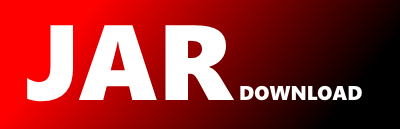
com.amazonaws.services.globalaccelerator.model.CreateEndpointGroupRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-globalaccelerator Show documentation
/*
* Copyright 2017-2022 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.globalaccelerator.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* @see AWS API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class CreateEndpointGroupRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* The Amazon Resource Name (ARN) of the listener.
*
*/
private String listenerArn;
/**
*
* The Amazon Web Services Region where the endpoint group is located. A listener can have only one endpoint group
* in a specific Region.
*
*/
private String endpointGroupRegion;
/**
*
* The list of endpoint objects.
*
*/
private java.util.List endpointConfigurations;
/**
*
* The percentage of traffic to send to an Amazon Web Services Region. Additional traffic is distributed to other
* endpoint groups for this listener.
*
*
* Use this action to increase (dial up) or decrease (dial down) traffic to a specific Region. The percentage is
* applied to the traffic that would otherwise have been routed to the Region based on optimal routing.
*
*
* The default value is 100.
*
*/
private Float trafficDialPercentage;
/**
*
* The port that Global Accelerator uses to check the health of endpoints that are part of this endpoint group. The
* default port is the listener port that this endpoint group is associated with. If listener port is a list of
* ports, Global Accelerator uses the first port in the list.
*
*/
private Integer healthCheckPort;
/**
*
* The protocol that Global Accelerator uses to check the health of endpoints that are part of this endpoint group.
* The default value is TCP.
*
*/
private String healthCheckProtocol;
/**
*
* If the protocol is HTTP/S, then this specifies the path that is the destination for health check targets. The
* default value is slash (/).
*
*/
private String healthCheckPath;
/**
*
* The time—10 seconds or 30 seconds—between each health check for an endpoint. The default value is 30.
*
*/
private Integer healthCheckIntervalSeconds;
/**
*
* The number of consecutive health checks required to set the state of a healthy endpoint to unhealthy, or to set
* an unhealthy endpoint to healthy. The default value is 3.
*
*/
private Integer thresholdCount;
/**
*
* A unique, case-sensitive identifier that you provide to ensure the idempotency—that is, the uniqueness—of the
* request.
*
*/
private String idempotencyToken;
/**
*
* Override specific listener ports used to route traffic to endpoints that are part of this endpoint group. For
* example, you can create a port override in which the listener receives user traffic on ports 80 and 443, but your
* accelerator routes that traffic to ports 1080 and 1443, respectively, on the endpoints.
*
*
* For more information, see
* Overriding listener ports in the Global Accelerator Developer Guide.
*
*/
private java.util.List portOverrides;
/**
*
* The Amazon Resource Name (ARN) of the listener.
*
*
* @param listenerArn
* The Amazon Resource Name (ARN) of the listener.
*/
public void setListenerArn(String listenerArn) {
this.listenerArn = listenerArn;
}
/**
*
* The Amazon Resource Name (ARN) of the listener.
*
*
* @return The Amazon Resource Name (ARN) of the listener.
*/
public String getListenerArn() {
return this.listenerArn;
}
/**
*
* The Amazon Resource Name (ARN) of the listener.
*
*
* @param listenerArn
* The Amazon Resource Name (ARN) of the listener.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateEndpointGroupRequest withListenerArn(String listenerArn) {
setListenerArn(listenerArn);
return this;
}
/**
*
* The Amazon Web Services Region where the endpoint group is located. A listener can have only one endpoint group
* in a specific Region.
*
*
* @param endpointGroupRegion
* The Amazon Web Services Region where the endpoint group is located. A listener can have only one endpoint
* group in a specific Region.
*/
public void setEndpointGroupRegion(String endpointGroupRegion) {
this.endpointGroupRegion = endpointGroupRegion;
}
/**
*
* The Amazon Web Services Region where the endpoint group is located. A listener can have only one endpoint group
* in a specific Region.
*
*
* @return The Amazon Web Services Region where the endpoint group is located. A listener can have only one endpoint
* group in a specific Region.
*/
public String getEndpointGroupRegion() {
return this.endpointGroupRegion;
}
/**
*
* The Amazon Web Services Region where the endpoint group is located. A listener can have only one endpoint group
* in a specific Region.
