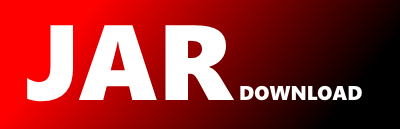
com.amazonaws.services.health.model.OrganizationEvent Maven / Gradle / Ivy
Show all versions of aws-java-sdk-health Show documentation
/*
* Copyright 2017-2022 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.health.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* Summary information about an event, returned by the DescribeEventsForOrganization operation.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class OrganizationEvent implements Serializable, Cloneable, StructuredPojo {
/**
*
* The unique identifier for the event. The event ARN has the
* arn:aws:health:event-region::event/SERVICE/EVENT_TYPE_CODE/EVENT_TYPE_PLUS_ID
* format.
*
*
* For example, an event ARN might look like the following:
*
*
* arn:aws:health:us-east-1::event/EC2/EC2_INSTANCE_RETIREMENT_SCHEDULED/EC2_INSTANCE_RETIREMENT_SCHEDULED_ABC123-DEF456
*
*/
private String arn;
/**
*
* The Amazon Web Services service that is affected by the event, such as EC2 and RDS.
*
*/
private String service;
/**
*
* The unique identifier for the event type. The format is AWS_SERVICE_DESCRIPTION
. For example,
* AWS_EC2_SYSTEM_MAINTENANCE_EVENT
.
*
*/
private String eventTypeCode;
/**
*
* A list of event type category codes. Possible values are issue
, accountNotification
, or
* scheduledChange
. Currently, the investigation
value isn't supported at this time.
*
*/
private String eventTypeCategory;
/**
*
* This parameter specifies if the Health event is a public Amazon Web Services service event or an account-specific
* event.
*
*
* -
*
* If the eventScopeCode
value is PUBLIC
, then the affectedAccounts
value is
* always empty.
*
*
* -
*
* If the eventScopeCode
value is ACCOUNT_SPECIFIC
, then the affectedAccounts
* value lists the affected Amazon Web Services accounts in your organization. For example, if an event affects a
* service such as Amazon Elastic Compute Cloud and you have Amazon Web Services accounts that use that service,
* those account IDs appear in the response.
*
*
* -
*
* If the eventScopeCode
value is NONE
, then the eventArn
that you specified
* in the request is invalid or doesn't exist.
*
*
*
*/
private String eventScopeCode;
/**
*
* The Amazon Web Services Region name of the event.
*
*/
private String region;
/**
*
* The date and time that the event began.
*
*/
private java.util.Date startTime;
/**
*
* The date and time that the event ended.
*
*/
private java.util.Date endTime;
/**
*
* The most recent date and time that the event was updated.
*
*/
private java.util.Date lastUpdatedTime;
/**
*
* The most recent status of the event. Possible values are open
, closed
, and
* upcoming
.
*
*/
private String statusCode;
/**
*
* The unique identifier for the event. The event ARN has the
* arn:aws:health:event-region::event/SERVICE/EVENT_TYPE_CODE/EVENT_TYPE_PLUS_ID
* format.
*
*
* For example, an event ARN might look like the following:
*
*
* arn:aws:health:us-east-1::event/EC2/EC2_INSTANCE_RETIREMENT_SCHEDULED/EC2_INSTANCE_RETIREMENT_SCHEDULED_ABC123-DEF456
*
*
* @param arn
* The unique identifier for the event. The event ARN has the
* arn:aws:health:event-region::event/SERVICE/EVENT_TYPE_CODE/EVENT_TYPE_PLUS_ID
* format.
*
* For example, an event ARN might look like the following:
*
*
* arn:aws:health:us-east-1::event/EC2/EC2_INSTANCE_RETIREMENT_SCHEDULED/EC2_INSTANCE_RETIREMENT_SCHEDULED_ABC123-DEF456
*/
public void setArn(String arn) {
this.arn = arn;
}
/**
*
* The unique identifier for the event. The event ARN has the
* arn:aws:health:event-region::event/SERVICE/EVENT_TYPE_CODE/EVENT_TYPE_PLUS_ID
* format.
