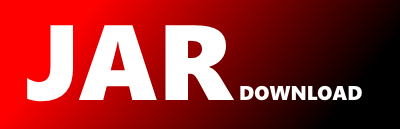
com.amazonaws.services.groundstation.AWSGroundStationClient Maven / Gradle / Ivy
Show all versions of aws-java-sdk-groundstation Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.groundstation;
import org.w3c.dom.*;
import java.net.*;
import java.util.*;
import javax.annotation.Generated;
import org.apache.commons.logging.*;
import com.amazonaws.*;
import com.amazonaws.annotation.SdkInternalApi;
import com.amazonaws.auth.*;
import com.amazonaws.handlers.*;
import com.amazonaws.http.*;
import com.amazonaws.internal.*;
import com.amazonaws.internal.auth.*;
import com.amazonaws.metrics.*;
import com.amazonaws.regions.*;
import com.amazonaws.transform.*;
import com.amazonaws.util.*;
import com.amazonaws.protocol.json.*;
import com.amazonaws.util.AWSRequestMetrics.Field;
import com.amazonaws.annotation.ThreadSafe;
import com.amazonaws.client.AwsSyncClientParams;
import com.amazonaws.client.builder.AdvancedConfig;
import com.amazonaws.services.groundstation.AWSGroundStationClientBuilder;
import com.amazonaws.services.groundstation.waiters.AWSGroundStationWaiters;
import com.amazonaws.AmazonServiceException;
import com.amazonaws.services.groundstation.model.*;
import com.amazonaws.services.groundstation.model.transform.*;
/**
* Client for accessing AWS Ground Station. All service calls made using this client are blocking, and will not return
* until the service call completes.
*
*
* Welcome to the AWS Ground Station API Reference. AWS Ground Station is a fully managed service that enables you to
* control satellite communications, downlink and process satellite data, and scale your satellite operations
* efficiently and cost-effectively without having to build or manage your own ground station infrastructure.
*
*/
@ThreadSafe
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class AWSGroundStationClient extends AmazonWebServiceClient implements AWSGroundStation {
/** Provider for AWS credentials. */
private final AWSCredentialsProvider awsCredentialsProvider;
private static final Log log = LogFactory.getLog(AWSGroundStation.class);
/** Default signing name for the service. */
private static final String DEFAULT_SIGNING_NAME = "groundstation";
private volatile AWSGroundStationWaiters waiters;
/** Client configuration factory providing ClientConfigurations tailored to this client */
protected static final ClientConfigurationFactory configFactory = new ClientConfigurationFactory();
private final AdvancedConfig advancedConfig;
private static final com.amazonaws.protocol.json.SdkJsonProtocolFactory protocolFactory = new com.amazonaws.protocol.json.SdkJsonProtocolFactory(
new JsonClientMetadata()
.withProtocolVersion("1.1")
.withSupportsCbor(false)
.withSupportsIon(false)
.withContentTypeOverride("application/json")
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("InvalidParameterException").withExceptionUnmarshaller(
com.amazonaws.services.groundstation.model.transform.InvalidParameterExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ResourceNotFoundException").withExceptionUnmarshaller(
com.amazonaws.services.groundstation.model.transform.ResourceNotFoundExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("DependencyException").withExceptionUnmarshaller(
com.amazonaws.services.groundstation.model.transform.DependencyExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ResourceLimitExceededException").withExceptionUnmarshaller(
com.amazonaws.services.groundstation.model.transform.ResourceLimitExceededExceptionUnmarshaller.getInstance()))
.withBaseServiceExceptionClass(com.amazonaws.services.groundstation.model.AWSGroundStationException.class));
public static AWSGroundStationClientBuilder builder() {
return AWSGroundStationClientBuilder.standard();
}
/**
* Constructs a new client to invoke service methods on AWS Ground Station using the specified parameters.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param clientParams
* Object providing client parameters.
*/
AWSGroundStationClient(AwsSyncClientParams clientParams) {
this(clientParams, false);
}
/**
* Constructs a new client to invoke service methods on AWS Ground Station using the specified parameters.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param clientParams
* Object providing client parameters.
*/
AWSGroundStationClient(AwsSyncClientParams clientParams, boolean endpointDiscoveryEnabled) {
super(clientParams);
this.awsCredentialsProvider = clientParams.getCredentialsProvider();
this.advancedConfig = clientParams.getAdvancedConfig();
init();
}
private void init() {
setServiceNameIntern(DEFAULT_SIGNING_NAME);
setEndpointPrefix(ENDPOINT_PREFIX);
// calling this.setEndPoint(...) will also modify the signer accordingly
setEndpoint("groundstation.us-east-1.amazonaws.com");
HandlerChainFactory chainFactory = new HandlerChainFactory();
requestHandler2s.addAll(chainFactory.newRequestHandlerChain("/com/amazonaws/services/groundstation/request.handlers"));
requestHandler2s.addAll(chainFactory.newRequestHandler2Chain("/com/amazonaws/services/groundstation/request.handler2s"));
requestHandler2s.addAll(chainFactory.getGlobalHandlers());
}
/**
*
* Cancels a contact with a specified contact ID.
