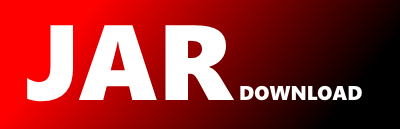
com.amazonaws.services.gamelift.model.ScalingPolicy Maven / Gradle / Ivy
Show all versions of aws-java-sdk-gamelift Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.gamelift.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* Rule that controls how a fleet is scaled. Scaling policies are uniquely identified by the combination of name and
* fleet ID.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class ScalingPolicy implements Serializable, Cloneable, StructuredPojo {
/**
*
* A unique identifier for the fleet that is associated with this scaling policy.
*
*/
private String fleetId;
/**
*
* The Amazon Resource Name (ARN)
* that is assigned to a Amazon GameLift fleet resource and uniquely identifies it. ARNs are unique across all
* Regions. Format is arn:aws:gamelift:<region>::fleet/fleet-a1234567-b8c9-0d1e-2fa3-b45c6d7e8912
* .
*
*/
private String fleetArn;
/**
*
* A descriptive label that is associated with a fleet's scaling policy. Policy names do not need to be unique.
*
*/
private String name;
/**
*
* Current status of the scaling policy. The scaling policy can be in force only when in an ACTIVE
* status. Scaling policies can be suspended for individual fleets. If the policy is suspended for a fleet, the
* policy status does not change.
*
*
* -
*
* ACTIVE -- The scaling policy can be used for auto-scaling a fleet.
*
*
* -
*
* UPDATE_REQUESTED -- A request to update the scaling policy has been received.
*
*
* -
*
* UPDATING -- A change is being made to the scaling policy.
*
*
* -
*
* DELETE_REQUESTED -- A request to delete the scaling policy has been received.
*
*
* -
*
* DELETING -- The scaling policy is being deleted.
*
*
* -
*
* DELETED -- The scaling policy has been deleted.
*
*
* -
*
* ERROR -- An error occurred in creating the policy. It should be removed and recreated.
*
*
*
*/
private String status;
/**
*
* Amount of adjustment to make, based on the scaling adjustment type.
*
*/
private Integer scalingAdjustment;
/**
*
* The type of adjustment to make to a fleet's instance count.
*
*
* -
*
* ChangeInCapacity -- add (or subtract) the scaling adjustment value from the current instance count.
* Positive values scale up while negative values scale down.
*
*
* -
*
* ExactCapacity -- set the instance count to the scaling adjustment value.
*
*
* -
*
* PercentChangeInCapacity -- increase or reduce the current instance count by the scaling adjustment, read
* as a percentage. Positive values scale up while negative values scale down.
*
*
*
*/
private String scalingAdjustmentType;
/**
*
* Comparison operator to use when measuring a metric against the threshold value.
*
*/
private String comparisonOperator;
/**
*
* Metric value used to trigger a scaling event.
*
*/
private Double threshold;
/**
*
* Length of time (in minutes) the metric must be at or beyond the threshold before a scaling event is triggered.
*
*/
private Integer evaluationPeriods;
/**
*
* Name of the Amazon GameLift-defined metric that is used to trigger a scaling adjustment. For detailed
* descriptions of fleet metrics, see Monitor Amazon
* GameLift with Amazon CloudWatch.
*
*
* -
*
* ActivatingGameSessions -- Game sessions in the process of being created.
*
*
* -
*
* ActiveGameSessions -- Game sessions that are currently running.
*
*
* -
*
* ActiveInstances -- Fleet instances that are currently running at least one game session.
*
*
* -
*
* AvailableGameSessions -- Additional game sessions that fleet could host simultaneously, given current
* capacity.
*
*
* -
*
* AvailablePlayerSessions -- Empty player slots in currently active game sessions. This includes game
* sessions that are not currently accepting players. Reserved player slots are not included.
*
*
* -
*
* CurrentPlayerSessions -- Player slots in active game sessions that are being used by a player or are
* reserved for a player.
*
*
* -
*
* IdleInstances -- Active instances that are currently hosting zero game sessions.
*
*
* -
*
* PercentAvailableGameSessions -- Unused percentage of the total number of game sessions that a fleet could
* host simultaneously, given current capacity. Use this metric for a target-based scaling policy.
*
*
* -
*
* PercentIdleInstances -- Percentage of the total number of active instances that are hosting zero game
* sessions.
*
*
* -
*
* QueueDepth -- Pending game session placement requests, in any queue, where the current fleet is the
* top-priority destination.
*
*
* -
*
* WaitTime -- Current wait time for pending game session placement requests, in any queue, where the current
* fleet is the top-priority destination.
*
*
*
*/
private String metricName;
/**
*
* The type of scaling policy to create. For a target-based policy, set the parameter MetricName to
* 'PercentAvailableGameSessions' and specify a TargetConfiguration. For a rule-based policy set the
* following parameters: MetricName, ComparisonOperator, Threshold, EvaluationPeriods,
* ScalingAdjustmentType, and ScalingAdjustment.
*
*/
private String policyType;
/**
*
* An object that contains settings for a target-based scaling policy.
*
*/
private TargetConfiguration targetConfiguration;
/**
*
* The current status of the fleet's scaling policies in a requested fleet location. The status
* PENDING_UPDATE
indicates that an update was requested for the fleet but has not yet been completed
* for the location.
*
*/
private String updateStatus;
/**
*
* The fleet location.
*
*/
private String location;
/**
*
* A unique identifier for the fleet that is associated with this scaling policy.
*
*
* @param fleetId
* A unique identifier for the fleet that is associated with this scaling policy.
*/
public void setFleetId(String fleetId) {
this.fleetId = fleetId;
}
/**
*
* A unique identifier for the fleet that is associated with this scaling policy.
*
*
* @return A unique identifier for the fleet that is associated with this scaling policy.
*/
public String getFleetId() {
return this.fleetId;
}
/**
*
* A unique identifier for the fleet that is associated with this scaling policy.
*
*
* @param fleetId
* A unique identifier for the fleet that is associated with this scaling policy.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ScalingPolicy withFleetId(String fleetId) {
setFleetId(fleetId);
return this;
}
/**
*
* The Amazon Resource Name (ARN)
* that is assigned to a Amazon GameLift fleet resource and uniquely identifies it. ARNs are unique across all
* Regions. Format is arn:aws:gamelift:<region>::fleet/fleet-a1234567-b8c9-0d1e-2fa3-b45c6d7e8912
* .
*
*
* @param fleetArn
* The Amazon Resource Name (ARN) that is assigned to a
* Amazon GameLift fleet resource and uniquely identifies it. ARNs are unique across all Regions. Format is
* arn:aws:gamelift:<region>::fleet/fleet-a1234567-b8c9-0d1e-2fa3-b45c6d7e8912
.
*/
public void setFleetArn(String fleetArn) {
this.fleetArn = fleetArn;
}
/**
*
* The Amazon Resource Name (ARN)
* that is assigned to a Amazon GameLift fleet resource and uniquely identifies it. ARNs are unique across all
* Regions. Format is arn:aws:gamelift:<region>::fleet/fleet-a1234567-b8c9-0d1e-2fa3-b45c6d7e8912
* .
*
*
* @return The Amazon Resource Name (ARN) that is assigned to a
* Amazon GameLift fleet resource and uniquely identifies it. ARNs are unique across all Regions. Format is
* arn:aws:gamelift:<region>::fleet/fleet-a1234567-b8c9-0d1e-2fa3-b45c6d7e8912
.