*
*
* @param endpointGroupRegion
* The Amazon Web Services Region where the endpoint group is located. A listener can have only one endpoint
* group in a specific Region.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateEndpointGroupRequest withEndpointGroupRegion(String endpointGroupRegion) {
setEndpointGroupRegion(endpointGroupRegion);
return this;
}
/**
*
* The list of endpoint objects.
*
*
* @return The list of endpoint objects.
*/
public java.util.List getEndpointConfigurations() {
return endpointConfigurations;
}
/**
*
* The list of endpoint objects.
*
*
* @param endpointConfigurations
* The list of endpoint objects.
*/
public void setEndpointConfigurations(java.util.Collection endpointConfigurations) {
if (endpointConfigurations == null) {
this.endpointConfigurations = null;
return;
}
this.endpointConfigurations = new java.util.ArrayList(endpointConfigurations);
}
/**
*
* The list of endpoint objects.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setEndpointConfigurations(java.util.Collection)} or
* {@link #withEndpointConfigurations(java.util.Collection)} if you want to override the existing values.
*
*
* @param endpointConfigurations
* The list of endpoint objects.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateEndpointGroupRequest withEndpointConfigurations(EndpointConfiguration... endpointConfigurations) {
if (this.endpointConfigurations == null) {
setEndpointConfigurations(new java.util.ArrayList(endpointConfigurations.length));
}
for (EndpointConfiguration ele : endpointConfigurations) {
this.endpointConfigurations.add(ele);
}
return this;
}
/**
*
* The list of endpoint objects.
*
*
* @param endpointConfigurations
* The list of endpoint objects.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateEndpointGroupRequest withEndpointConfigurations(java.util.Collection endpointConfigurations) {
setEndpointConfigurations(endpointConfigurations);
return this;
}
/**
*
* The percentage of traffic to send to an Amazon Web Services Region. Additional traffic is distributed to other
* endpoint groups for this listener.
*
*
* Use this action to increase (dial up) or decrease (dial down) traffic to a specific Region. The percentage is
* applied to the traffic that would otherwise have been routed to the Region based on optimal routing.
*
*
* The default value is 100.
*
*
* @param trafficDialPercentage
* The percentage of traffic to send to an Amazon Web Services Region. Additional traffic is distributed to
* other endpoint groups for this listener.
*
* Use this action to increase (dial up) or decrease (dial down) traffic to a specific Region. The percentage
* is applied to the traffic that would otherwise have been routed to the Region based on optimal routing.
*
*
* The default value is 100.
*/
public void setTrafficDialPercentage(Float trafficDialPercentage) {
this.trafficDialPercentage = trafficDialPercentage;
}
/**
*
* The percentage of traffic to send to an Amazon Web Services Region. Additional traffic is distributed to other
* endpoint groups for this listener.
*
*
* Use this action to increase (dial up) or decrease (dial down) traffic to a specific Region. The percentage is
* applied to the traffic that would otherwise have been routed to the Region based on optimal routing.
*
*
* The default value is 100.
*
*
* @return The percentage of traffic to send to an Amazon Web Services Region. Additional traffic is distributed to
* other endpoint groups for this listener.
*
* Use this action to increase (dial up) or decrease (dial down) traffic to a specific Region. The
* percentage is applied to the traffic that would otherwise have been routed to the Region based on optimal
* routing.
*
*
* The default value is 100.
*/
public Float getTrafficDialPercentage() {
return this.trafficDialPercentage;
}
/**
*
* The percentage of traffic to send to an Amazon Web Services Region. Additional traffic is distributed to other
* endpoint groups for this listener.
*
*
* Use this action to increase (dial up) or decrease (dial down) traffic to a specific Region. The percentage is
* applied to the traffic that would otherwise have been routed to the Region based on optimal routing.
*
*
* The default value is 100.
*
*
* @param trafficDialPercentage
* The percentage of traffic to send to an Amazon Web Services Region. Additional traffic is distributed to
* other endpoint groups for this listener.
*
* Use this action to increase (dial up) or decrease (dial down) traffic to a specific Region. The percentage
* is applied to the traffic that would otherwise have been routed to the Region based on optimal routing.
*
*
* The default value is 100.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateEndpointGroupRequest withTrafficDialPercentage(Float trafficDialPercentage) {
setTrafficDialPercentage(trafficDialPercentage);
return this;
}
/**
*
* The port that Global Accelerator uses to check the health of endpoints that are part of this endpoint group. The
* default port is the listener port that this endpoint group is associated with. If listener port is a list of
* ports, Global Accelerator uses the first port in the list.
