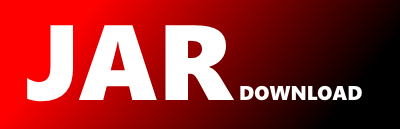
com.amazonaws.services.guardduty.AmazonGuardDutyAsync Maven / Gradle / Ivy
Show all versions of aws-java-sdk-guardduty Show documentation
/*
* Copyright 2013-2018 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.guardduty;
import javax.annotation.Generated;
import com.amazonaws.services.guardduty.model.*;
/**
* Interface for accessing Amazon GuardDuty asynchronously. Each asynchronous method will return a Java Future object
* representing the asynchronous operation; overloads which accept an {@code AsyncHandler} can be used to receive
* notification when an asynchronous operation completes.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.guardduty.AbstractAmazonGuardDutyAsync} instead.
*
*
* Assess, monitor, manage, and remediate security issues across your AWS infrastructure, applications, and data.
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AmazonGuardDutyAsync extends AmazonGuardDuty {
/**
* Accepts the invitation to be monitored by a master GuardDuty account.
*
* @param acceptInvitationRequest
* AcceptInvitation request body.
* @return A Java Future containing the result of the AcceptInvitation operation returned by the service.
* @sample AmazonGuardDutyAsync.AcceptInvitation
* @see AWS API
* Documentation
*/
java.util.concurrent.Future acceptInvitationAsync(AcceptInvitationRequest acceptInvitationRequest);
/**
* Accepts the invitation to be monitored by a master GuardDuty account.
*
* @param acceptInvitationRequest
* AcceptInvitation request body.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AcceptInvitation operation returned by the service.
* @sample AmazonGuardDutyAsyncHandler.AcceptInvitation
* @see AWS API
* Documentation
*/
java.util.concurrent.Future acceptInvitationAsync(AcceptInvitationRequest acceptInvitationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Archives Amazon GuardDuty findings specified by the list of finding IDs.
*
* @param archiveFindingsRequest
* ArchiveFindings request body.
* @return A Java Future containing the result of the ArchiveFindings operation returned by the service.
* @sample AmazonGuardDutyAsync.ArchiveFindings
* @see AWS API
* Documentation
*/
java.util.concurrent.Future archiveFindingsAsync(ArchiveFindingsRequest archiveFindingsRequest);
/**
* Archives Amazon GuardDuty findings specified by the list of finding IDs.
*
* @param archiveFindingsRequest
* ArchiveFindings request body.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ArchiveFindings operation returned by the service.
* @sample AmazonGuardDutyAsyncHandler.ArchiveFindings
* @see AWS API
* Documentation
*/
java.util.concurrent.Future archiveFindingsAsync(ArchiveFindingsRequest archiveFindingsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Creates a single Amazon GuardDuty detector. A detector is an object that represents the GuardDuty service. A
* detector must be created in order for GuardDuty to become operational.
*
* @param createDetectorRequest
* CreateDetector request body.
* @return A Java Future containing the result of the CreateDetector operation returned by the service.
* @sample AmazonGuardDutyAsync.CreateDetector
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createDetectorAsync(CreateDetectorRequest createDetectorRequest);
/**
* Creates a single Amazon GuardDuty detector. A detector is an object that represents the GuardDuty service. A
* detector must be created in order for GuardDuty to become operational.
*
* @param createDetectorRequest
* CreateDetector request body.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateDetector operation returned by the service.
* @sample AmazonGuardDutyAsyncHandler.CreateDetector
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createDetectorAsync(CreateDetectorRequest createDetectorRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Creates a filter using the specified finding criteria.
*
* @param createFilterRequest
* CreateFilterRequest request body.
* @return A Java Future containing the result of the CreateFilter operation returned by the service.
* @sample AmazonGuardDutyAsync.CreateFilter
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createFilterAsync(CreateFilterRequest createFilterRequest);
/**
* Creates a filter using the specified finding criteria.
*
* @param createFilterRequest
* CreateFilterRequest request body.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateFilter operation returned by the service.
