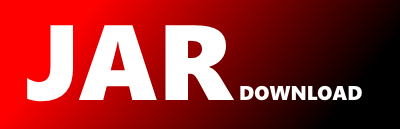
com.amazonaws.services.gamelift.model.GameServerGroup Maven / Gradle / Ivy
Show all versions of aws-java-sdk-gamelift Show documentation
/*
* Copyright 2017-2022 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.gamelift.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* This data type is used with the GameLift FleetIQ and game server groups.
*
*
* Properties that describe a game server group resource. A game server group manages certain properties related to a
* corresponding Amazon EC2 Auto Scaling group.
*
*
* A game server group is created by a successful call to CreateGameServerGroup
and deleted by calling
* DeleteGameServerGroup
. Game server group activity can be temporarily suspended and resumed by calling
* SuspendGameServerGroup
and ResumeGameServerGroup
, respectively.
*
*
* Related actions
*
*
* CreateGameServerGroup | ListGameServerGroups | DescribeGameServerGroup |
* UpdateGameServerGroup | DeleteGameServerGroup | ResumeGameServerGroup |
* SuspendGameServerGroup | DescribeGameServerInstances | All APIs by task
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class GameServerGroup implements Serializable, Cloneable, StructuredPojo {
/**
*
* A developer-defined identifier for the game server group. The name is unique for each Region in each Amazon Web
* Services account.
*
*/
private String gameServerGroupName;
/**
*
* A generated unique ID for the game server group.
*
*/
private String gameServerGroupArn;
/**
*
* The Amazon Resource Name (ARN)
* for an IAM role that allows Amazon Web Services to access your Amazon EC2 Auto Scaling groups.
*
*/
private String roleArn;
/**
*
* The set of Amazon EC2 instance types that GameLift FleetIQ can use when balancing and automatically scaling
* instances in the corresponding Auto Scaling group.
*
*/
private java.util.List instanceDefinitions;
/**
*
* Indicates how GameLift FleetIQ balances the use of Spot Instances and On-Demand Instances in the game server
* group. Method options include the following:
*
*
* -
*
* SPOT_ONLY
- Only Spot Instances are used in the game server group. If Spot Instances are unavailable
* or not viable for game hosting, the game server group provides no hosting capacity until Spot Instances can again
* be used. Until then, no new instances are started, and the existing nonviable Spot Instances are terminated
* (after current gameplay ends) and are not replaced.
*
*
* -
*
* SPOT_PREFERRED
- (default value) Spot Instances are used whenever available in the game server
* group. If Spot Instances are unavailable, the game server group continues to provide hosting capacity by falling
* back to On-Demand Instances. Existing nonviable Spot Instances are terminated (after current gameplay ends) and
* are replaced with new On-Demand Instances.
*
*
* -
*
* ON_DEMAND_ONLY
- Only On-Demand Instances are used in the game server group. No Spot Instances are
* used, even when available, while this balancing strategy is in force.
*
*
*
*/
private String balancingStrategy;
/**
*
* A flag that indicates whether instances in the game server group are protected from early termination.
* Unprotected instances that have active game servers running might be terminated during a scale-down event,
* causing players to be dropped from the game. Protected instances cannot be terminated while there are active game
* servers running except in the event of a forced game server group deletion (see ). An exception to this is with
* Spot Instances, which can be terminated by Amazon Web Services regardless of protection status.
*
*/
private String gameServerProtectionPolicy;
/**
*
* A generated unique ID for the Amazon EC2 Auto Scaling group that is associated with this game server group.
*
*/
private String autoScalingGroupArn;
/**
*
* The current status of the game server group. Possible statuses include:
*
*
* -
*
* NEW
- GameLift FleetIQ has validated the CreateGameServerGroup()
request.
*
*
* -
*
* ACTIVATING
- GameLift FleetIQ is setting up a game server group, which includes creating an Auto
* Scaling group in your Amazon Web Services account.
*
*
* -
*
* ACTIVE
- The game server group has been successfully created.
*
*
* -
*
* DELETE_SCHEDULED
- A request to delete the game server group has been received.
*
*
* -
*
* DELETING
- GameLift FleetIQ has received a valid DeleteGameServerGroup()
request and is
* processing it. GameLift FleetIQ must first complete and release hosts before it deletes the Auto Scaling group
* and the game server group.
*
*
* -
*
* DELETED
- The game server group has been successfully deleted.
*
*
* -
*
* ERROR
- The asynchronous processes of activating or deleting a game server group has failed,
* resulting in an error state.
*
*
*
*/
private String status;
/**
*
* Additional information about the current game server group status. This information might provide additional
* insight on groups that are in ERROR
status.
*
*/
private String statusReason;
/**
*
* A list of activities that are currently suspended for this game server group. If this property is empty, all
* activities are occurring.
*
*/
private java.util.List suspendedActions;
/**
*
* A time stamp indicating when this data object was created. Format is a number expressed in Unix time as
* milliseconds (for example "1469498468.057"
).
*
*/
private java.util.Date creationTime;
/**
*
* A timestamp that indicates when this game server group was last updated.
*
*/
private java.util.Date lastUpdatedTime;
/**
*
* A developer-defined identifier for the game server group. The name is unique for each Region in each Amazon Web
* Services account.
*
*
* @param gameServerGroupName
* A developer-defined identifier for the game server group. The name is unique for each Region in each
* Amazon Web Services account.
*/
public void setGameServerGroupName(String gameServerGroupName) {
this.gameServerGroupName = gameServerGroupName;
}
/**
*
* A developer-defined identifier for the game server group. The name is unique for each Region in each Amazon Web
* Services account.
