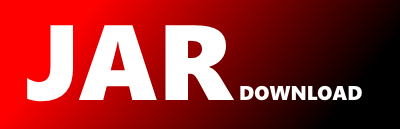
com.amazonaws.services.globalaccelerator.AWSGlobalAccelerator Maven / Gradle / Ivy
Show all versions of aws-java-sdk-globalaccelerator Show documentation
/*
* Copyright 2014-2019 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.globalaccelerator;
import javax.annotation.Generated;
import com.amazonaws.*;
import com.amazonaws.regions.*;
import com.amazonaws.services.globalaccelerator.model.*;
/**
* Interface for accessing AWS Global Accelerator.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.globalaccelerator.AbstractAWSGlobalAccelerator} instead.
*
*
* AWS Global Accelerator
*
* This is the AWS Global Accelerator API Reference. This guide is for developers who need detailed information
* about AWS Global Accelerator API actions, data types, and errors. For more information about Global Accelerator
* features, see the AWS Global
* Accelerator Developer Guide.
*
*
* AWS Global Accelerator is a network layer service in which you create accelerators to improve availability and
* performance for internet applications used by a global audience.
*
*
* Global Accelerator provides you with static IP addresses that you associate with your accelerator. These IP addresses
* are anycast from the AWS edge network and distribute incoming application traffic across multiple endpoint resources
* in multiple AWS Regions, which increases the availability of your applications. Endpoints can be Elastic IP
* addresses, Network Load Balancers, and Application Load Balancers that are located in one AWS Region or multiple
* Regions.
*
*
* Global Accelerator uses the AWS global network to route traffic to the optimal regional endpoint based on health,
* client location, and policies that you configure. The service reacts instantly to changes in health or configuration
* to ensure that internet traffic from clients is directed to only healthy endpoints.
*
*
* Global Accelerator includes components that work together to help you improve performance and availability for your
* applications:
*
*
* - Static IP address
* -
*
* AWS Global Accelerator provides you with a set of static IP addresses which are anycast from the AWS edge network and
* serve as the single fixed entry points for your clients. If you already have Elastic Load Balancing or Elastic IP
* address resources set up for your applications, you can easily add those to Global Accelerator to allow the resources
* to be accessed by a Global Accelerator static IP address.
*
*
* - Accelerator
* -
*
* An accelerator directs traffic to optimal endpoints over the AWS global network to improve availability and
* performance for your internet applications that have a global audience. Each accelerator includes one or more
* listeners.
*
*
* - Network zone
* -
*
* A network zone services the static IP addresses for your accelerator from a unique IP subnet. Similar to an AWS
* Availability Zone, a network zone is an isolated unit with its own set of physical infrastructure. When you configure
* an accelerator, Global Accelerator allocates two IPv4 addresses for it. If one IP address from a network zone becomes
* unavailable due to IP address blocking by certain client networks, or network disruptions, then client applications
* can retry on the healthy static IP address from the other isolated network zone.
*
*
* - Listener
* -
*
* A listener processes inbound connections from clients to Global Accelerator, based on the protocol and port that you
* configure. Each listener has one or more endpoint groups associated with it, and traffic is forwarded to endpoints in
* one of the groups. You associate endpoint groups with listeners by specifying the Regions that you want to distribute
* traffic to. Traffic is distributed to optimal endpoints within the endpoint groups associated with a listener.
*
*
* - Endpoint group
* -
*
* Each endpoint group is associated with a specific AWS Region. Endpoint groups include one or more endpoints in the
* Region. You can increase or reduce the percentage of traffic that would be otherwise directed to an endpoint group by
* adjusting a setting called a traffic dial. The traffic dial lets you easily do performance testing or
* blue/green deployment testing for new releases across different AWS Regions, for example.
*
*
* - Endpoint
* -
*
* An endpoint is an Elastic IP address, Network Load Balancer, or Application Load Balancer. Traffic is routed to
* endpoints based on several factors, including the geo-proximity to the user, the health of the endpoint, and the
* configuration options that you choose, such as endpoint weights. For each endpoint, you can configure weights, which
* are numbers that you can use to specify the proportion of traffic to route to each one. This can be useful, for
* example, to do performance testing within a Region.
*
*
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AWSGlobalAccelerator {
/**
* The region metadata service name for computing region endpoints. You can use this value to retrieve metadata
* (such as supported regions) of the service.
