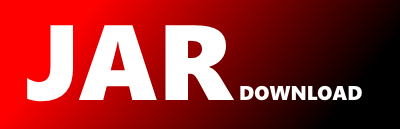
com.amazonaws.services.globalaccelerator.model.PortMapping Maven / Gradle / Ivy
Show all versions of aws-java-sdk-globalaccelerator Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.globalaccelerator.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* Returns the ports and associated IP addresses and ports of Amazon EC2 instances in your virtual private cloud (VPC)
* subnets. Custom routing is a port mapping protocol in Global Accelerator that statically associates port ranges with
* VPC subnets, which allows Global Accelerator to route to specific instances and ports within one or more subnets.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class PortMapping implements Serializable, Cloneable, StructuredPojo {
/**
*
* The accelerator port.
*
*/
private Integer acceleratorPort;
/**
*
* The Amazon Resource Name (ARN) of the endpoint group.
*
*/
private String endpointGroupArn;
/**
*
* The IP address of the VPC subnet (the subnet ID).
*
*/
private String endpointId;
/**
*
* The EC2 instance IP address and port number in the virtual private cloud (VPC) subnet.
*
*/
private SocketAddress destinationSocketAddress;
/**
*
* The protocols supported by the endpoint group.
*
*/
private java.util.List protocols;
/**
*
* Indicates whether or not a port mapping destination can receive traffic. The value is either ALLOW, if traffic is
* allowed to the destination, or DENY, if traffic is not allowed to the destination.
*
*/
private String destinationTrafficState;
/**
*
* The accelerator port.
*
*
* @param acceleratorPort
* The accelerator port.
*/
public void setAcceleratorPort(Integer acceleratorPort) {
this.acceleratorPort = acceleratorPort;
}
/**
*
* The accelerator port.
*
*
* @return The accelerator port.
*/
public Integer getAcceleratorPort() {
return this.acceleratorPort;
}
/**
*
* The accelerator port.
*
*
* @param acceleratorPort
* The accelerator port.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PortMapping withAcceleratorPort(Integer acceleratorPort) {
setAcceleratorPort(acceleratorPort);
return this;
}
/**
*
* The Amazon Resource Name (ARN) of the endpoint group.
*
*
* @param endpointGroupArn
* The Amazon Resource Name (ARN) of the endpoint group.
*/
public void setEndpointGroupArn(String endpointGroupArn) {
this.endpointGroupArn = endpointGroupArn;
}
/**
*
* The Amazon Resource Name (ARN) of the endpoint group.
*
*
* @return The Amazon Resource Name (ARN) of the endpoint group.
*/
public String getEndpointGroupArn() {
return this.endpointGroupArn;
}
/**
*
* The Amazon Resource Name (ARN) of the endpoint group.
*
*
* @param endpointGroupArn
* The Amazon Resource Name (ARN) of the endpoint group.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PortMapping withEndpointGroupArn(String endpointGroupArn) {
setEndpointGroupArn(endpointGroupArn);
return this;
}
/**
*
* The IP address of the VPC subnet (the subnet ID).
*
*
* @param endpointId
* The IP address of the VPC subnet (the subnet ID).
*/
public void setEndpointId(String endpointId) {
this.endpointId = endpointId;
}
/**
*
* The IP address of the VPC subnet (the subnet ID).
*
*
* @return The IP address of the VPC subnet (the subnet ID).
*/
public String getEndpointId() {
return this.endpointId;
}
/**
*
* The IP address of the VPC subnet (the subnet ID).
*
*
* @param endpointId
* The IP address of the VPC subnet (the subnet ID).
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PortMapping withEndpointId(String endpointId) {
setEndpointId(endpointId);
return this;
}
/**
*
* The EC2 instance IP address and port number in the virtual private cloud (VPC) subnet.
*
*
* @param destinationSocketAddress
* The EC2 instance IP address and port number in the virtual private cloud (VPC) subnet.
*/
public void setDestinationSocketAddress(SocketAddress destinationSocketAddress) {
this.destinationSocketAddress = destinationSocketAddress;
}
/**
*
* The EC2 instance IP address and port number in the virtual private cloud (VPC) subnet.
