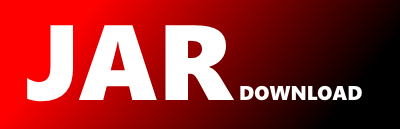
com.amazonaws.services.gamelift.model.PlayerSession Maven / Gradle / Ivy
/*
* Copyright 2017-2022 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.gamelift.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* Represents a player session. Player sessions are created either for a specific game session, or as part of a game
* session placement or matchmaking request. A player session can represents a reserved player slot in a game session
* (when status is RESERVED
) or actual player activity in a game session (when status is
* ACTIVE
). A player session object, including player data, is automatically passed to a game session when
* the player connects to the game session and is validated. After the game session ends, player sessions information is
* retained for 30 days and then removed.
*
*
* Related actions
*
*
* CreatePlayerSession | CreatePlayerSessions | DescribePlayerSessions |
* StartGameSessionPlacement | DescribeGameSessionPlacement | All APIs by task
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class PlayerSession implements Serializable, Cloneable, StructuredPojo {
/**
*
* A unique identifier for a player session.
*
*/
private String playerSessionId;
/**
*
* A unique identifier for a player that is associated with this player session.
*
*/
private String playerId;
/**
*
* A unique identifier for the game session that the player session is connected to.
*
*/
private String gameSessionId;
/**
*
* A unique identifier for the fleet that the player's game session is running on.
*
*/
private String fleetId;
/**
*
* The Amazon Resource Name (ARN)
* associated with the GameLift fleet that the player's game session is running on.
*
*/
private String fleetArn;
/**
*
* A time stamp indicating when this data object was created. Format is a number expressed in Unix time as
* milliseconds (for example "1469498468.057"
).
*
*/
private java.util.Date creationTime;
/**
*
* A time stamp indicating when this data object was terminated. Format is a number expressed in Unix time as
* milliseconds (for example "1469498468.057"
).
*
*/
private java.util.Date terminationTime;
/**
*
* Current status of the player session.
*
*
* Possible player session statuses include the following:
*
*
* -
*
* RESERVED -- The player session request has been received, but the player has not yet connected to the
* server process and/or been validated.
*
*
* -
*
* ACTIVE -- The player has been validated by the server process and is currently connected.
*
*
* -
*
* COMPLETED -- The player connection has been dropped.
*
*
* -
*
* TIMEDOUT -- A player session request was received, but the player did not connect and/or was not validated
* within the timeout limit (60 seconds).
*
*
*
*/
private String status;
/**
*
* The IP address of the game session. To connect to a GameLift game server, an app needs both the IP address and
* port number.
*
*/
private String ipAddress;
/**
*
* The DNS identifier assigned to the instance that is running the game session. Values have the following format:
*
*
* -
*
* TLS-enabled fleets: <unique identifier>.<region identifier>.amazongamelift.com
.
*
*
* -
*
* Non-TLS-enabled fleets: ec2-<unique identifier>.compute.amazonaws.com
. (See Amazon EC2 Instance IP Addressing.)
*
*
*
*
* When connecting to a game session that is running on a TLS-enabled fleet, you must use the DNS name, not the IP
* address.
*
*/
private String dnsName;
/**
*
* Port number for the game session. To connect to a Amazon Web Services server process, an app needs both the IP
* address and port number.
*
*/
private Integer port;
/**
*
* Developer-defined information related to a player. GameLift does not use this data, so it can be formatted as
* needed for use in the game.
*
*/
private String playerData;
/**
*
* A unique identifier for a player session.
*
*
* @param playerSessionId
* A unique identifier for a player session.
*/
public void setPlayerSessionId(String playerSessionId) {
this.playerSessionId = playerSessionId;
}
/**
*
* A unique identifier for a player session.
*
*
* @return A unique identifier for a player session.
*/
public String getPlayerSessionId() {
return this.playerSessionId;
}
/**
*
* A unique identifier for a player session.
*
*
* @param playerSessionId
* A unique identifier for a player session.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PlayerSession withPlayerSessionId(String playerSessionId) {
setPlayerSessionId(playerSessionId);
return this;
}
/**
*
* A unique identifier for a player that is associated with this player session.
*
*
* @param playerId
* A unique identifier for a player that is associated with this player session.
*/
public void setPlayerId(String playerId) {
this.playerId = playerId;
}
/**
*
* A unique identifier for a player that is associated with this player session.
*
*
* @return A unique identifier for a player that is associated with this player session.
