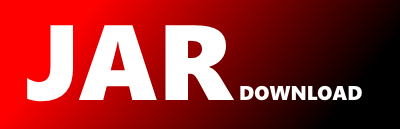
com.amazonaws.services.gamelift.model.MatchmakingTicket Maven / Gradle / Ivy
Show all versions of aws-java-sdk-gamelift Show documentation
/*
* Copyright 2017-2022 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.gamelift.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* Ticket generated to track the progress of a matchmaking request. Each ticket is uniquely identified by a ticket ID,
* supplied by the requester, when creating a matchmaking request with StartMatchmaking. Tickets can be retrieved
* by calling DescribeMatchmaking with the ticket ID.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class MatchmakingTicket implements Serializable, Cloneable, StructuredPojo {
/**
*
* A unique identifier for a matchmaking ticket.
*
*/
private String ticketId;
/**
*
* Name of the MatchmakingConfiguration that is used with this ticket. Matchmaking configurations determine
* how players are grouped into a match and how a new game session is created for the match.
*
*/
private String configurationName;
/**
*
* The Amazon Resource Name (ARN)
* associated with the GameLift matchmaking configuration resource that is used with this ticket.
*
*/
private String configurationArn;
/**
*
* Current status of the matchmaking request.
*
*
* -
*
* QUEUED -- The matchmaking request has been received and is currently waiting to be processed.
*
*
* -
*
* SEARCHING -- The matchmaking request is currently being processed.
*
*
* -
*
* REQUIRES_ACCEPTANCE -- A match has been proposed and the players must accept the match (see
* AcceptMatch). This status is used only with requests that use a matchmaking configuration with a player
* acceptance requirement.
*
*
* -
*
* PLACING -- The FlexMatch engine has matched players and is in the process of placing a new game session
* for the match.
*
*
* -
*
* COMPLETED -- Players have been matched and a game session is ready to host the players. A ticket in this
* state contains the necessary connection information for players.
*
*
* -
*
* FAILED -- The matchmaking request was not completed.
*
*
* -
*
* CANCELLED -- The matchmaking request was canceled. This may be the result of a call to
* StopMatchmaking or a proposed match that one or more players failed to accept.
*
*
* -
*
* TIMED_OUT -- The matchmaking request was not successful within the duration specified in the matchmaking
* configuration.
*
*
*
*
*
* Matchmaking requests that fail to successfully complete (statuses FAILED, CANCELLED, TIMED_OUT) can be
* resubmitted as new requests with new ticket IDs.
*
*
*/
private String status;
/**
*
* Code to explain the current status. For example, a status reason may indicate when a ticket has returned to
* SEARCHING
status after a proposed match fails to receive player acceptances.
*
*/
private String statusReason;
/**
*
* Additional information about the current status.
*
*/
private String statusMessage;
/**
*
* Time stamp indicating when this matchmaking request was received. Format is a number expressed in Unix time as
* milliseconds (for example "1469498468.057"
).
*
*/
private java.util.Date startTime;
/**
*
* Time stamp indicating when this matchmaking request stopped being processed due to success, failure, or
* cancellation. Format is a number expressed in Unix time as milliseconds (for example
* "1469498468.057"
).
*
*/
private java.util.Date endTime;
/**
*
* A set of Player
objects, each representing a player to find matches for. Players are identified by a
* unique player ID and may include latency data for use during matchmaking. If the ticket is in status
* COMPLETED
, the Player
objects include the team the players were assigned to in the
* resulting match.
*
*/
private java.util.List players;
/**
*
* Identifier and connection information of the game session created for the match. This information is added to the
* ticket only after the matchmaking request has been successfully completed. This parameter is not set when
* FlexMatch is being used without GameLift hosting.
*
*/
private GameSessionConnectionInfo gameSessionConnectionInfo;
/**
*
* Average amount of time (in seconds) that players are currently waiting for a match. If there is not enough recent
* data, this property may be empty.
*
*/
private Integer estimatedWaitTime;
/**
*
* A unique identifier for a matchmaking ticket.
*
*
* @param ticketId
* A unique identifier for a matchmaking ticket.