* @sample AmazonGuardDutyAsyncHandler.CreateFilter
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createFilterAsync(CreateFilterRequest createFilterRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Creates a new IPSet - a list of trusted IP addresses that have been whitelisted for secure communication with AWS
* infrastructure and applications.
*
* @param createIPSetRequest
* CreateIPSet request body.
* @return A Java Future containing the result of the CreateIPSet operation returned by the service.
* @sample AmazonGuardDutyAsync.CreateIPSet
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createIPSetAsync(CreateIPSetRequest createIPSetRequest);
/**
* Creates a new IPSet - a list of trusted IP addresses that have been whitelisted for secure communication with AWS
* infrastructure and applications.
*
* @param createIPSetRequest
* CreateIPSet request body.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateIPSet operation returned by the service.
* @sample AmazonGuardDutyAsyncHandler.CreateIPSet
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createIPSetAsync(CreateIPSetRequest createIPSetRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Creates member accounts of the current AWS account by specifying a list of AWS account IDs. The current AWS
* account can then invite these members to manage GuardDuty in their accounts.
*
* @param createMembersRequest
* CreateMembers request body.
* @return A Java Future containing the result of the CreateMembers operation returned by the service.
* @sample AmazonGuardDutyAsync.CreateMembers
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createMembersAsync(CreateMembersRequest createMembersRequest);
/**
* Creates member accounts of the current AWS account by specifying a list of AWS account IDs. The current AWS
* account can then invite these members to manage GuardDuty in their accounts.
*
* @param createMembersRequest
* CreateMembers request body.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateMembers operation returned by the service.
* @sample AmazonGuardDutyAsyncHandler.CreateMembers
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createMembersAsync(CreateMembersRequest createMembersRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Generates example findings of types specified by the list of finding types. If 'NULL' is specified for
* findingTypes, the API generates example findings of all supported finding types.
*
* @param createSampleFindingsRequest
* CreateSampleFindings request body.
* @return A Java Future containing the result of the CreateSampleFindings operation returned by the service.
* @sample AmazonGuardDutyAsync.CreateSampleFindings
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createSampleFindingsAsync(CreateSampleFindingsRequest createSampleFindingsRequest);
/**
* Generates example findings of types specified by the list of finding types. If 'NULL' is specified for
* findingTypes, the API generates example findings of all supported finding types.
*
* @param createSampleFindingsRequest
* CreateSampleFindings request body.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateSampleFindings operation returned by the service.
* @sample AmazonGuardDutyAsyncHandler.CreateSampleFindings
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createSampleFindingsAsync(CreateSampleFindingsRequest createSampleFindingsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Create a new ThreatIntelSet. ThreatIntelSets consist of known malicious IP addresses. GuardDuty generates
* findings based on ThreatIntelSets.
*
* @param createThreatIntelSetRequest
* CreateThreatIntelSet request body.
* @return A Java Future containing the result of the CreateThreatIntelSet operation returned by the service.
* @sample AmazonGuardDutyAsync.CreateThreatIntelSet
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createThreatIntelSetAsync(CreateThreatIntelSetRequest createThreatIntelSetRequest);
/**
* Create a new ThreatIntelSet. ThreatIntelSets consist of known malicious IP addresses. GuardDuty generates
* findings based on ThreatIntelSets.
*
* @param createThreatIntelSetRequest
* CreateThreatIntelSet request body.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateThreatIntelSet operation returned by the service.
* @sample AmazonGuardDutyAsyncHandler.CreateThreatIntelSet
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createThreatIntelSetAsync(CreateThreatIntelSetRequest createThreatIntelSetRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Declines invitations sent to the current member account by AWS account specified by their account IDs.
*
* @param declineInvitationsRequest
* DeclineInvitations request body.
* @return A Java Future containing the result of the DeclineInvitations operation returned by the service.
* @sample AmazonGuardDutyAsync.DeclineInvitations
* @see AWS
* API Documentation
*/
java.util.concurrent.Future declineInvitationsAsync(DeclineInvitationsRequest declineInvitationsRequest);
/**
* Declines invitations sent to the current member account by AWS account specified by their account IDs.
*
* @param declineInvitationsRequest
* DeclineInvitations request body.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeclineInvitations operation returned by the service.
