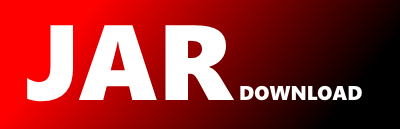
com.amazonaws.services.health.model.Event Maven / Gradle / Ivy
Show all versions of aws-java-sdk-health Show documentation
/*
* Copyright 2012-2017 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.health.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* Summary information about an event, returned by the DescribeEvents operation. The DescribeEventDetails
* operation also returns this information, as well as the EventDescription and additional event metadata.
*
*
* @see AWS API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class Event implements Serializable, Cloneable, StructuredPojo {
/**
*
* The unique identifier for the event. Format:
* arn:aws:health:event-region::event/EVENT_TYPE_PLUS_ID
. Example:
* arn:aws:health:us-east-1::event/AWS_EC2_MAINTENANCE_5331
*
*/
private String arn;
/**
*
* The AWS service that is affected by the event. For example, EC2
, RDS
.
*
*/
private String service;
/**
*
* The unique identifier for the event type. The format is AWS_SERVICE_DESCRIPTION
; for
* example, AWS_EC2_SYSTEM_MAINTENANCE_EVENT
.
*
*/
private String eventTypeCode;
/**
*
* The
*
*/
private String eventTypeCategory;
/**
*
* The AWS region name of the event.
*
*/
private String region;
/**
*
* The AWS Availability Zone of the event. For example, us-east-1a.
*
*/
private String availabilityZone;
/**
*
* The date and time that the event began.
*
*/
private java.util.Date startTime;
/**
*
* The date and time that the event ended.
*
*/
private java.util.Date endTime;
/**
*
* The most recent date and time that the event was updated.
*
*/
private java.util.Date lastUpdatedTime;
/**
*
* The most recent status of the event. Possible values are open
, closed
, and
* upcoming
.
*
*/
private String statusCode;
/**
*
* The unique identifier for the event. Format:
* arn:aws:health:event-region::event/EVENT_TYPE_PLUS_ID
. Example:
* arn:aws:health:us-east-1::event/AWS_EC2_MAINTENANCE_5331
*
*
* @param arn
* The unique identifier for the event. Format:
* arn:aws:health:event-region::event/EVENT_TYPE_PLUS_ID
. Example:
* arn:aws:health:us-east-1::event/AWS_EC2_MAINTENANCE_5331
*/
public void setArn(String arn) {
this.arn = arn;
}
/**
*
* The unique identifier for the event. Format:
* arn:aws:health:event-region::event/EVENT_TYPE_PLUS_ID
. Example:
* arn:aws:health:us-east-1::event/AWS_EC2_MAINTENANCE_5331
*
*
* @return The unique identifier for the event. Format:
* arn:aws:health:event-region::event/EVENT_TYPE_PLUS_ID
. Example:
* arn:aws:health:us-east-1::event/AWS_EC2_MAINTENANCE_5331
*/
public String getArn() {
return this.arn;
}
/**
*
* The unique identifier for the event. Format:
* arn:aws:health:event-region::event/EVENT_TYPE_PLUS_ID
. Example:
* arn:aws:health:us-east-1::event/AWS_EC2_MAINTENANCE_5331
*
*
* @param arn
* The unique identifier for the event. Format:
* arn:aws:health:event-region::event/EVENT_TYPE_PLUS_ID
. Example:
* arn:aws:health:us-east-1::event/AWS_EC2_MAINTENANCE_5331
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Event withArn(String arn) {
setArn(arn);
return this;
}
/**
*
* The AWS service that is affected by the event. For example, EC2
, RDS
.
*
*
* @param service
* The AWS service that is affected by the event. For example, EC2
, RDS
.
*/
public void setService(String service) {
this.service = service;
}
/**
*
* The AWS service that is affected by the event. For example, EC2
, RDS
.
*
*
* @return The AWS service that is affected by the event. For example, EC2
, RDS
.
*/
public String getService() {
return this.service;
}
/**
*
* The AWS service that is affected by the event. For example, EC2
, RDS
.