*/
public void setTicketId(String ticketId) {
this.ticketId = ticketId;
}
/**
*
* A unique identifier for a matchmaking ticket.
*
*
* @return A unique identifier for a matchmaking ticket.
*/
public String getTicketId() {
return this.ticketId;
}
/**
*
* A unique identifier for a matchmaking ticket.
*
*
* @param ticketId
* A unique identifier for a matchmaking ticket.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public MatchmakingTicket withTicketId(String ticketId) {
setTicketId(ticketId);
return this;
}
/**
*
* Name of the MatchmakingConfiguration that is used with this ticket. Matchmaking configurations determine
* how players are grouped into a match and how a new game session is created for the match.
*
*
* @param configurationName
* Name of the MatchmakingConfiguration that is used with this ticket. Matchmaking configurations
* determine how players are grouped into a match and how a new game session is created for the match.
*/
public void setConfigurationName(String configurationName) {
this.configurationName = configurationName;
}
/**
*
* Name of the MatchmakingConfiguration that is used with this ticket. Matchmaking configurations determine
* how players are grouped into a match and how a new game session is created for the match.
*
*
* @return Name of the MatchmakingConfiguration that is used with this ticket. Matchmaking configurations
* determine how players are grouped into a match and how a new game session is created for the match.
*/
public String getConfigurationName() {
return this.configurationName;
}
/**
*
* Name of the MatchmakingConfiguration that is used with this ticket. Matchmaking configurations determine
* how players are grouped into a match and how a new game session is created for the match.
*
*
* @param configurationName
* Name of the MatchmakingConfiguration that is used with this ticket. Matchmaking configurations
* determine how players are grouped into a match and how a new game session is created for the match.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public MatchmakingTicket withConfigurationName(String configurationName) {
setConfigurationName(configurationName);
return this;
}
/**
*
* The Amazon Resource Name (ARN)
* associated with the GameLift matchmaking configuration resource that is used with this ticket.
*
*
* @param configurationArn
* The Amazon Resource Name (ARN) associated with the
* GameLift matchmaking configuration resource that is used with this ticket.
*/
public void setConfigurationArn(String configurationArn) {
this.configurationArn = configurationArn;
}
/**
*
* The Amazon Resource Name (ARN)
* associated with the GameLift matchmaking configuration resource that is used with this ticket.
*
*
* @return The Amazon Resource Name (ARN) associated with the
* GameLift matchmaking configuration resource that is used with this ticket.
*/
public String getConfigurationArn() {
return this.configurationArn;
}
/**
*
* The Amazon Resource Name (ARN)
* associated with the GameLift matchmaking configuration resource that is used with this ticket.
*
*
* @param configurationArn
* The Amazon Resource Name (ARN) associated with the
* GameLift matchmaking configuration resource that is used with this ticket.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public MatchmakingTicket withConfigurationArn(String configurationArn) {
setConfigurationArn(configurationArn);
return this;
}
/**
*
* Current status of the matchmaking request.
*
*
* -
*
* QUEUED -- The matchmaking request has been received and is currently waiting to be processed.
*
*
* -
*
* SEARCHING -- The matchmaking request is currently being processed.
*
*
* -
*
* REQUIRES_ACCEPTANCE -- A match has been proposed and the players must accept the match (see
* AcceptMatch). This status is used only with requests that use a matchmaking configuration with a player
* acceptance requirement.
*
*
* -
*
* PLACING -- The FlexMatch engine has matched players and is in the process of placing a new game session
* for the match.
*
*
* -
*
* COMPLETED -- Players have been matched and a game session is ready to host the players. A ticket in this
* state contains the necessary connection information for players.
*
*
* -
*
* FAILED -- The matchmaking request was not completed.
*
*
* -
*
* CANCELLED -- The matchmaking request was canceled. This may be the result of a call to
* StopMatchmaking or a proposed match that one or more players failed to accept.
*
*
* -
*
* TIMED_OUT -- The matchmaking request was not successful within the duration specified in the matchmaking
* configuration.
*
*
*
*
*
* Matchmaking requests that fail to successfully complete (statuses FAILED, CANCELLED, TIMED_OUT) can be
* resubmitted as new requests with new ticket IDs.
