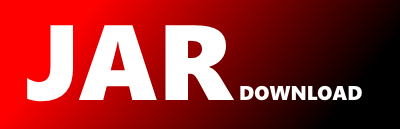
com.amazonaws.services.identitymanagement.AmazonIdentityManagementClient Maven / Gradle / Ivy
Show all versions of aws-java-sdk-iam Show documentation
/*
* Copyright 2011-2016 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.identitymanagement;
import org.w3c.dom.*;
import java.net.*;
import java.util.*;
import java.util.Map.Entry;
import org.apache.commons.logging.*;
import com.amazonaws.*;
import com.amazonaws.auth.*;
import com.amazonaws.handlers.*;
import com.amazonaws.http.*;
import com.amazonaws.internal.*;
import com.amazonaws.internal.auth.*;
import com.amazonaws.metrics.*;
import com.amazonaws.regions.*;
import com.amazonaws.transform.*;
import com.amazonaws.util.*;
import com.amazonaws.protocol.json.*;
import com.amazonaws.util.AWSRequestMetrics.Field;
import com.amazonaws.annotation.ThreadSafe;
import com.amazonaws.client.AwsSyncClientParams;
import com.amazonaws.services.identitymanagement.waiters.AmazonIdentityManagementWaiters;
import com.amazonaws.AmazonServiceException;
import com.amazonaws.services.identitymanagement.model.*;
import com.amazonaws.services.identitymanagement.model.transform.*;
/**
* Client for accessing IAM. All service calls made using this client are blocking, and will not return until the
* service call completes.
*
* AWS Identity and Access Management
*
* AWS Identity and Access Management (IAM) is a web service that you can use to manage users and user permissions under
* your AWS account. This guide provides descriptions of IAM actions that you can call programmatically. For general
* information about IAM, see AWS Identity and Access Management (IAM). For the
* user guide for IAM, see Using IAM.
*
*
*
* AWS provides SDKs that consist of libraries and sample code for various programming languages and platforms (Java,
* Ruby, .NET, iOS, Android, etc.). The SDKs provide a convenient way to create programmatic access to IAM and AWS. For
* example, the SDKs take care of tasks such as cryptographically signing requests (see below), managing errors, and
* retrying requests automatically. For information about the AWS SDKs, including how to download and install them, see
* the Tools for Amazon Web Services page.
*
*
*
* We recommend that you use the AWS SDKs to make programmatic API calls to IAM. However, you can also use the IAM Query
* API to make direct calls to the IAM web service. To learn more about the IAM Query API, see Making Query Requests in the
* Using IAM guide. IAM supports GET and POST requests for all actions. That is, the API does not require you to
* use GET for some actions and POST for others. However, GET requests are subject to the limitation size of a URL.
* Therefore, for operations that require larger sizes, use a POST request.
*
*
* Signing Requests
*
*
* Requests must be signed using an access key ID and a secret access key. We strongly recommend that you do not use
* your AWS account access key ID and secret access key for everyday work with IAM. You can use the access key ID and
* secret access key for an IAM user or you can use the AWS Security Token Service to generate temporary security
* credentials and use those to sign requests.
*
*
* To sign requests, we recommend that you use Signature Version 4. If you have an
* existing application that uses Signature Version 2, you do not have to update it to use Signature Version 4. However,
* some operations now require Signature Version 4. The documentation for operations that require version 4 indicate
* this requirement.
*
*
* Additional Resources
*
*
* For more information, see the following:
*
*
* -
*
* AWS Security Credentials.
* This topic provides general information about the types of credentials used for accessing AWS.
*
*
* -
*
* IAM Best Practices. This topic
* presents a list of suggestions for using the IAM service to help secure your AWS resources.
*
*
* -
*
* Signing AWS API Requests.
* This set of topics walk you through the process of signing a request using an access key ID and secret access key.
*
*
*
*/
@ThreadSafe
public class AmazonIdentityManagementClient extends AmazonWebServiceClient implements AmazonIdentityManagement {
/** Provider for AWS credentials. */
private final AWSCredentialsProvider awsCredentialsProvider;
private static final Log log = LogFactory.getLog(AmazonIdentityManagement.class);
/** Default signing name for the service. */
private static final String DEFAULT_SIGNING_NAME = "iam";
private volatile AmazonIdentityManagementWaiters waiters;
/** Client configuration factory providing ClientConfigurations tailored to this client */
protected static final ClientConfigurationFactory configFactory = new ClientConfigurationFactory();
/**
* List of exception unmarshallers for all modeled exceptions
*/
protected final List> exceptionUnmarshallers = new ArrayList>();
/**
* Constructs a new client to invoke service methods on IAM. A credentials provider chain will be used that searches
* for credentials in this order:
*
* - Environment Variables - AWS_ACCESS_KEY_ID and AWS_SECRET_KEY
* - Java System Properties - aws.accessKeyId and aws.secretKey
* - Instance profile credentials delivered through the Amazon EC2 metadata service
*
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @see DefaultAWSCredentialsProviderChain
*/
public AmazonIdentityManagementClient() {
this(DefaultAWSCredentialsProviderChain.getInstance(), configFactory.getConfig());
}
/**
* Constructs a new client to invoke service methods on IAM. A credentials provider chain will be used that searches
* for credentials in this order:
*
* - Environment Variables - AWS_ACCESS_KEY_ID and AWS_SECRET_KEY
* - Java System Properties - aws.accessKeyId and aws.secretKey
* - Instance profile credentials delivered through the Amazon EC2 metadata service
*
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param clientConfiguration
* The client configuration options controlling how this client connects to IAM (ex: proxy settings, retry
* counts, etc.).
*
* @see DefaultAWSCredentialsProviderChain
*/
public AmazonIdentityManagementClient(ClientConfiguration clientConfiguration) {
this(DefaultAWSCredentialsProviderChain.getInstance(), clientConfiguration);
}
/**
* Constructs a new client to invoke service methods on IAM using the specified AWS account credentials.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param awsCredentials
* The AWS credentials (access key ID and secret key) to use when authenticating with AWS services.
*/
public AmazonIdentityManagementClient(AWSCredentials awsCredentials) {
this(awsCredentials, configFactory.getConfig());
}
/**
* Constructs a new client to invoke service methods on IAM using the specified AWS account credentials and client
* configuration options.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param awsCredentials
* The AWS credentials (access key ID and secret key) to use when authenticating with AWS services.
* @param clientConfiguration
* The client configuration options controlling how this client connects to IAM (ex: proxy settings, retry
* counts, etc.).
*/
public AmazonIdentityManagementClient(AWSCredentials awsCredentials, ClientConfiguration clientConfiguration) {
super(clientConfiguration);
this.awsCredentialsProvider = new StaticCredentialsProvider(awsCredentials);
init();
}
/**
* Constructs a new client to invoke service methods on IAM using the specified AWS account credentials provider.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param awsCredentialsProvider
* The AWS credentials provider which will provide credentials to authenticate requests with AWS services.
*/
public AmazonIdentityManagementClient(AWSCredentialsProvider awsCredentialsProvider) {
this(awsCredentialsProvider, configFactory.getConfig());
}
/**
* Constructs a new client to invoke service methods on IAM using the specified AWS account credentials provider and
* client configuration options.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param awsCredentialsProvider
* The AWS credentials provider which will provide credentials to authenticate requests with AWS services.
* @param clientConfiguration
* The client configuration options controlling how this client connects to IAM (ex: proxy settings, retry
* counts, etc.).
*/
public AmazonIdentityManagementClient(AWSCredentialsProvider awsCredentialsProvider, ClientConfiguration clientConfiguration) {
this(awsCredentialsProvider, clientConfiguration, null);
}
/**
* Constructs a new client to invoke service methods on IAM using the specified AWS account credentials provider,
* client configuration options, and request metric collector.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param awsCredentialsProvider
* The AWS credentials provider which will provide credentials to authenticate requests with AWS services.
* @param clientConfiguration
* The client configuration options controlling how this client connects to IAM (ex: proxy settings, retry
* counts, etc.).
* @param requestMetricCollector
* optional request metric collector
*/
public AmazonIdentityManagementClient(AWSCredentialsProvider awsCredentialsProvider, ClientConfiguration clientConfiguration,
RequestMetricCollector requestMetricCollector) {
super(clientConfiguration, requestMetricCollector);
this.awsCredentialsProvider = awsCredentialsProvider;
init();
}
/**
* Constructs a new client to invoke service methods on IAM using the specified parameters.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param clientParams
* Object providing client parameters.