*
*
* @return A developer-defined identifier for the game server group. The name is unique for each Region in each
* Amazon Web Services account.
*/
public String getGameServerGroupName() {
return this.gameServerGroupName;
}
/**
*
* A developer-defined identifier for the game server group. The name is unique for each Region in each Amazon Web
* Services account.
*
*
* @param gameServerGroupName
* A developer-defined identifier for the game server group. The name is unique for each Region in each
* Amazon Web Services account.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GameServerGroup withGameServerGroupName(String gameServerGroupName) {
setGameServerGroupName(gameServerGroupName);
return this;
}
/**
*
* A generated unique ID for the game server group.
*
*
* @param gameServerGroupArn
* A generated unique ID for the game server group.
*/
public void setGameServerGroupArn(String gameServerGroupArn) {
this.gameServerGroupArn = gameServerGroupArn;
}
/**
*
* A generated unique ID for the game server group.
*
*
* @return A generated unique ID for the game server group.
*/
public String getGameServerGroupArn() {
return this.gameServerGroupArn;
}
/**
*
* A generated unique ID for the game server group.
*
*
* @param gameServerGroupArn
* A generated unique ID for the game server group.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GameServerGroup withGameServerGroupArn(String gameServerGroupArn) {
setGameServerGroupArn(gameServerGroupArn);
return this;
}
/**
*
* The Amazon Resource Name (ARN)
* for an IAM role that allows Amazon Web Services to access your Amazon EC2 Auto Scaling groups.
*
*
* @param roleArn
* The Amazon Resource Name (ARN) for an IAM role that
* allows Amazon Web Services to access your Amazon EC2 Auto Scaling groups.
*/
public void setRoleArn(String roleArn) {
this.roleArn = roleArn;
}
/**
*
* The Amazon Resource Name (ARN)
* for an IAM role that allows Amazon Web Services to access your Amazon EC2 Auto Scaling groups.
*
*
* @return The Amazon Resource Name (ARN) for an IAM role that
* allows Amazon Web Services to access your Amazon EC2 Auto Scaling groups.
*/
public String getRoleArn() {
return this.roleArn;
}
/**
*
* The Amazon Resource Name (ARN)
* for an IAM role that allows Amazon Web Services to access your Amazon EC2 Auto Scaling groups.
*
*
* @param roleArn
* The Amazon Resource Name (ARN) for an IAM role that
* allows Amazon Web Services to access your Amazon EC2 Auto Scaling groups.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GameServerGroup withRoleArn(String roleArn) {
setRoleArn(roleArn);
return this;
}
/**
*
* The set of Amazon EC2 instance types that GameLift FleetIQ can use when balancing and automatically scaling
* instances in the corresponding Auto Scaling group.
*
*
* @return The set of Amazon EC2 instance types that GameLift FleetIQ can use when balancing and automatically
* scaling instances in the corresponding Auto Scaling group.
*/
public java.util.List getInstanceDefinitions() {
return instanceDefinitions;
}
/**
*
* The set of Amazon EC2 instance types that GameLift FleetIQ can use when balancing and automatically scaling
* instances in the corresponding Auto Scaling group.
*
*
* @param instanceDefinitions
* The set of Amazon EC2 instance types that GameLift FleetIQ can use when balancing and automatically
* scaling instances in the corresponding Auto Scaling group.
*/
public void setInstanceDefinitions(java.util.Collection instanceDefinitions) {
if (instanceDefinitions == null) {
this.instanceDefinitions = null;
return;
}
this.instanceDefinitions = new java.util.ArrayList(instanceDefinitions);
}
/**
*
* The set of Amazon EC2 instance types that GameLift FleetIQ can use when balancing and automatically scaling
* instances in the corresponding Auto Scaling group.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setInstanceDefinitions(java.util.Collection)} or {@link #withInstanceDefinitions(java.util.Collection)}
* if you want to override the existing values.
*
*
* @param instanceDefinitions
* The set of Amazon EC2 instance types that GameLift FleetIQ can use when balancing and automatically
* scaling instances in the corresponding Auto Scaling group.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GameServerGroup withInstanceDefinitions(InstanceDefinition... instanceDefinitions) {
if (this.instanceDefinitions == null) {
setInstanceDefinitions(new java.util.ArrayList(instanceDefinitions.length));
}
for (InstanceDefinition ele : instanceDefinitions) {
this.instanceDefinitions.add(ele);
}
return this;
}
/**
*
* The set of Amazon EC2 instance types that GameLift FleetIQ can use when balancing and automatically scaling
* instances in the corresponding Auto Scaling group.
*
*
* @param instanceDefinitions
* The set of Amazon EC2 instance types that GameLift FleetIQ can use when balancing and automatically
* scaling instances in the corresponding Auto Scaling group.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GameServerGroup withInstanceDefinitions(java.util.Collection instanceDefinitions) {
setInstanceDefinitions(instanceDefinitions);
return this;
}
/**
*
* Indicates how GameLift FleetIQ balances the use of Spot Instances and On-Demand Instances in the game server
* group. Method options include the following:
*
*
* -
*
* SPOT_ONLY
- Only Spot Instances are used in the game server group. If Spot Instances are unavailable
* or not viable for game hosting, the game server group provides no hosting capacity until Spot Instances can again
* be used. Until then, no new instances are started, and the existing nonviable Spot Instances are terminated
* (after current gameplay ends) and are not replaced.