*
*
*
* @param status
* Current status of the matchmaking request.
*
* -
*
* QUEUED -- The matchmaking request has been received and is currently waiting to be processed.
*
*
* -
*
* SEARCHING -- The matchmaking request is currently being processed.
*
*
* -
*
* REQUIRES_ACCEPTANCE -- A match has been proposed and the players must accept the match (see
* AcceptMatch). This status is used only with requests that use a matchmaking configuration with a
* player acceptance requirement.
*
*
* -
*
* PLACING -- The FlexMatch engine has matched players and is in the process of placing a new game
* session for the match.
*
*
* -
*
* COMPLETED -- Players have been matched and a game session is ready to host the players. A ticket in
* this state contains the necessary connection information for players.
*
*
* -
*
* FAILED -- The matchmaking request was not completed.
*
*
* -
*
* CANCELLED -- The matchmaking request was canceled. This may be the result of a call to
* StopMatchmaking or a proposed match that one or more players failed to accept.
*
*
* -
*
* TIMED_OUT -- The matchmaking request was not successful within the duration specified in the
* matchmaking configuration.
*
*
*
*
*
* Matchmaking requests that fail to successfully complete (statuses FAILED, CANCELLED, TIMED_OUT) can be
* resubmitted as new requests with new ticket IDs.
*
* @see MatchmakingConfigurationStatus
*/
public void setStatus(String status) {
this.status = status;
}
/**
*
* Current status of the matchmaking request.
*
*
* -
*
* QUEUED -- The matchmaking request has been received and is currently waiting to be processed.
*
*
* -
*
* SEARCHING -- The matchmaking request is currently being processed.
*
*
* -
*
* REQUIRES_ACCEPTANCE -- A match has been proposed and the players must accept the match (see
* AcceptMatch). This status is used only with requests that use a matchmaking configuration with a player
* acceptance requirement.
*
*
* -
*
* PLACING -- The FlexMatch engine has matched players and is in the process of placing a new game session
* for the match.
*
*
* -
*
* COMPLETED -- Players have been matched and a game session is ready to host the players. A ticket in this
* state contains the necessary connection information for players.
*
*
* -
*
* FAILED -- The matchmaking request was not completed.
*
*
* -
*
* CANCELLED -- The matchmaking request was canceled. This may be the result of a call to
* StopMatchmaking or a proposed match that one or more players failed to accept.
*
*
* -
*
* TIMED_OUT -- The matchmaking request was not successful within the duration specified in the matchmaking
* configuration.
*
*
*
*
*
* Matchmaking requests that fail to successfully complete (statuses FAILED, CANCELLED, TIMED_OUT) can be
* resubmitted as new requests with new ticket IDs.
*
*
*
* @return Current status of the matchmaking request.
*
* -
*
* QUEUED -- The matchmaking request has been received and is currently waiting to be processed.
*
*
* -
*
* SEARCHING -- The matchmaking request is currently being processed.
*
*
* -
*
* REQUIRES_ACCEPTANCE -- A match has been proposed and the players must accept the match (see
* AcceptMatch). This status is used only with requests that use a matchmaking configuration with a
* player acceptance requirement.
*
*
* -
*
* PLACING -- The FlexMatch engine has matched players and is in the process of placing a new game
* session for the match.
*
*
* -
*
* COMPLETED -- Players have been matched and a game session is ready to host the players. A ticket
* in this state contains the necessary connection information for players.
*
*
* -
*
* FAILED -- The matchmaking request was not completed.
*
*
* -
*
* CANCELLED -- The matchmaking request was canceled. This may be the result of a call to
* StopMatchmaking or a proposed match that one or more players failed to accept.
*
*
* -
*
* TIMED_OUT -- The matchmaking request was not successful within the duration specified in the
* matchmaking configuration.
*
*
*
*
*
* Matchmaking requests that fail to successfully complete (statuses FAILED, CANCELLED, TIMED_OUT) can be
* resubmitted as new requests with new ticket IDs.
*
* @see MatchmakingConfigurationStatus
*/
public String getStatus() {
return this.status;
}
/**
*
* Current status of the matchmaking request.
