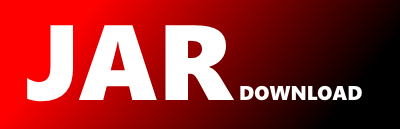
com.amazonaws.services.gamelift.model.GameSession Maven / Gradle / Ivy
Show all versions of aws-java-sdk-gamelift Show documentation
/*
* Copyright 2017-2022 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.gamelift.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* Properties describing a game session.
*
*
* A game session in ACTIVE status can host players. When a game session ends, its status is set to
* TERMINATED
.
*
*
* Once the session ends, the game session object is retained for 30 days. This means you can reuse idempotency token
* values after this time. Game session logs are retained for 14 days.
*
*
* Related actions
*
*
* CreateGameSession | DescribeGameSessions | DescribeGameSessionDetails |
* SearchGameSessions | UpdateGameSession | GetGameSessionLogUrl | StartGameSessionPlacement
* | DescribeGameSessionPlacement | StopGameSessionPlacement | All APIs by task
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class GameSession implements Serializable, Cloneable, StructuredPojo {
/**
*
* A unique identifier for the game session. A game session ARN has the following format:
* arn:aws:gamelift:<region>::gamesession/<fleet ID>/<custom ID string or idempotency token>
* .
*
*/
private String gameSessionId;
/**
*
* A descriptive label that is associated with a game session. Session names do not need to be unique.
*
*/
private String name;
/**
*
* A unique identifier for the fleet that the game session is running on.
*
*/
private String fleetId;
/**
*
* The Amazon Resource Name (ARN)
* associated with the GameLift fleet that this game session is running on.
*
*/
private String fleetArn;
/**
*
* A time stamp indicating when this data object was created. Format is a number expressed in Unix time as
* milliseconds (for example "1469498468.057"
).
*
*/
private java.util.Date creationTime;
/**
*
* A time stamp indicating when this data object was terminated. Format is a number expressed in Unix time as
* milliseconds (for example "1469498468.057"
).
*
*/
private java.util.Date terminationTime;
/**
*
* Number of players currently in the game session.
*
*/
private Integer currentPlayerSessionCount;
/**
*
* The maximum number of players that can be connected simultaneously to the game session.
*
*/
private Integer maximumPlayerSessionCount;
/**
*
* Current status of the game session. A game session must have an ACTIVE
status to have player
* sessions.
*
*/
private String status;
/**
*
* Provides additional information about game session status. INTERRUPTED
indicates that the game
* session was hosted on a spot instance that was reclaimed, causing the active game session to be terminated.
*
*/
private String statusReason;
/**
*
* A set of custom properties for a game session, formatted as key:value pairs. These properties are passed to a
* game server process in the GameSession object with a request to start a new game session (see Start a Game Session). You can search for active game sessions based on this custom data with
* SearchGameSessions.
*
*/
private java.util.List gameProperties;
/**
*
* The IP address of the game session. To connect to a GameLift game server, an app needs both the IP address and
* port number.
*
*/
private String ipAddress;
/**
*
* The DNS identifier assigned to the instance that is running the game session. Values have the following format:
*
*
* -
*
* TLS-enabled fleets: <unique identifier>.<region identifier>.amazongamelift.com
.
*
*
* -
*
* Non-TLS-enabled fleets: ec2-<unique identifier>.compute.amazonaws.com
. (See Amazon EC2 Instance IP Addressing.)
*
*
*
*
* When connecting to a game session that is running on a TLS-enabled fleet, you must use the DNS name, not the IP
* address.
*
*/
private String dnsName;
/**
*
* The port number for the game session. To connect to a GameLift game server, an app needs both the IP address and
* port number.
*
*/
private Integer port;
/**
*
* Indicates whether or not the game session is accepting new players.
*
*/
private String playerSessionCreationPolicy;
/**
*
* A unique identifier for a player. This ID is used to enforce a resource protection policy (if one exists), that
* limits the number of game sessions a player can create.
*
*/
private String creatorId;
/**
*
* A set of custom game session properties, formatted as a single string value. This data is passed to a game server
* process in the GameSession object with a request to start a new game session (see Start a Game Session).
*
*/
private String gameSessionData;
/**
*
* Information about the matchmaking process that was used to create the game session. It is in JSON syntax,
* formatted as a string. In addition the matchmaking configuration used, it contains data on all players assigned
* to the match, including player attributes and team assignments. For more details on matchmaker data, see Match
* Data. Matchmaker data is useful when requesting match backfills, and is updated whenever new players are
* added during a successful backfill (see StartMatchBackfill).
*
*/
private String matchmakerData;
/**
*
* The fleet location where the game session is running. This value might specify the fleet's home Region or a
* remote location. Location is expressed as an Amazon Web Services Region code such as us-west-2
.
*
*/
private String location;
/**
*
* A unique identifier for the game session. A game session ARN has the following format:
* arn:aws:gamelift:<region>::gamesession/<fleet ID>/<custom ID string or idempotency token>
* .
*
*
* @param gameSessionId
* A unique identifier for the game session. A game session ARN has the following format:
* arn:aws:gamelift:<region>::gamesession/<fleet ID>/<custom ID string or idempotency token>
* .
