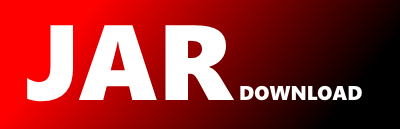
com.amazonaws.services.gamelift.model.SearchGameSessionsRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-gamelift Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.gamelift.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class SearchGameSessionsRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* A unique identifier for the fleet to search for active game sessions. You can use either the fleet ID or ARN
* value. Each request must reference either a fleet ID or alias ID, but not both.
*
*/
private String fleetId;
/**
*
* A unique identifier for the alias associated with the fleet to search for active game sessions. You can use
* either the alias ID or ARN value. Each request must reference either a fleet ID or alias ID, but not both.
*
*/
private String aliasId;
/**
*
* A fleet location to search for game sessions. You can specify a fleet's home Region or a remote location. Use the
* Amazon Web Services Region code format, such as us-west-2
.
*
*/
private String location;
/**
*
* String containing the search criteria for the session search. If no filter expression is included, the request
* returns results for all game sessions in the fleet that are in ACTIVE
status.
*
*
* A filter expression can contain one or multiple conditions. Each condition consists of the following:
*
*
* -
*
* Operand -- Name of a game session attribute. Valid values are gameSessionName
,
* gameSessionId
, gameSessionProperties
, maximumSessions
,
* creationTimeMillis
, playerSessionCount
, hasAvailablePlayerSessions
.
*
*
* -
*
* Comparator -- Valid comparators are: =
, <>
, <
,
* >
, <=
, >=
.
*
*
* -
*
* Value -- Value to be searched for. Values may be numbers, boolean values (true/false) or strings depending
* on the operand. String values are case sensitive and must be enclosed in single quotes. Special characters must
* be escaped. Boolean and string values can only be used with the comparators =
and
* <>
. For example, the following filter expression searches on gameSessionName
: "
* FilterExpression": "gameSessionName = 'Matt\\'s Awesome Game 1'"
.
*
*
*
*
* To chain multiple conditions in a single expression, use the logical keywords AND
, OR
,
* and NOT
and parentheses as needed. For example: x AND y AND NOT z
,
* NOT (x OR y)
.
*
*
* Session search evaluates conditions from left to right using the following precedence rules:
*
*
* -
*
* =
, <>
, <
, >
, <=
,
* >=
*
*
* -
*
* Parentheses
*
*
* -
*
* NOT
*
*
* -
*
* AND
*
*
* -
*
* OR
*
*
*
*
* For example, this filter expression retrieves game sessions hosting at least ten players that have an open player
* slot: "maximumSessions>=10 AND hasAvailablePlayerSessions=true"
.
*
*/
private String filterExpression;
/**
*
* Instructions on how to sort the search results. If no sort expression is included, the request returns results in
* random order. A sort expression consists of the following elements:
*
*
* -
*
* Operand -- Name of a game session attribute. Valid values are gameSessionName
,
* gameSessionId
, gameSessionProperties
, maximumSessions
,
* creationTimeMillis
, playerSessionCount
, hasAvailablePlayerSessions
.
*
*
* -
*
* Order -- Valid sort orders are ASC
(ascending) and DESC
(descending).
*
*
*
*
* For example, this sort expression returns the oldest active sessions first:
* "SortExpression": "creationTimeMillis ASC"
. Results with a null value for the sort operand are
* returned at the end of the list.
*
*/
private String sortExpression;
/**
*
* The maximum number of results to return. Use this parameter with NextToken
to get results as a set
* of sequential pages. The maximum number of results returned is 20, even if this value is not set or is set higher
* than 20.
*
*/
private Integer limit;
/**
*
* A token that indicates the start of the next sequential page of results. Use the token that is returned with a
* previous call to this operation. To start at the beginning of the result set, do not specify a value.
*
*/
private String nextToken;
/**
*
* A unique identifier for the fleet to search for active game sessions. You can use either the fleet ID or ARN
* value. Each request must reference either a fleet ID or alias ID, but not both.