*
*
* For example, an event ARN might look like the following:
*
*
* arn:aws:health:us-east-1::event/EC2/EC2_INSTANCE_RETIREMENT_SCHEDULED/EC2_INSTANCE_RETIREMENT_SCHEDULED_ABC123-DEF456
*
*
* @return The unique identifier for the event. The event ARN has the
* arn:aws:health:event-region::event/SERVICE/EVENT_TYPE_CODE/EVENT_TYPE_PLUS_ID
* format.
*
* For example, an event ARN might look like the following:
*
*
* arn:aws:health:us-east-1::event/EC2/EC2_INSTANCE_RETIREMENT_SCHEDULED/EC2_INSTANCE_RETIREMENT_SCHEDULED_ABC123-DEF456
*/
public String getArn() {
return this.arn;
}
/**
*
* The unique identifier for the event. The event ARN has the
* arn:aws:health:event-region::event/SERVICE/EVENT_TYPE_CODE/EVENT_TYPE_PLUS_ID
* format.
*
*
* For example, an event ARN might look like the following:
*
*
* arn:aws:health:us-east-1::event/EC2/EC2_INSTANCE_RETIREMENT_SCHEDULED/EC2_INSTANCE_RETIREMENT_SCHEDULED_ABC123-DEF456
*
*
* @param arn
* The unique identifier for the event. The event ARN has the
* arn:aws:health:event-region::event/SERVICE/EVENT_TYPE_CODE/EVENT_TYPE_PLUS_ID
* format.
*
* For example, an event ARN might look like the following:
*
*
* arn:aws:health:us-east-1::event/EC2/EC2_INSTANCE_RETIREMENT_SCHEDULED/EC2_INSTANCE_RETIREMENT_SCHEDULED_ABC123-DEF456
* @return Returns a reference to this object so that method calls can be chained together.
*/
public OrganizationEvent withArn(String arn) {
setArn(arn);
return this;
}
/**
*
* The Amazon Web Services service that is affected by the event, such as EC2 and RDS.
*
*
* @param service
* The Amazon Web Services service that is affected by the event, such as EC2 and RDS.
*/
public void setService(String service) {
this.service = service;
}
/**
*
* The Amazon Web Services service that is affected by the event, such as EC2 and RDS.
*
*
* @return The Amazon Web Services service that is affected by the event, such as EC2 and RDS.
*/
public String getService() {
return this.service;
}
/**
*
* The Amazon Web Services service that is affected by the event, such as EC2 and RDS.
*
*
* @param service
* The Amazon Web Services service that is affected by the event, such as EC2 and RDS.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public OrganizationEvent withService(String service) {
setService(service);
return this;
}
/**
*
* The unique identifier for the event type. The format is AWS_SERVICE_DESCRIPTION
. For example,
* AWS_EC2_SYSTEM_MAINTENANCE_EVENT
.
*
*
* @param eventTypeCode
* The unique identifier for the event type. The format is AWS_SERVICE_DESCRIPTION
. For example,
* AWS_EC2_SYSTEM_MAINTENANCE_EVENT
.
*/
public void setEventTypeCode(String eventTypeCode) {
this.eventTypeCode = eventTypeCode;
}
/**
*
* The unique identifier for the event type. The format is AWS_SERVICE_DESCRIPTION
. For example,
* AWS_EC2_SYSTEM_MAINTENANCE_EVENT
.
*
*
* @return The unique identifier for the event type. The format is AWS_SERVICE_DESCRIPTION
. For
* example, AWS_EC2_SYSTEM_MAINTENANCE_EVENT
.
*/
public String getEventTypeCode() {
return this.eventTypeCode;
}
/**
*
* The unique identifier for the event type. The format is AWS_SERVICE_DESCRIPTION
. For example,
* AWS_EC2_SYSTEM_MAINTENANCE_EVENT
.
*
*
* @param eventTypeCode
* The unique identifier for the event type. The format is AWS_SERVICE_DESCRIPTION
. For example,
* AWS_EC2_SYSTEM_MAINTENANCE_EVENT
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public OrganizationEvent withEventTypeCode(String eventTypeCode) {
setEventTypeCode(eventTypeCode);
return this;
}
/**
*
* A list of event type category codes. Possible values are issue
, accountNotification
, or
* scheduledChange
. Currently, the investigation
value isn't supported at this time.
