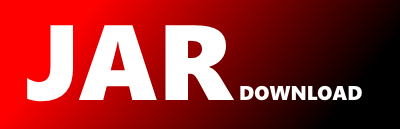
com.amazonaws.services.health.AWSHealthClient Maven / Gradle / Ivy
Show all versions of aws-java-sdk-health Show documentation
/*
* Copyright 2017-2022 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.health;
import org.w3c.dom.*;
import java.net.*;
import java.util.*;
import javax.annotation.Generated;
import org.apache.commons.logging.*;
import com.amazonaws.*;
import com.amazonaws.annotation.SdkInternalApi;
import com.amazonaws.auth.*;
import com.amazonaws.handlers.*;
import com.amazonaws.http.*;
import com.amazonaws.internal.*;
import com.amazonaws.internal.auth.*;
import com.amazonaws.metrics.*;
import com.amazonaws.regions.*;
import com.amazonaws.transform.*;
import com.amazonaws.util.*;
import com.amazonaws.protocol.json.*;
import com.amazonaws.util.AWSRequestMetrics.Field;
import com.amazonaws.annotation.ThreadSafe;
import com.amazonaws.client.AwsSyncClientParams;
import com.amazonaws.client.builder.AdvancedConfig;
import com.amazonaws.services.health.AWSHealthClientBuilder;
import com.amazonaws.AmazonServiceException;
import com.amazonaws.services.health.model.*;
import com.amazonaws.services.health.model.transform.*;
/**
* Client for accessing AWSHealth. All service calls made using this client are blocking, and will not return until the
* service call completes.
*
* Health
*
* The Health API provides programmatic access to the Health information that appears in the Personal Health Dashboard. You can use the API operations to get
* information about events that might affect your Amazon Web Services services and resources.
*
*
*
* -
*
* You must have a Business, Enterprise On-Ramp, or Enterprise Support plan from Amazon Web Services Support to use the Health API. If you call the
* Health API from an Amazon Web Services account that doesn't have a Business, Enterprise On-Ramp, or Enterprise
* Support plan, you receive a SubscriptionRequiredException
error.
*
*
* -
*
* You can use the Health endpoint health.us-east-1.amazonaws.com (HTTPS) to call the Health API operations. Health
* supports a multi-Region application architecture and has two regional endpoints in an active-passive configuration.
* You can use the high availability endpoint example to determine which Amazon Web Services Region is active, so that
* you can get the latest information from the API. For more information, see Accessing the Health API in the Health
* User Guide.
*
*
*
*
*
* For authentication of requests, Health uses the Signature Version 4 Signing
* Process.
*
*
* If your Amazon Web Services account is part of Organizations, you can use the Health organizational view feature.
* This feature provides a centralized view of Health events across all accounts in your organization. You can aggregate
* Health events in real time to identify accounts in your organization that are affected by an operational event or get
* notified of security vulnerabilities. Use the organizational view API operations to enable this feature and return
* event information. For more information, see Aggregating Health events in the
* Health User Guide.
*
*
*
* When you use the Health API operations to return Health events, see the following recommendations:
*
*
* -
*
* Use the eventScopeCode parameter to specify whether to return Health events that are public or account-specific.
*
*
* -
*
* Use pagination to view all events from the response. For example, if you call the
* DescribeEventsForOrganization
operation to get all events in your organization, you might receive
* several page results. Specify the nextToken
in the next request to return more results.
