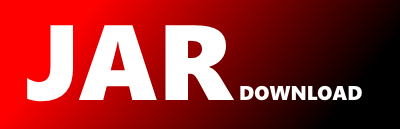
com.amazonaws.services.gamelift.model.Event Maven / Gradle / Ivy
/*
* Copyright 2017-2022 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.gamelift.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* Log entry describing an event that involves GameLift resources (such as a fleet). In addition to tracking activity,
* event codes and messages can provide additional information for troubleshooting and debugging problems.
*
*
* Related actions
*
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class Event implements Serializable, Cloneable, StructuredPojo {
/**
*
* A unique identifier for a fleet event.
*
*/
private String eventId;
/**
*
* A unique identifier for an event resource, such as a fleet ID.
*
*/
private String resourceId;
/**
*
* The type of event being logged.
*
*
* Fleet state transition events:
*
*
* -
*
* FLEET_CREATED -- A fleet resource was successfully created with a status of NEW
. Event messaging
* includes the fleet ID.
*
*
* -
*
* FLEET_STATE_DOWNLOADING -- Fleet status changed from NEW
to DOWNLOADING
. The compressed
* build has started downloading to a fleet instance for installation.
*
*
* -
*
* FLEET_STATE_VALIDATING -- Fleet status changed from DOWNLOADING
to VALIDATING
. GameLift
* has successfully downloaded the build and is now validating the build files.
*
*
* -
*
* FLEET_STATE_BUILDING -- Fleet status changed from VALIDATING
to BUILDING
. GameLift has
* successfully verified the build files and is now running the installation scripts.
*
*
* -
*
* FLEET_STATE_ACTIVATING -- Fleet status changed from BUILDING
to ACTIVATING
. GameLift is
* trying to launch an instance and test the connectivity between the build and the GameLift Service via the Server
* SDK.
*
*
* -
*
* FLEET_STATE_ACTIVE -- The fleet's status changed from ACTIVATING
to ACTIVE
. The fleet
* is now ready to host game sessions.
*
*
* -
*
* FLEET_STATE_ERROR -- The Fleet's status changed to ERROR
. Describe the fleet event message for more
* details.
*
*
*
*
* Fleet creation events (ordered by fleet creation activity):
*
*
* -
*
* FLEET_BINARY_DOWNLOAD_FAILED -- The build failed to download to the fleet instance.
*
*
* -
*
* FLEET_CREATION_EXTRACTING_BUILD -- The game server build was successfully downloaded to an instance, and the
* build files are now being extracted from the uploaded build and saved to an instance. Failure at this stage
* prevents a fleet from moving to ACTIVE status. Logs for this stage display a list of the files that are extracted
* and saved on the instance. Access the logs by using the URL in PreSignedLogUrl.
*
*
* -
*
* FLEET_CREATION_RUNNING_INSTALLER -- The game server build files were successfully extracted, and the GameLift is
* now running the build's install script (if one is included). Failure in this stage prevents a fleet from moving
* to ACTIVE status. Logs for this stage list the installation steps and whether or not the install completed
* successfully. Access the logs by using the URL in PreSignedLogUrl.
*
*
* -
*
* FLEET_CREATION_VALIDATING_RUNTIME_CONFIG -- The build process was successful, and the GameLift is now verifying
* that the game server launch paths, which are specified in the fleet's runtime configuration, exist. If any listed
* launch path exists, GameLift tries to launch a game server process and waits for the process to report ready.
* Failures in this stage prevent a fleet from moving to ACTIVE
status. Logs for this stage list the
* launch paths in the runtime configuration and indicate whether each is found. Access the logs by using the URL in
* PreSignedLogUrl.
*
*
* -
*
* FLEET_VALIDATION_LAUNCH_PATH_NOT_FOUND -- Validation of the runtime configuration failed because the executable
* specified in a launch path does not exist on the instance.
*
*
* -
*
* FLEET_VALIDATION_EXECUTABLE_RUNTIME_FAILURE -- Validation of the runtime configuration failed because the
* executable specified in a launch path failed to run on the fleet instance.
*
*
* -
*
* FLEET_VALIDATION_TIMED_OUT -- Validation of the fleet at the end of creation timed out. Try fleet creation again.
*
*
* -
*
* FLEET_ACTIVATION_FAILED -- The fleet failed to successfully complete one of the steps in the fleet activation
* process. This event code indicates that the game build was successfully downloaded to a fleet instance, built,
* and validated, but was not able to start a server process. For more information, see Debug Fleet Creation Issues.
*
*
* -
*
* FLEET_ACTIVATION_FAILED_NO_INSTANCES -- Fleet creation was not able to obtain any instances based on the input
* fleet attributes. Try again at a different time or choose a different combination of fleet attributes such as
* fleet type, instance type, etc.
*
*
* -
*
* FLEET_INITIALIZATION_FAILED -- A generic exception occurred during fleet creation. Describe the fleet event
* message for more details.
*
*
*
*
* VPC peering events:
*
*
* -
*
* FLEET_VPC_PEERING_SUCCEEDED -- A VPC peering connection has been established between the VPC for an GameLift
* fleet and a VPC in your Amazon Web Services account.
*
*
* -
*
* FLEET_VPC_PEERING_FAILED -- A requested VPC peering connection has failed. Event details and status information
* (see DescribeVpcPeeringConnections) provide additional detail. A common reason for peering failure is that
* the two VPCs have overlapping CIDR blocks of IPv4 addresses. To resolve this, change the CIDR block for the VPC
* in your Amazon Web Services account. For more information on VPC peering failures, see https://docs.aws.amazon.com/AmazonVPC/latest/PeeringGuide/invalid-peering-configurations.html
*
*
* -
*
* FLEET_VPC_PEERING_DELETED -- A VPC peering connection has been successfully deleted.
*
*
*
*
* Spot instance events:
*
*
* -
*
* INSTANCE_INTERRUPTED -- A spot instance was interrupted by EC2 with a two-minute notification.
*
*
*
*
* Spot process events:
*
*
* -
*
* SERVER_PROCESS_INVALID_PATH -- The game server executable or script could not be found based on the Fleet runtime
* configuration. Check that the launch path is correct based on the operating system of the Fleet.
*
*
* -
*
* SERVER_PROCESS_SDK_INITIALIZATION_TIMEOUT -- The server process did not call InitSDK() within the time expected.
* Check your game session log to see why InitSDK() was not called in time.
*
*
* -
*
* SERVER_PROCESS_PROCESS_READY_TIMEOUT -- The server process did not call ProcessReady() within the time expected
* after calling InitSDK(). Check your game session log to see why ProcessReady() was not called in time.
*
*
* -
*
* SERVER_PROCESS_CRASHED -- The server process exited without calling ProcessEnding(). Check your game session log
* to see why ProcessEnding() was not called.
*
*
* -
*
* SERVER_PROCESS_TERMINATED_UNHEALTHY -- The server process did not report a valid health check for too long and
* was therefore terminated by GameLift. Check your game session log to see if the thread became stuck processing a
* synchronous task for too long.
*
*
* -
*
* SERVER_PROCESS_FORCE_TERMINATED -- The server process did not exit cleanly after OnProcessTerminate() was sent
* within the time expected. Check your game session log to see why termination took longer than expected.
*
*
* -
*
* SERVER_PROCESS_PROCESS_EXIT_TIMEOUT -- The server process did not exit cleanly within the time expected after
* calling ProcessEnding(). Check your game session log to see why termination took longer than expected.
*
*
*
*
* Game session events:
*
*
* -
*
* GAME_SESSION_ACTIVATION_TIMEOUT -- GameSession failed to activate within the expected time. Check your game
* session log to see why ActivateGameSession() took longer to complete than expected.
*
*
*
*
* Other fleet events:
*
*
* -
*
* FLEET_SCALING_EVENT -- A change was made to the fleet's capacity settings (desired instances, minimum/maximum
* scaling limits). Event messaging includes the new capacity settings.
*
*
* -
*
* FLEET_NEW_GAME_SESSION_PROTECTION_POLICY_UPDATED -- A change was made to the fleet's game session protection
* policy setting. Event messaging includes both the old and new policy setting.
*
*
* -
*
* FLEET_DELETED -- A request to delete a fleet was initiated.
*
*
* -
*
* GENERIC_EVENT -- An unspecified event has occurred.
*
*
*
*/
private String eventCode;
/**
*
* Additional information related to the event.
*
*/
private String message;
/**
*
* Time stamp indicating when this event occurred. Format is a number expressed in Unix time as milliseconds (for
* example "1469498468.057"
).
*
*/
private java.util.Date eventTime;
/**
*
* Location of stored logs with additional detail that is related to the event. This is useful for debugging issues.
* The URL is valid for 15 minutes. You can also access fleet creation logs through the GameLift console.
*
*/
private String preSignedLogUrl;
/**
*
* A unique identifier for a fleet event.
*
*
* @param eventId
* A unique identifier for a fleet event.
*/
public void setEventId(String eventId) {
this.eventId = eventId;
}
/**
*
* A unique identifier for a fleet event.
*
*
* @return A unique identifier for a fleet event.
*/
public String getEventId() {
return this.eventId;
}
/**
*
* A unique identifier for a fleet event.
*
*
* @param eventId
* A unique identifier for a fleet event.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Event withEventId(String eventId) {
setEventId(eventId);
return this;
}
/**
*
* A unique identifier for an event resource, such as a fleet ID.
*
*
* @param resourceId
* A unique identifier for an event resource, such as a fleet ID.
*/
public void setResourceId(String resourceId) {
this.resourceId = resourceId;
}
/**
*
* A unique identifier for an event resource, such as a fleet ID.
*
*
* @return A unique identifier for an event resource, such as a fleet ID.
*/
public String getResourceId() {
return this.resourceId;
}
/**
*
* A unique identifier for an event resource, such as a fleet ID.
*
*
* @param resourceId
* A unique identifier for an event resource, such as a fleet ID.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Event withResourceId(String resourceId) {
setResourceId(resourceId);
return this;
}
/**
*
* The type of event being logged.
*
*
* Fleet state transition events:
*
*
* -
*
* FLEET_CREATED -- A fleet resource was successfully created with a status of NEW
. Event messaging
* includes the fleet ID.
*
*
* -
*
* FLEET_STATE_DOWNLOADING -- Fleet status changed from NEW
to DOWNLOADING
. The compressed
* build has started downloading to a fleet instance for installation.
*
*
* -
*
* FLEET_STATE_VALIDATING -- Fleet status changed from DOWNLOADING
to VALIDATING
. GameLift
* has successfully downloaded the build and is now validating the build files.
*
*
* -
*
* FLEET_STATE_BUILDING -- Fleet status changed from VALIDATING
to BUILDING
. GameLift has
* successfully verified the build files and is now running the installation scripts.
*
*
* -
*
* FLEET_STATE_ACTIVATING -- Fleet status changed from BUILDING
to ACTIVATING
. GameLift is
* trying to launch an instance and test the connectivity between the build and the GameLift Service via the Server
* SDK.
*
*
* -
*
* FLEET_STATE_ACTIVE -- The fleet's status changed from ACTIVATING
to ACTIVE
. The fleet
* is now ready to host game sessions.
*
*
* -
*
* FLEET_STATE_ERROR -- The Fleet's status changed to ERROR
. Describe the fleet event message for more
* details.
