name of the team that the player is assigned to in a match. Team names are defined in a matchmaking rule
* set.
*/
public void setTeam(String team) {
this.team = team;
}
/**
*
* Name of the team that the player is assigned to in a match. Team names are defined in a matchmaking rule set.
*
*
* @return Name of the team that the player is assigned to in a match. Team names are defined in a matchmaking rule
* set.
*/
public String getTeam() {
return this.team;
}
/**
*
* Name of the team that the player is assigned to in a match. Team names are defined in a matchmaking rule set.
*
*
* @param team
* Name of the team that the player is assigned to in a match. Team names are defined in a matchmaking rule
* set.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Player withTeam(String team) {
setTeam(team);
return this;
}
/**
*
* Set of values, expressed in milliseconds, indicating the amount of latency that a player experiences when
* connected to AWS regions. If this property is present, FlexMatch considers placing the match only in regions for
* which latency is reported.
*
*
* If a matchmaker has a rule that evaluates player latency, players must report latency in order to be matched. If
* no latency is reported in this scenario, FlexMatch assumes that no regions are available to the player and the
* ticket is not matchable.
*
*
* @return Set of values, expressed in milliseconds, indicating the amount of latency that a player experiences when
* connected to AWS regions. If this property is present, FlexMatch considers placing the match only in
* regions for which latency is reported.
*
* If a matchmaker has a rule that evaluates player latency, players must report latency in order to be
* matched. If no latency is reported in this scenario, FlexMatch assumes that no regions are available to
* the player and the ticket is not matchable.
*/
public java.util.Map getLatencyInMs() {
return latencyInMs;
}
/**
*
* Set of values, expressed in milliseconds, indicating the amount of latency that a player experiences when
* connected to AWS regions. If this property is present, FlexMatch considers placing the match only in regions for
* which latency is reported.
*
*
* If a matchmaker has a rule that evaluates player latency, players must report latency in order to be matched. If
* no latency is reported in this scenario, FlexMatch assumes that no regions are available to the player and the
* ticket is not matchable.
*
*
* @param latencyInMs
* Set of values, expressed in milliseconds, indicating the amount of latency that a player experiences when
* connected to AWS regions. If this property is present, FlexMatch considers placing the match only in
* regions for which latency is reported.
*
* If a matchmaker has a rule that evaluates player latency, players must report latency in order to be
* matched. If no latency is reported in this scenario, FlexMatch assumes that no regions are available to
* the player and the ticket is not matchable.
*/
public void setLatencyInMs(java.util.Map latencyInMs) {
this.latencyInMs = latencyInMs;
}
/**
*
* Set of values, expressed in milliseconds, indicating the amount of latency that a player experiences when
* connected to AWS regions. If this property is present, FlexMatch considers placing the match only in regions for
* which latency is reported.
*
*
* If a matchmaker has a rule that evaluates player latency, players must report latency in order to be matched. If
* no latency is reported in this scenario, FlexMatch assumes that no regions are available to the player and the
* ticket is not matchable.
*
*
* @param latencyInMs
* Set of values, expressed in milliseconds, indicating the amount of latency that a player experiences when
* connected to AWS regions. If this property is present, FlexMatch considers placing the match only in
* regions for which latency is reported.
*
* If a matchmaker has a rule that evaluates player latency, players must report latency in order to be
* matched. If no latency is reported in this scenario, FlexMatch assumes that no regions are available to
* the player and the ticket is not matchable.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Player withLatencyInMs(java.util.Map latencyInMs) {
setLatencyInMs(latencyInMs);
return this;
}
public Player addLatencyInMsEntry(String key, Integer value) {
if (null == this.latencyInMs) {
this.latencyInMs = new java.util.HashMap();
}
if (this.latencyInMs.containsKey(key))
throw new IllegalArgumentException("Duplicated keys (" + key.toString() + ") are provided.");
this.latencyInMs.put(key, value);
return this;
}
/**
* Removes all the entries added into LatencyInMs.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Player clearLatencyInMsEntries() {
this.latencyInMs = null;
return this;
}
/**
* Returns a string representation of this object; useful for testing and debugging.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getPlayerId() != null)
sb.append("PlayerId: ").append(getPlayerId()).append(",");
if (getPlayerAttributes() != null)
sb.append("PlayerAttributes: ").append(getPlayerAttributes()).append(",");
if (getTeam() != null)
sb.append("Team: ").append(getTeam()).append(",");
if (getLatencyInMs() != null)
sb.append("LatencyInMs: ").append(getLatencyInMs());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof Player == false)
return false;
Player other = (Player) obj;
if (other.getPlayerId() == null ^ this.getPlayerId() == null)
return false;
if (other.getPlayerId() != null && other.getPlayerId().equals(this.getPlayerId()) == false)
return false;
if (other.getPlayerAttributes() == null ^ this.getPlayerAttributes() == null)
return false;
if (other.getPlayerAttributes() != null && other.getPlayerAttributes().equals(this.getPlayerAttributes()) == false)
return false;
if (other.getTeam() == null ^ this.getTeam() == null)
return false;
if (other.getTeam() != null && other.getTeam().equals(this.getTeam()) == false)
return false;
if (other.getLatencyInMs() == null ^ this.getLatencyInMs() == null)
return false;
if (other.getLatencyInMs() != null && other.getLatencyInMs().equals(this.getLatencyInMs()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getPlayerId() == null) ? 0 : getPlayerId().hashCode());
hashCode = prime * hashCode + ((getPlayerAttributes() == null) ? 0 : getPlayerAttributes().hashCode());
hashCode = prime * hashCode + ((getTeam() == null) ? 0 : getTeam().hashCode());
hashCode = prime * hashCode + ((getLatencyInMs() == null) ? 0 : getLatencyInMs().hashCode());
return hashCode;
}
@Override
public Player clone() {
try {
return (Player) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.gamelift.model.transform.PlayerMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}