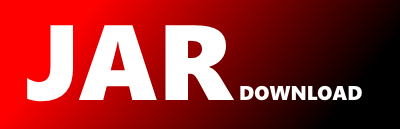
com.amazonaws.services.gamelift.model.MatchmakingConfiguration Maven / Gradle / Ivy
Show all versions of aws-java-sdk-gamelift Show documentation
/*
* Copyright 2017-2022 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.gamelift.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* Guidelines for use with FlexMatch to match players into games. All matchmaking requests must specify a matchmaking
* configuration.
*
*
* @see AWS
* API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class MatchmakingConfiguration implements Serializable, Cloneable, StructuredPojo {
/**
*
* A unique identifier for the matchmaking configuration. This name is used to identify the configuration associated
* with a matchmaking request or ticket.
*
*/
private String name;
/**
*
* The Amazon Resource Name (ARN)
* that is assigned to a GameLift matchmaking configuration resource and uniquely identifies it. ARNs are unique
* across all Regions. Format is
* arn:aws:gamelift:<region>::matchmakingconfiguration/<matchmaking configuration name>
. In
* a GameLift configuration ARN, the resource ID matches the Name value.
*
*/
private String configurationArn;
/**
*
* A descriptive label that is associated with matchmaking configuration.
*
*/
private String description;
/**
*
* The Amazon Resource Name (ARN)
* that is assigned to a GameLift game session queue resource and uniquely identifies it. ARNs are unique across all
* Regions. Format is arn:aws:gamelift:<region>::gamesessionqueue/<queue name>
. Queues can
* be located in any Region. Queues are used to start new GameLift-hosted game sessions for matches that are created
* with this matchmaking configuration. This property is not set when FlexMatchMode
is set to
* STANDALONE
.
*
*/
private java.util.List gameSessionQueueArns;
/**
*
* The maximum duration, in seconds, that a matchmaking ticket can remain in process before timing out. Requests
* that fail due to timing out can be resubmitted as needed.
*
*/
private Integer requestTimeoutSeconds;
/**
*
* The length of time (in seconds) to wait for players to accept a proposed match, if acceptance is required. If any
* player rejects the match or fails to accept before the timeout, the ticket continues to look for an acceptable
* match.
*
*/
private Integer acceptanceTimeoutSeconds;
/**
*
* A flag that indicates whether a match that was created with this configuration must be accepted by the matched
* players. To require acceptance, set to TRUE. When this option is enabled, matchmaking tickets use the status
* REQUIRES_ACCEPTANCE
to indicate when a completed potential match is waiting for player acceptance.
*
*/
private Boolean acceptanceRequired;
/**
*
* A unique identifier for the matchmaking rule set to use with this configuration. A matchmaking configuration can
* only use rule sets that are defined in the same Region.
*
*/
private String ruleSetName;
/**
*
* The Amazon Resource Name (ARN)
* associated with the GameLift matchmaking rule set resource that this configuration uses.
*
*/
private String ruleSetArn;
/**
*
* An SNS topic ARN that is set up to receive matchmaking notifications.
*
*/
private String notificationTarget;
/**
*
* The number of player slots in a match to keep open for future players. For example, if the configuration's rule
* set specifies a match for a single 12-person team, and the additional player count is set to 2, only 10 players
* are selected for the match. This parameter is not used when FlexMatchMode
is set to
* STANDALONE
.
*
*/
private Integer additionalPlayerCount;
/**
*
* Information to attach to all events related to the matchmaking configuration.
*
*/
private String customEventData;
/**
*
* A time stamp indicating when this data object was created. Format is a number expressed in Unix time as
* milliseconds (for example "1469498468.057"
).
*
*/
private java.util.Date creationTime;
/**
*
* A set of custom properties for a game session, formatted as key:value pairs. These properties are passed to a
* game server process in the GameSession object with a request to start a new game session (see Start a Game Session). This information is added to the new GameSession object that is created for a
* successful match. This parameter is not used when FlexMatchMode
is set to STANDALONE
.
*
*/
private java.util.List gameProperties;
/**
*
* A set of custom game session properties, formatted as a single string value. This data is passed to a game server
* process in the GameSession object with a request to start a new game session (see Start a Game Session). This information is added to the new GameSession object that is created for a
* successful match. This parameter is not used when FlexMatchMode
is set to STANDALONE
.
*
*/
private String gameSessionData;
/**
*
* The method used to backfill game sessions created with this matchmaking configuration. MANUAL indicates that the
* game makes backfill requests or does not use the match backfill feature. AUTOMATIC indicates that GameLift
* creates StartMatchBackfill requests whenever a game session has one or more open slots. Learn more about
* manual and automatic backfill in Backfill existing games
* with FlexMatch. Automatic backfill is not available when FlexMatchMode
is set to
* STANDALONE
.
*
*/
private String backfillMode;
/**
*
* Indicates whether this matchmaking configuration is being used with GameLift hosting or as a standalone
* matchmaking solution.
*
*
* -
*
* STANDALONE - FlexMatch forms matches and returns match information, including players and team
* assignments, in a
* MatchmakingSucceeded event.
*
*
* -
*
* WITH_QUEUE - FlexMatch forms matches and uses the specified GameLift queue to start a game session for the
* match.
*
*
*
*/
private String flexMatchMode;
/**
*
* A unique identifier for the matchmaking configuration. This name is used to identify the configuration associated
* with a matchmaking request or ticket.
*
*
* @param name
* A unique identifier for the matchmaking configuration. This name is used to identify the configuration
* associated with a matchmaking request or ticket.
*/
public void setName(String name) {
this.name = name;
}
/**
*
* A unique identifier for the matchmaking configuration. This name is used to identify the configuration associated
* with a matchmaking request or ticket.
*
*
* @return A unique identifier for the matchmaking configuration. This name is used to identify the configuration
* associated with a matchmaking request or ticket.
*/
public String getName() {
return this.name;
}
/**
*
* A unique identifier for the matchmaking configuration. This name is used to identify the configuration associated
* with a matchmaking request or ticket.