*
*
*
*
* Fleet creation events (ordered by fleet creation activity):
*
*
* -
*
* FLEET_BINARY_DOWNLOAD_FAILED -- The build failed to download to the fleet instance.
*
*
* -
*
* FLEET_CREATION_EXTRACTING_BUILD -- The game server build was successfully downloaded to an instance, and the
* build files are now being extracted from the uploaded build and saved to an instance. Failure at this stage
* prevents a fleet from moving to ACTIVE status. Logs for this stage display a list of the files that are extracted
* and saved on the instance. Access the logs by using the URL in PreSignedLogUrl.
*
*
* -
*
* FLEET_CREATION_RUNNING_INSTALLER -- The game server build files were successfully extracted, and the GameLift is
* now running the build's install script (if one is included). Failure in this stage prevents a fleet from moving
* to ACTIVE status. Logs for this stage list the installation steps and whether or not the install completed
* successfully. Access the logs by using the URL in PreSignedLogUrl.
*
*
* -
*
* FLEET_CREATION_VALIDATING_RUNTIME_CONFIG -- The build process was successful, and the GameLift is now verifying
* that the game server launch paths, which are specified in the fleet's runtime configuration, exist. If any listed
* launch path exists, GameLift tries to launch a game server process and waits for the process to report ready.
* Failures in this stage prevent a fleet from moving to ACTIVE
status. Logs for this stage list the
* launch paths in the runtime configuration and indicate whether each is found. Access the logs by using the URL in
* PreSignedLogUrl.
*
*
* -
*
* FLEET_VALIDATION_LAUNCH_PATH_NOT_FOUND -- Validation of the runtime configuration failed because the executable
* specified in a launch path does not exist on the instance.
*
*
* -
*
* FLEET_VALIDATION_EXECUTABLE_RUNTIME_FAILURE -- Validation of the runtime configuration failed because the
* executable specified in a launch path failed to run on the fleet instance.
*
*
* -
*
* FLEET_VALIDATION_TIMED_OUT -- Validation of the fleet at the end of creation timed out. Try fleet creation again.
*
*
* -
*
* FLEET_ACTIVATION_FAILED -- The fleet failed to successfully complete one of the steps in the fleet activation
* process. This event code indicates that the game build was successfully downloaded to a fleet instance, built,
* and validated, but was not able to start a server process. For more information, see Debug Fleet Creation Issues.
*
*
* -
*
* FLEET_ACTIVATION_FAILED_NO_INSTANCES -- Fleet creation was not able to obtain any instances based on the input
* fleet attributes. Try again at a different time or choose a different combination of fleet attributes such as
* fleet type, instance type, etc.
*
*
* -
*
* FLEET_INITIALIZATION_FAILED -- A generic exception occurred during fleet creation. Describe the fleet event
* message for more details.
*
*
*
*
* VPC peering events:
*
*
* -
*
* FLEET_VPC_PEERING_SUCCEEDED -- A VPC peering connection has been established between the VPC for an GameLift
* fleet and a VPC in your Amazon Web Services account.
*
*
* -
*
* FLEET_VPC_PEERING_FAILED -- A requested VPC peering connection has failed. Event details and status information
* (see DescribeVpcPeeringConnections) provide additional detail. A common reason for peering failure is that
* the two VPCs have overlapping CIDR blocks of IPv4 addresses. To resolve this, change the CIDR block for the VPC
* in your Amazon Web Services account. For more information on VPC peering failures, see https://docs.aws.amazon.com/AmazonVPC/latest/PeeringGuide/invalid-peering-configurations.html
*
*
* -
*
* FLEET_VPC_PEERING_DELETED -- A VPC peering connection has been successfully deleted.
*
*
*
*
* Spot instance events:
*
*
* -
*
* INSTANCE_INTERRUPTED -- A spot instance was interrupted by EC2 with a two-minute notification.
*
*
*
*
* Spot process events:
*
*
* -
*
* SERVER_PROCESS_INVALID_PATH -- The game server executable or script could not be found based on the Fleet runtime
* configuration. Check that the launch path is correct based on the operating system of the Fleet.
*
*
* -
*
* SERVER_PROCESS_SDK_INITIALIZATION_TIMEOUT -- The server process did not call InitSDK() within the time expected.
* Check your game session log to see why InitSDK() was not called in time.
*
*
* -
*
* SERVER_PROCESS_PROCESS_READY_TIMEOUT -- The server process did not call ProcessReady() within the time expected
* after calling InitSDK(). Check your game session log to see why ProcessReady() was not called in time.
*
*
* -
*
* SERVER_PROCESS_CRASHED -- The server process exited without calling ProcessEnding(). Check your game session log
* to see why ProcessEnding() was not called.
*
*
* -
*
* SERVER_PROCESS_TERMINATED_UNHEALTHY -- The server process did not report a valid health check for too long and
* was therefore terminated by GameLift. Check your game session log to see if the thread became stuck processing a
* synchronous task for too long.
*
*
* -
*
* SERVER_PROCESS_FORCE_TERMINATED -- The server process did not exit cleanly after OnProcessTerminate() was sent
* within the time expected. Check your game session log to see why termination took longer than expected.
*
*
* -
*
* SERVER_PROCESS_PROCESS_EXIT_TIMEOUT -- The server process did not exit cleanly within the time expected after
* calling ProcessEnding(). Check your game session log to see why termination took longer than expected.
*
*
*
*
* Game session events:
*
*
* -
*
* GAME_SESSION_ACTIVATION_TIMEOUT -- GameSession failed to activate within the expected time. Check your game
* session log to see why ActivateGameSession() took longer to complete than expected.
*
*
*
*
* Other fleet events:
*
*
* -
*
* FLEET_SCALING_EVENT -- A change was made to the fleet's capacity settings (desired instances, minimum/maximum
* scaling limits). Event messaging includes the new capacity settings.
*
*
* -
*
* FLEET_NEW_GAME_SESSION_PROTECTION_POLICY_UPDATED -- A change was made to the fleet's game session protection
* policy setting. Event messaging includes both the old and new policy setting.
*
*
* -
*
* FLEET_DELETED -- A request to delete a fleet was initiated.
*
*
* -
*
* GENERIC_EVENT -- An unspecified event has occurred.
*
*
*
*
* @param eventCode
* The type of event being logged.
*
* Fleet state transition events:
*
*
* -
*
* FLEET_CREATED -- A fleet resource was successfully created with a status of NEW
. Event
* messaging includes the fleet ID.
*
*
* -
*
* FLEET_STATE_DOWNLOADING -- Fleet status changed from NEW
to DOWNLOADING
. The
* compressed build has started downloading to a fleet instance for installation.
*
*
* -
*
* FLEET_STATE_VALIDATING -- Fleet status changed from DOWNLOADING
to VALIDATING
.
* GameLift has successfully downloaded the build and is now validating the build files.
*
*
* -
*
* FLEET_STATE_BUILDING -- Fleet status changed from VALIDATING
to BUILDING
.
* GameLift has successfully verified the build files and is now running the installation scripts.
*
*
* -
*
* FLEET_STATE_ACTIVATING -- Fleet status changed from BUILDING
to ACTIVATING
.
* GameLift is trying to launch an instance and test the connectivity between the build and the GameLift
* Service via the Server SDK.
*
*
* -
*
* FLEET_STATE_ACTIVE -- The fleet's status changed from ACTIVATING
to ACTIVE
. The
* fleet is now ready to host game sessions.
*
*
* -
*
* FLEET_STATE_ERROR -- The Fleet's status changed to ERROR
. Describe the fleet event message
* for more details.
*
*
*
*
* Fleet creation events (ordered by fleet creation activity):
*
*
* -
*
* FLEET_BINARY_DOWNLOAD_FAILED -- The build failed to download to the fleet instance.
*
*
* -
*
* FLEET_CREATION_EXTRACTING_BUILD -- The game server build was successfully downloaded to an instance, and
* the build files are now being extracted from the uploaded build and saved to an instance. Failure at this
* stage prevents a fleet from moving to ACTIVE status. Logs for this stage display a list of the files that
* are extracted and saved on the instance. Access the logs by using the URL in PreSignedLogUrl.
*
*
* -
*
* FLEET_CREATION_RUNNING_INSTALLER -- The game server build files were successfully extracted, and the
* GameLift is now running the build's install script (if one is included). Failure in this stage prevents a
* fleet from moving to ACTIVE status. Logs for this stage list the installation steps and whether or not the
* install completed successfully. Access the logs by using the URL in PreSignedLogUrl.
*
*
* -
*
* FLEET_CREATION_VALIDATING_RUNTIME_CONFIG -- The build process was successful, and the GameLift is now
* verifying that the game server launch paths, which are specified in the fleet's runtime configuration,
* exist. If any listed launch path exists, GameLift tries to launch a game server process and waits for the
* process to report ready. Failures in this stage prevent a fleet from moving to ACTIVE
status.
* Logs for this stage list the launch paths in the runtime configuration and indicate whether each is found.
* Access the logs by using the URL in PreSignedLogUrl.
*
*
* -
*
* FLEET_VALIDATION_LAUNCH_PATH_NOT_FOUND -- Validation of the runtime configuration failed because the
* executable specified in a launch path does not exist on the instance.
*
*
* -
*
* FLEET_VALIDATION_EXECUTABLE_RUNTIME_FAILURE -- Validation of the runtime configuration failed because the
* executable specified in a launch path failed to run on the fleet instance.
*
*
* -
*
* FLEET_VALIDATION_TIMED_OUT -- Validation of the fleet at the end of creation timed out. Try fleet creation
* again.
*
*
* -
*
* FLEET_ACTIVATION_FAILED -- The fleet failed to successfully complete one of the steps in the fleet
* activation process. This event code indicates that the game build was successfully downloaded to a fleet
* instance, built, and validated, but was not able to start a server process. For more information, see Debug Fleet Creation Issues.
*
*
* -
*
* FLEET_ACTIVATION_FAILED_NO_INSTANCES -- Fleet creation was not able to obtain any instances based on the
* input fleet attributes. Try again at a different time or choose a different combination of fleet
* attributes such as fleet type, instance type, etc.
*
*
* -
*
* FLEET_INITIALIZATION_FAILED -- A generic exception occurred during fleet creation. Describe the fleet
* event message for more details.
*
*
*
*
* VPC peering events:
*
*
* -
*
* FLEET_VPC_PEERING_SUCCEEDED -- A VPC peering connection has been established between the VPC for an
* GameLift fleet and a VPC in your Amazon Web Services account.
*
*
* -
*
* FLEET_VPC_PEERING_FAILED -- A requested VPC peering connection has failed. Event details and status
* information (see DescribeVpcPeeringConnections) provide additional detail. A common reason for
* peering failure is that the two VPCs have overlapping CIDR blocks of IPv4 addresses. To resolve this,
* change the CIDR block for the VPC in your Amazon Web Services account. For more information on VPC peering
* failures, see https://docs.aws.amazon.com/AmazonVPC/latest/PeeringGuide/invalid-peering-configurations.html
*
*
* -
*
* FLEET_VPC_PEERING_DELETED -- A VPC peering connection has been successfully deleted.
*
*
*
*
* Spot instance events:
*
*
* -
*
* INSTANCE_INTERRUPTED -- A spot instance was interrupted by EC2 with a two-minute notification.
*
*
*
*
* Spot process events:
*
*
* -
*
* SERVER_PROCESS_INVALID_PATH -- The game server executable or script could not be found based on the Fleet
* runtime configuration. Check that the launch path is correct based on the operating system of the Fleet.
