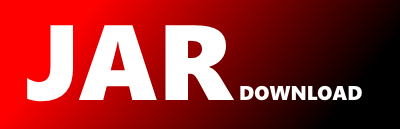
com.amazonaws.services.honeycode.AmazonHoneycode Maven / Gradle / Ivy
Show all versions of aws-java-sdk-honeycode Show documentation
/*
* Copyright 2017-2022 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.honeycode;
import javax.annotation.Generated;
import com.amazonaws.*;
import com.amazonaws.regions.*;
import com.amazonaws.services.honeycode.model.*;
/**
* Interface for accessing Honeycode.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.honeycode.AbstractAmazonHoneycode} instead.
*
*
*
* Amazon Honeycode is a fully managed service that allows you to quickly build mobile and web apps for teams—without
* programming. Build Honeycode apps for managing almost anything, like projects, customers, operations, approvals,
* resources, and even your team.
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AmazonHoneycode {
/**
* The region metadata service name for computing region endpoints. You can use this value to retrieve metadata
* (such as supported regions) of the service.
*
* @see RegionUtils#getRegionsForService(String)
*/
String ENDPOINT_PREFIX = "honeycode";
/**
*
* The BatchCreateTableRows API allows you to create one or more rows at the end of a table in a workbook. The API
* allows you to specify the values to set in some or all of the columns in the new rows.
*
*
* If a column is not explicitly set in a specific row, then the column level formula specified in the table will be
* applied to the new row. If there is no column level formula but the last row of the table has a formula, then
* that formula will be copied down to the new row. If there is no column level formula and no formula in the last
* row of the table, then that column will be left blank for the new rows.
*
*
* @param batchCreateTableRowsRequest
* @return Result of the BatchCreateTableRows operation returned by the service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action. Check that the workbook is owned by you and
* your IAM policy allows access to the resource in the request.
* @throws InternalServerException
* There were unexpected errors from the server.
* @throws RequestTimeoutException
* The request timed out.
* @throws ResourceNotFoundException
* A Workbook, Table, App, Screen or Screen Automation was not found with the given ID.
* @throws ServiceQuotaExceededException
* The request caused service quota to be breached.
* @throws ServiceUnavailableException
* Remote service is unreachable.
* @throws ThrottlingException
* Tps(transactions per second) rate reached.
* @throws ValidationException
* Request is invalid. The message in the response contains details on why the request is invalid.
* @sample AmazonHoneycode.BatchCreateTableRows
* @see AWS
* API Documentation
*/
BatchCreateTableRowsResult batchCreateTableRows(BatchCreateTableRowsRequest batchCreateTableRowsRequest);
/**
*
* The BatchDeleteTableRows API allows you to delete one or more rows from a table in a workbook. You need to
* specify the ids of the rows that you want to delete from the table.
*
*
* @param batchDeleteTableRowsRequest
* @return Result of the BatchDeleteTableRows operation returned by the service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action. Check that the workbook is owned by you and
* your IAM policy allows access to the resource in the request.
* @throws InternalServerException
* There were unexpected errors from the server.
* @throws ResourceNotFoundException
* A Workbook, Table, App, Screen or Screen Automation was not found with the given ID.
* @throws ServiceUnavailableException
* Remote service is unreachable.
* @throws ValidationException
* Request is invalid. The message in the response contains details on why the request is invalid.
* @throws RequestTimeoutException
* The request timed out.
* @throws ThrottlingException
* Tps(transactions per second) rate reached.
* @sample AmazonHoneycode.BatchDeleteTableRows
* @see AWS
* API Documentation
*/
BatchDeleteTableRowsResult batchDeleteTableRows(BatchDeleteTableRowsRequest batchDeleteTableRowsRequest);
/**
*
* The BatchUpdateTableRows API allows you to update one or more rows in a table in a workbook.