*
*
* @param healthCheckPort
* The port that Global Accelerator uses to check the health of endpoints that are part of this endpoint
* group. The default port is the listener port that this endpoint group is associated with. If listener port
* is a list of ports, Global Accelerator uses the first port in the list.
*/
public void setHealthCheckPort(Integer healthCheckPort) {
this.healthCheckPort = healthCheckPort;
}
/**
*
* The port that Global Accelerator uses to check the health of endpoints that are part of this endpoint group. The
* default port is the listener port that this endpoint group is associated with. If listener port is a list of
* ports, Global Accelerator uses the first port in the list.
*
*
* @return The port that Global Accelerator uses to check the health of endpoints that are part of this endpoint
* group. The default port is the listener port that this endpoint group is associated with. If listener
* port is a list of ports, Global Accelerator uses the first port in the list.
*/
public Integer getHealthCheckPort() {
return this.healthCheckPort;
}
/**
*
* The port that Global Accelerator uses to check the health of endpoints that are part of this endpoint group. The
* default port is the listener port that this endpoint group is associated with. If listener port is a list of
* ports, Global Accelerator uses the first port in the list.
*
*
* @param healthCheckPort
* The port that Global Accelerator uses to check the health of endpoints that are part of this endpoint
* group. The default port is the listener port that this endpoint group is associated with. If listener port
* is a list of ports, Global Accelerator uses the first port in the list.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateEndpointGroupRequest withHealthCheckPort(Integer healthCheckPort) {
setHealthCheckPort(healthCheckPort);
return this;
}
/**
*
* The protocol that Global Accelerator uses to check the health of endpoints that are part of this endpoint group.
* The default value is TCP.
*
*
* @param healthCheckProtocol
* The protocol that Global Accelerator uses to check the health of endpoints that are part of this endpoint
* group. The default value is TCP.
* @see HealthCheckProtocol
*/
public void setHealthCheckProtocol(String healthCheckProtocol) {
this.healthCheckProtocol = healthCheckProtocol;
}
/**
*
* The protocol that Global Accelerator uses to check the health of endpoints that are part of this endpoint group.
* The default value is TCP.
*
*
* @return The protocol that Global Accelerator uses to check the health of endpoints that are part of this endpoint
* group. The default value is TCP.
* @see HealthCheckProtocol
*/
public String getHealthCheckProtocol() {
return this.healthCheckProtocol;
}
/**
*
* The protocol that Global Accelerator uses to check the health of endpoints that are part of this endpoint group.
* The default value is TCP.
*
*
* @param healthCheckProtocol
* The protocol that Global Accelerator uses to check the health of endpoints that are part of this endpoint
* group. The default value is TCP.
* @return Returns a reference to this object so that method calls can be chained together.
* @see HealthCheckProtocol
*/
public CreateEndpointGroupRequest withHealthCheckProtocol(String healthCheckProtocol) {
setHealthCheckProtocol(healthCheckProtocol);
return this;
}
/**
*
* The protocol that Global Accelerator uses to check the health of endpoints that are part of this endpoint group.
* The default value is TCP.
*
*
* @param healthCheckProtocol
* The protocol that Global Accelerator uses to check the health of endpoints that are part of this endpoint
* group. The default value is TCP.
* @return Returns a reference to this object so that method calls can be chained together.
* @see HealthCheckProtocol
*/
public CreateEndpointGroupRequest withHealthCheckProtocol(HealthCheckProtocol healthCheckProtocol) {
this.healthCheckProtocol = healthCheckProtocol.toString();
return this;
}
/**
*
* If the protocol is HTTP/S, then this specifies the path that is the destination for health check targets. The
* default value is slash (/).
*
*
* @param healthCheckPath
* If the protocol is HTTP/S, then this specifies the path that is the destination for health check targets.
* The default value is slash (/).
*/
public void setHealthCheckPath(String healthCheckPath) {
this.healthCheckPath = healthCheckPath;
}
/**
*
* If the protocol is HTTP/S, then this specifies the path that is the destination for health check targets. The
* default value is slash (/).
*
*
* @return If the protocol is HTTP/S, then this specifies the path that is the destination for health check targets.
* The default value is slash (/).
*/
public String getHealthCheckPath() {
return this.healthCheckPath;
}
/**
*
* If the protocol is HTTP/S, then this specifies the path that is the destination for health check targets. The
* default value is slash (/).