*
*
* @param cancelContactRequest
* @return Result of the CancelContact operation returned by the service.
* @throws InvalidParameterException
* One or more parameters are not valid.
* @throws DependencyException
* Dependency encountered an error.
* @throws ResourceNotFoundException
* Resource was not found.
* @sample AWSGroundStation.CancelContact
* @see AWS
* API Documentation
*/
@Override
public CancelContactResult cancelContact(CancelContactRequest request) {
request = beforeClientExecution(request);
return executeCancelContact(request);
}
@SdkInternalApi
final CancelContactResult executeCancelContact(CancelContactRequest cancelContactRequest) {
ExecutionContext executionContext = createExecutionContext(cancelContactRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CancelContactRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(cancelContactRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "GroundStation");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CancelContact");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new CancelContactResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a Config
with the specified configData
parameters.
*
*
* Only one type of configData
can be specified.
*
*
* @param createConfigRequest
* @return Result of the CreateConfig operation returned by the service.
* @throws InvalidParameterException
* One or more parameters are not valid.
* @throws DependencyException
* Dependency encountered an error.
* @throws ResourceLimitExceededException
* Account limits for this resource have been exceeded.
* @throws ResourceNotFoundException
* Resource was not found.
* @sample AWSGroundStation.CreateConfig
* @see AWS API
* Documentation
*/
@Override
public CreateConfigResult createConfig(CreateConfigRequest request) {
request = beforeClientExecution(request);
return executeCreateConfig(request);
}
@SdkInternalApi
final CreateConfigResult executeCreateConfig(CreateConfigRequest createConfigRequest) {
ExecutionContext executionContext = createExecutionContext(createConfigRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateConfigRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createConfigRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "GroundStation");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateConfig");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new CreateConfigResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a DataflowEndpoint
group containing the specified list of DataflowEndpoint
* objects.
*
*
* The name
field in each endpoint is used in your mission profile DataflowEndpointConfig
* to specify which endpoints to use during a contact.
*
*
* When a contact uses multiple DataflowEndpointConfig
objects, each Config
must match a
* DataflowEndpoint
in the same group.
*
*
* @param createDataflowEndpointGroupRequest
* @return Result of the CreateDataflowEndpointGroup operation returned by the service.
* @throws InvalidParameterException
* One or more parameters are not valid.
* @throws DependencyException
* Dependency encountered an error.
* @throws ResourceNotFoundException
* Resource was not found.
* @sample AWSGroundStation.CreateDataflowEndpointGroup
* @see AWS API Documentation
*/
@Override
public CreateDataflowEndpointGroupResult createDataflowEndpointGroup(CreateDataflowEndpointGroupRequest request) {
request = beforeClientExecution(request);
return executeCreateDataflowEndpointGroup(request);
}
@SdkInternalApi
final CreateDataflowEndpointGroupResult executeCreateDataflowEndpointGroup(CreateDataflowEndpointGroupRequest createDataflowEndpointGroupRequest) {
ExecutionContext executionContext = createExecutionContext(createDataflowEndpointGroupRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateDataflowEndpointGroupRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(createDataflowEndpointGroupRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "GroundStation");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateDataflowEndpointGroup");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new CreateDataflowEndpointGroupResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates an Ephemeris with the specified EphemerisData
.
*
*
* @param createEphemerisRequest
* @return Result of the CreateEphemeris operation returned by the service.
* @throws InvalidParameterException
* One or more parameters are not valid.
* @throws DependencyException
* Dependency encountered an error.
* @throws ResourceNotFoundException
* Resource was not found.
* @sample AWSGroundStation.CreateEphemeris
* @see AWS
* API Documentation
*/
@Override
public CreateEphemerisResult createEphemeris(CreateEphemerisRequest request) {
request = beforeClientExecution(request);
return executeCreateEphemeris(request);
}
@SdkInternalApi
final CreateEphemerisResult executeCreateEphemeris(CreateEphemerisRequest createEphemerisRequest) {
ExecutionContext executionContext = createExecutionContext(createEphemerisRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateEphemerisRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createEphemerisRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "GroundStation");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateEphemeris");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new CreateEphemerisResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a mission profile.
*
*
* dataflowEdges
is a list of lists of strings. Each lower level list of strings has two elements: a
* from ARN and a to ARN.
*
*
* @param createMissionProfileRequest
* @return Result of the CreateMissionProfile operation returned by the service.
* @throws InvalidParameterException
* One or more parameters are not valid.
* @throws DependencyException
* Dependency encountered an error.
* @throws ResourceNotFoundException
* Resource was not found.
