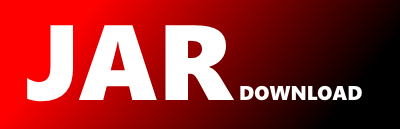
com.amazonaws.services.gamelift.model.ContainerGroupDefinition Maven / Gradle / Ivy
Show all versions of aws-java-sdk-gamelift Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.gamelift.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* This data type is used with the Amazon GameLift containers feature, which is currently in public preview.
*
*
* The properties that describe a container group resource. Container group definition properties can't be updated. To
* change a property, create a new container group definition.
*
*
* Used with: CreateContainerGroupDefinition
*
*
* Returned by: DescribeContainerGroupDefinition, ListContainerGroupDefinitions
*
*
* @see AWS
* API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class ContainerGroupDefinition implements Serializable, Cloneable, StructuredPojo {
/**
*
* The Amazon Resource Name (ARN)
* that is assigned to an Amazon GameLift ContainerGroupDefinition
resource. It uniquely identifies the
* resource across all Amazon Web Services Regions. Format is
* arn:aws:gamelift:<region>::containergroupdefinition/[container group definition name]
.
*
*/
private String containerGroupDefinitionArn;
/**
*
* A time stamp indicating when this data object was created. Format is a number expressed in Unix time as
* milliseconds (for example "1469498468.057"
).
*
*/
private java.util.Date creationTime;
/**
*
* The platform required for all containers in the container group definition.
*
*/
private String operatingSystem;
/**
*
* A descriptive identifier for the container group definition. The name value is unique in an Amazon Web Services
* Region.
*
*/
private String name;
/**
*
* The method for deploying the container group across fleet instances. A replica container group might have
* multiple copies on each fleet instance. A daemon container group maintains only one copy per fleet instance.
*
*/
private String schedulingStrategy;
/**
*
* The amount of memory (in MiB) on a fleet instance to allocate for the container group. All containers in the
* group share these resources.
*
*
* You can set additional limits for each ContainerDefinition in the group. If individual containers have
* limits, this value must meet the following requirements:
*
*
* -
*
* Equal to or greater than the sum of all container-specific soft memory limits in the group.
*
*
* -
*
* Equal to or greater than any container-specific hard limits in the group.
*
*
*
*
* For more details on memory allocation, see the Container fleet design
* guide.
*
*/
private Integer totalMemoryLimit;
/**
*
* The amount of CPU units on a fleet instance to allocate for the container group. All containers in the group
* share these resources. This property is an integer value in CPU units (1 vCPU is equal to 1024 CPU units).
*
*
* You can set additional limits for each ContainerDefinition in the group. If individual containers have
* limits, this value must be equal to or greater than the sum of all container-specific CPU limits in the group.
*
*
* For more details on memory allocation, see the Container fleet design
* guide.
*
*/
private Integer totalCpuLimit;
/**
*
* The set of container definitions that are included in the container group.
*
*/
private java.util.List containerDefinitions;
/**
*
* Current status of the container group definition resource. Values include:
*
*
* -
*
* COPYING
-- Amazon GameLift is in the process of making copies of all container images that are
* defined in the group. While in this state, the resource can't be used to create a container fleet.
*
*
* -
*
* READY
-- Amazon GameLift has copied the registry images for all containers that are defined in the
* group. You can use a container group definition in this status to create a container fleet.
*
*
* -
*
* FAILED
-- Amazon GameLift failed to create a valid container group definition resource. For more
* details on the cause of the failure, see StatusReason
. A container group definition resource in
* failed status will be deleted within a few minutes.
*
*
*
*/
private String status;
/**
*
* Additional information about a container group definition that's in FAILED
status. Possible reasons
* include:
*
*
* -
*
* An internal issue prevented Amazon GameLift from creating the container group definition resource. Delete the
* failed resource and call CreateContainerGroupDefinitionagain.
*
*
* -
*
* An access-denied message means that you don't have permissions to access the container image on ECR. See IAM
* permission examples for help setting up required IAM permissions for Amazon GameLift.
*
*
* -
*
* The ImageUri
value for at least one of the containers in the container group definition was invalid
* or not found in the current Amazon Web Services account.
*
*
* -
*
* At least one of the container images referenced in the container group definition exceeds the allowed size. For
* size limits, see Amazon GameLift endpoints
* and quotas.
*
*
* -
*
* At least one of the container images referenced in the container group definition uses a different operating
* system than the one defined for the container group.
