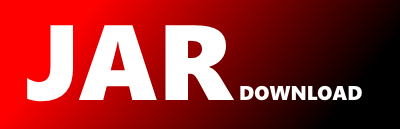
com.amazonaws.services.groundstation.model.GetSatelliteResult Maven / Gradle / Ivy
Show all versions of aws-java-sdk-groundstation Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.groundstation.model;
import java.io.Serializable;
import javax.annotation.Generated;
/**
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class GetSatelliteResult extends com.amazonaws.AmazonWebServiceResult implements Serializable, Cloneable {
/**
*
* The current ephemeris being used to compute the trajectory of the satellite.
*
*/
private EphemerisMetaData currentEphemeris;
/**
*
* A list of ground stations to which the satellite is on-boarded.
*
*/
private java.util.List groundStations;
/**
*
* NORAD satellite ID number.
*
*/
private Integer noradSatelliteID;
/**
*
* ARN of a satellite.
*
*/
private String satelliteArn;
/**
*
* UUID of a satellite.
*
*/
private String satelliteId;
/**
*
* The current ephemeris being used to compute the trajectory of the satellite.
*
*
* @param currentEphemeris
* The current ephemeris being used to compute the trajectory of the satellite.
*/
public void setCurrentEphemeris(EphemerisMetaData currentEphemeris) {
this.currentEphemeris = currentEphemeris;
}
/**
*
* The current ephemeris being used to compute the trajectory of the satellite.
*
*
* @return The current ephemeris being used to compute the trajectory of the satellite.
*/
public EphemerisMetaData getCurrentEphemeris() {
return this.currentEphemeris;
}
/**
*
* The current ephemeris being used to compute the trajectory of the satellite.
*
*
* @param currentEphemeris
* The current ephemeris being used to compute the trajectory of the satellite.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetSatelliteResult withCurrentEphemeris(EphemerisMetaData currentEphemeris) {
setCurrentEphemeris(currentEphemeris);
return this;
}
/**
*
* A list of ground stations to which the satellite is on-boarded.
*
*
* @return A list of ground stations to which the satellite is on-boarded.
*/
public java.util.List getGroundStations() {
return groundStations;
}
/**
*
* A list of ground stations to which the satellite is on-boarded.
*
*
* @param groundStations
* A list of ground stations to which the satellite is on-boarded.
*/
public void setGroundStations(java.util.Collection groundStations) {
if (groundStations == null) {
this.groundStations = null;
return;
}
this.groundStations = new java.util.ArrayList(groundStations);
}
/**
*
* A list of ground stations to which the satellite is on-boarded.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setGroundStations(java.util.Collection)} or {@link #withGroundStations(java.util.Collection)} if you want
* to override the existing values.
*
*
* @param groundStations
* A list of ground stations to which the satellite is on-boarded.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetSatelliteResult withGroundStations(String... groundStations) {
if (this.groundStations == null) {
setGroundStations(new java.util.ArrayList(groundStations.length));
}
for (String ele : groundStations) {
this.groundStations.add(ele);
}
return this;
}
/**
*
* A list of ground stations to which the satellite is on-boarded.
*
*
* @param groundStations
* A list of ground stations to which the satellite is on-boarded.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetSatelliteResult withGroundStations(java.util.Collection groundStations) {
setGroundStations(groundStations);
return this;
}
/**
*
* NORAD satellite ID number.
*
*
* @param noradSatelliteID
* NORAD satellite ID number.
*/
public void setNoradSatelliteID(Integer noradSatelliteID) {
this.noradSatelliteID = noradSatelliteID;
}
/**
*
* NORAD satellite ID number.
*
*
* @return NORAD satellite ID number.
*/
public Integer getNoradSatelliteID() {
return this.noradSatelliteID;
}
/**
*
* NORAD satellite ID number.
*
*
* @param noradSatelliteID
* NORAD satellite ID number.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetSatelliteResult withNoradSatelliteID(Integer noradSatelliteID) {
setNoradSatelliteID(noradSatelliteID);
return this;
}
/**
*
* ARN of a satellite.
*
*
* @param satelliteArn
* ARN of a satellite.
*/
public void setSatelliteArn(String satelliteArn) {
this.satelliteArn = satelliteArn;
}
/**
*
* ARN of a satellite.
*
*
* @return ARN of a satellite.
*/
public String getSatelliteArn() {
return this.satelliteArn;
}
/**
*
* ARN of a satellite.
*
*
* @param satelliteArn
* ARN of a satellite.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetSatelliteResult withSatelliteArn(String satelliteArn) {
setSatelliteArn(satelliteArn);
return this;
}
/**
*
* UUID of a satellite.
*
*
* @param satelliteId
* UUID of a satellite.
*/
public void setSatelliteId(String satelliteId) {
this.satelliteId = satelliteId;
}
/**
*
* UUID of a satellite.
*
*
* @return UUID of a satellite.
*/
public String getSatelliteId() {
return this.satelliteId;
}
/**
*
* UUID of a satellite.
*
*
* @param satelliteId
* UUID of a satellite.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetSatelliteResult withSatelliteId(String satelliteId) {
setSatelliteId(satelliteId);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getCurrentEphemeris() != null)
sb.append("CurrentEphemeris: ").append(getCurrentEphemeris()).append(",");
if (getGroundStations() != null)
sb.append("GroundStations: ").append(getGroundStations()).append(",");
if (getNoradSatelliteID() != null)
sb.append("NoradSatelliteID: ").append(getNoradSatelliteID()).append(",");
if (getSatelliteArn() != null)
sb.append("SatelliteArn: ").append(getSatelliteArn()).append(",");
if (getSatelliteId() != null)
sb.append("SatelliteId: ").append(getSatelliteId());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof GetSatelliteResult == false)
return false;
GetSatelliteResult other = (GetSatelliteResult) obj;
if (other.getCurrentEphemeris() == null ^ this.getCurrentEphemeris() == null)
return false;
if (other.getCurrentEphemeris() != null && other.getCurrentEphemeris().equals(this.getCurrentEphemeris()) == false)
return false;
if (other.getGroundStations() == null ^ this.getGroundStations() == null)
return false;
if (other.getGroundStations() != null && other.getGroundStations().equals(this.getGroundStations()) == false)
return false;
if (other.getNoradSatelliteID() == null ^ this.getNoradSatelliteID() == null)
return false;
if (other.getNoradSatelliteID() != null && other.getNoradSatelliteID().equals(this.getNoradSatelliteID()) == false)
return false;
if (other.getSatelliteArn() == null ^ this.getSatelliteArn() == null)
return false;
if (other.getSatelliteArn() != null && other.getSatelliteArn().equals(this.getSatelliteArn()) == false)
return false;
if (other.getSatelliteId() == null ^ this.getSatelliteId() == null)
return false;
if (other.getSatelliteId() != null && other.getSatelliteId().equals(this.getSatelliteId()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getCurrentEphemeris() == null) ? 0 : getCurrentEphemeris().hashCode());
hashCode = prime * hashCode + ((getGroundStations() == null) ? 0 : getGroundStations().hashCode());
hashCode = prime * hashCode + ((getNoradSatelliteID() == null) ? 0 : getNoradSatelliteID().hashCode());
hashCode = prime * hashCode + ((getSatelliteArn() == null) ? 0 : getSatelliteArn().hashCode());
hashCode = prime * hashCode + ((getSatelliteId() == null) ? 0 : getSatelliteId().hashCode());
return hashCode;
}
@Override
public GetSatelliteResult clone() {
try {
return (GetSatelliteResult) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
}