*/
public String getFleetArn() {
return this.fleetArn;
}
/**
*
* The Amazon Resource Name (ARN)
* that is assigned to a Amazon GameLift fleet resource and uniquely identifies it. ARNs are unique across all
* Regions. Format is arn:aws:gamelift:<region>::fleet/fleet-a1234567-b8c9-0d1e-2fa3-b45c6d7e8912
* .
*
*
* @param fleetArn
* The Amazon Resource Name (ARN) that is assigned to a
* Amazon GameLift fleet resource and uniquely identifies it. ARNs are unique across all Regions. Format is
* arn:aws:gamelift:<region>::fleet/fleet-a1234567-b8c9-0d1e-2fa3-b45c6d7e8912
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ScalingPolicy withFleetArn(String fleetArn) {
setFleetArn(fleetArn);
return this;
}
/**
*
* A descriptive label that is associated with a fleet's scaling policy. Policy names do not need to be unique.
*
*
* @param name
* A descriptive label that is associated with a fleet's scaling policy. Policy names do not need to be
* unique.
*/
public void setName(String name) {
this.name = name;
}
/**
*
* A descriptive label that is associated with a fleet's scaling policy. Policy names do not need to be unique.
*
*
* @return A descriptive label that is associated with a fleet's scaling policy. Policy names do not need to be
* unique.
*/
public String getName() {
return this.name;
}
/**
*
* A descriptive label that is associated with a fleet's scaling policy. Policy names do not need to be unique.
*
*
* @param name
* A descriptive label that is associated with a fleet's scaling policy. Policy names do not need to be
* unique.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ScalingPolicy withName(String name) {
setName(name);
return this;
}
/**
*
* Current status of the scaling policy. The scaling policy can be in force only when in an ACTIVE
* status. Scaling policies can be suspended for individual fleets. If the policy is suspended for a fleet, the
* policy status does not change.
*
*
* -
*
* ACTIVE -- The scaling policy can be used for auto-scaling a fleet.
*
*
* -
*
* UPDATE_REQUESTED -- A request to update the scaling policy has been received.
*
*
* -
*
* UPDATING -- A change is being made to the scaling policy.
*
*
* -
*
* DELETE_REQUESTED -- A request to delete the scaling policy has been received.
*
*
* -
*
* DELETING -- The scaling policy is being deleted.
*
*
* -
*
* DELETED -- The scaling policy has been deleted.
*
*
* -
*
* ERROR -- An error occurred in creating the policy. It should be removed and recreated.
*
*
*
*
* @param status
* Current status of the scaling policy. The scaling policy can be in force only when in an
* ACTIVE
status. Scaling policies can be suspended for individual fleets. If the policy is
* suspended for a fleet, the policy status does not change.
*
* -
*
* ACTIVE -- The scaling policy can be used for auto-scaling a fleet.
*
*
* -
*
* UPDATE_REQUESTED -- A request to update the scaling policy has been received.
*
*
* -
*
* UPDATING -- A change is being made to the scaling policy.
*
*
* -
*
* DELETE_REQUESTED -- A request to delete the scaling policy has been received.
*
*
* -
*
* DELETING -- The scaling policy is being deleted.
*
*
* -
*
* DELETED -- The scaling policy has been deleted.
*
*
* -
*
* ERROR -- An error occurred in creating the policy. It should be removed and recreated.
*
*
* @see ScalingStatusType
*/
public void setStatus(String status) {
this.status = status;
}
/**
*
* Current status of the scaling policy. The scaling policy can be in force only when in an ACTIVE
* status. Scaling policies can be suspended for individual fleets. If the policy is suspended for a fleet, the
* policy status does not change.
*
*
* -
*
* ACTIVE -- The scaling policy can be used for auto-scaling a fleet.
*
*
* -
*
* UPDATE_REQUESTED -- A request to update the scaling policy has been received.
*
*
* -
*
* UPDATING -- A change is being made to the scaling policy.
*
*
* -
*
* DELETE_REQUESTED -- A request to delete the scaling policy has been received.
*
*
* -
*
* DELETING -- The scaling policy is being deleted.
*
*
* -
*
* DELETED -- The scaling policy has been deleted.
*
*
* -
*
* ERROR -- An error occurred in creating the policy. It should be removed and recreated.
*
*
*
*
* @return Current status of the scaling policy. The scaling policy can be in force only when in an
* ACTIVE
status. Scaling policies can be suspended for individual fleets. If the policy is
* suspended for a fleet, the policy status does not change.
*
* -
*
* ACTIVE -- The scaling policy can be used for auto-scaling a fleet.
*
*
* -
*
* UPDATE_REQUESTED -- A request to update the scaling policy has been received.
*
*
* -
*
* UPDATING -- A change is being made to the scaling policy.
*
*
* -
*
* DELETE_REQUESTED -- A request to delete the scaling policy has been received.
*
*
* -
*
* DELETING -- The scaling policy is being deleted.
*
*
* -
*
* DELETED -- The scaling policy has been deleted.
*
*
* -
*
* ERROR -- An error occurred in creating the policy. It should be removed and recreated.
*
*
* @see ScalingStatusType
*/
public String getStatus() {
return this.status;
}
/**
*
* Current status of the scaling policy. The scaling policy can be in force only when in an ACTIVE
* status. Scaling policies can be suspended for individual fleets. If the policy is suspended for a fleet, the
* policy status does not change.
*
*
* -
*
* ACTIVE -- The scaling policy can be used for auto-scaling a fleet.
*
*
* -
*
* UPDATE_REQUESTED -- A request to update the scaling policy has been received.
*
*
* -
*
* UPDATING -- A change is being made to the scaling policy.
*
*
* -
*
* DELETE_REQUESTED -- A request to delete the scaling policy has been received.
*
*
* -
*
* DELETING -- The scaling policy is being deleted.
*
*
* -
*
* DELETED -- The scaling policy has been deleted.
*
*
* -
*
* ERROR -- An error occurred in creating the policy. It should be removed and recreated.
*
*
*
*
* @param status
* Current status of the scaling policy. The scaling policy can be in force only when in an
* ACTIVE
status. Scaling policies can be suspended for individual fleets. If the policy is
* suspended for a fleet, the policy status does not change.
*
* -
*
* ACTIVE -- The scaling policy can be used for auto-scaling a fleet.
*
*
* -
*
* UPDATE_REQUESTED -- A request to update the scaling policy has been received.
*
*
* -
*
* UPDATING -- A change is being made to the scaling policy.
*
*
* -
*
* DELETE_REQUESTED -- A request to delete the scaling policy has been received.
*
*
* -
*
* DELETING -- The scaling policy is being deleted.
*
*
* -
*
* DELETED -- The scaling policy has been deleted.
*
*
* -
*
* ERROR -- An error occurred in creating the policy. It should be removed and recreated.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see ScalingStatusType
*/
public ScalingPolicy withStatus(String status) {
setStatus(status);
return this;
}
/**
*
* Current status of the scaling policy. The scaling policy can be in force only when in an ACTIVE
* status. Scaling policies can be suspended for individual fleets. If the policy is suspended for a fleet, the
* policy status does not change.
*
*
* -
*
* ACTIVE -- The scaling policy can be used for auto-scaling a fleet.