*
*
* @param fleetId
* A unique identifier for the fleet to search for active game sessions. You can use either the fleet ID or
* ARN value. Each request must reference either a fleet ID or alias ID, but not both.
*/
public void setFleetId(String fleetId) {
this.fleetId = fleetId;
}
/**
*
* A unique identifier for the fleet to search for active game sessions. You can use either the fleet ID or ARN
* value. Each request must reference either a fleet ID or alias ID, but not both.
*
*
* @return A unique identifier for the fleet to search for active game sessions. You can use either the fleet ID or
* ARN value. Each request must reference either a fleet ID or alias ID, but not both.
*/
public String getFleetId() {
return this.fleetId;
}
/**
*
* A unique identifier for the fleet to search for active game sessions. You can use either the fleet ID or ARN
* value. Each request must reference either a fleet ID or alias ID, but not both.
*
*
* @param fleetId
* A unique identifier for the fleet to search for active game sessions. You can use either the fleet ID or
* ARN value. Each request must reference either a fleet ID or alias ID, but not both.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public SearchGameSessionsRequest withFleetId(String fleetId) {
setFleetId(fleetId);
return this;
}
/**
*
* A unique identifier for the alias associated with the fleet to search for active game sessions. You can use
* either the alias ID or ARN value. Each request must reference either a fleet ID or alias ID, but not both.
*
*
* @param aliasId
* A unique identifier for the alias associated with the fleet to search for active game sessions. You can
* use either the alias ID or ARN value. Each request must reference either a fleet ID or alias ID, but not
* both.
*/
public void setAliasId(String aliasId) {
this.aliasId = aliasId;
}
/**
*
* A unique identifier for the alias associated with the fleet to search for active game sessions. You can use
* either the alias ID or ARN value. Each request must reference either a fleet ID or alias ID, but not both.
*
*
* @return A unique identifier for the alias associated with the fleet to search for active game sessions. You can
* use either the alias ID or ARN value. Each request must reference either a fleet ID or alias ID, but not
* both.
*/
public String getAliasId() {
return this.aliasId;
}
/**
*
* A unique identifier for the alias associated with the fleet to search for active game sessions. You can use
* either the alias ID or ARN value. Each request must reference either a fleet ID or alias ID, but not both.
*
*
* @param aliasId
* A unique identifier for the alias associated with the fleet to search for active game sessions. You can
* use either the alias ID or ARN value. Each request must reference either a fleet ID or alias ID, but not
* both.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public SearchGameSessionsRequest withAliasId(String aliasId) {
setAliasId(aliasId);
return this;
}
/**
*
* A fleet location to search for game sessions. You can specify a fleet's home Region or a remote location. Use the
* Amazon Web Services Region code format, such as us-west-2
.
*
*
* @param location
* A fleet location to search for game sessions. You can specify a fleet's home Region or a remote location.
* Use the Amazon Web Services Region code format, such as us-west-2
.
*/
public void setLocation(String location) {
this.location = location;
}
/**
*
* A fleet location to search for game sessions. You can specify a fleet's home Region or a remote location. Use the
* Amazon Web Services Region code format, such as us-west-2
.
*
*
* @return A fleet location to search for game sessions. You can specify a fleet's home Region or a remote location.
* Use the Amazon Web Services Region code format, such as us-west-2
.
*/
public String getLocation() {
return this.location;
}
/**
*
* A fleet location to search for game sessions. You can specify a fleet's home Region or a remote location. Use the
* Amazon Web Services Region code format, such as us-west-2
.
*
*
* @param location
* A fleet location to search for game sessions. You can specify a fleet's home Region or a remote location.
* Use the Amazon Web Services Region code format, such as us-west-2
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public SearchGameSessionsRequest withLocation(String location) {
setLocation(location);
return this;
}
/**
*
* String containing the search criteria for the session search. If no filter expression is included, the request
* returns results for all game sessions in the fleet that are in ACTIVE
status.
*
*
* A filter expression can contain one or multiple conditions. Each condition consists of the following:
*
*
* -
*
* Operand -- Name of a game session attribute. Valid values are gameSessionName
,
* gameSessionId
, gameSessionProperties
, maximumSessions
,
* creationTimeMillis
, playerSessionCount
, hasAvailablePlayerSessions
.