*/
public void setGameSessionId(String gameSessionId) {
this.gameSessionId = gameSessionId;
}
/**
*
* A unique identifier for the game session. A game session ARN has the following format:
* arn:aws:gamelift:<region>::gamesession/<fleet ID>/<custom ID string or idempotency token>
* .
*
*
* @return A unique identifier for the game session. A game session ARN has the following format:
* arn:aws:gamelift:<region>::gamesession/<fleet ID>/<custom ID string or idempotency token>
* .
*/
public String getGameSessionId() {
return this.gameSessionId;
}
/**
*
* A unique identifier for the game session. A game session ARN has the following format:
* arn:aws:gamelift:<region>::gamesession/<fleet ID>/<custom ID string or idempotency token>
* .
*
*
* @param gameSessionId
* A unique identifier for the game session. A game session ARN has the following format:
* arn:aws:gamelift:<region>::gamesession/<fleet ID>/<custom ID string or idempotency token>
* .
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GameSession withGameSessionId(String gameSessionId) {
setGameSessionId(gameSessionId);
return this;
}
/**
*
* A descriptive label that is associated with a game session. Session names do not need to be unique.
*
*
* @param name
* A descriptive label that is associated with a game session. Session names do not need to be unique.
*/
public void setName(String name) {
this.name = name;
}
/**
*
* A descriptive label that is associated with a game session. Session names do not need to be unique.
*
*
* @return A descriptive label that is associated with a game session. Session names do not need to be unique.
*/
public String getName() {
return this.name;
}
/**
*
* A descriptive label that is associated with a game session. Session names do not need to be unique.
*
*
* @param name
* A descriptive label that is associated with a game session. Session names do not need to be unique.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GameSession withName(String name) {
setName(name);
return this;
}
/**
*
* A unique identifier for the fleet that the game session is running on.
*
*
* @param fleetId
* A unique identifier for the fleet that the game session is running on.
*/
public void setFleetId(String fleetId) {
this.fleetId = fleetId;
}
/**
*
* A unique identifier for the fleet that the game session is running on.
*
*
* @return A unique identifier for the fleet that the game session is running on.
*/
public String getFleetId() {
return this.fleetId;
}
/**
*
* A unique identifier for the fleet that the game session is running on.
*
*
* @param fleetId
* A unique identifier for the fleet that the game session is running on.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GameSession withFleetId(String fleetId) {
setFleetId(fleetId);
return this;
}
/**
*
* The Amazon Resource Name (ARN)
* associated with the GameLift fleet that this game session is running on.
*
*
* @param fleetArn
* The Amazon Resource Name (ARN) associated with the
* GameLift fleet that this game session is running on.
*/
public void setFleetArn(String fleetArn) {
this.fleetArn = fleetArn;
}
/**
*
* The Amazon Resource Name (ARN)
* associated with the GameLift fleet that this game session is running on.
*
*
* @return The Amazon Resource Name (ARN) associated with the
* GameLift fleet that this game session is running on.
*/
public String getFleetArn() {
return this.fleetArn;
}
/**
*
* The Amazon Resource Name (ARN)
* associated with the GameLift fleet that this game session is running on.
*
*
* @param fleetArn
* The Amazon Resource Name (ARN) associated with the
* GameLift fleet that this game session is running on.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GameSession withFleetArn(String fleetArn) {
setFleetArn(fleetArn);
return this;
}
/**
*
* A time stamp indicating when this data object was created. Format is a number expressed in Unix time as
* milliseconds (for example "1469498468.057"
).
*
*
* @param creationTime
* A time stamp indicating when this data object was created. Format is a number expressed in Unix time as
* milliseconds (for example "1469498468.057"
).
*/
public void setCreationTime(java.util.Date creationTime) {
this.creationTime = creationTime;
}
/**
*
* A time stamp indicating when this data object was created. Format is a number expressed in Unix time as
* milliseconds (for example "1469498468.057"
).
*
*
* @return A time stamp indicating when this data object was created. Format is a number expressed in Unix time as
* milliseconds (for example "1469498468.057"
).
*/
public java.util.Date getCreationTime() {
return this.creationTime;
}
/**
*
* A time stamp indicating when this data object was created. Format is a number expressed in Unix time as
* milliseconds (for example "1469498468.057"
).
*
*
* @param creationTime
* A time stamp indicating when this data object was created. Format is a number expressed in Unix time as
* milliseconds (for example "1469498468.057"
).
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GameSession withCreationTime(java.util.Date creationTime) {
setCreationTime(creationTime);
return this;
}
/**
*
* A time stamp indicating when this data object was terminated. Format is a number expressed in Unix time as
* milliseconds (for example "1469498468.057"
).
*
*
* @param terminationTime
* A time stamp indicating when this data object was terminated. Format is a number expressed in Unix time as
* milliseconds (for example "1469498468.057"
).
*/
public void setTerminationTime(java.util.Date terminationTime) {
this.terminationTime = terminationTime;
}
/**
*
* A time stamp indicating when this data object was terminated. Format is a number expressed in Unix time as
* milliseconds (for example "1469498468.057"
).
*
*
* @return A time stamp indicating when this data object was terminated. Format is a number expressed in Unix time
* as milliseconds (for example "1469498468.057"
).