*
*
* -
*
* UPDATE_REQUESTED -- A request to update the scaling policy has been received.
*
*
* -
*
* UPDATING -- A change is being made to the scaling policy.
*
*
* -
*
* DELETE_REQUESTED -- A request to delete the scaling policy has been received.
*
*
* -
*
* DELETING -- The scaling policy is being deleted.
*
*
* -
*
* DELETED -- The scaling policy has been deleted.
*
*
* -
*
* ERROR -- An error occurred in creating the policy. It should be removed and recreated.
*
*
*
*
* @param status
* Current status of the scaling policy. The scaling policy can be in force only when in an
* ACTIVE
status. Scaling policies can be suspended for individual fleets. If the policy is
* suspended for a fleet, the policy status does not change.
*
* -
*
* ACTIVE -- The scaling policy can be used for auto-scaling a fleet.
*
*
* -
*
* UPDATE_REQUESTED -- A request to update the scaling policy has been received.
*
*
* -
*
* UPDATING -- A change is being made to the scaling policy.
*
*
* -
*
* DELETE_REQUESTED -- A request to delete the scaling policy has been received.
*
*
* -
*
* DELETING -- The scaling policy is being deleted.
*
*
* -
*
* DELETED -- The scaling policy has been deleted.
*
*
* -
*
* ERROR -- An error occurred in creating the policy. It should be removed and recreated.
*
*
* @see ScalingStatusType
*/
public void setStatus(ScalingStatusType status) {
withStatus(status);
}
/**
*
* Current status of the scaling policy. The scaling policy can be in force only when in an ACTIVE
* status. Scaling policies can be suspended for individual fleets. If the policy is suspended for a fleet, the
* policy status does not change.
*
*
* -
*
* ACTIVE -- The scaling policy can be used for auto-scaling a fleet.
*
*
* -
*
* UPDATE_REQUESTED -- A request to update the scaling policy has been received.
*
*
* -
*
* UPDATING -- A change is being made to the scaling policy.
*
*
* -
*
* DELETE_REQUESTED -- A request to delete the scaling policy has been received.
*
*
* -
*
* DELETING -- The scaling policy is being deleted.
*
*
* -
*
* DELETED -- The scaling policy has been deleted.
*
*
* -
*
* ERROR -- An error occurred in creating the policy. It should be removed and recreated.
*
*
*
*
* @param status
* Current status of the scaling policy. The scaling policy can be in force only when in an
* ACTIVE
status. Scaling policies can be suspended for individual fleets. If the policy is
* suspended for a fleet, the policy status does not change.
*
* -
*
* ACTIVE -- The scaling policy can be used for auto-scaling a fleet.
*
*
* -
*
* UPDATE_REQUESTED -- A request to update the scaling policy has been received.
*
*
* -
*
* UPDATING -- A change is being made to the scaling policy.
*
*
* -
*
* DELETE_REQUESTED -- A request to delete the scaling policy has been received.
*
*
* -
*
* DELETING -- The scaling policy is being deleted.
*
*
* -
*
* DELETED -- The scaling policy has been deleted.
*
*
* -
*
* ERROR -- An error occurred in creating the policy. It should be removed and recreated.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see ScalingStatusType
*/
public ScalingPolicy withStatus(ScalingStatusType status) {
this.status = status.toString();
return this;
}
/**
*
* Amount of adjustment to make, based on the scaling adjustment type.
*
*
* @param scalingAdjustment
* Amount of adjustment to make, based on the scaling adjustment type.
*/
public void setScalingAdjustment(Integer scalingAdjustment) {
this.scalingAdjustment = scalingAdjustment;
}
/**
*
* Amount of adjustment to make, based on the scaling adjustment type.
*
*
* @return Amount of adjustment to make, based on the scaling adjustment type.
*/
public Integer getScalingAdjustment() {
return this.scalingAdjustment;
}
/**
*
* Amount of adjustment to make, based on the scaling adjustment type.
*
*
* @param scalingAdjustment
* Amount of adjustment to make, based on the scaling adjustment type.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ScalingPolicy withScalingAdjustment(Integer scalingAdjustment) {
setScalingAdjustment(scalingAdjustment);
return this;
}
/**
*
* The type of adjustment to make to a fleet's instance count.
*
*
* -
*
* ChangeInCapacity -- add (or subtract) the scaling adjustment value from the current instance count.
* Positive values scale up while negative values scale down.
*
*
* -
*
* ExactCapacity -- set the instance count to the scaling adjustment value.
*
*
* -
*
* PercentChangeInCapacity -- increase or reduce the current instance count by the scaling adjustment, read
* as a percentage. Positive values scale up while negative values scale down.
*
*
*
*
* @param scalingAdjustmentType
* The type of adjustment to make to a fleet's instance count.
*
* -
*
* ChangeInCapacity -- add (or subtract) the scaling adjustment value from the current instance count.
* Positive values scale up while negative values scale down.
*
*
* -
*
* ExactCapacity -- set the instance count to the scaling adjustment value.
*
*
* -
*
* PercentChangeInCapacity -- increase or reduce the current instance count by the scaling adjustment,
* read as a percentage. Positive values scale up while negative values scale down.
*
*
* @see ScalingAdjustmentType
*/
public void setScalingAdjustmentType(String scalingAdjustmentType) {
this.scalingAdjustmentType = scalingAdjustmentType;
}
/**
*
* The type of adjustment to make to a fleet's instance count.
*
*
* -
*
* ChangeInCapacity -- add (or subtract) the scaling adjustment value from the current instance count.
* Positive values scale up while negative values scale down.
*
*
* -
*
* ExactCapacity -- set the instance count to the scaling adjustment value.
*
*
* -
*
* PercentChangeInCapacity -- increase or reduce the current instance count by the scaling adjustment, read
* as a percentage. Positive values scale up while negative values scale down.
*
*
*
*
* @return The type of adjustment to make to a fleet's instance count.
*
* -
*
* ChangeInCapacity -- add (or subtract) the scaling adjustment value from the current instance
* count. Positive values scale up while negative values scale down.
*
*
* -
*
* ExactCapacity -- set the instance count to the scaling adjustment value.
*
*
* -
*
* PercentChangeInCapacity -- increase or reduce the current instance count by the scaling
* adjustment, read as a percentage. Positive values scale up while negative values scale down.
*
*
* @see ScalingAdjustmentType
*/
public String getScalingAdjustmentType() {
return this.scalingAdjustmentType;
}
/**
*
* The type of adjustment to make to a fleet's instance count.
*
*
* -
*
* ChangeInCapacity -- add (or subtract) the scaling adjustment value from the current instance count.
* Positive values scale up while negative values scale down.
*
*
* -
*
* ExactCapacity -- set the instance count to the scaling adjustment value.
*
*
* -
*
* PercentChangeInCapacity -- increase or reduce the current instance count by the scaling adjustment, read
* as a percentage. Positive values scale up while negative values scale down.
*
*
*
*
* @param scalingAdjustmentType
* The type of adjustment to make to a fleet's instance count.
*
* -
*
* ChangeInCapacity -- add (or subtract) the scaling adjustment value from the current instance count.
* Positive values scale up while negative values scale down.
*
*
* -
*
* ExactCapacity -- set the instance count to the scaling adjustment value.