*/
public String getPlayerId() {
return this.playerId;
}
/**
*
* A unique identifier for a player that is associated with this player session.
*
*
* @param playerId
* A unique identifier for a player that is associated with this player session.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PlayerSession withPlayerId(String playerId) {
setPlayerId(playerId);
return this;
}
/**
*
* A unique identifier for the game session that the player session is connected to.
*
*
* @param gameSessionId
* A unique identifier for the game session that the player session is connected to.
*/
public void setGameSessionId(String gameSessionId) {
this.gameSessionId = gameSessionId;
}
/**
*
* A unique identifier for the game session that the player session is connected to.
*
*
* @return A unique identifier for the game session that the player session is connected to.
*/
public String getGameSessionId() {
return this.gameSessionId;
}
/**
*
* A unique identifier for the game session that the player session is connected to.
*
*
* @param gameSessionId
* A unique identifier for the game session that the player session is connected to.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PlayerSession withGameSessionId(String gameSessionId) {
setGameSessionId(gameSessionId);
return this;
}
/**
*
* A unique identifier for the fleet that the player's game session is running on.
*
*
* @param fleetId
* A unique identifier for the fleet that the player's game session is running on.
*/
public void setFleetId(String fleetId) {
this.fleetId = fleetId;
}
/**
*
* A unique identifier for the fleet that the player's game session is running on.
*
*
* @return A unique identifier for the fleet that the player's game session is running on.
*/
public String getFleetId() {
return this.fleetId;
}
/**
*
* A unique identifier for the fleet that the player's game session is running on.
*
*
* @param fleetId
* A unique identifier for the fleet that the player's game session is running on.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PlayerSession withFleetId(String fleetId) {
setFleetId(fleetId);
return this;
}
/**
*
* The Amazon Resource Name (ARN)
* associated with the GameLift fleet that the player's game session is running on.
*
*
* @param fleetArn
* The Amazon Resource Name (ARN) associated with the
* GameLift fleet that the player's game session is running on.
*/
public void setFleetArn(String fleetArn) {
this.fleetArn = fleetArn;
}
/**
*
* The Amazon Resource Name (ARN)
* associated with the GameLift fleet that the player's game session is running on.
*
*
* @return The Amazon Resource Name (ARN) associated with the
* GameLift fleet that the player's game session is running on.
*/
public String getFleetArn() {
return this.fleetArn;
}
/**
*
* The Amazon Resource Name (ARN)
* associated with the GameLift fleet that the player's game session is running on.
*
*
* @param fleetArn
* The Amazon Resource Name (ARN) associated with the
* GameLift fleet that the player's game session is running on.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PlayerSession withFleetArn(String fleetArn) {
setFleetArn(fleetArn);
return this;
}
/**
*
* A time stamp indicating when this data object was created. Format is a number expressed in Unix time as
* milliseconds (for example "1469498468.057"
).
*
*
* @param creationTime
* A time stamp indicating when this data object was created. Format is a number expressed in Unix time as
* milliseconds (for example "1469498468.057"
).
*/
public void setCreationTime(java.util.Date creationTime) {
this.creationTime = creationTime;
}
/**
*
* A time stamp indicating when this data object was created. Format is a number expressed in Unix time as
* milliseconds (for example "1469498468.057"
).
*
*
* @return A time stamp indicating when this data object was created. Format is a number expressed in Unix time as
* milliseconds (for example "1469498468.057"
).
*/
public java.util.Date getCreationTime() {
return this.creationTime;
}
/**
*
* A time stamp indicating when this data object was created. Format is a number expressed in Unix time as
* milliseconds (for example "1469498468.057"
).
*
*
* @param creationTime
* A time stamp indicating when this data object was created. Format is a number expressed in Unix time as
* milliseconds (for example "1469498468.057"
).
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PlayerSession withCreationTime(java.util.Date creationTime) {
setCreationTime(creationTime);
return this;
}
/**
*
* A time stamp indicating when this data object was terminated. Format is a number expressed in Unix time as
* milliseconds (for example "1469498468.057"
).
*
*
* @param terminationTime
* A time stamp indicating when this data object was terminated. Format is a number expressed in Unix time as
* milliseconds (for example "1469498468.057"
).
*/
public void setTerminationTime(java.util.Date terminationTime) {
this.terminationTime = terminationTime;
}
/**
*
* A time stamp indicating when this data object was terminated. Format is a number expressed in Unix time as
* milliseconds (for example "1469498468.057"
).