*/
public java.util.Date getTerminationTime() {
return this.terminationTime;
}
/**
*
* A time stamp indicating when this data object was terminated. Format is a number expressed in Unix time as
* milliseconds (for example "1469498468.057"
).
*
*
* @param terminationTime
* A time stamp indicating when this data object was terminated. Format is a number expressed in Unix time as
* milliseconds (for example "1469498468.057"
).
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GameSession withTerminationTime(java.util.Date terminationTime) {
setTerminationTime(terminationTime);
return this;
}
/**
*
* Number of players currently in the game session.
*
*
* @param currentPlayerSessionCount
* Number of players currently in the game session.
*/
public void setCurrentPlayerSessionCount(Integer currentPlayerSessionCount) {
this.currentPlayerSessionCount = currentPlayerSessionCount;
}
/**
*
* Number of players currently in the game session.
*
*
* @return Number of players currently in the game session.
*/
public Integer getCurrentPlayerSessionCount() {
return this.currentPlayerSessionCount;
}
/**
*
* Number of players currently in the game session.
*
*
* @param currentPlayerSessionCount
* Number of players currently in the game session.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GameSession withCurrentPlayerSessionCount(Integer currentPlayerSessionCount) {
setCurrentPlayerSessionCount(currentPlayerSessionCount);
return this;
}
/**
*
* The maximum number of players that can be connected simultaneously to the game session.
*
*
* @param maximumPlayerSessionCount
* The maximum number of players that can be connected simultaneously to the game session.
*/
public void setMaximumPlayerSessionCount(Integer maximumPlayerSessionCount) {
this.maximumPlayerSessionCount = maximumPlayerSessionCount;
}
/**
*
* The maximum number of players that can be connected simultaneously to the game session.
*
*
* @return The maximum number of players that can be connected simultaneously to the game session.
*/
public Integer getMaximumPlayerSessionCount() {
return this.maximumPlayerSessionCount;
}
/**
*
* The maximum number of players that can be connected simultaneously to the game session.
*
*
* @param maximumPlayerSessionCount
* The maximum number of players that can be connected simultaneously to the game session.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GameSession withMaximumPlayerSessionCount(Integer maximumPlayerSessionCount) {
setMaximumPlayerSessionCount(maximumPlayerSessionCount);
return this;
}
/**
*
* Current status of the game session. A game session must have an ACTIVE
status to have player
* sessions.
*
*
* @param status
* Current status of the game session. A game session must have an ACTIVE
status to have player
* sessions.
* @see GameSessionStatus
*/
public void setStatus(String status) {
this.status = status;
}
/**
*
* Current status of the game session. A game session must have an ACTIVE
status to have player
* sessions.
*
*
* @return Current status of the game session. A game session must have an ACTIVE
status to have player
* sessions.
* @see GameSessionStatus
*/
public String getStatus() {
return this.status;
}
/**
*
* Current status of the game session. A game session must have an ACTIVE
status to have player
* sessions.
*
*
* @param status
* Current status of the game session. A game session must have an ACTIVE
status to have player
* sessions.
* @return Returns a reference to this object so that method calls can be chained together.
* @see GameSessionStatus
*/
public GameSession withStatus(String status) {
setStatus(status);
return this;
}
/**
*
* Current status of the game session. A game session must have an ACTIVE
status to have player
* sessions.
*
*
* @param status
* Current status of the game session. A game session must have an ACTIVE
status to have player
* sessions.
* @see GameSessionStatus
*/
public void setStatus(GameSessionStatus status) {
withStatus(status);
}
/**
*
* Current status of the game session. A game session must have an ACTIVE
status to have player
* sessions.
*
*
* @param status
* Current status of the game session. A game session must have an ACTIVE
status to have player
* sessions.
* @return Returns a reference to this object so that method calls can be chained together.
* @see GameSessionStatus
*/
public GameSession withStatus(GameSessionStatus status) {
this.status = status.toString();
return this;
}
/**
*
* Provides additional information about game session status. INTERRUPTED
indicates that the game
* session was hosted on a spot instance that was reclaimed, causing the active game session to be terminated.
*
*
* @param statusReason
* Provides additional information about game session status. INTERRUPTED
indicates that the
* game session was hosted on a spot instance that was reclaimed, causing the active game session to be
* terminated.
* @see GameSessionStatusReason
*/
public void setStatusReason(String statusReason) {
this.statusReason = statusReason;
}
/**
*
* Provides additional information about game session status. INTERRUPTED
indicates that the game
* session was hosted on a spot instance that was reclaimed, causing the active game session to be terminated.
*
*
* @return Provides additional information about game session status. INTERRUPTED
indicates that the
* game session was hosted on a spot instance that was reclaimed, causing the active game session to be
* terminated.
* @see GameSessionStatusReason
*/
public String getStatusReason() {
return this.statusReason;
}
/**
*
* Provides additional information about game session status. INTERRUPTED
indicates that the game
* session was hosted on a spot instance that was reclaimed, causing the active game session to be terminated.
*
*
* @param statusReason
* Provides additional information about game session status. INTERRUPTED
indicates that the
* game session was hosted on a spot instance that was reclaimed, causing the active game session to be
* terminated.