*
*
* -
*
* PercentChangeInCapacity -- increase or reduce the current instance count by the scaling adjustment,
* read as a percentage. Positive values scale up while negative values scale down.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see ScalingAdjustmentType
*/
public ScalingPolicy withScalingAdjustmentType(String scalingAdjustmentType) {
setScalingAdjustmentType(scalingAdjustmentType);
return this;
}
/**
*
* The type of adjustment to make to a fleet's instance count.
*
*
* -
*
* ChangeInCapacity -- add (or subtract) the scaling adjustment value from the current instance count.
* Positive values scale up while negative values scale down.
*
*
* -
*
* ExactCapacity -- set the instance count to the scaling adjustment value.
*
*
* -
*
* PercentChangeInCapacity -- increase or reduce the current instance count by the scaling adjustment, read
* as a percentage. Positive values scale up while negative values scale down.
*
*
*
*
* @param scalingAdjustmentType
* The type of adjustment to make to a fleet's instance count.
*
* -
*
* ChangeInCapacity -- add (or subtract) the scaling adjustment value from the current instance count.
* Positive values scale up while negative values scale down.
*
*
* -
*
* ExactCapacity -- set the instance count to the scaling adjustment value.
*
*
* -
*
* PercentChangeInCapacity -- increase or reduce the current instance count by the scaling adjustment,
* read as a percentage. Positive values scale up while negative values scale down.
*
*
* @see ScalingAdjustmentType
*/
public void setScalingAdjustmentType(ScalingAdjustmentType scalingAdjustmentType) {
withScalingAdjustmentType(scalingAdjustmentType);
}
/**
*
* The type of adjustment to make to a fleet's instance count.
*
*
* -
*
* ChangeInCapacity -- add (or subtract) the scaling adjustment value from the current instance count.
* Positive values scale up while negative values scale down.
*
*
* -
*
* ExactCapacity -- set the instance count to the scaling adjustment value.
*
*
* -
*
* PercentChangeInCapacity -- increase or reduce the current instance count by the scaling adjustment, read
* as a percentage. Positive values scale up while negative values scale down.
*
*
*
*
* @param scalingAdjustmentType
* The type of adjustment to make to a fleet's instance count.
*
* -
*
* ChangeInCapacity -- add (or subtract) the scaling adjustment value from the current instance count.
* Positive values scale up while negative values scale down.
*
*
* -
*
* ExactCapacity -- set the instance count to the scaling adjustment value.
*
*
* -
*
* PercentChangeInCapacity -- increase or reduce the current instance count by the scaling adjustment,
* read as a percentage. Positive values scale up while negative values scale down.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see ScalingAdjustmentType
*/
public ScalingPolicy withScalingAdjustmentType(ScalingAdjustmentType scalingAdjustmentType) {
this.scalingAdjustmentType = scalingAdjustmentType.toString();
return this;
}
/**
*
* Comparison operator to use when measuring a metric against the threshold value.
*
*
* @param comparisonOperator
* Comparison operator to use when measuring a metric against the threshold value.
* @see ComparisonOperatorType
*/
public void setComparisonOperator(String comparisonOperator) {
this.comparisonOperator = comparisonOperator;
}
/**
*
* Comparison operator to use when measuring a metric against the threshold value.
*
*
* @return Comparison operator to use when measuring a metric against the threshold value.
* @see ComparisonOperatorType
*/
public String getComparisonOperator() {
return this.comparisonOperator;
}
/**
*
* Comparison operator to use when measuring a metric against the threshold value.
*
*
* @param comparisonOperator
* Comparison operator to use when measuring a metric against the threshold value.
* @return Returns a reference to this object so that method calls can be chained together.
* @see ComparisonOperatorType
*/
public ScalingPolicy withComparisonOperator(String comparisonOperator) {
setComparisonOperator(comparisonOperator);
return this;
}
/**
*
* Comparison operator to use when measuring a metric against the threshold value.
*
*
* @param comparisonOperator
* Comparison operator to use when measuring a metric against the threshold value.
* @see ComparisonOperatorType
*/
public void setComparisonOperator(ComparisonOperatorType comparisonOperator) {
withComparisonOperator(comparisonOperator);
}
/**
*
* Comparison operator to use when measuring a metric against the threshold value.
*
*
* @param comparisonOperator
* Comparison operator to use when measuring a metric against the threshold value.
* @return Returns a reference to this object so that method calls can be chained together.
* @see ComparisonOperatorType
*/
public ScalingPolicy withComparisonOperator(ComparisonOperatorType comparisonOperator) {
this.comparisonOperator = comparisonOperator.toString();
return this;
}
/**
*
* Metric value used to trigger a scaling event.
*
*
* @param threshold
* Metric value used to trigger a scaling event.
*/
public void setThreshold(Double threshold) {
this.threshold = threshold;
}
/**
*
* Metric value used to trigger a scaling event.
*
*
* @return Metric value used to trigger a scaling event.
*/
public Double getThreshold() {
return this.threshold;
}
/**
*
* Metric value used to trigger a scaling event.
*
*
* @param threshold
* Metric value used to trigger a scaling event.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ScalingPolicy withThreshold(Double threshold) {
setThreshold(threshold);
return this;
}
/**
*
* Length of time (in minutes) the metric must be at or beyond the threshold before a scaling event is triggered.
*
*
* @param evaluationPeriods
* Length of time (in minutes) the metric must be at or beyond the threshold before a scaling event is
* triggered.
*/
public void setEvaluationPeriods(Integer evaluationPeriods) {
this.evaluationPeriods = evaluationPeriods;
}
/**
*
* Length of time (in minutes) the metric must be at or beyond the threshold before a scaling event is triggered.
*
*
* @return Length of time (in minutes) the metric must be at or beyond the threshold before a scaling event is
* triggered.
*/
public Integer getEvaluationPeriods() {
return this.evaluationPeriods;
}
/**
*
* Length of time (in minutes) the metric must be at or beyond the threshold before a scaling event is triggered.
*
*
* @param evaluationPeriods
* Length of time (in minutes) the metric must be at or beyond the threshold before a scaling event is
* triggered.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ScalingPolicy withEvaluationPeriods(Integer evaluationPeriods) {
setEvaluationPeriods(evaluationPeriods);
return this;
}
/**
*
* Name of the Amazon GameLift-defined metric that is used to trigger a scaling adjustment. For detailed
* descriptions of fleet metrics, see Monitor Amazon
* GameLift with Amazon CloudWatch.
*
*
* -
*
* ActivatingGameSessions -- Game sessions in the process of being created.
*
*
* -
*
* ActiveGameSessions -- Game sessions that are currently running.
*
*
* -
*
* ActiveInstances -- Fleet instances that are currently running at least one game session.
*
*
* -
*
* AvailableGameSessions -- Additional game sessions that fleet could host simultaneously, given current
* capacity.
*
*
* -
*
* AvailablePlayerSessions -- Empty player slots in currently active game sessions. This includes game
* sessions that are not currently accepting players. Reserved player slots are not included.
*
*
* -
*
* CurrentPlayerSessions -- Player slots in active game sessions that are being used by a player or are
* reserved for a player.
*
*
* -
*
* IdleInstances -- Active instances that are currently hosting zero game sessions.
*
*
* -
*
* PercentAvailableGameSessions -- Unused percentage of the total number of game sessions that a fleet could
* host simultaneously, given current capacity. Use this metric for a target-based scaling policy.