*
*
* @param service
* The AWS service that is affected by the event. For example, EC2
, RDS
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Event withService(String service) {
setService(service);
return this;
}
/**
*
* The unique identifier for the event type. The format is AWS_SERVICE_DESCRIPTION
; for
* example, AWS_EC2_SYSTEM_MAINTENANCE_EVENT
.
*
*
* @param eventTypeCode
* The unique identifier for the event type. The format is
* AWS_SERVICE_DESCRIPTION
; for example,
* AWS_EC2_SYSTEM_MAINTENANCE_EVENT
.
*/
public void setEventTypeCode(String eventTypeCode) {
this.eventTypeCode = eventTypeCode;
}
/**
*
* The unique identifier for the event type. The format is AWS_SERVICE_DESCRIPTION
; for
* example, AWS_EC2_SYSTEM_MAINTENANCE_EVENT
.
*
*
* @return The unique identifier for the event type. The format is
* AWS_SERVICE_DESCRIPTION
; for example,
* AWS_EC2_SYSTEM_MAINTENANCE_EVENT
.
*/
public String getEventTypeCode() {
return this.eventTypeCode;
}
/**
*
* The unique identifier for the event type. The format is AWS_SERVICE_DESCRIPTION
; for
* example, AWS_EC2_SYSTEM_MAINTENANCE_EVENT
.
*
*
* @param eventTypeCode
* The unique identifier for the event type. The format is
* AWS_SERVICE_DESCRIPTION
; for example,
* AWS_EC2_SYSTEM_MAINTENANCE_EVENT
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Event withEventTypeCode(String eventTypeCode) {
setEventTypeCode(eventTypeCode);
return this;
}
/**
*
* The
*
*
* @param eventTypeCategory
* The
* @see EventTypeCategory
*/
public void setEventTypeCategory(String eventTypeCategory) {
this.eventTypeCategory = eventTypeCategory;
}
/**
*
* The
*
*
* @return The
* @see EventTypeCategory
*/
public String getEventTypeCategory() {
return this.eventTypeCategory;
}
/**
*
* The
*
*
* @param eventTypeCategory
* The
* @return Returns a reference to this object so that method calls can be chained together.
* @see EventTypeCategory
*/
public Event withEventTypeCategory(String eventTypeCategory) {
setEventTypeCategory(eventTypeCategory);
return this;
}
/**
*
* The
*
*
* @param eventTypeCategory
* The
* @see EventTypeCategory
*/
public void setEventTypeCategory(EventTypeCategory eventTypeCategory) {
this.eventTypeCategory = eventTypeCategory.toString();
}
/**
*
* The
*
*
* @param eventTypeCategory
* The
* @return Returns a reference to this object so that method calls can be chained together.
* @see EventTypeCategory
*/
public Event withEventTypeCategory(EventTypeCategory eventTypeCategory) {
setEventTypeCategory(eventTypeCategory);
return this;
}
/**
*
* The AWS region name of the event.
*
*
* @param region
* The AWS region name of the event.
*/
public void setRegion(String region) {
this.region = region;
}
/**
*
* The AWS region name of the event.
*
*
* @return The AWS region name of the event.
*/
public String getRegion() {
return this.region;
}
/**
*
* The AWS region name of the event.
*
*
* @param region
* The AWS region name of the event.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Event withRegion(String region) {
setRegion(region);
return this;
}
/**
*
* The AWS Availability Zone of the event. For example, us-east-1a.
*
*
* @param availabilityZone
* The AWS Availability Zone of the event. For example, us-east-1a.
*/
public void setAvailabilityZone(String availabilityZone) {
this.availabilityZone = availabilityZone;
}
/**
*
* The AWS Availability Zone of the event. For example, us-east-1a.
*
*
* @return The AWS Availability Zone of the event. For example, us-east-1a.
*/
public String getAvailabilityZone() {
return this.availabilityZone;
}
/**
*
* The AWS Availability Zone of the event. For example, us-east-1a.
