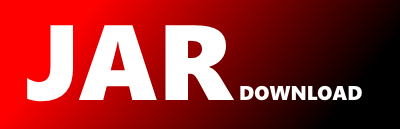
com.amazonaws.services.gamelift.model.AttributeValue Maven / Gradle / Ivy
Show all versions of aws-java-sdk-gamelift Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.gamelift.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* Values for use in player attribute key-value pairs. This object lets you specify an attribute value using any of the
* valid data types: string, number, string array, or data map. Each AttributeValue
object can use only one
* of the available properties.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class AttributeValue implements Serializable, Cloneable, StructuredPojo {
/**
*
* For single string values. Maximum string length is 100 characters.
*
*/
private String s;
/**
*
* For number values, expressed as double.
*
*/
private Double n;
/**
*
* For a list of up to 100 strings. Maximum length for each string is 100 characters. Duplicate values are not
* recognized; all occurrences of the repeated value after the first of a repeated value are ignored.
*
*/
private java.util.List sL;
/**
*
* For a map of up to 10 data type:value pairs. Maximum length for each string value is 100 characters.
*
*/
private java.util.Map sDM;
/**
*
* For single string values. Maximum string length is 100 characters.
*
*
* @param s
* For single string values. Maximum string length is 100 characters.
*/
public void setS(String s) {
this.s = s;
}
/**
*
* For single string values. Maximum string length is 100 characters.
*
*
* @return For single string values. Maximum string length is 100 characters.
*/
public String getS() {
return this.s;
}
/**
*
* For single string values. Maximum string length is 100 characters.
*
*
* @param s
* For single string values. Maximum string length is 100 characters.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AttributeValue withS(String s) {
setS(s);
return this;
}
/**
*
* For number values, expressed as double.
*
*
* @param n
* For number values, expressed as double.
*/
public void setN(Double n) {
this.n = n;
}
/**
*
* For number values, expressed as double.
*
*
* @return For number values, expressed as double.
*/
public Double getN() {
return this.n;
}
/**
*
* For number values, expressed as double.
*
*
* @param n
* For number values, expressed as double.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AttributeValue withN(Double n) {
setN(n);
return this;
}
/**
*
* For a list of up to 100 strings. Maximum length for each string is 100 characters. Duplicate values are not
* recognized; all occurrences of the repeated value after the first of a repeated value are ignored.
*
*
* @return For a list of up to 100 strings. Maximum length for each string is 100 characters. Duplicate values are
* not recognized; all occurrences of the repeated value after the first of a repeated value are ignored.
*/
public java.util.List getSL() {
return sL;
}
/**
*
* For a list of up to 100 strings. Maximum length for each string is 100 characters. Duplicate values are not
* recognized; all occurrences of the repeated value after the first of a repeated value are ignored.
*
*
* @param sL
* For a list of up to 100 strings. Maximum length for each string is 100 characters. Duplicate values are
* not recognized; all occurrences of the repeated value after the first of a repeated value are ignored.
*/
public void setSL(java.util.Collection sL) {
if (sL == null) {
this.sL = null;
return;
}
this.sL = new java.util.ArrayList(sL);
}
/**
*
* For a list of up to 100 strings. Maximum length for each string is 100 characters. Duplicate values are not
* recognized; all occurrences of the repeated value after the first of a repeated value are ignored.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setSL(java.util.Collection)} or {@link #withSL(java.util.Collection)} if you want to override the
* existing values.
*
*
* @param sL
* For a list of up to 100 strings. Maximum length for each string is 100 characters. Duplicate values are
* not recognized; all occurrences of the repeated value after the first of a repeated value are ignored.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AttributeValue withSL(String... sL) {
if (this.sL == null) {
setSL(new java.util.ArrayList(sL.length));
}
for (String ele : sL) {
this.sL.add(ele);
}
return this;
}
/**
*
* For a list of up to 100 strings. Maximum length for each string is 100 characters. Duplicate values are not
* recognized; all occurrences of the repeated value after the first of a repeated value are ignored.
*
*
* @param sL
* For a list of up to 100 strings. Maximum length for each string is 100 characters. Duplicate values are
* not recognized; all occurrences of the repeated value after the first of a repeated value are ignored.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AttributeValue withSL(java.util.Collection sL) {
setSL(sL);
return this;
}
/**
*
* For a map of up to 10 data type:value pairs. Maximum length for each string value is 100 characters.
*
*
* @return For a map of up to 10 data type:value pairs. Maximum length for each string value is 100 characters.
*/
public java.util.Map getSDM() {
return sDM;
}
/**
*
* For a map of up to 10 data type:value pairs. Maximum length for each string value is 100 characters.
*
*
* @param sDM
* For a map of up to 10 data type:value pairs. Maximum length for each string value is 100 characters.
*/
public void setSDM(java.util.Map sDM) {
this.sDM = sDM;
}
/**
*
* For a map of up to 10 data type:value pairs. Maximum length for each string value is 100 characters.
*
*
* @param sDM
* For a map of up to 10 data type:value pairs. Maximum length for each string value is 100 characters.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AttributeValue withSDM(java.util.Map sDM) {
setSDM(sDM);
return this;
}
/**
* Add a single SDM entry
*
* @see AttributeValue#withSDM
* @returns a reference to this object so that method calls can be chained together.
*/
public AttributeValue addSDMEntry(String key, Double value) {
if (null == this.sDM) {
this.sDM = new java.util.HashMap();
}
if (this.sDM.containsKey(key))
throw new IllegalArgumentException("Duplicated keys (" + key.toString() + ") are provided.");
this.sDM.put(key, value);
return this;
}
/**
* Removes all the entries added into SDM.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AttributeValue clearSDMEntries() {
this.sDM = null;
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getS() != null)
sb.append("S: ").append(getS()).append(",");
if (getN() != null)
sb.append("N: ").append(getN()).append(",");
if (getSL() != null)
sb.append("SL: ").append(getSL()).append(",");
if (getSDM() != null)
sb.append("SDM: ").append(getSDM());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof AttributeValue == false)
return false;
AttributeValue other = (AttributeValue) obj;
if (other.getS() == null ^ this.getS() == null)
return false;
if (other.getS() != null && other.getS().equals(this.getS()) == false)
return false;
if (other.getN() == null ^ this.getN() == null)
return false;
if (other.getN() != null && other.getN().equals(this.getN()) == false)
return false;
if (other.getSL() == null ^ this.getSL() == null)
return false;
if (other.getSL() != null && other.getSL().equals(this.getSL()) == false)
return false;
if (other.getSDM() == null ^ this.getSDM() == null)
return false;
if (other.getSDM() != null && other.getSDM().equals(this.getSDM()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getS() == null) ? 0 : getS().hashCode());
hashCode = prime * hashCode + ((getN() == null) ? 0 : getN().hashCode());
hashCode = prime * hashCode + ((getSL() == null) ? 0 : getSL().hashCode());
hashCode = prime * hashCode + ((getSDM() == null) ? 0 : getSDM().hashCode());
return hashCode;
}
@Override
public AttributeValue clone() {
try {
return (AttributeValue) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.gamelift.model.transform.AttributeValueMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}