*
*
* -
*
* SERVER_PROCESS_SDK_INITIALIZATION_TIMEOUT -- The server process did not call InitSDK() within the time
* expected. Check your game session log to see why InitSDK() was not called in time.
*
*
* -
*
* SERVER_PROCESS_PROCESS_READY_TIMEOUT -- The server process did not call ProcessReady() within the time
* expected after calling InitSDK(). Check your game session log to see why ProcessReady() was not called in
* time.
*
*
* -
*
* SERVER_PROCESS_CRASHED -- The server process exited without calling ProcessEnding(). Check your game
* session log to see why ProcessEnding() was not called.
*
*
* -
*
* SERVER_PROCESS_TERMINATED_UNHEALTHY -- The server process did not report a valid health check for too long
* and was therefore terminated by GameLift. Check your game session log to see if the thread became stuck
* processing a synchronous task for too long.
*
*
* -
*
* SERVER_PROCESS_FORCE_TERMINATED -- The server process did not exit cleanly after OnProcessTerminate() was
* sent within the time expected. Check your game session log to see why termination took longer than
* expected.
*
*
* -
*
* SERVER_PROCESS_PROCESS_EXIT_TIMEOUT -- The server process did not exit cleanly within the time expected
* after calling ProcessEnding(). Check your game session log to see why termination took longer than
* expected.
*
*
*
*
* Game session events:
*
*
* -
*
* GAME_SESSION_ACTIVATION_TIMEOUT -- GameSession failed to activate within the expected time. Check your
* game session log to see why ActivateGameSession() took longer to complete than expected.
*
*
*
*
* Other fleet events:
*
*
* -
*
* FLEET_SCALING_EVENT -- A change was made to the fleet's capacity settings (desired instances,
* minimum/maximum scaling limits). Event messaging includes the new capacity settings.
*
*
* -
*
* FLEET_NEW_GAME_SESSION_PROTECTION_POLICY_UPDATED -- A change was made to the fleet's game session
* protection policy setting. Event messaging includes both the old and new policy setting.
*
*
* -
*
* FLEET_DELETED -- A request to delete a fleet was initiated.
*
*
* -
*
* GENERIC_EVENT -- An unspecified event has occurred.
*
*
* @see EventCode
*/
public void setEventCode(String eventCode) {
this.eventCode = eventCode;
}
/**
*
* The type of event being logged.
*
*
* Fleet state transition events:
*
*
* -
*
* FLEET_CREATED -- A fleet resource was successfully created with a status of NEW
. Event messaging
* includes the fleet ID.
*
*
* -
*
* FLEET_STATE_DOWNLOADING -- Fleet status changed from NEW
to DOWNLOADING
. The compressed
* build has started downloading to a fleet instance for installation.
*
*
* -
*
* FLEET_STATE_VALIDATING -- Fleet status changed from DOWNLOADING
to VALIDATING
. GameLift
* has successfully downloaded the build and is now validating the build files.
*
*
* -
*
* FLEET_STATE_BUILDING -- Fleet status changed from VALIDATING
to BUILDING
. GameLift has
* successfully verified the build files and is now running the installation scripts.
*
*
* -
*
* FLEET_STATE_ACTIVATING -- Fleet status changed from BUILDING
to ACTIVATING
. GameLift is
* trying to launch an instance and test the connectivity between the build and the GameLift Service via the Server
* SDK.
*
*
* -
*
* FLEET_STATE_ACTIVE -- The fleet's status changed from ACTIVATING
to ACTIVE
. The fleet
* is now ready to host game sessions.
*
*
* -
*
* FLEET_STATE_ERROR -- The Fleet's status changed to ERROR
. Describe the fleet event message for more
* details.
*
*
*
*
* Fleet creation events (ordered by fleet creation activity):
*
*
* -
*
* FLEET_BINARY_DOWNLOAD_FAILED -- The build failed to download to the fleet instance.
*
*
* -
*
* FLEET_CREATION_EXTRACTING_BUILD -- The game server build was successfully downloaded to an instance, and the
* build files are now being extracted from the uploaded build and saved to an instance. Failure at this stage
* prevents a fleet from moving to ACTIVE status. Logs for this stage display a list of the files that are extracted
* and saved on the instance. Access the logs by using the URL in PreSignedLogUrl.
*
*
* -
*
* FLEET_CREATION_RUNNING_INSTALLER -- The game server build files were successfully extracted, and the GameLift is
* now running the build's install script (if one is included). Failure in this stage prevents a fleet from moving
* to ACTIVE status. Logs for this stage list the installation steps and whether or not the install completed
* successfully. Access the logs by using the URL in PreSignedLogUrl.
*
*
* -
*
* FLEET_CREATION_VALIDATING_RUNTIME_CONFIG -- The build process was successful, and the GameLift is now verifying
* that the game server launch paths, which are specified in the fleet's runtime configuration, exist. If any listed
* launch path exists, GameLift tries to launch a game server process and waits for the process to report ready.
* Failures in this stage prevent a fleet from moving to ACTIVE
status. Logs for this stage list the
* launch paths in the runtime configuration and indicate whether each is found. Access the logs by using the URL in
* PreSignedLogUrl.
*
*
* -
*
* FLEET_VALIDATION_LAUNCH_PATH_NOT_FOUND -- Validation of the runtime configuration failed because the executable
* specified in a launch path does not exist on the instance.
*
*
* -
*
* FLEET_VALIDATION_EXECUTABLE_RUNTIME_FAILURE -- Validation of the runtime configuration failed because the
* executable specified in a launch path failed to run on the fleet instance.
*
*
* -
*
* FLEET_VALIDATION_TIMED_OUT -- Validation of the fleet at the end of creation timed out. Try fleet creation again.
*
*
* -
*
* FLEET_ACTIVATION_FAILED -- The fleet failed to successfully complete one of the steps in the fleet activation
* process. This event code indicates that the game build was successfully downloaded to a fleet instance, built,
* and validated, but was not able to start a server process. For more information, see Debug Fleet Creation Issues.
*
*
* -
*
* FLEET_ACTIVATION_FAILED_NO_INSTANCES -- Fleet creation was not able to obtain any instances based on the input
* fleet attributes. Try again at a different time or choose a different combination of fleet attributes such as
* fleet type, instance type, etc.
*
*
* -
*
* FLEET_INITIALIZATION_FAILED -- A generic exception occurred during fleet creation. Describe the fleet event
* message for more details.
*
*
*
*
* VPC peering events:
*
*
* -
*
* FLEET_VPC_PEERING_SUCCEEDED -- A VPC peering connection has been established between the VPC for an GameLift
* fleet and a VPC in your Amazon Web Services account.
*
*
* -
*
* FLEET_VPC_PEERING_FAILED -- A requested VPC peering connection has failed. Event details and status information
* (see DescribeVpcPeeringConnections) provide additional detail. A common reason for peering failure is that
* the two VPCs have overlapping CIDR blocks of IPv4 addresses. To resolve this, change the CIDR block for the VPC
* in your Amazon Web Services account. For more information on VPC peering failures, see https://docs.aws.amazon.com/AmazonVPC/latest/PeeringGuide/invalid-peering-configurations.html
*
*
* -
*
* FLEET_VPC_PEERING_DELETED -- A VPC peering connection has been successfully deleted.
*
*
*
*
* Spot instance events:
*
*
* -
*
* INSTANCE_INTERRUPTED -- A spot instance was interrupted by EC2 with a two-minute notification.
*
*
*
*
* Spot process events:
*
*
* -
*
* SERVER_PROCESS_INVALID_PATH -- The game server executable or script could not be found based on the Fleet runtime
* configuration. Check that the launch path is correct based on the operating system of the Fleet.
*
*
* -
*
* SERVER_PROCESS_SDK_INITIALIZATION_TIMEOUT -- The server process did not call InitSDK() within the time expected.
* Check your game session log to see why InitSDK() was not called in time.
*
*
* -
*
* SERVER_PROCESS_PROCESS_READY_TIMEOUT -- The server process did not call ProcessReady() within the time expected
* after calling InitSDK(). Check your game session log to see why ProcessReady() was not called in time.
*
*
* -
*
* SERVER_PROCESS_CRASHED -- The server process exited without calling ProcessEnding(). Check your game session log
* to see why ProcessEnding() was not called.
*
*
* -
*
* SERVER_PROCESS_TERMINATED_UNHEALTHY -- The server process did not report a valid health check for too long and
* was therefore terminated by GameLift. Check your game session log to see if the thread became stuck processing a
* synchronous task for too long.
*
*
* -
*
* SERVER_PROCESS_FORCE_TERMINATED -- The server process did not exit cleanly after OnProcessTerminate() was sent
* within the time expected. Check your game session log to see why termination took longer than expected.
*
*
* -
*
* SERVER_PROCESS_PROCESS_EXIT_TIMEOUT -- The server process did not exit cleanly within the time expected after
* calling ProcessEnding(). Check your game session log to see why termination took longer than expected.
*
*
*
*
* Game session events:
*
*
* -
*
* GAME_SESSION_ACTIVATION_TIMEOUT -- GameSession failed to activate within the expected time. Check your game
* session log to see why ActivateGameSession() took longer to complete than expected.
*
*
*
*
* Other fleet events:
*
*
* -
*
* FLEET_SCALING_EVENT -- A change was made to the fleet's capacity settings (desired instances, minimum/maximum
* scaling limits). Event messaging includes the new capacity settings.
*
*
* -
*
* FLEET_NEW_GAME_SESSION_PROTECTION_POLICY_UPDATED -- A change was made to the fleet's game session protection
* policy setting. Event messaging includes both the old and new policy setting.
*
*
* -
*
* FLEET_DELETED -- A request to delete a fleet was initiated.
*
*
* -
*
* GENERIC_EVENT -- An unspecified event has occurred.
*
*
*
*
* @return The type of event being logged.
*
* Fleet state transition events:
*
*
* -
*
* FLEET_CREATED -- A fleet resource was successfully created with a status of NEW
. Event
* messaging includes the fleet ID.
*
*
* -
*
* FLEET_STATE_DOWNLOADING -- Fleet status changed from NEW
to DOWNLOADING
. The
* compressed build has started downloading to a fleet instance for installation.
*
*
* -
*
* FLEET_STATE_VALIDATING -- Fleet status changed from DOWNLOADING
to VALIDATING
.
* GameLift has successfully downloaded the build and is now validating the build files.
*
*
* -
*
* FLEET_STATE_BUILDING -- Fleet status changed from VALIDATING
to BUILDING
.
* GameLift has successfully verified the build files and is now running the installation scripts.
*
*
* -
*
* FLEET_STATE_ACTIVATING -- Fleet status changed from BUILDING
to ACTIVATING
.
* GameLift is trying to launch an instance and test the connectivity between the build and the GameLift
* Service via the Server SDK.
*
*
* -
*
* FLEET_STATE_ACTIVE -- The fleet's status changed from ACTIVATING
to ACTIVE
. The
* fleet is now ready to host game sessions.
*
*
* -
*
* FLEET_STATE_ERROR -- The Fleet's status changed to ERROR
. Describe the fleet event message
* for more details.
*
*
*
*
* Fleet creation events (ordered by fleet creation activity):
*
*
* -
*
* FLEET_BINARY_DOWNLOAD_FAILED -- The build failed to download to the fleet instance.