*
*
* @param availabilityZone
* The AWS Availability Zone of the event. For example, us-east-1a.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Event withAvailabilityZone(String availabilityZone) {
setAvailabilityZone(availabilityZone);
return this;
}
/**
*
* The date and time that the event began.
*
*
* @param startTime
* The date and time that the event began.
*/
public void setStartTime(java.util.Date startTime) {
this.startTime = startTime;
}
/**
*
* The date and time that the event began.
*
*
* @return The date and time that the event began.
*/
public java.util.Date getStartTime() {
return this.startTime;
}
/**
*
* The date and time that the event began.
*
*
* @param startTime
* The date and time that the event began.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Event withStartTime(java.util.Date startTime) {
setStartTime(startTime);
return this;
}
/**
*
* The date and time that the event ended.
*
*
* @param endTime
* The date and time that the event ended.
*/
public void setEndTime(java.util.Date endTime) {
this.endTime = endTime;
}
/**
*
* The date and time that the event ended.
*
*
* @return The date and time that the event ended.
*/
public java.util.Date getEndTime() {
return this.endTime;
}
/**
*
* The date and time that the event ended.
*
*
* @param endTime
* The date and time that the event ended.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Event withEndTime(java.util.Date endTime) {
setEndTime(endTime);
return this;
}
/**
*
* The most recent date and time that the event was updated.
*
*
* @param lastUpdatedTime
* The most recent date and time that the event was updated.
*/
public void setLastUpdatedTime(java.util.Date lastUpdatedTime) {
this.lastUpdatedTime = lastUpdatedTime;
}
/**
*
* The most recent date and time that the event was updated.
*
*
* @return The most recent date and time that the event was updated.
*/
public java.util.Date getLastUpdatedTime() {
return this.lastUpdatedTime;
}
/**
*
* The most recent date and time that the event was updated.
*
*
* @param lastUpdatedTime
* The most recent date and time that the event was updated.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Event withLastUpdatedTime(java.util.Date lastUpdatedTime) {
setLastUpdatedTime(lastUpdatedTime);
return this;
}
/**
*
* The most recent status of the event. Possible values are open
, closed
, and
* upcoming
.
*
*
* @param statusCode
* The most recent status of the event. Possible values are open
, closed
, and
* upcoming
.
* @see EventStatusCode
*/
public void setStatusCode(String statusCode) {
this.statusCode = statusCode;
}
/**
*
* The most recent status of the event. Possible values are open
, closed
, and
* upcoming
.
*
*
* @return The most recent status of the event. Possible values are open
, closed
, and
* upcoming
.
* @see EventStatusCode
*/
public String getStatusCode() {
return this.statusCode;
}
/**
*
* The most recent status of the event. Possible values are open
, closed
, and
* upcoming
.
*
*
* @param statusCode
* The most recent status of the event. Possible values are open
, closed
, and
* upcoming
.
* @return Returns a reference to this object so that method calls can be chained together.
* @see EventStatusCode
*/
public Event withStatusCode(String statusCode) {
setStatusCode(statusCode);
return this;
}
/**
*
* The most recent status of the event. Possible values are open
, closed
, and
* upcoming
.
*
*
* @param statusCode
* The most recent status of the event. Possible values are open
, closed
, and
* upcoming
.
* @see EventStatusCode
*/
public void setStatusCode(EventStatusCode statusCode) {
this.statusCode = statusCode.toString();
}
/**
*
* The most recent status of the event. Possible values are open
, closed
, and
* upcoming
.
*
*
* @param statusCode
* The most recent status of the event. Possible values are open
, closed
, and
* upcoming
.
* @return Returns a reference to this object so that method calls can be chained together.
