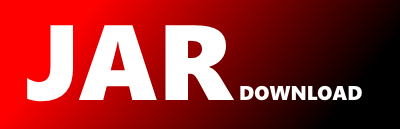
com.amazonaws.services.health.AWSHealthAsync Maven / Gradle / Ivy
Show all versions of aws-java-sdk-health Show documentation
/*
* Copyright 2012-2017 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.health;
import javax.annotation.Generated;
import com.amazonaws.services.health.model.*;
/**
* Interface for accessing AWSHealth asynchronously. Each asynchronous method will return a Java Future object
* representing the asynchronous operation; overloads which accept an {@code AsyncHandler} can be used to receive
* notification when an asynchronous operation completes.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.health.AbstractAWSHealthAsync} instead.
*
*
* AWS Health
*
* The AWS Health API provides programmatic access to the AWS Health information that is presented in the AWS Personal Health Dashboard. You can get information about events
* that affect your AWS resources:
*
*
* -
*
* DescribeEvents: Summary information about events.
*
*
* -
*
* DescribeEventDetails: Detailed information about one or more events.
*
*
* -
*
* DescribeAffectedEntities: Information about AWS resources that are affected by one or more events.
*
*
*
*
* In addition, these operations provide information about event types and summary counts of events or affected
* entities:
*
*
* -
*
* DescribeEventTypes: Information about the kinds of events that AWS Health tracks.
*
*
* -
*
* DescribeEventAggregates: A count of the number of events that meet specified criteria.
*
*
* -
*
* DescribeEntityAggregates: A count of the number of affected entities that meet specified criteria.
*
*
*
*
* The Health API requires a Business or Enterprise support plan from AWS Support. Calling the Health API from an account that does not
* have a Business or Enterprise support plan causes a SubscriptionRequiredException
.
*
*
* For authentication of requests, AWS Health uses the Signature Version 4 Signing Process.
*
*
* See the AWS Health User Guide for
* information about how to use the API.
*
*
* Service Endpoint
*
*
* The HTTP endpoint for the AWS Health API is:
*
*
* -
*
* https://health.us-east-1.amazonaws.com
*
*
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AWSHealthAsync extends AWSHealth {
/**
*
* Returns a list of entities that have been affected by the specified events, based on the specified filter
* criteria. Entities can refer to individual customer resources, groups of customer resources, or any other
* construct, depending on the AWS service. Events that have impact beyond that of the affected entities, or where
* the extent of impact is unknown, include at least one entity indicating this.
*
*
* At least one event ARN is required. Results are sorted by the lastUpdatedTime
of the entity,
* starting with the most recent.
*
*
* @param describeAffectedEntitiesRequest
* @return A Java Future containing the result of the DescribeAffectedEntities operation returned by the service.
* @sample AWSHealthAsync.DescribeAffectedEntities
* @see AWS API Documentation
*/
java.util.concurrent.Future describeAffectedEntitiesAsync(DescribeAffectedEntitiesRequest describeAffectedEntitiesRequest);
/**
*
* Returns a list of entities that have been affected by the specified events, based on the specified filter
* criteria. Entities can refer to individual customer resources, groups of customer resources, or any other
* construct, depending on the AWS service. Events that have impact beyond that of the affected entities, or where
* the extent of impact is unknown, include at least one entity indicating this.
*
*
* At least one event ARN is required. Results are sorted by the lastUpdatedTime
of the entity,
* starting with the most recent.
*
*
* @param describeAffectedEntitiesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeAffectedEntities operation returned by the service.
* @sample AWSHealthAsyncHandler.DescribeAffectedEntities
* @see AWS API Documentation
*/
java.util.concurrent.Future describeAffectedEntitiesAsync(DescribeAffectedEntitiesRequest describeAffectedEntitiesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns the number of entities that are affected by each of the specified events. If no events are specified, the
* counts of all affected entities are returned.
*
*
* @param describeEntityAggregatesRequest
* @return A Java Future containing the result of the DescribeEntityAggregates operation returned by the service.
* @sample AWSHealthAsync.DescribeEntityAggregates
* @see AWS API Documentation
*/
java.util.concurrent.Future describeEntityAggregatesAsync(DescribeEntityAggregatesRequest describeEntityAggregatesRequest);
/**
*
* Returns the number of entities that are affected by each of the specified events. If no events are specified, the
* counts of all affected entities are returned.