*
*
*
*/
private String statusReason;
/**
*
* The Amazon Resource Name (ARN)
* that is assigned to an Amazon GameLift ContainerGroupDefinition
resource. It uniquely identifies the
* resource across all Amazon Web Services Regions. Format is
* arn:aws:gamelift:<region>::containergroupdefinition/[container group definition name]
.
*
*
* @param containerGroupDefinitionArn
* The Amazon Resource Name (ARN) that is assigned to an
* Amazon GameLift ContainerGroupDefinition
resource. It uniquely identifies the resource across
* all Amazon Web Services Regions. Format is
* arn:aws:gamelift:<region>::containergroupdefinition/[container group definition name]
.
*/
public void setContainerGroupDefinitionArn(String containerGroupDefinitionArn) {
this.containerGroupDefinitionArn = containerGroupDefinitionArn;
}
/**
*
* The Amazon Resource Name (ARN)
* that is assigned to an Amazon GameLift ContainerGroupDefinition
resource. It uniquely identifies the
* resource across all Amazon Web Services Regions. Format is
* arn:aws:gamelift:<region>::containergroupdefinition/[container group definition name]
.
*
*
* @return The Amazon Resource Name (ARN) that is assigned to an
* Amazon GameLift ContainerGroupDefinition
resource. It uniquely identifies the resource
* across all Amazon Web Services Regions. Format is
* arn:aws:gamelift:<region>::containergroupdefinition/[container group definition name]
.
*/
public String getContainerGroupDefinitionArn() {
return this.containerGroupDefinitionArn;
}
/**
*
* The Amazon Resource Name (ARN)
* that is assigned to an Amazon GameLift ContainerGroupDefinition
resource. It uniquely identifies the
* resource across all Amazon Web Services Regions. Format is
* arn:aws:gamelift:<region>::containergroupdefinition/[container group definition name]
.
*
*
* @param containerGroupDefinitionArn
* The Amazon Resource Name (ARN) that is assigned to an
* Amazon GameLift ContainerGroupDefinition
resource. It uniquely identifies the resource across
* all Amazon Web Services Regions. Format is
* arn:aws:gamelift:<region>::containergroupdefinition/[container group definition name]
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ContainerGroupDefinition withContainerGroupDefinitionArn(String containerGroupDefinitionArn) {
setContainerGroupDefinitionArn(containerGroupDefinitionArn);
return this;
}
/**
*
* A time stamp indicating when this data object was created. Format is a number expressed in Unix time as
* milliseconds (for example "1469498468.057"
).
*
*
* @param creationTime
* A time stamp indicating when this data object was created. Format is a number expressed in Unix time as
* milliseconds (for example "1469498468.057"
).
*/
public void setCreationTime(java.util.Date creationTime) {
this.creationTime = creationTime;
}
/**
*
* A time stamp indicating when this data object was created. Format is a number expressed in Unix time as
* milliseconds (for example "1469498468.057"
).
*
*
* @return A time stamp indicating when this data object was created. Format is a number expressed in Unix time as
* milliseconds (for example "1469498468.057"
).
*/
public java.util.Date getCreationTime() {
return this.creationTime;
}
/**
*
* A time stamp indicating when this data object was created. Format is a number expressed in Unix time as
* milliseconds (for example "1469498468.057"
).
*
*
* @param creationTime
* A time stamp indicating when this data object was created. Format is a number expressed in Unix time as
* milliseconds (for example "1469498468.057"
).
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ContainerGroupDefinition withCreationTime(java.util.Date creationTime) {
setCreationTime(creationTime);
return this;
}
/**
*
* The platform required for all containers in the container group definition.
*
*
* @param operatingSystem
* The platform required for all containers in the container group definition.
* @see ContainerOperatingSystem
*/
public void setOperatingSystem(String operatingSystem) {
this.operatingSystem = operatingSystem;
}
/**
*
* The platform required for all containers in the container group definition.
*
*
* @return The platform required for all containers in the container group definition.
* @see ContainerOperatingSystem
*/
public String getOperatingSystem() {
return this.operatingSystem;
}
/**
*
* The platform required for all containers in the container group definition.
*
*
* @param operatingSystem
* The platform required for all containers in the container group definition.
* @return Returns a reference to this object so that method calls can be chained together.
* @see ContainerOperatingSystem
*/
public ContainerGroupDefinition withOperatingSystem(String operatingSystem) {
setOperatingSystem(operatingSystem);
return this;
}
/**
*
* The platform required for all containers in the container group definition.
*
*
* @param operatingSystem
* The platform required for all containers in the container group definition.
