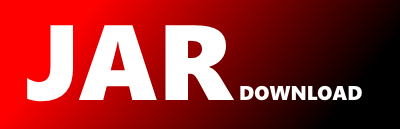
com.amazonaws.services.identitymanagement.AmazonIdentityManagementAsync Maven / Gradle / Ivy
Show all versions of aws-java-sdk-iam Show documentation
/*
* Copyright 2010-2016 Amazon.com, Inc. or its affiliates. All Rights
* Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License").
* You may not use this file except in compliance with the License.
* A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed
* on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either
* express or implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
package com.amazonaws.services.identitymanagement;
import com.amazonaws.services.identitymanagement.model.*;
/**
* Interface for accessing IAM asynchronously. Each asynchronous method will
* return a Java Future object representing the asynchronous operation;
* overloads which accept an {@code AsyncHandler} can be used to receive
* notification when an asynchronous operation completes.
*
* AWS Identity and Access Management
*
* AWS Identity and Access Management (IAM) is a web service that you can use to
* manage users and user permissions under your AWS account. This guide provides
* descriptions of IAM actions that you can call programmatically. For general
* information about IAM, see AWS Identity
* and Access Management (IAM). For the user guide for IAM, see Using IAM.
*
*
*
* AWS provides SDKs that consist of libraries and sample code for various
* programming languages and platforms (Java, Ruby, .NET, iOS, Android, etc.).
* The SDKs provide a convenient way to create programmatic access to IAM and
* AWS. For example, the SDKs take care of tasks such as cryptographically
* signing requests (see below), managing errors, and retrying requests
* automatically. For information about the AWS SDKs, including how to download
* and install them, see the Tools for
* Amazon Web Services page.
*
*
*
* We recommend that you use the AWS SDKs to make programmatic API calls to IAM.
* However, you can also use the IAM Query API to make direct calls to the IAM
* web service. To learn more about the IAM Query API, see Making Query Requests in the Using IAM guide. IAM supports GET
* and POST requests for all actions. That is, the API does not require you to
* use GET for some actions and POST for others. However, GET requests are
* subject to the limitation size of a URL. Therefore, for operations that
* require larger sizes, use a POST request.
*
*
* Signing Requests
*
*
* Requests must be signed using an access key ID and a secret access key. We
* strongly recommend that you do not use your AWS account access key ID and
* secret access key for everyday work with IAM. You can use the access key ID
* and secret access key for an IAM user or you can use the AWS Security Token
* Service to generate temporary security credentials and use those to sign
* requests.
*
*
* To sign requests, we recommend that you use Signature Version 4. If you have an existing application that uses
* Signature Version 2, you do not have to update it to use Signature Version 4.
* However, some operations now require Signature Version 4. The documentation
* for operations that require version 4 indicate this requirement.
*
*
* Additional Resources
*
*
* For more information, see the following:
*
*
* -
*
* AWS Security Credentials. This topic provides general information about
* the types of credentials used for accessing AWS.
*
*
* -
*
* IAM
* Best Practices. This topic presents a list of suggestions for using the
* IAM service to help secure your AWS resources.
*
*
* -
*
* Signing AWS API Requests. This set of topics walk you through the
* process of signing a request using an access key ID and secret access key.
*
*
*
*/
public interface AmazonIdentityManagementAsync extends AmazonIdentityManagement {
/**
*
* Adds a new client ID (also known as audience) to the list of client IDs
* already registered for the specified IAM OpenID Connect (OIDC) provider
* resource.
*
*
* This action is idempotent; it does not fail or return an error if you add
* an existing client ID to the provider.
*
*
* @param addClientIDToOpenIDConnectProviderRequest
* @return A Java Future containing the result of the
* AddClientIDToOpenIDConnectProvider operation returned by the
* service.
* @sample AmazonIdentityManagementAsync.AddClientIDToOpenIDConnectProvider
*/
java.util.concurrent.Future addClientIDToOpenIDConnectProviderAsync(
AddClientIDToOpenIDConnectProviderRequest addClientIDToOpenIDConnectProviderRequest);
/**
*
* Adds a new client ID (also known as audience) to the list of client IDs
* already registered for the specified IAM OpenID Connect (OIDC) provider
* resource.
*
*
* This action is idempotent; it does not fail or return an error if you add
* an existing client ID to the provider.
*
*
* @param addClientIDToOpenIDConnectProviderRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the
* AddClientIDToOpenIDConnectProvider operation returned by the
* service.