*
*
* -
*
* FLEET_CREATION_EXTRACTING_BUILD -- The game server build was successfully downloaded to an instance, and
* the build files are now being extracted from the uploaded build and saved to an instance. Failure at this
* stage prevents a fleet from moving to ACTIVE status. Logs for this stage display a list of the files that
* are extracted and saved on the instance. Access the logs by using the URL in PreSignedLogUrl.
*
*
* -
*
* FLEET_CREATION_RUNNING_INSTALLER -- The game server build files were successfully extracted, and the
* GameLift is now running the build's install script (if one is included). Failure in this stage prevents a
* fleet from moving to ACTIVE status. Logs for this stage list the installation steps and whether or not
* the install completed successfully. Access the logs by using the URL in PreSignedLogUrl.
*
*
* -
*
* FLEET_CREATION_VALIDATING_RUNTIME_CONFIG -- The build process was successful, and the GameLift is now
* verifying that the game server launch paths, which are specified in the fleet's runtime configuration,
* exist. If any listed launch path exists, GameLift tries to launch a game server process and waits for the
* process to report ready. Failures in this stage prevent a fleet from moving to ACTIVE
* status. Logs for this stage list the launch paths in the runtime configuration and indicate whether each
* is found. Access the logs by using the URL in PreSignedLogUrl.
*
*
* -
*
* FLEET_VALIDATION_LAUNCH_PATH_NOT_FOUND -- Validation of the runtime configuration failed because the
* executable specified in a launch path does not exist on the instance.
*
*
* -
*
* FLEET_VALIDATION_EXECUTABLE_RUNTIME_FAILURE -- Validation of the runtime configuration failed because the
* executable specified in a launch path failed to run on the fleet instance.
*
*
* -
*
* FLEET_VALIDATION_TIMED_OUT -- Validation of the fleet at the end of creation timed out. Try fleet
* creation again.
*
*
* -
*
* FLEET_ACTIVATION_FAILED -- The fleet failed to successfully complete one of the steps in the fleet
* activation process. This event code indicates that the game build was successfully downloaded to a fleet
* instance, built, and validated, but was not able to start a server process. For more information, see Debug Fleet Creation Issues.
*
*
* -
*
* FLEET_ACTIVATION_FAILED_NO_INSTANCES -- Fleet creation was not able to obtain any instances based on the
* input fleet attributes. Try again at a different time or choose a different combination of fleet
* attributes such as fleet type, instance type, etc.
*
*
* -
*
* FLEET_INITIALIZATION_FAILED -- A generic exception occurred during fleet creation. Describe the fleet
* event message for more details.
*
*
*
*
* VPC peering events:
*
*
* -
*
* FLEET_VPC_PEERING_SUCCEEDED -- A VPC peering connection has been established between the VPC for an
* GameLift fleet and a VPC in your Amazon Web Services account.
*
*
* -
*
* FLEET_VPC_PEERING_FAILED -- A requested VPC peering connection has failed. Event details and status
* information (see DescribeVpcPeeringConnections) provide additional detail. A common reason for
* peering failure is that the two VPCs have overlapping CIDR blocks of IPv4 addresses. To resolve this,
* change the CIDR block for the VPC in your Amazon Web Services account. For more information on VPC
* peering failures, see https://docs.aws.amazon.com/AmazonVPC/latest/PeeringGuide/invalid-peering-configurations.html
*
*
* -
*
* FLEET_VPC_PEERING_DELETED -- A VPC peering connection has been successfully deleted.
*
*
*
*
* Spot instance events:
*
*
* -
*
* INSTANCE_INTERRUPTED -- A spot instance was interrupted by EC2 with a two-minute notification.
*
*
*
*
* Spot process events:
*
*
* -
*
* SERVER_PROCESS_INVALID_PATH -- The game server executable or script could not be found based on the Fleet
* runtime configuration. Check that the launch path is correct based on the operating system of the Fleet.
*
*
* -
*
* SERVER_PROCESS_SDK_INITIALIZATION_TIMEOUT -- The server process did not call InitSDK() within the time
* expected. Check your game session log to see why InitSDK() was not called in time.
*
*
* -
*
* SERVER_PROCESS_PROCESS_READY_TIMEOUT -- The server process did not call ProcessReady() within the time
* expected after calling InitSDK(). Check your game session log to see why ProcessReady() was not called in
* time.
*
*
* -
*
* SERVER_PROCESS_CRASHED -- The server process exited without calling ProcessEnding(). Check your game
* session log to see why ProcessEnding() was not called.
*
*
* -
*
* SERVER_PROCESS_TERMINATED_UNHEALTHY -- The server process did not report a valid health check for too
* long and was therefore terminated by GameLift. Check your game session log to see if the thread became
* stuck processing a synchronous task for too long.
*
*
* -
*
* SERVER_PROCESS_FORCE_TERMINATED -- The server process did not exit cleanly after OnProcessTerminate() was
* sent within the time expected. Check your game session log to see why termination took longer than
* expected.
*
*
* -
*
* SERVER_PROCESS_PROCESS_EXIT_TIMEOUT -- The server process did not exit cleanly within the time expected
* after calling ProcessEnding(). Check your game session log to see why termination took longer than
* expected.
*
*
*
*
* Game session events:
*
*
* -
*
* GAME_SESSION_ACTIVATION_TIMEOUT -- GameSession failed to activate within the expected time. Check your
* game session log to see why ActivateGameSession() took longer to complete than expected.
*
*
*
*
* Other fleet events:
*
*
* -
*
* FLEET_SCALING_EVENT -- A change was made to the fleet's capacity settings (desired instances,
* minimum/maximum scaling limits). Event messaging includes the new capacity settings.
*
*
* -
*
* FLEET_NEW_GAME_SESSION_PROTECTION_POLICY_UPDATED -- A change was made to the fleet's game session
* protection policy setting. Event messaging includes both the old and new policy setting.
*
*
* -
*
* FLEET_DELETED -- A request to delete a fleet was initiated.
*
*
* -
*
* GENERIC_EVENT -- An unspecified event has occurred.
*
*
* @see EventCode
*/
public String getEventCode() {
return this.eventCode;
}
/**
*
* The type of event being logged.
*
*
* Fleet state transition events:
*
*
* -
*
* FLEET_CREATED -- A fleet resource was successfully created with a status of NEW
. Event messaging
* includes the fleet ID.
*
*
* -
*
* FLEET_STATE_DOWNLOADING -- Fleet status changed from NEW
to DOWNLOADING
. The compressed
* build has started downloading to a fleet instance for installation.
*
*
* -
*
* FLEET_STATE_VALIDATING -- Fleet status changed from DOWNLOADING
to VALIDATING
. GameLift
* has successfully downloaded the build and is now validating the build files.
*
*
* -
*
* FLEET_STATE_BUILDING -- Fleet status changed from VALIDATING
to BUILDING
. GameLift has
* successfully verified the build files and is now running the installation scripts.
*
*
* -
*
* FLEET_STATE_ACTIVATING -- Fleet status changed from BUILDING
to ACTIVATING
. GameLift is
* trying to launch an instance and test the connectivity between the build and the GameLift Service via the Server
* SDK.
*
*
* -
*
* FLEET_STATE_ACTIVE -- The fleet's status changed from ACTIVATING
to ACTIVE
. The fleet
* is now ready to host game sessions.
*
*
* -
*
* FLEET_STATE_ERROR -- The Fleet's status changed to ERROR
. Describe the fleet event message for more
* details.
*
*
*
*
* Fleet creation events (ordered by fleet creation activity):
*
*
* -
*
* FLEET_BINARY_DOWNLOAD_FAILED -- The build failed to download to the fleet instance.
*
*
* -
*
* FLEET_CREATION_EXTRACTING_BUILD -- The game server build was successfully downloaded to an instance, and the
* build files are now being extracted from the uploaded build and saved to an instance. Failure at this stage
* prevents a fleet from moving to ACTIVE status. Logs for this stage display a list of the files that are extracted
* and saved on the instance. Access the logs by using the URL in PreSignedLogUrl.
*
*
* -
*
* FLEET_CREATION_RUNNING_INSTALLER -- The game server build files were successfully extracted, and the GameLift is
* now running the build's install script (if one is included). Failure in this stage prevents a fleet from moving
* to ACTIVE status. Logs for this stage list the installation steps and whether or not the install completed
* successfully. Access the logs by using the URL in PreSignedLogUrl.
*
*
* -
*
* FLEET_CREATION_VALIDATING_RUNTIME_CONFIG -- The build process was successful, and the GameLift is now verifying
* that the game server launch paths, which are specified in the fleet's runtime configuration, exist. If any listed
* launch path exists, GameLift tries to launch a game server process and waits for the process to report ready.
* Failures in this stage prevent a fleet from moving to ACTIVE
status. Logs for this stage list the
* launch paths in the runtime configuration and indicate whether each is found. Access the logs by using the URL in
* PreSignedLogUrl.
*
*
* -
*
* FLEET_VALIDATION_LAUNCH_PATH_NOT_FOUND -- Validation of the runtime configuration failed because the executable
* specified in a launch path does not exist on the instance.
*
*
* -
*
* FLEET_VALIDATION_EXECUTABLE_RUNTIME_FAILURE -- Validation of the runtime configuration failed because the
* executable specified in a launch path failed to run on the fleet instance.
*
*
* -
*
* FLEET_VALIDATION_TIMED_OUT -- Validation of the fleet at the end of creation timed out. Try fleet creation again.
*
*
* -
*
* FLEET_ACTIVATION_FAILED -- The fleet failed to successfully complete one of the steps in the fleet activation
* process. This event code indicates that the game build was successfully downloaded to a fleet instance, built,
* and validated, but was not able to start a server process. For more information, see Debug Fleet Creation Issues.
*
*
* -
*
* FLEET_ACTIVATION_FAILED_NO_INSTANCES -- Fleet creation was not able to obtain any instances based on the input
* fleet attributes. Try again at a different time or choose a different combination of fleet attributes such as
* fleet type, instance type, etc.
*
*
* -
*
* FLEET_INITIALIZATION_FAILED -- A generic exception occurred during fleet creation. Describe the fleet event
* message for more details.
*
*
*
*
* VPC peering events:
*
*
* -
*
* FLEET_VPC_PEERING_SUCCEEDED -- A VPC peering connection has been established between the VPC for an GameLift
* fleet and a VPC in your Amazon Web Services account.
*
*
* -
*
* FLEET_VPC_PEERING_FAILED -- A requested VPC peering connection has failed. Event details and status information
* (see DescribeVpcPeeringConnections) provide additional detail. A common reason for peering failure is that
* the two VPCs have overlapping CIDR blocks of IPv4 addresses. To resolve this, change the CIDR block for the VPC
* in your Amazon Web Services account. For more information on VPC peering failures, see https://docs.aws.amazon.com/AmazonVPC/latest/PeeringGuide/invalid-peering-configurations.html
*
*
* -
*
* FLEET_VPC_PEERING_DELETED -- A VPC peering connection has been successfully deleted.
*
*
*
*
* Spot instance events:
*
*
* -
*
* INSTANCE_INTERRUPTED -- A spot instance was interrupted by EC2 with a two-minute notification.
*
*
*
*
* Spot process events:
*
*
* -
*
* SERVER_PROCESS_INVALID_PATH -- The game server executable or script could not be found based on the Fleet runtime
* configuration. Check that the launch path is correct based on the operating system of the Fleet.