*/
AmazonIdentityManagementClient(AwsSyncClientParams clientParams) {
super(clientParams);
this.awsCredentialsProvider = clientParams.getCredentialsProvider();
init();
}
private void init() {
exceptionUnmarshallers.add(new MalformedPolicyDocumentExceptionUnmarshaller());
exceptionUnmarshallers.add(new DeleteConflictExceptionUnmarshaller());
exceptionUnmarshallers.add(new InvalidCertificateExceptionUnmarshaller());
exceptionUnmarshallers.add(new PasswordPolicyViolationExceptionUnmarshaller());
exceptionUnmarshallers.add(new CredentialReportExpiredExceptionUnmarshaller());
exceptionUnmarshallers.add(new LimitExceededExceptionUnmarshaller());
exceptionUnmarshallers.add(new InvalidUserTypeExceptionUnmarshaller());
exceptionUnmarshallers.add(new NoSuchEntityExceptionUnmarshaller());
exceptionUnmarshallers.add(new EntityTemporarilyUnmodifiableExceptionUnmarshaller());
exceptionUnmarshallers.add(new DuplicateSSHPublicKeyExceptionUnmarshaller());
exceptionUnmarshallers.add(new DuplicateCertificateExceptionUnmarshaller());
exceptionUnmarshallers.add(new KeyPairMismatchExceptionUnmarshaller());
exceptionUnmarshallers.add(new CredentialReportNotReadyExceptionUnmarshaller());
exceptionUnmarshallers.add(new EntityAlreadyExistsExceptionUnmarshaller());
exceptionUnmarshallers.add(new ServiceFailureExceptionUnmarshaller());
exceptionUnmarshallers.add(new InvalidPublicKeyExceptionUnmarshaller());
exceptionUnmarshallers.add(new PolicyEvaluationExceptionUnmarshaller());
exceptionUnmarshallers.add(new InvalidAuthenticationCodeExceptionUnmarshaller());
exceptionUnmarshallers.add(new InvalidInputExceptionUnmarshaller());
exceptionUnmarshallers.add(new CredentialReportNotPresentExceptionUnmarshaller());
exceptionUnmarshallers.add(new UnrecognizedPublicKeyEncodingExceptionUnmarshaller());
exceptionUnmarshallers.add(new MalformedCertificateExceptionUnmarshaller());
exceptionUnmarshallers.add(new StandardErrorUnmarshaller(com.amazonaws.services.identitymanagement.model.AmazonIdentityManagementException.class));
setServiceNameIntern(DEFAULT_SIGNING_NAME);
setEndpointPrefix(ENDPOINT_PREFIX);
// calling this.setEndPoint(...) will also modify the signer accordingly
this.setEndpoint("iam.amazonaws.com");
HandlerChainFactory chainFactory = new HandlerChainFactory();
requestHandler2s.addAll(chainFactory.newRequestHandlerChain("/com/amazonaws/services/identitymanagement/request.handlers"));
requestHandler2s.addAll(chainFactory.newRequestHandler2Chain("/com/amazonaws/services/identitymanagement/request.handler2s"));
requestHandler2s.addAll(chainFactory.getGlobalHandlers());
}
/**
*
* Adds a new client ID (also known as audience) to the list of client IDs already registered for the specified IAM
* OpenID Connect (OIDC) provider resource.
*
*
* This action is idempotent; it does not fail or return an error if you add an existing client ID to the provider.
*
*
* @param addClientIDToOpenIDConnectProviderRequest
* @return Result of the AddClientIDToOpenIDConnectProvider operation returned by the service.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @throws NoSuchEntityException
* The request was rejected because it referenced an entity that does not exist. The error message describes
* the entity.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current AWS account limits.
* The error message describes the limit exceeded.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @sample AmazonIdentityManagement.AddClientIDToOpenIDConnectProvider
*/
@Override
public AddClientIDToOpenIDConnectProviderResult addClientIDToOpenIDConnectProvider(
AddClientIDToOpenIDConnectProviderRequest addClientIDToOpenIDConnectProviderRequest) {
ExecutionContext executionContext = createExecutionContext(addClientIDToOpenIDConnectProviderRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new AddClientIDToOpenIDConnectProviderRequestMarshaller()
.marshall(super.beforeMarshalling(addClientIDToOpenIDConnectProviderRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new AddClientIDToOpenIDConnectProviderResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Adds the specified IAM role to the specified instance profile.
*
*
*
* The caller of this API must be granted the PassRole
permission on the IAM role by a permission
* policy.
*
*
*
* For more information about roles, go to Working with Roles. For more
* information about instance profiles, go to About Instance Profiles.
*
*
* @param addRoleToInstanceProfileRequest
* @return Result of the AddRoleToInstanceProfile operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced an entity that does not exist. The error message describes
* the entity.
* @throws EntityAlreadyExistsException
* The request was rejected because it attempted to create a resource that already exists.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current AWS account limits.
* The error message describes the limit exceeded.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @sample AmazonIdentityManagement.AddRoleToInstanceProfile
*/
@Override
public AddRoleToInstanceProfileResult addRoleToInstanceProfile(AddRoleToInstanceProfileRequest addRoleToInstanceProfileRequest) {
ExecutionContext executionContext = createExecutionContext(addRoleToInstanceProfileRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new AddRoleToInstanceProfileRequestMarshaller().marshall(super.beforeMarshalling(addRoleToInstanceProfileRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new AddRoleToInstanceProfileResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Adds the specified user to the specified group.
*
*
* @param addUserToGroupRequest
* @return Result of the AddUserToGroup operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced an entity that does not exist. The error message describes
* the entity.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current AWS account limits.
* The error message describes the limit exceeded.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @sample AmazonIdentityManagement.AddUserToGroup
*/
@Override
public AddUserToGroupResult addUserToGroup(AddUserToGroupRequest addUserToGroupRequest) {
ExecutionContext executionContext = createExecutionContext(addUserToGroupRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new AddUserToGroupRequestMarshaller().marshall(super.beforeMarshalling(addUserToGroupRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new AddUserToGroupResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Attaches the specified managed policy to the specified IAM group.
*
*
* You use this API to attach a managed policy to a group. To embed an inline policy in a group, use
* PutGroupPolicy.
*
*
* For more information about policies, see Managed Policies and
* Inline Policies in the IAM User Guide.
*
*
* @param attachGroupPolicyRequest
* @return Result of the AttachGroupPolicy operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced an entity that does not exist. The error message describes
* the entity.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current AWS account limits.
* The error message describes the limit exceeded.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @sample AmazonIdentityManagement.AttachGroupPolicy
*/
@Override
public AttachGroupPolicyResult attachGroupPolicy(AttachGroupPolicyRequest attachGroupPolicyRequest) {
ExecutionContext executionContext = createExecutionContext(attachGroupPolicyRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new AttachGroupPolicyRequestMarshaller().marshall(super.beforeMarshalling(attachGroupPolicyRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new AttachGroupPolicyResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Attaches the specified managed policy to the specified IAM role.
*
*
* When you attach a managed policy to a role, the managed policy becomes part of the role's permission (access)
* policy. You cannot use a managed policy as the role's trust policy. The role's trust policy is created at the
* same time as the role, using CreateRole. You can update a role's trust policy using
* UpdateAssumeRolePolicy.
*
*
* Use this API to attach a managed policy to a role. To embed an inline policy in a role, use
* PutRolePolicy. For more information about policies, see Managed Policies and
* Inline Policies in the IAM User Guide.
*
*
* @param attachRolePolicyRequest
* @return Result of the AttachRolePolicy operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced an entity that does not exist. The error message describes
* the entity.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current AWS account limits.
* The error message describes the limit exceeded.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @sample AmazonIdentityManagement.AttachRolePolicy
*/
@Override
public AttachRolePolicyResult attachRolePolicy(AttachRolePolicyRequest attachRolePolicyRequest) {
ExecutionContext executionContext = createExecutionContext(attachRolePolicyRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new AttachRolePolicyRequestMarshaller().marshall(super.beforeMarshalling(attachRolePolicyRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new AttachRolePolicyResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Attaches the specified managed policy to the specified user.
*
*
* You use this API to attach a managed policy to a user. To embed an inline policy in a user, use
* PutUserPolicy.
*
*
* For more information about policies, see Managed Policies and
* Inline Policies in the IAM User Guide.
*
*
* @param attachUserPolicyRequest
* @return Result of the AttachUserPolicy operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced an entity that does not exist. The error message describes
* the entity.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current AWS account limits.
* The error message describes the limit exceeded.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @sample AmazonIdentityManagement.AttachUserPolicy
*/
@Override
public AttachUserPolicyResult attachUserPolicy(AttachUserPolicyRequest attachUserPolicyRequest) {
ExecutionContext executionContext = createExecutionContext(attachUserPolicyRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new AttachUserPolicyRequestMarshaller().marshall(super.beforeMarshalling(attachUserPolicyRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new AttachUserPolicyResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Changes the password of the IAM user who is calling this action. The root account password is not affected by
* this action.
*
*
* To change the password for a different user, see UpdateLoginProfile. For more information about modifying
* passwords, see Managing
* Passwords in the IAM User Guide.
*
*
* @param changePasswordRequest
* @return Result of the ChangePassword operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced an entity that does not exist. The error message describes
* the entity.
* @throws InvalidUserTypeException
* The request was rejected because the type of user for the transaction was incorrect.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current AWS account limits.
* The error message describes the limit exceeded.
* @throws EntityTemporarilyUnmodifiableException
* The request was rejected because it referenced an entity that is temporarily unmodifiable, such as a user
* name that was deleted and then recreated. The error indicates that the request is likely to succeed if
* you try again after waiting several minutes. The error message describes the entity.
* @throws PasswordPolicyViolationException
* The request was rejected because the provided password did not meet the requirements imposed by the
* account password policy.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @sample AmazonIdentityManagement.ChangePassword
*/
@Override
public ChangePasswordResult changePassword(ChangePasswordRequest changePasswordRequest) {
ExecutionContext executionContext = createExecutionContext(changePasswordRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ChangePasswordRequestMarshaller().marshall(super.beforeMarshalling(changePasswordRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new ChangePasswordResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a new AWS secret access key and corresponding AWS access key ID for the specified user. The default
* status for new keys is Active
.
*
*
* If you do not specify a user name, IAM determines the user name implicitly based on the AWS access key ID signing
* the request. Because this action works for access keys under the AWS account, you can use this action to manage
* root credentials even if the AWS account has no associated users.
*
*
* For information about limits on the number of keys you can create, see Limitations on IAM Entities
* in the IAM User Guide.