* @sample
* AmazonIdentityManagementAsyncHandler.AddClientIDToOpenIDConnectProvider
*/
java.util.concurrent.Future addClientIDToOpenIDConnectProviderAsync(
AddClientIDToOpenIDConnectProviderRequest addClientIDToOpenIDConnectProviderRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Adds the specified IAM role to the specified instance profile.
*
*
*
* The caller of this API must be granted the PassRole
* permission on the IAM role by a permission policy.
*
*
*
* For more information about roles, go to Working with Roles. For more information about instance profiles, go
* to About Instance Profiles.
*
*
* @param addRoleToInstanceProfileRequest
* @return A Java Future containing the result of the
* AddRoleToInstanceProfile operation returned by the service.
* @sample AmazonIdentityManagementAsync.AddRoleToInstanceProfile
*/
java.util.concurrent.Future addRoleToInstanceProfileAsync(
AddRoleToInstanceProfileRequest addRoleToInstanceProfileRequest);
/**
*
* Adds the specified IAM role to the specified instance profile.
*
*
*
* The caller of this API must be granted the PassRole
* permission on the IAM role by a permission policy.
*
*
*
* For more information about roles, go to Working with Roles. For more information about instance profiles, go
* to About Instance Profiles.
*
*
* @param addRoleToInstanceProfileRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the
* AddRoleToInstanceProfile operation returned by the service.
* @sample AmazonIdentityManagementAsyncHandler.AddRoleToInstanceProfile
*/
java.util.concurrent.Future addRoleToInstanceProfileAsync(
AddRoleToInstanceProfileRequest addRoleToInstanceProfileRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Adds the specified user to the specified group.
*
*
* @param addUserToGroupRequest
* @return A Java Future containing the result of the AddUserToGroup
* operation returned by the service.
* @sample AmazonIdentityManagementAsync.AddUserToGroup
*/
java.util.concurrent.Future addUserToGroupAsync(
AddUserToGroupRequest addUserToGroupRequest);
/**
*
* Adds the specified user to the specified group.
*
*
* @param addUserToGroupRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AddUserToGroup
* operation returned by the service.
* @sample AmazonIdentityManagementAsyncHandler.AddUserToGroup
*/
java.util.concurrent.Future addUserToGroupAsync(
AddUserToGroupRequest addUserToGroupRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Attaches the specified managed policy to the specified IAM group.
*
*
* You use this API to attach a managed policy to a group. To embed an
* inline policy in a group, use PutGroupPolicy.
*
*
* For more information about policies, see Managed Policies and Inline Policies in the IAM User Guide.
*
*
* @param attachGroupPolicyRequest
* @return A Java Future containing the result of the AttachGroupPolicy
* operation returned by the service.
* @sample AmazonIdentityManagementAsync.AttachGroupPolicy
*/
java.util.concurrent.Future attachGroupPolicyAsync(
AttachGroupPolicyRequest attachGroupPolicyRequest);
/**
*
* Attaches the specified managed policy to the specified IAM group.
*
*
* You use this API to attach a managed policy to a group. To embed an
* inline policy in a group, use PutGroupPolicy.
*
*
* For more information about policies, see Managed Policies and Inline Policies in the IAM User Guide.
*
*
* @param attachGroupPolicyRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AttachGroupPolicy
* operation returned by the service.
* @sample AmazonIdentityManagementAsyncHandler.AttachGroupPolicy
*/
java.util.concurrent.Future attachGroupPolicyAsync(
AttachGroupPolicyRequest attachGroupPolicyRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Attaches the specified managed policy to the specified IAM role.
*
*
* When you attach a managed policy to a role, the managed policy becomes
* part of the role's permission (access) policy. You cannot use a managed
* policy as the role's trust policy. The role's trust policy is created at
* the same time as the role, using CreateRole. You can update a
* role's trust policy using UpdateAssumeRolePolicy.
*
*
* Use this API to attach a managed policy to a role. To embed an
* inline policy in a role, use PutRolePolicy. For more information
* about policies, see Managed Policies and Inline Policies in the IAM User Guide.
*
*
* @param attachRolePolicyRequest
* @return A Java Future containing the result of the AttachRolePolicy
* operation returned by the service.
* @sample AmazonIdentityManagementAsync.AttachRolePolicy
*/
java.util.concurrent.Future attachRolePolicyAsync(
AttachRolePolicyRequest attachRolePolicyRequest);
/**
*
* Attaches the specified managed policy to the specified IAM role.
*
*
* When you attach a managed policy to a role, the managed policy becomes
* part of the role's permission (access) policy. You cannot use a managed
* policy as the role's trust policy. The role's trust policy is created at
* the same time as the role, using CreateRole. You can update a
* role's trust policy using UpdateAssumeRolePolicy.