*
*
*
*
*/
@ThreadSafe
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class AWSHealthClient extends AmazonWebServiceClient implements AWSHealth {
/** Provider for AWS credentials. */
private final AWSCredentialsProvider awsCredentialsProvider;
private static final Log log = LogFactory.getLog(AWSHealth.class);
/** Default signing name for the service. */
private static final String DEFAULT_SIGNING_NAME = "health";
/** Client configuration factory providing ClientConfigurations tailored to this client */
protected static final ClientConfigurationFactory configFactory = new ClientConfigurationFactory();
private final AdvancedConfig advancedConfig;
private static final com.amazonaws.protocol.json.SdkJsonProtocolFactory protocolFactory = new com.amazonaws.protocol.json.SdkJsonProtocolFactory(
new JsonClientMetadata()
.withProtocolVersion("1.1")
.withSupportsCbor(false)
.withSupportsIon(false)
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ConcurrentModificationException").withExceptionUnmarshaller(
com.amazonaws.services.health.model.transform.ConcurrentModificationExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("UnsupportedLocale").withExceptionUnmarshaller(
com.amazonaws.services.health.model.transform.UnsupportedLocaleExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("InvalidPaginationToken").withExceptionUnmarshaller(
com.amazonaws.services.health.model.transform.InvalidPaginationTokenExceptionUnmarshaller.getInstance()))
.withBaseServiceExceptionClass(com.amazonaws.services.health.model.AWSHealthException.class));
/**
* Constructs a new client to invoke service methods on AWSHealth. A credentials provider chain will be used that
* searches for credentials in this order:
*
* - Environment Variables - AWS_ACCESS_KEY_ID and AWS_SECRET_KEY
* - Java System Properties - aws.accessKeyId and aws.secretKey
* - Instance profile credentials delivered through the Amazon EC2 metadata service
*
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @see DefaultAWSCredentialsProviderChain
* @deprecated use {@link AWSHealthClientBuilder#defaultClient()}
*/
@Deprecated
public AWSHealthClient() {
this(DefaultAWSCredentialsProviderChain.getInstance(), configFactory.getConfig());
}
/**
* Constructs a new client to invoke service methods on AWSHealth. A credentials provider chain will be used that
* searches for credentials in this order:
*
* - Environment Variables - AWS_ACCESS_KEY_ID and AWS_SECRET_KEY
* - Java System Properties - aws.accessKeyId and aws.secretKey
* - Instance profile credentials delivered through the Amazon EC2 metadata service
*
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param clientConfiguration
* The client configuration options controlling how this client connects to AWSHealth (ex: proxy settings,
* retry counts, etc.).
*
* @see DefaultAWSCredentialsProviderChain
* @deprecated use {@link AWSHealthClientBuilder#withClientConfiguration(ClientConfiguration)}
*/
@Deprecated
public AWSHealthClient(ClientConfiguration clientConfiguration) {
this(DefaultAWSCredentialsProviderChain.getInstance(), clientConfiguration);
}
/**
* Constructs a new client to invoke service methods on AWSHealth using the specified AWS account credentials.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param awsCredentials
* The AWS credentials (access key ID and secret key) to use when authenticating with AWS services.
* @deprecated use {@link AWSHealthClientBuilder#withCredentials(AWSCredentialsProvider)} for example:
* {@code AWSHealthClientBuilder.standard().withCredentials(new AWSStaticCredentialsProvider(awsCredentials)).build();}
*/
@Deprecated
public AWSHealthClient(AWSCredentials awsCredentials) {
this(awsCredentials, configFactory.getConfig());
}
/**
* Constructs a new client to invoke service methods on AWSHealth using the specified AWS account credentials and
* client configuration options.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param awsCredentials
* The AWS credentials (access key ID and secret key) to use when authenticating with AWS services.
* @param clientConfiguration
* The client configuration options controlling how this client connects to AWSHealth (ex: proxy settings,
* retry counts, etc.).
* @deprecated use {@link AWSHealthClientBuilder#withCredentials(AWSCredentialsProvider)} and
* {@link AWSHealthClientBuilder#withClientConfiguration(ClientConfiguration)}
*/
@Deprecated
public AWSHealthClient(AWSCredentials awsCredentials, ClientConfiguration clientConfiguration) {
super(clientConfiguration);
this.awsCredentialsProvider = new StaticCredentialsProvider(awsCredentials);
this.advancedConfig = AdvancedConfig.EMPTY;
init();
}
/**
* Constructs a new client to invoke service methods on AWSHealth using the specified AWS account credentials
* provider.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param awsCredentialsProvider
* The AWS credentials provider which will provide credentials to authenticate requests with AWS services.
* @deprecated use {@link AWSHealthClientBuilder#withCredentials(AWSCredentialsProvider)}
*/
@Deprecated
public AWSHealthClient(AWSCredentialsProvider awsCredentialsProvider) {
this(awsCredentialsProvider, configFactory.getConfig());
}
/**
* Constructs a new client to invoke service methods on AWSHealth using the specified AWS account credentials
* provider and client configuration options.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param awsCredentialsProvider
* The AWS credentials provider which will provide credentials to authenticate requests with AWS services.
* @param clientConfiguration
* The client configuration options controlling how this client connects to AWSHealth (ex: proxy settings,
* retry counts, etc.).