* @see EventStatusCode
*/
public Event withStatusCode(EventStatusCode statusCode) {
setStatusCode(statusCode);
return this;
}
/**
* Returns a string representation of this object; useful for testing and debugging.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getArn() != null)
sb.append("Arn: ").append(getArn()).append(",");
if (getService() != null)
sb.append("Service: ").append(getService()).append(",");
if (getEventTypeCode() != null)
sb.append("EventTypeCode: ").append(getEventTypeCode()).append(",");
if (getEventTypeCategory() != null)
sb.append("EventTypeCategory: ").append(getEventTypeCategory()).append(",");
if (getRegion() != null)
sb.append("Region: ").append(getRegion()).append(",");
if (getAvailabilityZone() != null)
sb.append("AvailabilityZone: ").append(getAvailabilityZone()).append(",");
if (getStartTime() != null)
sb.append("StartTime: ").append(getStartTime()).append(",");
if (getEndTime() != null)
sb.append("EndTime: ").append(getEndTime()).append(",");
if (getLastUpdatedTime() != null)
sb.append("LastUpdatedTime: ").append(getLastUpdatedTime()).append(",");
if (getStatusCode() != null)
sb.append("StatusCode: ").append(getStatusCode());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof Event == false)
return false;
Event other = (Event) obj;
if (other.getArn() == null ^ this.getArn() == null)
return false;
if (other.getArn() != null && other.getArn().equals(this.getArn()) == false)
return false;
if (other.getService() == null ^ this.getService() == null)
return false;
if (other.getService() != null && other.getService().equals(this.getService()) == false)
return false;
if (other.getEventTypeCode() == null ^ this.getEventTypeCode() == null)
return false;
if (other.getEventTypeCode() != null && other.getEventTypeCode().equals(this.getEventTypeCode()) == false)
return false;
if (other.getEventTypeCategory() == null ^ this.getEventTypeCategory() == null)
return false;
if (other.getEventTypeCategory() != null && other.getEventTypeCategory().equals(this.getEventTypeCategory()) == false)
return false;
if (other.getRegion() == null ^ this.getRegion() == null)
return false;
if (other.getRegion() != null && other.getRegion().equals(this.getRegion()) == false)
return false;
if (other.getAvailabilityZone() == null ^ this.getAvailabilityZone() == null)
return false;
if (other.getAvailabilityZone() != null && other.getAvailabilityZone().equals(this.getAvailabilityZone()) == false)
return false;
if (other.getStartTime() == null ^ this.getStartTime() == null)
return false;
if (other.getStartTime() != null && other.getStartTime().equals(this.getStartTime()) == false)
return false;
if (other.getEndTime() == null ^ this.getEndTime() == null)
return false;
if (other.getEndTime() != null && other.getEndTime().equals(this.getEndTime()) == false)
return false;
if (other.getLastUpdatedTime() == null ^ this.getLastUpdatedTime() == null)
return false;
if (other.getLastUpdatedTime() != null && other.getLastUpdatedTime().equals(this.getLastUpdatedTime()) == false)
return false;
if (other.getStatusCode() == null ^ this.getStatusCode() == null)
return false;
if (other.getStatusCode() != null && other.getStatusCode().equals(this.getStatusCode()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getArn() == null) ? 0 : getArn().hashCode());
hashCode = prime * hashCode + ((getService() == null) ? 0 : getService().hashCode());
hashCode = prime * hashCode + ((getEventTypeCode() == null) ? 0 : getEventTypeCode().hashCode());
hashCode = prime * hashCode + ((getEventTypeCategory() == null) ? 0 : getEventTypeCategory().hashCode());
hashCode = prime * hashCode + ((getRegion() == null) ? 0 : getRegion().hashCode());
hashCode = prime * hashCode + ((getAvailabilityZone() == null) ? 0 : getAvailabilityZone().hashCode());
hashCode = prime * hashCode + ((getStartTime() == null) ? 0 : getStartTime().hashCode());
hashCode = prime * hashCode + ((getEndTime() == null) ? 0 : getEndTime().hashCode());
hashCode = prime * hashCode + ((getLastUpdatedTime() == null) ? 0 : getLastUpdatedTime().hashCode());
hashCode = prime * hashCode + ((getStatusCode() == null) ? 0 : getStatusCode().hashCode());
return hashCode;
}
@Override
public Event clone() {
try {
return (Event) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.health.model.transform.EventMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}