*
*
*
* To ensure the security of your AWS account, the secret access key is accessible only during key and user
* creation. You must save the key (for example, in a text file) if you want to be able to access it again. If a
* secret key is lost, you can delete the access keys for the associated user and then create new keys.
*
*
*
* @param createAccessKeyRequest
* @return Result of the CreateAccessKey operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced an entity that does not exist. The error message describes
* the entity.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current AWS account limits.
* The error message describes the limit exceeded.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @sample AmazonIdentityManagement.CreateAccessKey
*/
@Override
public CreateAccessKeyResult createAccessKey(CreateAccessKeyRequest createAccessKeyRequest) {
ExecutionContext executionContext = createExecutionContext(createAccessKeyRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateAccessKeyRequestMarshaller().marshall(super.beforeMarshalling(createAccessKeyRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new CreateAccessKeyResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
@Override
public CreateAccessKeyResult createAccessKey() {
return createAccessKey(new CreateAccessKeyRequest());
}
/**
*
* Creates an alias for your AWS account. For information about using an AWS account alias, see Using an Alias for Your AWS Account
* ID in the IAM User Guide.
*
*
* @param createAccountAliasRequest
* @return Result of the CreateAccountAlias operation returned by the service.
* @throws EntityAlreadyExistsException
* The request was rejected because it attempted to create a resource that already exists.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current AWS account limits.
* The error message describes the limit exceeded.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @sample AmazonIdentityManagement.CreateAccountAlias
*/
@Override
public CreateAccountAliasResult createAccountAlias(CreateAccountAliasRequest createAccountAliasRequest) {
ExecutionContext executionContext = createExecutionContext(createAccountAliasRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateAccountAliasRequestMarshaller().marshall(super.beforeMarshalling(createAccountAliasRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new CreateAccountAliasResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a new group.
*
*
* For information about the number of groups you can create, see Limitations on IAM Entities
* in the IAM User Guide.
*
*
* @param createGroupRequest
* @return Result of the CreateGroup operation returned by the service.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current AWS account limits.
* The error message describes the limit exceeded.
* @throws EntityAlreadyExistsException
* The request was rejected because it attempted to create a resource that already exists.
* @throws NoSuchEntityException
* The request was rejected because it referenced an entity that does not exist. The error message describes
* the entity.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @sample AmazonIdentityManagement.CreateGroup
*/
@Override
public CreateGroupResult createGroup(CreateGroupRequest createGroupRequest) {
ExecutionContext executionContext = createExecutionContext(createGroupRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateGroupRequestMarshaller().marshall(super.beforeMarshalling(createGroupRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(new CreateGroupResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a new instance profile. For information about instance profiles, go to About Instance Profiles.
*
*
* For information about the number of instance profiles you can create, see Limitations on IAM Entities
* in the IAM User Guide.
*
*
* @param createInstanceProfileRequest
* @return Result of the CreateInstanceProfile operation returned by the service.
* @throws EntityAlreadyExistsException
* The request was rejected because it attempted to create a resource that already exists.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current AWS account limits.
* The error message describes the limit exceeded.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @sample AmazonIdentityManagement.CreateInstanceProfile
*/
@Override
public CreateInstanceProfileResult createInstanceProfile(CreateInstanceProfileRequest createInstanceProfileRequest) {
ExecutionContext executionContext = createExecutionContext(createInstanceProfileRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateInstanceProfileRequestMarshaller().marshall(super.beforeMarshalling(createInstanceProfileRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new CreateInstanceProfileResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a password for the specified user, giving the user the ability to access AWS services through the AWS
* Management Console. For more information about managing passwords, see Managing Passwords in the
* IAM User Guide.
*
*
* @param createLoginProfileRequest
* @return Result of the CreateLoginProfile operation returned by the service.
* @throws EntityAlreadyExistsException
* The request was rejected because it attempted to create a resource that already exists.
* @throws NoSuchEntityException
* The request was rejected because it referenced an entity that does not exist. The error message describes
* the entity.
* @throws PasswordPolicyViolationException
* The request was rejected because the provided password did not meet the requirements imposed by the
* account password policy.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current AWS account limits.
* The error message describes the limit exceeded.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @sample AmazonIdentityManagement.CreateLoginProfile
*/
@Override
public CreateLoginProfileResult createLoginProfile(CreateLoginProfileRequest createLoginProfileRequest) {
ExecutionContext executionContext = createExecutionContext(createLoginProfileRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateLoginProfileRequestMarshaller().marshall(super.beforeMarshalling(createLoginProfileRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new CreateLoginProfileResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates an IAM entity to describe an identity provider (IdP) that supports OpenID Connect (OIDC).
*
*
* The OIDC provider that you create with this operation can be used as a principal in a role's trust policy to
* establish a trust relationship between AWS and the OIDC provider.
*
*
* When you create the IAM OIDC provider, you specify the URL of the OIDC identity provider (IdP) to trust, a list
* of client IDs (also known as audiences) that identify the application or applications that are allowed to
* authenticate using the OIDC provider, and a list of thumbprints of the server certificate(s) that the IdP uses.
* You get all of this information from the OIDC IdP that you want to use for access to AWS.
*
*
*
* Because trust for the OIDC provider is ultimately derived from the IAM provider that this action creates, it is a
* best practice to limit access to the CreateOpenIDConnectProvider action to highly-privileged users.
*
*
*
* @param createOpenIDConnectProviderRequest
* @return Result of the CreateOpenIDConnectProvider operation returned by the service.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @throws EntityAlreadyExistsException
* The request was rejected because it attempted to create a resource that already exists.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current AWS account limits.
* The error message describes the limit exceeded.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @sample AmazonIdentityManagement.CreateOpenIDConnectProvider
*/
@Override
public CreateOpenIDConnectProviderResult createOpenIDConnectProvider(CreateOpenIDConnectProviderRequest createOpenIDConnectProviderRequest) {
ExecutionContext executionContext = createExecutionContext(createOpenIDConnectProviderRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateOpenIDConnectProviderRequestMarshaller().marshall(super.beforeMarshalling(createOpenIDConnectProviderRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new CreateOpenIDConnectProviderResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a new managed policy for your AWS account.
*
*
* This operation creates a policy version with a version identifier of v1
and sets v1 as the policy's
* default version. For more information about policy versions, see Versioning for Managed
* Policies in the IAM User Guide.
*
*
* For more information about managed policies in general, see Managed Policies and
* Inline Policies in the IAM User Guide.
*
*
* @param createPolicyRequest
* @return Result of the CreatePolicy operation returned by the service.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current AWS account limits.
* The error message describes the limit exceeded.
* @throws EntityAlreadyExistsException
* The request was rejected because it attempted to create a resource that already exists.
* @throws MalformedPolicyDocumentException
* The request was rejected because the policy document was malformed. The error message describes the
* specific error.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @sample AmazonIdentityManagement.CreatePolicy
*/
@Override
public CreatePolicyResult createPolicy(CreatePolicyRequest createPolicyRequest) {
ExecutionContext executionContext = createExecutionContext(createPolicyRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreatePolicyRequestMarshaller().marshall(super.beforeMarshalling(createPolicyRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(new CreatePolicyResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a new version of the specified managed policy. To update a managed policy, you create a new policy
* version. A managed policy can have up to five versions. If the policy has five versions, you must delete an
* existing version using DeletePolicyVersion before you create a new version.
*
*
* Optionally, you can set the new version as the policy's default version. The default version is the version that
* is in effect for the IAM users, groups, and roles to which the policy is attached.
*
*
* For more information about managed policy versions, see Versioning for Managed
* Policies in the IAM User Guide.
*
*
* @param createPolicyVersionRequest
* @return Result of the CreatePolicyVersion operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced an entity that does not exist. The error message describes
* the entity.
* @throws MalformedPolicyDocumentException
* The request was rejected because the policy document was malformed. The error message describes the
* specific error.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current AWS account limits.
* The error message describes the limit exceeded.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @sample AmazonIdentityManagement.CreatePolicyVersion
*/
@Override
public CreatePolicyVersionResult createPolicyVersion(CreatePolicyVersionRequest createPolicyVersionRequest) {
ExecutionContext executionContext = createExecutionContext(createPolicyVersionRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreatePolicyVersionRequestMarshaller().marshall(super.beforeMarshalling(createPolicyVersionRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new CreatePolicyVersionResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a new role for your AWS account. For more information about roles, go to Working with Roles. For
* information about limitations on role names and the number of roles you can create, go to Limitations on IAM Entities
* in the IAM User Guide.
*
*
* @param createRoleRequest
* @return Result of the CreateRole operation returned by the service.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current AWS account limits.
* The error message describes the limit exceeded.
* @throws EntityAlreadyExistsException
* The request was rejected because it attempted to create a resource that already exists.
* @throws MalformedPolicyDocumentException
* The request was rejected because the policy document was malformed. The error message describes the
* specific error.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @sample AmazonIdentityManagement.CreateRole
*/
@Override
public CreateRoleResult createRole(CreateRoleRequest createRoleRequest) {
ExecutionContext executionContext = createExecutionContext(createRoleRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateRoleRequestMarshaller().marshall(super.beforeMarshalling(createRoleRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(new CreateRoleResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates an IAM resource that describes an identity provider (IdP) that supports SAML 2.0.
*
*
* The SAML provider resource that you create with this operation can be used as a principal in an IAM role's trust
* policy to enable federated users who sign-in using the SAML IdP to assume the role. You can create an IAM role
* that supports Web-based single sign-on (SSO) to the AWS Management Console or one that supports API access to
* AWS.