*
*
* Use this API to attach a managed policy to a role. To embed an
* inline policy in a role, use PutRolePolicy. For more information
* about policies, see Managed Policies and Inline Policies in the IAM User Guide.
*
*
* @param attachRolePolicyRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AttachRolePolicy
* operation returned by the service.
* @sample AmazonIdentityManagementAsyncHandler.AttachRolePolicy
*/
java.util.concurrent.Future attachRolePolicyAsync(
AttachRolePolicyRequest attachRolePolicyRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Attaches the specified managed policy to the specified user.
*
*
* You use this API to attach a managed policy to a user. To embed an
* inline policy in a user, use PutUserPolicy.
*
*
* For more information about policies, see Managed Policies and Inline Policies in the IAM User Guide.
*
*
* @param attachUserPolicyRequest
* @return A Java Future containing the result of the AttachUserPolicy
* operation returned by the service.
* @sample AmazonIdentityManagementAsync.AttachUserPolicy
*/
java.util.concurrent.Future attachUserPolicyAsync(
AttachUserPolicyRequest attachUserPolicyRequest);
/**
*
* Attaches the specified managed policy to the specified user.
*
*
* You use this API to attach a managed policy to a user. To embed an
* inline policy in a user, use PutUserPolicy.
*
*
* For more information about policies, see Managed Policies and Inline Policies in the IAM User Guide.
*
*
* @param attachUserPolicyRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AttachUserPolicy
* operation returned by the service.
* @sample AmazonIdentityManagementAsyncHandler.AttachUserPolicy
*/
java.util.concurrent.Future attachUserPolicyAsync(
AttachUserPolicyRequest attachUserPolicyRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Changes the password of the IAM user who is calling this action. The root
* account password is not affected by this action.
*
*
* To change the password for a different user, see
* UpdateLoginProfile. For more information about modifying
* passwords, see Managing Passwords in the IAM User Guide.
*
*
* @param changePasswordRequest
* @return A Java Future containing the result of the ChangePassword
* operation returned by the service.
* @sample AmazonIdentityManagementAsync.ChangePassword
*/
java.util.concurrent.Future changePasswordAsync(
ChangePasswordRequest changePasswordRequest);
/**
*
* Changes the password of the IAM user who is calling this action. The root
* account password is not affected by this action.
*
*
* To change the password for a different user, see
* UpdateLoginProfile. For more information about modifying
* passwords, see Managing Passwords in the IAM User Guide.
*
*
* @param changePasswordRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ChangePassword
* operation returned by the service.
* @sample AmazonIdentityManagementAsyncHandler.ChangePassword
*/
java.util.concurrent.Future changePasswordAsync(
ChangePasswordRequest changePasswordRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a new AWS secret access key and corresponding AWS access key ID
* for the specified user. The default status for new keys is
* Active
.
*
*
* If you do not specify a user name, IAM determines the user name
* implicitly based on the AWS access key ID signing the request. Because
* this action works for access keys under the AWS account, you can use this
* action to manage root credentials even if the AWS account has no
* associated users.
*
*
* For information about limits on the number of keys you can create, see Limitations on IAM Entities in the IAM User Guide.
*
*
*
* To ensure the security of your AWS account, the secret access key is
* accessible only during key and user creation. You must save the key (for
* example, in a text file) if you want to be able to access it again. If a
* secret key is lost, you can delete the access keys for the associated
* user and then create new keys.
*
*
*
* @param createAccessKeyRequest
* @return A Java Future containing the result of the CreateAccessKey
* operation returned by the service.
* @sample AmazonIdentityManagementAsync.CreateAccessKey
*/
java.util.concurrent.Future createAccessKeyAsync(
CreateAccessKeyRequest createAccessKeyRequest);
/**
*
* Creates a new AWS secret access key and corresponding AWS access key ID
* for the specified user. The default status for new keys is
* Active
.
*
*
* If you do not specify a user name, IAM determines the user name
* implicitly based on the AWS access key ID signing the request. Because
* this action works for access keys under the AWS account, you can use this
* action to manage root credentials even if the AWS account has no
* associated users.
*
*
* For information about limits on the number of keys you can create, see Limitations on IAM Entities in the IAM User Guide.
*
*
*
* To ensure the security of your AWS account, the secret access key is
* accessible only during key and user creation. You must save the key (for
* example, in a text file) if you want to be able to access it again. If a
* secret key is lost, you can delete the access keys for the associated
* user and then create new keys.
*
*
*
* @param createAccessKeyRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateAccessKey
* operation returned by the service.