*
*
* You can specify the values to set in some or all of the columns in the table for the specified rows. If a column
* is not explicitly specified in a particular row, then that column will not be updated for that row. To clear out
* the data in a specific cell, you need to set the value as an empty string ("").
*
*
* @param batchUpdateTableRowsRequest
* @return Result of the BatchUpdateTableRows operation returned by the service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action. Check that the workbook is owned by you and
* your IAM policy allows access to the resource in the request.
* @throws InternalServerException
* There were unexpected errors from the server.
* @throws ResourceNotFoundException
* A Workbook, Table, App, Screen or Screen Automation was not found with the given ID.
* @throws ServiceUnavailableException
* Remote service is unreachable.
* @throws ValidationException
* Request is invalid. The message in the response contains details on why the request is invalid.
* @throws RequestTimeoutException
* The request timed out.
* @throws ThrottlingException
* Tps(transactions per second) rate reached.
* @sample AmazonHoneycode.BatchUpdateTableRows
* @see AWS
* API Documentation
*/
BatchUpdateTableRowsResult batchUpdateTableRows(BatchUpdateTableRowsRequest batchUpdateTableRowsRequest);
/**
*
* The BatchUpsertTableRows API allows you to upsert one or more rows in a table. The upsert operation takes a
* filter expression as input and evaluates it to find matching rows on the destination table. If matching rows are
* found, it will update the cells in the matching rows to new values specified in the request. If no matching rows
* are found, a new row is added at the end of the table and the cells in that row are set to the new values
* specified in the request.
*
*
* You can specify the values to set in some or all of the columns in the table for the matching or newly appended
* rows. If a column is not explicitly specified for a particular row, then that column will not be updated for that
* row. To clear out the data in a specific cell, you need to set the value as an empty string ("").
*
*
* @param batchUpsertTableRowsRequest
* @return Result of the BatchUpsertTableRows operation returned by the service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action. Check that the workbook is owned by you and
* your IAM policy allows access to the resource in the request.
* @throws InternalServerException
* There were unexpected errors from the server.
* @throws RequestTimeoutException
* The request timed out.
* @throws ResourceNotFoundException
* A Workbook, Table, App, Screen or Screen Automation was not found with the given ID.
* @throws ServiceQuotaExceededException
* The request caused service quota to be breached.
* @throws ServiceUnavailableException
* Remote service is unreachable.
* @throws ThrottlingException
* Tps(transactions per second) rate reached.
* @throws ValidationException
* Request is invalid. The message in the response contains details on why the request is invalid.
* @sample AmazonHoneycode.BatchUpsertTableRows
* @see AWS
* API Documentation
*/
BatchUpsertTableRowsResult batchUpsertTableRows(BatchUpsertTableRowsRequest batchUpsertTableRowsRequest);
/**
*
* The DescribeTableDataImportJob API allows you to retrieve the status and details of a table data import job.
*
*
* @param describeTableDataImportJobRequest
* @return Result of the DescribeTableDataImportJob operation returned by the service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action. Check that the workbook is owned by you and
* your IAM policy allows access to the resource in the request.
* @throws InternalServerException
* There were unexpected errors from the server.
* @throws ResourceNotFoundException
* A Workbook, Table, App, Screen or Screen Automation was not found with the given ID.
* @throws ServiceUnavailableException
* Remote service is unreachable.
* @throws ThrottlingException
* Tps(transactions per second) rate reached.
* @throws ValidationException
* Request is invalid. The message in the response contains details on why the request is invalid.
* @sample AmazonHoneycode.DescribeTableDataImportJob
* @see AWS API Documentation
*/
DescribeTableDataImportJobResult describeTableDataImportJob(DescribeTableDataImportJobRequest describeTableDataImportJobRequest);
/**
*
* The GetScreenData API allows retrieval of data from a screen in a Honeycode app. The API allows setting local
* variables in the screen to filter, sort or otherwise affect what will be displayed on the screen.