* @sample AWSGroundStation.CreateMissionProfile
* @see AWS API Documentation
*/
@Override
public CreateMissionProfileResult createMissionProfile(CreateMissionProfileRequest request) {
request = beforeClientExecution(request);
return executeCreateMissionProfile(request);
}
@SdkInternalApi
final CreateMissionProfileResult executeCreateMissionProfile(CreateMissionProfileRequest createMissionProfileRequest) {
ExecutionContext executionContext = createExecutionContext(createMissionProfileRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateMissionProfileRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createMissionProfileRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "GroundStation");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateMissionProfile");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new CreateMissionProfileResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes a Config
.
*
*
* @param deleteConfigRequest
* @return Result of the DeleteConfig operation returned by the service.
* @throws InvalidParameterException
* One or more parameters are not valid.
* @throws DependencyException
* Dependency encountered an error.
* @throws ResourceNotFoundException
* Resource was not found.
* @sample AWSGroundStation.DeleteConfig
* @see AWS API
* Documentation
*/
@Override
public DeleteConfigResult deleteConfig(DeleteConfigRequest request) {
request = beforeClientExecution(request);
return executeDeleteConfig(request);
}
@SdkInternalApi
final DeleteConfigResult executeDeleteConfig(DeleteConfigRequest deleteConfigRequest) {
ExecutionContext executionContext = createExecutionContext(deleteConfigRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteConfigRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteConfigRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "GroundStation");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteConfig");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeleteConfigResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes a dataflow endpoint group.
*
*
* @param deleteDataflowEndpointGroupRequest
* @return Result of the DeleteDataflowEndpointGroup operation returned by the service.
* @throws InvalidParameterException
* One or more parameters are not valid.
* @throws DependencyException
* Dependency encountered an error.
* @throws ResourceNotFoundException
* Resource was not found.
* @sample AWSGroundStation.DeleteDataflowEndpointGroup
* @see AWS API Documentation
*/
@Override
public DeleteDataflowEndpointGroupResult deleteDataflowEndpointGroup(DeleteDataflowEndpointGroupRequest request) {
request = beforeClientExecution(request);
return executeDeleteDataflowEndpointGroup(request);
}
@SdkInternalApi
final DeleteDataflowEndpointGroupResult executeDeleteDataflowEndpointGroup(DeleteDataflowEndpointGroupRequest deleteDataflowEndpointGroupRequest) {
ExecutionContext executionContext = createExecutionContext(deleteDataflowEndpointGroupRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteDataflowEndpointGroupRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(deleteDataflowEndpointGroupRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "GroundStation");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteDataflowEndpointGroup");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DeleteDataflowEndpointGroupResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes an ephemeris
*
*
* @param deleteEphemerisRequest
* @return Result of the DeleteEphemeris operation returned by the service.
* @throws InvalidParameterException
* One or more parameters are not valid.
* @throws DependencyException
* Dependency encountered an error.
* @throws ResourceNotFoundException
* Resource was not found.
* @sample AWSGroundStation.DeleteEphemeris
* @see AWS
* API Documentation
*/
@Override
public DeleteEphemerisResult deleteEphemeris(DeleteEphemerisRequest request) {
request = beforeClientExecution(request);
return executeDeleteEphemeris(request);
}
@SdkInternalApi
final DeleteEphemerisResult executeDeleteEphemeris(DeleteEphemerisRequest deleteEphemerisRequest) {
ExecutionContext executionContext = createExecutionContext(deleteEphemerisRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteEphemerisRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteEphemerisRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "GroundStation");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteEphemeris");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeleteEphemerisResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes a mission profile.
*
*
* @param deleteMissionProfileRequest
* @return Result of the DeleteMissionProfile operation returned by the service.
* @throws InvalidParameterException
* One or more parameters are not valid.
* @throws DependencyException
* Dependency encountered an error.
* @throws ResourceNotFoundException
* Resource was not found.
* @sample AWSGroundStation.DeleteMissionProfile
* @see AWS API Documentation
*/
@Override
public DeleteMissionProfileResult deleteMissionProfile(DeleteMissionProfileRequest request) {
request = beforeClientExecution(request);
return executeDeleteMissionProfile(request);
}
@SdkInternalApi
final DeleteMissionProfileResult executeDeleteMissionProfile(DeleteMissionProfileRequest deleteMissionProfileRequest) {
ExecutionContext executionContext = createExecutionContext(deleteMissionProfileRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteMissionProfileRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteMissionProfileRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "GroundStation");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteMissionProfile");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeleteMissionProfileResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Describes an existing contact.
*
*
* @param describeContactRequest
* @return Result of the DescribeContact operation returned by the service.
* @throws InvalidParameterException
* One or more parameters are not valid.
* @throws DependencyException
* Dependency encountered an error.
* @throws ResourceNotFoundException
* Resource was not found.
* @sample AWSGroundStation.DescribeContact
* @see AWS
* API Documentation
*/
@Override
public DescribeContactResult describeContact(DescribeContactRequest request) {
request = beforeClientExecution(request);
return executeDescribeContact(request);
}
@SdkInternalApi
final DescribeContactResult executeDescribeContact(DescribeContactRequest describeContactRequest) {
ExecutionContext executionContext = createExecutionContext(describeContactRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeContactRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(describeContactRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "GroundStation");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeContact");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DescribeContactResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Describes an existing ephemeris.
