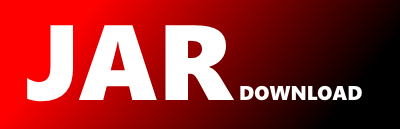
com.amazonaws.services.identitymanagement.model.GetOpenIDConnectProviderResult Maven / Gradle / Ivy
Show all versions of aws-java-sdk-iam Show documentation
/*
* Copyright 2011-2016 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.identitymanagement.model;
import java.io.Serializable;
/**
*
* Contains the response to a successful GetOpenIDConnectProvider request.
*
*/
public class GetOpenIDConnectProviderResult extends com.amazonaws.AmazonWebServiceResult implements Serializable, Cloneable {
/**
*
* The URL that the IAM OIDC provider resource object is associated with. For more information, see
* CreateOpenIDConnectProvider.
*
*/
private String url;
/**
*
* A list of client IDs (also known as audiences) that are associated with the specified IAM OIDC provider resource
* object. For more information, see CreateOpenIDConnectProvider.
*
*/
private com.amazonaws.internal.SdkInternalList clientIDList;
/**
*
* A list of certificate thumbprints that are associated with the specified IAM OIDC provider resource object. For
* more information, see CreateOpenIDConnectProvider.
*
*/
private com.amazonaws.internal.SdkInternalList thumbprintList;
/**
*
* The date and time when the IAM OIDC provider resource object was created in the AWS account.
*
*/
private java.util.Date createDate;
/**
*
* The URL that the IAM OIDC provider resource object is associated with. For more information, see
* CreateOpenIDConnectProvider.
*
*
* @param url
* The URL that the IAM OIDC provider resource object is associated with. For more information, see
* CreateOpenIDConnectProvider.
*/
public void setUrl(String url) {
this.url = url;
}
/**
*
* The URL that the IAM OIDC provider resource object is associated with. For more information, see
* CreateOpenIDConnectProvider.
*
*
* @return The URL that the IAM OIDC provider resource object is associated with. For more information, see
* CreateOpenIDConnectProvider.
*/
public String getUrl() {
return this.url;
}
/**
*
* The URL that the IAM OIDC provider resource object is associated with. For more information, see
* CreateOpenIDConnectProvider.
*
*
* @param url
* The URL that the IAM OIDC provider resource object is associated with. For more information, see
* CreateOpenIDConnectProvider.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetOpenIDConnectProviderResult withUrl(String url) {
setUrl(url);
return this;
}
/**
*
* A list of client IDs (also known as audiences) that are associated with the specified IAM OIDC provider resource
* object. For more information, see CreateOpenIDConnectProvider.
*
*
* @return A list of client IDs (also known as audiences) that are associated with the specified IAM OIDC provider
* resource object. For more information, see CreateOpenIDConnectProvider.
*/
public java.util.List getClientIDList() {
if (clientIDList == null) {
clientIDList = new com.amazonaws.internal.SdkInternalList();
}
return clientIDList;
}
/**
*
* A list of client IDs (also known as audiences) that are associated with the specified IAM OIDC provider resource
* object. For more information, see CreateOpenIDConnectProvider.
*
*
* @param clientIDList
* A list of client IDs (also known as audiences) that are associated with the specified IAM OIDC provider
* resource object. For more information, see CreateOpenIDConnectProvider.
*/
public void setClientIDList(java.util.Collection clientIDList) {
if (clientIDList == null) {
this.clientIDList = null;
return;
}
this.clientIDList = new com.amazonaws.internal.SdkInternalList(clientIDList);
}
/**
*
* A list of client IDs (also known as audiences) that are associated with the specified IAM OIDC provider resource
* object. For more information, see CreateOpenIDConnectProvider.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setClientIDList(java.util.Collection)} or {@link #withClientIDList(java.util.Collection)} if you want to
* override the existing values.
*
*
* @param clientIDList
* A list of client IDs (also known as audiences) that are associated with the specified IAM OIDC provider
* resource object. For more information, see CreateOpenIDConnectProvider.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetOpenIDConnectProviderResult withClientIDList(String... clientIDList) {
if (this.clientIDList == null) {
setClientIDList(new com.amazonaws.internal.SdkInternalList(clientIDList.length));
}
for (String ele : clientIDList) {
this.clientIDList.add(ele);
}
return this;
}
/**
*
* A list of client IDs (also known as audiences) that are associated with the specified IAM OIDC provider resource
* object. For more information, see CreateOpenIDConnectProvider.
*
*
* @param clientIDList
* A list of client IDs (also known as audiences) that are associated with the specified IAM OIDC provider
* resource object. For more information, see CreateOpenIDConnectProvider.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetOpenIDConnectProviderResult withClientIDList(java.util.Collection clientIDList) {
setClientIDList(clientIDList);
return this;
}
/**
*
* A list of certificate thumbprints that are associated with the specified IAM OIDC provider resource object. For
* more information, see CreateOpenIDConnectProvider.