* @return Returns a reference to this object so that method calls can be chained together.
* @see ContainerOperatingSystem
*/
public ContainerGroupDefinition withOperatingSystem(ContainerOperatingSystem operatingSystem) {
this.operatingSystem = operatingSystem.toString();
return this;
}
/**
*
* A descriptive identifier for the container group definition. The name value is unique in an Amazon Web Services
* Region.
*
*
* @param name
* A descriptive identifier for the container group definition. The name value is unique in an Amazon Web
* Services Region.
*/
public void setName(String name) {
this.name = name;
}
/**
*
* A descriptive identifier for the container group definition. The name value is unique in an Amazon Web Services
* Region.
*
*
* @return A descriptive identifier for the container group definition. The name value is unique in an Amazon Web
* Services Region.
*/
public String getName() {
return this.name;
}
/**
*
* A descriptive identifier for the container group definition. The name value is unique in an Amazon Web Services
* Region.
*
*
* @param name
* A descriptive identifier for the container group definition. The name value is unique in an Amazon Web
* Services Region.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ContainerGroupDefinition withName(String name) {
setName(name);
return this;
}
/**
*
* The method for deploying the container group across fleet instances. A replica container group might have
* multiple copies on each fleet instance. A daemon container group maintains only one copy per fleet instance.
*
*
* @param schedulingStrategy
* The method for deploying the container group across fleet instances. A replica container group might have
* multiple copies on each fleet instance. A daemon container group maintains only one copy per fleet
* instance.
* @see ContainerSchedulingStrategy
*/
public void setSchedulingStrategy(String schedulingStrategy) {
this.schedulingStrategy = schedulingStrategy;
}
/**
*
* The method for deploying the container group across fleet instances. A replica container group might have
* multiple copies on each fleet instance. A daemon container group maintains only one copy per fleet instance.
*
*
* @return The method for deploying the container group across fleet instances. A replica container group might have
* multiple copies on each fleet instance. A daemon container group maintains only one copy per fleet
* instance.
* @see ContainerSchedulingStrategy
*/
public String getSchedulingStrategy() {
return this.schedulingStrategy;
}
/**
*
* The method for deploying the container group across fleet instances. A replica container group might have
* multiple copies on each fleet instance. A daemon container group maintains only one copy per fleet instance.
*
*
* @param schedulingStrategy
* The method for deploying the container group across fleet instances. A replica container group might have
* multiple copies on each fleet instance. A daemon container group maintains only one copy per fleet
* instance.
* @return Returns a reference to this object so that method calls can be chained together.
* @see ContainerSchedulingStrategy
*/
public ContainerGroupDefinition withSchedulingStrategy(String schedulingStrategy) {
setSchedulingStrategy(schedulingStrategy);
return this;
}
/**
*
* The method for deploying the container group across fleet instances. A replica container group might have
* multiple copies on each fleet instance. A daemon container group maintains only one copy per fleet instance.
*
*
* @param schedulingStrategy
* The method for deploying the container group across fleet instances. A replica container group might have
* multiple copies on each fleet instance. A daemon container group maintains only one copy per fleet
* instance.
* @return Returns a reference to this object so that method calls can be chained together.
* @see ContainerSchedulingStrategy
*/
public ContainerGroupDefinition withSchedulingStrategy(ContainerSchedulingStrategy schedulingStrategy) {
this.schedulingStrategy = schedulingStrategy.toString();
return this;
}
/**
*
* The amount of memory (in MiB) on a fleet instance to allocate for the container group. All containers in the
* group share these resources.
*
*
* You can set additional limits for each ContainerDefinition in the group. If individual containers have
* limits, this value must meet the following requirements:
*
*
* -
*
* Equal to or greater than the sum of all container-specific soft memory limits in the group.
*
*
* -
*
* Equal to or greater than any container-specific hard limits in the group.
*
*
*
*
* For more details on memory allocation, see the Container fleet design
* guide.
*
*
* @param totalMemoryLimit
* The amount of memory (in MiB) on a fleet instance to allocate for the container group. All containers in
* the group share these resources.
*
* You can set additional limits for each ContainerDefinition in the group. If individual containers
* have limits, this value must meet the following requirements:
*
*
* -
*
* Equal to or greater than the sum of all container-specific soft memory limits in the group.
*
*
* -
*
* Equal to or greater than any container-specific hard limits in the group.
*
*
*
*
* For more details on memory allocation, see the Container fleet
* design guide.