* @return Returns a reference to this object so that method calls can be chained together.
* @see GameSessionStatusReason
*/
public GameSession withStatusReason(String statusReason) {
setStatusReason(statusReason);
return this;
}
/**
*
* Provides additional information about game session status. INTERRUPTED
indicates that the game
* session was hosted on a spot instance that was reclaimed, causing the active game session to be terminated.
*
*
* @param statusReason
* Provides additional information about game session status. INTERRUPTED
indicates that the
* game session was hosted on a spot instance that was reclaimed, causing the active game session to be
* terminated.
* @see GameSessionStatusReason
*/
public void setStatusReason(GameSessionStatusReason statusReason) {
withStatusReason(statusReason);
}
/**
*
* Provides additional information about game session status. INTERRUPTED
indicates that the game
* session was hosted on a spot instance that was reclaimed, causing the active game session to be terminated.
*
*
* @param statusReason
* Provides additional information about game session status. INTERRUPTED
indicates that the
* game session was hosted on a spot instance that was reclaimed, causing the active game session to be
* terminated.
* @return Returns a reference to this object so that method calls can be chained together.
* @see GameSessionStatusReason
*/
public GameSession withStatusReason(GameSessionStatusReason statusReason) {
this.statusReason = statusReason.toString();
return this;
}
/**
*
* A set of custom properties for a game session, formatted as key:value pairs. These properties are passed to a
* game server process in the GameSession object with a request to start a new game session (see Start a Game Session). You can search for active game sessions based on this custom data with
* SearchGameSessions.
*
*
* @return A set of custom properties for a game session, formatted as key:value pairs. These properties are passed
* to a game server process in the GameSession object with a request to start a new game session (see
* Start a Game Session). You can search for active game sessions based on this custom data with
* SearchGameSessions.
*/
public java.util.List getGameProperties() {
return gameProperties;
}
/**
*
* A set of custom properties for a game session, formatted as key:value pairs. These properties are passed to a
* game server process in the GameSession object with a request to start a new game session (see Start a Game Session). You can search for active game sessions based on this custom data with
* SearchGameSessions.
*
*
* @param gameProperties
* A set of custom properties for a game session, formatted as key:value pairs. These properties are passed
* to a game server process in the GameSession object with a request to start a new game session (see
* Start a Game Session). You can search for active game sessions based on this custom data with
* SearchGameSessions.
*/
public void setGameProperties(java.util.Collection gameProperties) {
if (gameProperties == null) {
this.gameProperties = null;
return;
}
this.gameProperties = new java.util.ArrayList(gameProperties);
}
/**
*
* A set of custom properties for a game session, formatted as key:value pairs. These properties are passed to a
* game server process in the GameSession object with a request to start a new game session (see Start a Game Session). You can search for active game sessions based on this custom data with
* SearchGameSessions.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setGameProperties(java.util.Collection)} or {@link #withGameProperties(java.util.Collection)} if you want
* to override the existing values.
*
*
* @param gameProperties
* A set of custom properties for a game session, formatted as key:value pairs. These properties are passed
* to a game server process in the GameSession object with a request to start a new game session (see
* Start a Game Session). You can search for active game sessions based on this custom data with
* SearchGameSessions.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GameSession withGameProperties(GameProperty... gameProperties) {
if (this.gameProperties == null) {
setGameProperties(new java.util.ArrayList(gameProperties.length));
}
for (GameProperty ele : gameProperties) {
this.gameProperties.add(ele);
}
return this;
}
/**
*
* A set of custom properties for a game session, formatted as key:value pairs. These properties are passed to a
* game server process in the GameSession object with a request to start a new game session (see Start a Game Session). You can search for active game sessions based on this custom data with
* SearchGameSessions.
*
*
* @param gameProperties
* A set of custom properties for a game session, formatted as key:value pairs. These properties are passed
* to a game server process in the GameSession object with a request to start a new game session (see
* Start a Game Session). You can search for active game sessions based on this custom data with
* SearchGameSessions.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GameSession withGameProperties(java.util.Collection gameProperties) {
setGameProperties(gameProperties);
return this;
}
/**
*
* The IP address of the game session. To connect to a GameLift game server, an app needs both the IP address and
* port number.
*
*
* @param ipAddress
* The IP address of the game session. To connect to a GameLift game server, an app needs both the IP address
* and port number.
*/
public void setIpAddress(String ipAddress) {
this.ipAddress = ipAddress;
}
/**
*
* The IP address of the game session. To connect to a GameLift game server, an app needs both the IP address and
* port number.
*
*
* @return The IP address of the game session. To connect to a GameLift game server, an app needs both the IP
* address and port number.
*/
public String getIpAddress() {
return this.ipAddress;
}
/**
*
* The IP address of the game session. To connect to a GameLift game server, an app needs both the IP address and
* port number.
*
*
* @param ipAddress
* The IP address of the game session. To connect to a GameLift game server, an app needs both the IP address
* and port number.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GameSession withIpAddress(String ipAddress) {
setIpAddress(ipAddress);
return this;
}
/**
*
* The DNS identifier assigned to the instance that is running the game session. Values have the following format:
*
*
* -
*
* TLS-enabled fleets: <unique identifier>.<region identifier>.amazongamelift.com
.