*
*
* @param describeEphemerisRequest
* @return Result of the DescribeEphemeris operation returned by the service.
* @throws InvalidParameterException
* One or more parameters are not valid.
* @throws DependencyException
* Dependency encountered an error.
* @throws ResourceNotFoundException
* Resource was not found.
* @sample AWSGroundStation.DescribeEphemeris
* @see AWS API Documentation
*/
@Override
public DescribeEphemerisResult describeEphemeris(DescribeEphemerisRequest request) {
request = beforeClientExecution(request);
return executeDescribeEphemeris(request);
}
@SdkInternalApi
final DescribeEphemerisResult executeDescribeEphemeris(DescribeEphemerisRequest describeEphemerisRequest) {
ExecutionContext executionContext = createExecutionContext(describeEphemerisRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeEphemerisRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(describeEphemerisRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "GroundStation");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeEphemeris");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DescribeEphemerisResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
*
* For use by AWS Ground Station Agent and shouldn't be called directly.
*
*
*
* Gets the latest configuration information for a registered agent.
*
*
* @param getAgentConfigurationRequest
* @return Result of the GetAgentConfiguration operation returned by the service.
* @throws InvalidParameterException
* One or more parameters are not valid.
* @throws DependencyException
* Dependency encountered an error.
* @throws ResourceNotFoundException
* Resource was not found.
* @sample AWSGroundStation.GetAgentConfiguration
* @see AWS API Documentation
*/
@Override
public GetAgentConfigurationResult getAgentConfiguration(GetAgentConfigurationRequest request) {
request = beforeClientExecution(request);
return executeGetAgentConfiguration(request);
}
@SdkInternalApi
final GetAgentConfigurationResult executeGetAgentConfiguration(GetAgentConfigurationRequest getAgentConfigurationRequest) {
ExecutionContext executionContext = createExecutionContext(getAgentConfigurationRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetAgentConfigurationRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getAgentConfigurationRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "GroundStation");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetAgentConfiguration");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new GetAgentConfigurationResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns Config
information.
*
*
* Only one Config
response can be returned.
*
*
* @param getConfigRequest
* @return Result of the GetConfig operation returned by the service.
* @throws InvalidParameterException
* One or more parameters are not valid.
* @throws DependencyException
* Dependency encountered an error.
* @throws ResourceNotFoundException
* Resource was not found.
* @sample AWSGroundStation.GetConfig
* @see AWS API
* Documentation
*/
@Override
public GetConfigResult getConfig(GetConfigRequest request) {
request = beforeClientExecution(request);
return executeGetConfig(request);
}
@SdkInternalApi
final GetConfigResult executeGetConfig(GetConfigRequest getConfigRequest) {
ExecutionContext executionContext = createExecutionContext(getConfigRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetConfigRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getConfigRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "GroundStation");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetConfig");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true).withHasStreamingSuccessResponse(false), new GetConfigResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns the dataflow endpoint group.
*
*
* @param getDataflowEndpointGroupRequest
* @return Result of the GetDataflowEndpointGroup operation returned by the service.
* @throws InvalidParameterException
* One or more parameters are not valid.
* @throws DependencyException
* Dependency encountered an error.
* @throws ResourceNotFoundException
* Resource was not found.
* @sample AWSGroundStation.GetDataflowEndpointGroup
* @see AWS API Documentation
*/
@Override
public GetDataflowEndpointGroupResult getDataflowEndpointGroup(GetDataflowEndpointGroupRequest request) {
request = beforeClientExecution(request);
return executeGetDataflowEndpointGroup(request);
}
@SdkInternalApi
final GetDataflowEndpointGroupResult executeGetDataflowEndpointGroup(GetDataflowEndpointGroupRequest getDataflowEndpointGroupRequest) {
ExecutionContext executionContext = createExecutionContext(getDataflowEndpointGroupRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetDataflowEndpointGroupRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(getDataflowEndpointGroupRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "GroundStation");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetDataflowEndpointGroup");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new GetDataflowEndpointGroupResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns the number of reserved minutes used by account.
*
*
* @param getMinuteUsageRequest
* @return Result of the GetMinuteUsage operation returned by the service.
* @throws InvalidParameterException
* One or more parameters are not valid.
* @throws DependencyException
* Dependency encountered an error.
* @throws ResourceNotFoundException
* Resource was not found.
* @sample AWSGroundStation.GetMinuteUsage
* @see AWS
* API Documentation
*/
@Override
public GetMinuteUsageResult getMinuteUsage(GetMinuteUsageRequest request) {
request = beforeClientExecution(request);
return executeGetMinuteUsage(request);
}
@SdkInternalApi
final GetMinuteUsageResult executeGetMinuteUsage(GetMinuteUsageRequest getMinuteUsageRequest) {
ExecutionContext executionContext = createExecutionContext(getMinuteUsageRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetMinuteUsageRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getMinuteUsageRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "GroundStation");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetMinuteUsage");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new GetMinuteUsageResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns a mission profile.