*
*
* -
*
* SPOT_PREFERRED
- (default value) Spot Instances are used whenever available in the game server
* group. If Spot Instances are unavailable, the game server group continues to provide hosting capacity by falling
* back to On-Demand Instances. Existing nonviable Spot Instances are terminated (after current gameplay ends) and
* are replaced with new On-Demand Instances.
*
*
* -
*
* ON_DEMAND_ONLY
- Only On-Demand Instances are used in the game server group. No Spot Instances are
* used, even when available, while this balancing strategy is in force.
*
*
*
*
* @param balancingStrategy
* Indicates how GameLift FleetIQ balances the use of Spot Instances and On-Demand Instances in the game
* server group. Method options include the following:
*
* -
*
* SPOT_ONLY
- Only Spot Instances are used in the game server group. If Spot Instances are
* unavailable or not viable for game hosting, the game server group provides no hosting capacity until Spot
* Instances can again be used. Until then, no new instances are started, and the existing nonviable Spot
* Instances are terminated (after current gameplay ends) and are not replaced.
*
*
* -
*
* SPOT_PREFERRED
- (default value) Spot Instances are used whenever available in the game
* server group. If Spot Instances are unavailable, the game server group continues to provide hosting
* capacity by falling back to On-Demand Instances. Existing nonviable Spot Instances are terminated (after
* current gameplay ends) and are replaced with new On-Demand Instances.
*
*
* -
*
* ON_DEMAND_ONLY
- Only On-Demand Instances are used in the game server group. No Spot
* Instances are used, even when available, while this balancing strategy is in force.
*
*
* @see BalancingStrategy
*/
public void setBalancingStrategy(String balancingStrategy) {
this.balancingStrategy = balancingStrategy;
}
/**
*
* Indicates how GameLift FleetIQ balances the use of Spot Instances and On-Demand Instances in the game server
* group. Method options include the following:
*
*
* -
*
* SPOT_ONLY
- Only Spot Instances are used in the game server group. If Spot Instances are unavailable
* or not viable for game hosting, the game server group provides no hosting capacity until Spot Instances can again
* be used. Until then, no new instances are started, and the existing nonviable Spot Instances are terminated
* (after current gameplay ends) and are not replaced.
*
*
* -
*
* SPOT_PREFERRED
- (default value) Spot Instances are used whenever available in the game server
* group. If Spot Instances are unavailable, the game server group continues to provide hosting capacity by falling
* back to On-Demand Instances. Existing nonviable Spot Instances are terminated (after current gameplay ends) and
* are replaced with new On-Demand Instances.
*
*
* -
*
* ON_DEMAND_ONLY
- Only On-Demand Instances are used in the game server group. No Spot Instances are
* used, even when available, while this balancing strategy is in force.
*
*
*
*
* @return Indicates how GameLift FleetIQ balances the use of Spot Instances and On-Demand Instances in the game
* server group. Method options include the following:
*
* -
*
* SPOT_ONLY
- Only Spot Instances are used in the game server group. If Spot Instances are
* unavailable or not viable for game hosting, the game server group provides no hosting capacity until Spot
* Instances can again be used. Until then, no new instances are started, and the existing nonviable Spot
* Instances are terminated (after current gameplay ends) and are not replaced.
*
*
* -
*
* SPOT_PREFERRED
- (default value) Spot Instances are used whenever available in the game
* server group. If Spot Instances are unavailable, the game server group continues to provide hosting
* capacity by falling back to On-Demand Instances. Existing nonviable Spot Instances are terminated (after
* current gameplay ends) and are replaced with new On-Demand Instances.
*
*
* -
*
* ON_DEMAND_ONLY
- Only On-Demand Instances are used in the game server group. No Spot
* Instances are used, even when available, while this balancing strategy is in force.
*
*
* @see BalancingStrategy
*/
public String getBalancingStrategy() {
return this.balancingStrategy;
}
/**
*
* Indicates how GameLift FleetIQ balances the use of Spot Instances and On-Demand Instances in the game server
* group. Method options include the following:
*
*
* -
*
* SPOT_ONLY
- Only Spot Instances are used in the game server group. If Spot Instances are unavailable
* or not viable for game hosting, the game server group provides no hosting capacity until Spot Instances can again
* be used. Until then, no new instances are started, and the existing nonviable Spot Instances are terminated
* (after current gameplay ends) and are not replaced.
*
*
* -
*
* SPOT_PREFERRED
- (default value) Spot Instances are used whenever available in the game server
* group. If Spot Instances are unavailable, the game server group continues to provide hosting capacity by falling
* back to On-Demand Instances. Existing nonviable Spot Instances are terminated (after current gameplay ends) and
* are replaced with new On-Demand Instances.
*
*
* -
*
* ON_DEMAND_ONLY
- Only On-Demand Instances are used in the game server group. No Spot Instances are
* used, even when available, while this balancing strategy is in force.
*
*
*
*
* @param balancingStrategy
* Indicates how GameLift FleetIQ balances the use of Spot Instances and On-Demand Instances in the game
* server group. Method options include the following:
*
* -
*
* SPOT_ONLY
- Only Spot Instances are used in the game server group. If Spot Instances are
* unavailable or not viable for game hosting, the game server group provides no hosting capacity until Spot
* Instances can again be used. Until then, no new instances are started, and the existing nonviable Spot
* Instances are terminated (after current gameplay ends) and are not replaced.
*
*
* -
*
* SPOT_PREFERRED
- (default value) Spot Instances are used whenever available in the game
* server group. If Spot Instances are unavailable, the game server group continues to provide hosting
* capacity by falling back to On-Demand Instances. Existing nonviable Spot Instances are terminated (after
* current gameplay ends) and are replaced with new On-Demand Instances.