*
*
* @param name
* A unique identifier for the matchmaking configuration. This name is used to identify the configuration
* associated with a matchmaking request or ticket.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public MatchmakingConfiguration withName(String name) {
setName(name);
return this;
}
/**
*
* The Amazon Resource Name (ARN)
* that is assigned to a GameLift matchmaking configuration resource and uniquely identifies it. ARNs are unique
* across all Regions. Format is
* arn:aws:gamelift:<region>::matchmakingconfiguration/<matchmaking configuration name>
. In
* a GameLift configuration ARN, the resource ID matches the Name value.
*
*
* @param configurationArn
* The Amazon Resource Name (ARN) that is assigned to a
* GameLift matchmaking configuration resource and uniquely identifies it. ARNs are unique across all
* Regions. Format is
* arn:aws:gamelift:<region>::matchmakingconfiguration/<matchmaking configuration name>
* . In a GameLift configuration ARN, the resource ID matches the Name value.
*/
public void setConfigurationArn(String configurationArn) {
this.configurationArn = configurationArn;
}
/**
*
* The Amazon Resource Name (ARN)
* that is assigned to a GameLift matchmaking configuration resource and uniquely identifies it. ARNs are unique
* across all Regions. Format is
* arn:aws:gamelift:<region>::matchmakingconfiguration/<matchmaking configuration name>
. In
* a GameLift configuration ARN, the resource ID matches the Name value.
*
*
* @return The Amazon Resource Name (ARN) that is assigned to a
* GameLift matchmaking configuration resource and uniquely identifies it. ARNs are unique across all
* Regions. Format is
* arn:aws:gamelift:<region>::matchmakingconfiguration/<matchmaking configuration name>
* . In a GameLift configuration ARN, the resource ID matches the Name value.
*/
public String getConfigurationArn() {
return this.configurationArn;
}
/**
*
* The Amazon Resource Name (ARN)
* that is assigned to a GameLift matchmaking configuration resource and uniquely identifies it. ARNs are unique
* across all Regions. Format is
* arn:aws:gamelift:<region>::matchmakingconfiguration/<matchmaking configuration name>
. In
* a GameLift configuration ARN, the resource ID matches the Name value.
*
*
* @param configurationArn
* The Amazon Resource Name (ARN) that is assigned to a
* GameLift matchmaking configuration resource and uniquely identifies it. ARNs are unique across all
* Regions. Format is
* arn:aws:gamelift:<region>::matchmakingconfiguration/<matchmaking configuration name>
* . In a GameLift configuration ARN, the resource ID matches the Name value.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public MatchmakingConfiguration withConfigurationArn(String configurationArn) {
setConfigurationArn(configurationArn);
return this;
}
/**
*
* A descriptive label that is associated with matchmaking configuration.
*
*
* @param description
* A descriptive label that is associated with matchmaking configuration.
*/
public void setDescription(String description) {
this.description = description;
}
/**
*
* A descriptive label that is associated with matchmaking configuration.
*
*
* @return A descriptive label that is associated with matchmaking configuration.
*/
public String getDescription() {
return this.description;
}
/**
*
* A descriptive label that is associated with matchmaking configuration.
*
*
* @param description
* A descriptive label that is associated with matchmaking configuration.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public MatchmakingConfiguration withDescription(String description) {
setDescription(description);
return this;
}
/**
*
* The Amazon Resource Name (ARN)
* that is assigned to a GameLift game session queue resource and uniquely identifies it. ARNs are unique across all
* Regions. Format is arn:aws:gamelift:<region>::gamesessionqueue/<queue name>
. Queues can
* be located in any Region. Queues are used to start new GameLift-hosted game sessions for matches that are created
* with this matchmaking configuration. This property is not set when FlexMatchMode
is set to
* STANDALONE
.
*
*
* @return The Amazon Resource Name (ARN) that is assigned to a
* GameLift game session queue resource and uniquely identifies it. ARNs are unique across all Regions.
* Format is arn:aws:gamelift:<region>::gamesessionqueue/<queue name>
. Queues can
* be located in any Region. Queues are used to start new GameLift-hosted game sessions for matches that are
* created with this matchmaking configuration. This property is not set when FlexMatchMode
is
* set to STANDALONE
.
*/
public java.util.List getGameSessionQueueArns() {
return gameSessionQueueArns;
}
/**
*
* The Amazon Resource Name (ARN)
* that is assigned to a GameLift game session queue resource and uniquely identifies it. ARNs are unique across all
* Regions. Format is arn:aws:gamelift:<region>::gamesessionqueue/<queue name>
. Queues can
* be located in any Region. Queues are used to start new GameLift-hosted game sessions for matches that are created
* with this matchmaking configuration. This property is not set when FlexMatchMode
is set to
* STANDALONE
.
*
*
* @param gameSessionQueueArns
* The Amazon Resource Name (ARN) that is assigned to a
* GameLift game session queue resource and uniquely identifies it. ARNs are unique across all Regions.
* Format is arn:aws:gamelift:<region>::gamesessionqueue/<queue name>
. Queues can be
* located in any Region. Queues are used to start new GameLift-hosted game sessions for matches that are
* created with this matchmaking configuration. This property is not set when FlexMatchMode
is
* set to STANDALONE
.
*/
public void setGameSessionQueueArns(java.util.Collection gameSessionQueueArns) {
if (gameSessionQueueArns == null) {
this.gameSessionQueueArns = null;
return;
}
this.gameSessionQueueArns = new java.util.ArrayList(gameSessionQueueArns);
}
/**
*
* The Amazon Resource Name (ARN)
* that is assigned to a GameLift game session queue resource and uniquely identifies it. ARNs are unique across all
* Regions. Format is arn:aws:gamelift:<region>::gamesessionqueue/<queue name>
. Queues can
* be located in any Region. Queues are used to start new GameLift-hosted game sessions for matches that are created
* with this matchmaking configuration. This property is not set when FlexMatchMode
is set to
* STANDALONE
.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setGameSessionQueueArns(java.util.Collection)} or {@link #withGameSessionQueueArns(java.util.Collection)}
* if you want to override the existing values.
*
*
* @param gameSessionQueueArns
* The Amazon Resource Name (ARN) that is assigned to a
* GameLift game session queue resource and uniquely identifies it. ARNs are unique across all Regions.