*
* @see RegionUtils#getRegionsForService(String)
*/
String ENDPOINT_PREFIX = "globalaccelerator";
/**
*
* Create an accelerator. An accelerator includes one or more listeners that process inbound connections and direct
* traffic to one or more endpoint groups, each of which includes endpoints, such as Network Load Balancers. To see
* an AWS CLI example of creating an accelerator, scroll down to Example.
*
*
* @param createAcceleratorRequest
* @return Result of the CreateAccelerator operation returned by the service.
* @throws InternalServiceErrorException
* There was an internal error for AWS Global Accelerator.
* @throws InvalidArgumentException
* An argument that you specified is invalid.
* @throws LimitExceededException
* Processing your request would cause you to exceed an AWS Global Accelerator limit.
* @sample AWSGlobalAccelerator.CreateAccelerator
* @see AWS API Documentation
*/
CreateAcceleratorResult createAccelerator(CreateAcceleratorRequest createAcceleratorRequest);
/**
*
* Create an endpoint group for the specified listener. An endpoint group is a collection of endpoints in one AWS
* Region. To see an AWS CLI example of creating an endpoint group, scroll down to Example.
*
*
* @param createEndpointGroupRequest
* @return Result of the CreateEndpointGroup operation returned by the service.
* @throws AcceleratorNotFoundException
* The accelerator that you specified doesn't exist.
* @throws EndpointGroupAlreadyExistsException
* The endpoint group that you specified already exists.
* @throws ListenerNotFoundException
* The listener that you specified doesn't exist.
* @throws InternalServiceErrorException
* There was an internal error for AWS Global Accelerator.
* @throws InvalidArgumentException
* An argument that you specified is invalid.
* @throws LimitExceededException
* Processing your request would cause you to exceed an AWS Global Accelerator limit.
* @sample AWSGlobalAccelerator.CreateEndpointGroup
* @see AWS API Documentation
*/
CreateEndpointGroupResult createEndpointGroup(CreateEndpointGroupRequest createEndpointGroupRequest);
/**
*
* Create a listener to process inbound connections from clients to an accelerator. Connections arrive to assigned
* static IP addresses on a port, port range, or list of port ranges that you specify. To see an AWS CLI example of
* creating a listener, scroll down to Example.
*
*
* @param createListenerRequest
* @return Result of the CreateListener operation returned by the service.
* @throws InvalidArgumentException
* An argument that you specified is invalid.
* @throws AcceleratorNotFoundException
* The accelerator that you specified doesn't exist.
* @throws InvalidPortRangeException
* The port numbers that you specified are not valid numbers or are not unique for this accelerator.
* @throws InternalServiceErrorException
* There was an internal error for AWS Global Accelerator.
* @throws LimitExceededException
* Processing your request would cause you to exceed an AWS Global Accelerator limit.
* @sample AWSGlobalAccelerator.CreateListener
* @see AWS API Documentation
*/
CreateListenerResult createListener(CreateListenerRequest createListenerRequest);
/**
*
* Delete an accelerator. Note: before you can delete an accelerator, you must disable it and remove all dependent
* resources (listeners and endpoint groups).
*
*
* @param deleteAcceleratorRequest
* @return Result of the DeleteAccelerator operation returned by the service.
* @throws AcceleratorNotFoundException
* The accelerator that you specified doesn't exist.
* @throws AcceleratorNotDisabledException
* The accelerator that you specified could not be disabled.
* @throws AssociatedListenerFoundException
* The accelerator that you specified has a listener associated with it. You must remove all dependent
* resources from an accelerator before you can delete it.
* @throws InternalServiceErrorException
* There was an internal error for AWS Global Accelerator.
* @throws InvalidArgumentException
* An argument that you specified is invalid.
* @sample AWSGlobalAccelerator.DeleteAccelerator
* @see AWS API Documentation
*/
DeleteAcceleratorResult deleteAccelerator(DeleteAcceleratorRequest deleteAcceleratorRequest);
/**
*
* Delete an endpoint group from a listener.
*
*
* @param deleteEndpointGroupRequest
* @return Result of the DeleteEndpointGroup operation returned by the service.
* @throws EndpointGroupNotFoundException
* The endpoint group that you specified doesn't exist.
* @throws InternalServiceErrorException
* There was an internal error for AWS Global Accelerator.
* @sample AWSGlobalAccelerator.DeleteEndpointGroup
* @see AWS API Documentation
*/
DeleteEndpointGroupResult deleteEndpointGroup(DeleteEndpointGroupRequest deleteEndpointGroupRequest);
/**
*
* Delete a listener from an accelerator.
