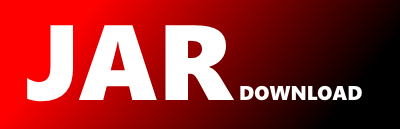
com.amazonaws.services.groundstation.AWSGroundStationAsync Maven / Gradle / Ivy
Show all versions of aws-java-sdk-groundstation Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.groundstation;
import javax.annotation.Generated;
import com.amazonaws.services.groundstation.model.*;
/**
* Interface for accessing AWS Ground Station asynchronously. Each asynchronous method will return a Java Future object
* representing the asynchronous operation; overloads which accept an {@code AsyncHandler} can be used to receive
* notification when an asynchronous operation completes.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.groundstation.AbstractAWSGroundStationAsync} instead.
*
*
*
* Welcome to the AWS Ground Station API Reference. AWS Ground Station is a fully managed service that enables you to
* control satellite communications, downlink and process satellite data, and scale your satellite operations
* efficiently and cost-effectively without having to build or manage your own ground station infrastructure.
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AWSGroundStationAsync extends AWSGroundStation {
/**
*
* Cancels a contact with a specified contact ID.
*
*
* @param cancelContactRequest
* @return A Java Future containing the result of the CancelContact operation returned by the service.
* @sample AWSGroundStationAsync.CancelContact
* @see AWS
* API Documentation
*/
java.util.concurrent.Future cancelContactAsync(CancelContactRequest cancelContactRequest);
/**
*
* Cancels a contact with a specified contact ID.
*
*
* @param cancelContactRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CancelContact operation returned by the service.
* @sample AWSGroundStationAsyncHandler.CancelContact
* @see AWS
* API Documentation
*/
java.util.concurrent.Future cancelContactAsync(CancelContactRequest cancelContactRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a Config
with the specified configData
parameters.
*
*
* Only one type of configData
can be specified.
*
*
* @param createConfigRequest
* @return A Java Future containing the result of the CreateConfig operation returned by the service.
* @sample AWSGroundStationAsync.CreateConfig
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createConfigAsync(CreateConfigRequest createConfigRequest);
/**
*
* Creates a Config
with the specified configData
parameters.
*
*
* Only one type of configData
can be specified.
*
*
* @param createConfigRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateConfig operation returned by the service.
* @sample AWSGroundStationAsyncHandler.CreateConfig
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createConfigAsync(CreateConfigRequest createConfigRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a DataflowEndpoint
group containing the specified list of DataflowEndpoint
* objects.
*
*
* The name
field in each endpoint is used in your mission profile DataflowEndpointConfig
* to specify which endpoints to use during a contact.
*
*
* When a contact uses multiple DataflowEndpointConfig
objects, each Config
must match a
* DataflowEndpoint
in the same group.
*
*
* @param createDataflowEndpointGroupRequest
* @return A Java Future containing the result of the CreateDataflowEndpointGroup operation returned by the service.
* @sample AWSGroundStationAsync.CreateDataflowEndpointGroup
* @see AWS API Documentation
*/
java.util.concurrent.Future createDataflowEndpointGroupAsync(
CreateDataflowEndpointGroupRequest createDataflowEndpointGroupRequest);
/**
*
* Creates a DataflowEndpoint
group containing the specified list of DataflowEndpoint
* objects.
*
*
* The name
field in each endpoint is used in your mission profile DataflowEndpointConfig
* to specify which endpoints to use during a contact.
*
*
* When a contact uses multiple DataflowEndpointConfig
objects, each Config
must match a
* DataflowEndpoint
in the same group.
*
*
* @param createDataflowEndpointGroupRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateDataflowEndpointGroup operation returned by the service.
* @sample AWSGroundStationAsyncHandler.CreateDataflowEndpointGroup
* @see AWS API Documentation
*/
java.util.concurrent.Future createDataflowEndpointGroupAsync(
CreateDataflowEndpointGroupRequest createDataflowEndpointGroupRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates an Ephemeris with the specified EphemerisData
.
*
*
* @param createEphemerisRequest
* @return A Java Future containing the result of the CreateEphemeris operation returned by the service.