*
*
* -
*
* ON_DEMAND_ONLY
- Only On-Demand Instances are used in the game server group. No Spot
* Instances are used, even when available, while this balancing strategy is in force.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see BalancingStrategy
*/
public GameServerGroup withBalancingStrategy(String balancingStrategy) {
setBalancingStrategy(balancingStrategy);
return this;
}
/**
*
* Indicates how GameLift FleetIQ balances the use of Spot Instances and On-Demand Instances in the game server
* group. Method options include the following:
*
*
* -
*
* SPOT_ONLY
- Only Spot Instances are used in the game server group. If Spot Instances are unavailable
* or not viable for game hosting, the game server group provides no hosting capacity until Spot Instances can again
* be used. Until then, no new instances are started, and the existing nonviable Spot Instances are terminated
* (after current gameplay ends) and are not replaced.
*
*
* -
*
* SPOT_PREFERRED
- (default value) Spot Instances are used whenever available in the game server
* group. If Spot Instances are unavailable, the game server group continues to provide hosting capacity by falling
* back to On-Demand Instances. Existing nonviable Spot Instances are terminated (after current gameplay ends) and
* are replaced with new On-Demand Instances.
*
*
* -
*
* ON_DEMAND_ONLY
- Only On-Demand Instances are used in the game server group. No Spot Instances are
* used, even when available, while this balancing strategy is in force.
*
*
*
*
* @param balancingStrategy
* Indicates how GameLift FleetIQ balances the use of Spot Instances and On-Demand Instances in the game
* server group. Method options include the following:
*
* -
*
* SPOT_ONLY
- Only Spot Instances are used in the game server group. If Spot Instances are
* unavailable or not viable for game hosting, the game server group provides no hosting capacity until Spot
* Instances can again be used. Until then, no new instances are started, and the existing nonviable Spot
* Instances are terminated (after current gameplay ends) and are not replaced.
*
*
* -
*
* SPOT_PREFERRED
- (default value) Spot Instances are used whenever available in the game
* server group. If Spot Instances are unavailable, the game server group continues to provide hosting
* capacity by falling back to On-Demand Instances. Existing nonviable Spot Instances are terminated (after
* current gameplay ends) and are replaced with new On-Demand Instances.
*
*
* -
*
* ON_DEMAND_ONLY
- Only On-Demand Instances are used in the game server group. No Spot
* Instances are used, even when available, while this balancing strategy is in force.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see BalancingStrategy
*/
public GameServerGroup withBalancingStrategy(BalancingStrategy balancingStrategy) {
this.balancingStrategy = balancingStrategy.toString();
return this;
}
/**
*
* A flag that indicates whether instances in the game server group are protected from early termination.
* Unprotected instances that have active game servers running might be terminated during a scale-down event,
* causing players to be dropped from the game. Protected instances cannot be terminated while there are active game
* servers running except in the event of a forced game server group deletion (see ). An exception to this is with
* Spot Instances, which can be terminated by Amazon Web Services regardless of protection status.
*
*
* @param gameServerProtectionPolicy
* A flag that indicates whether instances in the game server group are protected from early termination.
* Unprotected instances that have active game servers running might be terminated during a scale-down event,
* causing players to be dropped from the game. Protected instances cannot be terminated while there are
* active game servers running except in the event of a forced game server group deletion (see ). An
* exception to this is with Spot Instances, which can be terminated by Amazon Web Services regardless of
* protection status.
* @see GameServerProtectionPolicy
*/
public void setGameServerProtectionPolicy(String gameServerProtectionPolicy) {
this.gameServerProtectionPolicy = gameServerProtectionPolicy;
}
/**
*
* A flag that indicates whether instances in the game server group are protected from early termination.
* Unprotected instances that have active game servers running might be terminated during a scale-down event,
* causing players to be dropped from the game. Protected instances cannot be terminated while there are active game
* servers running except in the event of a forced game server group deletion (see ). An exception to this is with
* Spot Instances, which can be terminated by Amazon Web Services regardless of protection status.
*
*
* @return A flag that indicates whether instances in the game server group are protected from early termination.
* Unprotected instances that have active game servers running might be terminated during a scale-down
* event, causing players to be dropped from the game. Protected instances cannot be terminated while there
* are active game servers running except in the event of a forced game server group deletion (see ). An
* exception to this is with Spot Instances, which can be terminated by Amazon Web Services regardless of
* protection status.
* @see GameServerProtectionPolicy
*/
public String getGameServerProtectionPolicy() {
return this.gameServerProtectionPolicy;
}
/**
*
* A flag that indicates whether instances in the game server group are protected from early termination.
* Unprotected instances that have active game servers running might be terminated during a scale-down event,
* causing players to be dropped from the game. Protected instances cannot be terminated while there are active game
* servers running except in the event of a forced game server group deletion (see ). An exception to this is with
* Spot Instances, which can be terminated by Amazon Web Services regardless of protection status.
*
*
* @param gameServerProtectionPolicy
* A flag that indicates whether instances in the game server group are protected from early termination.
* Unprotected instances that have active game servers running might be terminated during a scale-down event,
* causing players to be dropped from the game. Protected instances cannot be terminated while there are
* active game servers running except in the event of a forced game server group deletion (see ). An
* exception to this is with Spot Instances, which can be terminated by Amazon Web Services regardless of
* protection status.
* @return Returns a reference to this object so that method calls can be chained together.