*
*
* -
*
* Non-TLS-enabled fleets: ec2-<unique identifier>.compute.amazonaws.com
. (See Amazon EC2 Instance IP Addressing.)
*
*
*
*
* When connecting to a game session that is running on a TLS-enabled fleet, you must use the DNS name, not the IP
* address.
*
*
* @param dnsName
* The DNS identifier assigned to the instance that is running the game session. Values have the following
* format:
*
* -
*
* TLS-enabled fleets: <unique identifier>.<region identifier>.amazongamelift.com
.
*
*
* -
*
* Non-TLS-enabled fleets: ec2-<unique identifier>.compute.amazonaws.com
. (See Amazon EC2 Instance IP Addressing.)
*
*
*
*
* When connecting to a game session that is running on a TLS-enabled fleet, you must use the DNS name, not
* the IP address.
*/
public void setDnsName(String dnsName) {
this.dnsName = dnsName;
}
/**
*
* The DNS identifier assigned to the instance that is running the game session. Values have the following format:
*
*
* -
*
* TLS-enabled fleets: <unique identifier>.<region identifier>.amazongamelift.com
.
*
*
* -
*
* Non-TLS-enabled fleets: ec2-<unique identifier>.compute.amazonaws.com
. (See Amazon EC2 Instance IP Addressing.)
*
*
*
*
* When connecting to a game session that is running on a TLS-enabled fleet, you must use the DNS name, not the IP
* address.
*
*
* @return The DNS identifier assigned to the instance that is running the game session. Values have the following
* format:
*
* -
*
* TLS-enabled fleets: <unique identifier>.<region identifier>.amazongamelift.com
.
*
*
* -
*
* Non-TLS-enabled fleets: ec2-<unique identifier>.compute.amazonaws.com
. (See Amazon EC2 Instance IP Addressing.)
*
*
*
*
* When connecting to a game session that is running on a TLS-enabled fleet, you must use the DNS name, not
* the IP address.
*/
public String getDnsName() {
return this.dnsName;
}
/**
*
* The DNS identifier assigned to the instance that is running the game session. Values have the following format:
*
*
* -
*
* TLS-enabled fleets: <unique identifier>.<region identifier>.amazongamelift.com
.
*
*
* -
*
* Non-TLS-enabled fleets: ec2-<unique identifier>.compute.amazonaws.com
. (See Amazon EC2 Instance IP Addressing.)
*
*
*
*
* When connecting to a game session that is running on a TLS-enabled fleet, you must use the DNS name, not the IP
* address.
*
*
* @param dnsName
* The DNS identifier assigned to the instance that is running the game session. Values have the following
* format:
*
* -
*
* TLS-enabled fleets: <unique identifier>.<region identifier>.amazongamelift.com
.
*
*
* -
*
* Non-TLS-enabled fleets: ec2-<unique identifier>.compute.amazonaws.com
. (See Amazon EC2 Instance IP Addressing.)
*
*
*
*
* When connecting to a game session that is running on a TLS-enabled fleet, you must use the DNS name, not
* the IP address.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GameSession withDnsName(String dnsName) {
setDnsName(dnsName);
return this;
}
/**
*
* The port number for the game session. To connect to a GameLift game server, an app needs both the IP address and
* port number.
*
*
* @param port
* The port number for the game session. To connect to a GameLift game server, an app needs both the IP
* address and port number.
*/
public void setPort(Integer port) {
this.port = port;
}
/**
*
* The port number for the game session. To connect to a GameLift game server, an app needs both the IP address and
* port number.
*
*
* @return The port number for the game session. To connect to a GameLift game server, an app needs both the IP
* address and port number.
*/
public Integer getPort() {
return this.port;
}
/**
*
* The port number for the game session. To connect to a GameLift game server, an app needs both the IP address and
* port number.
*
*
* @param port
* The port number for the game session. To connect to a GameLift game server, an app needs both the IP
* address and port number.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GameSession withPort(Integer port) {
setPort(port);
return this;
}
/**
*
* Indicates whether or not the game session is accepting new players.
*
*
* @param playerSessionCreationPolicy
* Indicates whether or not the game session is accepting new players.
* @see PlayerSessionCreationPolicy
*/
public void setPlayerSessionCreationPolicy(String playerSessionCreationPolicy) {
this.playerSessionCreationPolicy = playerSessionCreationPolicy;
}
/**
*
* Indicates whether or not the game session is accepting new players.
*
*
* @return Indicates whether or not the game session is accepting new players.
* @see PlayerSessionCreationPolicy
*/
public String getPlayerSessionCreationPolicy() {
return this.playerSessionCreationPolicy;
}
/**
*
* Indicates whether or not the game session is accepting new players.
*
*
* @param playerSessionCreationPolicy
* Indicates whether or not the game session is accepting new players.
* @return Returns a reference to this object so that method calls can be chained together.
* @see PlayerSessionCreationPolicy
*/
public GameSession withPlayerSessionCreationPolicy(String playerSessionCreationPolicy) {
setPlayerSessionCreationPolicy(playerSessionCreationPolicy);
return this;
}
/**
*
* Indicates whether or not the game session is accepting new players.
