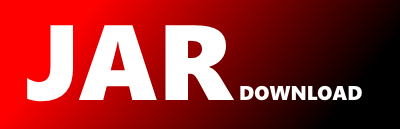
com.amazonaws.services.health.AWSHealth Maven / Gradle / Ivy
Show all versions of aws-java-sdk-health Show documentation
/*
* Copyright 2017-2022 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.health;
import javax.annotation.Generated;
import com.amazonaws.*;
import com.amazonaws.regions.*;
import com.amazonaws.services.health.model.*;
/**
* Interface for accessing AWSHealth.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.health.AbstractAWSHealth} instead.
*
*
* Health
*
* The Health API provides programmatic access to the Health information that appears in the Personal Health Dashboard. You can use the API operations to get
* information about events that might affect your Amazon Web Services services and resources.
*
*
*
* -
*
* You must have a Business, Enterprise On-Ramp, or Enterprise Support plan from Amazon Web Services Support to use the Health API. If you call the
* Health API from an Amazon Web Services account that doesn't have a Business, Enterprise On-Ramp, or Enterprise
* Support plan, you receive a SubscriptionRequiredException
error.
*
*
* -
*
* You can use the Health endpoint health.us-east-1.amazonaws.com (HTTPS) to call the Health API operations. Health
* supports a multi-Region application architecture and has two regional endpoints in an active-passive configuration.
* You can use the high availability endpoint example to determine which Amazon Web Services Region is active, so that
* you can get the latest information from the API. For more information, see Accessing the Health API in the Health
* User Guide.
*
*
*
*
*
* For authentication of requests, Health uses the Signature Version 4 Signing
* Process.
*
*
* If your Amazon Web Services account is part of Organizations, you can use the Health organizational view feature.
* This feature provides a centralized view of Health events across all accounts in your organization. You can aggregate
* Health events in real time to identify accounts in your organization that are affected by an operational event or get
* notified of security vulnerabilities. Use the organizational view API operations to enable this feature and return
* event information. For more information, see Aggregating Health events in the
* Health User Guide.
*
*
*
* When you use the Health API operations to return Health events, see the following recommendations:
*
*
* -
*
* Use the eventScopeCode parameter to specify whether to return Health events that are public or account-specific.
*
*
* -
*
* Use pagination to view all events from the response. For example, if you call the
* DescribeEventsForOrganization
operation to get all events in your organization, you might receive
* several page results. Specify the nextToken
in the next request to return more results.
*
*
*
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AWSHealth {
/**
* The region metadata service name for computing region endpoints. You can use this value to retrieve metadata
* (such as supported regions) of the service.
*
* @see RegionUtils#getRegionsForService(String)
*/
String ENDPOINT_PREFIX = "health";
/**
* Overrides the default endpoint for this client ("health.us-east-1.amazonaws.com"). Callers can use this method to
* control which AWS region they want to work with.
*
* Callers can pass in just the endpoint (ex: "health.us-east-1.amazonaws.com") or a full URL, including the
* protocol (ex: "health.us-east-1.amazonaws.com"). If the protocol is not specified here, the default protocol from
* this client's {@link ClientConfiguration} will be used, which by default is HTTPS.
*
* For more information on using AWS regions with the AWS SDK for Java, and a complete list of all available
* endpoints for all AWS services, see: https://docs.aws.amazon.com/sdk-for-java/v1/developer-guide/java-dg-region-selection.html#region-selection-
* choose-endpoint
*
* This method is not threadsafe. An endpoint should be configured when the client is created and before any
* service requests are made. Changing it afterwards creates inevitable race conditions for any service requests in
* transit or retrying.
*
* @param endpoint
* The endpoint (ex: "health.us-east-1.amazonaws.com") or a full URL, including the protocol (ex:
* "health.us-east-1.amazonaws.com") of the region specific AWS endpoint this client will communicate with.
* @deprecated use {@link AwsClientBuilder#setEndpointConfiguration(AwsClientBuilder.EndpointConfiguration)} for
* example:
* {@code builder.setEndpointConfiguration(new EndpointConfiguration(endpoint, signingRegion));}
*/
@Deprecated
void setEndpoint(String endpoint);
/**
* An alternative to {@link AWSHealth#setEndpoint(String)}, sets the regional endpoint for this client's service
* calls. Callers can use this method to control which AWS region they want to work with.