* @deprecated use {@link AWSHealthClientBuilder#withCredentials(AWSCredentialsProvider)} and
* {@link AWSHealthClientBuilder#withClientConfiguration(ClientConfiguration)}
*/
@Deprecated
public AWSHealthClient(AWSCredentialsProvider awsCredentialsProvider, ClientConfiguration clientConfiguration) {
this(awsCredentialsProvider, clientConfiguration, null);
}
/**
* Constructs a new client to invoke service methods on AWSHealth using the specified AWS account credentials
* provider, client configuration options, and request metric collector.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param awsCredentialsProvider
* The AWS credentials provider which will provide credentials to authenticate requests with AWS services.
* @param clientConfiguration
* The client configuration options controlling how this client connects to AWSHealth (ex: proxy settings,
* retry counts, etc.).
* @param requestMetricCollector
* optional request metric collector
* @deprecated use {@link AWSHealthClientBuilder#withCredentials(AWSCredentialsProvider)} and
* {@link AWSHealthClientBuilder#withClientConfiguration(ClientConfiguration)} and
* {@link AWSHealthClientBuilder#withMetricsCollector(RequestMetricCollector)}
*/
@Deprecated
public AWSHealthClient(AWSCredentialsProvider awsCredentialsProvider, ClientConfiguration clientConfiguration, RequestMetricCollector requestMetricCollector) {
super(clientConfiguration, requestMetricCollector);
this.awsCredentialsProvider = awsCredentialsProvider;
this.advancedConfig = AdvancedConfig.EMPTY;
init();
}
public static AWSHealthClientBuilder builder() {
return AWSHealthClientBuilder.standard();
}
/**
* Constructs a new client to invoke service methods on AWSHealth using the specified parameters.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param clientParams
* Object providing client parameters.
*/
AWSHealthClient(AwsSyncClientParams clientParams) {
this(clientParams, false);
}
/**
* Constructs a new client to invoke service methods on AWSHealth using the specified parameters.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param clientParams
* Object providing client parameters.
*/
AWSHealthClient(AwsSyncClientParams clientParams, boolean endpointDiscoveryEnabled) {
super(clientParams);
this.awsCredentialsProvider = clientParams.getCredentialsProvider();
this.advancedConfig = clientParams.getAdvancedConfig();
init();
}
private void init() {
setServiceNameIntern(DEFAULT_SIGNING_NAME);
setEndpointPrefix(ENDPOINT_PREFIX);
// calling this.setEndPoint(...) will also modify the signer accordingly
setEndpoint("health.us-east-1.amazonaws.com");
HandlerChainFactory chainFactory = new HandlerChainFactory();
requestHandler2s.addAll(chainFactory.newRequestHandlerChain("/com/amazonaws/services/health/request.handlers"));
requestHandler2s.addAll(chainFactory.newRequestHandler2Chain("/com/amazonaws/services/health/request.handler2s"));
requestHandler2s.addAll(chainFactory.getGlobalHandlers());
}
/**
*
* Returns a list of accounts in the organization from Organizations that are affected by the provided event. For
* more information about the different types of Health events, see Event.
*
*
* Before you can call this operation, you must first enable Health to work with Organizations. To do this, call the
* EnableHealthServiceAccessForOrganization operation from your organization's management account.
*
*
*
* This API operation uses pagination. Specify the nextToken
parameter in the next request to return
* more results.
*
*
*
* @param describeAffectedAccountsForOrganizationRequest
* @return Result of the DescribeAffectedAccountsForOrganization operation returned by the service.
* @throws InvalidPaginationTokenException
* The specified pagination token (nextToken
) is not valid.
* @sample AWSHealth.DescribeAffectedAccountsForOrganization
* @see AWS API Documentation
*/
@Override
public DescribeAffectedAccountsForOrganizationResult describeAffectedAccountsForOrganization(DescribeAffectedAccountsForOrganizationRequest request) {
request = beforeClientExecution(request);
return executeDescribeAffectedAccountsForOrganization(request);
}
@SdkInternalApi
final DescribeAffectedAccountsForOrganizationResult executeDescribeAffectedAccountsForOrganization(
DescribeAffectedAccountsForOrganizationRequest describeAffectedAccountsForOrganizationRequest) {
ExecutionContext executionContext = createExecutionContext(describeAffectedAccountsForOrganizationRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeAffectedAccountsForOrganizationRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(describeAffectedAccountsForOrganizationRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Health");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeAffectedAccountsForOrganization");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DescribeAffectedAccountsForOrganizationResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns a list of entities that have been affected by the specified events, based on the specified filter
* criteria. Entities can refer to individual customer resources, groups of customer resources, or any other
* construct, depending on the Amazon Web Services service. Events that have impact beyond that of the affected
* entities, or where the extent of impact is unknown, include at least one entity indicating this.
