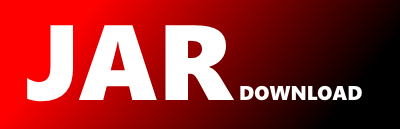
com.amazonaws.services.health.AWSHealthClient Maven / Gradle / Ivy
Show all versions of aws-java-sdk-health Show documentation
/*
* Copyright 2012-2017 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.health;
import org.w3c.dom.*;
import java.net.*;
import java.util.*;
import javax.annotation.Generated;
import org.apache.commons.logging.*;
import com.amazonaws.*;
import com.amazonaws.auth.*;
import com.amazonaws.handlers.*;
import com.amazonaws.http.*;
import com.amazonaws.internal.*;
import com.amazonaws.internal.auth.*;
import com.amazonaws.metrics.*;
import com.amazonaws.regions.*;
import com.amazonaws.transform.*;
import com.amazonaws.util.*;
import com.amazonaws.protocol.json.*;
import com.amazonaws.util.AWSRequestMetrics.Field;
import com.amazonaws.annotation.ThreadSafe;
import com.amazonaws.client.AwsSyncClientParams;
import com.amazonaws.services.health.AWSHealthClientBuilder;
import com.amazonaws.AmazonServiceException;
import com.amazonaws.services.health.model.*;
import com.amazonaws.services.health.model.transform.*;
/**
* Client for accessing AWSHealth. All service calls made using this client are blocking, and will not return until the
* service call completes.
*
* AWS Health
*
* The AWS Health API provides programmatic access to the AWS Health information that is presented in the AWS Personal Health Dashboard. You can get information about events
* that affect your AWS resources:
*
*
* -
*
* DescribeEvents: Summary information about events.
*
*
* -
*
* DescribeEventDetails: Detailed information about one or more events.
*
*
* -
*
* DescribeAffectedEntities: Information about AWS resources that are affected by one or more events.
*
*
*
*
* In addition, these operations provide information about event types and summary counts of events or affected
* entities:
*
*
* -
*
* DescribeEventTypes: Information about the kinds of events that AWS Health tracks.
*
*
* -
*
* DescribeEventAggregates: A count of the number of events that meet specified criteria.
*
*
* -
*
* DescribeEntityAggregates: A count of the number of affected entities that meet specified criteria.
*
*
*
*
* The Health API requires a Business or Enterprise support plan from AWS Support. Calling the Health API from an account that does not
* have a Business or Enterprise support plan causes a SubscriptionRequiredException
.
*
*
* For authentication of requests, AWS Health uses the Signature Version 4 Signing Process.
*
*
* See the AWS Health User Guide for
* information about how to use the API.
*
*
* Service Endpoint
*
*
* The HTTP endpoint for the AWS Health API is:
*
*
* -
*
* https://health.us-east-1.amazonaws.com
*
*
*
*/
@ThreadSafe
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class AWSHealthClient extends AmazonWebServiceClient implements AWSHealth {
/** Provider for AWS credentials. */
private final AWSCredentialsProvider awsCredentialsProvider;
private static final Log log = LogFactory.getLog(AWSHealth.class);
/** Default signing name for the service. */
private static final String DEFAULT_SIGNING_NAME = "health";
/** Client configuration factory providing ClientConfigurations tailored to this client */
protected static final ClientConfigurationFactory configFactory = new ClientConfigurationFactory();
private final com.amazonaws.protocol.json.SdkJsonProtocolFactory protocolFactory = new com.amazonaws.protocol.json.SdkJsonProtocolFactory(
new JsonClientMetadata()
.withProtocolVersion("1.1")
.withSupportsCbor(false)
.withSupportsIon(false)
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("UnsupportedLocale").withModeledClass(
com.amazonaws.services.health.model.UnsupportedLocaleException.class))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("InvalidPaginationToken").withModeledClass(
com.amazonaws.services.health.model.InvalidPaginationTokenException.class))
.withBaseServiceExceptionClass(com.amazonaws.services.health.model.AWSHealthException.class));
/**
* Constructs a new client to invoke service methods on AWSHealth. A credentials provider chain will be used that
* searches for credentials in this order:
*
* - Environment Variables - AWS_ACCESS_KEY_ID and AWS_SECRET_KEY
* - Java System Properties - aws.accessKeyId and aws.secretKey
* - Instance profile credentials delivered through the Amazon EC2 metadata service
*
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @see DefaultAWSCredentialsProviderChain
* @deprecated use {@link AWSHealthClientBuilder#defaultClient()}
*/
@Deprecated
public AWSHealthClient() {
this(DefaultAWSCredentialsProviderChain.getInstance(), configFactory.getConfig());
}
/**
* Constructs a new client to invoke service methods on AWSHealth. A credentials provider chain will be used that
* searches for credentials in this order:
*
* - Environment Variables - AWS_ACCESS_KEY_ID and AWS_SECRET_KEY
* - Java System Properties - aws.accessKeyId and aws.secretKey
* - Instance profile credentials delivered through the Amazon EC2 metadata service
*
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param clientConfiguration
* The client configuration options controlling how this client connects to AWSHealth (ex: proxy settings,
* retry counts, etc.).