*
*
* @param playerSessionCreationPolicy
* Indicates whether or not the game session is accepting new players.
* @see PlayerSessionCreationPolicy
*/
public void setPlayerSessionCreationPolicy(PlayerSessionCreationPolicy playerSessionCreationPolicy) {
withPlayerSessionCreationPolicy(playerSessionCreationPolicy);
}
/**
*
* Indicates whether or not the game session is accepting new players.
*
*
* @param playerSessionCreationPolicy
* Indicates whether or not the game session is accepting new players.
* @return Returns a reference to this object so that method calls can be chained together.
* @see PlayerSessionCreationPolicy
*/
public GameSession withPlayerSessionCreationPolicy(PlayerSessionCreationPolicy playerSessionCreationPolicy) {
this.playerSessionCreationPolicy = playerSessionCreationPolicy.toString();
return this;
}
/**
*
* A unique identifier for a player. This ID is used to enforce a resource protection policy (if one exists), that
* limits the number of game sessions a player can create.
*
*
* @param creatorId
* A unique identifier for a player. This ID is used to enforce a resource protection policy (if one exists),
* that limits the number of game sessions a player can create.
*/
public void setCreatorId(String creatorId) {
this.creatorId = creatorId;
}
/**
*
* A unique identifier for a player. This ID is used to enforce a resource protection policy (if one exists), that
* limits the number of game sessions a player can create.
*
*
* @return A unique identifier for a player. This ID is used to enforce a resource protection policy (if one
* exists), that limits the number of game sessions a player can create.
*/
public String getCreatorId() {
return this.creatorId;
}
/**
*
* A unique identifier for a player. This ID is used to enforce a resource protection policy (if one exists), that
* limits the number of game sessions a player can create.
*
*
* @param creatorId
* A unique identifier for a player. This ID is used to enforce a resource protection policy (if one exists),
* that limits the number of game sessions a player can create.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GameSession withCreatorId(String creatorId) {
setCreatorId(creatorId);
return this;
}
/**
*
* A set of custom game session properties, formatted as a single string value. This data is passed to a game server
* process in the GameSession object with a request to start a new game session (see Start a Game Session).
*
*
* @param gameSessionData
* A set of custom game session properties, formatted as a single string value. This data is passed to a game
* server process in the GameSession object with a request to start a new game session (see Start a Game Session).
*/
public void setGameSessionData(String gameSessionData) {
this.gameSessionData = gameSessionData;
}
/**
*
* A set of custom game session properties, formatted as a single string value. This data is passed to a game server
* process in the GameSession object with a request to start a new game session (see Start a Game Session).
*
*
* @return A set of custom game session properties, formatted as a single string value. This data is passed to a
* game server process in the GameSession object with a request to start a new game session (see Start a Game Session).
*/
public String getGameSessionData() {
return this.gameSessionData;
}
/**
*
* A set of custom game session properties, formatted as a single string value. This data is passed to a game server
* process in the GameSession object with a request to start a new game session (see Start a Game Session).
*
*
* @param gameSessionData
* A set of custom game session properties, formatted as a single string value. This data is passed to a game
* server process in the GameSession object with a request to start a new game session (see Start a Game Session).
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GameSession withGameSessionData(String gameSessionData) {
setGameSessionData(gameSessionData);
return this;
}
/**
*
* Information about the matchmaking process that was used to create the game session. It is in JSON syntax,
* formatted as a string. In addition the matchmaking configuration used, it contains data on all players assigned
* to the match, including player attributes and team assignments. For more details on matchmaker data, see Match
* Data. Matchmaker data is useful when requesting match backfills, and is updated whenever new players are
* added during a successful backfill (see StartMatchBackfill).
*
*
* @param matchmakerData
* Information about the matchmaking process that was used to create the game session. It is in JSON syntax,
* formatted as a string. In addition the matchmaking configuration used, it contains data on all players
* assigned to the match, including player attributes and team assignments. For more details on matchmaker
* data, see Match Data. Matchmaker data is useful when requesting match backfills, and is updated whenever new
* players are added during a successful backfill (see StartMatchBackfill).
*/
public void setMatchmakerData(String matchmakerData) {
this.matchmakerData = matchmakerData;
}
/**
*
* Information about the matchmaking process that was used to create the game session. It is in JSON syntax,
* formatted as a string. In addition the matchmaking configuration used, it contains data on all players assigned
* to the match, including player attributes and team assignments. For more details on matchmaker data, see Match
* Data. Matchmaker data is useful when requesting match backfills, and is updated whenever new players are
* added during a successful backfill (see StartMatchBackfill).
*
*
* @return Information about the matchmaking process that was used to create the game session. It is in JSON syntax,
* formatted as a string. In addition the matchmaking configuration used, it contains data on all players
* assigned to the match, including player attributes and team assignments. For more details on matchmaker
* data, see Match Data. Matchmaker data is useful when requesting match backfills, and is updated whenever new
* players are added during a successful backfill (see StartMatchBackfill).