*
*
* When you create the SAML provider resource, you upload an a SAML metadata document that you get from your IdP and
* that includes the issuer's name, expiration information, and keys that can be used to validate the SAML
* authentication response (assertions) that the IdP sends. You must generate the metadata document using the
* identity management software that is used as your organization's IdP.
*
*
*
* This operation requires Signature
* Version 4.
*
*
*
* For more information, see Enabling SAML
* 2.0 Federated Users to Access the AWS Management Console and About SAML 2.0-based
* Federation in the IAM User Guide.
*
*
* @param createSAMLProviderRequest
* @return Result of the CreateSAMLProvider operation returned by the service.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @throws EntityAlreadyExistsException
* The request was rejected because it attempted to create a resource that already exists.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current AWS account limits.
* The error message describes the limit exceeded.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @sample AmazonIdentityManagement.CreateSAMLProvider
*/
@Override
public CreateSAMLProviderResult createSAMLProvider(CreateSAMLProviderRequest createSAMLProviderRequest) {
ExecutionContext executionContext = createExecutionContext(createSAMLProviderRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateSAMLProviderRequestMarshaller().marshall(super.beforeMarshalling(createSAMLProviderRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new CreateSAMLProviderResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a new IAM user for your AWS account.
*
*
* For information about limitations on the number of IAM users you can create, see Limitations on IAM Entities
* in the IAM User Guide.
*
*
* @param createUserRequest
* @return Result of the CreateUser operation returned by the service.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current AWS account limits.
* The error message describes the limit exceeded.
* @throws EntityAlreadyExistsException
* The request was rejected because it attempted to create a resource that already exists.
* @throws NoSuchEntityException
* The request was rejected because it referenced an entity that does not exist. The error message describes
* the entity.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @sample AmazonIdentityManagement.CreateUser
*/
@Override
public CreateUserResult createUser(CreateUserRequest createUserRequest) {
ExecutionContext executionContext = createExecutionContext(createUserRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateUserRequestMarshaller().marshall(super.beforeMarshalling(createUserRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(new CreateUserResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a new virtual MFA device for the AWS account. After creating the virtual MFA, use EnableMFADevice
* to attach the MFA device to an IAM user. For more information about creating and working with virtual MFA
* devices, go to Using a Virtual
* MFA Device in the IAM User Guide.
*
*
* For information about limits on the number of MFA devices you can create, see Limitations on Entities in
* the IAM User Guide.
*
*
*
* The seed information contained in the QR code and the Base32 string should be treated like any other secret
* access information, such as your AWS access keys or your passwords. After you provision your virtual device, you
* should ensure that the information is destroyed following secure procedures.
*
*
*
* @param createVirtualMFADeviceRequest
* @return Result of the CreateVirtualMFADevice operation returned by the service.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current AWS account limits.
* The error message describes the limit exceeded.
* @throws EntityAlreadyExistsException
* The request was rejected because it attempted to create a resource that already exists.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @sample AmazonIdentityManagement.CreateVirtualMFADevice
*/
@Override
public CreateVirtualMFADeviceResult createVirtualMFADevice(CreateVirtualMFADeviceRequest createVirtualMFADeviceRequest) {
ExecutionContext executionContext = createExecutionContext(createVirtualMFADeviceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateVirtualMFADeviceRequestMarshaller().marshall(super.beforeMarshalling(createVirtualMFADeviceRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new CreateVirtualMFADeviceResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deactivates the specified MFA device and removes it from association with the user name for which it was
* originally enabled.
*
*
* For more information about creating and working with virtual MFA devices, go to Using a Virtual MFA Device in
* the IAM User Guide.
*
*
* @param deactivateMFADeviceRequest
* @return Result of the DeactivateMFADevice operation returned by the service.
* @throws EntityTemporarilyUnmodifiableException
* The request was rejected because it referenced an entity that is temporarily unmodifiable, such as a user
* name that was deleted and then recreated. The error indicates that the request is likely to succeed if
* you try again after waiting several minutes. The error message describes the entity.
* @throws NoSuchEntityException
* The request was rejected because it referenced an entity that does not exist. The error message describes
* the entity.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current AWS account limits.
* The error message describes the limit exceeded.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @sample AmazonIdentityManagement.DeactivateMFADevice
*/
@Override
public DeactivateMFADeviceResult deactivateMFADevice(DeactivateMFADeviceRequest deactivateMFADeviceRequest) {
ExecutionContext executionContext = createExecutionContext(deactivateMFADeviceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeactivateMFADeviceRequestMarshaller().marshall(super.beforeMarshalling(deactivateMFADeviceRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new DeactivateMFADeviceResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes the access key pair associated with the specified IAM user.
*
*
* If you do not specify a user name, IAM determines the user name implicitly based on the AWS access key ID signing
* the request. Because this action works for access keys under the AWS account, you can use this action to manage
* root credentials even if the AWS account has no associated users.
*
*
* @param deleteAccessKeyRequest
* @return Result of the DeleteAccessKey operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced an entity that does not exist. The error message describes
* the entity.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current AWS account limits.
* The error message describes the limit exceeded.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @sample AmazonIdentityManagement.DeleteAccessKey
*/
@Override
public DeleteAccessKeyResult deleteAccessKey(DeleteAccessKeyRequest deleteAccessKeyRequest) {
ExecutionContext executionContext = createExecutionContext(deleteAccessKeyRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteAccessKeyRequestMarshaller().marshall(super.beforeMarshalling(deleteAccessKeyRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new DeleteAccessKeyResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes the specified AWS account alias. For information about using an AWS account alias, see Using an Alias for Your AWS Account
* ID in the IAM User Guide.
*
*
* @param deleteAccountAliasRequest
* @return Result of the DeleteAccountAlias operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced an entity that does not exist. The error message describes
* the entity.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current AWS account limits.
* The error message describes the limit exceeded.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @sample AmazonIdentityManagement.DeleteAccountAlias
*/
@Override
public DeleteAccountAliasResult deleteAccountAlias(DeleteAccountAliasRequest deleteAccountAliasRequest) {
ExecutionContext executionContext = createExecutionContext(deleteAccountAliasRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteAccountAliasRequestMarshaller().marshall(super.beforeMarshalling(deleteAccountAliasRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new DeleteAccountAliasResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes the password policy for the AWS account. There are no parameters.
*
*
* @param deleteAccountPasswordPolicyRequest
* @return Result of the DeleteAccountPasswordPolicy operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced an entity that does not exist. The error message describes
* the entity.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current AWS account limits.
* The error message describes the limit exceeded.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @sample AmazonIdentityManagement.DeleteAccountPasswordPolicy
*/
@Override
public DeleteAccountPasswordPolicyResult deleteAccountPasswordPolicy(DeleteAccountPasswordPolicyRequest deleteAccountPasswordPolicyRequest) {
ExecutionContext executionContext = createExecutionContext(deleteAccountPasswordPolicyRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteAccountPasswordPolicyRequestMarshaller().marshall(super.beforeMarshalling(deleteAccountPasswordPolicyRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new DeleteAccountPasswordPolicyResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
@Override
public DeleteAccountPasswordPolicyResult deleteAccountPasswordPolicy() {
return deleteAccountPasswordPolicy(new DeleteAccountPasswordPolicyRequest());
}
/**
*
* Deletes the specified IAM group. The group must not contain any users or have any attached policies.
*
*
* @param deleteGroupRequest
* @return Result of the DeleteGroup operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced an entity that does not exist. The error message describes
* the entity.
* @throws DeleteConflictException
* The request was rejected because it attempted to delete a resource that has attached subordinate
* entities. The error message describes these entities.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current AWS account limits.
* The error message describes the limit exceeded.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @sample AmazonIdentityManagement.DeleteGroup
*/
@Override
public DeleteGroupResult deleteGroup(DeleteGroupRequest deleteGroupRequest) {
ExecutionContext executionContext = createExecutionContext(deleteGroupRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteGroupRequestMarshaller().marshall(super.beforeMarshalling(deleteGroupRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(new DeleteGroupResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes the specified inline policy that is embedded in the specified IAM group.
*
*
* A group can also have managed policies attached to it. To detach a managed policy from a group, use
* DetachGroupPolicy. For more information about policies, refer to Managed Policies and
* Inline Policies in the IAM User Guide.
*
*
* @param deleteGroupPolicyRequest
* @return Result of the DeleteGroupPolicy operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced an entity that does not exist. The error message describes
* the entity.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current AWS account limits.
* The error message describes the limit exceeded.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @sample AmazonIdentityManagement.DeleteGroupPolicy
*/
@Override
public DeleteGroupPolicyResult deleteGroupPolicy(DeleteGroupPolicyRequest deleteGroupPolicyRequest) {
ExecutionContext executionContext = createExecutionContext(deleteGroupPolicyRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteGroupPolicyRequestMarshaller().marshall(super.beforeMarshalling(deleteGroupPolicyRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new DeleteGroupPolicyResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes the specified instance profile. The instance profile must not have an associated role.
*
*
*
* Make sure you do not have any Amazon EC2 instances running with the instance profile you are about to delete.
* Deleting a role or instance profile that is associated with a running instance will break any applications
* running on the instance.
*
*
*
* For more information about instance profiles, go to About Instance Profiles.
*
*
* @param deleteInstanceProfileRequest
* @return Result of the DeleteInstanceProfile operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced an entity that does not exist. The error message describes
* the entity.