*
* By default, all service endpoints in all regions use the https protocol. To use http instead, specify it in the
* {@link ClientConfiguration} supplied at construction.
*
* This method is not threadsafe. A region should be configured when the client is created and before any service
* requests are made. Changing it afterwards creates inevitable race conditions for any service requests in transit
* or retrying.
*
* @param region
* The region this client will communicate with. See {@link Region#getRegion(com.amazonaws.regions.Regions)}
* for accessing a given region. Must not be null and must be a region where the service is available.
*
* @see Region#getRegion(com.amazonaws.regions.Regions)
* @see Region#createClient(Class, com.amazonaws.auth.AWSCredentialsProvider, ClientConfiguration)
* @see Region#isServiceSupported(String)
* @deprecated use {@link AwsClientBuilder#setRegion(String)}
*/
@Deprecated
void setRegion(Region region);
/**
*
* Returns a list of accounts in the organization from Organizations that are affected by the provided event. For
* more information about the different types of Health events, see Event.
*
*
* Before you can call this operation, you must first enable Health to work with Organizations. To do this, call the
* EnableHealthServiceAccessForOrganization operation from your organization's management account.
*
*
*
* This API operation uses pagination. Specify the nextToken
parameter in the next request to return
* more results.
*
*
*
* @param describeAffectedAccountsForOrganizationRequest
* @return Result of the DescribeAffectedAccountsForOrganization operation returned by the service.
* @throws InvalidPaginationTokenException
* The specified pagination token (nextToken
) is not valid.
* @sample AWSHealth.DescribeAffectedAccountsForOrganization
* @see AWS API Documentation
*/
DescribeAffectedAccountsForOrganizationResult describeAffectedAccountsForOrganization(
DescribeAffectedAccountsForOrganizationRequest describeAffectedAccountsForOrganizationRequest);
/**
*
* Returns a list of entities that have been affected by the specified events, based on the specified filter
* criteria. Entities can refer to individual customer resources, groups of customer resources, or any other
* construct, depending on the Amazon Web Services service. Events that have impact beyond that of the affected
* entities, or where the extent of impact is unknown, include at least one entity indicating this.
*
*
* At least one event ARN is required.
*
*
*
* -
*
* This API operation uses pagination. Specify the nextToken
parameter in the next request to return
* more results.
*
*
* -
*
* This operation supports resource-level permissions. You can use this operation to allow or deny access to
* specific Health events. For more information, see Resource- and action-based conditions in the Health User Guide.
*
*
*
*
*
* @param describeAffectedEntitiesRequest
* @return Result of the DescribeAffectedEntities operation returned by the service.
* @throws InvalidPaginationTokenException
* The specified pagination token (nextToken
) is not valid.
* @throws UnsupportedLocaleException
* The specified locale is not supported.
* @sample AWSHealth.DescribeAffectedEntities
* @see AWS API Documentation
*/
DescribeAffectedEntitiesResult describeAffectedEntities(DescribeAffectedEntitiesRequest describeAffectedEntitiesRequest);
/**
*
* Returns a list of entities that have been affected by one or more events for one or more accounts in your
* organization in Organizations, based on the filter criteria. Entities can refer to individual customer resources,
* groups of customer resources, or any other construct, depending on the Amazon Web Services service.
*
*
* At least one event Amazon Resource Name (ARN) and account ID are required.
*
*
* Before you can call this operation, you must first enable Health to work with Organizations. To do this, call the
* EnableHealthServiceAccessForOrganization operation from your organization's management account.
*
*
*
* -
*
* This API operation uses pagination. Specify the nextToken
parameter in the next request to return
* more results.
*
*
* -
*
* This operation doesn't support resource-level permissions. You can't use this operation to allow or deny access
* to specific Health events. For more information, see Resource- and action-based conditions in the Health User Guide.
*
*
*
*
*
* @param describeAffectedEntitiesForOrganizationRequest
* @return Result of the DescribeAffectedEntitiesForOrganization operation returned by the service.
* @throws InvalidPaginationTokenException
* The specified pagination token (nextToken
) is not valid.