*
*
* At least one event ARN is required.
*
*
*
* -
*
* This API operation uses pagination. Specify the nextToken
parameter in the next request to return
* more results.
*
*
* -
*
* This operation supports resource-level permissions. You can use this operation to allow or deny access to
* specific Health events. For more information, see Resource- and action-based conditions in the Health User Guide.
*
*
*
*
*
* @param describeAffectedEntitiesRequest
* @return Result of the DescribeAffectedEntities operation returned by the service.
* @throws InvalidPaginationTokenException
* The specified pagination token (nextToken
) is not valid.
* @throws UnsupportedLocaleException
* The specified locale is not supported.
* @sample AWSHealth.DescribeAffectedEntities
* @see AWS API Documentation
*/
@Override
public DescribeAffectedEntitiesResult describeAffectedEntities(DescribeAffectedEntitiesRequest request) {
request = beforeClientExecution(request);
return executeDescribeAffectedEntities(request);
}
@SdkInternalApi
final DescribeAffectedEntitiesResult executeDescribeAffectedEntities(DescribeAffectedEntitiesRequest describeAffectedEntitiesRequest) {
ExecutionContext executionContext = createExecutionContext(describeAffectedEntitiesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeAffectedEntitiesRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(describeAffectedEntitiesRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Health");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeAffectedEntities");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DescribeAffectedEntitiesResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns a list of entities that have been affected by one or more events for one or more accounts in your
* organization in Organizations, based on the filter criteria. Entities can refer to individual customer resources,
* groups of customer resources, or any other construct, depending on the Amazon Web Services service.
*
*
* At least one event Amazon Resource Name (ARN) and account ID are required.
*
*
* Before you can call this operation, you must first enable Health to work with Organizations. To do this, call the
* EnableHealthServiceAccessForOrganization operation from your organization's management account.
*
*
*
* -
*
* This API operation uses pagination. Specify the nextToken
parameter in the next request to return
* more results.
*
*
* -
*
* This operation doesn't support resource-level permissions. You can't use this operation to allow or deny access
* to specific Health events. For more information, see Resource- and action-based conditions in the Health User Guide.
*
*
*
*
*
* @param describeAffectedEntitiesForOrganizationRequest
* @return Result of the DescribeAffectedEntitiesForOrganization operation returned by the service.
* @throws InvalidPaginationTokenException
* The specified pagination token (nextToken
) is not valid.
* @throws UnsupportedLocaleException
* The specified locale is not supported.
* @sample AWSHealth.DescribeAffectedEntitiesForOrganization
* @see AWS API Documentation
*/
@Override
public DescribeAffectedEntitiesForOrganizationResult describeAffectedEntitiesForOrganization(DescribeAffectedEntitiesForOrganizationRequest request) {
request = beforeClientExecution(request);
return executeDescribeAffectedEntitiesForOrganization(request);
}
@SdkInternalApi
final DescribeAffectedEntitiesForOrganizationResult executeDescribeAffectedEntitiesForOrganization(
DescribeAffectedEntitiesForOrganizationRequest describeAffectedEntitiesForOrganizationRequest) {
ExecutionContext executionContext = createExecutionContext(describeAffectedEntitiesForOrganizationRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeAffectedEntitiesForOrganizationRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(describeAffectedEntitiesForOrganizationRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Health");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeAffectedEntitiesForOrganization");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DescribeAffectedEntitiesForOrganizationResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns the number of entities that are affected by each of the specified events.
*
*
* @param describeEntityAggregatesRequest
* @return Result of the DescribeEntityAggregates operation returned by the service.
* @sample AWSHealth.DescribeEntityAggregates
* @see AWS API Documentation
*/
@Override
public DescribeEntityAggregatesResult describeEntityAggregates(DescribeEntityAggregatesRequest request) {
request = beforeClientExecution(request);
return executeDescribeEntityAggregates(request);
}
@SdkInternalApi
final DescribeEntityAggregatesResult executeDescribeEntityAggregates(DescribeEntityAggregatesRequest describeEntityAggregatesRequest) {
ExecutionContext executionContext = createExecutionContext(describeEntityAggregatesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeEntityAggregatesRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(describeEntityAggregatesRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Health");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeEntityAggregates");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DescribeEntityAggregatesResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns the number of events of each event type (issue, scheduled change, and account notification). If no filter
* is specified, the counts of all events in each category are returned.