* @sample AmazonGuardDutyAsyncHandler.DeclineInvitations
* @see AWS
* API Documentation
*/
java.util.concurrent.Future declineInvitationsAsync(DeclineInvitationsRequest declineInvitationsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Deletes a Amazon GuardDuty detector specified by the detector ID.
*
* @param deleteDetectorRequest
* @return A Java Future containing the result of the DeleteDetector operation returned by the service.
* @sample AmazonGuardDutyAsync.DeleteDetector
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteDetectorAsync(DeleteDetectorRequest deleteDetectorRequest);
/**
* Deletes a Amazon GuardDuty detector specified by the detector ID.
*
* @param deleteDetectorRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteDetector operation returned by the service.
* @sample AmazonGuardDutyAsyncHandler.DeleteDetector
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteDetectorAsync(DeleteDetectorRequest deleteDetectorRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Deletes the filter specified by the filter name.
*
* @param deleteFilterRequest
* @return A Java Future containing the result of the DeleteFilter operation returned by the service.
* @sample AmazonGuardDutyAsync.DeleteFilter
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteFilterAsync(DeleteFilterRequest deleteFilterRequest);
/**
* Deletes the filter specified by the filter name.
*
* @param deleteFilterRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteFilter operation returned by the service.
* @sample AmazonGuardDutyAsyncHandler.DeleteFilter
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteFilterAsync(DeleteFilterRequest deleteFilterRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Deletes the IPSet specified by the IPSet ID.
*
* @param deleteIPSetRequest
* @return A Java Future containing the result of the DeleteIPSet operation returned by the service.
* @sample AmazonGuardDutyAsync.DeleteIPSet
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteIPSetAsync(DeleteIPSetRequest deleteIPSetRequest);
/**
* Deletes the IPSet specified by the IPSet ID.
*
* @param deleteIPSetRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteIPSet operation returned by the service.
* @sample AmazonGuardDutyAsyncHandler.DeleteIPSet
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteIPSetAsync(DeleteIPSetRequest deleteIPSetRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Deletes invitations sent to the current member account by AWS accounts specified by their account IDs.
*
* @param deleteInvitationsRequest
* DeleteInvitations request body.
* @return A Java Future containing the result of the DeleteInvitations operation returned by the service.
* @sample AmazonGuardDutyAsync.DeleteInvitations
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteInvitationsAsync(DeleteInvitationsRequest deleteInvitationsRequest);
/**
* Deletes invitations sent to the current member account by AWS accounts specified by their account IDs.
*
* @param deleteInvitationsRequest
* DeleteInvitations request body.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteInvitations operation returned by the service.
* @sample AmazonGuardDutyAsyncHandler.DeleteInvitations
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteInvitationsAsync(DeleteInvitationsRequest deleteInvitationsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Deletes GuardDuty member accounts (to the current GuardDuty master account) specified by the account IDs.
*
* @param deleteMembersRequest
* DeleteMembers request body.
* @return A Java Future containing the result of the DeleteMembers operation returned by the service.
* @sample AmazonGuardDutyAsync.DeleteMembers
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteMembersAsync(DeleteMembersRequest deleteMembersRequest);
/**
* Deletes GuardDuty member accounts (to the current GuardDuty master account) specified by the account IDs.
*
* @param deleteMembersRequest
* DeleteMembers request body.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteMembers operation returned by the service.
* @sample AmazonGuardDutyAsyncHandler.DeleteMembers
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteMembersAsync(DeleteMembersRequest deleteMembersRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Deletes ThreatIntelSet specified by the ThreatIntelSet ID.
*
* @param deleteThreatIntelSetRequest
* @return A Java Future containing the result of the DeleteThreatIntelSet operation returned by the service.
* @sample AmazonGuardDutyAsync.DeleteThreatIntelSet
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteThreatIntelSetAsync(DeleteThreatIntelSetRequest deleteThreatIntelSetRequest);
/**
* Deletes ThreatIntelSet specified by the ThreatIntelSet ID.
*
* @param deleteThreatIntelSetRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteThreatIntelSet operation returned by the service.