*
*
* @return A time stamp indicating when this data object was terminated. Format is a number expressed in Unix time
* as milliseconds (for example "1469498468.057"
).
*/
public java.util.Date getTerminationTime() {
return this.terminationTime;
}
/**
*
* A time stamp indicating when this data object was terminated. Format is a number expressed in Unix time as
* milliseconds (for example "1469498468.057"
).
*
*
* @param terminationTime
* A time stamp indicating when this data object was terminated. Format is a number expressed in Unix time as
* milliseconds (for example "1469498468.057"
).
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PlayerSession withTerminationTime(java.util.Date terminationTime) {
setTerminationTime(terminationTime);
return this;
}
/**
*
* Current status of the player session.
*
*
* Possible player session statuses include the following:
*
*
* -
*
* RESERVED -- The player session request has been received, but the player has not yet connected to the
* server process and/or been validated.
*
*
* -
*
* ACTIVE -- The player has been validated by the server process and is currently connected.
*
*
* -
*
* COMPLETED -- The player connection has been dropped.
*
*
* -
*
* TIMEDOUT -- A player session request was received, but the player did not connect and/or was not validated
* within the timeout limit (60 seconds).
*
*
*
*
* @param status
* Current status of the player session.
*
* Possible player session statuses include the following:
*
*
* -
*
* RESERVED -- The player session request has been received, but the player has not yet connected to
* the server process and/or been validated.
*
*
* -
*
* ACTIVE -- The player has been validated by the server process and is currently connected.
*
*
* -
*
* COMPLETED -- The player connection has been dropped.
*
*
* -
*
* TIMEDOUT -- A player session request was received, but the player did not connect and/or was not
* validated within the timeout limit (60 seconds).
*
*
* @see PlayerSessionStatus
*/
public void setStatus(String status) {
this.status = status;
}
/**
*
* Current status of the player session.
*
*
* Possible player session statuses include the following:
*
*
* -
*
* RESERVED -- The player session request has been received, but the player has not yet connected to the
* server process and/or been validated.
*
*
* -
*
* ACTIVE -- The player has been validated by the server process and is currently connected.
*
*
* -
*
* COMPLETED -- The player connection has been dropped.
*
*
* -
*
* TIMEDOUT -- A player session request was received, but the player did not connect and/or was not validated
* within the timeout limit (60 seconds).
*
*
*
*
* @return Current status of the player session.
*
* Possible player session statuses include the following:
*
*
* -
*
* RESERVED -- The player session request has been received, but the player has not yet connected to
* the server process and/or been validated.
*
*
* -
*
* ACTIVE -- The player has been validated by the server process and is currently connected.
*
*
* -
*
* COMPLETED -- The player connection has been dropped.
*
*
* -
*
* TIMEDOUT -- A player session request was received, but the player did not connect and/or was not
* validated within the timeout limit (60 seconds).
*
*
* @see PlayerSessionStatus
*/
public String getStatus() {
return this.status;
}
/**
*
* Current status of the player session.
*
*
* Possible player session statuses include the following:
*
*
* -
*
* RESERVED -- The player session request has been received, but the player has not yet connected to the
* server process and/or been validated.
*
*
* -
*
* ACTIVE -- The player has been validated by the server process and is currently connected.
*
*
* -
*
* COMPLETED -- The player connection has been dropped.
*
*
* -
*
* TIMEDOUT -- A player session request was received, but the player did not connect and/or was not validated
* within the timeout limit (60 seconds).
*
*
*
*
* @param status
* Current status of the player session.
*
* Possible player session statuses include the following:
*
*
* -
*
* RESERVED -- The player session request has been received, but the player has not yet connected to
* the server process and/or been validated.
*
*
* -
*
* ACTIVE -- The player has been validated by the server process and is currently connected.
*
*
* -
*
* COMPLETED -- The player connection has been dropped.
*
*
* -
*
* TIMEDOUT -- A player session request was received, but the player did not connect and/or was not
* validated within the timeout limit (60 seconds).
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see PlayerSessionStatus
*/
public PlayerSession withStatus(String status) {
setStatus(status);
return this;
}
/**
*
* Current status of the player session.
*
*
* Possible player session statuses include the following:
*
*
* -
*
* RESERVED -- The player session request has been received, but the player has not yet connected to the
* server process and/or been validated.
*
*
* -
*
* ACTIVE -- The player has been validated by the server process and is currently connected.