*/
public String getMatchmakerData() {
return this.matchmakerData;
}
/**
*
* Information about the matchmaking process that was used to create the game session. It is in JSON syntax,
* formatted as a string. In addition the matchmaking configuration used, it contains data on all players assigned
* to the match, including player attributes and team assignments. For more details on matchmaker data, see Match
* Data. Matchmaker data is useful when requesting match backfills, and is updated whenever new players are
* added during a successful backfill (see StartMatchBackfill).
*
*
* @param matchmakerData
* Information about the matchmaking process that was used to create the game session. It is in JSON syntax,
* formatted as a string. In addition the matchmaking configuration used, it contains data on all players
* assigned to the match, including player attributes and team assignments. For more details on matchmaker
* data, see Match Data. Matchmaker data is useful when requesting match backfills, and is updated whenever new
* players are added during a successful backfill (see StartMatchBackfill).
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GameSession withMatchmakerData(String matchmakerData) {
setMatchmakerData(matchmakerData);
return this;
}
/**
*
* The fleet location where the game session is running. This value might specify the fleet's home Region or a
* remote location. Location is expressed as an Amazon Web Services Region code such as us-west-2
.
*
*
* @param location
* The fleet location where the game session is running. This value might specify the fleet's home Region or
* a remote location. Location is expressed as an Amazon Web Services Region code such as
* us-west-2
.
*/
public void setLocation(String location) {
this.location = location;
}
/**
*
* The fleet location where the game session is running. This value might specify the fleet's home Region or a
* remote location. Location is expressed as an Amazon Web Services Region code such as us-west-2
.
*
*
* @return The fleet location where the game session is running. This value might specify the fleet's home Region or
* a remote location. Location is expressed as an Amazon Web Services Region code such as
* us-west-2
.
*/
public String getLocation() {
return this.location;
}
/**
*
* The fleet location where the game session is running. This value might specify the fleet's home Region or a
* remote location. Location is expressed as an Amazon Web Services Region code such as us-west-2
.
*
*
* @param location
* The fleet location where the game session is running. This value might specify the fleet's home Region or
* a remote location. Location is expressed as an Amazon Web Services Region code such as
* us-west-2
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GameSession withLocation(String location) {
setLocation(location);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getGameSessionId() != null)
sb.append("GameSessionId: ").append(getGameSessionId()).append(",");
if (getName() != null)
sb.append("Name: ").append(getName()).append(",");
if (getFleetId() != null)
sb.append("FleetId: ").append(getFleetId()).append(",");
if (getFleetArn() != null)
sb.append("FleetArn: ").append(getFleetArn()).append(",");
if (getCreationTime() != null)
sb.append("CreationTime: ").append(getCreationTime()).append(",");
if (getTerminationTime() != null)
sb.append("TerminationTime: ").append(getTerminationTime()).append(",");
if (getCurrentPlayerSessionCount() != null)
sb.append("CurrentPlayerSessionCount: ").append(getCurrentPlayerSessionCount()).append(",");
if (getMaximumPlayerSessionCount() != null)
sb.append("MaximumPlayerSessionCount: ").append(getMaximumPlayerSessionCount()).append(",");
if (getStatus() != null)
sb.append("Status: ").append(getStatus()).append(",");
if (getStatusReason() != null)
sb.append("StatusReason: ").append(getStatusReason()).append(",");
if (getGameProperties() != null)
sb.append("GameProperties: ").append(getGameProperties()).append(",");
if (getIpAddress() != null)
sb.append("IpAddress: ").append(getIpAddress()).append(",");
if (getDnsName() != null)
sb.append("DnsName: ").append(getDnsName()).append(",");
if (getPort() != null)
sb.append("Port: ").append(getPort()).append(",");
if (getPlayerSessionCreationPolicy() != null)
sb.append("PlayerSessionCreationPolicy: ").append(getPlayerSessionCreationPolicy()).append(",");
if (getCreatorId() != null)
sb.append("CreatorId: ").append(getCreatorId()).append(",");
if (getGameSessionData() != null)
sb.append("GameSessionData: ").append(getGameSessionData()).append(",");
if (getMatchmakerData() != null)
sb.append("MatchmakerData: ").append(getMatchmakerData()).append(",");
if (getLocation() != null)
sb.append("Location: ").append(getLocation());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof GameSession == false)
return false;
GameSession other = (GameSession) obj;
if (other.getGameSessionId() == null ^ this.getGameSessionId() == null)
return false;
if (other.getGameSessionId() != null && other.getGameSessionId().equals(this.getGameSessionId()) == false)
return false;
if (other.getName() == null ^ this.getName() == null)
return false;
if (other.getName() != null && other.getName().equals(this.getName()) == false)
return false;
if (other.getFleetId() == null ^ this.getFleetId() == null)
return false;
if (other.getFleetId() != null && other.getFleetId().equals(this.getFleetId()) == false)