* @sample AmazonGuardDutyAsyncHandler.DeleteThreatIntelSet
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteThreatIntelSetAsync(DeleteThreatIntelSetRequest deleteThreatIntelSetRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Disassociates the current GuardDuty member account from its master account.
*
* @param disassociateFromMasterAccountRequest
* @return A Java Future containing the result of the DisassociateFromMasterAccount operation returned by the
* service.
* @sample AmazonGuardDutyAsync.DisassociateFromMasterAccount
* @see AWS API Documentation
*/
java.util.concurrent.Future disassociateFromMasterAccountAsync(
DisassociateFromMasterAccountRequest disassociateFromMasterAccountRequest);
/**
* Disassociates the current GuardDuty member account from its master account.
*
* @param disassociateFromMasterAccountRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DisassociateFromMasterAccount operation returned by the
* service.
* @sample AmazonGuardDutyAsyncHandler.DisassociateFromMasterAccount
* @see AWS API Documentation
*/
java.util.concurrent.Future disassociateFromMasterAccountAsync(
DisassociateFromMasterAccountRequest disassociateFromMasterAccountRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Disassociates GuardDuty member accounts (to the current GuardDuty master account) specified by the account IDs.
*
* @param disassociateMembersRequest
* DisassociateMembers request body.
* @return A Java Future containing the result of the DisassociateMembers operation returned by the service.
* @sample AmazonGuardDutyAsync.DisassociateMembers
* @see AWS
* API Documentation
*/
java.util.concurrent.Future disassociateMembersAsync(DisassociateMembersRequest disassociateMembersRequest);
/**
* Disassociates GuardDuty member accounts (to the current GuardDuty master account) specified by the account IDs.
*
* @param disassociateMembersRequest
* DisassociateMembers request body.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DisassociateMembers operation returned by the service.
* @sample AmazonGuardDutyAsyncHandler.DisassociateMembers
* @see AWS
* API Documentation
*/
java.util.concurrent.Future disassociateMembersAsync(DisassociateMembersRequest disassociateMembersRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Retrieves an Amazon GuardDuty detector specified by the detectorId.
*
* @param getDetectorRequest
* @return A Java Future containing the result of the GetDetector operation returned by the service.
* @sample AmazonGuardDutyAsync.GetDetector
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getDetectorAsync(GetDetectorRequest getDetectorRequest);
/**
* Retrieves an Amazon GuardDuty detector specified by the detectorId.
*
* @param getDetectorRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetDetector operation returned by the service.
* @sample AmazonGuardDutyAsyncHandler.GetDetector
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getDetectorAsync(GetDetectorRequest getDetectorRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Returns the details of the filter specified by the filter name.
*
* @param getFilterRequest
* @return A Java Future containing the result of the GetFilter operation returned by the service.
* @sample AmazonGuardDutyAsync.GetFilter
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getFilterAsync(GetFilterRequest getFilterRequest);
/**
* Returns the details of the filter specified by the filter name.
*
* @param getFilterRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetFilter operation returned by the service.
* @sample AmazonGuardDutyAsyncHandler.GetFilter
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getFilterAsync(GetFilterRequest getFilterRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Describes Amazon GuardDuty findings specified by finding IDs.
*
* @param getFindingsRequest
* GetFindings request body.
* @return A Java Future containing the result of the GetFindings operation returned by the service.
* @sample AmazonGuardDutyAsync.GetFindings
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getFindingsAsync(GetFindingsRequest getFindingsRequest);
/**
* Describes Amazon GuardDuty findings specified by finding IDs.
*
* @param getFindingsRequest
* GetFindings request body.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetFindings operation returned by the service.
* @sample AmazonGuardDutyAsyncHandler.GetFindings
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getFindingsAsync(GetFindingsRequest getFindingsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Lists Amazon GuardDuty findings' statistics for the specified detector ID.
*
* @param getFindingsStatisticsRequest
* GetFindingsStatistics request body.
* @return A Java Future containing the result of the GetFindingsStatistics operation returned by the service.
* @sample AmazonGuardDutyAsync.GetFindingsStatistics
* @see AWS API Documentation
*/
java.util.concurrent.Future getFindingsStatisticsAsync(GetFindingsStatisticsRequest getFindingsStatisticsRequest);
/**
* Lists Amazon GuardDuty findings' statistics for the specified detector ID.