*
*
* -
*
* QUEUED -- The matchmaking request has been received and is currently waiting to be processed.
*
*
* -
*
* SEARCHING -- The matchmaking request is currently being processed.
*
*
* -
*
* REQUIRES_ACCEPTANCE -- A match has been proposed and the players must accept the match (see
* AcceptMatch). This status is used only with requests that use a matchmaking configuration with a player
* acceptance requirement.
*
*
* -
*
* PLACING -- The FlexMatch engine has matched players and is in the process of placing a new game session
* for the match.
*
*
* -
*
* COMPLETED -- Players have been matched and a game session is ready to host the players. A ticket in this
* state contains the necessary connection information for players.
*
*
* -
*
* FAILED -- The matchmaking request was not completed.
*
*
* -
*
* CANCELLED -- The matchmaking request was canceled. This may be the result of a call to
* StopMatchmaking or a proposed match that one or more players failed to accept.
*
*
* -
*
* TIMED_OUT -- The matchmaking request was not successful within the duration specified in the matchmaking
* configuration.
*
*
*
*
*
* Matchmaking requests that fail to successfully complete (statuses FAILED, CANCELLED, TIMED_OUT) can be
* resubmitted as new requests with new ticket IDs.
*
*
*
* @param status
* Current status of the matchmaking request.
*
* -
*
* QUEUED -- The matchmaking request has been received and is currently waiting to be processed.
*
*
* -
*
* SEARCHING -- The matchmaking request is currently being processed.
*
*
* -
*
* REQUIRES_ACCEPTANCE -- A match has been proposed and the players must accept the match (see
* AcceptMatch). This status is used only with requests that use a matchmaking configuration with a
* player acceptance requirement.
*
*
* -
*
* PLACING -- The FlexMatch engine has matched players and is in the process of placing a new game
* session for the match.
*
*
* -
*
* COMPLETED -- Players have been matched and a game session is ready to host the players. A ticket in
* this state contains the necessary connection information for players.
*
*
* -
*
* FAILED -- The matchmaking request was not completed.
*
*
* -
*
* CANCELLED -- The matchmaking request was canceled. This may be the result of a call to
* StopMatchmaking or a proposed match that one or more players failed to accept.
*
*
* -
*
* TIMED_OUT -- The matchmaking request was not successful within the duration specified in the
* matchmaking configuration.
*
*
*
*
*
* Matchmaking requests that fail to successfully complete (statuses FAILED, CANCELLED, TIMED_OUT) can be
* resubmitted as new requests with new ticket IDs.
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see MatchmakingConfigurationStatus
*/
public MatchmakingTicket withStatus(String status) {
setStatus(status);
return this;
}
/**
*
* Current status of the matchmaking request.
*
*
* -
*
* QUEUED -- The matchmaking request has been received and is currently waiting to be processed.
*
*
* -
*
* SEARCHING -- The matchmaking request is currently being processed.
*
*
* -
*
* REQUIRES_ACCEPTANCE -- A match has been proposed and the players must accept the match (see
* AcceptMatch). This status is used only with requests that use a matchmaking configuration with a player
* acceptance requirement.
*
*
* -
*
* PLACING -- The FlexMatch engine has matched players and is in the process of placing a new game session
* for the match.
*
*
* -
*
* COMPLETED -- Players have been matched and a game session is ready to host the players. A ticket in this
* state contains the necessary connection information for players.
*
*
* -
*
* FAILED -- The matchmaking request was not completed.
*
*
* -
*
* CANCELLED -- The matchmaking request was canceled. This may be the result of a call to
* StopMatchmaking or a proposed match that one or more players failed to accept.
*
*
* -
*
* TIMED_OUT -- The matchmaking request was not successful within the duration specified in the matchmaking
* configuration.
*
*
*
*
*
* Matchmaking requests that fail to successfully complete (statuses FAILED, CANCELLED, TIMED_OUT) can be
* resubmitted as new requests with new ticket IDs.
*
*
*
* @param status
* Current status of the matchmaking request.
*
* -
*
* QUEUED -- The matchmaking request has been received and is currently waiting to be processed.
*
*
* -
*
* SEARCHING -- The matchmaking request is currently being processed.