* @see GameServerProtectionPolicy
*/
public GameServerGroup withGameServerProtectionPolicy(String gameServerProtectionPolicy) {
setGameServerProtectionPolicy(gameServerProtectionPolicy);
return this;
}
/**
*
* A flag that indicates whether instances in the game server group are protected from early termination.
* Unprotected instances that have active game servers running might be terminated during a scale-down event,
* causing players to be dropped from the game. Protected instances cannot be terminated while there are active game
* servers running except in the event of a forced game server group deletion (see ). An exception to this is with
* Spot Instances, which can be terminated by Amazon Web Services regardless of protection status.
*
*
* @param gameServerProtectionPolicy
* A flag that indicates whether instances in the game server group are protected from early termination.
* Unprotected instances that have active game servers running might be terminated during a scale-down event,
* causing players to be dropped from the game. Protected instances cannot be terminated while there are
* active game servers running except in the event of a forced game server group deletion (see ). An
* exception to this is with Spot Instances, which can be terminated by Amazon Web Services regardless of
* protection status.
* @return Returns a reference to this object so that method calls can be chained together.
* @see GameServerProtectionPolicy
*/
public GameServerGroup withGameServerProtectionPolicy(GameServerProtectionPolicy gameServerProtectionPolicy) {
this.gameServerProtectionPolicy = gameServerProtectionPolicy.toString();
return this;
}
/**
*
* A generated unique ID for the Amazon EC2 Auto Scaling group that is associated with this game server group.
*
*
* @param autoScalingGroupArn
* A generated unique ID for the Amazon EC2 Auto Scaling group that is associated with this game server
* group.
*/
public void setAutoScalingGroupArn(String autoScalingGroupArn) {
this.autoScalingGroupArn = autoScalingGroupArn;
}
/**
*
* A generated unique ID for the Amazon EC2 Auto Scaling group that is associated with this game server group.
*
*
* @return A generated unique ID for the Amazon EC2 Auto Scaling group that is associated with this game server
* group.
*/
public String getAutoScalingGroupArn() {
return this.autoScalingGroupArn;
}
/**
*
* A generated unique ID for the Amazon EC2 Auto Scaling group that is associated with this game server group.
*
*
* @param autoScalingGroupArn
* A generated unique ID for the Amazon EC2 Auto Scaling group that is associated with this game server
* group.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GameServerGroup withAutoScalingGroupArn(String autoScalingGroupArn) {
setAutoScalingGroupArn(autoScalingGroupArn);
return this;
}
/**
*
* The current status of the game server group. Possible statuses include:
*
*
* -
*
* NEW
- GameLift FleetIQ has validated the CreateGameServerGroup()
request.
*
*
* -
*
* ACTIVATING
- GameLift FleetIQ is setting up a game server group, which includes creating an Auto
* Scaling group in your Amazon Web Services account.
*
*
* -
*
* ACTIVE
- The game server group has been successfully created.
*
*
* -
*
* DELETE_SCHEDULED
- A request to delete the game server group has been received.
*
*
* -
*
* DELETING
- GameLift FleetIQ has received a valid DeleteGameServerGroup()
request and is
* processing it. GameLift FleetIQ must first complete and release hosts before it deletes the Auto Scaling group
* and the game server group.
*
*
* -
*
* DELETED
- The game server group has been successfully deleted.
*
*
* -
*
* ERROR
- The asynchronous processes of activating or deleting a game server group has failed,
* resulting in an error state.
*
*
*
*
* @param status
* The current status of the game server group. Possible statuses include:
*
* -
*
* NEW
- GameLift FleetIQ has validated the CreateGameServerGroup()
request.
*
*
* -
*
* ACTIVATING
- GameLift FleetIQ is setting up a game server group, which includes creating an
* Auto Scaling group in your Amazon Web Services account.
*
*
* -
*
* ACTIVE
- The game server group has been successfully created.
*
*
* -
*
* DELETE_SCHEDULED
- A request to delete the game server group has been received.
*
*
* -
*
* DELETING
- GameLift FleetIQ has received a valid DeleteGameServerGroup()
request
* and is processing it. GameLift FleetIQ must first complete and release hosts before it deletes the Auto
* Scaling group and the game server group.
*
*
* -
*
* DELETED
- The game server group has been successfully deleted.
*
*
* -
*
* ERROR
- The asynchronous processes of activating or deleting a game server group has failed,
* resulting in an error state.
*
*
* @see GameServerGroupStatus
*/
public void setStatus(String status) {
this.status = status;
}
/**
*
* The current status of the game server group. Possible statuses include:
*
*
* -
*
* NEW
- GameLift FleetIQ has validated the CreateGameServerGroup()
request.
*
*
* -
*
* ACTIVATING
- GameLift FleetIQ is setting up a game server group, which includes creating an Auto
* Scaling group in your Amazon Web Services account.
*
*
* -
*
* ACTIVE
- The game server group has been successfully created.
*
*
* -
*
* DELETE_SCHEDULED
- A request to delete the game server group has been received.
*
*
* -
*
* DELETING
- GameLift FleetIQ has received a valid DeleteGameServerGroup()
request and is
* processing it. GameLift FleetIQ must first complete and release hosts before it deletes the Auto Scaling group
* and the game server group.
*
*
* -
*
* DELETED
- The game server group has been successfully deleted.
*
*
* -
*
* ERROR
- The asynchronous processes of activating or deleting a game server group has failed,
* resulting in an error state.
*
*
*
*
* @return The current status of the game server group. Possible statuses include:
*
* -
*
* NEW
- GameLift FleetIQ has validated the CreateGameServerGroup()
request.