* @throws UnsupportedLocaleException
* The specified locale is not supported.
* @sample AWSHealth.DescribeAffectedEntitiesForOrganization
* @see AWS API Documentation
*/
DescribeAffectedEntitiesForOrganizationResult describeAffectedEntitiesForOrganization(
DescribeAffectedEntitiesForOrganizationRequest describeAffectedEntitiesForOrganizationRequest);
/**
*
* Returns the number of entities that are affected by each of the specified events.
*
*
* @param describeEntityAggregatesRequest
* @return Result of the DescribeEntityAggregates operation returned by the service.
* @sample AWSHealth.DescribeEntityAggregates
* @see AWS API Documentation
*/
DescribeEntityAggregatesResult describeEntityAggregates(DescribeEntityAggregatesRequest describeEntityAggregatesRequest);
/**
*
* Returns the number of events of each event type (issue, scheduled change, and account notification). If no filter
* is specified, the counts of all events in each category are returned.
*
*
*
* This API operation uses pagination. Specify the nextToken
parameter in the next request to return
* more results.
*
*
*
* @param describeEventAggregatesRequest
* @return Result of the DescribeEventAggregates operation returned by the service.
* @throws InvalidPaginationTokenException
* The specified pagination token (nextToken
) is not valid.
* @sample AWSHealth.DescribeEventAggregates
* @see AWS
* API Documentation
*/
DescribeEventAggregatesResult describeEventAggregates(DescribeEventAggregatesRequest describeEventAggregatesRequest);
/**
*
* Returns detailed information about one or more specified events. Information includes standard event data (Amazon
* Web Services Region, service, and so on, as returned by DescribeEvents), a
* detailed event description, and possible additional metadata that depends upon the nature of the event. Affected
* entities are not included. To retrieve the entities, use the DescribeAffectedEntities operation.
*
*
* If a specified event can't be retrieved, an error message is returned for that event.
*
*
*
* This operation supports resource-level permissions. You can use this operation to allow or deny access to
* specific Health events. For more information, see Resource- and action-based conditions in the Health User Guide.
*
*
*
* @param describeEventDetailsRequest
* @return Result of the DescribeEventDetails operation returned by the service.
* @throws UnsupportedLocaleException
* The specified locale is not supported.
* @sample AWSHealth.DescribeEventDetails
* @see AWS
* API Documentation
*/
DescribeEventDetailsResult describeEventDetails(DescribeEventDetailsRequest describeEventDetailsRequest);
/**
*
* Returns detailed information about one or more specified events for one or more Amazon Web Services accounts in
* your organization. This information includes standard event data (such as the Amazon Web Services Region and
* service), an event description, and (depending on the event) possible metadata. This operation doesn't return
* affected entities, such as the resources related to the event. To return affected entities, use the DescribeAffectedEntitiesForOrganization operation.
*
*
*
* Before you can call this operation, you must first enable Health to work with Organizations. To do this, call the
* EnableHealthServiceAccessForOrganization operation from your organization's management account.
*
*
*
* When you call the DescribeEventDetailsForOrganization
operation, specify the
* organizationEventDetailFilters
object in the request. Depending on the Health event type, note the
* following differences:
*
*
* -
*
* To return event details for a public event, you must specify a null value for the awsAccountId
* parameter. If you specify an account ID for a public event, Health returns an error message because public events
* aren't specific to an account.
*
*
* -
*
* To return event details for an event that is specific to an account in your organization, you must specify the
* awsAccountId
parameter in the request. If you don't specify an account ID, Health returns an error
* message because the event is specific to an account in your organization.
*
*
*
*
* For more information, see Event.
*
*
*
* This operation doesn't support resource-level permissions. You can't use this operation to allow or deny access
* to specific Health events. For more information, see Resource- and action-based conditions in the Health User Guide.
*
*
*
* @param describeEventDetailsForOrganizationRequest
* @return Result of the DescribeEventDetailsForOrganization operation returned by the service.
* @throws UnsupportedLocaleException
* The specified locale is not supported.