*
*
* -
*
* REQUIRES_ACCEPTANCE -- A match has been proposed and the players must accept the match (see
* AcceptMatch). This status is used only with requests that use a matchmaking configuration with a
* player acceptance requirement.
*
*
* -
*
* PLACING -- The FlexMatch engine has matched players and is in the process of placing a new game
* session for the match.
*
*
* -
*
* COMPLETED -- Players have been matched and a game session is ready to host the players. A ticket in
* this state contains the necessary connection information for players.
*
*
* -
*
* FAILED -- The matchmaking request was not completed.
*
*
* -
*
* CANCELLED -- The matchmaking request was canceled. This may be the result of a call to
* StopMatchmaking or a proposed match that one or more players failed to accept.
*
*
* -
*
* TIMED_OUT -- The matchmaking request was not successful within the duration specified in the
* matchmaking configuration.
*
*
*
*
*
* Matchmaking requests that fail to successfully complete (statuses FAILED, CANCELLED, TIMED_OUT) can be
* resubmitted as new requests with new ticket IDs.
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see MatchmakingConfigurationStatus
*/
public MatchmakingTicket withStatus(MatchmakingConfigurationStatus status) {
this.status = status.toString();
return this;
}
/**
*
* Code to explain the current status. For example, a status reason may indicate when a ticket has returned to
* SEARCHING
status after a proposed match fails to receive player acceptances.
*
*
* @param statusReason
* Code to explain the current status. For example, a status reason may indicate when a ticket has returned
* to SEARCHING
status after a proposed match fails to receive player acceptances.
*/
public void setStatusReason(String statusReason) {
this.statusReason = statusReason;
}
/**
*
* Code to explain the current status. For example, a status reason may indicate when a ticket has returned to
* SEARCHING
status after a proposed match fails to receive player acceptances.
*
*
* @return Code to explain the current status. For example, a status reason may indicate when a ticket has returned
* to SEARCHING
status after a proposed match fails to receive player acceptances.
*/
public String getStatusReason() {
return this.statusReason;
}
/**
*
* Code to explain the current status. For example, a status reason may indicate when a ticket has returned to
* SEARCHING
status after a proposed match fails to receive player acceptances.
*
*
* @param statusReason
* Code to explain the current status. For example, a status reason may indicate when a ticket has returned
* to SEARCHING
status after a proposed match fails to receive player acceptances.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public MatchmakingTicket withStatusReason(String statusReason) {
setStatusReason(statusReason);
return this;
}
/**
*
* Additional information about the current status.
*
*
* @param statusMessage
* Additional information about the current status.
*/
public void setStatusMessage(String statusMessage) {
this.statusMessage = statusMessage;
}
/**
*
* Additional information about the current status.
*
*
* @return Additional information about the current status.
*/
public String getStatusMessage() {
return this.statusMessage;
}
/**
*
* Additional information about the current status.
*
*
* @param statusMessage
* Additional information about the current status.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public MatchmakingTicket withStatusMessage(String statusMessage) {
setStatusMessage(statusMessage);
return this;
}
/**
*
* Time stamp indicating when this matchmaking request was received. Format is a number expressed in Unix time as
* milliseconds (for example "1469498468.057"
).
*
*
* @param startTime
* Time stamp indicating when this matchmaking request was received. Format is a number expressed in Unix
* time as milliseconds (for example "1469498468.057"
).
*/
public void setStartTime(java.util.Date startTime) {
this.startTime = startTime;
}
/**
*
* Time stamp indicating when this matchmaking request was received. Format is a number expressed in Unix time as
* milliseconds (for example "1469498468.057"
).
*
*
* @return Time stamp indicating when this matchmaking request was received. Format is a number expressed in Unix
* time as milliseconds (for example "1469498468.057"
).
*/
public java.util.Date getStartTime() {
return this.startTime;
}
/**
*
* Time stamp indicating when this matchmaking request was received. Format is a number expressed in Unix time as
* milliseconds (for example "1469498468.057"
).
*
*
* @param startTime
* Time stamp indicating when this matchmaking request was received. Format is a number expressed in Unix
* time as milliseconds (for example "1469498468.057"
).