*
*
* -
*
* Comparator -- Valid comparators are: =
, <>
, <
,
* >
, <=
, >=
.
*
*
* -
*
* Value -- Value to be searched for. Values may be numbers, boolean values (true/false) or strings depending
* on the operand. String values are case sensitive and must be enclosed in single quotes. Special characters must
* be escaped. Boolean and string values can only be used with the comparators =
and
* <>
. For example, the following filter expression searches on gameSessionName
: "
* FilterExpression": "gameSessionName = 'Matt\\'s Awesome Game 1'"
.
*
*
*
*
* To chain multiple conditions in a single expression, use the logical keywords AND
, OR
,
* and NOT
and parentheses as needed. For example: x AND y AND NOT z
,
* NOT (x OR y)
.
*
*
* Session search evaluates conditions from left to right using the following precedence rules:
*
*
* -
*
* =
, <>
, <
, >
, <=
,
* >=
*
*
* -
*
* Parentheses
*
*
* -
*
* NOT
*
*
* -
*
* AND
*
*
* -
*
* OR
*
*
*
*
* For example, this filter expression retrieves game sessions hosting at least ten players that have an open player
* slot: "maximumSessions>=10 AND hasAvailablePlayerSessions=true"
.
*
*
* @param filterExpression
* String containing the search criteria for the session search. If no filter expression is included, the
* request returns results for all game sessions in the fleet that are in ACTIVE
status.
*
* A filter expression can contain one or multiple conditions. Each condition consists of the following:
*
*
* -
*
* Operand -- Name of a game session attribute. Valid values are gameSessionName
,
* gameSessionId
, gameSessionProperties
, maximumSessions
,
* creationTimeMillis
, playerSessionCount
, hasAvailablePlayerSessions
.
*
*
* -
*
* Comparator -- Valid comparators are: =
, <>
, <
,
* >
, <=
, >=
.
*
*
* -
*
* Value -- Value to be searched for. Values may be numbers, boolean values (true/false) or strings
* depending on the operand. String values are case sensitive and must be enclosed in single quotes. Special
* characters must be escaped. Boolean and string values can only be used with the comparators =
* and <>
. For example, the following filter expression searches on
* gameSessionName
: "
* FilterExpression": "gameSessionName = 'Matt\\'s Awesome Game 1'"
.
*
*
*
*
* To chain multiple conditions in a single expression, use the logical keywords AND
,
* OR
, and NOT
and parentheses as needed. For example:
* x AND y AND NOT z
, NOT (x OR y)
.
*
*
* Session search evaluates conditions from left to right using the following precedence rules:
*
*
* -
*
* =
, <>
, <
, >
, <=
,
* >=
*
*
* -
*
* Parentheses
*
*
* -
*
* NOT
*
*
* -
*
* AND
*
*
* -
*
* OR
*
*
*
*
* For example, this filter expression retrieves game sessions hosting at least ten players that have an open
* player slot: "maximumSessions>=10 AND hasAvailablePlayerSessions=true"
.
*/
public void setFilterExpression(String filterExpression) {
this.filterExpression = filterExpression;
}
/**
*
* String containing the search criteria for the session search. If no filter expression is included, the request
* returns results for all game sessions in the fleet that are in ACTIVE
status.
*
*
* A filter expression can contain one or multiple conditions. Each condition consists of the following:
*
*
* -
*
* Operand -- Name of a game session attribute. Valid values are gameSessionName
,
* gameSessionId
, gameSessionProperties
, maximumSessions
,
* creationTimeMillis
, playerSessionCount
, hasAvailablePlayerSessions
.
*
*
* -
*
* Comparator -- Valid comparators are: =
, <>
, <
,
* >
, <=
, >=
.
*
*
* -
*
* Value -- Value to be searched for. Values may be numbers, boolean values (true/false) or strings depending
* on the operand. String values are case sensitive and must be enclosed in single quotes. Special characters must
* be escaped. Boolean and string values can only be used with the comparators =
and
* <>
. For example, the following filter expression searches on gameSessionName
: "
* FilterExpression": "gameSessionName = 'Matt\\'s Awesome Game 1'"
.