*
*
*
* This API operation uses pagination. Specify the nextToken
parameter in the next request to return
* more results.
*
*
*
* @param describeEventAggregatesRequest
* @return Result of the DescribeEventAggregates operation returned by the service.
* @throws InvalidPaginationTokenException
* The specified pagination token (nextToken
) is not valid.
* @sample AWSHealth.DescribeEventAggregates
* @see AWS
* API Documentation
*/
@Override
public DescribeEventAggregatesResult describeEventAggregates(DescribeEventAggregatesRequest request) {
request = beforeClientExecution(request);
return executeDescribeEventAggregates(request);
}
@SdkInternalApi
final DescribeEventAggregatesResult executeDescribeEventAggregates(DescribeEventAggregatesRequest describeEventAggregatesRequest) {
ExecutionContext executionContext = createExecutionContext(describeEventAggregatesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeEventAggregatesRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(describeEventAggregatesRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Health");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeEventAggregates");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DescribeEventAggregatesResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns detailed information about one or more specified events. Information includes standard event data (Amazon
* Web Services Region, service, and so on, as returned by DescribeEvents), a
* detailed event description, and possible additional metadata that depends upon the nature of the event. Affected
* entities are not included. To retrieve the entities, use the DescribeAffectedEntities operation.
*
*
* If a specified event can't be retrieved, an error message is returned for that event.
*
*
*
* This operation supports resource-level permissions. You can use this operation to allow or deny access to
* specific Health events. For more information, see Resource- and action-based conditions in the Health User Guide.
*
*
*
* @param describeEventDetailsRequest
* @return Result of the DescribeEventDetails operation returned by the service.
* @throws UnsupportedLocaleException
* The specified locale is not supported.
* @sample AWSHealth.DescribeEventDetails
* @see AWS
* API Documentation
*/
@Override
public DescribeEventDetailsResult describeEventDetails(DescribeEventDetailsRequest request) {
request = beforeClientExecution(request);
return executeDescribeEventDetails(request);
}
@SdkInternalApi
final DescribeEventDetailsResult executeDescribeEventDetails(DescribeEventDetailsRequest describeEventDetailsRequest) {
ExecutionContext executionContext = createExecutionContext(describeEventDetailsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeEventDetailsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(describeEventDetailsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Health");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeEventDetails");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DescribeEventDetailsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns detailed information about one or more specified events for one or more Amazon Web Services accounts in
* your organization. This information includes standard event data (such as the Amazon Web Services Region and
* service), an event description, and (depending on the event) possible metadata. This operation doesn't return
* affected entities, such as the resources related to the event. To return affected entities, use the DescribeAffectedEntitiesForOrganization operation.
*
*
*
* Before you can call this operation, you must first enable Health to work with Organizations. To do this, call the
* EnableHealthServiceAccessForOrganization operation from your organization's management account.
*
*
*
* When you call the DescribeEventDetailsForOrganization
operation, specify the
* organizationEventDetailFilters
object in the request. Depending on the Health event type, note the
* following differences:
*
*
* -
*
* To return event details for a public event, you must specify a null value for the awsAccountId
* parameter. If you specify an account ID for a public event, Health returns an error message because public events
* aren't specific to an account.
*
*
* -
*
* To return event details for an event that is specific to an account in your organization, you must specify the
* awsAccountId
parameter in the request. If you don't specify an account ID, Health returns an error
* message because the event is specific to an account in your organization.
*
*
*
*
* For more information, see Event.
*
*
*
* This operation doesn't support resource-level permissions. You can't use this operation to allow or deny access
* to specific Health events. For more information, see Resource- and action-based conditions in the Health User Guide.
*
*
*
* @param describeEventDetailsForOrganizationRequest
* @return Result of the DescribeEventDetailsForOrganization operation returned by the service.
* @throws UnsupportedLocaleException
* The specified locale is not supported.
* @sample AWSHealth.DescribeEventDetailsForOrganization
* @see AWS API Documentation
*/
@Override
public DescribeEventDetailsForOrganizationResult describeEventDetailsForOrganization(DescribeEventDetailsForOrganizationRequest request) {
request = beforeClientExecution(request);
return executeDescribeEventDetailsForOrganization(request);
}
@SdkInternalApi
final DescribeEventDetailsForOrganizationResult executeDescribeEventDetailsForOrganization(
DescribeEventDetailsForOrganizationRequest describeEventDetailsForOrganizationRequest) {
ExecutionContext executionContext = createExecutionContext(describeEventDetailsForOrganizationRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeEventDetailsForOrganizationRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(describeEventDetailsForOrganizationRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Health");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeEventDetailsForOrganization");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DescribeEventDetailsForOrganizationResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns the event types that meet the specified filter criteria. You can use this API operation to find
* information about the Health event, such as the category, Amazon Web Services service, and event code. The
* metadata for each event appears in the EventType object.