*
*
* @param getMissionProfileRequest
* @return Result of the GetMissionProfile operation returned by the service.
* @throws InvalidParameterException
* One or more parameters are not valid.
* @throws DependencyException
* Dependency encountered an error.
* @throws ResourceNotFoundException
* Resource was not found.
* @sample AWSGroundStation.GetMissionProfile
* @see AWS API Documentation
*/
@Override
public GetMissionProfileResult getMissionProfile(GetMissionProfileRequest request) {
request = beforeClientExecution(request);
return executeGetMissionProfile(request);
}
@SdkInternalApi
final GetMissionProfileResult executeGetMissionProfile(GetMissionProfileRequest getMissionProfileRequest) {
ExecutionContext executionContext = createExecutionContext(getMissionProfileRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetMissionProfileRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getMissionProfileRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "GroundStation");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetMissionProfile");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new GetMissionProfileResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns a satellite.
*
*
* @param getSatelliteRequest
* @return Result of the GetSatellite operation returned by the service.
* @throws InvalidParameterException
* One or more parameters are not valid.
* @throws DependencyException
* Dependency encountered an error.
* @throws ResourceNotFoundException
* Resource was not found.
* @sample AWSGroundStation.GetSatellite
* @see AWS API
* Documentation
*/
@Override
public GetSatelliteResult getSatellite(GetSatelliteRequest request) {
request = beforeClientExecution(request);
return executeGetSatellite(request);
}
@SdkInternalApi
final GetSatelliteResult executeGetSatellite(GetSatelliteRequest getSatelliteRequest) {
ExecutionContext executionContext = createExecutionContext(getSatelliteRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetSatelliteRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getSatelliteRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "GroundStation");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetSatellite");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new GetSatelliteResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns a list of Config
objects.
*
*
* @param listConfigsRequest
* @return Result of the ListConfigs operation returned by the service.
* @throws InvalidParameterException
* One or more parameters are not valid.
* @throws DependencyException
* Dependency encountered an error.
* @throws ResourceNotFoundException
* Resource was not found.
* @sample AWSGroundStation.ListConfigs
* @see AWS API
* Documentation
*/
@Override
public ListConfigsResult listConfigs(ListConfigsRequest request) {
request = beforeClientExecution(request);
return executeListConfigs(request);
}
@SdkInternalApi
final ListConfigsResult executeListConfigs(ListConfigsRequest listConfigsRequest) {
ExecutionContext executionContext = createExecutionContext(listConfigsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListConfigsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listConfigsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "GroundStation");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListConfigs");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListConfigsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns a list of contacts.
*
*
* If statusList
contains AVAILABLE, the request must include groundStation
,
* missionprofileArn
, and satelliteArn
.
*
*
* @param listContactsRequest
* @return Result of the ListContacts operation returned by the service.
* @throws InvalidParameterException
* One or more parameters are not valid.
* @throws DependencyException
* Dependency encountered an error.
* @throws ResourceNotFoundException
* Resource was not found.
* @sample AWSGroundStation.ListContacts
* @see AWS API
* Documentation
*/
@Override
public ListContactsResult listContacts(ListContactsRequest request) {
request = beforeClientExecution(request);
return executeListContacts(request);
}
@SdkInternalApi
final ListContactsResult executeListContacts(ListContactsRequest listContactsRequest) {
ExecutionContext executionContext = createExecutionContext(listContactsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListContactsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listContactsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "GroundStation");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListContacts");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListContactsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns a list of DataflowEndpoint
groups.
*
*
* @param listDataflowEndpointGroupsRequest
* @return Result of the ListDataflowEndpointGroups operation returned by the service.
* @throws InvalidParameterException
* One or more parameters are not valid.
* @throws DependencyException
* Dependency encountered an error.
* @throws ResourceNotFoundException
* Resource was not found.
* @sample AWSGroundStation.ListDataflowEndpointGroups
* @see AWS API Documentation
*/
@Override
public ListDataflowEndpointGroupsResult listDataflowEndpointGroups(ListDataflowEndpointGroupsRequest request) {
request = beforeClientExecution(request);
return executeListDataflowEndpointGroups(request);
}
@SdkInternalApi
final ListDataflowEndpointGroupsResult executeListDataflowEndpointGroups(ListDataflowEndpointGroupsRequest listDataflowEndpointGroupsRequest) {
ExecutionContext executionContext = createExecutionContext(listDataflowEndpointGroupsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListDataflowEndpointGroupsRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(listDataflowEndpointGroupsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "GroundStation");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListDataflowEndpointGroups");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new ListDataflowEndpointGroupsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* List existing ephemerides.
*
*
* @param listEphemeridesRequest
* @return Result of the ListEphemerides operation returned by the service.