*
*
* -
*
* PercentIdleInstances -- Percentage of the total number of active instances that are hosting zero game
* sessions.
*
*
* -
*
* QueueDepth -- Pending game session placement requests, in any queue, where the current fleet is the
* top-priority destination.
*
*
* -
*
* WaitTime -- Current wait time for pending game session placement requests, in any queue, where the current
* fleet is the top-priority destination.
*
*
*
*
* @param metricName
* Name of the Amazon GameLift-defined metric that is used to trigger a scaling adjustment. For detailed
* descriptions of fleet metrics, see Monitor
* Amazon GameLift with Amazon CloudWatch.
*
* -
*
* ActivatingGameSessions -- Game sessions in the process of being created.
*
*
* -
*
* ActiveGameSessions -- Game sessions that are currently running.
*
*
* -
*
* ActiveInstances -- Fleet instances that are currently running at least one game session.
*
*
* -
*
* AvailableGameSessions -- Additional game sessions that fleet could host simultaneously, given
* current capacity.
*
*
* -
*
* AvailablePlayerSessions -- Empty player slots in currently active game sessions. This includes game
* sessions that are not currently accepting players. Reserved player slots are not included.
*
*
* -
*
* CurrentPlayerSessions -- Player slots in active game sessions that are being used by a player or
* are reserved for a player.
*
*
* -
*
* IdleInstances -- Active instances that are currently hosting zero game sessions.
*
*
* -
*
* PercentAvailableGameSessions -- Unused percentage of the total number of game sessions that a fleet
* could host simultaneously, given current capacity. Use this metric for a target-based scaling policy.
*
*
* -
*
* PercentIdleInstances -- Percentage of the total number of active instances that are hosting zero
* game sessions.
*
*
* -
*
* QueueDepth -- Pending game session placement requests, in any queue, where the current fleet is the
* top-priority destination.
*
*
* -
*
* WaitTime -- Current wait time for pending game session placement requests, in any queue, where the
* current fleet is the top-priority destination.
*
*
* @see MetricName
*/
public void setMetricName(String metricName) {
this.metricName = metricName;
}
/**
*
* Name of the Amazon GameLift-defined metric that is used to trigger a scaling adjustment. For detailed
* descriptions of fleet metrics, see Monitor Amazon
* GameLift with Amazon CloudWatch.
*
*
* -
*
* ActivatingGameSessions -- Game sessions in the process of being created.
*
*
* -
*
* ActiveGameSessions -- Game sessions that are currently running.
*
*
* -
*
* ActiveInstances -- Fleet instances that are currently running at least one game session.
*
*
* -
*
* AvailableGameSessions -- Additional game sessions that fleet could host simultaneously, given current
* capacity.
*
*
* -
*
* AvailablePlayerSessions -- Empty player slots in currently active game sessions. This includes game
* sessions that are not currently accepting players. Reserved player slots are not included.
*
*
* -
*
* CurrentPlayerSessions -- Player slots in active game sessions that are being used by a player or are
* reserved for a player.
*
*
* -
*
* IdleInstances -- Active instances that are currently hosting zero game sessions.
*
*
* -
*
* PercentAvailableGameSessions -- Unused percentage of the total number of game sessions that a fleet could
* host simultaneously, given current capacity. Use this metric for a target-based scaling policy.
*
*
* -
*
* PercentIdleInstances -- Percentage of the total number of active instances that are hosting zero game
* sessions.
*
*
* -
*
* QueueDepth -- Pending game session placement requests, in any queue, where the current fleet is the
* top-priority destination.
*
*
* -
*
* WaitTime -- Current wait time for pending game session placement requests, in any queue, where the current
* fleet is the top-priority destination.
*
*
*
*
* @return Name of the Amazon GameLift-defined metric that is used to trigger a scaling adjustment. For detailed
* descriptions of fleet metrics, see Monitor
* Amazon GameLift with Amazon CloudWatch.
*
* -
*
* ActivatingGameSessions -- Game sessions in the process of being created.
*
*
* -
*
* ActiveGameSessions -- Game sessions that are currently running.
*
*
* -
*
* ActiveInstances -- Fleet instances that are currently running at least one game session.
*
*
* -
*
* AvailableGameSessions -- Additional game sessions that fleet could host simultaneously, given
* current capacity.
*
*
* -
*
* AvailablePlayerSessions -- Empty player slots in currently active game sessions. This includes
* game sessions that are not currently accepting players. Reserved player slots are not included.
*
*
* -
*
* CurrentPlayerSessions -- Player slots in active game sessions that are being used by a player or
* are reserved for a player.
*
*
* -
*
* IdleInstances -- Active instances that are currently hosting zero game sessions.
*
*
* -
*
* PercentAvailableGameSessions -- Unused percentage of the total number of game sessions that a
* fleet could host simultaneously, given current capacity. Use this metric for a target-based scaling
* policy.
*
*
* -
*
* PercentIdleInstances -- Percentage of the total number of active instances that are hosting zero
* game sessions.
*
*
* -
*
* QueueDepth -- Pending game session placement requests, in any queue, where the current fleet is
* the top-priority destination.
*
*
* -
*
* WaitTime -- Current wait time for pending game session placement requests, in any queue, where the
* current fleet is the top-priority destination.
*
*
* @see MetricName
*/
public String getMetricName() {
return this.metricName;
}
/**
*
* Name of the Amazon GameLift-defined metric that is used to trigger a scaling adjustment. For detailed
* descriptions of fleet metrics, see Monitor Amazon
* GameLift with Amazon CloudWatch.
*
*
* -
*
* ActivatingGameSessions -- Game sessions in the process of being created.
*
*
* -
*
* ActiveGameSessions -- Game sessions that are currently running.
*
*
* -
*
* ActiveInstances -- Fleet instances that are currently running at least one game session.
*
*
* -
*
* AvailableGameSessions -- Additional game sessions that fleet could host simultaneously, given current
* capacity.
*
*
* -
*
* AvailablePlayerSessions -- Empty player slots in currently active game sessions. This includes game
* sessions that are not currently accepting players. Reserved player slots are not included.
*
*
* -
*
* CurrentPlayerSessions -- Player slots in active game sessions that are being used by a player or are
* reserved for a player.
*
*
* -
*
* IdleInstances -- Active instances that are currently hosting zero game sessions.
*
*
* -
*
* PercentAvailableGameSessions -- Unused percentage of the total number of game sessions that a fleet could
* host simultaneously, given current capacity. Use this metric for a target-based scaling policy.
*
*
* -
*
* PercentIdleInstances -- Percentage of the total number of active instances that are hosting zero game
* sessions.
*
*
* -
*
* QueueDepth -- Pending game session placement requests, in any queue, where the current fleet is the
* top-priority destination.
*
*
* -
*
* WaitTime -- Current wait time for pending game session placement requests, in any queue, where the current
* fleet is the top-priority destination.
*
*
*
*
* @param metricName
* Name of the Amazon GameLift-defined metric that is used to trigger a scaling adjustment. For detailed
* descriptions of fleet metrics, see Monitor
* Amazon GameLift with Amazon CloudWatch.
*
* -
*
* ActivatingGameSessions -- Game sessions in the process of being created.
*
*
* -
*
* ActiveGameSessions -- Game sessions that are currently running.