*
*
* @param healthCheckPath
* If the protocol is HTTP/S, then this specifies the path that is the destination for health check targets.
* The default value is slash (/).
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateEndpointGroupRequest withHealthCheckPath(String healthCheckPath) {
setHealthCheckPath(healthCheckPath);
return this;
}
/**
*
* The time—10 seconds or 30 seconds—between each health check for an endpoint. The default value is 30.
*
*
* @param healthCheckIntervalSeconds
* The time—10 seconds or 30 seconds—between each health check for an endpoint. The default value is 30.
*/
public void setHealthCheckIntervalSeconds(Integer healthCheckIntervalSeconds) {
this.healthCheckIntervalSeconds = healthCheckIntervalSeconds;
}
/**
*
* The time—10 seconds or 30 seconds—between each health check for an endpoint. The default value is 30.
*
*
* @return The time—10 seconds or 30 seconds—between each health check for an endpoint. The default value is 30.
*/
public Integer getHealthCheckIntervalSeconds() {
return this.healthCheckIntervalSeconds;
}
/**
*
* The time—10 seconds or 30 seconds—between each health check for an endpoint. The default value is 30.
*
*
* @param healthCheckIntervalSeconds
* The time—10 seconds or 30 seconds—between each health check for an endpoint. The default value is 30.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateEndpointGroupRequest withHealthCheckIntervalSeconds(Integer healthCheckIntervalSeconds) {
setHealthCheckIntervalSeconds(healthCheckIntervalSeconds);
return this;
}
/**
*
* The number of consecutive health checks required to set the state of a healthy endpoint to unhealthy, or to set
* an unhealthy endpoint to healthy. The default value is 3.
*
*
* @param thresholdCount
* The number of consecutive health checks required to set the state of a healthy endpoint to unhealthy, or
* to set an unhealthy endpoint to healthy. The default value is 3.
*/
public void setThresholdCount(Integer thresholdCount) {
this.thresholdCount = thresholdCount;
}
/**
*
* The number of consecutive health checks required to set the state of a healthy endpoint to unhealthy, or to set
* an unhealthy endpoint to healthy. The default value is 3.
*
*
* @return The number of consecutive health checks required to set the state of a healthy endpoint to unhealthy, or
* to set an unhealthy endpoint to healthy. The default value is 3.
*/
public Integer getThresholdCount() {
return this.thresholdCount;
}
/**
*
* The number of consecutive health checks required to set the state of a healthy endpoint to unhealthy, or to set
* an unhealthy endpoint to healthy. The default value is 3.
*
*
* @param thresholdCount
* The number of consecutive health checks required to set the state of a healthy endpoint to unhealthy, or
* to set an unhealthy endpoint to healthy. The default value is 3.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateEndpointGroupRequest withThresholdCount(Integer thresholdCount) {
setThresholdCount(thresholdCount);
return this;
}
/**
*
* A unique, case-sensitive identifier that you provide to ensure the idempotency—that is, the uniqueness—of the
* request.
*
*
* @param idempotencyToken
* A unique, case-sensitive identifier that you provide to ensure the idempotency—that is, the uniqueness—of
* the request.
*/
public void setIdempotencyToken(String idempotencyToken) {
this.idempotencyToken = idempotencyToken;
}
/**
*
* A unique, case-sensitive identifier that you provide to ensure the idempotency—that is, the uniqueness—of the
* request.
*
*
* @return A unique, case-sensitive identifier that you provide to ensure the idempotency—that is, the uniqueness—of
* the request.
*/
public String getIdempotencyToken() {
return this.idempotencyToken;
}
/**
*
* A unique, case-sensitive identifier that you provide to ensure the idempotency—that is, the uniqueness—of the
* request.
*
*
* @param idempotencyToken
* A unique, case-sensitive identifier that you provide to ensure the idempotency—that is, the uniqueness—of
* the request.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateEndpointGroupRequest withIdempotencyToken(String idempotencyToken) {
setIdempotencyToken(idempotencyToken);
return this;
}
/**
*
* Override specific listener ports used to route traffic to endpoints that are part of this endpoint group. For
* example, you can create a port override in which the listener receives user traffic on ports 80 and 443, but your
* accelerator routes that traffic to ports 1080 and 1443, respectively, on the endpoints.
*
*
* For more information, see
* Overriding listener ports in the Global Accelerator Developer Guide.
*
*
* @return Override specific listener ports used to route traffic to endpoints that are part of this endpoint group.