* @return Returns a reference to this object so that method calls can be chained together.
*/
public MatchmakingTicket withStartTime(java.util.Date startTime) {
setStartTime(startTime);
return this;
}
/**
*
* Time stamp indicating when this matchmaking request stopped being processed due to success, failure, or
* cancellation. Format is a number expressed in Unix time as milliseconds (for example
* "1469498468.057"
).
*
*
* @param endTime
* Time stamp indicating when this matchmaking request stopped being processed due to success, failure, or
* cancellation. Format is a number expressed in Unix time as milliseconds (for example
* "1469498468.057"
).
*/
public void setEndTime(java.util.Date endTime) {
this.endTime = endTime;
}
/**
*
* Time stamp indicating when this matchmaking request stopped being processed due to success, failure, or
* cancellation. Format is a number expressed in Unix time as milliseconds (for example
* "1469498468.057"
).
*
*
* @return Time stamp indicating when this matchmaking request stopped being processed due to success, failure, or
* cancellation. Format is a number expressed in Unix time as milliseconds (for example
* "1469498468.057"
).
*/
public java.util.Date getEndTime() {
return this.endTime;
}
/**
*
* Time stamp indicating when this matchmaking request stopped being processed due to success, failure, or
* cancellation. Format is a number expressed in Unix time as milliseconds (for example
* "1469498468.057"
).
*
*
* @param endTime
* Time stamp indicating when this matchmaking request stopped being processed due to success, failure, or
* cancellation. Format is a number expressed in Unix time as milliseconds (for example
* "1469498468.057"
).
* @return Returns a reference to this object so that method calls can be chained together.
*/
public MatchmakingTicket withEndTime(java.util.Date endTime) {
setEndTime(endTime);
return this;
}
/**
*
* A set of Player
objects, each representing a player to find matches for. Players are identified by a
* unique player ID and may include latency data for use during matchmaking. If the ticket is in status
* COMPLETED
, the Player
objects include the team the players were assigned to in the
* resulting match.
*
*
* @return A set of Player
objects, each representing a player to find matches for. Players are
* identified by a unique player ID and may include latency data for use during matchmaking. If the ticket
* is in status COMPLETED
, the Player
objects include the team the players were
* assigned to in the resulting match.
*/
public java.util.List getPlayers() {
return players;
}
/**
*
* A set of Player
objects, each representing a player to find matches for. Players are identified by a
* unique player ID and may include latency data for use during matchmaking. If the ticket is in status
* COMPLETED
, the Player
objects include the team the players were assigned to in the
* resulting match.
*
*
* @param players
* A set of Player
objects, each representing a player to find matches for. Players are
* identified by a unique player ID and may include latency data for use during matchmaking. If the ticket is
* in status COMPLETED
, the Player
objects include the team the players were
* assigned to in the resulting match.
*/
public void setPlayers(java.util.Collection players) {
if (players == null) {
this.players = null;
return;
}
this.players = new java.util.ArrayList(players);
}
/**
*
* A set of Player
objects, each representing a player to find matches for. Players are identified by a
* unique player ID and may include latency data for use during matchmaking. If the ticket is in status
* COMPLETED
, the Player
objects include the team the players were assigned to in the
* resulting match.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setPlayers(java.util.Collection)} or {@link #withPlayers(java.util.Collection)} if you want to override
* the existing values.
*
*
* @param players
* A set of Player
objects, each representing a player to find matches for. Players are
* identified by a unique player ID and may include latency data for use during matchmaking. If the ticket is
* in status COMPLETED
, the Player
objects include the team the players were
* assigned to in the resulting match.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public MatchmakingTicket withPlayers(Player... players) {
if (this.players == null) {
setPlayers(new java.util.ArrayList(players.length));
}
for (Player ele : players) {
this.players.add(ele);
}
return this;
}
/**
*
* A set of Player
objects, each representing a player to find matches for. Players are identified by a
* unique player ID and may include latency data for use during matchmaking. If the ticket is in status
* COMPLETED
, the Player
objects include the team the players were assigned to in the
* resulting match.