* @throws DeleteConflictException
* The request was rejected because it attempted to delete a resource that has attached subordinate
* entities. The error message describes these entities.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current AWS account limits.
* The error message describes the limit exceeded.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @sample AmazonIdentityManagement.DeleteInstanceProfile
*/
@Override
public DeleteInstanceProfileResult deleteInstanceProfile(DeleteInstanceProfileRequest deleteInstanceProfileRequest) {
ExecutionContext executionContext = createExecutionContext(deleteInstanceProfileRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteInstanceProfileRequestMarshaller().marshall(super.beforeMarshalling(deleteInstanceProfileRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new DeleteInstanceProfileResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes the password for the specified IAM user, which terminates the user's ability to access AWS services
* through the AWS Management Console.
*
*
*
* Deleting a user's password does not prevent a user from accessing AWS through the command line interface or the
* API. To prevent all user access you must also either make any access keys inactive or delete them. For more
* information about making keys inactive or deleting them, see UpdateAccessKey and DeleteAccessKey.
*
*
*
* @param deleteLoginProfileRequest
* @return Result of the DeleteLoginProfile operation returned by the service.
* @throws EntityTemporarilyUnmodifiableException
* The request was rejected because it referenced an entity that is temporarily unmodifiable, such as a user
* name that was deleted and then recreated. The error indicates that the request is likely to succeed if
* you try again after waiting several minutes. The error message describes the entity.
* @throws NoSuchEntityException
* The request was rejected because it referenced an entity that does not exist. The error message describes
* the entity.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current AWS account limits.
* The error message describes the limit exceeded.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @sample AmazonIdentityManagement.DeleteLoginProfile
*/
@Override
public DeleteLoginProfileResult deleteLoginProfile(DeleteLoginProfileRequest deleteLoginProfileRequest) {
ExecutionContext executionContext = createExecutionContext(deleteLoginProfileRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteLoginProfileRequestMarshaller().marshall(super.beforeMarshalling(deleteLoginProfileRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new DeleteLoginProfileResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes an OpenID Connect identity provider (IdP) resource object in IAM.
*
*
* Deleting an IAM OIDC provider resource does not update any roles that reference the provider as a principal in
* their trust policies. Any attempt to assume a role that references a deleted provider fails.
*
*
* This action is idempotent; it does not fail or return an error if you call the action for a provider that does
* not exist.
*
*
* @param deleteOpenIDConnectProviderRequest
* @return Result of the DeleteOpenIDConnectProvider operation returned by the service.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @throws NoSuchEntityException
* The request was rejected because it referenced an entity that does not exist. The error message describes
* the entity.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @sample AmazonIdentityManagement.DeleteOpenIDConnectProvider
*/
@Override
public DeleteOpenIDConnectProviderResult deleteOpenIDConnectProvider(DeleteOpenIDConnectProviderRequest deleteOpenIDConnectProviderRequest) {
ExecutionContext executionContext = createExecutionContext(deleteOpenIDConnectProviderRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteOpenIDConnectProviderRequestMarshaller().marshall(super.beforeMarshalling(deleteOpenIDConnectProviderRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new DeleteOpenIDConnectProviderResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes the specified managed policy.
*
*
* Before you can delete a managed policy, you must first detach the policy from all users, groups, and roles that
* it is attached to, and you must delete all of the policy's versions. The following steps describe the process for
* deleting a managed policy:
*
*
* -
*
* Detach the policy from all users, groups, and roles that the policy is attached to, using the
* DetachUserPolicy, DetachGroupPolicy, or DetachRolePolicy APIs. To list all the users,
* groups, and roles that a policy is attached to, use ListEntitiesForPolicy.
*
*
* -
*
* Delete all versions of the policy using DeletePolicyVersion. To list the policy's versions, use
* ListPolicyVersions. You cannot use DeletePolicyVersion to delete the version that is marked as the
* default version. You delete the policy's default version in the next step of the process.
*
*
* -
*
* Delete the policy (this automatically deletes the policy's default version) using this API.
*
*
*
*
* For information about managed policies, see Managed Policies and
* Inline Policies in the IAM User Guide.
*
*
* @param deletePolicyRequest
* @return Result of the DeletePolicy operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced an entity that does not exist. The error message describes
* the entity.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current AWS account limits.
* The error message describes the limit exceeded.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @throws DeleteConflictException
* The request was rejected because it attempted to delete a resource that has attached subordinate
* entities. The error message describes these entities.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @sample AmazonIdentityManagement.DeletePolicy
*/
@Override
public DeletePolicyResult deletePolicy(DeletePolicyRequest deletePolicyRequest) {
ExecutionContext executionContext = createExecutionContext(deletePolicyRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeletePolicyRequestMarshaller().marshall(super.beforeMarshalling(deletePolicyRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(new DeletePolicyResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes the specified version from the specified managed policy.
*
*
* You cannot delete the default version from a policy using this API. To delete the default version from a policy,
* use DeletePolicy. To find out which version of a policy is marked as the default version, use
* ListPolicyVersions.
*
*
* For information about versions for managed policies, see Versioning for Managed
* Policies in the IAM User Guide.
*
*
* @param deletePolicyVersionRequest
* @return Result of the DeletePolicyVersion operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced an entity that does not exist. The error message describes
* the entity.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current AWS account limits.
* The error message describes the limit exceeded.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @throws DeleteConflictException
* The request was rejected because it attempted to delete a resource that has attached subordinate
* entities. The error message describes these entities.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @sample AmazonIdentityManagement.DeletePolicyVersion
*/
@Override
public DeletePolicyVersionResult deletePolicyVersion(DeletePolicyVersionRequest deletePolicyVersionRequest) {
ExecutionContext executionContext = createExecutionContext(deletePolicyVersionRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeletePolicyVersionRequestMarshaller().marshall(super.beforeMarshalling(deletePolicyVersionRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new DeletePolicyVersionResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes the specified role. The role must not have any policies attached. For more information about roles, go to
* Working with Roles.
*
*
*
* Make sure you do not have any Amazon EC2 instances running with the role you are about to delete. Deleting a role
* or instance profile that is associated with a running instance will break any applications running on the
* instance.
*
*
*
* @param deleteRoleRequest
* @return Result of the DeleteRole operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced an entity that does not exist. The error message describes
* the entity.
* @throws DeleteConflictException
* The request was rejected because it attempted to delete a resource that has attached subordinate
* entities. The error message describes these entities.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current AWS account limits.
* The error message describes the limit exceeded.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @sample AmazonIdentityManagement.DeleteRole
*/
@Override
public DeleteRoleResult deleteRole(DeleteRoleRequest deleteRoleRequest) {
ExecutionContext executionContext = createExecutionContext(deleteRoleRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteRoleRequestMarshaller().marshall(super.beforeMarshalling(deleteRoleRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(new DeleteRoleResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes the specified inline policy that is embedded in the specified IAM role.
*
*
* A role can also have managed policies attached to it. To detach a managed policy from a role, use
* DetachRolePolicy. For more information about policies, refer to Managed Policies and
* Inline Policies in the IAM User Guide.
*
*
* @param deleteRolePolicyRequest
* @return Result of the DeleteRolePolicy operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced an entity that does not exist. The error message describes
* the entity.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current AWS account limits.
* The error message describes the limit exceeded.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @sample AmazonIdentityManagement.DeleteRolePolicy
*/
@Override
public DeleteRolePolicyResult deleteRolePolicy(DeleteRolePolicyRequest deleteRolePolicyRequest) {
ExecutionContext executionContext = createExecutionContext(deleteRolePolicyRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteRolePolicyRequestMarshaller().marshall(super.beforeMarshalling(deleteRolePolicyRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new DeleteRolePolicyResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes a SAML provider resource in IAM.
*
*
* Deleting the provider resource from IAM does not update any roles that reference the SAML provider resource's ARN
* as a principal in their trust policies. Any attempt to assume a role that references a non-existent provider
* resource ARN fails.
*
*
*
* This operation requires Signature
* Version 4.
*
*
*
* @param deleteSAMLProviderRequest
* @return Result of the DeleteSAMLProvider operation returned by the service.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current AWS account limits.
* The error message describes the limit exceeded.
* @throws NoSuchEntityException
* The request was rejected because it referenced an entity that does not exist. The error message describes
* the entity.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @sample AmazonIdentityManagement.DeleteSAMLProvider
*/
@Override
public DeleteSAMLProviderResult deleteSAMLProvider(DeleteSAMLProviderRequest deleteSAMLProviderRequest) {
ExecutionContext executionContext = createExecutionContext(deleteSAMLProviderRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteSAMLProviderRequestMarshaller().marshall(super.beforeMarshalling(deleteSAMLProviderRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new DeleteSAMLProviderResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes the specified SSH public key.
*
*
* The SSH public key deleted by this action is used only for authenticating the associated IAM user to an AWS
* CodeCommit repository. For more information about using SSH keys to authenticate to an AWS CodeCommit repository,
* see Set up AWS
* CodeCommit for SSH Connections in the AWS CodeCommit User Guide.
*
*
* @param deleteSSHPublicKeyRequest
* @return Result of the DeleteSSHPublicKey operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced an entity that does not exist. The error message describes
* the entity.
* @sample AmazonIdentityManagement.DeleteSSHPublicKey
*/
@Override
public DeleteSSHPublicKeyResult deleteSSHPublicKey(DeleteSSHPublicKeyRequest deleteSSHPublicKeyRequest) {
ExecutionContext executionContext = createExecutionContext(deleteSSHPublicKeyRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteSSHPublicKeyRequestMarshaller().marshall(super.beforeMarshalling(deleteSSHPublicKeyRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new DeleteSSHPublicKeyResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes the specified server certificate.