* @sample AWSGroundStationAsync.CreateEphemeris
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createEphemerisAsync(CreateEphemerisRequest createEphemerisRequest);
/**
*
* Creates an Ephemeris with the specified EphemerisData
.
*
*
* @param createEphemerisRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateEphemeris operation returned by the service.
* @sample AWSGroundStationAsyncHandler.CreateEphemeris
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createEphemerisAsync(CreateEphemerisRequest createEphemerisRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a mission profile.
*
*
* dataflowEdges
is a list of lists of strings. Each lower level list of strings has two elements: a
* from ARN and a to ARN.
*
*
* @param createMissionProfileRequest
* @return A Java Future containing the result of the CreateMissionProfile operation returned by the service.
* @sample AWSGroundStationAsync.CreateMissionProfile
* @see AWS API Documentation
*/
java.util.concurrent.Future createMissionProfileAsync(CreateMissionProfileRequest createMissionProfileRequest);
/**
*
* Creates a mission profile.
*
*
* dataflowEdges
is a list of lists of strings. Each lower level list of strings has two elements: a
* from ARN and a to ARN.
*
*
* @param createMissionProfileRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateMissionProfile operation returned by the service.
* @sample AWSGroundStationAsyncHandler.CreateMissionProfile
* @see AWS API Documentation
*/
java.util.concurrent.Future createMissionProfileAsync(CreateMissionProfileRequest createMissionProfileRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a Config
.
*
*
* @param deleteConfigRequest
* @return A Java Future containing the result of the DeleteConfig operation returned by the service.
* @sample AWSGroundStationAsync.DeleteConfig
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteConfigAsync(DeleteConfigRequest deleteConfigRequest);
/**
*
* Deletes a Config
.
*
*
* @param deleteConfigRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteConfig operation returned by the service.
* @sample AWSGroundStationAsyncHandler.DeleteConfig
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteConfigAsync(DeleteConfigRequest deleteConfigRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a dataflow endpoint group.
*
*
* @param deleteDataflowEndpointGroupRequest
* @return A Java Future containing the result of the DeleteDataflowEndpointGroup operation returned by the service.
* @sample AWSGroundStationAsync.DeleteDataflowEndpointGroup
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteDataflowEndpointGroupAsync(
DeleteDataflowEndpointGroupRequest deleteDataflowEndpointGroupRequest);
/**
*
* Deletes a dataflow endpoint group.
*
*
* @param deleteDataflowEndpointGroupRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteDataflowEndpointGroup operation returned by the service.
* @sample AWSGroundStationAsyncHandler.DeleteDataflowEndpointGroup
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteDataflowEndpointGroupAsync(
DeleteDataflowEndpointGroupRequest deleteDataflowEndpointGroupRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes an ephemeris
*
*
* @param deleteEphemerisRequest
* @return A Java Future containing the result of the DeleteEphemeris operation returned by the service.
* @sample AWSGroundStationAsync.DeleteEphemeris
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteEphemerisAsync(DeleteEphemerisRequest deleteEphemerisRequest);
/**
*
* Deletes an ephemeris
*
*
* @param deleteEphemerisRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteEphemeris operation returned by the service.
* @sample AWSGroundStationAsyncHandler.DeleteEphemeris
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteEphemerisAsync(DeleteEphemerisRequest deleteEphemerisRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a mission profile.
*
*
* @param deleteMissionProfileRequest
* @return A Java Future containing the result of the DeleteMissionProfile operation returned by the service.
* @sample AWSGroundStationAsync.DeleteMissionProfile
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteMissionProfileAsync(DeleteMissionProfileRequest deleteMissionProfileRequest);
/**
*
* Deletes a mission profile.
*
*
* @param deleteMissionProfileRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteMissionProfile operation returned by the service.
* @sample AWSGroundStationAsyncHandler.DeleteMissionProfile
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteMissionProfileAsync(DeleteMissionProfileRequest deleteMissionProfileRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Describes an existing contact.
*
*
* @param describeContactRequest
* @return A Java Future containing the result of the DescribeContact operation returned by the service.