*
* @param getFindingsStatisticsRequest
* GetFindingsStatistics request body.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetFindingsStatistics operation returned by the service.
* @sample AmazonGuardDutyAsyncHandler.GetFindingsStatistics
* @see AWS API Documentation
*/
java.util.concurrent.Future getFindingsStatisticsAsync(GetFindingsStatisticsRequest getFindingsStatisticsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Retrieves the IPSet specified by the IPSet ID.
*
* @param getIPSetRequest
* @return A Java Future containing the result of the GetIPSet operation returned by the service.
* @sample AmazonGuardDutyAsync.GetIPSet
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getIPSetAsync(GetIPSetRequest getIPSetRequest);
/**
* Retrieves the IPSet specified by the IPSet ID.
*
* @param getIPSetRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetIPSet operation returned by the service.
* @sample AmazonGuardDutyAsyncHandler.GetIPSet
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getIPSetAsync(GetIPSetRequest getIPSetRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Returns the count of all GuardDuty membership invitations that were sent to the current member account except the
* currently accepted invitation.
*
* @param getInvitationsCountRequest
* @return A Java Future containing the result of the GetInvitationsCount operation returned by the service.
* @sample AmazonGuardDutyAsync.GetInvitationsCount
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getInvitationsCountAsync(GetInvitationsCountRequest getInvitationsCountRequest);
/**
* Returns the count of all GuardDuty membership invitations that were sent to the current member account except the
* currently accepted invitation.
*
* @param getInvitationsCountRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetInvitationsCount operation returned by the service.
* @sample AmazonGuardDutyAsyncHandler.GetInvitationsCount
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getInvitationsCountAsync(GetInvitationsCountRequest getInvitationsCountRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Provides the details for the GuardDuty master account to the current GuardDuty member account.
*
* @param getMasterAccountRequest
* @return A Java Future containing the result of the GetMasterAccount operation returned by the service.
* @sample AmazonGuardDutyAsync.GetMasterAccount
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getMasterAccountAsync(GetMasterAccountRequest getMasterAccountRequest);
/**
* Provides the details for the GuardDuty master account to the current GuardDuty member account.
*
* @param getMasterAccountRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetMasterAccount operation returned by the service.
* @sample AmazonGuardDutyAsyncHandler.GetMasterAccount
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getMasterAccountAsync(GetMasterAccountRequest getMasterAccountRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Retrieves GuardDuty member accounts (to the current GuardDuty master account) specified by the account IDs.
*
* @param getMembersRequest
* GetMembers request body.
* @return A Java Future containing the result of the GetMembers operation returned by the service.
* @sample AmazonGuardDutyAsync.GetMembers
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getMembersAsync(GetMembersRequest getMembersRequest);
/**
* Retrieves GuardDuty member accounts (to the current GuardDuty master account) specified by the account IDs.
*
* @param getMembersRequest
* GetMembers request body.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetMembers operation returned by the service.
* @sample AmazonGuardDutyAsyncHandler.GetMembers
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getMembersAsync(GetMembersRequest getMembersRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Retrieves the ThreatIntelSet that is specified by the ThreatIntelSet ID.
*
* @param getThreatIntelSetRequest
* @return A Java Future containing the result of the GetThreatIntelSet operation returned by the service.
* @sample AmazonGuardDutyAsync.GetThreatIntelSet
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getThreatIntelSetAsync(GetThreatIntelSetRequest getThreatIntelSetRequest);
/**
* Retrieves the ThreatIntelSet that is specified by the ThreatIntelSet ID.
*
* @param getThreatIntelSetRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetThreatIntelSet operation returned by the service.
* @sample AmazonGuardDutyAsyncHandler.GetThreatIntelSet
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getThreatIntelSetAsync(GetThreatIntelSetRequest getThreatIntelSetRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Invites other AWS accounts (created as members of the current AWS account by CreateMembers) to enable GuardDuty
* and allow the current AWS account to view and manage these accounts' GuardDuty findings on their behalf as the
* master account.
*
* @param inviteMembersRequest
* InviteMembers request body.