*
*
*
*
* To chain multiple conditions in a single expression, use the logical keywords AND
, OR
,
* and NOT
and parentheses as needed. For example: x AND y AND NOT z
,
* NOT (x OR y)
.
*
*
* Session search evaluates conditions from left to right using the following precedence rules:
*
*
* -
*
* =
, <>
, <
, >
, <=
,
* >=
*
*
* -
*
* Parentheses
*
*
* -
*
* NOT
*
*
* -
*
* AND
*
*
* -
*
* OR
*
*
*
*
* For example, this filter expression retrieves game sessions hosting at least ten players that have an open player
* slot: "maximumSessions>=10 AND hasAvailablePlayerSessions=true"
.
*
*
* @return String containing the search criteria for the session search. If no filter expression is included, the
* request returns results for all game sessions in the fleet that are in ACTIVE
status.
*
* A filter expression can contain one or multiple conditions. Each condition consists of the following:
*
*
* -
*
* Operand -- Name of a game session attribute. Valid values are gameSessionName
,
* gameSessionId
, gameSessionProperties
, maximumSessions
,
* creationTimeMillis
, playerSessionCount
, hasAvailablePlayerSessions
* .
*
*
* -
*
* Comparator -- Valid comparators are: =
, <>
, <
,
* >
, <=
, >=
.
*
*
* -
*
* Value -- Value to be searched for. Values may be numbers, boolean values (true/false) or strings
* depending on the operand. String values are case sensitive and must be enclosed in single quotes. Special
* characters must be escaped. Boolean and string values can only be used with the comparators
* =
and <>
. For example, the following filter expression searches on
* gameSessionName
: "
* FilterExpression": "gameSessionName = 'Matt\\'s Awesome Game 1'"
.
*
*
*
*
* To chain multiple conditions in a single expression, use the logical keywords AND
,
* OR
, and NOT
and parentheses as needed. For example:
* x AND y AND NOT z
, NOT (x OR y)
.
*
*
* Session search evaluates conditions from left to right using the following precedence rules:
*
*
* -
*
* =
, <>
, <
, >
, <=
,
* >=
*
*
* -
*
* Parentheses
*
*
* -
*
* NOT
*
*
* -
*
* AND
*
*
* -
*
* OR
*
*
*
*
* For example, this filter expression retrieves game sessions hosting at least ten players that have an
* open player slot: "maximumSessions>=10 AND hasAvailablePlayerSessions=true"
.
*/
public String getFilterExpression() {
return this.filterExpression;
}
/**
*
* String containing the search criteria for the session search. If no filter expression is included, the request
* returns results for all game sessions in the fleet that are in ACTIVE
status.
*
*
* A filter expression can contain one or multiple conditions. Each condition consists of the following:
*
*
* -
*
* Operand -- Name of a game session attribute. Valid values are gameSessionName
,
* gameSessionId
, gameSessionProperties
, maximumSessions
,
* creationTimeMillis
, playerSessionCount
, hasAvailablePlayerSessions
.
*
*
* -
*
* Comparator -- Valid comparators are: =
, <>
, <
,
* >
, <=
, >=
.
*
*
* -
*
* Value -- Value to be searched for. Values may be numbers, boolean values (true/false) or strings depending
* on the operand. String values are case sensitive and must be enclosed in single quotes. Special characters must
* be escaped. Boolean and string values can only be used with the comparators =
and
* <>
. For example, the following filter expression searches on gameSessionName
: "
* FilterExpression": "gameSessionName = 'Matt\\'s Awesome Game 1'"
.
*
*
*
*
* To chain multiple conditions in a single expression, use the logical keywords AND
, OR
,
* and NOT
and parentheses as needed. For example: x AND y AND NOT z
,
* NOT (x OR y)
.