*
*
* -
*
* ActiveInstances -- Fleet instances that are currently running at least one game session.
*
*
* -
*
* AvailableGameSessions -- Additional game sessions that fleet could host simultaneously, given
* current capacity.
*
*
* -
*
* AvailablePlayerSessions -- Empty player slots in currently active game sessions. This includes game
* sessions that are not currently accepting players. Reserved player slots are not included.
*
*
* -
*
* CurrentPlayerSessions -- Player slots in active game sessions that are being used by a player or
* are reserved for a player.
*
*
* -
*
* IdleInstances -- Active instances that are currently hosting zero game sessions.
*
*
* -
*
* PercentAvailableGameSessions -- Unused percentage of the total number of game sessions that a fleet
* could host simultaneously, given current capacity. Use this metric for a target-based scaling policy.
*
*
* -
*
* PercentIdleInstances -- Percentage of the total number of active instances that are hosting zero
* game sessions.
*
*
* -
*
* QueueDepth -- Pending game session placement requests, in any queue, where the current fleet is the
* top-priority destination.
*
*
* -
*
* WaitTime -- Current wait time for pending game session placement requests, in any queue, where the
* current fleet is the top-priority destination.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see MetricName
*/
public ScalingPolicy withMetricName(String metricName) {
setMetricName(metricName);
return this;
}
/**
*
* Name of the Amazon GameLift-defined metric that is used to trigger a scaling adjustment. For detailed
* descriptions of fleet metrics, see Monitor Amazon
* GameLift with Amazon CloudWatch.
*
*
* -
*
* ActivatingGameSessions -- Game sessions in the process of being created.
*
*
* -
*
* ActiveGameSessions -- Game sessions that are currently running.
*
*
* -
*
* ActiveInstances -- Fleet instances that are currently running at least one game session.
*
*
* -
*
* AvailableGameSessions -- Additional game sessions that fleet could host simultaneously, given current
* capacity.
*
*
* -
*
* AvailablePlayerSessions -- Empty player slots in currently active game sessions. This includes game
* sessions that are not currently accepting players. Reserved player slots are not included.
*
*
* -
*
* CurrentPlayerSessions -- Player slots in active game sessions that are being used by a player or are
* reserved for a player.
*
*
* -
*
* IdleInstances -- Active instances that are currently hosting zero game sessions.
*
*
* -
*
* PercentAvailableGameSessions -- Unused percentage of the total number of game sessions that a fleet could
* host simultaneously, given current capacity. Use this metric for a target-based scaling policy.
*
*
* -
*
* PercentIdleInstances -- Percentage of the total number of active instances that are hosting zero game
* sessions.
*
*
* -
*
* QueueDepth -- Pending game session placement requests, in any queue, where the current fleet is the
* top-priority destination.
*
*
* -
*
* WaitTime -- Current wait time for pending game session placement requests, in any queue, where the current
* fleet is the top-priority destination.
*
*
*
*
* @param metricName
* Name of the Amazon GameLift-defined metric that is used to trigger a scaling adjustment. For detailed
* descriptions of fleet metrics, see Monitor
* Amazon GameLift with Amazon CloudWatch.
*
* -
*
* ActivatingGameSessions -- Game sessions in the process of being created.
*
*
* -
*
* ActiveGameSessions -- Game sessions that are currently running.
*
*
* -
*
* ActiveInstances -- Fleet instances that are currently running at least one game session.
*
*
* -
*
* AvailableGameSessions -- Additional game sessions that fleet could host simultaneously, given
* current capacity.
*
*
* -
*
* AvailablePlayerSessions -- Empty player slots in currently active game sessions. This includes game
* sessions that are not currently accepting players. Reserved player slots are not included.
*
*
* -
*
* CurrentPlayerSessions -- Player slots in active game sessions that are being used by a player or
* are reserved for a player.
*
*
* -
*
* IdleInstances -- Active instances that are currently hosting zero game sessions.
*
*
* -
*
* PercentAvailableGameSessions -- Unused percentage of the total number of game sessions that a fleet
* could host simultaneously, given current capacity. Use this metric for a target-based scaling policy.
*
*
* -
*
* PercentIdleInstances -- Percentage of the total number of active instances that are hosting zero
* game sessions.
*
*
* -
*
* QueueDepth -- Pending game session placement requests, in any queue, where the current fleet is the
* top-priority destination.
*
*
* -
*
* WaitTime -- Current wait time for pending game session placement requests, in any queue, where the
* current fleet is the top-priority destination.
*
*
* @see MetricName
*/
public void setMetricName(MetricName metricName) {
withMetricName(metricName);
}
/**
*
* Name of the Amazon GameLift-defined metric that is used to trigger a scaling adjustment. For detailed
* descriptions of fleet metrics, see Monitor Amazon
* GameLift with Amazon CloudWatch.
*
*
* -
*
* ActivatingGameSessions -- Game sessions in the process of being created.
*
*
* -
*
* ActiveGameSessions -- Game sessions that are currently running.
*
*
* -
*
* ActiveInstances -- Fleet instances that are currently running at least one game session.
*
*
* -
*
* AvailableGameSessions -- Additional game sessions that fleet could host simultaneously, given current
* capacity.
*
*
* -
*
* AvailablePlayerSessions -- Empty player slots in currently active game sessions. This includes game
* sessions that are not currently accepting players. Reserved player slots are not included.
*
*
* -
*
* CurrentPlayerSessions -- Player slots in active game sessions that are being used by a player or are
* reserved for a player.
*
*
* -
*
* IdleInstances -- Active instances that are currently hosting zero game sessions.
*
*
* -
*
* PercentAvailableGameSessions -- Unused percentage of the total number of game sessions that a fleet could
* host simultaneously, given current capacity. Use this metric for a target-based scaling policy.
*
*
* -
*
* PercentIdleInstances -- Percentage of the total number of active instances that are hosting zero game
* sessions.
*
*
* -
*
* QueueDepth -- Pending game session placement requests, in any queue, where the current fleet is the
* top-priority destination.
*
*
* -
*
* WaitTime -- Current wait time for pending game session placement requests, in any queue, where the current
* fleet is the top-priority destination.
*
*
*
*
* @param metricName
* Name of the Amazon GameLift-defined metric that is used to trigger a scaling adjustment. For detailed
* descriptions of fleet metrics, see Monitor
* Amazon GameLift with Amazon CloudWatch.
*
* -
*
* ActivatingGameSessions -- Game sessions in the process of being created.
*
*
* -
*
* ActiveGameSessions -- Game sessions that are currently running.
*
*
* -
*
* ActiveInstances -- Fleet instances that are currently running at least one game session.
*
*
* -
*
* AvailableGameSessions -- Additional game sessions that fleet could host simultaneously, given
* current capacity.
*
*
* -
*
* AvailablePlayerSessions -- Empty player slots in currently active game sessions. This includes game
* sessions that are not currently accepting players. Reserved player slots are not included.
*
*
* -
*
* CurrentPlayerSessions -- Player slots in active game sessions that are being used by a player or
* are reserved for a player.
*
*
* -
*
* IdleInstances -- Active instances that are currently hosting zero game sessions.
*
*
* -
*
* PercentAvailableGameSessions -- Unused percentage of the total number of game sessions that a fleet
* could host simultaneously, given current capacity. Use this metric for a target-based scaling policy.