*
* @see DefaultAWSCredentialsProviderChain
* @deprecated use {@link AWSHealthClientBuilder#withClientConfiguration(ClientConfiguration)}
*/
@Deprecated
public AWSHealthClient(ClientConfiguration clientConfiguration) {
this(DefaultAWSCredentialsProviderChain.getInstance(), clientConfiguration);
}
/**
* Constructs a new client to invoke service methods on AWSHealth using the specified AWS account credentials.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param awsCredentials
* The AWS credentials (access key ID and secret key) to use when authenticating with AWS services.
* @deprecated use {@link AWSHealthClientBuilder#withCredentials(AWSCredentialsProvider)} for example:
* {@code AWSHealthClientBuilder.standard().withCredentials(new AWSStaticCredentialsProvider(awsCredentials)).build();}
*/
@Deprecated
public AWSHealthClient(AWSCredentials awsCredentials) {
this(awsCredentials, configFactory.getConfig());
}
/**
* Constructs a new client to invoke service methods on AWSHealth using the specified AWS account credentials and
* client configuration options.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param awsCredentials
* The AWS credentials (access key ID and secret key) to use when authenticating with AWS services.
* @param clientConfiguration
* The client configuration options controlling how this client connects to AWSHealth (ex: proxy settings,
* retry counts, etc.).
* @deprecated use {@link AWSHealthClientBuilder#withCredentials(AWSCredentialsProvider)} and
* {@link AWSHealthClientBuilder#withClientConfiguration(ClientConfiguration)}
*/
@Deprecated
public AWSHealthClient(AWSCredentials awsCredentials, ClientConfiguration clientConfiguration) {
super(clientConfiguration);
this.awsCredentialsProvider = new StaticCredentialsProvider(awsCredentials);
init();
}
/**
* Constructs a new client to invoke service methods on AWSHealth using the specified AWS account credentials
* provider.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param awsCredentialsProvider
* The AWS credentials provider which will provide credentials to authenticate requests with AWS services.
* @deprecated use {@link AWSHealthClientBuilder#withCredentials(AWSCredentialsProvider)}
*/
@Deprecated
public AWSHealthClient(AWSCredentialsProvider awsCredentialsProvider) {
this(awsCredentialsProvider, configFactory.getConfig());
}
/**
* Constructs a new client to invoke service methods on AWSHealth using the specified AWS account credentials
* provider and client configuration options.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param awsCredentialsProvider
* The AWS credentials provider which will provide credentials to authenticate requests with AWS services.
* @param clientConfiguration
* The client configuration options controlling how this client connects to AWSHealth (ex: proxy settings,
* retry counts, etc.).
* @deprecated use {@link AWSHealthClientBuilder#withCredentials(AWSCredentialsProvider)} and
* {@link AWSHealthClientBuilder#withClientConfiguration(ClientConfiguration)}
*/
@Deprecated
public AWSHealthClient(AWSCredentialsProvider awsCredentialsProvider, ClientConfiguration clientConfiguration) {
this(awsCredentialsProvider, clientConfiguration, null);
}
/**
* Constructs a new client to invoke service methods on AWSHealth using the specified AWS account credentials
* provider, client configuration options, and request metric collector.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param awsCredentialsProvider
* The AWS credentials provider which will provide credentials to authenticate requests with AWS services.
* @param clientConfiguration
* The client configuration options controlling how this client connects to AWSHealth (ex: proxy settings,
* retry counts, etc.).