*
*
* @param eventTypeCategory
* A list of event type category codes. Possible values are issue
,
* accountNotification
, or scheduledChange
. Currently, the
* investigation
value isn't supported at this time.
* @see EventTypeCategory
*/
public void setEventTypeCategory(String eventTypeCategory) {
this.eventTypeCategory = eventTypeCategory;
}
/**
*
* A list of event type category codes. Possible values are issue
, accountNotification
, or
* scheduledChange
. Currently, the investigation
value isn't supported at this time.
*
*
* @return A list of event type category codes. Possible values are issue
,
* accountNotification
, or scheduledChange
. Currently, the
* investigation
value isn't supported at this time.
* @see EventTypeCategory
*/
public String getEventTypeCategory() {
return this.eventTypeCategory;
}
/**
*
* A list of event type category codes. Possible values are issue
, accountNotification
, or
* scheduledChange
. Currently, the investigation
value isn't supported at this time.
*
*
* @param eventTypeCategory
* A list of event type category codes. Possible values are issue
,
* accountNotification
, or scheduledChange
. Currently, the
* investigation
value isn't supported at this time.
* @return Returns a reference to this object so that method calls can be chained together.
* @see EventTypeCategory
*/
public OrganizationEvent withEventTypeCategory(String eventTypeCategory) {
setEventTypeCategory(eventTypeCategory);
return this;
}
/**
*
* A list of event type category codes. Possible values are issue
, accountNotification
, or
* scheduledChange
. Currently, the investigation
value isn't supported at this time.
*
*
* @param eventTypeCategory
* A list of event type category codes. Possible values are issue
,
* accountNotification
, or scheduledChange
. Currently, the
* investigation
value isn't supported at this time.
* @return Returns a reference to this object so that method calls can be chained together.
* @see EventTypeCategory
*/
public OrganizationEvent withEventTypeCategory(EventTypeCategory eventTypeCategory) {
this.eventTypeCategory = eventTypeCategory.toString();
return this;
}
/**
*
* This parameter specifies if the Health event is a public Amazon Web Services service event or an account-specific
* event.
*
*
* -
*
* If the eventScopeCode
value is PUBLIC
, then the affectedAccounts
value is
* always empty.
*
*
* -
*
* If the eventScopeCode
value is ACCOUNT_SPECIFIC
, then the affectedAccounts
* value lists the affected Amazon Web Services accounts in your organization. For example, if an event affects a
* service such as Amazon Elastic Compute Cloud and you have Amazon Web Services accounts that use that service,
* those account IDs appear in the response.
*
*
* -
*
* If the eventScopeCode
value is NONE
, then the eventArn
that you specified
* in the request is invalid or doesn't exist.
*
*
*
*
* @param eventScopeCode
* This parameter specifies if the Health event is a public Amazon Web Services service event or an
* account-specific event.
*
* -
*
* If the eventScopeCode
value is PUBLIC
, then the affectedAccounts
* value is always empty.
*
*
* -
*
* If the eventScopeCode
value is ACCOUNT_SPECIFIC
, then the
* affectedAccounts
value lists the affected Amazon Web Services accounts in your organization.
* For example, if an event affects a service such as Amazon Elastic Compute Cloud and you have Amazon Web
* Services accounts that use that service, those account IDs appear in the response.
*
*
* -
*
* If the eventScopeCode
value is NONE
, then the eventArn
that you
* specified in the request is invalid or doesn't exist.
*
*
* @see EventScopeCode
*/
public void setEventScopeCode(String eventScopeCode) {
this.eventScopeCode = eventScopeCode;
}
/**
*
* This parameter specifies if the Health event is a public Amazon Web Services service event or an account-specific
* event.
*
*
* -
*
* If the eventScopeCode
value is PUBLIC
, then the affectedAccounts
value is
* always empty.
*
*
* -
*
* If the eventScopeCode
value is ACCOUNT_SPECIFIC
, then the affectedAccounts
* value lists the affected Amazon Web Services accounts in your organization. For example, if an event affects a
* service such as Amazon Elastic Compute Cloud and you have Amazon Web Services accounts that use that service,
* those account IDs appear in the response.