*
*
* -
*
* ACTIVATING
- GameLift FleetIQ is setting up a game server group, which includes creating an
* Auto Scaling group in your Amazon Web Services account.
*
*
* -
*
* ACTIVE
- The game server group has been successfully created.
*
*
* -
*
* DELETE_SCHEDULED
- A request to delete the game server group has been received.
*
*
* -
*
* DELETING
- GameLift FleetIQ has received a valid DeleteGameServerGroup()
* request and is processing it. GameLift FleetIQ must first complete and release hosts before it deletes
* the Auto Scaling group and the game server group.
*
*
* -
*
* DELETED
- The game server group has been successfully deleted.
*
*
* -
*
* ERROR
- The asynchronous processes of activating or deleting a game server group has failed,
* resulting in an error state.
*
*
* @see GameServerGroupStatus
*/
public String getStatus() {
return this.status;
}
/**
*
* The current status of the game server group. Possible statuses include:
*
*
* -
*
* NEW
- GameLift FleetIQ has validated the CreateGameServerGroup()
request.
*
*
* -
*
* ACTIVATING
- GameLift FleetIQ is setting up a game server group, which includes creating an Auto
* Scaling group in your Amazon Web Services account.
*
*
* -
*
* ACTIVE
- The game server group has been successfully created.
*
*
* -
*
* DELETE_SCHEDULED
- A request to delete the game server group has been received.
*
*
* -
*
* DELETING
- GameLift FleetIQ has received a valid DeleteGameServerGroup()
request and is
* processing it. GameLift FleetIQ must first complete and release hosts before it deletes the Auto Scaling group
* and the game server group.
*
*
* -
*
* DELETED
- The game server group has been successfully deleted.
*
*
* -
*
* ERROR
- The asynchronous processes of activating or deleting a game server group has failed,
* resulting in an error state.
*
*
*
*
* @param status
* The current status of the game server group. Possible statuses include:
*
* -
*
* NEW
- GameLift FleetIQ has validated the CreateGameServerGroup()
request.
*
*
* -
*
* ACTIVATING
- GameLift FleetIQ is setting up a game server group, which includes creating an
* Auto Scaling group in your Amazon Web Services account.
*
*
* -
*
* ACTIVE
- The game server group has been successfully created.
*
*
* -
*
* DELETE_SCHEDULED
- A request to delete the game server group has been received.
*
*
* -
*
* DELETING
- GameLift FleetIQ has received a valid DeleteGameServerGroup()
request
* and is processing it. GameLift FleetIQ must first complete and release hosts before it deletes the Auto
* Scaling group and the game server group.
*
*
* -
*
* DELETED
- The game server group has been successfully deleted.
*
*
* -
*
* ERROR
- The asynchronous processes of activating or deleting a game server group has failed,
* resulting in an error state.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see GameServerGroupStatus
*/
public GameServerGroup withStatus(String status) {
setStatus(status);
return this;
}
/**
*
* The current status of the game server group. Possible statuses include:
*
*
* -
*
* NEW
- GameLift FleetIQ has validated the CreateGameServerGroup()
request.
*
*
* -
*
* ACTIVATING
- GameLift FleetIQ is setting up a game server group, which includes creating an Auto
* Scaling group in your Amazon Web Services account.
*
*
* -
*
* ACTIVE
- The game server group has been successfully created.
*
*
* -
*
* DELETE_SCHEDULED
- A request to delete the game server group has been received.
*
*
* -
*
* DELETING
- GameLift FleetIQ has received a valid DeleteGameServerGroup()
request and is
* processing it. GameLift FleetIQ must first complete and release hosts before it deletes the Auto Scaling group
* and the game server group.
*
*
* -
*
* DELETED
- The game server group has been successfully deleted.
*
*
* -
*
* ERROR
- The asynchronous processes of activating or deleting a game server group has failed,
* resulting in an error state.
*
*
*
*
* @param status
* The current status of the game server group. Possible statuses include:
*
* -
*
* NEW
- GameLift FleetIQ has validated the CreateGameServerGroup()
request.
*
*
* -
*
* ACTIVATING
- GameLift FleetIQ is setting up a game server group, which includes creating an
* Auto Scaling group in your Amazon Web Services account.
*
*
* -
*
* ACTIVE
- The game server group has been successfully created.
*
*
* -
*
* DELETE_SCHEDULED
- A request to delete the game server group has been received.
*
*
* -
*
* DELETING
- GameLift FleetIQ has received a valid DeleteGameServerGroup()
request
* and is processing it. GameLift FleetIQ must first complete and release hosts before it deletes the Auto
* Scaling group and the game server group.
*
*
* -
*
* DELETED
- The game server group has been successfully deleted.
*
*
* -
*
* ERROR
- The asynchronous processes of activating or deleting a game server group has failed,
* resulting in an error state.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see GameServerGroupStatus
*/
public GameServerGroup withStatus(GameServerGroupStatus status) {
this.status = status.toString();
return this;
}
/**
*
* Additional information about the current game server group status. This information might provide additional
* insight on groups that are in ERROR
status.
*
*
* @param statusReason
* Additional information about the current game server group status. This information might provide
* additional insight on groups that are in ERROR
status.
*/
public void setStatusReason(String statusReason) {
this.statusReason = statusReason;
}
/**
*
* Additional information about the current game server group status. This information might provide additional
* insight on groups that are in ERROR
status.
*
*
* @return Additional information about the current game server group status. This information might provide
* additional insight on groups that are in ERROR
status.