* Format is arn:aws:gamelift:<region>::gamesessionqueue/<queue name>
. Queues can be
* located in any Region. Queues are used to start new GameLift-hosted game sessions for matches that are
* created with this matchmaking configuration. This property is not set when FlexMatchMode
is
* set to STANDALONE
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public MatchmakingConfiguration withGameSessionQueueArns(String... gameSessionQueueArns) {
if (this.gameSessionQueueArns == null) {
setGameSessionQueueArns(new java.util.ArrayList(gameSessionQueueArns.length));
}
for (String ele : gameSessionQueueArns) {
this.gameSessionQueueArns.add(ele);
}
return this;
}
/**
*
* The Amazon Resource Name (ARN)
* that is assigned to a GameLift game session queue resource and uniquely identifies it. ARNs are unique across all
* Regions. Format is arn:aws:gamelift:<region>::gamesessionqueue/<queue name>
. Queues can
* be located in any Region. Queues are used to start new GameLift-hosted game sessions for matches that are created
* with this matchmaking configuration. This property is not set when FlexMatchMode
is set to
* STANDALONE
.
*
*
* @param gameSessionQueueArns
* The Amazon Resource Name (ARN) that is assigned to a
* GameLift game session queue resource and uniquely identifies it. ARNs are unique across all Regions.
* Format is arn:aws:gamelift:<region>::gamesessionqueue/<queue name>
. Queues can be
* located in any Region. Queues are used to start new GameLift-hosted game sessions for matches that are
* created with this matchmaking configuration. This property is not set when FlexMatchMode
is
* set to STANDALONE
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public MatchmakingConfiguration withGameSessionQueueArns(java.util.Collection gameSessionQueueArns) {
setGameSessionQueueArns(gameSessionQueueArns);
return this;
}
/**
*
* The maximum duration, in seconds, that a matchmaking ticket can remain in process before timing out. Requests
* that fail due to timing out can be resubmitted as needed.
*
*
* @param requestTimeoutSeconds
* The maximum duration, in seconds, that a matchmaking ticket can remain in process before timing out.
* Requests that fail due to timing out can be resubmitted as needed.
*/
public void setRequestTimeoutSeconds(Integer requestTimeoutSeconds) {
this.requestTimeoutSeconds = requestTimeoutSeconds;
}
/**
*
* The maximum duration, in seconds, that a matchmaking ticket can remain in process before timing out. Requests
* that fail due to timing out can be resubmitted as needed.
*
*
* @return The maximum duration, in seconds, that a matchmaking ticket can remain in process before timing out.
* Requests that fail due to timing out can be resubmitted as needed.
*/
public Integer getRequestTimeoutSeconds() {
return this.requestTimeoutSeconds;
}
/**
*
* The maximum duration, in seconds, that a matchmaking ticket can remain in process before timing out. Requests
* that fail due to timing out can be resubmitted as needed.
*
*
* @param requestTimeoutSeconds
* The maximum duration, in seconds, that a matchmaking ticket can remain in process before timing out.
* Requests that fail due to timing out can be resubmitted as needed.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public MatchmakingConfiguration withRequestTimeoutSeconds(Integer requestTimeoutSeconds) {
setRequestTimeoutSeconds(requestTimeoutSeconds);
return this;
}
/**
*
* The length of time (in seconds) to wait for players to accept a proposed match, if acceptance is required. If any
* player rejects the match or fails to accept before the timeout, the ticket continues to look for an acceptable
* match.
*
*
* @param acceptanceTimeoutSeconds
* The length of time (in seconds) to wait for players to accept a proposed match, if acceptance is required.
* If any player rejects the match or fails to accept before the timeout, the ticket continues to look for an
* acceptable match.
*/
public void setAcceptanceTimeoutSeconds(Integer acceptanceTimeoutSeconds) {
this.acceptanceTimeoutSeconds = acceptanceTimeoutSeconds;
}
/**
*
* The length of time (in seconds) to wait for players to accept a proposed match, if acceptance is required. If any
* player rejects the match or fails to accept before the timeout, the ticket continues to look for an acceptable
* match.
*
*
* @return The length of time (in seconds) to wait for players to accept a proposed match, if acceptance is
* required. If any player rejects the match or fails to accept before the timeout, the ticket continues to
* look for an acceptable match.
*/
public Integer getAcceptanceTimeoutSeconds() {
return this.acceptanceTimeoutSeconds;
}
/**
*
* The length of time (in seconds) to wait for players to accept a proposed match, if acceptance is required. If any
* player rejects the match or fails to accept before the timeout, the ticket continues to look for an acceptable
* match.
*
*
* @param acceptanceTimeoutSeconds
* The length of time (in seconds) to wait for players to accept a proposed match, if acceptance is required.
* If any player rejects the match or fails to accept before the timeout, the ticket continues to look for an
* acceptable match.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public MatchmakingConfiguration withAcceptanceTimeoutSeconds(Integer acceptanceTimeoutSeconds) {
setAcceptanceTimeoutSeconds(acceptanceTimeoutSeconds);
return this;
}
/**
*
* A flag that indicates whether a match that was created with this configuration must be accepted by the matched
* players. To require acceptance, set to TRUE. When this option is enabled, matchmaking tickets use the status
* REQUIRES_ACCEPTANCE
to indicate when a completed potential match is waiting for player acceptance.
*
*
* @param acceptanceRequired
* A flag that indicates whether a match that was created with this configuration must be accepted by the
* matched players. To require acceptance, set to TRUE. When this option is enabled, matchmaking tickets use
* the status REQUIRES_ACCEPTANCE
to indicate when a completed potential match is waiting for
* player acceptance.
*/
public void setAcceptanceRequired(Boolean acceptanceRequired) {
this.acceptanceRequired = acceptanceRequired;
}
/**
*
* A flag that indicates whether a match that was created with this configuration must be accepted by the matched
* players. To require acceptance, set to TRUE. When this option is enabled, matchmaking tickets use the status
* REQUIRES_ACCEPTANCE
to indicate when a completed potential match is waiting for player acceptance.