*
*
* @param getScreenDataRequest
* @return Result of the GetScreenData operation returned by the service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action. Check that the workbook is owned by you and
* your IAM policy allows access to the resource in the request.
* @throws InternalServerException
* There were unexpected errors from the server.
* @throws RequestTimeoutException
* The request timed out.
* @throws ResourceNotFoundException
* A Workbook, Table, App, Screen or Screen Automation was not found with the given ID.
* @throws ServiceUnavailableException
* Remote service is unreachable.
* @throws ThrottlingException
* Tps(transactions per second) rate reached.
* @throws ValidationException
* Request is invalid. The message in the response contains details on why the request is invalid.
* @sample AmazonHoneycode.GetScreenData
* @see AWS API
* Documentation
*/
GetScreenDataResult getScreenData(GetScreenDataRequest getScreenDataRequest);
/**
*
* The InvokeScreenAutomation API allows invoking an action defined in a screen in a Honeycode app. The API allows
* setting local variables, which can then be used in the automation being invoked. This allows automating the
* Honeycode app interactions to write, update or delete data in the workbook.
*
*
* @param invokeScreenAutomationRequest
* @return Result of the InvokeScreenAutomation operation returned by the service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action. Check that the workbook is owned by you and
* your IAM policy allows access to the resource in the request.
* @throws InternalServerException
* There were unexpected errors from the server.
* @throws ResourceNotFoundException
* A Workbook, Table, App, Screen or Screen Automation was not found with the given ID.
* @throws ValidationException
* Request is invalid. The message in the response contains details on why the request is invalid.
* @throws ThrottlingException
* Tps(transactions per second) rate reached.
* @throws ServiceUnavailableException
* Remote service is unreachable.
* @throws AutomationExecutionException
* The automation execution did not end successfully.
* @throws AutomationExecutionTimeoutException
* The automation execution timed out.
* @throws RequestTimeoutException
* The request timed out.
* @sample AmazonHoneycode.InvokeScreenAutomation
* @see AWS API Documentation
*/
InvokeScreenAutomationResult invokeScreenAutomation(InvokeScreenAutomationRequest invokeScreenAutomationRequest);
/**
*
* The ListTableColumns API allows you to retrieve a list of all the columns in a table in a workbook.
*
*
* @param listTableColumnsRequest
* @return Result of the ListTableColumns operation returned by the service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action. Check that the workbook is owned by you and
* your IAM policy allows access to the resource in the request.
* @throws InternalServerException
* There were unexpected errors from the server.
* @throws RequestTimeoutException
* The request timed out.
* @throws ResourceNotFoundException
* A Workbook, Table, App, Screen or Screen Automation was not found with the given ID.
* @throws ServiceUnavailableException
* Remote service is unreachable.
* @throws ThrottlingException
* Tps(transactions per second) rate reached.
* @throws ValidationException
* Request is invalid. The message in the response contains details on why the request is invalid.
* @sample AmazonHoneycode.ListTableColumns
* @see AWS API
* Documentation
*/
ListTableColumnsResult listTableColumns(ListTableColumnsRequest listTableColumnsRequest);
/**
*
* The ListTableRows API allows you to retrieve a list of all the rows in a table in a workbook.
*
*
* @param listTableRowsRequest
* @return Result of the ListTableRows operation returned by the service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action. Check that the workbook is owned by you and
* your IAM policy allows access to the resource in the request.
* @throws InternalServerException
* There were unexpected errors from the server.
* @throws ResourceNotFoundException
* A Workbook, Table, App, Screen or Screen Automation was not found with the given ID.
* @throws ServiceUnavailableException
* Remote service is unreachable.
* @throws ValidationException
* Request is invalid. The message in the response contains details on why the request is invalid.
* @throws RequestTimeoutException
* The request timed out.
* @throws ThrottlingException
* Tps(transactions per second) rate reached.