* @sample AWSGroundStationAsync.DescribeContact
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeContactAsync(DescribeContactRequest describeContactRequest);
/**
*
* Describes an existing contact.
*
*
* @param describeContactRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeContact operation returned by the service.
* @sample AWSGroundStationAsyncHandler.DescribeContact
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeContactAsync(DescribeContactRequest describeContactRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Describes an existing ephemeris.
*
*
* @param describeEphemerisRequest
* @return A Java Future containing the result of the DescribeEphemeris operation returned by the service.
* @sample AWSGroundStationAsync.DescribeEphemeris
* @see AWS API Documentation
*/
java.util.concurrent.Future describeEphemerisAsync(DescribeEphemerisRequest describeEphemerisRequest);
/**
*
* Describes an existing ephemeris.
*
*
* @param describeEphemerisRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeEphemeris operation returned by the service.
* @sample AWSGroundStationAsyncHandler.DescribeEphemeris
* @see AWS API Documentation
*/
java.util.concurrent.Future describeEphemerisAsync(DescribeEphemerisRequest describeEphemerisRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
*
* For use by AWS Ground Station Agent and shouldn't be called directly.
*
*
*
* Gets the latest configuration information for a registered agent.
*
*
* @param getAgentConfigurationRequest
* @return A Java Future containing the result of the GetAgentConfiguration operation returned by the service.
* @sample AWSGroundStationAsync.GetAgentConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future getAgentConfigurationAsync(GetAgentConfigurationRequest getAgentConfigurationRequest);
/**
*
*
* For use by AWS Ground Station Agent and shouldn't be called directly.
*
*
*
* Gets the latest configuration information for a registered agent.
*
*
* @param getAgentConfigurationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetAgentConfiguration operation returned by the service.
* @sample AWSGroundStationAsyncHandler.GetAgentConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future getAgentConfigurationAsync(GetAgentConfigurationRequest getAgentConfigurationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns Config
information.
*
*
* Only one Config
response can be returned.
*
*
* @param getConfigRequest
* @return A Java Future containing the result of the GetConfig operation returned by the service.
* @sample AWSGroundStationAsync.GetConfig
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getConfigAsync(GetConfigRequest getConfigRequest);
/**
*
* Returns Config
information.
*
*
* Only one Config
response can be returned.
*
*
* @param getConfigRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetConfig operation returned by the service.
* @sample AWSGroundStationAsyncHandler.GetConfig
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getConfigAsync(GetConfigRequest getConfigRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns the dataflow endpoint group.
*
*
* @param getDataflowEndpointGroupRequest
* @return A Java Future containing the result of the GetDataflowEndpointGroup operation returned by the service.
* @sample AWSGroundStationAsync.GetDataflowEndpointGroup
* @see AWS API Documentation
*/
java.util.concurrent.Future getDataflowEndpointGroupAsync(GetDataflowEndpointGroupRequest getDataflowEndpointGroupRequest);
/**
*
* Returns the dataflow endpoint group.
*
*
* @param getDataflowEndpointGroupRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetDataflowEndpointGroup operation returned by the service.
* @sample AWSGroundStationAsyncHandler.GetDataflowEndpointGroup
* @see AWS API Documentation
*/
java.util.concurrent.Future getDataflowEndpointGroupAsync(GetDataflowEndpointGroupRequest getDataflowEndpointGroupRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns the number of reserved minutes used by account.
*
*
* @param getMinuteUsageRequest
* @return A Java Future containing the result of the GetMinuteUsage operation returned by the service.
* @sample AWSGroundStationAsync.GetMinuteUsage
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getMinuteUsageAsync(GetMinuteUsageRequest getMinuteUsageRequest);
/**
*
* Returns the number of reserved minutes used by account.
*
*
* @param getMinuteUsageRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetMinuteUsage operation returned by the service.
* @sample AWSGroundStationAsyncHandler.GetMinuteUsage
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getMinuteUsageAsync(GetMinuteUsageRequest getMinuteUsageRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a mission profile.
*
*
* @param getMissionProfileRequest
* @return A Java Future containing the result of the GetMissionProfile operation returned by the service.