* @return A Java Future containing the result of the InviteMembers operation returned by the service.
* @sample AmazonGuardDutyAsync.InviteMembers
* @see AWS API
* Documentation
*/
java.util.concurrent.Future inviteMembersAsync(InviteMembersRequest inviteMembersRequest);
/**
* Invites other AWS accounts (created as members of the current AWS account by CreateMembers) to enable GuardDuty
* and allow the current AWS account to view and manage these accounts' GuardDuty findings on their behalf as the
* master account.
*
* @param inviteMembersRequest
* InviteMembers request body.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the InviteMembers operation returned by the service.
* @sample AmazonGuardDutyAsyncHandler.InviteMembers
* @see AWS API
* Documentation
*/
java.util.concurrent.Future inviteMembersAsync(InviteMembersRequest inviteMembersRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Lists detectorIds of all the existing Amazon GuardDuty detector resources.
*
* @param listDetectorsRequest
* @return A Java Future containing the result of the ListDetectors operation returned by the service.
* @sample AmazonGuardDutyAsync.ListDetectors
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listDetectorsAsync(ListDetectorsRequest listDetectorsRequest);
/**
* Lists detectorIds of all the existing Amazon GuardDuty detector resources.
*
* @param listDetectorsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListDetectors operation returned by the service.
* @sample AmazonGuardDutyAsyncHandler.ListDetectors
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listDetectorsAsync(ListDetectorsRequest listDetectorsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Returns a paginated list of the current filters.
*
* @param listFiltersRequest
* @return A Java Future containing the result of the ListFilters operation returned by the service.
* @sample AmazonGuardDutyAsync.ListFilters
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listFiltersAsync(ListFiltersRequest listFiltersRequest);
/**
* Returns a paginated list of the current filters.
*
* @param listFiltersRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListFilters operation returned by the service.
* @sample AmazonGuardDutyAsyncHandler.ListFilters
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listFiltersAsync(ListFiltersRequest listFiltersRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Lists Amazon GuardDuty findings for the specified detector ID.
*
* @param listFindingsRequest
* ListFindings request body.
* @return A Java Future containing the result of the ListFindings operation returned by the service.
* @sample AmazonGuardDutyAsync.ListFindings
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listFindingsAsync(ListFindingsRequest listFindingsRequest);
/**
* Lists Amazon GuardDuty findings for the specified detector ID.
*
* @param listFindingsRequest
* ListFindings request body.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListFindings operation returned by the service.
* @sample AmazonGuardDutyAsyncHandler.ListFindings
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listFindingsAsync(ListFindingsRequest listFindingsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Lists the IPSets of the GuardDuty service specified by the detector ID.
*
* @param listIPSetsRequest
* @return A Java Future containing the result of the ListIPSets operation returned by the service.
* @sample AmazonGuardDutyAsync.ListIPSets
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listIPSetsAsync(ListIPSetsRequest listIPSetsRequest);
/**
* Lists the IPSets of the GuardDuty service specified by the detector ID.
*
* @param listIPSetsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListIPSets operation returned by the service.
* @sample AmazonGuardDutyAsyncHandler.ListIPSets
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listIPSetsAsync(ListIPSetsRequest listIPSetsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Lists all GuardDuty membership invitations that were sent to the current AWS account.
*
* @param listInvitationsRequest
* @return A Java Future containing the result of the ListInvitations operation returned by the service.
* @sample AmazonGuardDutyAsync.ListInvitations
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listInvitationsAsync(ListInvitationsRequest listInvitationsRequest);
/**
* Lists all GuardDuty membership invitations that were sent to the current AWS account.
*
* @param listInvitationsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListInvitations operation returned by the service.
* @sample AmazonGuardDutyAsyncHandler.ListInvitations
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listInvitationsAsync(ListInvitationsRequest listInvitationsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Lists details about all member accounts for the current GuardDuty master account.
*
* @param listMembersRequest
* @return A Java Future containing the result of the ListMembers operation returned by the service.
* @sample AmazonGuardDutyAsync.ListMembers
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listMembersAsync(ListMembersRequest listMembersRequest);
/**
* Lists details about all member accounts for the current GuardDuty master account.