*/
public void setTotalMemoryLimit(Integer totalMemoryLimit) {
this.totalMemoryLimit = totalMemoryLimit;
}
/**
*
* The amount of memory (in MiB) on a fleet instance to allocate for the container group. All containers in the
* group share these resources.
*
*
* You can set additional limits for each ContainerDefinition in the group. If individual containers have
* limits, this value must meet the following requirements:
*
*
* -
*
* Equal to or greater than the sum of all container-specific soft memory limits in the group.
*
*
* -
*
* Equal to or greater than any container-specific hard limits in the group.
*
*
*
*
* For more details on memory allocation, see the Container fleet design
* guide.
*
*
* @return The amount of memory (in MiB) on a fleet instance to allocate for the container group. All containers in
* the group share these resources.
*
* You can set additional limits for each ContainerDefinition in the group. If individual containers
* have limits, this value must meet the following requirements:
*
*
* -
*
* Equal to or greater than the sum of all container-specific soft memory limits in the group.
*
*
* -
*
* Equal to or greater than any container-specific hard limits in the group.
*
*
*
*
* For more details on memory allocation, see the Container fleet
* design guide.
*/
public Integer getTotalMemoryLimit() {
return this.totalMemoryLimit;
}
/**
*
* The amount of memory (in MiB) on a fleet instance to allocate for the container group. All containers in the
* group share these resources.
*
*
* You can set additional limits for each ContainerDefinition in the group. If individual containers have
* limits, this value must meet the following requirements:
*
*
* -
*
* Equal to or greater than the sum of all container-specific soft memory limits in the group.
*
*
* -
*
* Equal to or greater than any container-specific hard limits in the group.
*
*
*
*
* For more details on memory allocation, see the Container fleet design
* guide.
*
*
* @param totalMemoryLimit
* The amount of memory (in MiB) on a fleet instance to allocate for the container group. All containers in
* the group share these resources.
*
* You can set additional limits for each ContainerDefinition in the group. If individual containers
* have limits, this value must meet the following requirements:
*
*
* -
*
* Equal to or greater than the sum of all container-specific soft memory limits in the group.
*
*
* -
*
* Equal to or greater than any container-specific hard limits in the group.
*
*
*
*
* For more details on memory allocation, see the Container fleet
* design guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ContainerGroupDefinition withTotalMemoryLimit(Integer totalMemoryLimit) {
setTotalMemoryLimit(totalMemoryLimit);
return this;
}
/**
*
* The amount of CPU units on a fleet instance to allocate for the container group. All containers in the group
* share these resources. This property is an integer value in CPU units (1 vCPU is equal to 1024 CPU units).
*
*
* You can set additional limits for each ContainerDefinition in the group. If individual containers have
* limits, this value must be equal to or greater than the sum of all container-specific CPU limits in the group.
*
*
* For more details on memory allocation, see the Container fleet design
* guide.
*
*
* @param totalCpuLimit
* The amount of CPU units on a fleet instance to allocate for the container group. All containers in the
* group share these resources. This property is an integer value in CPU units (1 vCPU is equal to 1024 CPU
* units).
*
* You can set additional limits for each ContainerDefinition in the group. If individual containers
* have limits, this value must be equal to or greater than the sum of all container-specific CPU limits in
* the group.
*
*
* For more details on memory allocation, see the Container fleet
* design guide.
*/
public void setTotalCpuLimit(Integer totalCpuLimit) {
this.totalCpuLimit = totalCpuLimit;
}
/**
*
* The amount of CPU units on a fleet instance to allocate for the container group. All containers in the group
* share these resources. This property is an integer value in CPU units (1 vCPU is equal to 1024 CPU units).
*
*
* You can set additional limits for each ContainerDefinition in the group. If individual containers have
* limits, this value must be equal to or greater than the sum of all container-specific CPU limits in the group.
*
*
* For more details on memory allocation, see the Container fleet design
* guide.
*
*
* @return The amount of CPU units on a fleet instance to allocate for the container group. All containers in the
* group share these resources. This property is an integer value in CPU units (1 vCPU is equal to 1024 CPU
* units).
*
* You can set additional limits for each ContainerDefinition in the group. If individual containers
* have limits, this value must be equal to or greater than the sum of all container-specific CPU limits in
* the group.
*
*
* For more details on memory allocation, see the Container fleet
* design guide.
*/
public Integer getTotalCpuLimit() {
return this.totalCpuLimit;
}
/**
*
* The amount of CPU units on a fleet instance to allocate for the container group. All containers in the group
* share these resources. This property is an integer value in CPU units (1 vCPU is equal to 1024 CPU units).