* @sample AmazonIdentityManagementAsyncHandler.CreateAccessKey
*/
java.util.concurrent.Future createAccessKeyAsync(
CreateAccessKeyRequest createAccessKeyRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Simplified method form for invoking the CreateAccessKey operation.
*
* @see #createAccessKeyAsync(CreateAccessKeyRequest)
*/
java.util.concurrent.Future createAccessKeyAsync();
/**
* Simplified method form for invoking the CreateAccessKey operation with an
* AsyncHandler.
*
* @see #createAccessKeyAsync(CreateAccessKeyRequest,
* com.amazonaws.handlers.AsyncHandler)
*/
java.util.concurrent.Future createAccessKeyAsync(
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates an alias for your AWS account. For information about using an AWS
* account alias, see Using
* an Alias for Your AWS Account ID in the IAM User Guide.
*
*
* @param createAccountAliasRequest
* @return A Java Future containing the result of the CreateAccountAlias
* operation returned by the service.
* @sample AmazonIdentityManagementAsync.CreateAccountAlias
*/
java.util.concurrent.Future createAccountAliasAsync(
CreateAccountAliasRequest createAccountAliasRequest);
/**
*
* Creates an alias for your AWS account. For information about using an AWS
* account alias, see Using
* an Alias for Your AWS Account ID in the IAM User Guide.
*
*
* @param createAccountAliasRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateAccountAlias
* operation returned by the service.
* @sample AmazonIdentityManagementAsyncHandler.CreateAccountAlias
*/
java.util.concurrent.Future createAccountAliasAsync(
CreateAccountAliasRequest createAccountAliasRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a new group.
*
*
* For information about the number of groups you can create, see Limitations on IAM Entities in the IAM User Guide.
*
*
* @param createGroupRequest
* @return A Java Future containing the result of the CreateGroup operation
* returned by the service.
* @sample AmazonIdentityManagementAsync.CreateGroup
*/
java.util.concurrent.Future createGroupAsync(
CreateGroupRequest createGroupRequest);
/**
*
* Creates a new group.
*
*
* For information about the number of groups you can create, see Limitations on IAM Entities in the IAM User Guide.
*
*
* @param createGroupRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateGroup operation
* returned by the service.
* @sample AmazonIdentityManagementAsyncHandler.CreateGroup
*/
java.util.concurrent.Future createGroupAsync(
CreateGroupRequest createGroupRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a new instance profile. For information about instance profiles,
* go to About Instance Profiles.
*
*
* For information about the number of instance profiles you can create, see
* Limitations on IAM Entities in the IAM User Guide.
*
*
* @param createInstanceProfileRequest
* @return A Java Future containing the result of the CreateInstanceProfile
* operation returned by the service.
* @sample AmazonIdentityManagementAsync.CreateInstanceProfile
*/
java.util.concurrent.Future createInstanceProfileAsync(
CreateInstanceProfileRequest createInstanceProfileRequest);
/**
*
* Creates a new instance profile. For information about instance profiles,
* go to About Instance Profiles.
*
*
* For information about the number of instance profiles you can create, see
* Limitations on IAM Entities in the IAM User Guide.
*
*
* @param createInstanceProfileRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateInstanceProfile
* operation returned by the service.
* @sample AmazonIdentityManagementAsyncHandler.CreateInstanceProfile
*/
java.util.concurrent.Future createInstanceProfileAsync(
CreateInstanceProfileRequest createInstanceProfileRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a password for the specified user, giving the user the ability to
* access AWS services through the AWS Management Console. For more
* information about managing passwords, see Managing Passwords in the IAM User Guide.
*
*
* @param createLoginProfileRequest
* @return A Java Future containing the result of the CreateLoginProfile
* operation returned by the service.
* @sample AmazonIdentityManagementAsync.CreateLoginProfile
*/
java.util.concurrent.Future createLoginProfileAsync(
CreateLoginProfileRequest createLoginProfileRequest);
/**
*
* Creates a password for the specified user, giving the user the ability to
* access AWS services through the AWS Management Console. For more
* information about managing passwords, see Managing Passwords in the IAM User Guide.
*
*
* @param createLoginProfileRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateLoginProfile
* operation returned by the service.
* @sample AmazonIdentityManagementAsyncHandler.CreateLoginProfile
*/
java.util.concurrent.Future createLoginProfileAsync(
CreateLoginProfileRequest createLoginProfileRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates an IAM entity to describe an identity provider (IdP) that
* supports OpenID Connect (OIDC).
*
*
* The OIDC provider that you create with this operation can be used as a
* principal in a role's trust policy to establish a trust relationship
* between AWS and the OIDC provider.
