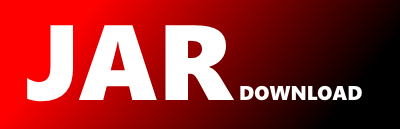
com.amazonaws.services.honeycode.model.InvokeScreenAutomationRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-honeycode Show documentation
/*
* Copyright 2017-2022 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.honeycode.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* @see AWS
* API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class InvokeScreenAutomationRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* The ID of the workbook that contains the screen automation.
*
*/
private String workbookId;
/**
*
* The ID of the app that contains the screen automation.
*
*/
private String appId;
/**
*
* The ID of the screen that contains the screen automation.
*
*/
private String screenId;
/**
*
* The ID of the automation action to be performed.
*
*/
private String screenAutomationId;
/**
*
* Variables are specified as a map where the key is the name of the variable as defined on the screen. The value is
* an object which currently has only one property, rawValue, which holds the value of the variable to be passed to
* the screen. Any variables defined in a screen are required to be passed in the call.
*
*/
private java.util.Map variables;
/**
*
* The row ID for the automation if the automation is defined inside a block with source or list.
*
*/
private String rowId;
/**
*
* The request token for performing the automation action. Request tokens help to identify duplicate requests. If a
* call times out or fails due to a transient error like a failed network connection, you can retry the call with
* the same request token. The service ensures that if the first call using that request token is successfully
* performed, the second call will return the response of the previous call rather than performing the action again.
*
*
* Note that request tokens are valid only for a few minutes. You cannot use request tokens to dedupe requests
* spanning hours or days.
*
*/
private String clientRequestToken;
/**
*
* The ID of the workbook that contains the screen automation.
*
*
* @param workbookId
* The ID of the workbook that contains the screen automation.
*/
public void setWorkbookId(String workbookId) {
this.workbookId = workbookId;
}
/**
*
* The ID of the workbook that contains the screen automation.
*
*
* @return The ID of the workbook that contains the screen automation.
*/
public String getWorkbookId() {
return this.workbookId;
}
/**
*
* The ID of the workbook that contains the screen automation.
*
*
* @param workbookId
* The ID of the workbook that contains the screen automation.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public InvokeScreenAutomationRequest withWorkbookId(String workbookId) {
setWorkbookId(workbookId);
return this;
}
/**
*
* The ID of the app that contains the screen automation.
*
*
* @param appId
* The ID of the app that contains the screen automation.
*/
public void setAppId(String appId) {
this.appId = appId;
}
/**
*
* The ID of the app that contains the screen automation.
*
*
* @return The ID of the app that contains the screen automation.
*/
public String getAppId() {
return this.appId;
}
/**
*
* The ID of the app that contains the screen automation.
*
*
* @param appId
* The ID of the app that contains the screen automation.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public InvokeScreenAutomationRequest withAppId(String appId) {
setAppId(appId);
return this;
}
/**
*
* The ID of the screen that contains the screen automation.
*
*
* @param screenId
* The ID of the screen that contains the screen automation.
*/
public void setScreenId(String screenId) {
this.screenId = screenId;
}
/**
*
* The ID of the screen that contains the screen automation.
*
*
* @return The ID of the screen that contains the screen automation.
*/
public String getScreenId() {
return this.screenId;
}
/**
*
* The ID of the screen that contains the screen automation.
*
*
* @param screenId
* The ID of the screen that contains the screen automation.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public InvokeScreenAutomationRequest withScreenId(String screenId) {
setScreenId(screenId);
return this;
}
/**
*
* The ID of the automation action to be performed.
*
*
* @param screenAutomationId
* The ID of the automation action to be performed.
*/
public void setScreenAutomationId(String screenAutomationId) {
this.screenAutomationId = screenAutomationId;
}
/**
*
* The ID of the automation action to be performed.
*
*
* @return The ID of the automation action to be performed.
*/
public String getScreenAutomationId() {
return this.screenAutomationId;
}
/**
*
* The ID of the automation action to be performed.
*
*
* @param screenAutomationId
* The ID of the automation action to be performed.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public InvokeScreenAutomationRequest withScreenAutomationId(String screenAutomationId) {
setScreenAutomationId(screenAutomationId);
return this;
}
/**
*
* Variables are specified as a map where the key is the name of the variable as defined on the screen. The value is
* an object which currently has only one property, rawValue, which holds the value of the variable to be passed to
* the screen. Any variables defined in a screen are required to be passed in the call.