* @throws InvalidParameterException
* One or more parameters are not valid.
* @throws DependencyException
* Dependency encountered an error.
* @throws ResourceNotFoundException
* Resource was not found.
* @sample AWSGroundStation.ListEphemerides
* @see AWS
* API Documentation
*/
@Override
public ListEphemeridesResult listEphemerides(ListEphemeridesRequest request) {
request = beforeClientExecution(request);
return executeListEphemerides(request);
}
@SdkInternalApi
final ListEphemeridesResult executeListEphemerides(ListEphemeridesRequest listEphemeridesRequest) {
ExecutionContext executionContext = createExecutionContext(listEphemeridesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListEphemeridesRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listEphemeridesRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "GroundStation");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListEphemerides");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListEphemeridesResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns a list of ground stations.
*
*
* @param listGroundStationsRequest
* @return Result of the ListGroundStations operation returned by the service.
* @throws InvalidParameterException
* One or more parameters are not valid.
* @throws DependencyException
* Dependency encountered an error.
* @throws ResourceNotFoundException
* Resource was not found.
* @sample AWSGroundStation.ListGroundStations
* @see AWS API Documentation
*/
@Override
public ListGroundStationsResult listGroundStations(ListGroundStationsRequest request) {
request = beforeClientExecution(request);
return executeListGroundStations(request);
}
@SdkInternalApi
final ListGroundStationsResult executeListGroundStations(ListGroundStationsRequest listGroundStationsRequest) {
ExecutionContext executionContext = createExecutionContext(listGroundStationsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListGroundStationsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listGroundStationsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "GroundStation");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListGroundStations");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListGroundStationsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns a list of mission profiles.
*
*
* @param listMissionProfilesRequest
* @return Result of the ListMissionProfiles operation returned by the service.
* @throws InvalidParameterException
* One or more parameters are not valid.
* @throws DependencyException
* Dependency encountered an error.
* @throws ResourceNotFoundException
* Resource was not found.
* @sample AWSGroundStation.ListMissionProfiles
* @see AWS API Documentation
*/
@Override
public ListMissionProfilesResult listMissionProfiles(ListMissionProfilesRequest request) {
request = beforeClientExecution(request);
return executeListMissionProfiles(request);
}
@SdkInternalApi
final ListMissionProfilesResult executeListMissionProfiles(ListMissionProfilesRequest listMissionProfilesRequest) {
ExecutionContext executionContext = createExecutionContext(listMissionProfilesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListMissionProfilesRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listMissionProfilesRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "GroundStation");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListMissionProfiles");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListMissionProfilesResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns a list of satellites.
*
*
* @param listSatellitesRequest
* @return Result of the ListSatellites operation returned by the service.
* @throws InvalidParameterException
* One or more parameters are not valid.
* @throws DependencyException
* Dependency encountered an error.
* @throws ResourceNotFoundException
* Resource was not found.
* @sample AWSGroundStation.ListSatellites
* @see AWS
* API Documentation
*/
@Override
public ListSatellitesResult listSatellites(ListSatellitesRequest request) {
request = beforeClientExecution(request);
return executeListSatellites(request);
}
@SdkInternalApi
final ListSatellitesResult executeListSatellites(ListSatellitesRequest listSatellitesRequest) {
ExecutionContext executionContext = createExecutionContext(listSatellitesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListSatellitesRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listSatellitesRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "GroundStation");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListSatellites");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListSatellitesResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns a list of tags for a specified resource.
*
*
* @param listTagsForResourceRequest
* @return Result of the ListTagsForResource operation returned by the service.
* @throws InvalidParameterException
* One or more parameters are not valid.
* @throws DependencyException
* Dependency encountered an error.
* @throws ResourceNotFoundException
* Resource was not found.
* @sample AWSGroundStation.ListTagsForResource
* @see AWS API Documentation
*/
@Override
public ListTagsForResourceResult listTagsForResource(ListTagsForResourceRequest request) {
request = beforeClientExecution(request);
return executeListTagsForResource(request);
}
@SdkInternalApi
final ListTagsForResourceResult executeListTagsForResource(ListTagsForResourceRequest listTagsForResourceRequest) {
ExecutionContext executionContext = createExecutionContext(listTagsForResourceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListTagsForResourceRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listTagsForResourceRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "GroundStation");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListTagsForResource");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListTagsForResourceResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
*
* For use by AWS Ground Station Agent and shouldn't be called directly.
*
*
*
* Registers a new agent with AWS Ground Station.
*
*
* @param registerAgentRequest
* @return Result of the RegisterAgent operation returned by the service.
* @throws InvalidParameterException
* One or more parameters are not valid.
* @throws DependencyException
* Dependency encountered an error.
* @throws ResourceNotFoundException
* Resource was not found.