*
*
* @return The EC2 instance IP address and port number in the virtual private cloud (VPC) subnet.
*/
public SocketAddress getDestinationSocketAddress() {
return this.destinationSocketAddress;
}
/**
*
* The EC2 instance IP address and port number in the virtual private cloud (VPC) subnet.
*
*
* @param destinationSocketAddress
* The EC2 instance IP address and port number in the virtual private cloud (VPC) subnet.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PortMapping withDestinationSocketAddress(SocketAddress destinationSocketAddress) {
setDestinationSocketAddress(destinationSocketAddress);
return this;
}
/**
*
* The protocols supported by the endpoint group.
*
*
* @return The protocols supported by the endpoint group.
* @see CustomRoutingProtocol
*/
public java.util.List getProtocols() {
return protocols;
}
/**
*
* The protocols supported by the endpoint group.
*
*
* @param protocols
* The protocols supported by the endpoint group.
* @see CustomRoutingProtocol
*/
public void setProtocols(java.util.Collection protocols) {
if (protocols == null) {
this.protocols = null;
return;
}
this.protocols = new java.util.ArrayList(protocols);
}
/**
*
* The protocols supported by the endpoint group.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setProtocols(java.util.Collection)} or {@link #withProtocols(java.util.Collection)} if you want to
* override the existing values.
*
*
* @param protocols
* The protocols supported by the endpoint group.
* @return Returns a reference to this object so that method calls can be chained together.
* @see CustomRoutingProtocol
*/
public PortMapping withProtocols(String... protocols) {
if (this.protocols == null) {
setProtocols(new java.util.ArrayList(protocols.length));
}
for (String ele : protocols) {
this.protocols.add(ele);
}
return this;
}
/**
*
* The protocols supported by the endpoint group.
*
*
* @param protocols
* The protocols supported by the endpoint group.
* @return Returns a reference to this object so that method calls can be chained together.
* @see CustomRoutingProtocol
*/
public PortMapping withProtocols(java.util.Collection protocols) {
setProtocols(protocols);
return this;
}
/**
*
* The protocols supported by the endpoint group.
*
*
* @param protocols
* The protocols supported by the endpoint group.
* @return Returns a reference to this object so that method calls can be chained together.
* @see CustomRoutingProtocol
*/
public PortMapping withProtocols(CustomRoutingProtocol... protocols) {
java.util.ArrayList protocolsCopy = new java.util.ArrayList(protocols.length);
for (CustomRoutingProtocol value : protocols) {
protocolsCopy.add(value.toString());
}
if (getProtocols() == null) {
setProtocols(protocolsCopy);
} else {
getProtocols().addAll(protocolsCopy);
}
return this;
}
/**
*
* Indicates whether or not a port mapping destination can receive traffic. The value is either ALLOW, if traffic is
* allowed to the destination, or DENY, if traffic is not allowed to the destination.
*
*
* @param destinationTrafficState
* Indicates whether or not a port mapping destination can receive traffic. The value is either ALLOW, if
* traffic is allowed to the destination, or DENY, if traffic is not allowed to the destination.
* @see CustomRoutingDestinationTrafficState
*/
public void setDestinationTrafficState(String destinationTrafficState) {
this.destinationTrafficState = destinationTrafficState;
}
/**
*
* Indicates whether or not a port mapping destination can receive traffic. The value is either ALLOW, if traffic is
* allowed to the destination, or DENY, if traffic is not allowed to the destination.
*
*
* @return Indicates whether or not a port mapping destination can receive traffic. The value is either ALLOW, if
* traffic is allowed to the destination, or DENY, if traffic is not allowed to the destination.
* @see CustomRoutingDestinationTrafficState
*/
public String getDestinationTrafficState() {
return this.destinationTrafficState;
}
/**
*
* Indicates whether or not a port mapping destination can receive traffic. The value is either ALLOW, if traffic is
* allowed to the destination, or DENY, if traffic is not allowed to the destination.