*
*
* @param deleteListenerRequest
* @return Result of the DeleteListener operation returned by the service.
* @throws ListenerNotFoundException
* The listener that you specified doesn't exist.
* @throws AssociatedEndpointGroupFoundException
* The listener that you specified has an endpoint group associated with it. You must remove all dependent
* resources from a listener before you can delete it.
* @throws InternalServiceErrorException
* There was an internal error for AWS Global Accelerator.
* @sample AWSGlobalAccelerator.DeleteListener
* @see AWS API Documentation
*/
DeleteListenerResult deleteListener(DeleteListenerRequest deleteListenerRequest);
/**
*
* Describe an accelerator. To see an AWS CLI example of describing an accelerator, scroll down to Example.
*
*
* @param describeAcceleratorRequest
* @return Result of the DescribeAccelerator operation returned by the service.
* @throws AcceleratorNotFoundException
* The accelerator that you specified doesn't exist.
* @throws InternalServiceErrorException
* There was an internal error for AWS Global Accelerator.
* @throws InvalidArgumentException
* An argument that you specified is invalid.
* @sample AWSGlobalAccelerator.DescribeAccelerator
* @see AWS API Documentation
*/
DescribeAcceleratorResult describeAccelerator(DescribeAcceleratorRequest describeAcceleratorRequest);
/**
*
* Describe the attributes of an accelerator.
*
*
* @param describeAcceleratorAttributesRequest
* @return Result of the DescribeAcceleratorAttributes operation returned by the service.
* @throws AcceleratorNotFoundException
* The accelerator that you specified doesn't exist.
* @throws InternalServiceErrorException
* There was an internal error for AWS Global Accelerator.
* @throws InvalidArgumentException
* An argument that you specified is invalid.
* @sample AWSGlobalAccelerator.DescribeAcceleratorAttributes
* @see AWS API Documentation
*/
DescribeAcceleratorAttributesResult describeAcceleratorAttributes(DescribeAcceleratorAttributesRequest describeAcceleratorAttributesRequest);
/**
*
* Describe an endpoint group.
*
*
* @param describeEndpointGroupRequest
* @return Result of the DescribeEndpointGroup operation returned by the service.
* @throws EndpointGroupNotFoundException
* The endpoint group that you specified doesn't exist.
* @throws InternalServiceErrorException
* There was an internal error for AWS Global Accelerator.
* @sample AWSGlobalAccelerator.DescribeEndpointGroup
* @see AWS API Documentation
*/
DescribeEndpointGroupResult describeEndpointGroup(DescribeEndpointGroupRequest describeEndpointGroupRequest);
/**
*
* Describe a listener.
*
*
* @param describeListenerRequest
* @return Result of the DescribeListener operation returned by the service.
* @throws InvalidArgumentException
* An argument that you specified is invalid.
* @throws ListenerNotFoundException
* The listener that you specified doesn't exist.
* @throws InternalServiceErrorException
* There was an internal error for AWS Global Accelerator.
* @sample AWSGlobalAccelerator.DescribeListener
* @see AWS API Documentation
*/
DescribeListenerResult describeListener(DescribeListenerRequest describeListenerRequest);
/**
*
* List the accelerators for an AWS account.
*
*
* @param listAcceleratorsRequest
* @return Result of the ListAccelerators operation returned by the service.
* @throws InvalidNextTokenException
* There isn't another item to return.
* @throws InternalServiceErrorException
* There was an internal error for AWS Global Accelerator.
* @sample AWSGlobalAccelerator.ListAccelerators
* @see AWS API Documentation
*/
ListAcceleratorsResult listAccelerators(ListAcceleratorsRequest listAcceleratorsRequest);
/**
*
* List the endpoint groups that are associated with a listener.
*
*
* @param listEndpointGroupsRequest
* @return Result of the ListEndpointGroups operation returned by the service.
* @throws ListenerNotFoundException
* The listener that you specified doesn't exist.
* @throws InvalidNextTokenException
* There isn't another item to return.
* @throws InvalidArgumentException
* An argument that you specified is invalid.
* @throws InternalServiceErrorException
* There was an internal error for AWS Global Accelerator.
* @sample AWSGlobalAccelerator.ListEndpointGroups
* @see AWS API Documentation
*/
ListEndpointGroupsResult listEndpointGroups(ListEndpointGroupsRequest listEndpointGroupsRequest);
/**
*
* List the listeners for an accelerator.