*
*
* Session search evaluates conditions from left to right using the following precedence rules:
*
*
* -
*
* =
, <>
, <
, >
, <=
,
* >=
*
*
* -
*
* Parentheses
*
*
* -
*
* NOT
*
*
* -
*
* AND
*
*
* -
*
* OR
*
*
*
*
* For example, this filter expression retrieves game sessions hosting at least ten players that have an open player
* slot: "maximumSessions>=10 AND hasAvailablePlayerSessions=true"
.
*
*
* @param filterExpression
* String containing the search criteria for the session search. If no filter expression is included, the
* request returns results for all game sessions in the fleet that are in ACTIVE
status.
*
* A filter expression can contain one or multiple conditions. Each condition consists of the following:
*
*
* -
*
* Operand -- Name of a game session attribute. Valid values are gameSessionName
,
* gameSessionId
, gameSessionProperties
, maximumSessions
,
* creationTimeMillis
, playerSessionCount
, hasAvailablePlayerSessions
.
*
*
* -
*
* Comparator -- Valid comparators are: =
, <>
, <
,
* >
, <=
, >=
.
*
*
* -
*
* Value -- Value to be searched for. Values may be numbers, boolean values (true/false) or strings
* depending on the operand. String values are case sensitive and must be enclosed in single quotes. Special
* characters must be escaped. Boolean and string values can only be used with the comparators =
* and <>
. For example, the following filter expression searches on
* gameSessionName
: "
* FilterExpression": "gameSessionName = 'Matt\\'s Awesome Game 1'"
.
*
*
*
*
* To chain multiple conditions in a single expression, use the logical keywords AND
,
* OR
, and NOT
and parentheses as needed. For example:
* x AND y AND NOT z
, NOT (x OR y)
.
*
*
* Session search evaluates conditions from left to right using the following precedence rules:
*
*
* -
*
* =
, <>
, <
, >
, <=
,
* >=
*
*
* -
*
* Parentheses
*
*
* -
*
* NOT
*
*
* -
*
* AND
*
*
* -
*
* OR
*
*
*
*
* For example, this filter expression retrieves game sessions hosting at least ten players that have an open
* player slot: "maximumSessions>=10 AND hasAvailablePlayerSessions=true"
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public SearchGameSessionsRequest withFilterExpression(String filterExpression) {
setFilterExpression(filterExpression);
return this;
}
/**
*
* Instructions on how to sort the search results. If no sort expression is included, the request returns results in
* random order. A sort expression consists of the following elements:
*
*
* -
*
* Operand -- Name of a game session attribute. Valid values are gameSessionName
,
* gameSessionId
, gameSessionProperties
, maximumSessions
,
* creationTimeMillis
, playerSessionCount
, hasAvailablePlayerSessions
.
*
*
* -
*
* Order -- Valid sort orders are ASC
(ascending) and DESC
(descending).
*
*
*
*
* For example, this sort expression returns the oldest active sessions first:
* "SortExpression": "creationTimeMillis ASC"
. Results with a null value for the sort operand are
* returned at the end of the list.
*
*
* @param sortExpression
* Instructions on how to sort the search results. If no sort expression is included, the request returns
* results in random order. A sort expression consists of the following elements:
*
* -
*
* Operand -- Name of a game session attribute. Valid values are gameSessionName
,
* gameSessionId
, gameSessionProperties
, maximumSessions
,
* creationTimeMillis
, playerSessionCount
, hasAvailablePlayerSessions
.
*
*
* -
*
* Order -- Valid sort orders are ASC
(ascending) and DESC
(descending).
*
*
*
*
* For example, this sort expression returns the oldest active sessions first:
* "SortExpression": "creationTimeMillis ASC"
. Results with a null value for the sort operand
* are returned at the end of the list.
*/
public void setSortExpression(String sortExpression) {
this.sortExpression = sortExpression;
}
/**
*
* Instructions on how to sort the search results. If no sort expression is included, the request returns results in
* random order. A sort expression consists of the following elements:
*
*
* -
*
* Operand -- Name of a game session attribute. Valid values are gameSessionName
,
* gameSessionId
, gameSessionProperties
, maximumSessions
,
* creationTimeMillis
, playerSessionCount
, hasAvailablePlayerSessions
.