*
*
* @param destinationTrafficState
* Indicates whether or not a port mapping destination can receive traffic. The value is either ALLOW, if
* traffic is allowed to the destination, or DENY, if traffic is not allowed to the destination.
* @return Returns a reference to this object so that method calls can be chained together.
* @see CustomRoutingDestinationTrafficState
*/
public PortMapping withDestinationTrafficState(String destinationTrafficState) {
setDestinationTrafficState(destinationTrafficState);
return this;
}
/**
*
* Indicates whether or not a port mapping destination can receive traffic. The value is either ALLOW, if traffic is
* allowed to the destination, or DENY, if traffic is not allowed to the destination.
*
*
* @param destinationTrafficState
* Indicates whether or not a port mapping destination can receive traffic. The value is either ALLOW, if
* traffic is allowed to the destination, or DENY, if traffic is not allowed to the destination.
* @return Returns a reference to this object so that method calls can be chained together.
* @see CustomRoutingDestinationTrafficState
*/
public PortMapping withDestinationTrafficState(CustomRoutingDestinationTrafficState destinationTrafficState) {
this.destinationTrafficState = destinationTrafficState.toString();
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getAcceleratorPort() != null)
sb.append("AcceleratorPort: ").append(getAcceleratorPort()).append(",");
if (getEndpointGroupArn() != null)
sb.append("EndpointGroupArn: ").append(getEndpointGroupArn()).append(",");
if (getEndpointId() != null)
sb.append("EndpointId: ").append(getEndpointId()).append(",");
if (getDestinationSocketAddress() != null)
sb.append("DestinationSocketAddress: ").append(getDestinationSocketAddress()).append(",");
if (getProtocols() != null)
sb.append("Protocols: ").append(getProtocols()).append(",");
if (getDestinationTrafficState() != null)
sb.append("DestinationTrafficState: ").append(getDestinationTrafficState());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof PortMapping == false)
return false;
PortMapping other = (PortMapping) obj;
if (other.getAcceleratorPort() == null ^ this.getAcceleratorPort() == null)
return false;
if (other.getAcceleratorPort() != null && other.getAcceleratorPort().equals(this.getAcceleratorPort()) == false)
return false;
if (other.getEndpointGroupArn() == null ^ this.getEndpointGroupArn() == null)
return false;
if (other.getEndpointGroupArn() != null && other.getEndpointGroupArn().equals(this.getEndpointGroupArn()) == false)
return false;
if (other.getEndpointId() == null ^ this.getEndpointId() == null)
return false;
if (other.getEndpointId() != null && other.getEndpointId().equals(this.getEndpointId()) == false)
return false;
if (other.getDestinationSocketAddress() == null ^ this.getDestinationSocketAddress() == null)
return false;
if (other.getDestinationSocketAddress() != null && other.getDestinationSocketAddress().equals(this.getDestinationSocketAddress()) == false)
return false;
if (other.getProtocols() == null ^ this.getProtocols() == null)
return false;
if (other.getProtocols() != null && other.getProtocols().equals(this.getProtocols()) == false)
return false;
if (other.getDestinationTrafficState() == null ^ this.getDestinationTrafficState() == null)
return false;
if (other.getDestinationTrafficState() != null && other.getDestinationTrafficState().equals(this.getDestinationTrafficState()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getAcceleratorPort() == null) ? 0 : getAcceleratorPort().hashCode());
hashCode = prime * hashCode + ((getEndpointGroupArn() == null) ? 0 : getEndpointGroupArn().hashCode());
hashCode = prime * hashCode + ((getEndpointId() == null) ? 0 : getEndpointId().hashCode());
hashCode = prime * hashCode + ((getDestinationSocketAddress() == null) ? 0 : getDestinationSocketAddress().hashCode());
hashCode = prime * hashCode + ((getProtocols() == null) ? 0 : getProtocols().hashCode());
hashCode = prime * hashCode + ((getDestinationTrafficState() == null) ? 0 : getDestinationTrafficState().hashCode());
return hashCode;
}
@Override
public PortMapping clone() {
try {
return (PortMapping) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.globalaccelerator.model.transform.PortMappingMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}