*
*
* -
*
* COMPLETED -- The player connection has been dropped.
*
*
* -
*
* TIMEDOUT -- A player session request was received, but the player did not connect and/or was not validated
* within the timeout limit (60 seconds).
*
*
*
*
* @param status
* Current status of the player session.
*
* Possible player session statuses include the following:
*
*
* -
*
* RESERVED -- The player session request has been received, but the player has not yet connected to
* the server process and/or been validated.
*
*
* -
*
* ACTIVE -- The player has been validated by the server process and is currently connected.
*
*
* -
*
* COMPLETED -- The player connection has been dropped.
*
*
* -
*
* TIMEDOUT -- A player session request was received, but the player did not connect and/or was not
* validated within the timeout limit (60 seconds).
*
*
* @see PlayerSessionStatus
*/
public void setStatus(PlayerSessionStatus status) {
withStatus(status);
}
/**
*
* Current status of the player session.
*
*
* Possible player session statuses include the following:
*
*
* -
*
* RESERVED -- The player session request has been received, but the player has not yet connected to the
* server process and/or been validated.
*
*
* -
*
* ACTIVE -- The player has been validated by the server process and is currently connected.
*
*
* -
*
* COMPLETED -- The player connection has been dropped.
*
*
* -
*
* TIMEDOUT -- A player session request was received, but the player did not connect and/or was not validated
* within the timeout limit (60 seconds).
*
*
*
*
* @param status
* Current status of the player session.
*
* Possible player session statuses include the following:
*
*
* -
*
* RESERVED -- The player session request has been received, but the player has not yet connected to
* the server process and/or been validated.
*
*
* -
*
* ACTIVE -- The player has been validated by the server process and is currently connected.
*
*
* -
*
* COMPLETED -- The player connection has been dropped.
*
*
* -
*
* TIMEDOUT -- A player session request was received, but the player did not connect and/or was not
* validated within the timeout limit (60 seconds).
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see PlayerSessionStatus
*/
public PlayerSession withStatus(PlayerSessionStatus status) {
this.status = status.toString();
return this;
}
/**
*
* The IP address of the game session. To connect to a GameLift game server, an app needs both the IP address and
* port number.
*
*
* @param ipAddress
* The IP address of the game session. To connect to a GameLift game server, an app needs both the IP address
* and port number.
*/
public void setIpAddress(String ipAddress) {
this.ipAddress = ipAddress;
}
/**
*
* The IP address of the game session. To connect to a GameLift game server, an app needs both the IP address and
* port number.
*
*
* @return The IP address of the game session. To connect to a GameLift game server, an app needs both the IP
* address and port number.
*/
public String getIpAddress() {
return this.ipAddress;
}
/**
*
* The IP address of the game session. To connect to a GameLift game server, an app needs both the IP address and
* port number.
*
*
* @param ipAddress
* The IP address of the game session. To connect to a GameLift game server, an app needs both the IP address
* and port number.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PlayerSession withIpAddress(String ipAddress) {
setIpAddress(ipAddress);
return this;
}
/**
*
* The DNS identifier assigned to the instance that is running the game session. Values have the following format:
*
*
* -
*
* TLS-enabled fleets: <unique identifier>.<region identifier>.amazongamelift.com
.
*
*
* -
*
* Non-TLS-enabled fleets: ec2-<unique identifier>.compute.amazonaws.com
. (See Amazon EC2 Instance IP Addressing.)
*
*
*
*
* When connecting to a game session that is running on a TLS-enabled fleet, you must use the DNS name, not the IP
* address.
*
*
* @param dnsName
* The DNS identifier assigned to the instance that is running the game session. Values have the following
* format:
*
* -
*
* TLS-enabled fleets: <unique identifier>.<region identifier>.amazongamelift.com
.
*
*
* -
*
* Non-TLS-enabled fleets: ec2-<unique identifier>.compute.amazonaws.com
. (See Amazon EC2 Instance IP Addressing.)
*
*
*
*
* When connecting to a game session that is running on a TLS-enabled fleet, you must use the DNS name, not
* the IP address.
*/
public void setDnsName(String dnsName) {
this.dnsName = dnsName;
}
/**
*
* The DNS identifier assigned to the instance that is running the game session. Values have the following format:
*
*
* -
*
* TLS-enabled fleets: <unique identifier>.<region identifier>.amazongamelift.com
.