*
*
* -
*
* If the eventScopeCode
value is NONE
, then the eventArn
that you specified
* in the request is invalid or doesn't exist.
*
*
*
*
* @return This parameter specifies if the Health event is a public Amazon Web Services service event or an
* account-specific event.
*
* -
*
* If the eventScopeCode
value is PUBLIC
, then the affectedAccounts
* value is always empty.
*
*
* -
*
* If the eventScopeCode
value is ACCOUNT_SPECIFIC
, then the
* affectedAccounts
value lists the affected Amazon Web Services accounts in your organization.
* For example, if an event affects a service such as Amazon Elastic Compute Cloud and you have Amazon Web
* Services accounts that use that service, those account IDs appear in the response.
*
*
* -
*
* If the eventScopeCode
value is NONE
, then the eventArn
that you
* specified in the request is invalid or doesn't exist.
*
*
* @see EventScopeCode
*/
public String getEventScopeCode() {
return this.eventScopeCode;
}
/**
*
* This parameter specifies if the Health event is a public Amazon Web Services service event or an account-specific
* event.
*
*
* -
*
* If the eventScopeCode
value is PUBLIC
, then the affectedAccounts
value is
* always empty.
*
*
* -
*
* If the eventScopeCode
value is ACCOUNT_SPECIFIC
, then the affectedAccounts
* value lists the affected Amazon Web Services accounts in your organization. For example, if an event affects a
* service such as Amazon Elastic Compute Cloud and you have Amazon Web Services accounts that use that service,
* those account IDs appear in the response.
*
*
* -
*
* If the eventScopeCode
value is NONE
, then the eventArn
that you specified
* in the request is invalid or doesn't exist.
*
*
*
*
* @param eventScopeCode
* This parameter specifies if the Health event is a public Amazon Web Services service event or an
* account-specific event.
*
* -
*
* If the eventScopeCode
value is PUBLIC
, then the affectedAccounts
* value is always empty.
*
*
* -
*
* If the eventScopeCode
value is ACCOUNT_SPECIFIC
, then the
* affectedAccounts
value lists the affected Amazon Web Services accounts in your organization.
* For example, if an event affects a service such as Amazon Elastic Compute Cloud and you have Amazon Web
* Services accounts that use that service, those account IDs appear in the response.
*
*
* -
*
* If the eventScopeCode
value is NONE
, then the eventArn
that you
* specified in the request is invalid or doesn't exist.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see EventScopeCode
*/
public OrganizationEvent withEventScopeCode(String eventScopeCode) {
setEventScopeCode(eventScopeCode);
return this;
}
/**
*
* This parameter specifies if the Health event is a public Amazon Web Services service event or an account-specific
* event.
*
*
* -
*
* If the eventScopeCode
value is PUBLIC
, then the affectedAccounts
value is
* always empty.
*
*
* -
*
* If the eventScopeCode
value is ACCOUNT_SPECIFIC
, then the affectedAccounts
* value lists the affected Amazon Web Services accounts in your organization. For example, if an event affects a
* service such as Amazon Elastic Compute Cloud and you have Amazon Web Services accounts that use that service,
* those account IDs appear in the response.
*
*
* -
*
* If the eventScopeCode
value is NONE
, then the eventArn
that you specified
* in the request is invalid or doesn't exist.
*
*
*
*
* @param eventScopeCode
* This parameter specifies if the Health event is a public Amazon Web Services service event or an
* account-specific event.
*
* -
*
* If the eventScopeCode
value is PUBLIC
, then the affectedAccounts
* value is always empty.
*
*
* -
*
* If the eventScopeCode
value is ACCOUNT_SPECIFIC
, then the
* affectedAccounts
value lists the affected Amazon Web Services accounts in your organization.
* For example, if an event affects a service such as Amazon Elastic Compute Cloud and you have Amazon Web
* Services accounts that use that service, those account IDs appear in the response.