*
*
* @return A list of certificate thumbprints that are associated with the specified IAM OIDC provider resource
* object. For more information, see CreateOpenIDConnectProvider.
*/
public java.util.List getThumbprintList() {
if (thumbprintList == null) {
thumbprintList = new com.amazonaws.internal.SdkInternalList();
}
return thumbprintList;
}
/**
*
* A list of certificate thumbprints that are associated with the specified IAM OIDC provider resource object. For
* more information, see CreateOpenIDConnectProvider.
*
*
* @param thumbprintList
* A list of certificate thumbprints that are associated with the specified IAM OIDC provider resource
* object. For more information, see CreateOpenIDConnectProvider.
*/
public void setThumbprintList(java.util.Collection thumbprintList) {
if (thumbprintList == null) {
this.thumbprintList = null;
return;
}
this.thumbprintList = new com.amazonaws.internal.SdkInternalList(thumbprintList);
}
/**
*
* A list of certificate thumbprints that are associated with the specified IAM OIDC provider resource object. For
* more information, see CreateOpenIDConnectProvider.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setThumbprintList(java.util.Collection)} or {@link #withThumbprintList(java.util.Collection)} if you want
* to override the existing values.
*
*
* @param thumbprintList
* A list of certificate thumbprints that are associated with the specified IAM OIDC provider resource
* object. For more information, see CreateOpenIDConnectProvider.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetOpenIDConnectProviderResult withThumbprintList(String... thumbprintList) {
if (this.thumbprintList == null) {
setThumbprintList(new com.amazonaws.internal.SdkInternalList(thumbprintList.length));
}
for (String ele : thumbprintList) {
this.thumbprintList.add(ele);
}
return this;
}
/**
*
* A list of certificate thumbprints that are associated with the specified IAM OIDC provider resource object. For
* more information, see CreateOpenIDConnectProvider.
*
*
* @param thumbprintList
* A list of certificate thumbprints that are associated with the specified IAM OIDC provider resource
* object. For more information, see CreateOpenIDConnectProvider.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetOpenIDConnectProviderResult withThumbprintList(java.util.Collection thumbprintList) {
setThumbprintList(thumbprintList);
return this;
}
/**
*
* The date and time when the IAM OIDC provider resource object was created in the AWS account.
*
*
* @param createDate
* The date and time when the IAM OIDC provider resource object was created in the AWS account.
*/
public void setCreateDate(java.util.Date createDate) {
this.createDate = createDate;
}
/**
*
* The date and time when the IAM OIDC provider resource object was created in the AWS account.
*
*
* @return The date and time when the IAM OIDC provider resource object was created in the AWS account.
*/
public java.util.Date getCreateDate() {
return this.createDate;
}
/**
*
* The date and time when the IAM OIDC provider resource object was created in the AWS account.
*
*
* @param createDate
* The date and time when the IAM OIDC provider resource object was created in the AWS account.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetOpenIDConnectProviderResult withCreateDate(java.util.Date createDate) {
setCreateDate(createDate);
return this;
}
/**
* Returns a string representation of this object; useful for testing and debugging.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getUrl() != null)
sb.append("Url: " + getUrl() + ",");
if (getClientIDList() != null)
sb.append("ClientIDList: " + getClientIDList() + ",");
if (getThumbprintList() != null)
sb.append("ThumbprintList: " + getThumbprintList() + ",");
if (getCreateDate() != null)
sb.append("CreateDate: " + getCreateDate());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof GetOpenIDConnectProviderResult == false)
return false;
GetOpenIDConnectProviderResult other = (GetOpenIDConnectProviderResult) obj;
if (other.getUrl() == null ^ this.getUrl() == null)
return false;
if (other.getUrl() != null && other.getUrl().equals(this.getUrl()) == false)
return false;
if (other.getClientIDList() == null ^ this.getClientIDList() == null)
return false;
if (other.getClientIDList() != null && other.getClientIDList().equals(this.getClientIDList()) == false)
return false;
if (other.getThumbprintList() == null ^ this.getThumbprintList() == null)
return false;
if (other.getThumbprintList() != null && other.getThumbprintList().equals(this.getThumbprintList()) == false)
return false;
if (other.getCreateDate() == null ^ this.getCreateDate() == null)
return false;
if (other.getCreateDate() != null && other.getCreateDate().equals(this.getCreateDate()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getUrl() == null) ? 0 : getUrl().hashCode());
hashCode = prime * hashCode + ((getClientIDList() == null) ? 0 : getClientIDList().hashCode());
hashCode = prime * hashCode + ((getThumbprintList() == null) ? 0 : getThumbprintList().hashCode());
hashCode = prime * hashCode + ((getCreateDate() == null) ? 0 : getCreateDate().hashCode());
return hashCode;
}
@Override
public GetOpenIDConnectProviderResult clone() {
try {
return (GetOpenIDConnectProviderResult) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
}