*
*
* -
*
* Non-TLS-enabled fleets: ec2-<unique identifier>.compute.amazonaws.com
. (See Amazon EC2 Instance IP Addressing.)
*
*
*
*
* When connecting to a game session that is running on a TLS-enabled fleet, you must use the DNS name, not the IP
* address.
*
*
* @return The DNS identifier assigned to the instance that is running the game session. Values have the following
* format:
*
* -
*
* TLS-enabled fleets: <unique identifier>.<region identifier>.amazongamelift.com
.
*
*
* -
*
* Non-TLS-enabled fleets: ec2-<unique identifier>.compute.amazonaws.com
. (See Amazon EC2 Instance IP Addressing.)
*
*
*
*
* When connecting to a game session that is running on a TLS-enabled fleet, you must use the DNS name, not
* the IP address.
*/
public String getDnsName() {
return this.dnsName;
}
/**
*
* The DNS identifier assigned to the instance that is running the game session. Values have the following format:
*
*
* -
*
* TLS-enabled fleets: <unique identifier>.<region identifier>.amazongamelift.com
.
*
*
* -
*
* Non-TLS-enabled fleets: ec2-<unique identifier>.compute.amazonaws.com
. (See Amazon EC2 Instance IP Addressing.)
*
*
*
*
* When connecting to a game session that is running on a TLS-enabled fleet, you must use the DNS name, not the IP
* address.
*
*
* @param dnsName
* The DNS identifier assigned to the instance that is running the game session. Values have the following
* format:
*
* -
*
* TLS-enabled fleets: <unique identifier>.<region identifier>.amazongamelift.com
.
*
*
* -
*
* Non-TLS-enabled fleets: ec2-<unique identifier>.compute.amazonaws.com
. (See Amazon EC2 Instance IP Addressing.)
*
*
*
*
* When connecting to a game session that is running on a TLS-enabled fleet, you must use the DNS name, not
* the IP address.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PlayerSession withDnsName(String dnsName) {
setDnsName(dnsName);
return this;
}
/**
*
* Port number for the game session. To connect to a Amazon Web Services server process, an app needs both the IP
* address and port number.
*
*
* @param port
* Port number for the game session. To connect to a Amazon Web Services server process, an app needs both
* the IP address and port number.
*/
public void setPort(Integer port) {
this.port = port;
}
/**
*
* Port number for the game session. To connect to a Amazon Web Services server process, an app needs both the IP
* address and port number.
*
*
* @return Port number for the game session. To connect to a Amazon Web Services server process, an app needs both
* the IP address and port number.
*/
public Integer getPort() {
return this.port;
}
/**
*
* Port number for the game session. To connect to a Amazon Web Services server process, an app needs both the IP
* address and port number.
*
*
* @param port
* Port number for the game session. To connect to a Amazon Web Services server process, an app needs both
* the IP address and port number.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PlayerSession withPort(Integer port) {
setPort(port);
return this;
}
/**
*
* Developer-defined information related to a player. GameLift does not use this data, so it can be formatted as
* needed for use in the game.
*
*
* @param playerData
* Developer-defined information related to a player. GameLift does not use this data, so it can be formatted
* as needed for use in the game.
*/
public void setPlayerData(String playerData) {
this.playerData = playerData;
}
/**
*
* Developer-defined information related to a player. GameLift does not use this data, so it can be formatted as
* needed for use in the game.
*
*
* @return Developer-defined information related to a player. GameLift does not use this data, so it can be
* formatted as needed for use in the game.
*/
public String getPlayerData() {
return this.playerData;
}
/**
*
* Developer-defined information related to a player. GameLift does not use this data, so it can be formatted as
* needed for use in the game.