*
*
* -
*
* If the eventScopeCode
value is NONE
, then the eventArn
that you
* specified in the request is invalid or doesn't exist.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see EventScopeCode
*/
public OrganizationEvent withEventScopeCode(EventScopeCode eventScopeCode) {
this.eventScopeCode = eventScopeCode.toString();
return this;
}
/**
*
* The Amazon Web Services Region name of the event.
*
*
* @param region
* The Amazon Web Services Region name of the event.
*/
public void setRegion(String region) {
this.region = region;
}
/**
*
* The Amazon Web Services Region name of the event.
*
*
* @return The Amazon Web Services Region name of the event.
*/
public String getRegion() {
return this.region;
}
/**
*
* The Amazon Web Services Region name of the event.
*
*
* @param region
* The Amazon Web Services Region name of the event.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public OrganizationEvent withRegion(String region) {
setRegion(region);
return this;
}
/**
*
* The date and time that the event began.
*
*
* @param startTime
* The date and time that the event began.
*/
public void setStartTime(java.util.Date startTime) {
this.startTime = startTime;
}
/**
*
* The date and time that the event began.
*
*
* @return The date and time that the event began.
*/
public java.util.Date getStartTime() {
return this.startTime;
}
/**
*
* The date and time that the event began.
*
*
* @param startTime
* The date and time that the event began.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public OrganizationEvent withStartTime(java.util.Date startTime) {
setStartTime(startTime);
return this;
}
/**
*
* The date and time that the event ended.
*
*
* @param endTime
* The date and time that the event ended.
*/
public void setEndTime(java.util.Date endTime) {
this.endTime = endTime;
}
/**
*
* The date and time that the event ended.
*
*
* @return The date and time that the event ended.
*/
public java.util.Date getEndTime() {
return this.endTime;
}
/**
*
* The date and time that the event ended.
*
*
* @param endTime
* The date and time that the event ended.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public OrganizationEvent withEndTime(java.util.Date endTime) {
setEndTime(endTime);
return this;
}
/**
*
* The most recent date and time that the event was updated.
*
*
* @param lastUpdatedTime
* The most recent date and time that the event was updated.
*/
public void setLastUpdatedTime(java.util.Date lastUpdatedTime) {
this.lastUpdatedTime = lastUpdatedTime;
}
/**
*
* The most recent date and time that the event was updated.
*
*
* @return The most recent date and time that the event was updated.
*/
public java.util.Date getLastUpdatedTime() {
return this.lastUpdatedTime;
}
/**
*
* The most recent date and time that the event was updated.
*
*
* @param lastUpdatedTime
* The most recent date and time that the event was updated.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public OrganizationEvent withLastUpdatedTime(java.util.Date lastUpdatedTime) {
setLastUpdatedTime(lastUpdatedTime);
return this;
}
/**
*
* The most recent status of the event. Possible values are open
, closed
, and
* upcoming
.
*
*
* @param statusCode
* The most recent status of the event. Possible values are open
, closed
, and
* upcoming
.
* @see EventStatusCode
*/
public void setStatusCode(String statusCode) {
this.statusCode = statusCode;
}
/**
*
* The most recent status of the event. Possible values are open
, closed
, and
* upcoming
.
*
*
* @return The most recent status of the event. Possible values are open
, closed
, and
* upcoming
.
* @see EventStatusCode
*/
public String getStatusCode() {
return this.statusCode;
}
/**
*
* The most recent status of the event. Possible values are open
, closed
, and
* upcoming
.
*
*
* @param statusCode
* The most recent status of the event. Possible values are open
, closed
, and
* upcoming
.
* @return Returns a reference to this object so that method calls can be chained together.
* @see EventStatusCode
*/
public OrganizationEvent withStatusCode(String statusCode) {
setStatusCode(statusCode);
return this;
}
/**
*
* The most recent status of the event. Possible values are open
, closed
, and
* upcoming
.
*
*
* @param statusCode
* The most recent status of the event. Possible values are open
, closed
, and
* upcoming
.
* @return Returns a reference to this object so that method calls can be chained together.