* @sample AWSGroundStationAsync.GetMissionProfile
* @see AWS API Documentation
*/
java.util.concurrent.Future getMissionProfileAsync(GetMissionProfileRequest getMissionProfileRequest);
/**
*
* Returns a mission profile.
*
*
* @param getMissionProfileRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetMissionProfile operation returned by the service.
* @sample AWSGroundStationAsyncHandler.GetMissionProfile
* @see AWS API Documentation
*/
java.util.concurrent.Future getMissionProfileAsync(GetMissionProfileRequest getMissionProfileRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a satellite.
*
*
* @param getSatelliteRequest
* @return A Java Future containing the result of the GetSatellite operation returned by the service.
* @sample AWSGroundStationAsync.GetSatellite
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getSatelliteAsync(GetSatelliteRequest getSatelliteRequest);
/**
*
* Returns a satellite.
*
*
* @param getSatelliteRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetSatellite operation returned by the service.
* @sample AWSGroundStationAsyncHandler.GetSatellite
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getSatelliteAsync(GetSatelliteRequest getSatelliteRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of Config
objects.
*
*
* @param listConfigsRequest
* @return A Java Future containing the result of the ListConfigs operation returned by the service.
* @sample AWSGroundStationAsync.ListConfigs
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listConfigsAsync(ListConfigsRequest listConfigsRequest);
/**
*
* Returns a list of Config
objects.
*
*
* @param listConfigsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListConfigs operation returned by the service.
* @sample AWSGroundStationAsyncHandler.ListConfigs
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listConfigsAsync(ListConfigsRequest listConfigsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of contacts.
*
*
* If statusList
contains AVAILABLE, the request must include groundStation
,
* missionprofileArn
, and satelliteArn
.
*
*
* @param listContactsRequest
* @return A Java Future containing the result of the ListContacts operation returned by the service.
* @sample AWSGroundStationAsync.ListContacts
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listContactsAsync(ListContactsRequest listContactsRequest);
/**
*
* Returns a list of contacts.
*
*
* If statusList
contains AVAILABLE, the request must include groundStation
,
* missionprofileArn
, and satelliteArn
.
*
*
* @param listContactsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListContacts operation returned by the service.
* @sample AWSGroundStationAsyncHandler.ListContacts
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listContactsAsync(ListContactsRequest listContactsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of DataflowEndpoint
groups.
*
*
* @param listDataflowEndpointGroupsRequest
* @return A Java Future containing the result of the ListDataflowEndpointGroups operation returned by the service.
* @sample AWSGroundStationAsync.ListDataflowEndpointGroups
* @see AWS API Documentation
*/
java.util.concurrent.Future listDataflowEndpointGroupsAsync(
ListDataflowEndpointGroupsRequest listDataflowEndpointGroupsRequest);
/**
*
* Returns a list of DataflowEndpoint
groups.
*
*
* @param listDataflowEndpointGroupsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListDataflowEndpointGroups operation returned by the service.
* @sample AWSGroundStationAsyncHandler.ListDataflowEndpointGroups
* @see AWS API Documentation
*/
java.util.concurrent.Future listDataflowEndpointGroupsAsync(
ListDataflowEndpointGroupsRequest listDataflowEndpointGroupsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* List existing ephemerides.
*
*
* @param listEphemeridesRequest
* @return A Java Future containing the result of the ListEphemerides operation returned by the service.
* @sample AWSGroundStationAsync.ListEphemerides
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listEphemeridesAsync(ListEphemeridesRequest listEphemeridesRequest);
/**
*
* List existing ephemerides.
*
*
* @param listEphemeridesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListEphemerides operation returned by the service.
* @sample AWSGroundStationAsyncHandler.ListEphemerides
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listEphemeridesAsync(ListEphemeridesRequest listEphemeridesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of ground stations.
*
*
* @param listGroundStationsRequest
* @return A Java Future containing the result of the ListGroundStations operation returned by the service.
* @sample AWSGroundStationAsync.ListGroundStations
* @see AWS API Documentation
*/
java.util.concurrent.Future listGroundStationsAsync(ListGroundStationsRequest listGroundStationsRequest);
/**
*
* Returns a list of ground stations.