*
*
* You can set additional limits for each ContainerDefinition in the group. If individual containers have
* limits, this value must be equal to or greater than the sum of all container-specific CPU limits in the group.
*
*
* For more details on memory allocation, see the Container fleet design
* guide.
*
*
* @param totalCpuLimit
* The amount of CPU units on a fleet instance to allocate for the container group. All containers in the
* group share these resources. This property is an integer value in CPU units (1 vCPU is equal to 1024 CPU
* units).
*
* You can set additional limits for each ContainerDefinition in the group. If individual containers
* have limits, this value must be equal to or greater than the sum of all container-specific CPU limits in
* the group.
*
*
* For more details on memory allocation, see the Container fleet
* design guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ContainerGroupDefinition withTotalCpuLimit(Integer totalCpuLimit) {
setTotalCpuLimit(totalCpuLimit);
return this;
}
/**
*
* The set of container definitions that are included in the container group.
*
*
* @return The set of container definitions that are included in the container group.
*/
public java.util.List getContainerDefinitions() {
return containerDefinitions;
}
/**
*
* The set of container definitions that are included in the container group.
*
*
* @param containerDefinitions
* The set of container definitions that are included in the container group.
*/
public void setContainerDefinitions(java.util.Collection containerDefinitions) {
if (containerDefinitions == null) {
this.containerDefinitions = null;
return;
}
this.containerDefinitions = new java.util.ArrayList(containerDefinitions);
}
/**
*
* The set of container definitions that are included in the container group.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setContainerDefinitions(java.util.Collection)} or {@link #withContainerDefinitions(java.util.Collection)}
* if you want to override the existing values.
*
*
* @param containerDefinitions
* The set of container definitions that are included in the container group.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ContainerGroupDefinition withContainerDefinitions(ContainerDefinition... containerDefinitions) {
if (this.containerDefinitions == null) {
setContainerDefinitions(new java.util.ArrayList(containerDefinitions.length));
}
for (ContainerDefinition ele : containerDefinitions) {
this.containerDefinitions.add(ele);
}
return this;
}
/**
*
* The set of container definitions that are included in the container group.
*
*
* @param containerDefinitions
* The set of container definitions that are included in the container group.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ContainerGroupDefinition withContainerDefinitions(java.util.Collection containerDefinitions) {
setContainerDefinitions(containerDefinitions);
return this;
}
/**
*
* Current status of the container group definition resource. Values include:
*
*
* -
*
* COPYING
-- Amazon GameLift is in the process of making copies of all container images that are
* defined in the group. While in this state, the resource can't be used to create a container fleet.
*
*
* -
*
* READY
-- Amazon GameLift has copied the registry images for all containers that are defined in the
* group. You can use a container group definition in this status to create a container fleet.
*
*
* -
*
* FAILED
-- Amazon GameLift failed to create a valid container group definition resource. For more
* details on the cause of the failure, see StatusReason
. A container group definition resource in
* failed status will be deleted within a few minutes.
*
*
*
*
* @param status
* Current status of the container group definition resource. Values include:
*
* -
*
* COPYING
-- Amazon GameLift is in the process of making copies of all container images that
* are defined in the group. While in this state, the resource can't be used to create a container fleet.
*
*
* -
*
* READY
-- Amazon GameLift has copied the registry images for all containers that are defined
* in the group. You can use a container group definition in this status to create a container fleet.
*
*
* -
*
* FAILED
-- Amazon GameLift failed to create a valid container group definition resource. For
* more details on the cause of the failure, see StatusReason
. A container group definition
* resource in failed status will be deleted within a few minutes.
*
*
* @see ContainerGroupDefinitionStatus
*/
public void setStatus(String status) {
this.status = status;
}
/**
*
* Current status of the container group definition resource. Values include:
*
*
* -
*
* COPYING
-- Amazon GameLift is in the process of making copies of all container images that are
* defined in the group. While in this state, the resource can't be used to create a container fleet.
*
*
* -
*
* READY
-- Amazon GameLift has copied the registry images for all containers that are defined in the
* group. You can use a container group definition in this status to create a container fleet.
*
*
* -
*
* FAILED
-- Amazon GameLift failed to create a valid container group definition resource. For more
* details on the cause of the failure, see StatusReason
. A container group definition resource in
* failed status will be deleted within a few minutes.
*
*
*
*
* @return Current status of the container group definition resource. Values include:
*
* -
*
* COPYING
-- Amazon GameLift is in the process of making copies of all container images that
* are defined in the group. While in this state, the resource can't be used to create a container fleet.