*
*
* @param players
* A set of Player
objects, each representing a player to find matches for. Players are
* identified by a unique player ID and may include latency data for use during matchmaking. If the ticket is
* in status COMPLETED
, the Player
objects include the team the players were
* assigned to in the resulting match.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public MatchmakingTicket withPlayers(java.util.Collection players) {
setPlayers(players);
return this;
}
/**
*
* Identifier and connection information of the game session created for the match. This information is added to the
* ticket only after the matchmaking request has been successfully completed. This parameter is not set when
* FlexMatch is being used without GameLift hosting.
*
*
* @param gameSessionConnectionInfo
* Identifier and connection information of the game session created for the match. This information is added
* to the ticket only after the matchmaking request has been successfully completed. This parameter is not
* set when FlexMatch is being used without GameLift hosting.
*/
public void setGameSessionConnectionInfo(GameSessionConnectionInfo gameSessionConnectionInfo) {
this.gameSessionConnectionInfo = gameSessionConnectionInfo;
}
/**
*
* Identifier and connection information of the game session created for the match. This information is added to the
* ticket only after the matchmaking request has been successfully completed. This parameter is not set when
* FlexMatch is being used without GameLift hosting.
*
*
* @return Identifier and connection information of the game session created for the match. This information is
* added to the ticket only after the matchmaking request has been successfully completed. This parameter is
* not set when FlexMatch is being used without GameLift hosting.
*/
public GameSessionConnectionInfo getGameSessionConnectionInfo() {
return this.gameSessionConnectionInfo;
}
/**
*
* Identifier and connection information of the game session created for the match. This information is added to the
* ticket only after the matchmaking request has been successfully completed. This parameter is not set when
* FlexMatch is being used without GameLift hosting.
*
*
* @param gameSessionConnectionInfo
* Identifier and connection information of the game session created for the match. This information is added
* to the ticket only after the matchmaking request has been successfully completed. This parameter is not
* set when FlexMatch is being used without GameLift hosting.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public MatchmakingTicket withGameSessionConnectionInfo(GameSessionConnectionInfo gameSessionConnectionInfo) {
setGameSessionConnectionInfo(gameSessionConnectionInfo);
return this;
}
/**
*
* Average amount of time (in seconds) that players are currently waiting for a match. If there is not enough recent
* data, this property may be empty.
*
*
* @param estimatedWaitTime
* Average amount of time (in seconds) that players are currently waiting for a match. If there is not enough
* recent data, this property may be empty.
*/
public void setEstimatedWaitTime(Integer estimatedWaitTime) {
this.estimatedWaitTime = estimatedWaitTime;
}
/**
*
* Average amount of time (in seconds) that players are currently waiting for a match. If there is not enough recent
* data, this property may be empty.
*
*
* @return Average amount of time (in seconds) that players are currently waiting for a match. If there is not
* enough recent data, this property may be empty.
*/
public Integer getEstimatedWaitTime() {
return this.estimatedWaitTime;
}
/**
*
* Average amount of time (in seconds) that players are currently waiting for a match. If there is not enough recent
* data, this property may be empty.