*
*
* If you don't specify a filter criteria, the API operation returns all event types, in no particular order.
*
*
*
* This API operation uses pagination. Specify the nextToken
parameter in the next request to return
* more results.
*
*
*
* @param describeEventTypesRequest
* @return Result of the DescribeEventTypes operation returned by the service.
* @throws InvalidPaginationTokenException
* The specified pagination token (nextToken
) is not valid.
* @throws UnsupportedLocaleException
* The specified locale is not supported.
* @sample AWSHealth.DescribeEventTypes
* @see AWS API
* Documentation
*/
@Override
public DescribeEventTypesResult describeEventTypes(DescribeEventTypesRequest request) {
request = beforeClientExecution(request);
return executeDescribeEventTypes(request);
}
@SdkInternalApi
final DescribeEventTypesResult executeDescribeEventTypes(DescribeEventTypesRequest describeEventTypesRequest) {
ExecutionContext executionContext = createExecutionContext(describeEventTypesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeEventTypesRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(describeEventTypesRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Health");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeEventTypes");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DescribeEventTypesResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns information about events that meet the specified filter criteria. Events are returned in a summary form
* and do not include the detailed description, any additional metadata that depends on the event type, or any
* affected resources. To retrieve that information, use the DescribeEventDetails and DescribeAffectedEntities operations.
*
*
* If no filter criteria are specified, all events are returned. Results are sorted by lastModifiedTime
* , starting with the most recent event.
*
*
*
* -
*
* When you call the DescribeEvents
operation and specify an entity for the entityValues
* parameter, Health might return public events that aren't specific to that resource. For example, if you call
* DescribeEvents
and specify an ID for an Amazon Elastic Compute Cloud (Amazon EC2) instance, Health
* might return events that aren't specific to that resource or service. To get events that are specific to a
* service, use the services
parameter in the filter
object. For more information, see Event.
*
*
* -
*
* This API operation uses pagination. Specify the nextToken
parameter in the next request to return
* more results.
*
*
*
*
*
* @param describeEventsRequest
* @return Result of the DescribeEvents operation returned by the service.
* @throws InvalidPaginationTokenException
* The specified pagination token (nextToken
) is not valid.
* @throws UnsupportedLocaleException
* The specified locale is not supported.
* @sample AWSHealth.DescribeEvents
* @see AWS API
* Documentation
*/
@Override
public DescribeEventsResult describeEvents(DescribeEventsRequest request) {
request = beforeClientExecution(request);
return executeDescribeEvents(request);
}
@SdkInternalApi
final DescribeEventsResult executeDescribeEvents(DescribeEventsRequest describeEventsRequest) {
ExecutionContext executionContext = createExecutionContext(describeEventsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeEventsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(describeEventsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Health");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeEvents");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DescribeEventsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns information about events across your organization in Organizations. You can use thefilters
* parameter to specify the events that you want to return. Events are returned in a summary form and don't include
* the affected accounts, detailed description, any additional metadata that depends on the event type, or any
* affected resources. To retrieve that information, use the following operations:
*
*
* -
*
*
* -
*
*
* -
*
*
*
*
* If you don't specify a filter
, the DescribeEventsForOrganizations
returns all events
* across your organization. Results are sorted by lastModifiedTime
, starting with the most recent
* event.
*
*
* For more information about the different types of Health events, see Event.
*
*
* Before you can call this operation, you must first enable Health to work with Organizations. To do this, call the
* EnableHealthServiceAccessForOrganization operation from your organization's management account.
*
*
*
* This API operation uses pagination. Specify the nextToken
parameter in the next request to return
* more results.
*
*
*
* @param describeEventsForOrganizationRequest
* @return Result of the DescribeEventsForOrganization operation returned by the service.
* @throws InvalidPaginationTokenException
* The specified pagination token (nextToken
) is not valid.
* @throws UnsupportedLocaleException
* The specified locale is not supported.