* @sample AWSGroundStation.RegisterAgent
* @see AWS
* API Documentation
*/
@Override
public RegisterAgentResult registerAgent(RegisterAgentRequest request) {
request = beforeClientExecution(request);
return executeRegisterAgent(request);
}
@SdkInternalApi
final RegisterAgentResult executeRegisterAgent(RegisterAgentRequest registerAgentRequest) {
ExecutionContext executionContext = createExecutionContext(registerAgentRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new RegisterAgentRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(registerAgentRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "GroundStation");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "RegisterAgent");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new RegisterAgentResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Reserves a contact using specified parameters.
*
*
* @param reserveContactRequest
* @return Result of the ReserveContact operation returned by the service.
* @throws InvalidParameterException
* One or more parameters are not valid.
* @throws DependencyException
* Dependency encountered an error.
* @throws ResourceNotFoundException
* Resource was not found.
* @sample AWSGroundStation.ReserveContact
* @see AWS
* API Documentation
*/
@Override
public ReserveContactResult reserveContact(ReserveContactRequest request) {
request = beforeClientExecution(request);
return executeReserveContact(request);
}
@SdkInternalApi
final ReserveContactResult executeReserveContact(ReserveContactRequest reserveContactRequest) {
ExecutionContext executionContext = createExecutionContext(reserveContactRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ReserveContactRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(reserveContactRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "GroundStation");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ReserveContact");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ReserveContactResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Assigns a tag to a resource.
*
*
* @param tagResourceRequest
* @return Result of the TagResource operation returned by the service.
* @throws InvalidParameterException
* One or more parameters are not valid.
* @throws DependencyException
* Dependency encountered an error.
* @throws ResourceNotFoundException
* Resource was not found.
* @sample AWSGroundStation.TagResource
* @see AWS API
* Documentation
*/
@Override
public TagResourceResult tagResource(TagResourceRequest request) {
request = beforeClientExecution(request);
return executeTagResource(request);
}
@SdkInternalApi
final TagResourceResult executeTagResource(TagResourceRequest tagResourceRequest) {
ExecutionContext executionContext = createExecutionContext(tagResourceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new TagResourceRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(tagResourceRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "GroundStation");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "TagResource");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new TagResourceResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deassigns a resource tag.
*
*
* @param untagResourceRequest
* @return Result of the UntagResource operation returned by the service.
* @throws InvalidParameterException
* One or more parameters are not valid.
* @throws DependencyException
* Dependency encountered an error.
* @throws ResourceNotFoundException
* Resource was not found.
* @sample AWSGroundStation.UntagResource
* @see AWS
* API Documentation
*/
@Override
public UntagResourceResult untagResource(UntagResourceRequest request) {
request = beforeClientExecution(request);
return executeUntagResource(request);
}
@SdkInternalApi
final UntagResourceResult executeUntagResource(UntagResourceRequest untagResourceRequest) {
ExecutionContext executionContext = createExecutionContext(untagResourceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UntagResourceRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(untagResourceRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "GroundStation");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UntagResource");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new UntagResourceResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
*
* For use by AWS Ground Station Agent and shouldn't be called directly.
*
*
*
* Update the status of the agent.
*
*
* @param updateAgentStatusRequest
* @return Result of the UpdateAgentStatus operation returned by the service.
* @throws InvalidParameterException
* One or more parameters are not valid.
* @throws DependencyException
* Dependency encountered an error.
* @throws ResourceNotFoundException
* Resource was not found.
* @sample AWSGroundStation.UpdateAgentStatus
* @see AWS API Documentation
*/
@Override
public UpdateAgentStatusResult updateAgentStatus(UpdateAgentStatusRequest request) {
request = beforeClientExecution(request);
return executeUpdateAgentStatus(request);
}
@SdkInternalApi
final UpdateAgentStatusResult executeUpdateAgentStatus(UpdateAgentStatusRequest updateAgentStatusRequest) {
ExecutionContext executionContext = createExecutionContext(updateAgentStatusRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateAgentStatusRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(updateAgentStatusRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "GroundStation");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UpdateAgentStatus");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new UpdateAgentStatusResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Updates the Config
used when scheduling contacts.
*
*
* Updating a Config
will not update the execution parameters for existing future contacts scheduled
* with this Config
.
*
*
* @param updateConfigRequest
* @return Result of the UpdateConfig operation returned by the service.
* @throws InvalidParameterException
* One or more parameters are not valid.
* @throws DependencyException
* Dependency encountered an error.
* @throws ResourceNotFoundException
* Resource was not found.
* @sample AWSGroundStation.UpdateConfig
* @see AWS API
* Documentation
*/
@Override
public UpdateConfigResult updateConfig(UpdateConfigRequest request) {
request = beforeClientExecution(request);
return executeUpdateConfig(request);
}
@SdkInternalApi
final UpdateConfigResult executeUpdateConfig(UpdateConfigRequest updateConfigRequest) {
ExecutionContext executionContext = createExecutionContext(updateConfigRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateConfigRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(updateConfigRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "GroundStation");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UpdateConfig");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new UpdateConfigResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Updates an existing ephemeris
*
*
* @param updateEphemerisRequest
* @return Result of the UpdateEphemeris operation returned by the service.