*
*
* @return A flag that indicates whether a match that was created with this configuration must be accepted by the
* matched players. To require acceptance, set to TRUE. When this option is enabled, matchmaking tickets use
* the status REQUIRES_ACCEPTANCE
to indicate when a completed potential match is waiting for
* player acceptance.
*/
public Boolean getAcceptanceRequired() {
return this.acceptanceRequired;
}
/**
*
* A flag that indicates whether a match that was created with this configuration must be accepted by the matched
* players. To require acceptance, set to TRUE. When this option is enabled, matchmaking tickets use the status
* REQUIRES_ACCEPTANCE
to indicate when a completed potential match is waiting for player acceptance.
*
*
* @param acceptanceRequired
* A flag that indicates whether a match that was created with this configuration must be accepted by the
* matched players. To require acceptance, set to TRUE. When this option is enabled, matchmaking tickets use
* the status REQUIRES_ACCEPTANCE
to indicate when a completed potential match is waiting for
* player acceptance.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public MatchmakingConfiguration withAcceptanceRequired(Boolean acceptanceRequired) {
setAcceptanceRequired(acceptanceRequired);
return this;
}
/**
*
* A flag that indicates whether a match that was created with this configuration must be accepted by the matched
* players. To require acceptance, set to TRUE. When this option is enabled, matchmaking tickets use the status
* REQUIRES_ACCEPTANCE
to indicate when a completed potential match is waiting for player acceptance.
*
*
* @return A flag that indicates whether a match that was created with this configuration must be accepted by the
* matched players. To require acceptance, set to TRUE. When this option is enabled, matchmaking tickets use
* the status REQUIRES_ACCEPTANCE
to indicate when a completed potential match is waiting for
* player acceptance.
*/
public Boolean isAcceptanceRequired() {
return this.acceptanceRequired;
}
/**
*
* A unique identifier for the matchmaking rule set to use with this configuration. A matchmaking configuration can
* only use rule sets that are defined in the same Region.
*
*
* @param ruleSetName
* A unique identifier for the matchmaking rule set to use with this configuration. A matchmaking
* configuration can only use rule sets that are defined in the same Region.
*/
public void setRuleSetName(String ruleSetName) {
this.ruleSetName = ruleSetName;
}
/**
*
* A unique identifier for the matchmaking rule set to use with this configuration. A matchmaking configuration can
* only use rule sets that are defined in the same Region.
*
*
* @return A unique identifier for the matchmaking rule set to use with this configuration. A matchmaking
* configuration can only use rule sets that are defined in the same Region.
*/
public String getRuleSetName() {
return this.ruleSetName;
}
/**
*
* A unique identifier for the matchmaking rule set to use with this configuration. A matchmaking configuration can
* only use rule sets that are defined in the same Region.
*
*
* @param ruleSetName
* A unique identifier for the matchmaking rule set to use with this configuration. A matchmaking
* configuration can only use rule sets that are defined in the same Region.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public MatchmakingConfiguration withRuleSetName(String ruleSetName) {
setRuleSetName(ruleSetName);
return this;
}
/**
*
* The Amazon Resource Name (ARN)
* associated with the GameLift matchmaking rule set resource that this configuration uses.
*
*
* @param ruleSetArn
* The Amazon Resource Name (ARN) associated with the
* GameLift matchmaking rule set resource that this configuration uses.
*/
public void setRuleSetArn(String ruleSetArn) {
this.ruleSetArn = ruleSetArn;
}
/**
*
* The Amazon Resource Name (ARN)
* associated with the GameLift matchmaking rule set resource that this configuration uses.
*
*
* @return The Amazon Resource Name (ARN) associated with the
* GameLift matchmaking rule set resource that this configuration uses.
*/
public String getRuleSetArn() {
return this.ruleSetArn;
}
/**
*
* The Amazon Resource Name (ARN)
* associated with the GameLift matchmaking rule set resource that this configuration uses.
*
*
* @param ruleSetArn
* The Amazon Resource Name (ARN) associated with the
* GameLift matchmaking rule set resource that this configuration uses.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public MatchmakingConfiguration withRuleSetArn(String ruleSetArn) {
setRuleSetArn(ruleSetArn);
return this;
}
/**
*
* An SNS topic ARN that is set up to receive matchmaking notifications.
*
*
* @param notificationTarget
* An SNS topic ARN that is set up to receive matchmaking notifications.
*/
public void setNotificationTarget(String notificationTarget) {
this.notificationTarget = notificationTarget;
}
/**
*
* An SNS topic ARN that is set up to receive matchmaking notifications.
*
*
* @return An SNS topic ARN that is set up to receive matchmaking notifications.
*/
public String getNotificationTarget() {
return this.notificationTarget;
}
/**
*
* An SNS topic ARN that is set up to receive matchmaking notifications.
*
*
* @param notificationTarget
* An SNS topic ARN that is set up to receive matchmaking notifications.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public MatchmakingConfiguration withNotificationTarget(String notificationTarget) {
setNotificationTarget(notificationTarget);
return this;
}
/**
*
* The number of player slots in a match to keep open for future players. For example, if the configuration's rule
* set specifies a match for a single 12-person team, and the additional player count is set to 2, only 10 players
* are selected for the match. This parameter is not used when FlexMatchMode
is set to
* STANDALONE
.
*
*
* @param additionalPlayerCount
* The number of player slots in a match to keep open for future players. For example, if the configuration's
* rule set specifies a match for a single 12-person team, and the additional player count is set to 2, only
* 10 players are selected for the match. This parameter is not used when FlexMatchMode
is set
* to STANDALONE
.
*/
public void setAdditionalPlayerCount(Integer additionalPlayerCount) {
this.additionalPlayerCount = additionalPlayerCount;
}
/**
*
* The number of player slots in a match to keep open for future players. For example, if the configuration's rule
* set specifies a match for a single 12-person team, and the additional player count is set to 2, only 10 players
* are selected for the match. This parameter is not used when FlexMatchMode
is set to
* STANDALONE
.
*
*
* @return The number of player slots in a match to keep open for future players. For example, if the
* configuration's rule set specifies a match for a single 12-person team, and the additional player count
* is set to 2, only 10 players are selected for the match. This parameter is not used when
* FlexMatchMode
is set to STANDALONE
.