* @sample AWSHealth.DescribeEventsForOrganization
* @see AWS API Documentation
*/
@Override
public DescribeEventsForOrganizationResult describeEventsForOrganization(DescribeEventsForOrganizationRequest request) {
request = beforeClientExecution(request);
return executeDescribeEventsForOrganization(request);
}
@SdkInternalApi
final DescribeEventsForOrganizationResult executeDescribeEventsForOrganization(DescribeEventsForOrganizationRequest describeEventsForOrganizationRequest) {
ExecutionContext executionContext = createExecutionContext(describeEventsForOrganizationRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeEventsForOrganizationRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(describeEventsForOrganizationRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Health");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeEventsForOrganization");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DescribeEventsForOrganizationResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* This operation provides status information on enabling or disabling Health to work with your organization. To
* call this operation, you must sign in as an IAM user, assume an IAM role, or sign in as the root user (not
* recommended) in the organization's management account.
*
*
* @param describeHealthServiceStatusForOrganizationRequest
* @return Result of the DescribeHealthServiceStatusForOrganization operation returned by the service.
* @sample AWSHealth.DescribeHealthServiceStatusForOrganization
* @see AWS API Documentation
*/
@Override
public DescribeHealthServiceStatusForOrganizationResult describeHealthServiceStatusForOrganization(DescribeHealthServiceStatusForOrganizationRequest request) {
request = beforeClientExecution(request);
return executeDescribeHealthServiceStatusForOrganization(request);
}
@SdkInternalApi
final DescribeHealthServiceStatusForOrganizationResult executeDescribeHealthServiceStatusForOrganization(
DescribeHealthServiceStatusForOrganizationRequest describeHealthServiceStatusForOrganizationRequest) {
ExecutionContext executionContext = createExecutionContext(describeHealthServiceStatusForOrganizationRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeHealthServiceStatusForOrganizationRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(describeHealthServiceStatusForOrganizationRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Health");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeHealthServiceStatusForOrganization");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DescribeHealthServiceStatusForOrganizationResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Disables Health from working with Organizations. To call this operation, you must sign in as an Identity and
* Access Management (IAM) user, assume an IAM role, or sign in as the root user (not recommended) in the
* organization's management account. For more information, see Aggregating Health events in the
* Health User Guide.
*
*
* This operation doesn't remove the service-linked role from the management account in your organization. You must
* use the IAM console, API, or Command Line Interface (CLI) to remove the service-linked role. For more
* information, see Deleting a Service-Linked Role in the IAM User Guide.
*
*
*
* You can also disable the organizational feature by using the Organizations DisableAWSServiceAccess API operation. After you call this operation, Health stops aggregating events for
* all other Amazon Web Services accounts in your organization. If you call the Health API operations for
* organizational view, Health returns an error. Health continues to aggregate health events for your Amazon Web
* Services account.
*
*
*
* @param disableHealthServiceAccessForOrganizationRequest
* @return Result of the DisableHealthServiceAccessForOrganization operation returned by the service.
* @throws ConcurrentModificationException
* EnableHealthServiceAccessForOrganization is already in progress. Wait for the action to complete
* before trying again. To get the current status, use the DescribeHealthServiceStatusForOrganization operation.
* @sample AWSHealth.DisableHealthServiceAccessForOrganization
* @see AWS API Documentation
*/
@Override
public DisableHealthServiceAccessForOrganizationResult disableHealthServiceAccessForOrganization(DisableHealthServiceAccessForOrganizationRequest request) {
request = beforeClientExecution(request);
return executeDisableHealthServiceAccessForOrganization(request);
}
@SdkInternalApi
final DisableHealthServiceAccessForOrganizationResult executeDisableHealthServiceAccessForOrganization(
DisableHealthServiceAccessForOrganizationRequest disableHealthServiceAccessForOrganizationRequest) {
ExecutionContext executionContext = createExecutionContext(disableHealthServiceAccessForOrganizationRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DisableHealthServiceAccessForOrganizationRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(disableHealthServiceAccessForOrganizationRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Health");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DisableHealthServiceAccessForOrganization");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DisableHealthServiceAccessForOrganizationResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Enables Health to work with Organizations. You can use the organizational view feature to aggregate events from
* all Amazon Web Services accounts in your organization in a centralized location.
*
*
* This operation also creates a service-linked role for the management account in the organization.
*
*
*
* To call this operation, you must meet the following requirements:
*
*
* -
*
* You must have a Business, Enterprise On-Ramp, or Enterprise Support plan from Amazon Web Services Support to use the Health API. If you call
* the Health API from an Amazon Web Services account that doesn't have a Business, Enterprise On-Ramp, or
* Enterprise Support plan, you receive a SubscriptionRequiredException
error.