*
*
* When you create the IAM OIDC provider, you specify the URL of the OIDC
* identity provider (IdP) to trust, a list of client IDs (also known as
* audiences) that identify the application or applications that are allowed
* to authenticate using the OIDC provider, and a list of thumbprints of the
* server certificate(s) that the IdP uses. You get all of this information
* from the OIDC IdP that you want to use for access to AWS.
*
*
*
* Because trust for the OIDC provider is ultimately derived from the IAM
* provider that this action creates, it is a best practice to limit access
* to the CreateOpenIDConnectProvider action to highly-privileged
* users.
*
*
*
* @param createOpenIDConnectProviderRequest
* @return A Java Future containing the result of the
* CreateOpenIDConnectProvider operation returned by the service.
* @sample AmazonIdentityManagementAsync.CreateOpenIDConnectProvider
*/
java.util.concurrent.Future createOpenIDConnectProviderAsync(
CreateOpenIDConnectProviderRequest createOpenIDConnectProviderRequest);
/**
*
* Creates an IAM entity to describe an identity provider (IdP) that
* supports OpenID Connect (OIDC).
*
*
* The OIDC provider that you create with this operation can be used as a
* principal in a role's trust policy to establish a trust relationship
* between AWS and the OIDC provider.
*
*
* When you create the IAM OIDC provider, you specify the URL of the OIDC
* identity provider (IdP) to trust, a list of client IDs (also known as
* audiences) that identify the application or applications that are allowed
* to authenticate using the OIDC provider, and a list of thumbprints of the
* server certificate(s) that the IdP uses. You get all of this information
* from the OIDC IdP that you want to use for access to AWS.
*
*
*
* Because trust for the OIDC provider is ultimately derived from the IAM
* provider that this action creates, it is a best practice to limit access
* to the CreateOpenIDConnectProvider action to highly-privileged
* users.
*
*
*
* @param createOpenIDConnectProviderRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the
* CreateOpenIDConnectProvider operation returned by the service.
* @sample AmazonIdentityManagementAsyncHandler.CreateOpenIDConnectProvider
*/
java.util.concurrent.Future createOpenIDConnectProviderAsync(
CreateOpenIDConnectProviderRequest createOpenIDConnectProviderRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a new managed policy for your AWS account.
*
*
* This operation creates a policy version with a version identifier of
* v1
and sets v1 as the policy's default version. For more
* information about policy versions, see Versioning for Managed Policies in the IAM User Guide.
*
*
* For more information about managed policies in general, see Managed Policies and Inline Policies in the IAM User Guide.
*
*
* @param createPolicyRequest
* @return A Java Future containing the result of the CreatePolicy operation
* returned by the service.
* @sample AmazonIdentityManagementAsync.CreatePolicy
*/
java.util.concurrent.Future createPolicyAsync(
CreatePolicyRequest createPolicyRequest);
/**
*
* Creates a new managed policy for your AWS account.
*
*
* This operation creates a policy version with a version identifier of
* v1
and sets v1 as the policy's default version. For more
* information about policy versions, see Versioning for Managed Policies in the IAM User Guide.
*
*
* For more information about managed policies in general, see Managed Policies and Inline Policies in the IAM User Guide.
*
*
* @param createPolicyRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreatePolicy operation
* returned by the service.
* @sample AmazonIdentityManagementAsyncHandler.CreatePolicy
*/
java.util.concurrent.Future createPolicyAsync(
CreatePolicyRequest createPolicyRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a new version of the specified managed policy. To update a
* managed policy, you create a new policy version. A managed policy can
* have up to five versions. If the policy has five versions, you must
* delete an existing version using DeletePolicyVersion before you
* create a new version.
*
*
* Optionally, you can set the new version as the policy's default version.
* The default version is the version that is in effect for the IAM users,
* groups, and roles to which the policy is attached.
*
*
* For more information about managed policy versions, see Versioning for Managed Policies in the IAM User Guide.
*
*
* @param createPolicyVersionRequest
* @return A Java Future containing the result of the CreatePolicyVersion
* operation returned by the service.
* @sample AmazonIdentityManagementAsync.CreatePolicyVersion
*/
java.util.concurrent.Future createPolicyVersionAsync(
CreatePolicyVersionRequest createPolicyVersionRequest);
/**
*
* Creates a new version of the specified managed policy. To update a
* managed policy, you create a new policy version. A managed policy can
* have up to five versions. If the policy has five versions, you must
* delete an existing version using DeletePolicyVersion before you
* create a new version.
*
*
* Optionally, you can set the new version as the policy's default version.
* The default version is the version that is in effect for the IAM users,
* groups, and roles to which the policy is attached.
*
*
* For more information about managed policy versions, see Versioning for Managed Policies in the IAM User Guide.