*
*
* -
*
* PercentIdleInstances -- Percentage of the total number of active instances that are hosting zero
* game sessions.
*
*
* -
*
* QueueDepth -- Pending game session placement requests, in any queue, where the current fleet is the
* top-priority destination.
*
*
* -
*
* WaitTime -- Current wait time for pending game session placement requests, in any queue, where the
* current fleet is the top-priority destination.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see MetricName
*/
public ScalingPolicy withMetricName(MetricName metricName) {
this.metricName = metricName.toString();
return this;
}
/**
*
* The type of scaling policy to create. For a target-based policy, set the parameter MetricName to
* 'PercentAvailableGameSessions' and specify a TargetConfiguration. For a rule-based policy set the
* following parameters: MetricName, ComparisonOperator, Threshold, EvaluationPeriods,
* ScalingAdjustmentType, and ScalingAdjustment.
*
*
* @param policyType
* The type of scaling policy to create. For a target-based policy, set the parameter MetricName to
* 'PercentAvailableGameSessions' and specify a TargetConfiguration. For a rule-based policy set the
* following parameters: MetricName, ComparisonOperator, Threshold,
* EvaluationPeriods, ScalingAdjustmentType, and ScalingAdjustment.
* @see PolicyType
*/
public void setPolicyType(String policyType) {
this.policyType = policyType;
}
/**
*
* The type of scaling policy to create. For a target-based policy, set the parameter MetricName to
* 'PercentAvailableGameSessions' and specify a TargetConfiguration. For a rule-based policy set the
* following parameters: MetricName, ComparisonOperator, Threshold, EvaluationPeriods,
* ScalingAdjustmentType, and ScalingAdjustment.
*
*
* @return The type of scaling policy to create. For a target-based policy, set the parameter MetricName to
* 'PercentAvailableGameSessions' and specify a TargetConfiguration. For a rule-based policy set the
* following parameters: MetricName, ComparisonOperator, Threshold,
* EvaluationPeriods, ScalingAdjustmentType, and ScalingAdjustment.
* @see PolicyType
*/
public String getPolicyType() {
return this.policyType;
}
/**
*
* The type of scaling policy to create. For a target-based policy, set the parameter MetricName to
* 'PercentAvailableGameSessions' and specify a TargetConfiguration. For a rule-based policy set the
* following parameters: MetricName, ComparisonOperator, Threshold, EvaluationPeriods,
* ScalingAdjustmentType, and ScalingAdjustment.
*
*
* @param policyType
* The type of scaling policy to create. For a target-based policy, set the parameter MetricName to
* 'PercentAvailableGameSessions' and specify a TargetConfiguration. For a rule-based policy set the
* following parameters: MetricName, ComparisonOperator, Threshold,
* EvaluationPeriods, ScalingAdjustmentType, and ScalingAdjustment.
* @return Returns a reference to this object so that method calls can be chained together.
* @see PolicyType
*/
public ScalingPolicy withPolicyType(String policyType) {
setPolicyType(policyType);
return this;
}
/**
*
* The type of scaling policy to create. For a target-based policy, set the parameter MetricName to
* 'PercentAvailableGameSessions' and specify a TargetConfiguration. For a rule-based policy set the
* following parameters: MetricName, ComparisonOperator, Threshold, EvaluationPeriods,
* ScalingAdjustmentType, and ScalingAdjustment.
*
*
* @param policyType
* The type of scaling policy to create. For a target-based policy, set the parameter MetricName to
* 'PercentAvailableGameSessions' and specify a TargetConfiguration. For a rule-based policy set the
* following parameters: MetricName, ComparisonOperator, Threshold,
* EvaluationPeriods, ScalingAdjustmentType, and ScalingAdjustment.
* @see PolicyType
*/
public void setPolicyType(PolicyType policyType) {
withPolicyType(policyType);
}
/**
*
* The type of scaling policy to create. For a target-based policy, set the parameter MetricName to
* 'PercentAvailableGameSessions' and specify a TargetConfiguration. For a rule-based policy set the
* following parameters: MetricName, ComparisonOperator, Threshold, EvaluationPeriods,
* ScalingAdjustmentType, and ScalingAdjustment.
*
*
* @param policyType
* The type of scaling policy to create. For a target-based policy, set the parameter MetricName to
* 'PercentAvailableGameSessions' and specify a TargetConfiguration. For a rule-based policy set the
* following parameters: MetricName, ComparisonOperator, Threshold,
* EvaluationPeriods, ScalingAdjustmentType, and ScalingAdjustment.
* @return Returns a reference to this object so that method calls can be chained together.
* @see PolicyType
*/
public ScalingPolicy withPolicyType(PolicyType policyType) {
this.policyType = policyType.toString();
return this;
}
/**
*
* An object that contains settings for a target-based scaling policy.
*
*
* @param targetConfiguration
* An object that contains settings for a target-based scaling policy.
*/
public void setTargetConfiguration(TargetConfiguration targetConfiguration) {
this.targetConfiguration = targetConfiguration;
}
/**
*
* An object that contains settings for a target-based scaling policy.
*
*
* @return An object that contains settings for a target-based scaling policy.
*/
public TargetConfiguration getTargetConfiguration() {
return this.targetConfiguration;
}
/**
*
* An object that contains settings for a target-based scaling policy.
*
*
* @param targetConfiguration
* An object that contains settings for a target-based scaling policy.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ScalingPolicy withTargetConfiguration(TargetConfiguration targetConfiguration) {
setTargetConfiguration(targetConfiguration);
return this;
}
/**
*
* The current status of the fleet's scaling policies in a requested fleet location. The status
* PENDING_UPDATE
indicates that an update was requested for the fleet but has not yet been completed
* for the location.
*
*
* @param updateStatus
* The current status of the fleet's scaling policies in a requested fleet location. The status
* PENDING_UPDATE
indicates that an update was requested for the fleet but has not yet been
* completed for the location.
* @see LocationUpdateStatus
*/
public void setUpdateStatus(String updateStatus) {
this.updateStatus = updateStatus;
}
/**
*
* The current status of the fleet's scaling policies in a requested fleet location. The status
* PENDING_UPDATE
indicates that an update was requested for the fleet but has not yet been completed
* for the location.
*
*
* @return The current status of the fleet's scaling policies in a requested fleet location. The status
* PENDING_UPDATE
indicates that an update was requested for the fleet but has not yet been
* completed for the location.
* @see LocationUpdateStatus
*/
public String getUpdateStatus() {
return this.updateStatus;
}
/**
*
* The current status of the fleet's scaling policies in a requested fleet location. The status
* PENDING_UPDATE
indicates that an update was requested for the fleet but has not yet been completed
* for the location.
*
*
* @param updateStatus
* The current status of the fleet's scaling policies in a requested fleet location. The status
* PENDING_UPDATE
indicates that an update was requested for the fleet but has not yet been
* completed for the location.
* @return Returns a reference to this object so that method calls can be chained together.
* @see LocationUpdateStatus
*/
public ScalingPolicy withUpdateStatus(String updateStatus) {
setUpdateStatus(updateStatus);
return this;
}
/**
*
* The current status of the fleet's scaling policies in a requested fleet location. The status
* PENDING_UPDATE
indicates that an update was requested for the fleet but has not yet been completed
* for the location.