*
*
* -
*
* You must have permission to call this operation from the organization's management account. For example IAM
* policies, see Health
* identity-based policy examples.
*
*
*
*
*
* If you don't have the required support plan, you can instead use the Health console to enable the organizational
* view feature. For more information, see Aggregating Health events in the
* Health User Guide.
*
*
* @param enableHealthServiceAccessForOrganizationRequest
* @return Result of the EnableHealthServiceAccessForOrganization operation returned by the service.
* @throws ConcurrentModificationException
* EnableHealthServiceAccessForOrganization is already in progress. Wait for the action to complete
* before trying again. To get the current status, use the DescribeHealthServiceStatusForOrganization operation.
* @sample AWSHealth.EnableHealthServiceAccessForOrganization
* @see AWS API Documentation
*/
@Override
public EnableHealthServiceAccessForOrganizationResult enableHealthServiceAccessForOrganization(EnableHealthServiceAccessForOrganizationRequest request) {
request = beforeClientExecution(request);
return executeEnableHealthServiceAccessForOrganization(request);
}
@SdkInternalApi
final EnableHealthServiceAccessForOrganizationResult executeEnableHealthServiceAccessForOrganization(
EnableHealthServiceAccessForOrganizationRequest enableHealthServiceAccessForOrganizationRequest) {
ExecutionContext executionContext = createExecutionContext(enableHealthServiceAccessForOrganizationRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new EnableHealthServiceAccessForOrganizationRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(enableHealthServiceAccessForOrganizationRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Health");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "EnableHealthServiceAccessForOrganization");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new EnableHealthServiceAccessForOrganizationResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
* Returns additional metadata for a previously executed successful, request, typically used for debugging issues
* where a service isn't acting as expected. This data isn't considered part of the result data returned by an
* operation, so it's available through this separate, diagnostic interface.
*
* Response metadata is only cached for a limited period of time, so if you need to access this extra diagnostic
* information for an executed request, you should use this method to retrieve it as soon as possible after
* executing the request.
*
* @param request
* The originally executed request
*
* @return The response metadata for the specified request, or null if none is available.
*/
public ResponseMetadata getCachedResponseMetadata(AmazonWebServiceRequest request) {
return client.getResponseMetadataForRequest(request);
}
/**
* Normal invoke with authentication. Credentials are required and may be overriden at the request level.
**/
private Response invoke(Request request, HttpResponseHandler> responseHandler,
ExecutionContext executionContext) {
return invoke(request, responseHandler, executionContext, null, null);
}
/**
* Normal invoke with authentication. Credentials are required and may be overriden at the request level.
**/
private Response invoke(Request request, HttpResponseHandler> responseHandler,
ExecutionContext executionContext, URI cachedEndpoint, URI uriFromEndpointTrait) {
executionContext.setCredentialsProvider(CredentialUtils.getCredentialsProvider(request.getOriginalRequest(), awsCredentialsProvider));
return doInvoke(request, responseHandler, executionContext, cachedEndpoint, uriFromEndpointTrait);
}
/**
* Invoke with no authentication. Credentials are not required and any credentials set on the client or request will
* be ignored for this operation.
**/
private Response anonymousInvoke(Request request,
HttpResponseHandler> responseHandler, ExecutionContext executionContext) {
return doInvoke(request, responseHandler, executionContext, null, null);
}
/**
* Invoke the request using the http client. Assumes credentials (or lack thereof) have been configured in the
* ExecutionContext beforehand.
**/
private Response doInvoke(Request request, HttpResponseHandler> responseHandler,
ExecutionContext executionContext, URI discoveredEndpoint, URI uriFromEndpointTrait) {
if (discoveredEndpoint != null) {
request.setEndpoint(discoveredEndpoint);
request.getOriginalRequest().getRequestClientOptions().appendUserAgent("endpoint-discovery");
} else if (uriFromEndpointTrait != null) {
request.setEndpoint(uriFromEndpointTrait);
} else {
request.setEndpoint(endpoint);
}
request.setTimeOffset(timeOffset);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler(new JsonErrorResponseMetadata());
return client.execute(request, responseHandler, errorResponseHandler, executionContext);
}
@com.amazonaws.annotation.SdkInternalApi
static com.amazonaws.protocol.json.SdkJsonProtocolFactory getProtocolFactory() {
return protocolFactory;
}
@Override
public void shutdown() {
super.shutdown();
}
}