*
*
* -
*
* Order -- Valid sort orders are ASC
(ascending) and DESC
(descending).
*
*
*
*
* For example, this sort expression returns the oldest active sessions first:
* "SortExpression": "creationTimeMillis ASC"
. Results with a null value for the sort operand are
* returned at the end of the list.
*
*
* @return Instructions on how to sort the search results. If no sort expression is included, the request returns
* results in random order. A sort expression consists of the following elements:
*
* -
*
* Operand -- Name of a game session attribute. Valid values are gameSessionName
,
* gameSessionId
, gameSessionProperties
, maximumSessions
,
* creationTimeMillis
, playerSessionCount
, hasAvailablePlayerSessions
* .
*
*
* -
*
* Order -- Valid sort orders are ASC
(ascending) and DESC
(descending).
*
*
*
*
* For example, this sort expression returns the oldest active sessions first:
* "SortExpression": "creationTimeMillis ASC"
. Results with a null value for the sort operand
* are returned at the end of the list.
*/
public String getSortExpression() {
return this.sortExpression;
}
/**
*
* Instructions on how to sort the search results. If no sort expression is included, the request returns results in
* random order. A sort expression consists of the following elements:
*
*
* -
*
* Operand -- Name of a game session attribute. Valid values are gameSessionName
,
* gameSessionId
, gameSessionProperties
, maximumSessions
,
* creationTimeMillis
, playerSessionCount
, hasAvailablePlayerSessions
.
*
*
* -
*
* Order -- Valid sort orders are ASC
(ascending) and DESC
(descending).
*
*
*
*
* For example, this sort expression returns the oldest active sessions first:
* "SortExpression": "creationTimeMillis ASC"
. Results with a null value for the sort operand are
* returned at the end of the list.
*
*
* @param sortExpression
* Instructions on how to sort the search results. If no sort expression is included, the request returns
* results in random order. A sort expression consists of the following elements:
*
* -
*
* Operand -- Name of a game session attribute. Valid values are gameSessionName
,
* gameSessionId
, gameSessionProperties
, maximumSessions
,
* creationTimeMillis
, playerSessionCount
, hasAvailablePlayerSessions
.
*
*
* -
*
* Order -- Valid sort orders are ASC
(ascending) and DESC
(descending).
*
*
*
*
* For example, this sort expression returns the oldest active sessions first:
* "SortExpression": "creationTimeMillis ASC"
. Results with a null value for the sort operand
* are returned at the end of the list.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public SearchGameSessionsRequest withSortExpression(String sortExpression) {
setSortExpression(sortExpression);
return this;
}
/**
*
* The maximum number of results to return. Use this parameter with NextToken
to get results as a set
* of sequential pages. The maximum number of results returned is 20, even if this value is not set or is set higher
* than 20.
*
*
* @param limit
* The maximum number of results to return. Use this parameter with NextToken
to get results as
* a set of sequential pages. The maximum number of results returned is 20, even if this value is not set or
* is set higher than 20.
*/
public void setLimit(Integer limit) {
this.limit = limit;
}
/**
*
* The maximum number of results to return. Use this parameter with NextToken
to get results as a set
* of sequential pages. The maximum number of results returned is 20, even if this value is not set or is set higher
* than 20.
*
*
* @return The maximum number of results to return. Use this parameter with NextToken
to get results as
* a set of sequential pages. The maximum number of results returned is 20, even if this value is not set or
* is set higher than 20.
*/
public Integer getLimit() {
return this.limit;
}
/**
*
* The maximum number of results to return. Use this parameter with NextToken
to get results as a set
* of sequential pages. The maximum number of results returned is 20, even if this value is not set or is set higher
* than 20.
*
*
* @param limit
* The maximum number of results to return. Use this parameter with NextToken
to get results as
* a set of sequential pages. The maximum number of results returned is 20, even if this value is not set or
* is set higher than 20.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public SearchGameSessionsRequest withLimit(Integer limit) {
setLimit(limit);
return this;
}
/**
*
* A token that indicates the start of the next sequential page of results. Use the token that is returned with a
* previous call to this operation. To start at the beginning of the result set, do not specify a value.