*
*
* @param updateStatus
* The current status of the fleet's scaling policies in a requested fleet location. The status
* PENDING_UPDATE
indicates that an update was requested for the fleet but has not yet been
* completed for the location.
* @see LocationUpdateStatus
*/
public void setUpdateStatus(LocationUpdateStatus updateStatus) {
withUpdateStatus(updateStatus);
}
/**
*
* The current status of the fleet's scaling policies in a requested fleet location. The status
* PENDING_UPDATE
indicates that an update was requested for the fleet but has not yet been completed
* for the location.
*
*
* @param updateStatus
* The current status of the fleet's scaling policies in a requested fleet location. The status
* PENDING_UPDATE
indicates that an update was requested for the fleet but has not yet been
* completed for the location.
* @return Returns a reference to this object so that method calls can be chained together.
* @see LocationUpdateStatus
*/
public ScalingPolicy withUpdateStatus(LocationUpdateStatus updateStatus) {
this.updateStatus = updateStatus.toString();
return this;
}
/**
*
* The fleet location.
*
*
* @param location
* The fleet location.
*/
public void setLocation(String location) {
this.location = location;
}
/**
*
* The fleet location.
*
*
* @return The fleet location.
*/
public String getLocation() {
return this.location;
}
/**
*
* The fleet location.
*
*
* @param location
* The fleet location.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ScalingPolicy withLocation(String location) {
setLocation(location);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getFleetId() != null)
sb.append("FleetId: ").append(getFleetId()).append(",");
if (getFleetArn() != null)
sb.append("FleetArn: ").append(getFleetArn()).append(",");
if (getName() != null)
sb.append("Name: ").append(getName()).append(",");
if (getStatus() != null)
sb.append("Status: ").append(getStatus()).append(",");
if (getScalingAdjustment() != null)
sb.append("ScalingAdjustment: ").append(getScalingAdjustment()).append(",");
if (getScalingAdjustmentType() != null)
sb.append("ScalingAdjustmentType: ").append(getScalingAdjustmentType()).append(",");
if (getComparisonOperator() != null)
sb.append("ComparisonOperator: ").append(getComparisonOperator()).append(",");
if (getThreshold() != null)
sb.append("Threshold: ").append(getThreshold()).append(",");
if (getEvaluationPeriods() != null)
sb.append("EvaluationPeriods: ").append(getEvaluationPeriods()).append(",");
if (getMetricName() != null)
sb.append("MetricName: ").append(getMetricName()).append(",");
if (getPolicyType() != null)
sb.append("PolicyType: ").append(getPolicyType()).append(",");
if (getTargetConfiguration() != null)
sb.append("TargetConfiguration: ").append(getTargetConfiguration()).append(",");
if (getUpdateStatus() != null)
sb.append("UpdateStatus: ").append(getUpdateStatus()).append(",");
if (getLocation() != null)
sb.append("Location: ").append(getLocation());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof ScalingPolicy == false)
return false;
ScalingPolicy other = (ScalingPolicy) obj;
if (other.getFleetId() == null ^ this.getFleetId() == null)
return false;
if (other.getFleetId() != null && other.getFleetId().equals(this.getFleetId()) == false)
return false;
if (other.getFleetArn() == null ^ this.getFleetArn() == null)
return false;
if (other.getFleetArn() != null && other.getFleetArn().equals(this.getFleetArn()) == false)
return false;
if (other.getName() == null ^ this.getName() == null)
return false;
if (other.getName() != null && other.getName().equals(this.getName()) == false)
return false;
if (other.getStatus() == null ^ this.getStatus() == null)
return false;
if (other.getStatus() != null && other.getStatus().equals(this.getStatus()) == false)
return false;
if (other.getScalingAdjustment() == null ^ this.getScalingAdjustment() == null)
return false;
if (other.getScalingAdjustment() != null && other.getScalingAdjustment().equals(this.getScalingAdjustment()) == false)
return false;
if (other.getScalingAdjustmentType() == null ^ this.getScalingAdjustmentType() == null)
return false;
if (other.getScalingAdjustmentType() != null && other.getScalingAdjustmentType().equals(this.getScalingAdjustmentType()) == false)
return false;
if (other.getComparisonOperator() == null ^ this.getComparisonOperator() == null)
return false;
if (other.getComparisonOperator() != null && other.getComparisonOperator().equals(this.getComparisonOperator()) == false)
return false;
if (other.getThreshold() == null ^ this.getThreshold() == null)
return false;
if (other.getThreshold() != null && other.getThreshold().equals(this.getThreshold()) == false)
return false;
if (other.getEvaluationPeriods() == null ^ this.getEvaluationPeriods() == null)
return false;
if (other.getEvaluationPeriods() != null && other.getEvaluationPeriods().equals(this.getEvaluationPeriods()) == false)
return false;
if (other.getMetricName() == null ^ this.getMetricName() == null)
return false;
if (other.getMetricName() != null && other.getMetricName().equals(this.getMetricName()) == false)
return false;
if (other.getPolicyType() == null ^ this.getPolicyType() == null)
return false;
if (other.getPolicyType() != null && other.getPolicyType().equals(this.getPolicyType()) == false)
return false;
if (other.getTargetConfiguration() == null ^ this.getTargetConfiguration() == null)
return false;
if (other.getTargetConfiguration() != null && other.getTargetConfiguration().equals(this.getTargetConfiguration()) == false)
return false;
if (other.getUpdateStatus() == null ^ this.getUpdateStatus() == null)
return false;
if (other.getUpdateStatus() != null && other.getUpdateStatus().equals(this.getUpdateStatus()) == false)
return false;
if (other.getLocation() == null ^ this.getLocation() == null)
return false;
if (other.getLocation() != null && other.getLocation().equals(this.getLocation()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getFleetId() == null) ? 0 : getFleetId().hashCode());
hashCode = prime * hashCode + ((getFleetArn() == null) ? 0 : getFleetArn().hashCode());
hashCode = prime * hashCode + ((getName() == null) ? 0 : getName().hashCode());
hashCode = prime * hashCode + ((getStatus() == null) ? 0 : getStatus().hashCode());
hashCode = prime * hashCode + ((getScalingAdjustment() == null) ? 0 : getScalingAdjustment().hashCode());
hashCode = prime * hashCode + ((getScalingAdjustmentType() == null) ? 0 : getScalingAdjustmentType().hashCode());
hashCode = prime * hashCode + ((getComparisonOperator() == null) ? 0 : getComparisonOperator().hashCode());
hashCode = prime * hashCode + ((getThreshold() == null) ? 0 : getThreshold().hashCode());
hashCode = prime * hashCode + ((getEvaluationPeriods() == null) ? 0 : getEvaluationPeriods().hashCode());
hashCode = prime * hashCode + ((getMetricName() == null) ? 0 : getMetricName().hashCode());
hashCode = prime * hashCode + ((getPolicyType() == null) ? 0 : getPolicyType().hashCode());
hashCode = prime * hashCode + ((getTargetConfiguration() == null) ? 0 : getTargetConfiguration().hashCode());
hashCode = prime * hashCode + ((getUpdateStatus() == null) ? 0 : getUpdateStatus().hashCode());
hashCode = prime * hashCode + ((getLocation() == null) ? 0 : getLocation().hashCode());
return hashCode;
}
@Override
public ScalingPolicy clone() {
try {
return (ScalingPolicy) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.gamelift.model.transform.ScalingPolicyMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}