*
*
* @param listGroundStationsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListGroundStations operation returned by the service.
* @sample AWSGroundStationAsyncHandler.ListGroundStations
* @see AWS API Documentation
*/
java.util.concurrent.Future listGroundStationsAsync(ListGroundStationsRequest listGroundStationsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of mission profiles.
*
*
* @param listMissionProfilesRequest
* @return A Java Future containing the result of the ListMissionProfiles operation returned by the service.
* @sample AWSGroundStationAsync.ListMissionProfiles
* @see AWS API Documentation
*/
java.util.concurrent.Future listMissionProfilesAsync(ListMissionProfilesRequest listMissionProfilesRequest);
/**
*
* Returns a list of mission profiles.
*
*
* @param listMissionProfilesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListMissionProfiles operation returned by the service.
* @sample AWSGroundStationAsyncHandler.ListMissionProfiles
* @see AWS API Documentation
*/
java.util.concurrent.Future listMissionProfilesAsync(ListMissionProfilesRequest listMissionProfilesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of satellites.
*
*
* @param listSatellitesRequest
* @return A Java Future containing the result of the ListSatellites operation returned by the service.
* @sample AWSGroundStationAsync.ListSatellites
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listSatellitesAsync(ListSatellitesRequest listSatellitesRequest);
/**
*
* Returns a list of satellites.
*
*
* @param listSatellitesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListSatellites operation returned by the service.
* @sample AWSGroundStationAsyncHandler.ListSatellites
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listSatellitesAsync(ListSatellitesRequest listSatellitesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of tags for a specified resource.
*
*
* @param listTagsForResourceRequest
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* @sample AWSGroundStationAsync.ListTagsForResource
* @see AWS API Documentation
*/
java.util.concurrent.Future listTagsForResourceAsync(ListTagsForResourceRequest listTagsForResourceRequest);
/**
*
* Returns a list of tags for a specified resource.
*
*
* @param listTagsForResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* @sample AWSGroundStationAsyncHandler.ListTagsForResource
* @see AWS API Documentation
*/
java.util.concurrent.Future listTagsForResourceAsync(ListTagsForResourceRequest listTagsForResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
*
* For use by AWS Ground Station Agent and shouldn't be called directly.
*
*
*
* Registers a new agent with AWS Ground Station.
*
*
* @param registerAgentRequest
* @return A Java Future containing the result of the RegisterAgent operation returned by the service.
* @sample AWSGroundStationAsync.RegisterAgent
* @see AWS
* API Documentation
*/
java.util.concurrent.Future registerAgentAsync(RegisterAgentRequest registerAgentRequest);
/**
*
*
* For use by AWS Ground Station Agent and shouldn't be called directly.
*
*
*
* Registers a new agent with AWS Ground Station.
*
*
* @param registerAgentRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the RegisterAgent operation returned by the service.
* @sample AWSGroundStationAsyncHandler.RegisterAgent
* @see AWS
* API Documentation
*/
java.util.concurrent.Future registerAgentAsync(RegisterAgentRequest registerAgentRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Reserves a contact using specified parameters.
*
*
* @param reserveContactRequest
* @return A Java Future containing the result of the ReserveContact operation returned by the service.
* @sample AWSGroundStationAsync.ReserveContact
* @see AWS
* API Documentation
*/
java.util.concurrent.Future reserveContactAsync(ReserveContactRequest reserveContactRequest);
/**
*
* Reserves a contact using specified parameters.
*
*
* @param reserveContactRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ReserveContact operation returned by the service.
* @sample AWSGroundStationAsyncHandler.ReserveContact
* @see AWS
* API Documentation
*/
java.util.concurrent.Future reserveContactAsync(ReserveContactRequest reserveContactRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Assigns a tag to a resource.
*
*
* @param tagResourceRequest
* @return A Java Future containing the result of the TagResource operation returned by the service.
* @sample AWSGroundStationAsync.TagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future tagResourceAsync(TagResourceRequest tagResourceRequest);
/**
*
* Assigns a tag to a resource.