*
*
* -
*
* SERVER_PROCESS_SDK_INITIALIZATION_TIMEOUT -- The server process did not call InitSDK() within the time expected.
* Check your game session log to see why InitSDK() was not called in time.
*
*
* -
*
* SERVER_PROCESS_PROCESS_READY_TIMEOUT -- The server process did not call ProcessReady() within the time expected
* after calling InitSDK(). Check your game session log to see why ProcessReady() was not called in time.
*
*
* -
*
* SERVER_PROCESS_CRASHED -- The server process exited without calling ProcessEnding(). Check your game session log
* to see why ProcessEnding() was not called.
*
*
* -
*
* SERVER_PROCESS_TERMINATED_UNHEALTHY -- The server process did not report a valid health check for too long and
* was therefore terminated by GameLift. Check your game session log to see if the thread became stuck processing a
* synchronous task for too long.
*
*
* -
*
* SERVER_PROCESS_FORCE_TERMINATED -- The server process did not exit cleanly after OnProcessTerminate() was sent
* within the time expected. Check your game session log to see why termination took longer than expected.
*
*
* -
*
* SERVER_PROCESS_PROCESS_EXIT_TIMEOUT -- The server process did not exit cleanly within the time expected after
* calling ProcessEnding(). Check your game session log to see why termination took longer than expected.
*
*
*
*
* Game session events:
*
*
* -
*
* GAME_SESSION_ACTIVATION_TIMEOUT -- GameSession failed to activate within the expected time. Check your game
* session log to see why ActivateGameSession() took longer to complete than expected.
*
*
*
*
* Other fleet events:
*
*
* -
*
* FLEET_SCALING_EVENT -- A change was made to the fleet's capacity settings (desired instances, minimum/maximum
* scaling limits). Event messaging includes the new capacity settings.
*
*
* -
*
* FLEET_NEW_GAME_SESSION_PROTECTION_POLICY_UPDATED -- A change was made to the fleet's game session protection
* policy setting. Event messaging includes both the old and new policy setting.
*
*
* -
*
* FLEET_DELETED -- A request to delete a fleet was initiated.
*
*
* -
*
* GENERIC_EVENT -- An unspecified event has occurred.
*
*
*
*
* @param eventCode
* The type of event being logged.
*
* Fleet state transition events:
*
*
* -
*
* FLEET_CREATED -- A fleet resource was successfully created with a status of NEW
. Event
* messaging includes the fleet ID.
*
*
* -
*
* FLEET_STATE_DOWNLOADING -- Fleet status changed from NEW
to DOWNLOADING
. The
* compressed build has started downloading to a fleet instance for installation.
*
*
* -
*
* FLEET_STATE_VALIDATING -- Fleet status changed from DOWNLOADING
to VALIDATING
.
* GameLift has successfully downloaded the build and is now validating the build files.
*
*
* -
*
* FLEET_STATE_BUILDING -- Fleet status changed from VALIDATING
to BUILDING
.
* GameLift has successfully verified the build files and is now running the installation scripts.
*
*
* -
*
* FLEET_STATE_ACTIVATING -- Fleet status changed from BUILDING
to ACTIVATING
.
* GameLift is trying to launch an instance and test the connectivity between the build and the GameLift
* Service via the Server SDK.
*
*
* -
*
* FLEET_STATE_ACTIVE -- The fleet's status changed from ACTIVATING
to ACTIVE
. The
* fleet is now ready to host game sessions.
*
*
* -
*
* FLEET_STATE_ERROR -- The Fleet's status changed to ERROR
. Describe the fleet event message
* for more details.
*
*
*
*
* Fleet creation events (ordered by fleet creation activity):
*
*
* -
*
* FLEET_BINARY_DOWNLOAD_FAILED -- The build failed to download to the fleet instance.
*
*
* -
*
* FLEET_CREATION_EXTRACTING_BUILD -- The game server build was successfully downloaded to an instance, and
* the build files are now being extracted from the uploaded build and saved to an instance. Failure at this
* stage prevents a fleet from moving to ACTIVE status. Logs for this stage display a list of the files that
* are extracted and saved on the instance. Access the logs by using the URL in PreSignedLogUrl.
*
*
* -
*
* FLEET_CREATION_RUNNING_INSTALLER -- The game server build files were successfully extracted, and the
* GameLift is now running the build's install script (if one is included). Failure in this stage prevents a
* fleet from moving to ACTIVE status. Logs for this stage list the installation steps and whether or not the
* install completed successfully. Access the logs by using the URL in PreSignedLogUrl.
*
*
* -
*
* FLEET_CREATION_VALIDATING_RUNTIME_CONFIG -- The build process was successful, and the GameLift is now
* verifying that the game server launch paths, which are specified in the fleet's runtime configuration,
* exist. If any listed launch path exists, GameLift tries to launch a game server process and waits for the
* process to report ready. Failures in this stage prevent a fleet from moving to ACTIVE
status.
* Logs for this stage list the launch paths in the runtime configuration and indicate whether each is found.
* Access the logs by using the URL in PreSignedLogUrl.
*
*
* -
*
* FLEET_VALIDATION_LAUNCH_PATH_NOT_FOUND -- Validation of the runtime configuration failed because the
* executable specified in a launch path does not exist on the instance.
*
*
* -
*
* FLEET_VALIDATION_EXECUTABLE_RUNTIME_FAILURE -- Validation of the runtime configuration failed because the
* executable specified in a launch path failed to run on the fleet instance.
*
*
* -
*
* FLEET_VALIDATION_TIMED_OUT -- Validation of the fleet at the end of creation timed out. Try fleet creation
* again.
*
*
* -
*
* FLEET_ACTIVATION_FAILED -- The fleet failed to successfully complete one of the steps in the fleet
* activation process. This event code indicates that the game build was successfully downloaded to a fleet
* instance, built, and validated, but was not able to start a server process. For more information, see Debug Fleet Creation Issues.
*
*
* -
*
* FLEET_ACTIVATION_FAILED_NO_INSTANCES -- Fleet creation was not able to obtain any instances based on the
* input fleet attributes. Try again at a different time or choose a different combination of fleet
* attributes such as fleet type, instance type, etc.
*
*
* -
*
* FLEET_INITIALIZATION_FAILED -- A generic exception occurred during fleet creation. Describe the fleet
* event message for more details.
*
*
*
*
* VPC peering events:
*
*
* -
*
* FLEET_VPC_PEERING_SUCCEEDED -- A VPC peering connection has been established between the VPC for an
* GameLift fleet and a VPC in your Amazon Web Services account.
*
*
* -
*
* FLEET_VPC_PEERING_FAILED -- A requested VPC peering connection has failed. Event details and status
* information (see DescribeVpcPeeringConnections) provide additional detail. A common reason for
* peering failure is that the two VPCs have overlapping CIDR blocks of IPv4 addresses. To resolve this,
* change the CIDR block for the VPC in your Amazon Web Services account. For more information on VPC peering
* failures, see https://docs.aws.amazon.com/AmazonVPC/latest/PeeringGuide/invalid-peering-configurations.html
*
*
* -
*
* FLEET_VPC_PEERING_DELETED -- A VPC peering connection has been successfully deleted.
*
*
*
*
* Spot instance events:
*
*
* -
*
* INSTANCE_INTERRUPTED -- A spot instance was interrupted by EC2 with a two-minute notification.
*
*
*
*
* Spot process events:
*
*
* -
*
* SERVER_PROCESS_INVALID_PATH -- The game server executable or script could not be found based on the Fleet
* runtime configuration. Check that the launch path is correct based on the operating system of the Fleet.
*
*
* -
*
* SERVER_PROCESS_SDK_INITIALIZATION_TIMEOUT -- The server process did not call InitSDK() within the time
* expected. Check your game session log to see why InitSDK() was not called in time.
*
*
* -
*
* SERVER_PROCESS_PROCESS_READY_TIMEOUT -- The server process did not call ProcessReady() within the time
* expected after calling InitSDK(). Check your game session log to see why ProcessReady() was not called in
* time.
*
*
* -
*
* SERVER_PROCESS_CRASHED -- The server process exited without calling ProcessEnding(). Check your game
* session log to see why ProcessEnding() was not called.
*
*
* -
*
* SERVER_PROCESS_TERMINATED_UNHEALTHY -- The server process did not report a valid health check for too long
* and was therefore terminated by GameLift. Check your game session log to see if the thread became stuck
* processing a synchronous task for too long.
*
*
* -
*
* SERVER_PROCESS_FORCE_TERMINATED -- The server process did not exit cleanly after OnProcessTerminate() was
* sent within the time expected. Check your game session log to see why termination took longer than
* expected.
*
*
* -
*
* SERVER_PROCESS_PROCESS_EXIT_TIMEOUT -- The server process did not exit cleanly within the time expected
* after calling ProcessEnding(). Check your game session log to see why termination took longer than
* expected.
*
*
*
*
* Game session events:
*
*
* -
*
* GAME_SESSION_ACTIVATION_TIMEOUT -- GameSession failed to activate within the expected time. Check your
* game session log to see why ActivateGameSession() took longer to complete than expected.
*
*
*
*
* Other fleet events:
*
*
* -
*
* FLEET_SCALING_EVENT -- A change was made to the fleet's capacity settings (desired instances,
* minimum/maximum scaling limits). Event messaging includes the new capacity settings.
*
*
* -
*
* FLEET_NEW_GAME_SESSION_PROTECTION_POLICY_UPDATED -- A change was made to the fleet's game session
* protection policy setting. Event messaging includes both the old and new policy setting.
*
*
* -
*
* FLEET_DELETED -- A request to delete a fleet was initiated.
*
*
* -
*
* GENERIC_EVENT -- An unspecified event has occurred.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see EventCode
*/
public Event withEventCode(String eventCode) {
setEventCode(eventCode);
return this;
}
/**
*
* The type of event being logged.
*
*
* Fleet state transition events:
*
*
* -
*
* FLEET_CREATED -- A fleet resource was successfully created with a status of NEW
. Event messaging
* includes the fleet ID.
*
*
* -
*
* FLEET_STATE_DOWNLOADING -- Fleet status changed from NEW
to DOWNLOADING
. The compressed
* build has started downloading to a fleet instance for installation.
*
*
* -
*
* FLEET_STATE_VALIDATING -- Fleet status changed from DOWNLOADING
to VALIDATING
. GameLift
* has successfully downloaded the build and is now validating the build files.
*
*
* -
*
* FLEET_STATE_BUILDING -- Fleet status changed from VALIDATING
to BUILDING
. GameLift has
* successfully verified the build files and is now running the installation scripts.
*
*
* -
*
* FLEET_STATE_ACTIVATING -- Fleet status changed from BUILDING
to ACTIVATING
. GameLift is
* trying to launch an instance and test the connectivity between the build and the GameLift Service via the Server
* SDK.
*
*
* -
*
* FLEET_STATE_ACTIVE -- The fleet's status changed from ACTIVATING
to ACTIVE
. The fleet
* is now ready to host game sessions.
*
*
* -
*
* FLEET_STATE_ERROR -- The Fleet's status changed to ERROR
. Describe the fleet event message for more
* details.
*
*
*
*
* Fleet creation events (ordered by fleet creation activity):
*
*
* -
*
* FLEET_BINARY_DOWNLOAD_FAILED -- The build failed to download to the fleet instance.