*/
public Integer getAdditionalPlayerCount() {
return this.additionalPlayerCount;
}
/**
*
* The number of player slots in a match to keep open for future players. For example, if the configuration's rule
* set specifies a match for a single 12-person team, and the additional player count is set to 2, only 10 players
* are selected for the match. This parameter is not used when FlexMatchMode
is set to
* STANDALONE
.
*
*
* @param additionalPlayerCount
* The number of player slots in a match to keep open for future players. For example, if the configuration's
* rule set specifies a match for a single 12-person team, and the additional player count is set to 2, only
* 10 players are selected for the match. This parameter is not used when FlexMatchMode
is set
* to STANDALONE
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public MatchmakingConfiguration withAdditionalPlayerCount(Integer additionalPlayerCount) {
setAdditionalPlayerCount(additionalPlayerCount);
return this;
}
/**
*
* Information to attach to all events related to the matchmaking configuration.
*
*
* @param customEventData
* Information to attach to all events related to the matchmaking configuration.
*/
public void setCustomEventData(String customEventData) {
this.customEventData = customEventData;
}
/**
*
* Information to attach to all events related to the matchmaking configuration.
*
*
* @return Information to attach to all events related to the matchmaking configuration.
*/
public String getCustomEventData() {
return this.customEventData;
}
/**
*
* Information to attach to all events related to the matchmaking configuration.
*
*
* @param customEventData
* Information to attach to all events related to the matchmaking configuration.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public MatchmakingConfiguration withCustomEventData(String customEventData) {
setCustomEventData(customEventData);
return this;
}
/**
*
* A time stamp indicating when this data object was created. Format is a number expressed in Unix time as
* milliseconds (for example "1469498468.057"
).
*
*
* @param creationTime
* A time stamp indicating when this data object was created. Format is a number expressed in Unix time as
* milliseconds (for example "1469498468.057"
).
*/
public void setCreationTime(java.util.Date creationTime) {
this.creationTime = creationTime;
}
/**
*
* A time stamp indicating when this data object was created. Format is a number expressed in Unix time as
* milliseconds (for example "1469498468.057"
).
*
*
* @return A time stamp indicating when this data object was created. Format is a number expressed in Unix time as
* milliseconds (for example "1469498468.057"
).
*/
public java.util.Date getCreationTime() {
return this.creationTime;
}
/**
*
* A time stamp indicating when this data object was created. Format is a number expressed in Unix time as
* milliseconds (for example "1469498468.057"
).
*
*
* @param creationTime
* A time stamp indicating when this data object was created. Format is a number expressed in Unix time as
* milliseconds (for example "1469498468.057"
).
* @return Returns a reference to this object so that method calls can be chained together.
*/
public MatchmakingConfiguration withCreationTime(java.util.Date creationTime) {
setCreationTime(creationTime);
return this;
}
/**
*
* A set of custom properties for a game session, formatted as key:value pairs. These properties are passed to a
* game server process in the GameSession object with a request to start a new game session (see Start a Game Session). This information is added to the new GameSession object that is created for a
* successful match. This parameter is not used when FlexMatchMode
is set to STANDALONE
.
*
*
* @return A set of custom properties for a game session, formatted as key:value pairs. These properties are passed
* to a game server process in the GameSession object with a request to start a new game session (see
* Start a Game Session). This information is added to the new GameSession object that is
* created for a successful match. This parameter is not used when FlexMatchMode
is set to
* STANDALONE
.
*/
public java.util.List getGameProperties() {
return gameProperties;
}
/**
*
* A set of custom properties for a game session, formatted as key:value pairs. These properties are passed to a
* game server process in the GameSession object with a request to start a new game session (see Start a Game Session). This information is added to the new GameSession object that is created for a
* successful match. This parameter is not used when FlexMatchMode
is set to STANDALONE
.
*
*
* @param gameProperties
* A set of custom properties for a game session, formatted as key:value pairs. These properties are passed
* to a game server process in the GameSession object with a request to start a new game session (see
* Start a Game Session). This information is added to the new GameSession object that is created
* for a successful match. This parameter is not used when FlexMatchMode
is set to
* STANDALONE
.
*/
public void setGameProperties(java.util.Collection gameProperties) {
if (gameProperties == null) {
this.gameProperties = null;
return;
}
this.gameProperties = new java.util.ArrayList(gameProperties);
}
/**
*
* A set of custom properties for a game session, formatted as key:value pairs. These properties are passed to a
* game server process in the GameSession object with a request to start a new game session (see Start a Game Session). This information is added to the new GameSession object that is created for a
* successful match. This parameter is not used when FlexMatchMode
is set to STANDALONE
.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setGameProperties(java.util.Collection)} or {@link #withGameProperties(java.util.Collection)} if you want
* to override the existing values.
*
*
* @param gameProperties
* A set of custom properties for a game session, formatted as key:value pairs. These properties are passed
* to a game server process in the GameSession object with a request to start a new game session (see
* Start a Game Session). This information is added to the new GameSession object that is created
* for a successful match. This parameter is not used when FlexMatchMode
is set to
* STANDALONE
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public MatchmakingConfiguration withGameProperties(GameProperty... gameProperties) {
if (this.gameProperties == null) {
setGameProperties(new java.util.ArrayList(gameProperties.length));
}
for (GameProperty ele : gameProperties) {
this.gameProperties.add(ele);
}
return this;
}
/**
*
* A set of custom properties for a game session, formatted as key:value pairs. These properties are passed to a
* game server process in the GameSession object with a request to start a new game session (see Start a Game Session). This information is added to the new GameSession object that is created for a
* successful match. This parameter is not used when FlexMatchMode
is set to STANDALONE
.
*
*
* @param gameProperties
* A set of custom properties for a game session, formatted as key:value pairs. These properties are passed
* to a game server process in the GameSession object with a request to start a new game session (see
* Start a Game Session). This information is added to the new GameSession object that is created
* for a successful match. This parameter is not used when FlexMatchMode
is set to
* STANDALONE
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public MatchmakingConfiguration withGameProperties(java.util.Collection gameProperties) {
setGameProperties(gameProperties);
return this;
}
/**
*
* A set of custom game session properties, formatted as a single string value. This data is passed to a game server
* process in the GameSession object with a request to start a new game session (see Start a Game Session). This information is added to the new GameSession object that is created for a
* successful match. This parameter is not used when FlexMatchMode
is set to STANDALONE
.