* @sample AWSHealth.DescribeEventDetailsForOrganization
* @see AWS API Documentation
*/
DescribeEventDetailsForOrganizationResult describeEventDetailsForOrganization(
DescribeEventDetailsForOrganizationRequest describeEventDetailsForOrganizationRequest);
/**
*
* Returns the event types that meet the specified filter criteria. You can use this API operation to find
* information about the Health event, such as the category, Amazon Web Services service, and event code. The
* metadata for each event appears in the EventType object.
*
*
* If you don't specify a filter criteria, the API operation returns all event types, in no particular order.
*
*
*
* This API operation uses pagination. Specify the nextToken
parameter in the next request to return
* more results.
*
*
*
* @param describeEventTypesRequest
* @return Result of the DescribeEventTypes operation returned by the service.
* @throws InvalidPaginationTokenException
* The specified pagination token (nextToken
) is not valid.
* @throws UnsupportedLocaleException
* The specified locale is not supported.
* @sample AWSHealth.DescribeEventTypes
* @see AWS API
* Documentation
*/
DescribeEventTypesResult describeEventTypes(DescribeEventTypesRequest describeEventTypesRequest);
/**
*
* Returns information about events that meet the specified filter criteria. Events are returned in a summary form
* and do not include the detailed description, any additional metadata that depends on the event type, or any
* affected resources. To retrieve that information, use the DescribeEventDetails and DescribeAffectedEntities operations.
*
*
* If no filter criteria are specified, all events are returned. Results are sorted by lastModifiedTime
* , starting with the most recent event.
*
*
*
* -
*
* When you call the DescribeEvents
operation and specify an entity for the entityValues
* parameter, Health might return public events that aren't specific to that resource. For example, if you call
* DescribeEvents
and specify an ID for an Amazon Elastic Compute Cloud (Amazon EC2) instance, Health
* might return events that aren't specific to that resource or service. To get events that are specific to a
* service, use the services
parameter in the filter
object. For more information, see Event.
*
*
* -
*
* This API operation uses pagination. Specify the nextToken
parameter in the next request to return
* more results.
*
*
*
*
*
* @param describeEventsRequest
* @return Result of the DescribeEvents operation returned by the service.
* @throws InvalidPaginationTokenException
* The specified pagination token (nextToken
) is not valid.
* @throws UnsupportedLocaleException
* The specified locale is not supported.
* @sample AWSHealth.DescribeEvents
* @see AWS API
* Documentation
*/
DescribeEventsResult describeEvents(DescribeEventsRequest describeEventsRequest);
/**
*
* Returns information about events across your organization in Organizations. You can use thefilters
* parameter to specify the events that you want to return. Events are returned in a summary form and don't include
* the affected accounts, detailed description, any additional metadata that depends on the event type, or any
* affected resources. To retrieve that information, use the following operations:
*
*
* -
*
*
* -
*
*
* -
*
*
*
*
* If you don't specify a filter
, the DescribeEventsForOrganizations
returns all events
* across your organization. Results are sorted by lastModifiedTime
, starting with the most recent
* event.
*
*
* For more information about the different types of Health events, see Event.
*
*
* Before you can call this operation, you must first enable Health to work with Organizations. To do this, call the
* EnableHealthServiceAccessForOrganization operation from your organization's management account.
*
*
*
* This API operation uses pagination. Specify the nextToken
parameter in the next request to return
* more results.
*
*
*
* @param describeEventsForOrganizationRequest
* @return Result of the DescribeEventsForOrganization operation returned by the service.
* @throws InvalidPaginationTokenException
* The specified pagination token (nextToken
) is not valid.
* @throws UnsupportedLocaleException
* The specified locale is not supported.
* @sample AWSHealth.DescribeEventsForOrganization
* @see AWS API Documentation
*/
DescribeEventsForOrganizationResult describeEventsForOrganization(DescribeEventsForOrganizationRequest describeEventsForOrganizationRequest);
/**
*
* This operation provides status information on enabling or disabling Health to work with your organization. To
* call this operation, you must sign in as an IAM user, assume an IAM role, or sign in as the root user (not
* recommended) in the organization's management account.
*
*
* @param describeHealthServiceStatusForOrganizationRequest
* @return Result of the DescribeHealthServiceStatusForOrganization operation returned by the service.