* For example, you can create a port override in which the listener receives user traffic on ports 80 and
* 443, but your accelerator routes that traffic to ports 1080 and 1443, respectively, on the endpoints.
*
* For more information, see
* Overriding listener ports in the Global Accelerator Developer Guide.
*/
public java.util.List getPortOverrides() {
return portOverrides;
}
/**
*
* Override specific listener ports used to route traffic to endpoints that are part of this endpoint group. For
* example, you can create a port override in which the listener receives user traffic on ports 80 and 443, but your
* accelerator routes that traffic to ports 1080 and 1443, respectively, on the endpoints.
*
*
* For more information, see
* Overriding listener ports in the Global Accelerator Developer Guide.
*
*
* @param portOverrides
* Override specific listener ports used to route traffic to endpoints that are part of this endpoint group.
* For example, you can create a port override in which the listener receives user traffic on ports 80 and
* 443, but your accelerator routes that traffic to ports 1080 and 1443, respectively, on the endpoints.
*
* For more information, see
* Overriding listener ports in the Global Accelerator Developer Guide.
*/
public void setPortOverrides(java.util.Collection portOverrides) {
if (portOverrides == null) {
this.portOverrides = null;
return;
}
this.portOverrides = new java.util.ArrayList(portOverrides);
}
/**
*
* Override specific listener ports used to route traffic to endpoints that are part of this endpoint group. For
* example, you can create a port override in which the listener receives user traffic on ports 80 and 443, but your
* accelerator routes that traffic to ports 1080 and 1443, respectively, on the endpoints.
*
*
* For more information, see
* Overriding listener ports in the Global Accelerator Developer Guide.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setPortOverrides(java.util.Collection)} or {@link #withPortOverrides(java.util.Collection)} if you want
* to override the existing values.
*
*
* @param portOverrides
* Override specific listener ports used to route traffic to endpoints that are part of this endpoint group.
* For example, you can create a port override in which the listener receives user traffic on ports 80 and
* 443, but your accelerator routes that traffic to ports 1080 and 1443, respectively, on the endpoints.
*
* For more information, see
* Overriding listener ports in the Global Accelerator Developer Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateEndpointGroupRequest withPortOverrides(PortOverride... portOverrides) {
if (this.portOverrides == null) {
setPortOverrides(new java.util.ArrayList(portOverrides.length));
}
for (PortOverride ele : portOverrides) {
this.portOverrides.add(ele);
}
return this;
}
/**
*
* Override specific listener ports used to route traffic to endpoints that are part of this endpoint group. For
* example, you can create a port override in which the listener receives user traffic on ports 80 and 443, but your
* accelerator routes that traffic to ports 1080 and 1443, respectively, on the endpoints.
*
*
* For more information, see
* Overriding listener ports in the Global Accelerator Developer Guide.
*
*
* @param portOverrides
* Override specific listener ports used to route traffic to endpoints that are part of this endpoint group.
* For example, you can create a port override in which the listener receives user traffic on ports 80 and
* 443, but your accelerator routes that traffic to ports 1080 and 1443, respectively, on the endpoints.
*
* For more information, see
* Overriding listener ports in the Global Accelerator Developer Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateEndpointGroupRequest withPortOverrides(java.util.Collection portOverrides) {
setPortOverrides(portOverrides);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getListenerArn() != null)
sb.append("ListenerArn: ").append(getListenerArn()).append(",");
if (getEndpointGroupRegion() != null)
sb.append("EndpointGroupRegion: ").append(getEndpointGroupRegion()).append(",");
if (getEndpointConfigurations() != null)
sb.append("EndpointConfigurations: ").append(getEndpointConfigurations()).append(",");
if (getTrafficDialPercentage() != null)
sb.append("TrafficDialPercentage: ").append(getTrafficDialPercentage()).append(",");
if (getHealthCheckPort() != null)
sb.append("HealthCheckPort: ").append(getHealthCheckPort()).append(",");
if (getHealthCheckProtocol() != null)
sb.append("HealthCheckProtocol: ").append(getHealthCheckProtocol()).append(",");