* @param requestMetricCollector
* optional request metric collector
* @deprecated use {@link AWSHealthClientBuilder#withCredentials(AWSCredentialsProvider)} and
* {@link AWSHealthClientBuilder#withClientConfiguration(ClientConfiguration)} and
* {@link AWSHealthClientBuilder#withMetricsCollector(RequestMetricCollector)}
*/
@Deprecated
public AWSHealthClient(AWSCredentialsProvider awsCredentialsProvider, ClientConfiguration clientConfiguration, RequestMetricCollector requestMetricCollector) {
super(clientConfiguration, requestMetricCollector);
this.awsCredentialsProvider = awsCredentialsProvider;
init();
}
public static AWSHealthClientBuilder builder() {
return AWSHealthClientBuilder.standard();
}
/**
* Constructs a new client to invoke service methods on AWSHealth using the specified parameters.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param clientParams
* Object providing client parameters.
*/
AWSHealthClient(AwsSyncClientParams clientParams) {
super(clientParams);
this.awsCredentialsProvider = clientParams.getCredentialsProvider();
init();
}
private void init() {
setServiceNameIntern(DEFAULT_SIGNING_NAME);
setEndpointPrefix(ENDPOINT_PREFIX);
// calling this.setEndPoint(...) will also modify the signer accordingly
setEndpoint("health.us-east-1.amazonaws.com");
HandlerChainFactory chainFactory = new HandlerChainFactory();
requestHandler2s.addAll(chainFactory.newRequestHandlerChain("/com/amazonaws/services/health/request.handlers"));
requestHandler2s.addAll(chainFactory.newRequestHandler2Chain("/com/amazonaws/services/health/request.handler2s"));
requestHandler2s.addAll(chainFactory.getGlobalHandlers());
}
/**
*
* Returns a list of entities that have been affected by the specified events, based on the specified filter
* criteria. Entities can refer to individual customer resources, groups of customer resources, or any other
* construct, depending on the AWS service. Events that have impact beyond that of the affected entities, or where
* the extent of impact is unknown, include at least one entity indicating this.
*
*
* At least one event ARN is required. Results are sorted by the lastUpdatedTime
of the entity,
* starting with the most recent.
*
*
* @param describeAffectedEntitiesRequest
* @return Result of the DescribeAffectedEntities operation returned by the service.
* @throws InvalidPaginationTokenException
* The specified pagination token (nextToken
) is not valid.
* @throws UnsupportedLocaleException
* The specified locale is not supported.
* @sample AWSHealth.DescribeAffectedEntities
* @see AWS API Documentation
*/
@Override
public DescribeAffectedEntitiesResult describeAffectedEntities(DescribeAffectedEntitiesRequest describeAffectedEntitiesRequest) {
ExecutionContext executionContext = createExecutionContext(describeAffectedEntitiesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeAffectedEntitiesRequestMarshaller(protocolFactory).marshall(super.beforeMarshalling(describeAffectedEntitiesRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DescribeAffectedEntitiesResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns the number of entities that are affected by each of the specified events. If no events are specified, the
* counts of all affected entities are returned.
*
*
* @param describeEntityAggregatesRequest
* @return Result of the DescribeEntityAggregates operation returned by the service.
* @sample AWSHealth.DescribeEntityAggregates
* @see AWS API Documentation
*/
@Override
public DescribeEntityAggregatesResult describeEntityAggregates(DescribeEntityAggregatesRequest describeEntityAggregatesRequest) {
ExecutionContext executionContext = createExecutionContext(describeEntityAggregatesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeEntityAggregatesRequestMarshaller(protocolFactory).marshall(super.beforeMarshalling(describeEntityAggregatesRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DescribeEntityAggregatesResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns the number of events of each event type (issue, scheduled change, and account notification). If no filter
* is specified, the counts of all events in each category are returned.
*
*
* @param describeEventAggregatesRequest
* @return Result of the DescribeEventAggregates operation returned by the service.
* @throws InvalidPaginationTokenException
* The specified pagination token (nextToken
) is not valid.
* @sample AWSHealth.DescribeEventAggregates
* @see AWS
* API Documentation
*/
@Override
public DescribeEventAggregatesResult describeEventAggregates(DescribeEventAggregatesRequest describeEventAggregatesRequest) {
ExecutionContext executionContext = createExecutionContext(describeEventAggregatesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeEventAggregatesRequestMarshaller(protocolFactory).marshall(super.beforeMarshalling(describeEventAggregatesRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DescribeEventAggregatesResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns detailed information about one or more specified events. Information includes standard event data
* (region, service, etc., as returned by DescribeEvents), a detailed event description, and possible
* additional metadata that depends upon the nature of the event. Affected entities are not included; to retrieve
* those, use the DescribeAffectedEntities operation.