*
*
* @param playerData
* Developer-defined information related to a player. GameLift does not use this data, so it can be formatted
* as needed for use in the game.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PlayerSession withPlayerData(String playerData) {
setPlayerData(playerData);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getPlayerSessionId() != null)
sb.append("PlayerSessionId: ").append(getPlayerSessionId()).append(",");
if (getPlayerId() != null)
sb.append("PlayerId: ").append(getPlayerId()).append(",");
if (getGameSessionId() != null)
sb.append("GameSessionId: ").append(getGameSessionId()).append(",");
if (getFleetId() != null)
sb.append("FleetId: ").append(getFleetId()).append(",");
if (getFleetArn() != null)
sb.append("FleetArn: ").append(getFleetArn()).append(",");
if (getCreationTime() != null)
sb.append("CreationTime: ").append(getCreationTime()).append(",");
if (getTerminationTime() != null)
sb.append("TerminationTime: ").append(getTerminationTime()).append(",");
if (getStatus() != null)
sb.append("Status: ").append(getStatus()).append(",");
if (getIpAddress() != null)
sb.append("IpAddress: ").append(getIpAddress()).append(",");
if (getDnsName() != null)
sb.append("DnsName: ").append(getDnsName()).append(",");
if (getPort() != null)
sb.append("Port: ").append(getPort()).append(",");
if (getPlayerData() != null)
sb.append("PlayerData: ").append(getPlayerData());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof PlayerSession == false)
return false;
PlayerSession other = (PlayerSession) obj;
if (other.getPlayerSessionId() == null ^ this.getPlayerSessionId() == null)
return false;
if (other.getPlayerSessionId() != null && other.getPlayerSessionId().equals(this.getPlayerSessionId()) == false)
return false;
if (other.getPlayerId() == null ^ this.getPlayerId() == null)
return false;
if (other.getPlayerId() != null && other.getPlayerId().equals(this.getPlayerId()) == false)
return false;
if (other.getGameSessionId() == null ^ this.getGameSessionId() == null)
return false;
if (other.getGameSessionId() != null && other.getGameSessionId().equals(this.getGameSessionId()) == false)
return false;
if (other.getFleetId() == null ^ this.getFleetId() == null)
return false;
if (other.getFleetId() != null && other.getFleetId().equals(this.getFleetId()) == false)
return false;
if (other.getFleetArn() == null ^ this.getFleetArn() == null)
return false;
if (other.getFleetArn() != null && other.getFleetArn().equals(this.getFleetArn()) == false)
return false;
if (other.getCreationTime() == null ^ this.getCreationTime() == null)
return false;
if (other.getCreationTime() != null && other.getCreationTime().equals(this.getCreationTime()) == false)
return false;
if (other.getTerminationTime() == null ^ this.getTerminationTime() == null)
return false;
if (other.getTerminationTime() != null && other.getTerminationTime().equals(this.getTerminationTime()) == false)
return false;
if (other.getStatus() == null ^ this.getStatus() == null)
return false;
if (other.getStatus() != null && other.getStatus().equals(this.getStatus()) == false)
return false;
if (other.getIpAddress() == null ^ this.getIpAddress() == null)
return false;
if (other.getIpAddress() != null && other.getIpAddress().equals(this.getIpAddress()) == false)
return false;
if (other.getDnsName() == null ^ this.getDnsName() == null)
return false;
if (other.getDnsName() != null && other.getDnsName().equals(this.getDnsName()) == false)
return false;
if (other.getPort() == null ^ this.getPort() == null)
return false;
if (other.getPort() != null && other.getPort().equals(this.getPort()) == false)
return false;
if (other.getPlayerData() == null ^ this.getPlayerData() == null)
return false;
if (other.getPlayerData() != null && other.getPlayerData().equals(this.getPlayerData()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getPlayerSessionId() == null) ? 0 : getPlayerSessionId().hashCode());
hashCode = prime * hashCode + ((getPlayerId() == null) ? 0 : getPlayerId().hashCode());
hashCode = prime * hashCode + ((getGameSessionId() == null) ? 0 : getGameSessionId().hashCode());
hashCode = prime * hashCode + ((getFleetId() == null) ? 0 : getFleetId().hashCode());
hashCode = prime * hashCode + ((getFleetArn() == null) ? 0 : getFleetArn().hashCode());
hashCode = prime * hashCode + ((getCreationTime() == null) ? 0 : getCreationTime().hashCode());
hashCode = prime * hashCode + ((getTerminationTime() == null) ? 0 : getTerminationTime().hashCode());
hashCode = prime * hashCode + ((getStatus() == null) ? 0 : getStatus().hashCode());
hashCode = prime * hashCode + ((getIpAddress() == null) ? 0 : getIpAddress().hashCode());
hashCode = prime * hashCode + ((getDnsName() == null) ? 0 : getDnsName().hashCode());
hashCode = prime * hashCode + ((getPort() == null) ? 0 : getPort().hashCode());
hashCode = prime * hashCode + ((getPlayerData() == null) ? 0 : getPlayerData().hashCode());
return hashCode;
}
@Override
public PlayerSession clone() {
try {
return (PlayerSession) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.gamelift.model.transform.PlayerSessionMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}