*/
public String getStatusReason() {
return this.statusReason;
}
/**
*
* Additional information about the current game server group status. This information might provide additional
* insight on groups that are in ERROR
status.
*
*
* @param statusReason
* Additional information about the current game server group status. This information might provide
* additional insight on groups that are in ERROR
status.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GameServerGroup withStatusReason(String statusReason) {
setStatusReason(statusReason);
return this;
}
/**
*
* A list of activities that are currently suspended for this game server group. If this property is empty, all
* activities are occurring.
*
*
* @return A list of activities that are currently suspended for this game server group. If this property is empty,
* all activities are occurring.
* @see GameServerGroupAction
*/
public java.util.List getSuspendedActions() {
return suspendedActions;
}
/**
*
* A list of activities that are currently suspended for this game server group. If this property is empty, all
* activities are occurring.
*
*
* @param suspendedActions
* A list of activities that are currently suspended for this game server group. If this property is empty,
* all activities are occurring.
* @see GameServerGroupAction
*/
public void setSuspendedActions(java.util.Collection suspendedActions) {
if (suspendedActions == null) {
this.suspendedActions = null;
return;
}
this.suspendedActions = new java.util.ArrayList(suspendedActions);
}
/**
*
* A list of activities that are currently suspended for this game server group. If this property is empty, all
* activities are occurring.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setSuspendedActions(java.util.Collection)} or {@link #withSuspendedActions(java.util.Collection)} if you
* want to override the existing values.
*
*
* @param suspendedActions
* A list of activities that are currently suspended for this game server group. If this property is empty,
* all activities are occurring.
* @return Returns a reference to this object so that method calls can be chained together.
* @see GameServerGroupAction
*/
public GameServerGroup withSuspendedActions(String... suspendedActions) {
if (this.suspendedActions == null) {
setSuspendedActions(new java.util.ArrayList(suspendedActions.length));
}
for (String ele : suspendedActions) {
this.suspendedActions.add(ele);
}
return this;
}
/**
*
* A list of activities that are currently suspended for this game server group. If this property is empty, all
* activities are occurring.
*
*
* @param suspendedActions
* A list of activities that are currently suspended for this game server group. If this property is empty,
* all activities are occurring.
* @return Returns a reference to this object so that method calls can be chained together.
* @see GameServerGroupAction
*/
public GameServerGroup withSuspendedActions(java.util.Collection suspendedActions) {
setSuspendedActions(suspendedActions);
return this;
}
/**
*
* A list of activities that are currently suspended for this game server group. If this property is empty, all
* activities are occurring.
*
*
* @param suspendedActions
* A list of activities that are currently suspended for this game server group. If this property is empty,
* all activities are occurring.
* @return Returns a reference to this object so that method calls can be chained together.
* @see GameServerGroupAction
*/
public GameServerGroup withSuspendedActions(GameServerGroupAction... suspendedActions) {
java.util.ArrayList suspendedActionsCopy = new java.util.ArrayList(suspendedActions.length);
for (GameServerGroupAction value : suspendedActions) {
suspendedActionsCopy.add(value.toString());
}
if (getSuspendedActions() == null) {
setSuspendedActions(suspendedActionsCopy);
} else {
getSuspendedActions().addAll(suspendedActionsCopy);
}
return this;
}
/**
*
* A time stamp indicating when this data object was created. Format is a number expressed in Unix time as
* milliseconds (for example "1469498468.057"
).
*
*
* @param creationTime
* A time stamp indicating when this data object was created. Format is a number expressed in Unix time as
* milliseconds (for example "1469498468.057"
).
*/
public void setCreationTime(java.util.Date creationTime) {
this.creationTime = creationTime;
}
/**
*
* A time stamp indicating when this data object was created. Format is a number expressed in Unix time as
* milliseconds (for example "1469498468.057"
).
*
*
* @return A time stamp indicating when this data object was created. Format is a number expressed in Unix time as
* milliseconds (for example "1469498468.057"
).
*/
public java.util.Date getCreationTime() {
return this.creationTime;
}
/**
*
* A time stamp indicating when this data object was created. Format is a number expressed in Unix time as
* milliseconds (for example "1469498468.057"
).
*
*
* @param creationTime
* A time stamp indicating when this data object was created. Format is a number expressed in Unix time as
* milliseconds (for example "1469498468.057"
).
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GameServerGroup withCreationTime(java.util.Date creationTime) {
setCreationTime(creationTime);
return this;
}
/**
*
* A timestamp that indicates when this game server group was last updated.
*
*
* @param lastUpdatedTime
* A timestamp that indicates when this game server group was last updated.
*/
public void setLastUpdatedTime(java.util.Date lastUpdatedTime) {
this.lastUpdatedTime = lastUpdatedTime;
}
/**
*
* A timestamp that indicates when this game server group was last updated.
*
*
* @return A timestamp that indicates when this game server group was last updated.
*/
public java.util.Date getLastUpdatedTime() {
return this.lastUpdatedTime;
}
/**
*
* A timestamp that indicates when this game server group was last updated.