*
*
* If a specified event cannot be retrieved, an error message is returned for that event.
*
*
* @param describeEventDetailsRequest
* @return Result of the DescribeEventDetails operation returned by the service.
* @throws UnsupportedLocaleException
* The specified locale is not supported.
* @sample AWSHealth.DescribeEventDetails
* @see AWS
* API Documentation
*/
@Override
public DescribeEventDetailsResult describeEventDetails(DescribeEventDetailsRequest describeEventDetailsRequest) {
ExecutionContext executionContext = createExecutionContext(describeEventDetailsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeEventDetailsRequestMarshaller(protocolFactory).marshall(super.beforeMarshalling(describeEventDetailsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DescribeEventDetailsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns the event types that meet the specified filter criteria. If no filter criteria are specified, all event
* types are returned, in no particular order.
*
*
* @param describeEventTypesRequest
* @return Result of the DescribeEventTypes operation returned by the service.
* @throws InvalidPaginationTokenException
* The specified pagination token (nextToken
) is not valid.
* @throws UnsupportedLocaleException
* The specified locale is not supported.
* @sample AWSHealth.DescribeEventTypes
* @see AWS API
* Documentation
*/
@Override
public DescribeEventTypesResult describeEventTypes(DescribeEventTypesRequest describeEventTypesRequest) {
ExecutionContext executionContext = createExecutionContext(describeEventTypesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeEventTypesRequestMarshaller(protocolFactory).marshall(super.beforeMarshalling(describeEventTypesRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DescribeEventTypesResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns information about events that meet the specified filter criteria. Events are returned in a summary form
* and do not include the detailed description, any additional metadata that depends on the event type, or any
* affected resources. To retrieve that information, use the DescribeEventDetails and
* DescribeAffectedEntities operations.
*
*
* If no filter criteria are specified, all events are returned. Results are sorted by lastModifiedTime
* , starting with the most recent.
*
*
* @param describeEventsRequest
* @return Result of the DescribeEvents operation returned by the service.
* @throws InvalidPaginationTokenException
* The specified pagination token (nextToken
) is not valid.
* @throws UnsupportedLocaleException
* The specified locale is not supported.
* @sample AWSHealth.DescribeEvents
* @see AWS API
* Documentation
*/
@Override
public DescribeEventsResult describeEvents(DescribeEventsRequest describeEventsRequest) {
ExecutionContext executionContext = createExecutionContext(describeEventsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeEventsRequestMarshaller(protocolFactory).marshall(super.beforeMarshalling(describeEventsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DescribeEventsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
* Returns additional metadata for a previously executed successful, request, typically used for debugging issues
* where a service isn't acting as expected. This data isn't considered part of the result data returned by an
* operation, so it's available through this separate, diagnostic interface.
*
* Response metadata is only cached for a limited period of time, so if you need to access this extra diagnostic
* information for an executed request, you should use this method to retrieve it as soon as possible after
* executing the request.
*
* @param request
* The originally executed request
*
* @return The response metadata for the specified request, or null if none is available.
*/
public ResponseMetadata getCachedResponseMetadata(AmazonWebServiceRequest request) {
return client.getResponseMetadataForRequest(request);
}
/**
* Normal invoke with authentication. Credentials are required and may be overriden at the request level.
**/
private Response invoke(Request request, HttpResponseHandler> responseHandler,
ExecutionContext executionContext) {
executionContext.setCredentialsProvider(CredentialUtils.getCredentialsProvider(request.getOriginalRequest(), awsCredentialsProvider));
return doInvoke(request, responseHandler, executionContext);
}
/**
* Invoke with no authentication. Credentials are not required and any credentials set on the client or request will
* be ignored for this operation.
**/
private Response anonymousInvoke(Request request,
HttpResponseHandler> responseHandler, ExecutionContext executionContext) {
return doInvoke(request, responseHandler, executionContext);
}
/**
* Invoke the request using the http client. Assumes credentials (or lack thereof) have been configured in the
* ExecutionContext beforehand.
**/
private Response doInvoke(Request request, HttpResponseHandler> responseHandler,
ExecutionContext executionContext) {
request.setEndpoint(endpoint);
request.setTimeOffset(timeOffset);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler(new JsonErrorResponseMetadata());
return client.execute(request, responseHandler, errorResponseHandler, executionContext);
}
}