*
*
* -
*
* FLEET_CREATION_EXTRACTING_BUILD -- The game server build was successfully downloaded to an instance, and the
* build files are now being extracted from the uploaded build and saved to an instance. Failure at this stage
* prevents a fleet from moving to ACTIVE status. Logs for this stage display a list of the files that are extracted
* and saved on the instance. Access the logs by using the URL in PreSignedLogUrl.
*
*
* -
*
* FLEET_CREATION_RUNNING_INSTALLER -- The game server build files were successfully extracted, and the GameLift is
* now running the build's install script (if one is included). Failure in this stage prevents a fleet from moving
* to ACTIVE status. Logs for this stage list the installation steps and whether or not the install completed
* successfully. Access the logs by using the URL in PreSignedLogUrl.
*
*
* -
*
* FLEET_CREATION_VALIDATING_RUNTIME_CONFIG -- The build process was successful, and the GameLift is now verifying
* that the game server launch paths, which are specified in the fleet's runtime configuration, exist. If any listed
* launch path exists, GameLift tries to launch a game server process and waits for the process to report ready.
* Failures in this stage prevent a fleet from moving to ACTIVE
status. Logs for this stage list the
* launch paths in the runtime configuration and indicate whether each is found. Access the logs by using the URL in
* PreSignedLogUrl.
*
*
* -
*
* FLEET_VALIDATION_LAUNCH_PATH_NOT_FOUND -- Validation of the runtime configuration failed because the executable
* specified in a launch path does not exist on the instance.
*
*
* -
*
* FLEET_VALIDATION_EXECUTABLE_RUNTIME_FAILURE -- Validation of the runtime configuration failed because the
* executable specified in a launch path failed to run on the fleet instance.
*
*
* -
*
* FLEET_VALIDATION_TIMED_OUT -- Validation of the fleet at the end of creation timed out. Try fleet creation again.
*
*
* -
*
* FLEET_ACTIVATION_FAILED -- The fleet failed to successfully complete one of the steps in the fleet activation
* process. This event code indicates that the game build was successfully downloaded to a fleet instance, built,
* and validated, but was not able to start a server process. For more information, see Debug Fleet Creation Issues.
*
*
* -
*
* FLEET_ACTIVATION_FAILED_NO_INSTANCES -- Fleet creation was not able to obtain any instances based on the input
* fleet attributes. Try again at a different time or choose a different combination of fleet attributes such as
* fleet type, instance type, etc.
*
*
* -
*
* FLEET_INITIALIZATION_FAILED -- A generic exception occurred during fleet creation. Describe the fleet event
* message for more details.
*
*
*
*
* VPC peering events:
*
*
* -
*
* FLEET_VPC_PEERING_SUCCEEDED -- A VPC peering connection has been established between the VPC for an GameLift
* fleet and a VPC in your Amazon Web Services account.
*
*
* -
*
* FLEET_VPC_PEERING_FAILED -- A requested VPC peering connection has failed. Event details and status information
* (see DescribeVpcPeeringConnections) provide additional detail. A common reason for peering failure is that
* the two VPCs have overlapping CIDR blocks of IPv4 addresses. To resolve this, change the CIDR block for the VPC
* in your Amazon Web Services account. For more information on VPC peering failures, see https://docs.aws.amazon.com/AmazonVPC/latest/PeeringGuide/invalid-peering-configurations.html
*
*
* -
*
* FLEET_VPC_PEERING_DELETED -- A VPC peering connection has been successfully deleted.
*
*
*
*
* Spot instance events:
*
*
* -
*
* INSTANCE_INTERRUPTED -- A spot instance was interrupted by EC2 with a two-minute notification.
*
*
*
*
* Spot process events:
*
*
* -
*
* SERVER_PROCESS_INVALID_PATH -- The game server executable or script could not be found based on the Fleet runtime
* configuration. Check that the launch path is correct based on the operating system of the Fleet.
*
*
* -
*
* SERVER_PROCESS_SDK_INITIALIZATION_TIMEOUT -- The server process did not call InitSDK() within the time expected.
* Check your game session log to see why InitSDK() was not called in time.
*
*
* -
*
* SERVER_PROCESS_PROCESS_READY_TIMEOUT -- The server process did not call ProcessReady() within the time expected
* after calling InitSDK(). Check your game session log to see why ProcessReady() was not called in time.
*
*
* -
*
* SERVER_PROCESS_CRASHED -- The server process exited without calling ProcessEnding(). Check your game session log
* to see why ProcessEnding() was not called.
*
*
* -
*
* SERVER_PROCESS_TERMINATED_UNHEALTHY -- The server process did not report a valid health check for too long and
* was therefore terminated by GameLift. Check your game session log to see if the thread became stuck processing a
* synchronous task for too long.
*
*
* -
*
* SERVER_PROCESS_FORCE_TERMINATED -- The server process did not exit cleanly after OnProcessTerminate() was sent
* within the time expected. Check your game session log to see why termination took longer than expected.
*
*
* -
*
* SERVER_PROCESS_PROCESS_EXIT_TIMEOUT -- The server process did not exit cleanly within the time expected after
* calling ProcessEnding(). Check your game session log to see why termination took longer than expected.
*
*
*
*
* Game session events:
*
*
* -
*
* GAME_SESSION_ACTIVATION_TIMEOUT -- GameSession failed to activate within the expected time. Check your game
* session log to see why ActivateGameSession() took longer to complete than expected.
*
*
*
*
* Other fleet events:
*
*
* -
*
* FLEET_SCALING_EVENT -- A change was made to the fleet's capacity settings (desired instances, minimum/maximum
* scaling limits). Event messaging includes the new capacity settings.
*
*
* -
*
* FLEET_NEW_GAME_SESSION_PROTECTION_POLICY_UPDATED -- A change was made to the fleet's game session protection
* policy setting. Event messaging includes both the old and new policy setting.
*
*
* -
*
* FLEET_DELETED -- A request to delete a fleet was initiated.
*
*
* -
*
* GENERIC_EVENT -- An unspecified event has occurred.
*
*
*
*
* @param eventCode
* The type of event being logged.
*
* Fleet state transition events:
*
*
* -
*
* FLEET_CREATED -- A fleet resource was successfully created with a status of NEW
. Event
* messaging includes the fleet ID.
*
*
* -
*
* FLEET_STATE_DOWNLOADING -- Fleet status changed from NEW
to DOWNLOADING
. The
* compressed build has started downloading to a fleet instance for installation.
*
*
* -
*
* FLEET_STATE_VALIDATING -- Fleet status changed from DOWNLOADING
to VALIDATING
.
* GameLift has successfully downloaded the build and is now validating the build files.
*
*
* -
*
* FLEET_STATE_BUILDING -- Fleet status changed from VALIDATING
to BUILDING
.
* GameLift has successfully verified the build files and is now running the installation scripts.
*
*
* -
*
* FLEET_STATE_ACTIVATING -- Fleet status changed from BUILDING
to ACTIVATING
.
* GameLift is trying to launch an instance and test the connectivity between the build and the GameLift
* Service via the Server SDK.
*
*
* -
*
* FLEET_STATE_ACTIVE -- The fleet's status changed from ACTIVATING
to ACTIVE
. The
* fleet is now ready to host game sessions.
*
*
* -
*
* FLEET_STATE_ERROR -- The Fleet's status changed to ERROR
. Describe the fleet event message
* for more details.
*
*
*
*
* Fleet creation events (ordered by fleet creation activity):
*
*
* -
*
* FLEET_BINARY_DOWNLOAD_FAILED -- The build failed to download to the fleet instance.
*
*
* -
*
* FLEET_CREATION_EXTRACTING_BUILD -- The game server build was successfully downloaded to an instance, and
* the build files are now being extracted from the uploaded build and saved to an instance. Failure at this
* stage prevents a fleet from moving to ACTIVE status. Logs for this stage display a list of the files that
* are extracted and saved on the instance. Access the logs by using the URL in PreSignedLogUrl.
*
*
* -
*
* FLEET_CREATION_RUNNING_INSTALLER -- The game server build files were successfully extracted, and the
* GameLift is now running the build's install script (if one is included). Failure in this stage prevents a
* fleet from moving to ACTIVE status. Logs for this stage list the installation steps and whether or not the
* install completed successfully. Access the logs by using the URL in PreSignedLogUrl.
*
*
* -
*
* FLEET_CREATION_VALIDATING_RUNTIME_CONFIG -- The build process was successful, and the GameLift is now
* verifying that the game server launch paths, which are specified in the fleet's runtime configuration,
* exist. If any listed launch path exists, GameLift tries to launch a game server process and waits for the
* process to report ready. Failures in this stage prevent a fleet from moving to ACTIVE
status.
* Logs for this stage list the launch paths in the runtime configuration and indicate whether each is found.
* Access the logs by using the URL in PreSignedLogUrl.
*
*
* -
*
* FLEET_VALIDATION_LAUNCH_PATH_NOT_FOUND -- Validation of the runtime configuration failed because the
* executable specified in a launch path does not exist on the instance.
*
*
* -
*
* FLEET_VALIDATION_EXECUTABLE_RUNTIME_FAILURE -- Validation of the runtime configuration failed because the
* executable specified in a launch path failed to run on the fleet instance.
*
*
* -
*
* FLEET_VALIDATION_TIMED_OUT -- Validation of the fleet at the end of creation timed out. Try fleet creation
* again.
*
*
* -
*
* FLEET_ACTIVATION_FAILED -- The fleet failed to successfully complete one of the steps in the fleet
* activation process. This event code indicates that the game build was successfully downloaded to a fleet
* instance, built, and validated, but was not able to start a server process. For more information, see Debug Fleet Creation Issues.
*
*
* -
*
* FLEET_ACTIVATION_FAILED_NO_INSTANCES -- Fleet creation was not able to obtain any instances based on the
* input fleet attributes. Try again at a different time or choose a different combination of fleet
* attributes such as fleet type, instance type, etc.
*
*
* -
*
* FLEET_INITIALIZATION_FAILED -- A generic exception occurred during fleet creation. Describe the fleet
* event message for more details.
*
*
*
*
* VPC peering events:
*
*
* -
*
* FLEET_VPC_PEERING_SUCCEEDED -- A VPC peering connection has been established between the VPC for an
* GameLift fleet and a VPC in your Amazon Web Services account.
*
*
* -
*
* FLEET_VPC_PEERING_FAILED -- A requested VPC peering connection has failed. Event details and status
* information (see DescribeVpcPeeringConnections) provide additional detail. A common reason for
* peering failure is that the two VPCs have overlapping CIDR blocks of IPv4 addresses. To resolve this,
* change the CIDR block for the VPC in your Amazon Web Services account. For more information on VPC peering
* failures, see https://docs.aws.amazon.com/AmazonVPC/latest/PeeringGuide/invalid-peering-configurations.html
*
*
* -
*
* FLEET_VPC_PEERING_DELETED -- A VPC peering connection has been successfully deleted.
*
*
*
*
* Spot instance events:
*
*
* -
*
* INSTANCE_INTERRUPTED -- A spot instance was interrupted by EC2 with a two-minute notification.
*
*
*
*
* Spot process events:
*
*
* -
*
* SERVER_PROCESS_INVALID_PATH -- The game server executable or script could not be found based on the Fleet
* runtime configuration. Check that the launch path is correct based on the operating system of the Fleet.
*
*
* -
*
* SERVER_PROCESS_SDK_INITIALIZATION_TIMEOUT -- The server process did not call InitSDK() within the time
* expected. Check your game session log to see why InitSDK() was not called in time.