*
*
* @param createPolicyVersionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreatePolicyVersion
* operation returned by the service.
* @sample AmazonIdentityManagementAsyncHandler.CreatePolicyVersion
*/
java.util.concurrent.Future createPolicyVersionAsync(
CreatePolicyVersionRequest createPolicyVersionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a new role for your AWS account. For more information about
* roles, go to Working with Roles. For information about limitations on role names
* and the number of roles you can create, go to Limitations on IAM Entities in the IAM User Guide.
*
*
* @param createRoleRequest
* @return A Java Future containing the result of the CreateRole operation
* returned by the service.
* @sample AmazonIdentityManagementAsync.CreateRole
*/
java.util.concurrent.Future createRoleAsync(
CreateRoleRequest createRoleRequest);
/**
*
* Creates a new role for your AWS account. For more information about
* roles, go to Working with Roles. For information about limitations on role names
* and the number of roles you can create, go to Limitations on IAM Entities in the IAM User Guide.
*
*
* @param createRoleRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateRole operation
* returned by the service.
* @sample AmazonIdentityManagementAsyncHandler.CreateRole
*/
java.util.concurrent.Future createRoleAsync(
CreateRoleRequest createRoleRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates an IAM resource that describes an identity provider (IdP) that
* supports SAML 2.0.
*
*
* The SAML provider resource that you create with this operation can be
* used as a principal in an IAM role's trust policy to enable federated
* users who sign-in using the SAML IdP to assume the role. You can create
* an IAM role that supports Web-based single sign-on (SSO) to the AWS
* Management Console or one that supports API access to AWS.
*
*
* When you create the SAML provider resource, you upload an a SAML metadata
* document that you get from your IdP and that includes the issuer's name,
* expiration information, and keys that can be used to validate the SAML
* authentication response (assertions) that the IdP sends. You must
* generate the metadata document using the identity management software
* that is used as your organization's IdP.
*
*
*
* This operation requires Signature Version 4.
*
*
*
* For more information, see Enabling SAML 2.0 Federated Users to Access the AWS Management
* Console and About SAML 2.0-based Federation in the IAM User Guide.
*
*
* @param createSAMLProviderRequest
* @return A Java Future containing the result of the CreateSAMLProvider
* operation returned by the service.
* @sample AmazonIdentityManagementAsync.CreateSAMLProvider
*/
java.util.concurrent.Future createSAMLProviderAsync(
CreateSAMLProviderRequest createSAMLProviderRequest);
/**
*
* Creates an IAM resource that describes an identity provider (IdP) that
* supports SAML 2.0.
*
*
* The SAML provider resource that you create with this operation can be
* used as a principal in an IAM role's trust policy to enable federated
* users who sign-in using the SAML IdP to assume the role. You can create
* an IAM role that supports Web-based single sign-on (SSO) to the AWS
* Management Console or one that supports API access to AWS.
*
*
* When you create the SAML provider resource, you upload an a SAML metadata
* document that you get from your IdP and that includes the issuer's name,
* expiration information, and keys that can be used to validate the SAML
* authentication response (assertions) that the IdP sends. You must
* generate the metadata document using the identity management software
* that is used as your organization's IdP.
*
*
*
* This operation requires Signature Version 4.
*
*
*
* For more information, see Enabling SAML 2.0 Federated Users to Access the AWS Management
* Console and About SAML 2.0-based Federation in the IAM User Guide.
*
*
* @param createSAMLProviderRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateSAMLProvider
* operation returned by the service.
* @sample AmazonIdentityManagementAsyncHandler.CreateSAMLProvider
*/
java.util.concurrent.Future createSAMLProviderAsync(
CreateSAMLProviderRequest createSAMLProviderRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a new IAM user for your AWS account.
*
*
* For information about limitations on the number of IAM users you can
* create, see Limitations on IAM Entities in the IAM User Guide.
*
*
* @param createUserRequest
* @return A Java Future containing the result of the CreateUser operation
* returned by the service.
* @sample AmazonIdentityManagementAsync.CreateUser
*/
java.util.concurrent.Future createUserAsync(
CreateUserRequest createUserRequest);
/**
*
* Creates a new IAM user for your AWS account.
*
*
* For information about limitations on the number of IAM users you can
* create, see Limitations on IAM Entities in the IAM User Guide.
*
*
* @param createUserRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateUser operation
* returned by the service.
* @sample AmazonIdentityManagementAsyncHandler.CreateUser
*/
java.util.concurrent.Future createUserAsync(
CreateUserRequest createUserRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a new virtual MFA device for the AWS account. After creating the
* virtual MFA, use EnableMFADevice to attach the MFA device to an
* IAM user. For more information about creating and working with virtual
* MFA devices, go to Using a Virtual MFA Device in the IAM User Guide.