*
*
* @param lastUpdatedTime
* A timestamp that indicates when this game server group was last updated.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GameServerGroup withLastUpdatedTime(java.util.Date lastUpdatedTime) {
setLastUpdatedTime(lastUpdatedTime);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getGameServerGroupName() != null)
sb.append("GameServerGroupName: ").append(getGameServerGroupName()).append(",");
if (getGameServerGroupArn() != null)
sb.append("GameServerGroupArn: ").append(getGameServerGroupArn()).append(",");
if (getRoleArn() != null)
sb.append("RoleArn: ").append(getRoleArn()).append(",");
if (getInstanceDefinitions() != null)
sb.append("InstanceDefinitions: ").append(getInstanceDefinitions()).append(",");
if (getBalancingStrategy() != null)
sb.append("BalancingStrategy: ").append(getBalancingStrategy()).append(",");
if (getGameServerProtectionPolicy() != null)
sb.append("GameServerProtectionPolicy: ").append(getGameServerProtectionPolicy()).append(",");
if (getAutoScalingGroupArn() != null)
sb.append("AutoScalingGroupArn: ").append(getAutoScalingGroupArn()).append(",");
if (getStatus() != null)
sb.append("Status: ").append(getStatus()).append(",");
if (getStatusReason() != null)
sb.append("StatusReason: ").append(getStatusReason()).append(",");
if (getSuspendedActions() != null)
sb.append("SuspendedActions: ").append(getSuspendedActions()).append(",");
if (getCreationTime() != null)
sb.append("CreationTime: ").append(getCreationTime()).append(",");
if (getLastUpdatedTime() != null)
sb.append("LastUpdatedTime: ").append(getLastUpdatedTime());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof GameServerGroup == false)
return false;
GameServerGroup other = (GameServerGroup) obj;
if (other.getGameServerGroupName() == null ^ this.getGameServerGroupName() == null)
return false;
if (other.getGameServerGroupName() != null && other.getGameServerGroupName().equals(this.getGameServerGroupName()) == false)
return false;
if (other.getGameServerGroupArn() == null ^ this.getGameServerGroupArn() == null)
return false;
if (other.getGameServerGroupArn() != null && other.getGameServerGroupArn().equals(this.getGameServerGroupArn()) == false)
return false;
if (other.getRoleArn() == null ^ this.getRoleArn() == null)
return false;
if (other.getRoleArn() != null && other.getRoleArn().equals(this.getRoleArn()) == false)
return false;
if (other.getInstanceDefinitions() == null ^ this.getInstanceDefinitions() == null)
return false;
if (other.getInstanceDefinitions() != null && other.getInstanceDefinitions().equals(this.getInstanceDefinitions()) == false)
return false;
if (other.getBalancingStrategy() == null ^ this.getBalancingStrategy() == null)
return false;
if (other.getBalancingStrategy() != null && other.getBalancingStrategy().equals(this.getBalancingStrategy()) == false)
return false;
if (other.getGameServerProtectionPolicy() == null ^ this.getGameServerProtectionPolicy() == null)
return false;
if (other.getGameServerProtectionPolicy() != null && other.getGameServerProtectionPolicy().equals(this.getGameServerProtectionPolicy()) == false)
return false;
if (other.getAutoScalingGroupArn() == null ^ this.getAutoScalingGroupArn() == null)
return false;
if (other.getAutoScalingGroupArn() != null && other.getAutoScalingGroupArn().equals(this.getAutoScalingGroupArn()) == false)
return false;
if (other.getStatus() == null ^ this.getStatus() == null)
return false;
if (other.getStatus() != null && other.getStatus().equals(this.getStatus()) == false)
return false;
if (other.getStatusReason() == null ^ this.getStatusReason() == null)
return false;
if (other.getStatusReason() != null && other.getStatusReason().equals(this.getStatusReason()) == false)
return false;
if (other.getSuspendedActions() == null ^ this.getSuspendedActions() == null)
return false;
if (other.getSuspendedActions() != null && other.getSuspendedActions().equals(this.getSuspendedActions()) == false)
return false;
if (other.getCreationTime() == null ^ this.getCreationTime() == null)
return false;
if (other.getCreationTime() != null && other.getCreationTime().equals(this.getCreationTime()) == false)
return false;
if (other.getLastUpdatedTime() == null ^ this.getLastUpdatedTime() == null)
return false;
if (other.getLastUpdatedTime() != null && other.getLastUpdatedTime().equals(this.getLastUpdatedTime()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getGameServerGroupName() == null) ? 0 : getGameServerGroupName().hashCode());
hashCode = prime * hashCode + ((getGameServerGroupArn() == null) ? 0 : getGameServerGroupArn().hashCode());
hashCode = prime * hashCode + ((getRoleArn() == null) ? 0 : getRoleArn().hashCode());
hashCode = prime * hashCode + ((getInstanceDefinitions() == null) ? 0 : getInstanceDefinitions().hashCode());
hashCode = prime * hashCode + ((getBalancingStrategy() == null) ? 0 : getBalancingStrategy().hashCode());
hashCode = prime * hashCode + ((getGameServerProtectionPolicy() == null) ? 0 : getGameServerProtectionPolicy().hashCode());
hashCode = prime * hashCode + ((getAutoScalingGroupArn() == null) ? 0 : getAutoScalingGroupArn().hashCode());
hashCode = prime * hashCode + ((getStatus() == null) ? 0 : getStatus().hashCode());
hashCode = prime * hashCode + ((getStatusReason() == null) ? 0 : getStatusReason().hashCode());
hashCode = prime * hashCode + ((getSuspendedActions() == null) ? 0 : getSuspendedActions().hashCode());
hashCode = prime * hashCode + ((getCreationTime() == null) ? 0 : getCreationTime().hashCode());
hashCode = prime * hashCode + ((getLastUpdatedTime() == null) ? 0 : getLastUpdatedTime().hashCode());
return hashCode;
}
@Override
public GameServerGroup clone() {
try {
return (GameServerGroup) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.gamelift.model.transform.GameServerGroupMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}