*
*
* -
*
* SERVER_PROCESS_PROCESS_READY_TIMEOUT -- The server process did not call ProcessReady() within the time
* expected after calling InitSDK(). Check your game session log to see why ProcessReady() was not called in
* time.
*
*
* -
*
* SERVER_PROCESS_CRASHED -- The server process exited without calling ProcessEnding(). Check your game
* session log to see why ProcessEnding() was not called.
*
*
* -
*
* SERVER_PROCESS_TERMINATED_UNHEALTHY -- The server process did not report a valid health check for too long
* and was therefore terminated by GameLift. Check your game session log to see if the thread became stuck
* processing a synchronous task for too long.
*
*
* -
*
* SERVER_PROCESS_FORCE_TERMINATED -- The server process did not exit cleanly after OnProcessTerminate() was
* sent within the time expected. Check your game session log to see why termination took longer than
* expected.
*
*
* -
*
* SERVER_PROCESS_PROCESS_EXIT_TIMEOUT -- The server process did not exit cleanly within the time expected
* after calling ProcessEnding(). Check your game session log to see why termination took longer than
* expected.
*
*
*
*
* Game session events:
*
*
* -
*
* GAME_SESSION_ACTIVATION_TIMEOUT -- GameSession failed to activate within the expected time. Check your
* game session log to see why ActivateGameSession() took longer to complete than expected.
*
*
*
*
* Other fleet events:
*
*
* -
*
* FLEET_SCALING_EVENT -- A change was made to the fleet's capacity settings (desired instances,
* minimum/maximum scaling limits). Event messaging includes the new capacity settings.
*
*
* -
*
* FLEET_NEW_GAME_SESSION_PROTECTION_POLICY_UPDATED -- A change was made to the fleet's game session
* protection policy setting. Event messaging includes both the old and new policy setting.
*
*
* -
*
* FLEET_DELETED -- A request to delete a fleet was initiated.
*
*
* -
*
* GENERIC_EVENT -- An unspecified event has occurred.
*
*
* @see EventCode
*/
public void setEventCode(EventCode eventCode) {
withEventCode(eventCode);
}
/**
*
* The type of event being logged.
*
*
* Fleet state transition events:
*
*
* -
*
* FLEET_CREATED -- A fleet resource was successfully created with a status of NEW
. Event messaging
* includes the fleet ID.
*
*
* -
*
* FLEET_STATE_DOWNLOADING -- Fleet status changed from NEW
to DOWNLOADING
. The compressed
* build has started downloading to a fleet instance for installation.
*
*
* -
*
* FLEET_STATE_VALIDATING -- Fleet status changed from DOWNLOADING
to VALIDATING
. GameLift
* has successfully downloaded the build and is now validating the build files.
*
*
* -
*
* FLEET_STATE_BUILDING -- Fleet status changed from VALIDATING
to BUILDING
. GameLift has
* successfully verified the build files and is now running the installation scripts.
*
*
* -
*
* FLEET_STATE_ACTIVATING -- Fleet status changed from BUILDING
to ACTIVATING
. GameLift is
* trying to launch an instance and test the connectivity between the build and the GameLift Service via the Server
* SDK.
*
*
* -
*
* FLEET_STATE_ACTIVE -- The fleet's status changed from ACTIVATING
to ACTIVE
. The fleet
* is now ready to host game sessions.
*
*
* -
*
* FLEET_STATE_ERROR -- The Fleet's status changed to ERROR
. Describe the fleet event message for more
* details.
*
*
*
*
* Fleet creation events (ordered by fleet creation activity):
*
*
* -
*
* FLEET_BINARY_DOWNLOAD_FAILED -- The build failed to download to the fleet instance.
*
*
* -
*
* FLEET_CREATION_EXTRACTING_BUILD -- The game server build was successfully downloaded to an instance, and the
* build files are now being extracted from the uploaded build and saved to an instance. Failure at this stage
* prevents a fleet from moving to ACTIVE status. Logs for this stage display a list of the files that are extracted
* and saved on the instance. Access the logs by using the URL in PreSignedLogUrl.
*
*
* -
*
* FLEET_CREATION_RUNNING_INSTALLER -- The game server build files were successfully extracted, and the GameLift is
* now running the build's install script (if one is included). Failure in this stage prevents a fleet from moving
* to ACTIVE status. Logs for this stage list the installation steps and whether or not the install completed
* successfully. Access the logs by using the URL in PreSignedLogUrl.
*
*
* -
*
* FLEET_CREATION_VALIDATING_RUNTIME_CONFIG -- The build process was successful, and the GameLift is now verifying
* that the game server launch paths, which are specified in the fleet's runtime configuration, exist. If any listed
* launch path exists, GameLift tries to launch a game server process and waits for the process to report ready.
* Failures in this stage prevent a fleet from moving to ACTIVE
status. Logs for this stage list the
* launch paths in the runtime configuration and indicate whether each is found. Access the logs by using the URL in
* PreSignedLogUrl.
*
*
* -
*
* FLEET_VALIDATION_LAUNCH_PATH_NOT_FOUND -- Validation of the runtime configuration failed because the executable
* specified in a launch path does not exist on the instance.
*
*
* -
*
* FLEET_VALIDATION_EXECUTABLE_RUNTIME_FAILURE -- Validation of the runtime configuration failed because the
* executable specified in a launch path failed to run on the fleet instance.
*
*
* -
*
* FLEET_VALIDATION_TIMED_OUT -- Validation of the fleet at the end of creation timed out. Try fleet creation again.
*
*
* -
*
* FLEET_ACTIVATION_FAILED -- The fleet failed to successfully complete one of the steps in the fleet activation
* process. This event code indicates that the game build was successfully downloaded to a fleet instance, built,
* and validated, but was not able to start a server process. For more information, see Debug Fleet Creation Issues.
*
*
* -
*
* FLEET_ACTIVATION_FAILED_NO_INSTANCES -- Fleet creation was not able to obtain any instances based on the input
* fleet attributes. Try again at a different time or choose a different combination of fleet attributes such as
* fleet type, instance type, etc.
*
*
* -
*
* FLEET_INITIALIZATION_FAILED -- A generic exception occurred during fleet creation. Describe the fleet event
* message for more details.
*
*
*
*
* VPC peering events:
*
*
* -
*
* FLEET_VPC_PEERING_SUCCEEDED -- A VPC peering connection has been established between the VPC for an GameLift
* fleet and a VPC in your Amazon Web Services account.
*
*
* -
*
* FLEET_VPC_PEERING_FAILED -- A requested VPC peering connection has failed. Event details and status information
* (see DescribeVpcPeeringConnections) provide additional detail. A common reason for peering failure is that
* the two VPCs have overlapping CIDR blocks of IPv4 addresses. To resolve this, change the CIDR block for the VPC
* in your Amazon Web Services account. For more information on VPC peering failures, see https://docs.aws.amazon.com/AmazonVPC/latest/PeeringGuide/invalid-peering-configurations.html
*
*
* -
*
* FLEET_VPC_PEERING_DELETED -- A VPC peering connection has been successfully deleted.
*
*
*
*
* Spot instance events:
*
*
* -
*
* INSTANCE_INTERRUPTED -- A spot instance was interrupted by EC2 with a two-minute notification.
*
*
*
*
* Spot process events:
*
*
* -
*
* SERVER_PROCESS_INVALID_PATH -- The game server executable or script could not be found based on the Fleet runtime
* configuration. Check that the launch path is correct based on the operating system of the Fleet.
*
*
* -
*
* SERVER_PROCESS_SDK_INITIALIZATION_TIMEOUT -- The server process did not call InitSDK() within the time expected.
* Check your game session log to see why InitSDK() was not called in time.
*
*
* -
*
* SERVER_PROCESS_PROCESS_READY_TIMEOUT -- The server process did not call ProcessReady() within the time expected
* after calling InitSDK(). Check your game session log to see why ProcessReady() was not called in time.
*
*
* -
*
* SERVER_PROCESS_CRASHED -- The server process exited without calling ProcessEnding(). Check your game session log
* to see why ProcessEnding() was not called.
*
*
* -
*
* SERVER_PROCESS_TERMINATED_UNHEALTHY -- The server process did not report a valid health check for too long and
* was therefore terminated by GameLift. Check your game session log to see if the thread became stuck processing a
* synchronous task for too long.
*
*
* -
*
* SERVER_PROCESS_FORCE_TERMINATED -- The server process did not exit cleanly after OnProcessTerminate() was sent
* within the time expected. Check your game session log to see why termination took longer than expected.
*
*
* -
*
* SERVER_PROCESS_PROCESS_EXIT_TIMEOUT -- The server process did not exit cleanly within the time expected after
* calling ProcessEnding(). Check your game session log to see why termination took longer than expected.
*
*
*
*
* Game session events:
*
*
* -
*
* GAME_SESSION_ACTIVATION_TIMEOUT -- GameSession failed to activate within the expected time. Check your game
* session log to see why ActivateGameSession() took longer to complete than expected.
*
*
*
*
* Other fleet events:
*
*
* -
*
* FLEET_SCALING_EVENT -- A change was made to the fleet's capacity settings (desired instances, minimum/maximum
* scaling limits). Event messaging includes the new capacity settings.
*
*
* -
*
* FLEET_NEW_GAME_SESSION_PROTECTION_POLICY_UPDATED -- A change was made to the fleet's game session protection
* policy setting. Event messaging includes both the old and new policy setting.
*
*
* -
*
* FLEET_DELETED -- A request to delete a fleet was initiated.
*
*
* -
*
* GENERIC_EVENT -- An unspecified event has occurred.
*
*
*
*
* @param eventCode
* The type of event being logged.
*
* Fleet state transition events:
*
*
* -
*
* FLEET_CREATED -- A fleet resource was successfully created with a status of NEW
. Event
* messaging includes the fleet ID.
*
*
* -
*
* FLEET_STATE_DOWNLOADING -- Fleet status changed from NEW
to DOWNLOADING
. The
* compressed build has started downloading to a fleet instance for installation.
*
*
* -
*
* FLEET_STATE_VALIDATING -- Fleet status changed from DOWNLOADING
to VALIDATING
.
* GameLift has successfully downloaded the build and is now validating the build files.
*
*
* -
*
* FLEET_STATE_BUILDING -- Fleet status changed from VALIDATING
to BUILDING
.
* GameLift has successfully verified the build files and is now running the installation scripts.
*
*
* -
*
* FLEET_STATE_ACTIVATING -- Fleet status changed from BUILDING
to ACTIVATING
.
* GameLift is trying to launch an instance and test the connectivity between the build and the GameLift
* Service via the Server SDK.
*
*
* -
*
* FLEET_STATE_ACTIVE -- The fleet's status changed from ACTIVATING
to ACTIVE
. The
* fleet is now ready to host game sessions.
*
*
* -
*
* FLEET_STATE_ERROR -- The Fleet's status changed to ERROR
. Describe the fleet event message
* for more details.
*
*
*
*
* Fleet creation events (ordered by fleet creation activity):
*
*
* -
*
* FLEET_BINARY_DOWNLOAD_FAILED -- The build failed to download to the fleet instance.
*
*
* -
*
* FLEET_CREATION_EXTRACTING_BUILD -- The game server build was successfully downloaded to an instance, and
* the build files are now being extracted from the uploaded build and saved to an instance. Failure at this
* stage prevents a fleet from moving to ACTIVE status. Logs for this stage display a list of the files that
* are extracted and saved on the instance. Access the logs by using the URL in PreSignedLogUrl.