*
* @param listMembersRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListMembers operation returned by the service.
* @sample AmazonGuardDutyAsyncHandler.ListMembers
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listMembersAsync(ListMembersRequest listMembersRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Lists the ThreatIntelSets of the GuardDuty service specified by the detector ID.
*
* @param listThreatIntelSetsRequest
* @return A Java Future containing the result of the ListThreatIntelSets operation returned by the service.
* @sample AmazonGuardDutyAsync.ListThreatIntelSets
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listThreatIntelSetsAsync(ListThreatIntelSetsRequest listThreatIntelSetsRequest);
/**
* Lists the ThreatIntelSets of the GuardDuty service specified by the detector ID.
*
* @param listThreatIntelSetsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListThreatIntelSets operation returned by the service.
* @sample AmazonGuardDutyAsyncHandler.ListThreatIntelSets
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listThreatIntelSetsAsync(ListThreatIntelSetsRequest listThreatIntelSetsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Re-enables GuardDuty to monitor findings of the member accounts specified by the account IDs. A master GuardDuty
* account can run this command after disabling GuardDuty from monitoring these members' findings by running
* StopMonitoringMembers.
*
* @param startMonitoringMembersRequest
* StartMonitoringMembers request body.
* @return A Java Future containing the result of the StartMonitoringMembers operation returned by the service.
* @sample AmazonGuardDutyAsync.StartMonitoringMembers
* @see AWS API Documentation
*/
java.util.concurrent.Future startMonitoringMembersAsync(StartMonitoringMembersRequest startMonitoringMembersRequest);
/**
* Re-enables GuardDuty to monitor findings of the member accounts specified by the account IDs. A master GuardDuty
* account can run this command after disabling GuardDuty from monitoring these members' findings by running
* StopMonitoringMembers.
*
* @param startMonitoringMembersRequest
* StartMonitoringMembers request body.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the StartMonitoringMembers operation returned by the service.
* @sample AmazonGuardDutyAsyncHandler.StartMonitoringMembers
* @see AWS API Documentation
*/
java.util.concurrent.Future startMonitoringMembersAsync(StartMonitoringMembersRequest startMonitoringMembersRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Disables GuardDuty from monitoring findings of the member accounts specified by the account IDs. After running
* this command, a master GuardDuty account can run StartMonitoringMembers to re-enable GuardDuty to monitor these
* members’ findings.
*
* @param stopMonitoringMembersRequest
* StopMonitoringMembers request body.
* @return A Java Future containing the result of the StopMonitoringMembers operation returned by the service.
* @sample AmazonGuardDutyAsync.StopMonitoringMembers
* @see AWS API Documentation
*/
java.util.concurrent.Future stopMonitoringMembersAsync(StopMonitoringMembersRequest stopMonitoringMembersRequest);
/**
* Disables GuardDuty from monitoring findings of the member accounts specified by the account IDs. After running
* this command, a master GuardDuty account can run StartMonitoringMembers to re-enable GuardDuty to monitor these
* members’ findings.
*
* @param stopMonitoringMembersRequest
* StopMonitoringMembers request body.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the StopMonitoringMembers operation returned by the service.
* @sample AmazonGuardDutyAsyncHandler.StopMonitoringMembers
* @see AWS API Documentation
*/
java.util.concurrent.Future stopMonitoringMembersAsync(StopMonitoringMembersRequest stopMonitoringMembersRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Unarchives Amazon GuardDuty findings specified by the list of finding IDs.
*
* @param unarchiveFindingsRequest
* UnarchiveFindings request body.
* @return A Java Future containing the result of the UnarchiveFindings operation returned by the service.
* @sample AmazonGuardDutyAsync.UnarchiveFindings
* @see AWS
* API Documentation
*/
java.util.concurrent.Future unarchiveFindingsAsync(UnarchiveFindingsRequest unarchiveFindingsRequest);
/**
* Unarchives Amazon GuardDuty findings specified by the list of finding IDs.
*
* @param unarchiveFindingsRequest
* UnarchiveFindings request body.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UnarchiveFindings operation returned by the service.