*
*
* For more information about working with server certificates, including a list of AWS services that can use the
* server certificates that you manage with IAM, go to Working with Server
* Certificates in the IAM User Guide.
*
*
*
* If you are using a server certificate with Elastic Load Balancing, deleting the certificate could have
* implications for your application. If Elastic Load Balancing doesn't detect the deletion of bound certificates,
* it may continue to use the certificates. This could cause Elastic Load Balancing to stop accepting traffic. We
* recommend that you remove the reference to the certificate from Elastic Load Balancing before using this command
* to delete the certificate. For more information, go to DeleteLoadBalancerListeners in the Elastic Load Balancing API Reference.
*
*
*
* @param deleteServerCertificateRequest
* @return Result of the DeleteServerCertificate operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced an entity that does not exist. The error message describes
* the entity.
* @throws DeleteConflictException
* The request was rejected because it attempted to delete a resource that has attached subordinate
* entities. The error message describes these entities.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current AWS account limits.
* The error message describes the limit exceeded.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @sample AmazonIdentityManagement.DeleteServerCertificate
*/
@Override
public DeleteServerCertificateResult deleteServerCertificate(DeleteServerCertificateRequest deleteServerCertificateRequest) {
ExecutionContext executionContext = createExecutionContext(deleteServerCertificateRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteServerCertificateRequestMarshaller().marshall(super.beforeMarshalling(deleteServerCertificateRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new DeleteServerCertificateResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes a signing certificate associated with the specified IAM user.
*
*
* If you do not specify a user name, IAM determines the user name implicitly based on the AWS access key ID signing
* the request. Because this action works for access keys under the AWS account, you can use this action to manage
* root credentials even if the AWS account has no associated IAM users.
*
*
* @param deleteSigningCertificateRequest
* @return Result of the DeleteSigningCertificate operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced an entity that does not exist. The error message describes
* the entity.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current AWS account limits.
* The error message describes the limit exceeded.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @sample AmazonIdentityManagement.DeleteSigningCertificate
*/
@Override
public DeleteSigningCertificateResult deleteSigningCertificate(DeleteSigningCertificateRequest deleteSigningCertificateRequest) {
ExecutionContext executionContext = createExecutionContext(deleteSigningCertificateRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteSigningCertificateRequestMarshaller().marshall(super.beforeMarshalling(deleteSigningCertificateRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new DeleteSigningCertificateResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes the specified IAM user. The user must not belong to any groups or have any access keys, signing
* certificates, or attached policies.
*
*
* @param deleteUserRequest
* @return Result of the DeleteUser operation returned by the service.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current AWS account limits.
* The error message describes the limit exceeded.
* @throws NoSuchEntityException
* The request was rejected because it referenced an entity that does not exist. The error message describes
* the entity.
* @throws DeleteConflictException
* The request was rejected because it attempted to delete a resource that has attached subordinate
* entities. The error message describes these entities.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @sample AmazonIdentityManagement.DeleteUser
*/
@Override
public DeleteUserResult deleteUser(DeleteUserRequest deleteUserRequest) {
ExecutionContext executionContext = createExecutionContext(deleteUserRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteUserRequestMarshaller().marshall(super.beforeMarshalling(deleteUserRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(new DeleteUserResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes the specified inline policy that is embedded in the specified IAM user.
*
*
* A user can also have managed policies attached to it. To detach a managed policy from a user, use
* DetachUserPolicy. For more information about policies, refer to Managed Policies and
* Inline Policies in the IAM User Guide.
*
*
* @param deleteUserPolicyRequest
* @return Result of the DeleteUserPolicy operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced an entity that does not exist. The error message describes
* the entity.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current AWS account limits.
* The error message describes the limit exceeded.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @sample AmazonIdentityManagement.DeleteUserPolicy
*/
@Override
public DeleteUserPolicyResult deleteUserPolicy(DeleteUserPolicyRequest deleteUserPolicyRequest) {
ExecutionContext executionContext = createExecutionContext(deleteUserPolicyRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteUserPolicyRequestMarshaller().marshall(super.beforeMarshalling(deleteUserPolicyRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new DeleteUserPolicyResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes a virtual MFA device.
*
*
*
* You must deactivate a user's virtual MFA device before you can delete it. For information about deactivating MFA
* devices, see DeactivateMFADevice.
*
*
*
* @param deleteVirtualMFADeviceRequest
* @return Result of the DeleteVirtualMFADevice operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced an entity that does not exist. The error message describes
* the entity.
* @throws DeleteConflictException
* The request was rejected because it attempted to delete a resource that has attached subordinate
* entities. The error message describes these entities.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current AWS account limits.
* The error message describes the limit exceeded.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @sample AmazonIdentityManagement.DeleteVirtualMFADevice
*/
@Override
public DeleteVirtualMFADeviceResult deleteVirtualMFADevice(DeleteVirtualMFADeviceRequest deleteVirtualMFADeviceRequest) {
ExecutionContext executionContext = createExecutionContext(deleteVirtualMFADeviceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteVirtualMFADeviceRequestMarshaller().marshall(super.beforeMarshalling(deleteVirtualMFADeviceRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new DeleteVirtualMFADeviceResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Removes the specified managed policy from the specified IAM group.
*
*
* A group can also have inline policies embedded with it. To delete an inline policy, use the
* DeleteGroupPolicy API. For information about policies, see Managed Policies and
* Inline Policies in the IAM User Guide.
*
*
* @param detachGroupPolicyRequest
* @return Result of the DetachGroupPolicy operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced an entity that does not exist. The error message describes
* the entity.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current AWS account limits.
* The error message describes the limit exceeded.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @sample AmazonIdentityManagement.DetachGroupPolicy
*/
@Override
public DetachGroupPolicyResult detachGroupPolicy(DetachGroupPolicyRequest detachGroupPolicyRequest) {
ExecutionContext executionContext = createExecutionContext(detachGroupPolicyRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DetachGroupPolicyRequestMarshaller().marshall(super.beforeMarshalling(detachGroupPolicyRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new DetachGroupPolicyResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Removes the specified managed policy from the specified role.
*
*
* A role can also have inline policies embedded with it. To delete an inline policy, use the
* DeleteRolePolicy API. For information about policies, see Managed Policies and
* Inline Policies in the IAM User Guide.
*
*
* @param detachRolePolicyRequest
* @return Result of the DetachRolePolicy operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced an entity that does not exist. The error message describes
* the entity.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current AWS account limits.
* The error message describes the limit exceeded.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @sample AmazonIdentityManagement.DetachRolePolicy
*/
@Override
public DetachRolePolicyResult detachRolePolicy(DetachRolePolicyRequest detachRolePolicyRequest) {
ExecutionContext executionContext = createExecutionContext(detachRolePolicyRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DetachRolePolicyRequestMarshaller().marshall(super.beforeMarshalling(detachRolePolicyRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new DetachRolePolicyResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Removes the specified managed policy from the specified user.
*
*
* A user can also have inline policies embedded with it. To delete an inline policy, use the
* DeleteUserPolicy API. For information about policies, see Managed Policies and
* Inline Policies in the IAM User Guide.
*
*
* @param detachUserPolicyRequest
* @return Result of the DetachUserPolicy operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced an entity that does not exist. The error message describes
* the entity.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current AWS account limits.
* The error message describes the limit exceeded.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @sample AmazonIdentityManagement.DetachUserPolicy
*/
@Override
public DetachUserPolicyResult detachUserPolicy(DetachUserPolicyRequest detachUserPolicyRequest) {
ExecutionContext executionContext = createExecutionContext(detachUserPolicyRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DetachUserPolicyRequestMarshaller().marshall(super.beforeMarshalling(detachUserPolicyRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new DetachUserPolicyResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Enables the specified MFA device and associates it with the specified IAM user. When enabled, the MFA device is
* required for every subsequent login by the IAM user associated with the device.
*
*
* @param enableMFADeviceRequest
* @return Result of the EnableMFADevice operation returned by the service.
* @throws EntityAlreadyExistsException
* The request was rejected because it attempted to create a resource that already exists.
* @throws EntityTemporarilyUnmodifiableException
* The request was rejected because it referenced an entity that is temporarily unmodifiable, such as a user
* name that was deleted and then recreated. The error indicates that the request is likely to succeed if
* you try again after waiting several minutes. The error message describes the entity.
* @throws InvalidAuthenticationCodeException
* The request was rejected because the authentication code was not recognized. The error message describes
* the specific error.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current AWS account limits.
* The error message describes the limit exceeded.
* @throws NoSuchEntityException
* The request was rejected because it referenced an entity that does not exist. The error message describes
* the entity.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @sample AmazonIdentityManagement.EnableMFADevice
*/
@Override
public EnableMFADeviceResult enableMFADevice(EnableMFADeviceRequest enableMFADeviceRequest) {
ExecutionContext executionContext = createExecutionContext(enableMFADeviceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new EnableMFADeviceRequestMarshaller().marshall(super.beforeMarshalling(enableMFADeviceRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new EnableMFADeviceResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Generates a credential report for the AWS account. For more information about the credential report, see Getting Credential Reports in
* the IAM User Guide.
*
*
* @param generateCredentialReportRequest
* @return Result of the GenerateCredentialReport operation returned by the service.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current AWS account limits.