* @see EventStatusCode
*/
public OrganizationEvent withStatusCode(EventStatusCode statusCode) {
this.statusCode = statusCode.toString();
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getArn() != null)
sb.append("Arn: ").append(getArn()).append(",");
if (getService() != null)
sb.append("Service: ").append(getService()).append(",");
if (getEventTypeCode() != null)
sb.append("EventTypeCode: ").append(getEventTypeCode()).append(",");
if (getEventTypeCategory() != null)
sb.append("EventTypeCategory: ").append(getEventTypeCategory()).append(",");
if (getEventScopeCode() != null)
sb.append("EventScopeCode: ").append(getEventScopeCode()).append(",");
if (getRegion() != null)
sb.append("Region: ").append(getRegion()).append(",");
if (getStartTime() != null)
sb.append("StartTime: ").append(getStartTime()).append(",");
if (getEndTime() != null)
sb.append("EndTime: ").append(getEndTime()).append(",");
if (getLastUpdatedTime() != null)
sb.append("LastUpdatedTime: ").append(getLastUpdatedTime()).append(",");
if (getStatusCode() != null)
sb.append("StatusCode: ").append(getStatusCode());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof OrganizationEvent == false)
return false;
OrganizationEvent other = (OrganizationEvent) obj;
if (other.getArn() == null ^ this.getArn() == null)
return false;
if (other.getArn() != null && other.getArn().equals(this.getArn()) == false)
return false;
if (other.getService() == null ^ this.getService() == null)
return false;
if (other.getService() != null && other.getService().equals(this.getService()) == false)
return false;
if (other.getEventTypeCode() == null ^ this.getEventTypeCode() == null)
return false;
if (other.getEventTypeCode() != null && other.getEventTypeCode().equals(this.getEventTypeCode()) == false)
return false;
if (other.getEventTypeCategory() == null ^ this.getEventTypeCategory() == null)
return false;
if (other.getEventTypeCategory() != null && other.getEventTypeCategory().equals(this.getEventTypeCategory()) == false)
return false;
if (other.getEventScopeCode() == null ^ this.getEventScopeCode() == null)
return false;
if (other.getEventScopeCode() != null && other.getEventScopeCode().equals(this.getEventScopeCode()) == false)
return false;
if (other.getRegion() == null ^ this.getRegion() == null)
return false;
if (other.getRegion() != null && other.getRegion().equals(this.getRegion()) == false)
return false;
if (other.getStartTime() == null ^ this.getStartTime() == null)
return false;
if (other.getStartTime() != null && other.getStartTime().equals(this.getStartTime()) == false)
return false;
if (other.getEndTime() == null ^ this.getEndTime() == null)
return false;
if (other.getEndTime() != null && other.getEndTime().equals(this.getEndTime()) == false)
return false;
if (other.getLastUpdatedTime() == null ^ this.getLastUpdatedTime() == null)
return false;
if (other.getLastUpdatedTime() != null && other.getLastUpdatedTime().equals(this.getLastUpdatedTime()) == false)
return false;
if (other.getStatusCode() == null ^ this.getStatusCode() == null)
return false;
if (other.getStatusCode() != null && other.getStatusCode().equals(this.getStatusCode()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getArn() == null) ? 0 : getArn().hashCode());
hashCode = prime * hashCode + ((getService() == null) ? 0 : getService().hashCode());
hashCode = prime * hashCode + ((getEventTypeCode() == null) ? 0 : getEventTypeCode().hashCode());
hashCode = prime * hashCode + ((getEventTypeCategory() == null) ? 0 : getEventTypeCategory().hashCode());
hashCode = prime * hashCode + ((getEventScopeCode() == null) ? 0 : getEventScopeCode().hashCode());
hashCode = prime * hashCode + ((getRegion() == null) ? 0 : getRegion().hashCode());
hashCode = prime * hashCode + ((getStartTime() == null) ? 0 : getStartTime().hashCode());
hashCode = prime * hashCode + ((getEndTime() == null) ? 0 : getEndTime().hashCode());
hashCode = prime * hashCode + ((getLastUpdatedTime() == null) ? 0 : getLastUpdatedTime().hashCode());
hashCode = prime * hashCode + ((getStatusCode() == null) ? 0 : getStatusCode().hashCode());
return hashCode;
}
@Override
public OrganizationEvent clone() {
try {
return (OrganizationEvent) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.health.model.transform.OrganizationEventMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}