return false;
if (other.getFleetArn() == null ^ this.getFleetArn() == null)
return false;
if (other.getFleetArn() != null && other.getFleetArn().equals(this.getFleetArn()) == false)
return false;
if (other.getCreationTime() == null ^ this.getCreationTime() == null)
return false;
if (other.getCreationTime() != null && other.getCreationTime().equals(this.getCreationTime()) == false)
return false;
if (other.getTerminationTime() == null ^ this.getTerminationTime() == null)
return false;
if (other.getTerminationTime() != null && other.getTerminationTime().equals(this.getTerminationTime()) == false)
return false;
if (other.getCurrentPlayerSessionCount() == null ^ this.getCurrentPlayerSessionCount() == null)
return false;
if (other.getCurrentPlayerSessionCount() != null && other.getCurrentPlayerSessionCount().equals(this.getCurrentPlayerSessionCount()) == false)
return false;
if (other.getMaximumPlayerSessionCount() == null ^ this.getMaximumPlayerSessionCount() == null)
return false;
if (other.getMaximumPlayerSessionCount() != null && other.getMaximumPlayerSessionCount().equals(this.getMaximumPlayerSessionCount()) == false)
return false;
if (other.getStatus() == null ^ this.getStatus() == null)
return false;
if (other.getStatus() != null && other.getStatus().equals(this.getStatus()) == false)
return false;
if (other.getStatusReason() == null ^ this.getStatusReason() == null)
return false;
if (other.getStatusReason() != null && other.getStatusReason().equals(this.getStatusReason()) == false)
return false;
if (other.getGameProperties() == null ^ this.getGameProperties() == null)
return false;
if (other.getGameProperties() != null && other.getGameProperties().equals(this.getGameProperties()) == false)
return false;
if (other.getIpAddress() == null ^ this.getIpAddress() == null)
return false;
if (other.getIpAddress() != null && other.getIpAddress().equals(this.getIpAddress()) == false)
return false;
if (other.getDnsName() == null ^ this.getDnsName() == null)
return false;
if (other.getDnsName() != null && other.getDnsName().equals(this.getDnsName()) == false)
return false;
if (other.getPort() == null ^ this.getPort() == null)
return false;
if (other.getPort() != null && other.getPort().equals(this.getPort()) == false)
return false;
if (other.getPlayerSessionCreationPolicy() == null ^ this.getPlayerSessionCreationPolicy() == null)
return false;
if (other.getPlayerSessionCreationPolicy() != null && other.getPlayerSessionCreationPolicy().equals(this.getPlayerSessionCreationPolicy()) == false)
return false;
if (other.getCreatorId() == null ^ this.getCreatorId() == null)
return false;
if (other.getCreatorId() != null && other.getCreatorId().equals(this.getCreatorId()) == false)
return false;
if (other.getGameSessionData() == null ^ this.getGameSessionData() == null)
return false;
if (other.getGameSessionData() != null && other.getGameSessionData().equals(this.getGameSessionData()) == false)
return false;
if (other.getMatchmakerData() == null ^ this.getMatchmakerData() == null)
return false;
if (other.getMatchmakerData() != null && other.getMatchmakerData().equals(this.getMatchmakerData()) == false)
return false;
if (other.getLocation() == null ^ this.getLocation() == null)
return false;
if (other.getLocation() != null && other.getLocation().equals(this.getLocation()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getGameSessionId() == null) ? 0 : getGameSessionId().hashCode());
hashCode = prime * hashCode + ((getName() == null) ? 0 : getName().hashCode());
hashCode = prime * hashCode + ((getFleetId() == null) ? 0 : getFleetId().hashCode());
hashCode = prime * hashCode + ((getFleetArn() == null) ? 0 : getFleetArn().hashCode());
hashCode = prime * hashCode + ((getCreationTime() == null) ? 0 : getCreationTime().hashCode());
hashCode = prime * hashCode + ((getTerminationTime() == null) ? 0 : getTerminationTime().hashCode());
hashCode = prime * hashCode + ((getCurrentPlayerSessionCount() == null) ? 0 : getCurrentPlayerSessionCount().hashCode());
hashCode = prime * hashCode + ((getMaximumPlayerSessionCount() == null) ? 0 : getMaximumPlayerSessionCount().hashCode());
hashCode = prime * hashCode + ((getStatus() == null) ? 0 : getStatus().hashCode());
hashCode = prime * hashCode + ((getStatusReason() == null) ? 0 : getStatusReason().hashCode());
hashCode = prime * hashCode + ((getGameProperties() == null) ? 0 : getGameProperties().hashCode());
hashCode = prime * hashCode + ((getIpAddress() == null) ? 0 : getIpAddress().hashCode());
hashCode = prime * hashCode + ((getDnsName() == null) ? 0 : getDnsName().hashCode());
hashCode = prime * hashCode + ((getPort() == null) ? 0 : getPort().hashCode());
hashCode = prime * hashCode + ((getPlayerSessionCreationPolicy() == null) ? 0 : getPlayerSessionCreationPolicy().hashCode());
hashCode = prime * hashCode + ((getCreatorId() == null) ? 0 : getCreatorId().hashCode());
hashCode = prime * hashCode + ((getGameSessionData() == null) ? 0 : getGameSessionData().hashCode());
hashCode = prime * hashCode + ((getMatchmakerData() == null) ? 0 : getMatchmakerData().hashCode());
hashCode = prime * hashCode + ((getLocation() == null) ? 0 : getLocation().hashCode());
return hashCode;
}
@Override
public GameSession clone() {
try {
return (GameSession) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.gamelift.model.transform.GameSessionMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}