* The error message describes the limit exceeded.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @sample AmazonIdentityManagement.GenerateCredentialReport
*/
@Override
public GenerateCredentialReportResult generateCredentialReport(GenerateCredentialReportRequest generateCredentialReportRequest) {
ExecutionContext executionContext = createExecutionContext(generateCredentialReportRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GenerateCredentialReportRequestMarshaller().marshall(super.beforeMarshalling(generateCredentialReportRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new GenerateCredentialReportResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
@Override
public GenerateCredentialReportResult generateCredentialReport() {
return generateCredentialReport(new GenerateCredentialReportRequest());
}
/**
*
* Retrieves information about when the specified access key was last used. The information includes the date and
* time of last use, along with the AWS service and region that were specified in the last request made with that
* key.
*
*
* @param getAccessKeyLastUsedRequest
* @return Result of the GetAccessKeyLastUsed operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced an entity that does not exist. The error message describes
* the entity.
* @sample AmazonIdentityManagement.GetAccessKeyLastUsed
*/
@Override
public GetAccessKeyLastUsedResult getAccessKeyLastUsed(GetAccessKeyLastUsedRequest getAccessKeyLastUsedRequest) {
ExecutionContext executionContext = createExecutionContext(getAccessKeyLastUsedRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetAccessKeyLastUsedRequestMarshaller().marshall(super.beforeMarshalling(getAccessKeyLastUsedRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new GetAccessKeyLastUsedResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieves information about all IAM users, groups, roles, and policies in your AWS account, including their
* relationships to one another. Use this API to obtain a snapshot of the configuration of IAM permissions (users,
* groups, roles, and policies) in your account.
*
*
* You can optionally filter the results using the Filter
parameter. You can paginate the results using
* the MaxItems
and Marker
parameters.
*
*
* @param getAccountAuthorizationDetailsRequest
* @return Result of the GetAccountAuthorizationDetails operation returned by the service.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @sample AmazonIdentityManagement.GetAccountAuthorizationDetails
*/
@Override
public GetAccountAuthorizationDetailsResult getAccountAuthorizationDetails(GetAccountAuthorizationDetailsRequest getAccountAuthorizationDetailsRequest) {
ExecutionContext executionContext = createExecutionContext(getAccountAuthorizationDetailsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetAccountAuthorizationDetailsRequestMarshaller().marshall(super.beforeMarshalling(getAccountAuthorizationDetailsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new GetAccountAuthorizationDetailsResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
@Override
public GetAccountAuthorizationDetailsResult getAccountAuthorizationDetails() {
return getAccountAuthorizationDetails(new GetAccountAuthorizationDetailsRequest());
}
/**
*
* Retrieves the password policy for the AWS account. For more information about using a password policy, go to Managing an IAM
* Password Policy.
*
*
* @param getAccountPasswordPolicyRequest
* @return Result of the GetAccountPasswordPolicy operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced an entity that does not exist. The error message describes
* the entity.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @sample AmazonIdentityManagement.GetAccountPasswordPolicy
*/
@Override
public GetAccountPasswordPolicyResult getAccountPasswordPolicy(GetAccountPasswordPolicyRequest getAccountPasswordPolicyRequest) {
ExecutionContext executionContext = createExecutionContext(getAccountPasswordPolicyRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetAccountPasswordPolicyRequestMarshaller().marshall(super.beforeMarshalling(getAccountPasswordPolicyRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new GetAccountPasswordPolicyResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
@Override
public GetAccountPasswordPolicyResult getAccountPasswordPolicy() {
return getAccountPasswordPolicy(new GetAccountPasswordPolicyRequest());
}
/**
*
* Retrieves information about IAM entity usage and IAM quotas in the AWS account.
*
*
* For information about limitations on IAM entities, see Limitations on IAM Entities
* in the IAM User Guide.
*
*
* @param getAccountSummaryRequest
* @return Result of the GetAccountSummary operation returned by the service.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @sample AmazonIdentityManagement.GetAccountSummary
*/
@Override
public GetAccountSummaryResult getAccountSummary(GetAccountSummaryRequest getAccountSummaryRequest) {
ExecutionContext executionContext = createExecutionContext(getAccountSummaryRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetAccountSummaryRequestMarshaller().marshall(super.beforeMarshalling(getAccountSummaryRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new GetAccountSummaryResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
@Override
public GetAccountSummaryResult getAccountSummary() {
return getAccountSummary(new GetAccountSummaryRequest());
}
/**
*
* Gets a list of all of the context keys referenced in the input policies. The policies are supplied as a list of
* one or more strings. To get the context keys from policies associated with an IAM user, group, or role, use
* GetContextKeysForPrincipalPolicy.
*
*
* Context keys are variables maintained by AWS and its services that provide details about the context of an API
* query request, and can be evaluated by testing against a value specified in an IAM policy. Use
* GetContextKeysForCustomPolicy to understand what key names and values you must supply when you call
* SimulateCustomPolicy. Note that all parameters are shown in unencoded form here for clarity, but must be
* URL encoded to be included as a part of a real HTML request.
*
*
* @param getContextKeysForCustomPolicyRequest
* @return Result of the GetContextKeysForCustomPolicy operation returned by the service.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @sample AmazonIdentityManagement.GetContextKeysForCustomPolicy
*/
@Override
public GetContextKeysForCustomPolicyResult getContextKeysForCustomPolicy(GetContextKeysForCustomPolicyRequest getContextKeysForCustomPolicyRequest) {
ExecutionContext executionContext = createExecutionContext(getContextKeysForCustomPolicyRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetContextKeysForCustomPolicyRequestMarshaller().marshall(super.beforeMarshalling(getContextKeysForCustomPolicyRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new GetContextKeysForCustomPolicyResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Gets a list of all of the context keys referenced in all of the IAM policies attached to the specified IAM
* entity. The entity can be an IAM user, group, or role. If you specify a user, then the request also includes all
* of the policies attached to groups that the user is a member of.
*
*
* You can optionally include a list of one or more additional policies, specified as strings. If you want to
* include only a list of policies by string, use GetContextKeysForCustomPolicy instead.
*
*
* Note: This API discloses information about the permissions granted to other users. If you do not want
* users to see other user's permissions, then consider allowing them to use GetContextKeysForCustomPolicy
* instead.
*
*
* Context keys are variables maintained by AWS and its services that provide details about the context of an API
* query request, and can be evaluated by testing against a value in an IAM policy. Use
* GetContextKeysForPrincipalPolicy to understand what key names and values you must supply when you call
* SimulatePrincipalPolicy.
*
*
* @param getContextKeysForPrincipalPolicyRequest
* @return Result of the GetContextKeysForPrincipalPolicy operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced an entity that does not exist. The error message describes
* the entity.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @sample AmazonIdentityManagement.GetContextKeysForPrincipalPolicy
*/
@Override
public GetContextKeysForPrincipalPolicyResult getContextKeysForPrincipalPolicy(
GetContextKeysForPrincipalPolicyRequest getContextKeysForPrincipalPolicyRequest) {
ExecutionContext executionContext = createExecutionContext(getContextKeysForPrincipalPolicyRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetContextKeysForPrincipalPolicyRequestMarshaller().marshall(super.beforeMarshalling(getContextKeysForPrincipalPolicyRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new GetContextKeysForPrincipalPolicyResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieves a credential report for the AWS account. For more information about the credential report, see Getting Credential Reports in
* the IAM User Guide.
*
*
* @param getCredentialReportRequest
* @return Result of the GetCredentialReport operation returned by the service.
* @throws CredentialReportNotPresentException
* The request was rejected because the credential report does not exist. To generate a credential report,
* use GenerateCredentialReport.
* @throws CredentialReportExpiredException
* The request was rejected because the most recent credential report has expired. To generate a new
* credential report, use GenerateCredentialReport. For more information about credential report
* expiration, see Getting
* Credential Reports in the IAM User Guide.
* @throws CredentialReportNotReadyException
* The request was rejected because the credential report is still being generated.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @sample AmazonIdentityManagement.GetCredentialReport
*/
@Override
public GetCredentialReportResult getCredentialReport(GetCredentialReportRequest getCredentialReportRequest) {
ExecutionContext executionContext = createExecutionContext(getCredentialReportRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetCredentialReportRequestMarshaller().marshall(super.beforeMarshalling(getCredentialReportRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new GetCredentialReportResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
@Override
public GetCredentialReportResult getCredentialReport() {
return getCredentialReport(new GetCredentialReportRequest());
}
/**
*
* Returns a list of IAM users that are in the specified IAM group. You can paginate the results using the
* MaxItems
and Marker
parameters.
*
*
* @param getGroupRequest
* @return Result of the GetGroup operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced an entity that does not exist. The error message describes
* the entity.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @sample AmazonIdentityManagement.GetGroup
*/
@Override
public GetGroupResult getGroup(GetGroupRequest getGroupRequest) {
ExecutionContext executionContext = createExecutionContext(getGroupRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetGroupRequestMarshaller().marshall(super.beforeMarshalling(getGroupRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(new GetGroupResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieves the specified inline policy document that is embedded in the specified IAM group.
*
*
*
* Policies returned by this API are URL-encoded compliant with RFC
* 3986. You can use a URL decoding method to convert the policy back to plain JSON text. For example, if you
* use Java, you can use the decode
method of the java.net.URLDecoder
utility class in the
* Java SDK. Other languages and SDKs provide similar functionality.
*
*
*
* An IAM group can also have managed policies attached to it. To retrieve a managed policy document that is
* attached to a group, use GetPolicy to determine the policy's default version, then use
* GetPolicyVersion to retrieve the policy document.