* @sample AmazonHoneycode.ListTableRows
* @see AWS API
* Documentation
*/
ListTableRowsResult listTableRows(ListTableRowsRequest listTableRowsRequest);
/**
*
* The ListTables API allows you to retrieve a list of all the tables in a workbook.
*
*
* @param listTablesRequest
* @return Result of the ListTables operation returned by the service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action. Check that the workbook is owned by you and
* your IAM policy allows access to the resource in the request.
* @throws InternalServerException
* There were unexpected errors from the server.
* @throws RequestTimeoutException
* The request timed out.
* @throws ResourceNotFoundException
* A Workbook, Table, App, Screen or Screen Automation was not found with the given ID.
* @throws ServiceUnavailableException
* Remote service is unreachable.
* @throws ThrottlingException
* Tps(transactions per second) rate reached.
* @throws ValidationException
* Request is invalid. The message in the response contains details on why the request is invalid.
* @sample AmazonHoneycode.ListTables
* @see AWS API
* Documentation
*/
ListTablesResult listTables(ListTablesRequest listTablesRequest);
/**
*
* The QueryTableRows API allows you to use a filter formula to query for specific rows in a table.
*
*
* @param queryTableRowsRequest
* @return Result of the QueryTableRows operation returned by the service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action. Check that the workbook is owned by you and
* your IAM policy allows access to the resource in the request.
* @throws InternalServerException
* There were unexpected errors from the server.
* @throws RequestTimeoutException
* The request timed out.
* @throws ResourceNotFoundException
* A Workbook, Table, App, Screen or Screen Automation was not found with the given ID.
* @throws ServiceUnavailableException
* Remote service is unreachable.
* @throws ThrottlingException
* Tps(transactions per second) rate reached.
* @throws ValidationException
* Request is invalid. The message in the response contains details on why the request is invalid.
* @sample AmazonHoneycode.QueryTableRows
* @see AWS API
* Documentation
*/
QueryTableRowsResult queryTableRows(QueryTableRowsRequest queryTableRowsRequest);
/**
*
* The StartTableDataImportJob API allows you to start an import job on a table. This API will only return the id of
* the job that was started. To find out the status of the import request, you need to call the
* DescribeTableDataImportJob API.
*
*
* @param startTableDataImportJobRequest
* @return Result of the StartTableDataImportJob operation returned by the service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action. Check that the workbook is owned by you and
* your IAM policy allows access to the resource in the request.
* @throws InternalServerException
* There were unexpected errors from the server.
* @throws ResourceNotFoundException
* A Workbook, Table, App, Screen or Screen Automation was not found with the given ID.
* @throws ServiceUnavailableException
* Remote service is unreachable.
* @throws ThrottlingException
* Tps(transactions per second) rate reached.
* @throws ValidationException
* Request is invalid. The message in the response contains details on why the request is invalid.
* @sample AmazonHoneycode.StartTableDataImportJob
* @see AWS API Documentation
*/
StartTableDataImportJobResult startTableDataImportJob(StartTableDataImportJobRequest startTableDataImportJobRequest);
/**
* Shuts down this client object, releasing any resources that might be held open. This is an optional method, and
* callers are not expected to call it, but can if they want to explicitly release any open resources. Once a client
* has been shutdown, it should not be used to make any more requests.
*/
void shutdown();
/**
* Returns additional metadata for a previously executed successful request, typically used for debugging issues
* where a service isn't acting as expected. This data isn't considered part of the result data returned by an
* operation, so it's available through this separate, diagnostic interface.
*
* Response metadata is only cached for a limited period of time, so if you need to access this extra diagnostic
* information for an executed request, you should use this method to retrieve it as soon as possible after
* executing a request.
*
* @param request
* The originally executed request.
*
* @return The response metadata for the specified request, or null if none is available.
*/
ResponseMetadata getCachedResponseMetadata(AmazonWebServiceRequest request);
}