*
*
* -
*
* READY
-- Amazon GameLift has copied the registry images for all containers that are defined
* in the group. You can use a container group definition in this status to create a container fleet.
*
*
* -
*
* FAILED
-- Amazon GameLift failed to create a valid container group definition resource. For
* more details on the cause of the failure, see StatusReason
. A container group definition
* resource in failed status will be deleted within a few minutes.
*
*
* @see ContainerGroupDefinitionStatus
*/
public String getStatus() {
return this.status;
}
/**
*
* Current status of the container group definition resource. Values include:
*
*
* -
*
* COPYING
-- Amazon GameLift is in the process of making copies of all container images that are
* defined in the group. While in this state, the resource can't be used to create a container fleet.
*
*
* -
*
* READY
-- Amazon GameLift has copied the registry images for all containers that are defined in the
* group. You can use a container group definition in this status to create a container fleet.
*
*
* -
*
* FAILED
-- Amazon GameLift failed to create a valid container group definition resource. For more
* details on the cause of the failure, see StatusReason
. A container group definition resource in
* failed status will be deleted within a few minutes.
*
*
*
*
* @param status
* Current status of the container group definition resource. Values include:
*
* -
*
* COPYING
-- Amazon GameLift is in the process of making copies of all container images that
* are defined in the group. While in this state, the resource can't be used to create a container fleet.
*
*
* -
*
* READY
-- Amazon GameLift has copied the registry images for all containers that are defined
* in the group. You can use a container group definition in this status to create a container fleet.
*
*
* -
*
* FAILED
-- Amazon GameLift failed to create a valid container group definition resource. For
* more details on the cause of the failure, see StatusReason
. A container group definition
* resource in failed status will be deleted within a few minutes.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see ContainerGroupDefinitionStatus
*/
public ContainerGroupDefinition withStatus(String status) {
setStatus(status);
return this;
}
/**
*
* Current status of the container group definition resource. Values include:
*
*
* -
*
* COPYING
-- Amazon GameLift is in the process of making copies of all container images that are
* defined in the group. While in this state, the resource can't be used to create a container fleet.
*
*
* -
*
* READY
-- Amazon GameLift has copied the registry images for all containers that are defined in the
* group. You can use a container group definition in this status to create a container fleet.
*
*
* -
*
* FAILED
-- Amazon GameLift failed to create a valid container group definition resource. For more
* details on the cause of the failure, see StatusReason
. A container group definition resource in
* failed status will be deleted within a few minutes.
*
*
*
*
* @param status
* Current status of the container group definition resource. Values include:
*
* -
*
* COPYING
-- Amazon GameLift is in the process of making copies of all container images that
* are defined in the group. While in this state, the resource can't be used to create a container fleet.
*
*
* -
*
* READY
-- Amazon GameLift has copied the registry images for all containers that are defined
* in the group. You can use a container group definition in this status to create a container fleet.
*
*
* -
*
* FAILED
-- Amazon GameLift failed to create a valid container group definition resource. For
* more details on the cause of the failure, see StatusReason
. A container group definition
* resource in failed status will be deleted within a few minutes.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see ContainerGroupDefinitionStatus
*/
public ContainerGroupDefinition withStatus(ContainerGroupDefinitionStatus status) {
this.status = status.toString();
return this;
}
/**
*
* Additional information about a container group definition that's in FAILED
status. Possible reasons
* include:
*
*
* -
*
* An internal issue prevented Amazon GameLift from creating the container group definition resource. Delete the
* failed resource and call CreateContainerGroupDefinitionagain.
*
*
* -
*
* An access-denied message means that you don't have permissions to access the container image on ECR. See IAM
* permission examples for help setting up required IAM permissions for Amazon GameLift.
*
*
* -
*
* The ImageUri
value for at least one of the containers in the container group definition was invalid
* or not found in the current Amazon Web Services account.
*
*
* -
*
* At least one of the container images referenced in the container group definition exceeds the allowed size. For
* size limits, see Amazon GameLift endpoints
* and quotas.
*
*
* -
*
* At least one of the container images referenced in the container group definition uses a different operating
* system than the one defined for the container group.
*
*
*
*
* @param statusReason
* Additional information about a container group definition that's in FAILED
status. Possible
* reasons include:
*
* -
*
* An internal issue prevented Amazon GameLift from creating the container group definition resource. Delete
* the failed resource and call CreateContainerGroupDefinitionagain.