*
*
* -
*
* FLEET_CREATION_RUNNING_INSTALLER -- The game server build files were successfully extracted, and the
* GameLift is now running the build's install script (if one is included). Failure in this stage prevents a
* fleet from moving to ACTIVE status. Logs for this stage list the installation steps and whether or not the
* install completed successfully. Access the logs by using the URL in PreSignedLogUrl.
*
*
* -
*
* FLEET_CREATION_VALIDATING_RUNTIME_CONFIG -- The build process was successful, and the GameLift is now
* verifying that the game server launch paths, which are specified in the fleet's runtime configuration,
* exist. If any listed launch path exists, GameLift tries to launch a game server process and waits for the
* process to report ready. Failures in this stage prevent a fleet from moving to ACTIVE
status.
* Logs for this stage list the launch paths in the runtime configuration and indicate whether each is found.
* Access the logs by using the URL in PreSignedLogUrl.
*
*
* -
*
* FLEET_VALIDATION_LAUNCH_PATH_NOT_FOUND -- Validation of the runtime configuration failed because the
* executable specified in a launch path does not exist on the instance.
*
*
* -
*
* FLEET_VALIDATION_EXECUTABLE_RUNTIME_FAILURE -- Validation of the runtime configuration failed because the
* executable specified in a launch path failed to run on the fleet instance.
*
*
* -
*
* FLEET_VALIDATION_TIMED_OUT -- Validation of the fleet at the end of creation timed out. Try fleet creation
* again.
*
*
* -
*
* FLEET_ACTIVATION_FAILED -- The fleet failed to successfully complete one of the steps in the fleet
* activation process. This event code indicates that the game build was successfully downloaded to a fleet
* instance, built, and validated, but was not able to start a server process. For more information, see Debug Fleet Creation Issues.
*
*
* -
*
* FLEET_ACTIVATION_FAILED_NO_INSTANCES -- Fleet creation was not able to obtain any instances based on the
* input fleet attributes. Try again at a different time or choose a different combination of fleet
* attributes such as fleet type, instance type, etc.
*
*
* -
*
* FLEET_INITIALIZATION_FAILED -- A generic exception occurred during fleet creation. Describe the fleet
* event message for more details.
*
*
*
*
* VPC peering events:
*
*
* -
*
* FLEET_VPC_PEERING_SUCCEEDED -- A VPC peering connection has been established between the VPC for an
* GameLift fleet and a VPC in your Amazon Web Services account.
*
*
* -
*
* FLEET_VPC_PEERING_FAILED -- A requested VPC peering connection has failed. Event details and status
* information (see DescribeVpcPeeringConnections) provide additional detail. A common reason for
* peering failure is that the two VPCs have overlapping CIDR blocks of IPv4 addresses. To resolve this,
* change the CIDR block for the VPC in your Amazon Web Services account. For more information on VPC peering
* failures, see https://docs.aws.amazon.com/AmazonVPC/latest/PeeringGuide/invalid-peering-configurations.html
*
*
* -
*
* FLEET_VPC_PEERING_DELETED -- A VPC peering connection has been successfully deleted.
*
*
*
*
* Spot instance events:
*
*
* -
*
* INSTANCE_INTERRUPTED -- A spot instance was interrupted by EC2 with a two-minute notification.
*
*
*
*
* Spot process events:
*
*
* -
*
* SERVER_PROCESS_INVALID_PATH -- The game server executable or script could not be found based on the Fleet
* runtime configuration. Check that the launch path is correct based on the operating system of the Fleet.
*
*
* -
*
* SERVER_PROCESS_SDK_INITIALIZATION_TIMEOUT -- The server process did not call InitSDK() within the time
* expected. Check your game session log to see why InitSDK() was not called in time.
*
*
* -
*
* SERVER_PROCESS_PROCESS_READY_TIMEOUT -- The server process did not call ProcessReady() within the time
* expected after calling InitSDK(). Check your game session log to see why ProcessReady() was not called in
* time.
*
*
* -
*
* SERVER_PROCESS_CRASHED -- The server process exited without calling ProcessEnding(). Check your game
* session log to see why ProcessEnding() was not called.
*
*
* -
*
* SERVER_PROCESS_TERMINATED_UNHEALTHY -- The server process did not report a valid health check for too long
* and was therefore terminated by GameLift. Check your game session log to see if the thread became stuck
* processing a synchronous task for too long.
*
*
* -
*
* SERVER_PROCESS_FORCE_TERMINATED -- The server process did not exit cleanly after OnProcessTerminate() was
* sent within the time expected. Check your game session log to see why termination took longer than
* expected.
*
*
* -
*
* SERVER_PROCESS_PROCESS_EXIT_TIMEOUT -- The server process did not exit cleanly within the time expected
* after calling ProcessEnding(). Check your game session log to see why termination took longer than
* expected.
*
*
*
*
* Game session events:
*
*
* -
*
* GAME_SESSION_ACTIVATION_TIMEOUT -- GameSession failed to activate within the expected time. Check your
* game session log to see why ActivateGameSession() took longer to complete than expected.
*
*
*
*
* Other fleet events:
*
*
* -
*
* FLEET_SCALING_EVENT -- A change was made to the fleet's capacity settings (desired instances,
* minimum/maximum scaling limits). Event messaging includes the new capacity settings.
*
*
* -
*
* FLEET_NEW_GAME_SESSION_PROTECTION_POLICY_UPDATED -- A change was made to the fleet's game session
* protection policy setting. Event messaging includes both the old and new policy setting.
*
*
* -
*
* FLEET_DELETED -- A request to delete a fleet was initiated.
*
*
* -
*
* GENERIC_EVENT -- An unspecified event has occurred.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see EventCode
*/
public Event withEventCode(EventCode eventCode) {
this.eventCode = eventCode.toString();
return this;
}
/**
*
* Additional information related to the event.
*
*
* @param message
* Additional information related to the event.
*/
public void setMessage(String message) {
this.message = message;
}
/**
*
* Additional information related to the event.
*
*
* @return Additional information related to the event.
*/
public String getMessage() {
return this.message;
}
/**
*
* Additional information related to the event.
*
*
* @param message
* Additional information related to the event.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Event withMessage(String message) {
setMessage(message);
return this;
}
/**
*
* Time stamp indicating when this event occurred. Format is a number expressed in Unix time as milliseconds (for
* example "1469498468.057"
).
*
*
* @param eventTime
* Time stamp indicating when this event occurred. Format is a number expressed in Unix time as milliseconds
* (for example "1469498468.057"
).
*/
public void setEventTime(java.util.Date eventTime) {
this.eventTime = eventTime;
}
/**
*
* Time stamp indicating when this event occurred. Format is a number expressed in Unix time as milliseconds (for
* example "1469498468.057"
).
*
*
* @return Time stamp indicating when this event occurred. Format is a number expressed in Unix time as milliseconds
* (for example "1469498468.057"
).
*/
public java.util.Date getEventTime() {
return this.eventTime;
}
/**
*
* Time stamp indicating when this event occurred. Format is a number expressed in Unix time as milliseconds (for
* example "1469498468.057"
).
*
*
* @param eventTime
* Time stamp indicating when this event occurred. Format is a number expressed in Unix time as milliseconds
* (for example "1469498468.057"
).
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Event withEventTime(java.util.Date eventTime) {
setEventTime(eventTime);
return this;
}
/**
*
* Location of stored logs with additional detail that is related to the event. This is useful for debugging issues.
* The URL is valid for 15 minutes. You can also access fleet creation logs through the GameLift console.
*
*
* @param preSignedLogUrl
* Location of stored logs with additional detail that is related to the event. This is useful for debugging
* issues. The URL is valid for 15 minutes. You can also access fleet creation logs through the GameLift
* console.
*/
public void setPreSignedLogUrl(String preSignedLogUrl) {
this.preSignedLogUrl = preSignedLogUrl;
}
/**
*
* Location of stored logs with additional detail that is related to the event. This is useful for debugging issues.
* The URL is valid for 15 minutes. You can also access fleet creation logs through the GameLift console.
*
*
* @return Location of stored logs with additional detail that is related to the event. This is useful for debugging
* issues. The URL is valid for 15 minutes. You can also access fleet creation logs through the GameLift
* console.
*/
public String getPreSignedLogUrl() {
return this.preSignedLogUrl;
}
/**
*
* Location of stored logs with additional detail that is related to the event. This is useful for debugging issues.
* The URL is valid for 15 minutes. You can also access fleet creation logs through the GameLift console.
*
*
* @param preSignedLogUrl
* Location of stored logs with additional detail that is related to the event. This is useful for debugging
* issues. The URL is valid for 15 minutes. You can also access fleet creation logs through the GameLift
* console.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Event withPreSignedLogUrl(String preSignedLogUrl) {
setPreSignedLogUrl(preSignedLogUrl);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getEventId() != null)
sb.append("EventId: ").append(getEventId()).append(",");
if (getResourceId() != null)
sb.append("ResourceId: ").append(getResourceId()).append(",");
if (getEventCode() != null)
sb.append("EventCode: ").append(getEventCode()).append(",");
if (getMessage() != null)
sb.append("Message: ").append(getMessage()).append(",");
if (getEventTime() != null)
sb.append("EventTime: ").append(getEventTime()).append(",");
if (getPreSignedLogUrl() != null)
sb.append("PreSignedLogUrl: ").append(getPreSignedLogUrl());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof Event == false)
return false;
Event other = (Event) obj;
if (other.getEventId() == null ^ this.getEventId() == null)
return false;
if (other.getEventId() != null && other.getEventId().equals(this.getEventId()) == false)
return false;
if (other.getResourceId() == null ^ this.getResourceId() == null)
return false;
if (other.getResourceId() != null && other.getResourceId().equals(this.getResourceId()) == false)
return false;
if (other.getEventCode() == null ^ this.getEventCode() == null)
return false;
if (other.getEventCode() != null && other.getEventCode().equals(this.getEventCode()) == false)
return false;
if (other.getMessage() == null ^ this.getMessage() == null)
return false;
if (other.getMessage() != null && other.getMessage().equals(this.getMessage()) == false)
return false;
if (other.getEventTime() == null ^ this.getEventTime() == null)
return false;
if (other.getEventTime() != null && other.getEventTime().equals(this.getEventTime()) == false)
return false;
if (other.getPreSignedLogUrl() == null ^ this.getPreSignedLogUrl() == null)
return false;
if (other.getPreSignedLogUrl() != null && other.getPreSignedLogUrl().equals(this.getPreSignedLogUrl()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getEventId() == null) ? 0 : getEventId().hashCode());
hashCode = prime * hashCode + ((getResourceId() == null) ? 0 : getResourceId().hashCode());
hashCode = prime * hashCode + ((getEventCode() == null) ? 0 : getEventCode().hashCode());
hashCode = prime * hashCode + ((getMessage() == null) ? 0 : getMessage().hashCode());
hashCode = prime * hashCode + ((getEventTime() == null) ? 0 : getEventTime().hashCode());
hashCode = prime * hashCode + ((getPreSignedLogUrl() == null) ? 0 : getPreSignedLogUrl().hashCode());
return hashCode;
}
@Override
public Event clone() {
try {
return (Event) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.gamelift.model.transform.EventMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}