*
*
* @param tagResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the TagResource operation returned by the service.
* @sample AWSGroundStationAsyncHandler.TagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future tagResourceAsync(TagResourceRequest tagResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deassigns a resource tag.
*
*
* @param untagResourceRequest
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* @sample AWSGroundStationAsync.UntagResource
* @see AWS
* API Documentation
*/
java.util.concurrent.Future untagResourceAsync(UntagResourceRequest untagResourceRequest);
/**
*
* Deassigns a resource tag.
*
*
* @param untagResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* @sample AWSGroundStationAsyncHandler.UntagResource
* @see AWS
* API Documentation
*/
java.util.concurrent.Future untagResourceAsync(UntagResourceRequest untagResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
*
* For use by AWS Ground Station Agent and shouldn't be called directly.
*
*
*
* Update the status of the agent.
*
*
* @param updateAgentStatusRequest
* @return A Java Future containing the result of the UpdateAgentStatus operation returned by the service.
* @sample AWSGroundStationAsync.UpdateAgentStatus
* @see AWS API Documentation
*/
java.util.concurrent.Future updateAgentStatusAsync(UpdateAgentStatusRequest updateAgentStatusRequest);
/**
*
*
* For use by AWS Ground Station Agent and shouldn't be called directly.
*
*
*
* Update the status of the agent.
*
*
* @param updateAgentStatusRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateAgentStatus operation returned by the service.
* @sample AWSGroundStationAsyncHandler.UpdateAgentStatus
* @see AWS API Documentation
*/
java.util.concurrent.Future updateAgentStatusAsync(UpdateAgentStatusRequest updateAgentStatusRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates the Config
used when scheduling contacts.
*
*
* Updating a Config
will not update the execution parameters for existing future contacts scheduled
* with this Config
.
*
*
* @param updateConfigRequest
* @return A Java Future containing the result of the UpdateConfig operation returned by the service.
* @sample AWSGroundStationAsync.UpdateConfig
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateConfigAsync(UpdateConfigRequest updateConfigRequest);
/**
*
* Updates the Config
used when scheduling contacts.
*
*
* Updating a Config
will not update the execution parameters for existing future contacts scheduled
* with this Config
.
*
*
* @param updateConfigRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateConfig operation returned by the service.
* @sample AWSGroundStationAsyncHandler.UpdateConfig
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateConfigAsync(UpdateConfigRequest updateConfigRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates an existing ephemeris
*
*
* @param updateEphemerisRequest
* @return A Java Future containing the result of the UpdateEphemeris operation returned by the service.
* @sample AWSGroundStationAsync.UpdateEphemeris
* @see AWS
* API Documentation
*/
java.util.concurrent.Future updateEphemerisAsync(UpdateEphemerisRequest updateEphemerisRequest);
/**
*
* Updates an existing ephemeris
*
*
* @param updateEphemerisRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateEphemeris operation returned by the service.
* @sample AWSGroundStationAsyncHandler.UpdateEphemeris
* @see AWS
* API Documentation
*/
java.util.concurrent.Future updateEphemerisAsync(UpdateEphemerisRequest updateEphemerisRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates a mission profile.
*
*
* Updating a mission profile will not update the execution parameters for existing future contacts.
*
*
* @param updateMissionProfileRequest
* @return A Java Future containing the result of the UpdateMissionProfile operation returned by the service.
* @sample AWSGroundStationAsync.UpdateMissionProfile
* @see AWS API Documentation
*/
java.util.concurrent.Future updateMissionProfileAsync(UpdateMissionProfileRequest updateMissionProfileRequest);
/**
*
* Updates a mission profile.
*
*
* Updating a mission profile will not update the execution parameters for existing future contacts.
*
*
* @param updateMissionProfileRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateMissionProfile operation returned by the service.
* @sample AWSGroundStationAsyncHandler.UpdateMissionProfile
* @see AWS API Documentation
*/
java.util.concurrent.Future updateMissionProfileAsync(UpdateMissionProfileRequest updateMissionProfileRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
}