*
*
* -
*
* An access-denied message means that you don't have permissions to access the container image on ECR. See
*
* IAM permission examples for help setting up required IAM permissions for Amazon GameLift.
*
*
* -
*
* The ImageUri
value for at least one of the containers in the container group definition was
* invalid or not found in the current Amazon Web Services account.
*
*
* -
*
* At least one of the container images referenced in the container group definition exceeds the allowed
* size. For size limits, see Amazon
* GameLift endpoints and quotas.
*
*
* -
*
* At least one of the container images referenced in the container group definition uses a different
* operating system than the one defined for the container group.
*
*
*/
public void setStatusReason(String statusReason) {
this.statusReason = statusReason;
}
/**
*
* Additional information about a container group definition that's in FAILED
status. Possible reasons
* include:
*
*
* -
*
* An internal issue prevented Amazon GameLift from creating the container group definition resource. Delete the
* failed resource and call CreateContainerGroupDefinitionagain.
*
*
* -
*
* An access-denied message means that you don't have permissions to access the container image on ECR. See IAM
* permission examples for help setting up required IAM permissions for Amazon GameLift.
*
*
* -
*
* The ImageUri
value for at least one of the containers in the container group definition was invalid
* or not found in the current Amazon Web Services account.
*
*
* -
*
* At least one of the container images referenced in the container group definition exceeds the allowed size. For
* size limits, see Amazon GameLift endpoints
* and quotas.
*
*
* -
*
* At least one of the container images referenced in the container group definition uses a different operating
* system than the one defined for the container group.
*
*
*
*
* @return Additional information about a container group definition that's in FAILED
status. Possible
* reasons include:
*
* -
*
* An internal issue prevented Amazon GameLift from creating the container group definition resource. Delete
* the failed resource and call CreateContainerGroupDefinitionagain.
*
*
* -
*
* An access-denied message means that you don't have permissions to access the container image on ECR. See
*
* IAM permission examples for help setting up required IAM permissions for Amazon GameLift.
*
*
* -
*
* The ImageUri
value for at least one of the containers in the container group definition was
* invalid or not found in the current Amazon Web Services account.
*
*
* -
*
* At least one of the container images referenced in the container group definition exceeds the allowed
* size. For size limits, see Amazon
* GameLift endpoints and quotas.
*
*
* -
*
* At least one of the container images referenced in the container group definition uses a different
* operating system than the one defined for the container group.
*
*
*/
public String getStatusReason() {
return this.statusReason;
}
/**
*
* Additional information about a container group definition that's in FAILED
status. Possible reasons
* include:
*
*
* -
*
* An internal issue prevented Amazon GameLift from creating the container group definition resource. Delete the
* failed resource and call CreateContainerGroupDefinitionagain.
*
*
* -
*
* An access-denied message means that you don't have permissions to access the container image on ECR. See IAM
* permission examples for help setting up required IAM permissions for Amazon GameLift.
*
*
* -
*
* The ImageUri
value for at least one of the containers in the container group definition was invalid
* or not found in the current Amazon Web Services account.
*
*
* -
*
* At least one of the container images referenced in the container group definition exceeds the allowed size. For
* size limits, see Amazon GameLift endpoints
* and quotas.
*
*
* -
*
* At least one of the container images referenced in the container group definition uses a different operating
* system than the one defined for the container group.
*
*
*
*
* @param statusReason
* Additional information about a container group definition that's in FAILED
status. Possible
* reasons include:
*
* -
*
* An internal issue prevented Amazon GameLift from creating the container group definition resource. Delete
* the failed resource and call CreateContainerGroupDefinitionagain.
*
*
* -
*
* An access-denied message means that you don't have permissions to access the container image on ECR. See
*
* IAM permission examples for help setting up required IAM permissions for Amazon GameLift.
*
*
* -
*
* The ImageUri
value for at least one of the containers in the container group definition was
* invalid or not found in the current Amazon Web Services account.
*
*
* -
*
* At least one of the container images referenced in the container group definition exceeds the allowed
* size. For size limits, see Amazon
* GameLift endpoints and quotas.