* @sample AWSHealth.DescribeHealthServiceStatusForOrganization
* @see AWS API Documentation
*/
DescribeHealthServiceStatusForOrganizationResult describeHealthServiceStatusForOrganization(
DescribeHealthServiceStatusForOrganizationRequest describeHealthServiceStatusForOrganizationRequest);
/**
*
* Disables Health from working with Organizations. To call this operation, you must sign in as an Identity and
* Access Management (IAM) user, assume an IAM role, or sign in as the root user (not recommended) in the
* organization's management account. For more information, see Aggregating Health events in the
* Health User Guide.
*
*
* This operation doesn't remove the service-linked role from the management account in your organization. You must
* use the IAM console, API, or Command Line Interface (CLI) to remove the service-linked role. For more
* information, see Deleting a Service-Linked Role in the IAM User Guide.
*
*
*
* You can also disable the organizational feature by using the Organizations DisableAWSServiceAccess API operation. After you call this operation, Health stops aggregating events for
* all other Amazon Web Services accounts in your organization. If you call the Health API operations for
* organizational view, Health returns an error. Health continues to aggregate health events for your Amazon Web
* Services account.
*
*
*
* @param disableHealthServiceAccessForOrganizationRequest
* @return Result of the DisableHealthServiceAccessForOrganization operation returned by the service.
* @throws ConcurrentModificationException
* EnableHealthServiceAccessForOrganization is already in progress. Wait for the action to complete
* before trying again. To get the current status, use the DescribeHealthServiceStatusForOrganization operation.
* @sample AWSHealth.DisableHealthServiceAccessForOrganization
* @see AWS API Documentation
*/
DisableHealthServiceAccessForOrganizationResult disableHealthServiceAccessForOrganization(
DisableHealthServiceAccessForOrganizationRequest disableHealthServiceAccessForOrganizationRequest);
/**
*
* Enables Health to work with Organizations. You can use the organizational view feature to aggregate events from
* all Amazon Web Services accounts in your organization in a centralized location.
*
*
* This operation also creates a service-linked role for the management account in the organization.
*
*
*
* To call this operation, you must meet the following requirements:
*
*
* -
*
* You must have a Business, Enterprise On-Ramp, or Enterprise Support plan from Amazon Web Services Support to use the Health API. If you call
* the Health API from an Amazon Web Services account that doesn't have a Business, Enterprise On-Ramp, or
* Enterprise Support plan, you receive a SubscriptionRequiredException
error.
*
*
* -
*
* You must have permission to call this operation from the organization's management account. For example IAM
* policies, see Health
* identity-based policy examples.
*
*
*
*
*
* If you don't have the required support plan, you can instead use the Health console to enable the organizational
* view feature. For more information, see Aggregating Health events in the
* Health User Guide.
*
*
* @param enableHealthServiceAccessForOrganizationRequest
* @return Result of the EnableHealthServiceAccessForOrganization operation returned by the service.
* @throws ConcurrentModificationException
* EnableHealthServiceAccessForOrganization is already in progress. Wait for the action to complete
* before trying again. To get the current status, use the DescribeHealthServiceStatusForOrganization operation.
* @sample AWSHealth.EnableHealthServiceAccessForOrganization
* @see AWS API Documentation
*/
EnableHealthServiceAccessForOrganizationResult enableHealthServiceAccessForOrganization(
EnableHealthServiceAccessForOrganizationRequest enableHealthServiceAccessForOrganizationRequest);
/**
* Shuts down this client object, releasing any resources that might be held open. This is an optional method, and
* callers are not expected to call it, but can if they want to explicitly release any open resources. Once a client
* has been shutdown, it should not be used to make any more requests.
*/
void shutdown();
/**
* Returns additional metadata for a previously executed successful request, typically used for debugging issues
* where a service isn't acting as expected. This data isn't considered part of the result data returned by an
* operation, so it's available through this separate, diagnostic interface.
*
* Response metadata is only cached for a limited period of time, so if you need to access this extra diagnostic
* information for an executed request, you should use this method to retrieve it as soon as possible after
* executing a request.
*
* @param request
* The originally executed request.
*
* @return The response metadata for the specified request, or null if none is available.
*/
ResponseMetadata getCachedResponseMetadata(AmazonWebServiceRequest request);
}