*
*
* @param describeEntityAggregatesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeEntityAggregates operation returned by the service.
* @sample AWSHealthAsyncHandler.DescribeEntityAggregates
* @see AWS API Documentation
*/
java.util.concurrent.Future describeEntityAggregatesAsync(DescribeEntityAggregatesRequest describeEntityAggregatesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns the number of events of each event type (issue, scheduled change, and account notification). If no filter
* is specified, the counts of all events in each category are returned.
*
*
* @param describeEventAggregatesRequest
* @return A Java Future containing the result of the DescribeEventAggregates operation returned by the service.
* @sample AWSHealthAsync.DescribeEventAggregates
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeEventAggregatesAsync(DescribeEventAggregatesRequest describeEventAggregatesRequest);
/**
*
* Returns the number of events of each event type (issue, scheduled change, and account notification). If no filter
* is specified, the counts of all events in each category are returned.
*
*
* @param describeEventAggregatesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeEventAggregates operation returned by the service.
* @sample AWSHealthAsyncHandler.DescribeEventAggregates
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeEventAggregatesAsync(DescribeEventAggregatesRequest describeEventAggregatesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns detailed information about one or more specified events. Information includes standard event data
* (region, service, etc., as returned by DescribeEvents), a detailed event description, and possible
* additional metadata that depends upon the nature of the event. Affected entities are not included; to retrieve
* those, use the DescribeAffectedEntities operation.
*
*
* If a specified event cannot be retrieved, an error message is returned for that event.
*
*
* @param describeEventDetailsRequest
* @return A Java Future containing the result of the DescribeEventDetails operation returned by the service.
* @sample AWSHealthAsync.DescribeEventDetails
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeEventDetailsAsync(DescribeEventDetailsRequest describeEventDetailsRequest);
/**
*
* Returns detailed information about one or more specified events. Information includes standard event data
* (region, service, etc., as returned by DescribeEvents), a detailed event description, and possible
* additional metadata that depends upon the nature of the event. Affected entities are not included; to retrieve
* those, use the DescribeAffectedEntities operation.
*
*
* If a specified event cannot be retrieved, an error message is returned for that event.
*
*
* @param describeEventDetailsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeEventDetails operation returned by the service.
* @sample AWSHealthAsyncHandler.DescribeEventDetails
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeEventDetailsAsync(DescribeEventDetailsRequest describeEventDetailsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns the event types that meet the specified filter criteria. If no filter criteria are specified, all event
* types are returned, in no particular order.
*
*
* @param describeEventTypesRequest
* @return A Java Future containing the result of the DescribeEventTypes operation returned by the service.
* @sample AWSHealthAsync.DescribeEventTypes
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeEventTypesAsync(DescribeEventTypesRequest describeEventTypesRequest);
/**
*
* Returns the event types that meet the specified filter criteria. If no filter criteria are specified, all event
* types are returned, in no particular order.
*
*
* @param describeEventTypesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeEventTypes operation returned by the service.
* @sample AWSHealthAsyncHandler.DescribeEventTypes
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeEventTypesAsync(DescribeEventTypesRequest describeEventTypesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns information about events that meet the specified filter criteria. Events are returned in a summary form
* and do not include the detailed description, any additional metadata that depends on the event type, or any
* affected resources. To retrieve that information, use the DescribeEventDetails and
* DescribeAffectedEntities operations.
*
*
* If no filter criteria are specified, all events are returned. Results are sorted by lastModifiedTime
* , starting with the most recent.
*
*
* @param describeEventsRequest
* @return A Java Future containing the result of the DescribeEvents operation returned by the service.
* @sample AWSHealthAsync.DescribeEvents
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeEventsAsync(DescribeEventsRequest describeEventsRequest);
/**
*
* Returns information about events that meet the specified filter criteria. Events are returned in a summary form
* and do not include the detailed description, any additional metadata that depends on the event type, or any
* affected resources. To retrieve that information, use the DescribeEventDetails and
* DescribeAffectedEntities operations.
*
*
* If no filter criteria are specified, all events are returned. Results are sorted by lastModifiedTime
* , starting with the most recent.
*
*
* @param describeEventsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeEvents operation returned by the service.
* @sample AWSHealthAsyncHandler.DescribeEvents
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeEventsAsync(DescribeEventsRequest describeEventsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
}