if (getHealthCheckPath() != null)
sb.append("HealthCheckPath: ").append(getHealthCheckPath()).append(",");
if (getHealthCheckIntervalSeconds() != null)
sb.append("HealthCheckIntervalSeconds: ").append(getHealthCheckIntervalSeconds()).append(",");
if (getThresholdCount() != null)
sb.append("ThresholdCount: ").append(getThresholdCount()).append(",");
if (getIdempotencyToken() != null)
sb.append("IdempotencyToken: ").append(getIdempotencyToken()).append(",");
if (getPortOverrides() != null)
sb.append("PortOverrides: ").append(getPortOverrides());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof CreateEndpointGroupRequest == false)
return false;
CreateEndpointGroupRequest other = (CreateEndpointGroupRequest) obj;
if (other.getListenerArn() == null ^ this.getListenerArn() == null)
return false;
if (other.getListenerArn() != null && other.getListenerArn().equals(this.getListenerArn()) == false)
return false;
if (other.getEndpointGroupRegion() == null ^ this.getEndpointGroupRegion() == null)
return false;
if (other.getEndpointGroupRegion() != null && other.getEndpointGroupRegion().equals(this.getEndpointGroupRegion()) == false)
return false;
if (other.getEndpointConfigurations() == null ^ this.getEndpointConfigurations() == null)
return false;
if (other.getEndpointConfigurations() != null && other.getEndpointConfigurations().equals(this.getEndpointConfigurations()) == false)
return false;
if (other.getTrafficDialPercentage() == null ^ this.getTrafficDialPercentage() == null)
return false;
if (other.getTrafficDialPercentage() != null && other.getTrafficDialPercentage().equals(this.getTrafficDialPercentage()) == false)
return false;
if (other.getHealthCheckPort() == null ^ this.getHealthCheckPort() == null)
return false;
if (other.getHealthCheckPort() != null && other.getHealthCheckPort().equals(this.getHealthCheckPort()) == false)
return false;
if (other.getHealthCheckProtocol() == null ^ this.getHealthCheckProtocol() == null)
return false;
if (other.getHealthCheckProtocol() != null && other.getHealthCheckProtocol().equals(this.getHealthCheckProtocol()) == false)
return false;
if (other.getHealthCheckPath() == null ^ this.getHealthCheckPath() == null)
return false;
if (other.getHealthCheckPath() != null && other.getHealthCheckPath().equals(this.getHealthCheckPath()) == false)
return false;
if (other.getHealthCheckIntervalSeconds() == null ^ this.getHealthCheckIntervalSeconds() == null)
return false;
if (other.getHealthCheckIntervalSeconds() != null && other.getHealthCheckIntervalSeconds().equals(this.getHealthCheckIntervalSeconds()) == false)
return false;
if (other.getThresholdCount() == null ^ this.getThresholdCount() == null)
return false;
if (other.getThresholdCount() != null && other.getThresholdCount().equals(this.getThresholdCount()) == false)
return false;
if (other.getIdempotencyToken() == null ^ this.getIdempotencyToken() == null)
return false;
if (other.getIdempotencyToken() != null && other.getIdempotencyToken().equals(this.getIdempotencyToken()) == false)
return false;
if (other.getPortOverrides() == null ^ this.getPortOverrides() == null)
return false;
if (other.getPortOverrides() != null && other.getPortOverrides().equals(this.getPortOverrides()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getListenerArn() == null) ? 0 : getListenerArn().hashCode());
hashCode = prime * hashCode + ((getEndpointGroupRegion() == null) ? 0 : getEndpointGroupRegion().hashCode());
hashCode = prime * hashCode + ((getEndpointConfigurations() == null) ? 0 : getEndpointConfigurations().hashCode());
hashCode = prime * hashCode + ((getTrafficDialPercentage() == null) ? 0 : getTrafficDialPercentage().hashCode());
hashCode = prime * hashCode + ((getHealthCheckPort() == null) ? 0 : getHealthCheckPort().hashCode());
hashCode = prime * hashCode + ((getHealthCheckProtocol() == null) ? 0 : getHealthCheckProtocol().hashCode());
hashCode = prime * hashCode + ((getHealthCheckPath() == null) ? 0 : getHealthCheckPath().hashCode());
hashCode = prime * hashCode + ((getHealthCheckIntervalSeconds() == null) ? 0 : getHealthCheckIntervalSeconds().hashCode());
hashCode = prime * hashCode + ((getThresholdCount() == null) ? 0 : getThresholdCount().hashCode());
hashCode = prime * hashCode + ((getIdempotencyToken() == null) ? 0 : getIdempotencyToken().hashCode());
hashCode = prime * hashCode + ((getPortOverrides() == null) ? 0 : getPortOverrides().hashCode());
return hashCode;
}
@Override
public CreateEndpointGroupRequest clone() {
return (CreateEndpointGroupRequest) super.clone();
}
}