*
*
* @param estimatedWaitTime
* Average amount of time (in seconds) that players are currently waiting for a match. If there is not enough
* recent data, this property may be empty.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public MatchmakingTicket withEstimatedWaitTime(Integer estimatedWaitTime) {
setEstimatedWaitTime(estimatedWaitTime);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getTicketId() != null)
sb.append("TicketId: ").append(getTicketId()).append(",");
if (getConfigurationName() != null)
sb.append("ConfigurationName: ").append(getConfigurationName()).append(",");
if (getConfigurationArn() != null)
sb.append("ConfigurationArn: ").append(getConfigurationArn()).append(",");
if (getStatus() != null)
sb.append("Status: ").append(getStatus()).append(",");
if (getStatusReason() != null)
sb.append("StatusReason: ").append(getStatusReason()).append(",");
if (getStatusMessage() != null)
sb.append("StatusMessage: ").append(getStatusMessage()).append(",");
if (getStartTime() != null)
sb.append("StartTime: ").append(getStartTime()).append(",");
if (getEndTime() != null)
sb.append("EndTime: ").append(getEndTime()).append(",");
if (getPlayers() != null)
sb.append("Players: ").append(getPlayers()).append(",");
if (getGameSessionConnectionInfo() != null)
sb.append("GameSessionConnectionInfo: ").append(getGameSessionConnectionInfo()).append(",");
if (getEstimatedWaitTime() != null)
sb.append("EstimatedWaitTime: ").append(getEstimatedWaitTime());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof MatchmakingTicket == false)
return false;
MatchmakingTicket other = (MatchmakingTicket) obj;
if (other.getTicketId() == null ^ this.getTicketId() == null)
return false;
if (other.getTicketId() != null && other.getTicketId().equals(this.getTicketId()) == false)
return false;
if (other.getConfigurationName() == null ^ this.getConfigurationName() == null)
return false;
if (other.getConfigurationName() != null && other.getConfigurationName().equals(this.getConfigurationName()) == false)
return false;
if (other.getConfigurationArn() == null ^ this.getConfigurationArn() == null)
return false;
if (other.getConfigurationArn() != null && other.getConfigurationArn().equals(this.getConfigurationArn()) == false)
return false;
if (other.getStatus() == null ^ this.getStatus() == null)
return false;
if (other.getStatus() != null && other.getStatus().equals(this.getStatus()) == false)
return false;
if (other.getStatusReason() == null ^ this.getStatusReason() == null)
return false;
if (other.getStatusReason() != null && other.getStatusReason().equals(this.getStatusReason()) == false)
return false;
if (other.getStatusMessage() == null ^ this.getStatusMessage() == null)
return false;
if (other.getStatusMessage() != null && other.getStatusMessage().equals(this.getStatusMessage()) == false)
return false;
if (other.getStartTime() == null ^ this.getStartTime() == null)
return false;
if (other.getStartTime() != null && other.getStartTime().equals(this.getStartTime()) == false)
return false;
if (other.getEndTime() == null ^ this.getEndTime() == null)
return false;
if (other.getEndTime() != null && other.getEndTime().equals(this.getEndTime()) == false)
return false;
if (other.getPlayers() == null ^ this.getPlayers() == null)
return false;
if (other.getPlayers() != null && other.getPlayers().equals(this.getPlayers()) == false)
return false;
if (other.getGameSessionConnectionInfo() == null ^ this.getGameSessionConnectionInfo() == null)
return false;
if (other.getGameSessionConnectionInfo() != null && other.getGameSessionConnectionInfo().equals(this.getGameSessionConnectionInfo()) == false)
return false;
if (other.getEstimatedWaitTime() == null ^ this.getEstimatedWaitTime() == null)
return false;
if (other.getEstimatedWaitTime() != null && other.getEstimatedWaitTime().equals(this.getEstimatedWaitTime()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getTicketId() == null) ? 0 : getTicketId().hashCode());
hashCode = prime * hashCode + ((getConfigurationName() == null) ? 0 : getConfigurationName().hashCode());
hashCode = prime * hashCode + ((getConfigurationArn() == null) ? 0 : getConfigurationArn().hashCode());
hashCode = prime * hashCode + ((getStatus() == null) ? 0 : getStatus().hashCode());
hashCode = prime * hashCode + ((getStatusReason() == null) ? 0 : getStatusReason().hashCode());
hashCode = prime * hashCode + ((getStatusMessage() == null) ? 0 : getStatusMessage().hashCode());
hashCode = prime * hashCode + ((getStartTime() == null) ? 0 : getStartTime().hashCode());
hashCode = prime * hashCode + ((getEndTime() == null) ? 0 : getEndTime().hashCode());
hashCode = prime * hashCode + ((getPlayers() == null) ? 0 : getPlayers().hashCode());
hashCode = prime * hashCode + ((getGameSessionConnectionInfo() == null) ? 0 : getGameSessionConnectionInfo().hashCode());
hashCode = prime * hashCode + ((getEstimatedWaitTime() == null) ? 0 : getEstimatedWaitTime().hashCode());
return hashCode;
}
@Override
public MatchmakingTicket clone() {
try {
return (MatchmakingTicket) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.gamelift.model.transform.MatchmakingTicketMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}