*
*
* @param nextToken
* A token that indicates the start of the next sequential page of results. Use the token that is returned
* with a previous call to this operation. To start at the beginning of the result set, do not specify a
* value.
*/
public void setNextToken(String nextToken) {
this.nextToken = nextToken;
}
/**
*
* A token that indicates the start of the next sequential page of results. Use the token that is returned with a
* previous call to this operation. To start at the beginning of the result set, do not specify a value.
*
*
* @return A token that indicates the start of the next sequential page of results. Use the token that is returned
* with a previous call to this operation. To start at the beginning of the result set, do not specify a
* value.
*/
public String getNextToken() {
return this.nextToken;
}
/**
*
* A token that indicates the start of the next sequential page of results. Use the token that is returned with a
* previous call to this operation. To start at the beginning of the result set, do not specify a value.
*
*
* @param nextToken
* A token that indicates the start of the next sequential page of results. Use the token that is returned
* with a previous call to this operation. To start at the beginning of the result set, do not specify a
* value.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public SearchGameSessionsRequest withNextToken(String nextToken) {
setNextToken(nextToken);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getFleetId() != null)
sb.append("FleetId: ").append(getFleetId()).append(",");
if (getAliasId() != null)
sb.append("AliasId: ").append(getAliasId()).append(",");
if (getLocation() != null)
sb.append("Location: ").append(getLocation()).append(",");
if (getFilterExpression() != null)
sb.append("FilterExpression: ").append(getFilterExpression()).append(",");
if (getSortExpression() != null)
sb.append("SortExpression: ").append(getSortExpression()).append(",");
if (getLimit() != null)
sb.append("Limit: ").append(getLimit()).append(",");
if (getNextToken() != null)
sb.append("NextToken: ").append(getNextToken());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof SearchGameSessionsRequest == false)
return false;
SearchGameSessionsRequest other = (SearchGameSessionsRequest) obj;
if (other.getFleetId() == null ^ this.getFleetId() == null)
return false;
if (other.getFleetId() != null && other.getFleetId().equals(this.getFleetId()) == false)
return false;
if (other.getAliasId() == null ^ this.getAliasId() == null)
return false;
if (other.getAliasId() != null && other.getAliasId().equals(this.getAliasId()) == false)
return false;
if (other.getLocation() == null ^ this.getLocation() == null)
return false;
if (other.getLocation() != null && other.getLocation().equals(this.getLocation()) == false)
return false;
if (other.getFilterExpression() == null ^ this.getFilterExpression() == null)
return false;
if (other.getFilterExpression() != null && other.getFilterExpression().equals(this.getFilterExpression()) == false)
return false;
if (other.getSortExpression() == null ^ this.getSortExpression() == null)
return false;
if (other.getSortExpression() != null && other.getSortExpression().equals(this.getSortExpression()) == false)
return false;
if (other.getLimit() == null ^ this.getLimit() == null)
return false;
if (other.getLimit() != null && other.getLimit().equals(this.getLimit()) == false)
return false;
if (other.getNextToken() == null ^ this.getNextToken() == null)
return false;
if (other.getNextToken() != null && other.getNextToken().equals(this.getNextToken()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getFleetId() == null) ? 0 : getFleetId().hashCode());
hashCode = prime * hashCode + ((getAliasId() == null) ? 0 : getAliasId().hashCode());
hashCode = prime * hashCode + ((getLocation() == null) ? 0 : getLocation().hashCode());
hashCode = prime * hashCode + ((getFilterExpression() == null) ? 0 : getFilterExpression().hashCode());
hashCode = prime * hashCode + ((getSortExpression() == null) ? 0 : getSortExpression().hashCode());
hashCode = prime * hashCode + ((getLimit() == null) ? 0 : getLimit().hashCode());
hashCode = prime * hashCode + ((getNextToken() == null) ? 0 : getNextToken().hashCode());
return hashCode;
}
@Override
public SearchGameSessionsRequest clone() {
return (SearchGameSessionsRequest) super.clone();
}
}