*
*
* For more information about policies, see Managed Policies and
* Inline Policies in the IAM User Guide.
*
*
* @param getGroupPolicyRequest
* @return Result of the GetGroupPolicy operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced an entity that does not exist. The error message describes
* the entity.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @sample AmazonIdentityManagement.GetGroupPolicy
*/
@Override
public GetGroupPolicyResult getGroupPolicy(GetGroupPolicyRequest getGroupPolicyRequest) {
ExecutionContext executionContext = createExecutionContext(getGroupPolicyRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetGroupPolicyRequestMarshaller().marshall(super.beforeMarshalling(getGroupPolicyRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new GetGroupPolicyResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieves information about the specified instance profile, including the instance profile's path, GUID, ARN, and
* role. For more information about instance profiles, see About Instance Profiles in
* the IAM User Guide.
*
*
* @param getInstanceProfileRequest
* @return Result of the GetInstanceProfile operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced an entity that does not exist. The error message describes
* the entity.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @sample AmazonIdentityManagement.GetInstanceProfile
*/
@Override
public GetInstanceProfileResult getInstanceProfile(GetInstanceProfileRequest getInstanceProfileRequest) {
ExecutionContext executionContext = createExecutionContext(getInstanceProfileRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetInstanceProfileRequestMarshaller().marshall(super.beforeMarshalling(getInstanceProfileRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new GetInstanceProfileResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieves the user name and password-creation date for the specified IAM user. If the user has not been assigned
* a password, the action returns a 404 (NoSuchEntity
) error.
*
*
* @param getLoginProfileRequest
* @return Result of the GetLoginProfile operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced an entity that does not exist. The error message describes
* the entity.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @sample AmazonIdentityManagement.GetLoginProfile
*/
@Override
public GetLoginProfileResult getLoginProfile(GetLoginProfileRequest getLoginProfileRequest) {
ExecutionContext executionContext = createExecutionContext(getLoginProfileRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetLoginProfileRequestMarshaller().marshall(super.beforeMarshalling(getLoginProfileRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new GetLoginProfileResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns information about the specified OpenID Connect (OIDC) provider resource object in IAM.
*
*
* @param getOpenIDConnectProviderRequest
* @return Result of the GetOpenIDConnectProvider operation returned by the service.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @throws NoSuchEntityException
* The request was rejected because it referenced an entity that does not exist. The error message describes
* the entity.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @sample AmazonIdentityManagement.GetOpenIDConnectProvider
*/
@Override
public GetOpenIDConnectProviderResult getOpenIDConnectProvider(GetOpenIDConnectProviderRequest getOpenIDConnectProviderRequest) {
ExecutionContext executionContext = createExecutionContext(getOpenIDConnectProviderRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetOpenIDConnectProviderRequestMarshaller().marshall(super.beforeMarshalling(getOpenIDConnectProviderRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new GetOpenIDConnectProviderResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieves information about the specified managed policy, including the policy's default version and the total
* number of IAM users, groups, and roles to which the policy is attached. To retrieve the list of the specific
* users, groups, and roles that the policy is attached to, use the ListEntitiesForPolicy API. This API
* returns metadata about the policy. To retrieve the actual policy document for a specific version of the policy,
* use GetPolicyVersion.
*
*
* This API retrieves information about managed policies. To retrieve information about an inline policy that is
* embedded with an IAM user, group, or role, use the GetUserPolicy, GetGroupPolicy, or
* GetRolePolicy API.
*
*
* For more information about policies, see Managed Policies and
* Inline Policies in the IAM User Guide.
*
*
* @param getPolicyRequest
* @return Result of the GetPolicy operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced an entity that does not exist. The error message describes
* the entity.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @sample AmazonIdentityManagement.GetPolicy
*/
@Override
public GetPolicyResult getPolicy(GetPolicyRequest getPolicyRequest) {
ExecutionContext executionContext = createExecutionContext(getPolicyRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetPolicyRequestMarshaller().marshall(super.beforeMarshalling(getPolicyRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(new GetPolicyResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieves information about the specified version of the specified managed policy, including the policy document.
*
*
*
* Policies returned by this API are URL-encoded compliant with RFC
* 3986. You can use a URL decoding method to convert the policy back to plain JSON text. For example, if you
* use Java, you can use the decode
method of the java.net.URLDecoder
utility class in the
* Java SDK. Other languages and SDKs provide similar functionality.
*
*
*
* To list the available versions for a policy, use ListPolicyVersions.
*
*
* This API retrieves information about managed policies. To retrieve information about an inline policy that is
* embedded in a user, group, or role, use the GetUserPolicy, GetGroupPolicy, or GetRolePolicy
* API.
*
*
* For more information about the types of policies, see Managed Policies and
* Inline Policies in the IAM User Guide.
*
*
* For more information about managed policy versions, see Versioning for Managed
* Policies in the IAM User Guide.
*
*
* @param getPolicyVersionRequest
* @return Result of the GetPolicyVersion operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced an entity that does not exist. The error message describes
* the entity.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @sample AmazonIdentityManagement.GetPolicyVersion
*/
@Override
public GetPolicyVersionResult getPolicyVersion(GetPolicyVersionRequest getPolicyVersionRequest) {
ExecutionContext executionContext = createExecutionContext(getPolicyVersionRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetPolicyVersionRequestMarshaller().marshall(super.beforeMarshalling(getPolicyVersionRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new GetPolicyVersionResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieves information about the specified role, including the role's path, GUID, ARN, and the role's trust policy
* that grants permission to assume the role. For more information about roles, see Working with Roles.
*
*
*
* Policies returned by this API are URL-encoded compliant with RFC
* 3986. You can use a URL decoding method to convert the policy back to plain JSON text. For example, if you
* use Java, you can use the decode
method of the java.net.URLDecoder
utility class in the
* Java SDK. Other languages and SDKs provide similar functionality.
*
*
*
* @param getRoleRequest
* @return Result of the GetRole operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced an entity that does not exist. The error message describes
* the entity.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @sample AmazonIdentityManagement.GetRole
*/
@Override
public GetRoleResult getRole(GetRoleRequest getRoleRequest) {
ExecutionContext executionContext = createExecutionContext(getRoleRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetRoleRequestMarshaller().marshall(super.beforeMarshalling(getRoleRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(new GetRoleResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieves the specified inline policy document that is embedded with the specified IAM role.
*
*
*
* Policies returned by this API are URL-encoded compliant with RFC
* 3986. You can use a URL decoding method to convert the policy back to plain JSON text. For example, if you
* use Java, you can use the decode
method of the java.net.URLDecoder
utility class in the
* Java SDK. Other languages and SDKs provide similar functionality.
*
*
*
* An IAM role can also have managed policies attached to it. To retrieve a managed policy document that is attached
* to a role, use GetPolicy to determine the policy's default version, then use GetPolicyVersion to
* retrieve the policy document.
*
*
* For more information about policies, see Managed Policies and
* Inline Policies in the IAM User Guide.
*
*
* For more information about roles, see Using Roles to Delegate Permissions
* and Federate Identities.
*
*
* @param getRolePolicyRequest
* @return Result of the GetRolePolicy operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced an entity that does not exist. The error message describes
* the entity.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @sample AmazonIdentityManagement.GetRolePolicy
*/
@Override
public GetRolePolicyResult getRolePolicy(GetRolePolicyRequest getRolePolicyRequest) {
ExecutionContext executionContext = createExecutionContext(getRolePolicyRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetRolePolicyRequestMarshaller().marshall(super.beforeMarshalling(getRolePolicyRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(new GetRolePolicyResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns the SAML provider metadocument that was uploaded when the IAM SAML provider resource object was created
* or updated.
*
*
*
* This operation requires Signature
* Version 4.
*
*
*
* @param getSAMLProviderRequest
* @return Result of the GetSAMLProvider operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced an entity that does not exist. The error message describes
* the entity.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @throws ServiceFailureException
* The request processing has failed because of an unknown error, exception or failure.
* @sample AmazonIdentityManagement.GetSAMLProvider
*/
@Override
public GetSAMLProviderResult getSAMLProvider(GetSAMLProviderRequest getSAMLProviderRequest) {
ExecutionContext executionContext = createExecutionContext(getSAMLProviderRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetSAMLProviderRequestMarshaller().marshall(super.beforeMarshalling(getSAMLProviderRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new GetSAMLProviderResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieves the specified SSH public key, including metadata about the key.
*
*
* The SSH public key retrieved by this action is used only for authenticating the associated IAM user to an AWS
* CodeCommit repository. For more information about using SSH keys to authenticate to an AWS CodeCommit repository,
* see Set up AWS
* CodeCommit for SSH Connections in the AWS CodeCommit User Guide.
*
*
* @param getSSHPublicKeyRequest
* @return Result of the GetSSHPublicKey operation returned by the service.
* @throws NoSuchEntityException
* The request was rejected because it referenced an entity that does not exist. The error message describes
* the entity.
* @throws UnrecognizedPublicKeyEncodingException
* The request was rejected because the public key encoding format is unsupported or unrecognized.
* @sample AmazonIdentityManagement.GetSSHPublicKey
*/
@Override
public GetSSHPublicKeyResult getSSHPublicKey(GetSSHPublicKeyRequest getSSHPublicKeyRequest) {
ExecutionContext executionContext = createExecutionContext(getSSHPublicKeyRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetSSHPublicKeyRequestMarshaller().marshall(super.beforeMarshalling(getSSHPublicKeyRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new GetSSHPublicKeyResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*