*
*
* -
*
* At least one of the container images referenced in the container group definition uses a different
* operating system than the one defined for the container group.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ContainerGroupDefinition withStatusReason(String statusReason) {
setStatusReason(statusReason);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getContainerGroupDefinitionArn() != null)
sb.append("ContainerGroupDefinitionArn: ").append(getContainerGroupDefinitionArn()).append(",");
if (getCreationTime() != null)
sb.append("CreationTime: ").append(getCreationTime()).append(",");
if (getOperatingSystem() != null)
sb.append("OperatingSystem: ").append(getOperatingSystem()).append(",");
if (getName() != null)
sb.append("Name: ").append(getName()).append(",");
if (getSchedulingStrategy() != null)
sb.append("SchedulingStrategy: ").append(getSchedulingStrategy()).append(",");
if (getTotalMemoryLimit() != null)
sb.append("TotalMemoryLimit: ").append(getTotalMemoryLimit()).append(",");
if (getTotalCpuLimit() != null)
sb.append("TotalCpuLimit: ").append(getTotalCpuLimit()).append(",");
if (getContainerDefinitions() != null)
sb.append("ContainerDefinitions: ").append(getContainerDefinitions()).append(",");
if (getStatus() != null)
sb.append("Status: ").append(getStatus()).append(",");
if (getStatusReason() != null)
sb.append("StatusReason: ").append(getStatusReason());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof ContainerGroupDefinition == false)
return false;
ContainerGroupDefinition other = (ContainerGroupDefinition) obj;
if (other.getContainerGroupDefinitionArn() == null ^ this.getContainerGroupDefinitionArn() == null)
return false;
if (other.getContainerGroupDefinitionArn() != null && other.getContainerGroupDefinitionArn().equals(this.getContainerGroupDefinitionArn()) == false)
return false;
if (other.getCreationTime() == null ^ this.getCreationTime() == null)
return false;
if (other.getCreationTime() != null && other.getCreationTime().equals(this.getCreationTime()) == false)
return false;
if (other.getOperatingSystem() == null ^ this.getOperatingSystem() == null)
return false;
if (other.getOperatingSystem() != null && other.getOperatingSystem().equals(this.getOperatingSystem()) == false)
return false;
if (other.getName() == null ^ this.getName() == null)
return false;
if (other.getName() != null && other.getName().equals(this.getName()) == false)
return false;
if (other.getSchedulingStrategy() == null ^ this.getSchedulingStrategy() == null)
return false;
if (other.getSchedulingStrategy() != null && other.getSchedulingStrategy().equals(this.getSchedulingStrategy()) == false)
return false;
if (other.getTotalMemoryLimit() == null ^ this.getTotalMemoryLimit() == null)
return false;
if (other.getTotalMemoryLimit() != null && other.getTotalMemoryLimit().equals(this.getTotalMemoryLimit()) == false)
return false;
if (other.getTotalCpuLimit() == null ^ this.getTotalCpuLimit() == null)
return false;
if (other.getTotalCpuLimit() != null && other.getTotalCpuLimit().equals(this.getTotalCpuLimit()) == false)
return false;
if (other.getContainerDefinitions() == null ^ this.getContainerDefinitions() == null)
return false;
if (other.getContainerDefinitions() != null && other.getContainerDefinitions().equals(this.getContainerDefinitions()) == false)
return false;
if (other.getStatus() == null ^ this.getStatus() == null)
return false;
if (other.getStatus() != null && other.getStatus().equals(this.getStatus()) == false)
return false;
if (other.getStatusReason() == null ^ this.getStatusReason() == null)
return false;
if (other.getStatusReason() != null && other.getStatusReason().equals(this.getStatusReason()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getContainerGroupDefinitionArn() == null) ? 0 : getContainerGroupDefinitionArn().hashCode());
hashCode = prime * hashCode + ((getCreationTime() == null) ? 0 : getCreationTime().hashCode());
hashCode = prime * hashCode + ((getOperatingSystem() == null) ? 0 : getOperatingSystem().hashCode());
hashCode = prime * hashCode + ((getName() == null) ? 0 : getName().hashCode());
hashCode = prime * hashCode + ((getSchedulingStrategy() == null) ? 0 : getSchedulingStrategy().hashCode());
hashCode = prime * hashCode + ((getTotalMemoryLimit() == null) ? 0 : getTotalMemoryLimit().hashCode());
hashCode = prime * hashCode + ((getTotalCpuLimit() == null) ? 0 : getTotalCpuLimit().hashCode());
hashCode = prime * hashCode + ((getContainerDefinitions() == null) ? 0 : getContainerDefinitions().hashCode());
hashCode = prime * hashCode + ((getStatus() == null) ? 0 : getStatus().hashCode());
hashCode = prime * hashCode + ((getStatusReason() == null) ? 0 : getStatusReason().hashCode());
return hashCode;
}
@Override
public ContainerGroupDefinition clone() {
try {
return (ContainerGroupDefinition) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.gamelift.model.transform.ContainerGroupDefinitionMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}