*
*
* For information about limits on the number of MFA devices you can create,
* see Limitations on Entities in the IAM User Guide.
*
*
*
* The seed information contained in the QR code and the Base32 string
* should be treated like any other secret access information, such as your
* AWS access keys or your passwords. After you provision your virtual
* device, you should ensure that the information is destroyed following
* secure procedures.
*
*
*
* @param createVirtualMFADeviceRequest
* @return A Java Future containing the result of the CreateVirtualMFADevice
* operation returned by the service.
* @sample AmazonIdentityManagementAsync.CreateVirtualMFADevice
*/
java.util.concurrent.Future createVirtualMFADeviceAsync(
CreateVirtualMFADeviceRequest createVirtualMFADeviceRequest);
/**
*
* Creates a new virtual MFA device for the AWS account. After creating the
* virtual MFA, use EnableMFADevice to attach the MFA device to an
* IAM user. For more information about creating and working with virtual
* MFA devices, go to Using a Virtual MFA Device in the IAM User Guide.
*
*
* For information about limits on the number of MFA devices you can create,
* see Limitations on Entities in the IAM User Guide.
*
*
*
* The seed information contained in the QR code and the Base32 string
* should be treated like any other secret access information, such as your
* AWS access keys or your passwords. After you provision your virtual
* device, you should ensure that the information is destroyed following
* secure procedures.
*
*
*
* @param createVirtualMFADeviceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateVirtualMFADevice
* operation returned by the service.
* @sample AmazonIdentityManagementAsyncHandler.CreateVirtualMFADevice
*/
java.util.concurrent.Future createVirtualMFADeviceAsync(
CreateVirtualMFADeviceRequest createVirtualMFADeviceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deactivates the specified MFA device and removes it from association with
* the user name for which it was originally enabled.
*
*
* For more information about creating and working with virtual MFA devices,
* go to Using a Virtual MFA Device in the IAM User Guide.
*
*
* @param deactivateMFADeviceRequest
* @return A Java Future containing the result of the DeactivateMFADevice
* operation returned by the service.
* @sample AmazonIdentityManagementAsync.DeactivateMFADevice
*/
java.util.concurrent.Future deactivateMFADeviceAsync(
DeactivateMFADeviceRequest deactivateMFADeviceRequest);
/**
*
* Deactivates the specified MFA device and removes it from association with
* the user name for which it was originally enabled.
*
*
* For more information about creating and working with virtual MFA devices,
* go to Using a Virtual MFA Device in the IAM User Guide.
*
*
* @param deactivateMFADeviceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeactivateMFADevice
* operation returned by the service.
* @sample AmazonIdentityManagementAsyncHandler.DeactivateMFADevice
*/
java.util.concurrent.Future deactivateMFADeviceAsync(
DeactivateMFADeviceRequest deactivateMFADeviceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the access key pair associated with the specified IAM user.
*
*
* If you do not specify a user name, IAM determines the user name
* implicitly based on the AWS access key ID signing the request. Because
* this action works for access keys under the AWS account, you can use this
* action to manage root credentials even if the AWS account has no
* associated users.
*
*
* @param deleteAccessKeyRequest
* @return A Java Future containing the result of the DeleteAccessKey
* operation returned by the service.
* @sample AmazonIdentityManagementAsync.DeleteAccessKey
*/
java.util.concurrent.Future deleteAccessKeyAsync(
DeleteAccessKeyRequest deleteAccessKeyRequest);
/**
*
* Deletes the access key pair associated with the specified IAM user.
*
*
* If you do not specify a user name, IAM determines the user name
* implicitly based on the AWS access key ID signing the request. Because
* this action works for access keys under the AWS account, you can use this
* action to manage root credentials even if the AWS account has no
* associated users.
*
*
* @param deleteAccessKeyRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteAccessKey
* operation returned by the service.
* @sample AmazonIdentityManagementAsyncHandler.DeleteAccessKey
*/
java.util.concurrent.Future deleteAccessKeyAsync(
DeleteAccessKeyRequest deleteAccessKeyRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the specified AWS account alias. For information about using an
* AWS account alias, see Using
* an Alias for Your AWS Account ID in the IAM User Guide.
*
*
* @param deleteAccountAliasRequest
* @return A Java Future containing the result of the DeleteAccountAlias
* operation returned by the service.