*
*
* @return Variables are specified as a map where the key is the name of the variable as defined on the screen. The
* value is an object which currently has only one property, rawValue, which holds the value of the variable
* to be passed to the screen. Any variables defined in a screen are required to be passed in the call.
*/
public java.util.Map getVariables() {
return variables;
}
/**
*
* Variables are specified as a map where the key is the name of the variable as defined on the screen. The value is
* an object which currently has only one property, rawValue, which holds the value of the variable to be passed to
* the screen. Any variables defined in a screen are required to be passed in the call.
*
*
* @param variables
* Variables are specified as a map where the key is the name of the variable as defined on the screen. The
* value is an object which currently has only one property, rawValue, which holds the value of the variable
* to be passed to the screen. Any variables defined in a screen are required to be passed in the call.
*/
public void setVariables(java.util.Map variables) {
this.variables = variables;
}
/**
*
* Variables are specified as a map where the key is the name of the variable as defined on the screen. The value is
* an object which currently has only one property, rawValue, which holds the value of the variable to be passed to
* the screen. Any variables defined in a screen are required to be passed in the call.
*
*
* @param variables
* Variables are specified as a map where the key is the name of the variable as defined on the screen. The
* value is an object which currently has only one property, rawValue, which holds the value of the variable
* to be passed to the screen. Any variables defined in a screen are required to be passed in the call.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public InvokeScreenAutomationRequest withVariables(java.util.Map variables) {
setVariables(variables);
return this;
}
/**
* Add a single Variables entry
*
* @see InvokeScreenAutomationRequest#withVariables
* @returns a reference to this object so that method calls can be chained together.
*/
public InvokeScreenAutomationRequest addVariablesEntry(String key, VariableValue value) {
if (null == this.variables) {
this.variables = new java.util.HashMap();
}
if (this.variables.containsKey(key))
throw new IllegalArgumentException("Duplicated keys (" + key.toString() + ") are provided.");
this.variables.put(key, value);
return this;
}
/**
* Removes all the entries added into Variables.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public InvokeScreenAutomationRequest clearVariablesEntries() {
this.variables = null;
return this;
}
/**
*
* The row ID for the automation if the automation is defined inside a block with source or list.
*
*
* @param rowId
* The row ID for the automation if the automation is defined inside a block with source or list.
*/
public void setRowId(String rowId) {
this.rowId = rowId;
}
/**
*
* The row ID for the automation if the automation is defined inside a block with source or list.
*
*
* @return The row ID for the automation if the automation is defined inside a block with source or list.
*/
public String getRowId() {
return this.rowId;
}
/**
*
* The row ID for the automation if the automation is defined inside a block with source or list.
*
*
* @param rowId
* The row ID for the automation if the automation is defined inside a block with source or list.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public InvokeScreenAutomationRequest withRowId(String rowId) {
setRowId(rowId);
return this;
}
/**
*
* The request token for performing the automation action. Request tokens help to identify duplicate requests. If a
* call times out or fails due to a transient error like a failed network connection, you can retry the call with
* the same request token. The service ensures that if the first call using that request token is successfully
* performed, the second call will return the response of the previous call rather than performing the action again.
*
*
* Note that request tokens are valid only for a few minutes. You cannot use request tokens to dedupe requests
* spanning hours or days.
*
*
* @param clientRequestToken
* The request token for performing the automation action. Request tokens help to identify duplicate
* requests. If a call times out or fails due to a transient error like a failed network connection, you can
* retry the call with the same request token. The service ensures that if the first call using that request
* token is successfully performed, the second call will return the response of the previous call rather than
* performing the action again.
*
* Note that request tokens are valid only for a few minutes. You cannot use request tokens to dedupe
* requests spanning hours or days.
*/
public void setClientRequestToken(String clientRequestToken) {
this.clientRequestToken = clientRequestToken;
}
/**
*
* The request token for performing the automation action. Request tokens help to identify duplicate requests. If a
* call times out or fails due to a transient error like a failed network connection, you can retry the call with
* the same request token. The service ensures that if the first call using that request token is successfully
* performed, the second call will return the response of the previous call rather than performing the action again.
*
*
* Note that request tokens are valid only for a few minutes. You cannot use request tokens to dedupe requests
* spanning hours or days.