*
*
* @param listListenersRequest
* @return Result of the ListListeners operation returned by the service.
* @throws AcceleratorNotFoundException
* The accelerator that you specified doesn't exist.
* @throws InvalidNextTokenException
* There isn't another item to return.
* @throws InternalServiceErrorException
* There was an internal error for AWS Global Accelerator.
* @sample AWSGlobalAccelerator.ListListeners
* @see AWS API Documentation
*/
ListListenersResult listListeners(ListListenersRequest listListenersRequest);
/**
*
* Update an accelerator.
*
*
* @param updateAcceleratorRequest
* @return Result of the UpdateAccelerator operation returned by the service.
* @throws AcceleratorNotFoundException
* The accelerator that you specified doesn't exist.
* @throws InternalServiceErrorException
* There was an internal error for AWS Global Accelerator.
* @throws InvalidArgumentException
* An argument that you specified is invalid.
* @sample AWSGlobalAccelerator.UpdateAccelerator
* @see AWS API Documentation
*/
UpdateAcceleratorResult updateAccelerator(UpdateAcceleratorRequest updateAcceleratorRequest);
/**
*
* Update the attributes for an accelerator. To see an AWS CLI example of updating an accelerator to enable flow
* logs, scroll down to Example.
*
*
* @param updateAcceleratorAttributesRequest
* @return Result of the UpdateAcceleratorAttributes operation returned by the service.
* @throws AcceleratorNotFoundException
* The accelerator that you specified doesn't exist.
* @throws InternalServiceErrorException
* There was an internal error for AWS Global Accelerator.
* @throws InvalidArgumentException
* An argument that you specified is invalid.
* @sample AWSGlobalAccelerator.UpdateAcceleratorAttributes
* @see AWS API Documentation
*/
UpdateAcceleratorAttributesResult updateAcceleratorAttributes(UpdateAcceleratorAttributesRequest updateAcceleratorAttributesRequest);
/**
*
* Update an endpoint group. To see an AWS CLI example of updating an endpoint group, scroll down to Example.
*
*
* @param updateEndpointGroupRequest
* @return Result of the UpdateEndpointGroup operation returned by the service.
* @throws InvalidArgumentException
* An argument that you specified is invalid.
* @throws EndpointGroupNotFoundException
* The endpoint group that you specified doesn't exist.
* @throws InternalServiceErrorException
* There was an internal error for AWS Global Accelerator.
* @throws LimitExceededException
* Processing your request would cause you to exceed an AWS Global Accelerator limit.
* @sample AWSGlobalAccelerator.UpdateEndpointGroup
* @see AWS API Documentation
*/
UpdateEndpointGroupResult updateEndpointGroup(UpdateEndpointGroupRequest updateEndpointGroupRequest);
/**
*
* Update a listener.
*
*
* @param updateListenerRequest
* @return Result of the UpdateListener operation returned by the service.
* @throws InvalidArgumentException
* An argument that you specified is invalid.
* @throws InvalidPortRangeException
* The port numbers that you specified are not valid numbers or are not unique for this accelerator.
* @throws ListenerNotFoundException
* The listener that you specified doesn't exist.
* @throws InternalServiceErrorException
* There was an internal error for AWS Global Accelerator.
* @throws LimitExceededException
* Processing your request would cause you to exceed an AWS Global Accelerator limit.
* @sample AWSGlobalAccelerator.UpdateListener
* @see AWS API Documentation
*/
UpdateListenerResult updateListener(UpdateListenerRequest updateListenerRequest);
/**
* Shuts down this client object, releasing any resources that might be held open. This is an optional method, and
* callers are not expected to call it, but can if they want to explicitly release any open resources. Once a client
* has been shutdown, it should not be used to make any more requests.
*/
void shutdown();
/**
* Returns additional metadata for a previously executed successful request, typically used for debugging issues
* where a service isn't acting as expected. This data isn't considered part of the result data returned by an
* operation, so it's available through this separate, diagnostic interface.
*
* Response metadata is only cached for a limited period of time, so if you need to access this extra diagnostic
* information for an executed request, you should use this method to retrieve it as soon as possible after
* executing a request.
*
* @param request
* The originally executed request.
*
* @return The response metadata for the specified request, or null if none is available.
*/
ResponseMetadata getCachedResponseMetadata(AmazonWebServiceRequest request);
}