*
*
* @param gameSessionData
* A set of custom game session properties, formatted as a single string value. This data is passed to a game
* server process in the GameSession object with a request to start a new game session (see Start a Game Session). This information is added to the new GameSession object that is created
* for a successful match. This parameter is not used when FlexMatchMode
is set to
* STANDALONE
.
*/
public void setGameSessionData(String gameSessionData) {
this.gameSessionData = gameSessionData;
}
/**
*
* A set of custom game session properties, formatted as a single string value. This data is passed to a game server
* process in the GameSession object with a request to start a new game session (see Start a Game Session). This information is added to the new GameSession object that is created for a
* successful match. This parameter is not used when FlexMatchMode
is set to STANDALONE
.
*
*
* @return A set of custom game session properties, formatted as a single string value. This data is passed to a
* game server process in the GameSession object with a request to start a new game session (see Start a Game Session). This information is added to the new GameSession object that is
* created for a successful match. This parameter is not used when FlexMatchMode
is set to
* STANDALONE
.
*/
public String getGameSessionData() {
return this.gameSessionData;
}
/**
*
* A set of custom game session properties, formatted as a single string value. This data is passed to a game server
* process in the GameSession object with a request to start a new game session (see Start a Game Session). This information is added to the new GameSession object that is created for a
* successful match. This parameter is not used when FlexMatchMode
is set to STANDALONE
.
*
*
* @param gameSessionData
* A set of custom game session properties, formatted as a single string value. This data is passed to a game
* server process in the GameSession object with a request to start a new game session (see Start a Game Session). This information is added to the new GameSession object that is created
* for a successful match. This parameter is not used when FlexMatchMode
is set to
* STANDALONE
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public MatchmakingConfiguration withGameSessionData(String gameSessionData) {
setGameSessionData(gameSessionData);
return this;
}
/**
*
* The method used to backfill game sessions created with this matchmaking configuration. MANUAL indicates that the
* game makes backfill requests or does not use the match backfill feature. AUTOMATIC indicates that GameLift
* creates StartMatchBackfill requests whenever a game session has one or more open slots. Learn more about
* manual and automatic backfill in Backfill existing games
* with FlexMatch. Automatic backfill is not available when FlexMatchMode
is set to
* STANDALONE
.
*
*
* @param backfillMode
* The method used to backfill game sessions created with this matchmaking configuration. MANUAL indicates
* that the game makes backfill requests or does not use the match backfill feature. AUTOMATIC indicates that
* GameLift creates StartMatchBackfill requests whenever a game session has one or more open slots.
* Learn more about manual and automatic backfill in Backfill existing
* games with FlexMatch. Automatic backfill is not available when FlexMatchMode
is set to
* STANDALONE
.
* @see BackfillMode
*/
public void setBackfillMode(String backfillMode) {
this.backfillMode = backfillMode;
}
/**
*
* The method used to backfill game sessions created with this matchmaking configuration. MANUAL indicates that the
* game makes backfill requests or does not use the match backfill feature. AUTOMATIC indicates that GameLift
* creates StartMatchBackfill requests whenever a game session has one or more open slots. Learn more about
* manual and automatic backfill in Backfill existing games
* with FlexMatch. Automatic backfill is not available when FlexMatchMode
is set to
* STANDALONE
.
*
*
* @return The method used to backfill game sessions created with this matchmaking configuration. MANUAL indicates
* that the game makes backfill requests or does not use the match backfill feature. AUTOMATIC indicates
* that GameLift creates StartMatchBackfill requests whenever a game session has one or more open
* slots. Learn more about manual and automatic backfill in Backfill existing
* games with FlexMatch. Automatic backfill is not available when FlexMatchMode
is set to
* STANDALONE
.
* @see BackfillMode
*/
public String getBackfillMode() {
return this.backfillMode;
}
/**
*
* The method used to backfill game sessions created with this matchmaking configuration. MANUAL indicates that the
* game makes backfill requests or does not use the match backfill feature. AUTOMATIC indicates that GameLift
* creates StartMatchBackfill requests whenever a game session has one or more open slots. Learn more about
* manual and automatic backfill in Backfill existing games
* with FlexMatch. Automatic backfill is not available when FlexMatchMode
is set to
* STANDALONE
.
*
*
* @param backfillMode
* The method used to backfill game sessions created with this matchmaking configuration. MANUAL indicates
* that the game makes backfill requests or does not use the match backfill feature. AUTOMATIC indicates that
* GameLift creates StartMatchBackfill requests whenever a game session has one or more open slots.
* Learn more about manual and automatic backfill in Backfill existing
* games with FlexMatch. Automatic backfill is not available when FlexMatchMode
is set to
* STANDALONE
.
* @return Returns a reference to this object so that method calls can be chained together.
* @see BackfillMode
*/
public MatchmakingConfiguration withBackfillMode(String backfillMode) {
setBackfillMode(backfillMode);
return this;
}
/**
*
* The method used to backfill game sessions created with this matchmaking configuration. MANUAL indicates that the
* game makes backfill requests or does not use the match backfill feature. AUTOMATIC indicates that GameLift
* creates StartMatchBackfill requests whenever a game session has one or more open slots. Learn more about
* manual and automatic backfill in Backfill existing games
* with FlexMatch. Automatic backfill is not available when FlexMatchMode
is set to
* STANDALONE
.
*
*
* @param backfillMode
* The method used to backfill game sessions created with this matchmaking configuration. MANUAL indicates
* that the game makes backfill requests or does not use the match backfill feature. AUTOMATIC indicates that
* GameLift creates StartMatchBackfill requests whenever a game session has one or more open slots.
* Learn more about manual and automatic backfill in Backfill existing
* games with FlexMatch. Automatic backfill is not available when FlexMatchMode
is set to
* STANDALONE
.
* @return Returns a reference to this object so that method calls can be chained together.