*
*
* @return The request token for performing the automation action. Request tokens help to identify duplicate
* requests. If a call times out or fails due to a transient error like a failed network connection, you can
* retry the call with the same request token. The service ensures that if the first call using that request
* token is successfully performed, the second call will return the response of the previous call rather
* than performing the action again.
*
* Note that request tokens are valid only for a few minutes. You cannot use request tokens to dedupe
* requests spanning hours or days.
*/
public String getClientRequestToken() {
return this.clientRequestToken;
}
/**
*
* The request token for performing the automation action. Request tokens help to identify duplicate requests. If a
* call times out or fails due to a transient error like a failed network connection, you can retry the call with
* the same request token. The service ensures that if the first call using that request token is successfully
* performed, the second call will return the response of the previous call rather than performing the action again.
*
*
* Note that request tokens are valid only for a few minutes. You cannot use request tokens to dedupe requests
* spanning hours or days.
*
*
* @param clientRequestToken
* The request token for performing the automation action. Request tokens help to identify duplicate
* requests. If a call times out or fails due to a transient error like a failed network connection, you can
* retry the call with the same request token. The service ensures that if the first call using that request
* token is successfully performed, the second call will return the response of the previous call rather than
* performing the action again.
*
* Note that request tokens are valid only for a few minutes. You cannot use request tokens to dedupe
* requests spanning hours or days.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public InvokeScreenAutomationRequest withClientRequestToken(String clientRequestToken) {
setClientRequestToken(clientRequestToken);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getWorkbookId() != null)
sb.append("WorkbookId: ").append(getWorkbookId()).append(",");
if (getAppId() != null)
sb.append("AppId: ").append(getAppId()).append(",");
if (getScreenId() != null)
sb.append("ScreenId: ").append(getScreenId()).append(",");
if (getScreenAutomationId() != null)
sb.append("ScreenAutomationId: ").append(getScreenAutomationId()).append(",");
if (getVariables() != null)
sb.append("Variables: ").append("***Sensitive Data Redacted***").append(",");
if (getRowId() != null)
sb.append("RowId: ").append(getRowId()).append(",");
if (getClientRequestToken() != null)
sb.append("ClientRequestToken: ").append(getClientRequestToken());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof InvokeScreenAutomationRequest == false)
return false;
InvokeScreenAutomationRequest other = (InvokeScreenAutomationRequest) obj;
if (other.getWorkbookId() == null ^ this.getWorkbookId() == null)
return false;
if (other.getWorkbookId() != null && other.getWorkbookId().equals(this.getWorkbookId()) == false)
return false;
if (other.getAppId() == null ^ this.getAppId() == null)
return false;
if (other.getAppId() != null && other.getAppId().equals(this.getAppId()) == false)
return false;
if (other.getScreenId() == null ^ this.getScreenId() == null)
return false;
if (other.getScreenId() != null && other.getScreenId().equals(this.getScreenId()) == false)
return false;
if (other.getScreenAutomationId() == null ^ this.getScreenAutomationId() == null)
return false;
if (other.getScreenAutomationId() != null && other.getScreenAutomationId().equals(this.getScreenAutomationId()) == false)
return false;
if (other.getVariables() == null ^ this.getVariables() == null)
return false;
if (other.getVariables() != null && other.getVariables().equals(this.getVariables()) == false)
return false;
if (other.getRowId() == null ^ this.getRowId() == null)
return false;
if (other.getRowId() != null && other.getRowId().equals(this.getRowId()) == false)
return false;
if (other.getClientRequestToken() == null ^ this.getClientRequestToken() == null)
return false;
if (other.getClientRequestToken() != null && other.getClientRequestToken().equals(this.getClientRequestToken()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getWorkbookId() == null) ? 0 : getWorkbookId().hashCode());
hashCode = prime * hashCode + ((getAppId() == null) ? 0 : getAppId().hashCode());
hashCode = prime * hashCode + ((getScreenId() == null) ? 0 : getScreenId().hashCode());
hashCode = prime * hashCode + ((getScreenAutomationId() == null) ? 0 : getScreenAutomationId().hashCode());
hashCode = prime * hashCode + ((getVariables() == null) ? 0 : getVariables().hashCode());
hashCode = prime * hashCode + ((getRowId() == null) ? 0 : getRowId().hashCode());
hashCode = prime * hashCode + ((getClientRequestToken() == null) ? 0 : getClientRequestToken().hashCode());
return hashCode;
}
@Override
public InvokeScreenAutomationRequest clone() {
return (InvokeScreenAutomationRequest) super.clone();
}
}