* @throws InvalidParameterException
* One or more parameters are not valid.
* @throws DependencyException
* Dependency encountered an error.
* @throws ResourceNotFoundException
* Resource was not found.
* @sample AWSGroundStation.UpdateEphemeris
* @see AWS
* API Documentation
*/
@Override
public UpdateEphemerisResult updateEphemeris(UpdateEphemerisRequest request) {
request = beforeClientExecution(request);
return executeUpdateEphemeris(request);
}
@SdkInternalApi
final UpdateEphemerisResult executeUpdateEphemeris(UpdateEphemerisRequest updateEphemerisRequest) {
ExecutionContext executionContext = createExecutionContext(updateEphemerisRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateEphemerisRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(updateEphemerisRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "GroundStation");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UpdateEphemeris");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new UpdateEphemerisResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Updates a mission profile.
*
*
* Updating a mission profile will not update the execution parameters for existing future contacts.
*
*
* @param updateMissionProfileRequest
* @return Result of the UpdateMissionProfile operation returned by the service.
* @throws InvalidParameterException
* One or more parameters are not valid.
* @throws DependencyException
* Dependency encountered an error.
* @throws ResourceNotFoundException
* Resource was not found.
* @sample AWSGroundStation.UpdateMissionProfile
* @see AWS API Documentation
*/
@Override
public UpdateMissionProfileResult updateMissionProfile(UpdateMissionProfileRequest request) {
request = beforeClientExecution(request);
return executeUpdateMissionProfile(request);
}
@SdkInternalApi
final UpdateMissionProfileResult executeUpdateMissionProfile(UpdateMissionProfileRequest updateMissionProfileRequest) {
ExecutionContext executionContext = createExecutionContext(updateMissionProfileRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateMissionProfileRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(updateMissionProfileRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "GroundStation");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UpdateMissionProfile");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new UpdateMissionProfileResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
* Returns additional metadata for a previously executed successful, request, typically used for debugging issues
* where a service isn't acting as expected. This data isn't considered part of the result data returned by an
* operation, so it's available through this separate, diagnostic interface.
*
* Response metadata is only cached for a limited period of time, so if you need to access this extra diagnostic
* information for an executed request, you should use this method to retrieve it as soon as possible after
* executing the request.
*
* @param request
* The originally executed request
*
* @return The response metadata for the specified request, or null if none is available.
*/
public ResponseMetadata getCachedResponseMetadata(AmazonWebServiceRequest request) {
return client.getResponseMetadataForRequest(request);
}
/**
* Normal invoke with authentication. Credentials are required and may be overriden at the request level.
**/
private Response invoke(Request request, HttpResponseHandler> responseHandler,
ExecutionContext executionContext) {
return invoke(request, responseHandler, executionContext, null, null);
}
/**
* Normal invoke with authentication. Credentials are required and may be overriden at the request level.
**/
private Response invoke(Request request, HttpResponseHandler> responseHandler,
ExecutionContext executionContext, URI cachedEndpoint, URI uriFromEndpointTrait) {
executionContext.setCredentialsProvider(CredentialUtils.getCredentialsProvider(request.getOriginalRequest(), awsCredentialsProvider));
return doInvoke(request, responseHandler, executionContext, cachedEndpoint, uriFromEndpointTrait);
}
/**
* Invoke with no authentication. Credentials are not required and any credentials set on the client or request will
* be ignored for this operation.
**/
private Response anonymousInvoke(Request request,
HttpResponseHandler> responseHandler, ExecutionContext executionContext) {
return doInvoke(request, responseHandler, executionContext, null, null);
}
/**
* Invoke the request using the http client. Assumes credentials (or lack thereof) have been configured in the
* ExecutionContext beforehand.
**/
private Response doInvoke(Request request, HttpResponseHandler> responseHandler,
ExecutionContext executionContext, URI discoveredEndpoint, URI uriFromEndpointTrait) {
if (discoveredEndpoint != null) {
request.setEndpoint(discoveredEndpoint);
request.getOriginalRequest().getRequestClientOptions().appendUserAgent("endpoint-discovery");
} else if (uriFromEndpointTrait != null) {
request.setEndpoint(uriFromEndpointTrait);
} else {
request.setEndpoint(endpoint);
}
request.setTimeOffset(timeOffset);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler(new JsonErrorResponseMetadata());
return client.execute(request, responseHandler, errorResponseHandler, executionContext);
}
@com.amazonaws.annotation.SdkInternalApi
static com.amazonaws.protocol.json.SdkJsonProtocolFactory getProtocolFactory() {
return protocolFactory;
}
@Override
public AWSGroundStationWaiters waiters() {
if (waiters == null) {
synchronized (this) {
if (waiters == null) {
waiters = new AWSGroundStationWaiters(this);
}
}
}
return waiters;
}
@Override
public void shutdown() {
super.shutdown();
if (waiters != null) {
waiters.shutdown();
}
}
}