* @see BackfillMode
*/
public MatchmakingConfiguration withBackfillMode(BackfillMode backfillMode) {
this.backfillMode = backfillMode.toString();
return this;
}
/**
*
* Indicates whether this matchmaking configuration is being used with GameLift hosting or as a standalone
* matchmaking solution.
*
*
* -
*
* STANDALONE - FlexMatch forms matches and returns match information, including players and team
* assignments, in a
* MatchmakingSucceeded event.
*
*
* -
*
* WITH_QUEUE - FlexMatch forms matches and uses the specified GameLift queue to start a game session for the
* match.
*
*
*
*
* @param flexMatchMode
* Indicates whether this matchmaking configuration is being used with GameLift hosting or as a standalone
* matchmaking solution.
*
* -
*
* STANDALONE - FlexMatch forms matches and returns match information, including players and team
* assignments, in a MatchmakingSucceeded event.
*
*
* -
*
* WITH_QUEUE - FlexMatch forms matches and uses the specified GameLift queue to start a game session
* for the match.
*
*
* @see FlexMatchMode
*/
public void setFlexMatchMode(String flexMatchMode) {
this.flexMatchMode = flexMatchMode;
}
/**
*
* Indicates whether this matchmaking configuration is being used with GameLift hosting or as a standalone
* matchmaking solution.
*
*
* -
*
* STANDALONE - FlexMatch forms matches and returns match information, including players and team
* assignments, in a
* MatchmakingSucceeded event.
*
*
* -
*
* WITH_QUEUE - FlexMatch forms matches and uses the specified GameLift queue to start a game session for the
* match.
*
*
*
*
* @return Indicates whether this matchmaking configuration is being used with GameLift hosting or as a standalone
* matchmaking solution.
*
* -
*
* STANDALONE - FlexMatch forms matches and returns match information, including players and team
* assignments, in a MatchmakingSucceeded event.
*
*
* -
*
* WITH_QUEUE - FlexMatch forms matches and uses the specified GameLift queue to start a game session
* for the match.
*
*
* @see FlexMatchMode
*/
public String getFlexMatchMode() {
return this.flexMatchMode;
}
/**
*
* Indicates whether this matchmaking configuration is being used with GameLift hosting or as a standalone
* matchmaking solution.
*
*
* -
*
* STANDALONE - FlexMatch forms matches and returns match information, including players and team
* assignments, in a
* MatchmakingSucceeded event.
*
*
* -
*
* WITH_QUEUE - FlexMatch forms matches and uses the specified GameLift queue to start a game session for the
* match.
*
*
*
*
* @param flexMatchMode
* Indicates whether this matchmaking configuration is being used with GameLift hosting or as a standalone
* matchmaking solution.
*
* -
*
* STANDALONE - FlexMatch forms matches and returns match information, including players and team
* assignments, in a MatchmakingSucceeded event.
*
*
* -
*
* WITH_QUEUE - FlexMatch forms matches and uses the specified GameLift queue to start a game session
* for the match.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see FlexMatchMode
*/
public MatchmakingConfiguration withFlexMatchMode(String flexMatchMode) {
setFlexMatchMode(flexMatchMode);
return this;
}
/**
*
* Indicates whether this matchmaking configuration is being used with GameLift hosting or as a standalone
* matchmaking solution.
*
*
* -
*
* STANDALONE - FlexMatch forms matches and returns match information, including players and team
* assignments, in a
* MatchmakingSucceeded event.
*
*
* -
*
* WITH_QUEUE - FlexMatch forms matches and uses the specified GameLift queue to start a game session for the
* match.
*
*
*
*
* @param flexMatchMode
* Indicates whether this matchmaking configuration is being used with GameLift hosting or as a standalone
* matchmaking solution.
*
* -
*
* STANDALONE - FlexMatch forms matches and returns match information, including players and team
* assignments, in a MatchmakingSucceeded event.
*
*
* -
*
* WITH_QUEUE - FlexMatch forms matches and uses the specified GameLift queue to start a game session
* for the match.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see FlexMatchMode
*/
public MatchmakingConfiguration withFlexMatchMode(FlexMatchMode flexMatchMode) {
this.flexMatchMode = flexMatchMode.toString();
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getName() != null)
sb.append("Name: ").append(getName()).append(",");
if (getConfigurationArn() != null)
sb.append("ConfigurationArn: ").append(getConfigurationArn()).append(",");
if (getDescription() != null)
sb.append("Description: ").append(getDescription()).append(",");
if (getGameSessionQueueArns() != null)
sb.append("GameSessionQueueArns: ").append(getGameSessionQueueArns()).append(",");
if (getRequestTimeoutSeconds() != null)
sb.append("RequestTimeoutSeconds: ").append(getRequestTimeoutSeconds()).append(",");
if (getAcceptanceTimeoutSeconds() != null)
sb.append("AcceptanceTimeoutSeconds: ").append(getAcceptanceTimeoutSeconds()).append(",");
if (getAcceptanceRequired() != null)
sb.append("AcceptanceRequired: ").append(getAcceptanceRequired()).append(",");
if (getRuleSetName() != null)
sb.append("RuleSetName: ").append(getRuleSetName()).append(",");
if (getRuleSetArn() != null)
sb.append("RuleSetArn: ").append(getRuleSetArn()).append(",");
if (getNotificationTarget() != null)
sb.append("NotificationTarget: ").append(getNotificationTarget()).append(",");
if (getAdditionalPlayerCount() != null)
sb.append("AdditionalPlayerCount: ").append(getAdditionalPlayerCount()).append(",");
if (getCustomEventData() != null)
sb.append("CustomEventData: ").append(getCustomEventData()).append(",");
if (getCreationTime() != null)
sb.append("CreationTime: ").append(getCreationTime()).append(",");
if (getGameProperties() != null)
sb.append("GameProperties: ").append(getGameProperties()).append(",");
if (getGameSessionData() != null)
sb.append("GameSessionData: ").append(getGameSessionData()).append(",");
if (getBackfillMode() != null)
sb.append("BackfillMode: ").append(getBackfillMode()).append(",");