* @sample AmazonGuardDutyAsyncHandler.UnarchiveFindings
* @see AWS
* API Documentation
*/
java.util.concurrent.Future unarchiveFindingsAsync(UnarchiveFindingsRequest unarchiveFindingsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Updates an Amazon GuardDuty detector specified by the detectorId.
*
* @param updateDetectorRequest
* UpdateDetector request body.
* @return A Java Future containing the result of the UpdateDetector operation returned by the service.
* @sample AmazonGuardDutyAsync.UpdateDetector
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateDetectorAsync(UpdateDetectorRequest updateDetectorRequest);
/**
* Updates an Amazon GuardDuty detector specified by the detectorId.
*
* @param updateDetectorRequest
* UpdateDetector request body.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateDetector operation returned by the service.
* @sample AmazonGuardDutyAsyncHandler.UpdateDetector
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateDetectorAsync(UpdateDetectorRequest updateDetectorRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Updates the filter specified by the filter name.
*
* @param updateFilterRequest
* UpdateFilterRequest request body.
* @return A Java Future containing the result of the UpdateFilter operation returned by the service.
* @sample AmazonGuardDutyAsync.UpdateFilter
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateFilterAsync(UpdateFilterRequest updateFilterRequest);
/**
* Updates the filter specified by the filter name.
*
* @param updateFilterRequest
* UpdateFilterRequest request body.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateFilter operation returned by the service.
* @sample AmazonGuardDutyAsyncHandler.UpdateFilter
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateFilterAsync(UpdateFilterRequest updateFilterRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Marks specified Amazon GuardDuty findings as useful or not useful.
*
* @param updateFindingsFeedbackRequest
* UpdateFindingsFeedback request body.
* @return A Java Future containing the result of the UpdateFindingsFeedback operation returned by the service.
* @sample AmazonGuardDutyAsync.UpdateFindingsFeedback
* @see AWS API Documentation
*/
java.util.concurrent.Future updateFindingsFeedbackAsync(UpdateFindingsFeedbackRequest updateFindingsFeedbackRequest);
/**
* Marks specified Amazon GuardDuty findings as useful or not useful.
*
* @param updateFindingsFeedbackRequest
* UpdateFindingsFeedback request body.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateFindingsFeedback operation returned by the service.
* @sample AmazonGuardDutyAsyncHandler.UpdateFindingsFeedback
* @see AWS API Documentation
*/
java.util.concurrent.Future updateFindingsFeedbackAsync(UpdateFindingsFeedbackRequest updateFindingsFeedbackRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Updates the IPSet specified by the IPSet ID.
*
* @param updateIPSetRequest
* UpdateIPSet request body.
* @return A Java Future containing the result of the UpdateIPSet operation returned by the service.
* @sample AmazonGuardDutyAsync.UpdateIPSet
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateIPSetAsync(UpdateIPSetRequest updateIPSetRequest);
/**
* Updates the IPSet specified by the IPSet ID.
*
* @param updateIPSetRequest
* UpdateIPSet request body.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateIPSet operation returned by the service.
* @sample AmazonGuardDutyAsyncHandler.UpdateIPSet
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateIPSetAsync(UpdateIPSetRequest updateIPSetRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Updates the ThreatIntelSet specified by ThreatIntelSet ID.
*
* @param updateThreatIntelSetRequest
* UpdateThreatIntelSet request body.
* @return A Java Future containing the result of the UpdateThreatIntelSet operation returned by the service.
* @sample AmazonGuardDutyAsync.UpdateThreatIntelSet
* @see AWS
* API Documentation
*/
java.util.concurrent.Future updateThreatIntelSetAsync(UpdateThreatIntelSetRequest updateThreatIntelSetRequest);
/**
* Updates the ThreatIntelSet specified by ThreatIntelSet ID.
*
* @param updateThreatIntelSetRequest
* UpdateThreatIntelSet request body.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateThreatIntelSet operation returned by the service.
* @sample AmazonGuardDutyAsyncHandler.UpdateThreatIntelSet
* @see AWS
* API Documentation
*/
java.util.concurrent.Future updateThreatIntelSetAsync(UpdateThreatIntelSetRequest updateThreatIntelSetRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
}