if (getFlexMatchMode() != null)
sb.append("FlexMatchMode: ").append(getFlexMatchMode());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof MatchmakingConfiguration == false)
return false;
MatchmakingConfiguration other = (MatchmakingConfiguration) obj;
if (other.getName() == null ^ this.getName() == null)
return false;
if (other.getName() != null && other.getName().equals(this.getName()) == false)
return false;
if (other.getConfigurationArn() == null ^ this.getConfigurationArn() == null)
return false;
if (other.getConfigurationArn() != null && other.getConfigurationArn().equals(this.getConfigurationArn()) == false)
return false;
if (other.getDescription() == null ^ this.getDescription() == null)
return false;
if (other.getDescription() != null && other.getDescription().equals(this.getDescription()) == false)
return false;
if (other.getGameSessionQueueArns() == null ^ this.getGameSessionQueueArns() == null)
return false;
if (other.getGameSessionQueueArns() != null && other.getGameSessionQueueArns().equals(this.getGameSessionQueueArns()) == false)
return false;
if (other.getRequestTimeoutSeconds() == null ^ this.getRequestTimeoutSeconds() == null)
return false;
if (other.getRequestTimeoutSeconds() != null && other.getRequestTimeoutSeconds().equals(this.getRequestTimeoutSeconds()) == false)
return false;
if (other.getAcceptanceTimeoutSeconds() == null ^ this.getAcceptanceTimeoutSeconds() == null)
return false;
if (other.getAcceptanceTimeoutSeconds() != null && other.getAcceptanceTimeoutSeconds().equals(this.getAcceptanceTimeoutSeconds()) == false)
return false;
if (other.getAcceptanceRequired() == null ^ this.getAcceptanceRequired() == null)
return false;
if (other.getAcceptanceRequired() != null && other.getAcceptanceRequired().equals(this.getAcceptanceRequired()) == false)
return false;
if (other.getRuleSetName() == null ^ this.getRuleSetName() == null)
return false;
if (other.getRuleSetName() != null && other.getRuleSetName().equals(this.getRuleSetName()) == false)
return false;
if (other.getRuleSetArn() == null ^ this.getRuleSetArn() == null)
return false;
if (other.getRuleSetArn() != null && other.getRuleSetArn().equals(this.getRuleSetArn()) == false)
return false;
if (other.getNotificationTarget() == null ^ this.getNotificationTarget() == null)
return false;
if (other.getNotificationTarget() != null && other.getNotificationTarget().equals(this.getNotificationTarget()) == false)
return false;
if (other.getAdditionalPlayerCount() == null ^ this.getAdditionalPlayerCount() == null)
return false;
if (other.getAdditionalPlayerCount() != null && other.getAdditionalPlayerCount().equals(this.getAdditionalPlayerCount()) == false)
return false;
if (other.getCustomEventData() == null ^ this.getCustomEventData() == null)
return false;
if (other.getCustomEventData() != null && other.getCustomEventData().equals(this.getCustomEventData()) == false)
return false;
if (other.getCreationTime() == null ^ this.getCreationTime() == null)
return false;
if (other.getCreationTime() != null && other.getCreationTime().equals(this.getCreationTime()) == false)
return false;
if (other.getGameProperties() == null ^ this.getGameProperties() == null)
return false;
if (other.getGameProperties() != null && other.getGameProperties().equals(this.getGameProperties()) == false)
return false;
if (other.getGameSessionData() == null ^ this.getGameSessionData() == null)
return false;
if (other.getGameSessionData() != null && other.getGameSessionData().equals(this.getGameSessionData()) == false)
return false;
if (other.getBackfillMode() == null ^ this.getBackfillMode() == null)
return false;
if (other.getBackfillMode() != null && other.getBackfillMode().equals(this.getBackfillMode()) == false)
return false;
if (other.getFlexMatchMode() == null ^ this.getFlexMatchMode() == null)
return false;
if (other.getFlexMatchMode() != null && other.getFlexMatchMode().equals(this.getFlexMatchMode()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getName() == null) ? 0 : getName().hashCode());
hashCode = prime * hashCode + ((getConfigurationArn() == null) ? 0 : getConfigurationArn().hashCode());
hashCode = prime * hashCode + ((getDescription() == null) ? 0 : getDescription().hashCode());
hashCode = prime * hashCode + ((getGameSessionQueueArns() == null) ? 0 : getGameSessionQueueArns().hashCode());
hashCode = prime * hashCode + ((getRequestTimeoutSeconds() == null) ? 0 : getRequestTimeoutSeconds().hashCode());
hashCode = prime * hashCode + ((getAcceptanceTimeoutSeconds() == null) ? 0 : getAcceptanceTimeoutSeconds().hashCode());
hashCode = prime * hashCode + ((getAcceptanceRequired() == null) ? 0 : getAcceptanceRequired().hashCode());
hashCode = prime * hashCode + ((getRuleSetName() == null) ? 0 : getRuleSetName().hashCode());
hashCode = prime * hashCode + ((getRuleSetArn() == null) ? 0 : getRuleSetArn().hashCode());
hashCode = prime * hashCode + ((getNotificationTarget() == null) ? 0 : getNotificationTarget().hashCode());
hashCode = prime * hashCode + ((getAdditionalPlayerCount() == null) ? 0 : getAdditionalPlayerCount().hashCode());
hashCode = prime * hashCode + ((getCustomEventData() == null) ? 0 : getCustomEventData().hashCode());
hashCode = prime * hashCode + ((getCreationTime() == null) ? 0 : getCreationTime().hashCode());
hashCode = prime * hashCode + ((getGameProperties() == null) ? 0 : getGameProperties().hashCode());
hashCode = prime * hashCode + ((getGameSessionData() == null) ? 0 : getGameSessionData().hashCode());
hashCode = prime * hashCode + ((getBackfillMode() == null) ? 0 : getBackfillMode().hashCode());
hashCode = prime * hashCode + ((getFlexMatchMode() == null) ? 0 : getFlexMatchMode().hashCode());
return hashCode;
}
@Override
public MatchmakingConfiguration clone() {
try {
return (MatchmakingConfiguration) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.gamelift.model.transform.MatchmakingConfigurationMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}