* @sample AmazonIdentityManagementAsync.DeleteAccountAlias
*/
java.util.concurrent.Future deleteAccountAliasAsync(
DeleteAccountAliasRequest deleteAccountAliasRequest);
/**
*
* Deletes the specified AWS account alias. For information about using an
* AWS account alias, see Using
* an Alias for Your AWS Account ID in the IAM User Guide.
*
*
* @param deleteAccountAliasRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteAccountAlias
* operation returned by the service.
* @sample AmazonIdentityManagementAsyncHandler.DeleteAccountAlias
*/
java.util.concurrent.Future deleteAccountAliasAsync(
DeleteAccountAliasRequest deleteAccountAliasRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the password policy for the AWS account. There are no parameters.
*
*
* @param deleteAccountPasswordPolicyRequest
* @return A Java Future containing the result of the
* DeleteAccountPasswordPolicy operation returned by the service.
* @sample AmazonIdentityManagementAsync.DeleteAccountPasswordPolicy
*/
java.util.concurrent.Future deleteAccountPasswordPolicyAsync(
DeleteAccountPasswordPolicyRequest deleteAccountPasswordPolicyRequest);
/**
*
* Deletes the password policy for the AWS account. There are no parameters.
*
*
* @param deleteAccountPasswordPolicyRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the
* DeleteAccountPasswordPolicy operation returned by the service.
* @sample AmazonIdentityManagementAsyncHandler.DeleteAccountPasswordPolicy
*/
java.util.concurrent.Future deleteAccountPasswordPolicyAsync(
DeleteAccountPasswordPolicyRequest deleteAccountPasswordPolicyRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Simplified method form for invoking the DeleteAccountPasswordPolicy
* operation.
*
* @see #deleteAccountPasswordPolicyAsync(DeleteAccountPasswordPolicyRequest)
*/
java.util.concurrent.Future deleteAccountPasswordPolicyAsync();
/**
* Simplified method form for invoking the DeleteAccountPasswordPolicy
* operation with an AsyncHandler.
*
* @see #deleteAccountPasswordPolicyAsync(DeleteAccountPasswordPolicyRequest,
* com.amazonaws.handlers.AsyncHandler)
*/
java.util.concurrent.Future deleteAccountPasswordPolicyAsync(
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the specified IAM group. The group must not contain any users or
* have any attached policies.
*
*
* @param deleteGroupRequest
* @return A Java Future containing the result of the DeleteGroup operation
* returned by the service.
* @sample AmazonIdentityManagementAsync.DeleteGroup
*/
java.util.concurrent.Future deleteGroupAsync(
DeleteGroupRequest deleteGroupRequest);
/**
*
* Deletes the specified IAM group. The group must not contain any users or
* have any attached policies.
*
*
* @param deleteGroupRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteGroup operation
* returned by the service.
* @sample AmazonIdentityManagementAsyncHandler.DeleteGroup
*/
java.util.concurrent.Future deleteGroupAsync(
DeleteGroupRequest deleteGroupRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the specified inline policy that is embedded in the specified IAM
* group.
*
*
* A group can also have managed policies attached to it. To detach a
* managed policy from a group, use DetachGroupPolicy. For more
* information about policies, refer to Managed Policies and Inline Policies in the IAM User Guide.
*
*
* @param deleteGroupPolicyRequest
* @return A Java Future containing the result of the DeleteGroupPolicy
* operation returned by the service.
* @sample AmazonIdentityManagementAsync.DeleteGroupPolicy
*/
java.util.concurrent.Future deleteGroupPolicyAsync(
DeleteGroupPolicyRequest deleteGroupPolicyRequest);
/**
*
* Deletes the specified inline policy that is embedded in the specified IAM
* group.
*
*
* A group can also have managed policies attached to it. To detach a
* managed policy from a group, use DetachGroupPolicy. For more
* information about policies, refer to Managed Policies and Inline Policies in the IAM User Guide.
*
*
* @param deleteGroupPolicyRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteGroupPolicy
* operation returned by the service.
* @sample AmazonIdentityManagementAsyncHandler.DeleteGroupPolicy
*/
java.util.concurrent.Future deleteGroupPolicyAsync(
DeleteGroupPolicyRequest deleteGroupPolicyRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the specified instance profile. The instance profile must not
* have an associated role.
*
*
*
* Make sure you do not have any Amazon EC2 instances running with the
* instance profile you are about to delete. Deleting a role or instance
* profile that is associated with a running instance will break any
* applications running on the instance.
*
*
*
* For more information about instance profiles, go to About Instance Profiles.
*
*
* @param deleteInstanceProfileRequest
* @return A Java Future containing the result of the DeleteInstanceProfile
* operation returned by the service.
* @sample AmazonIdentityManagementAsync.DeleteInstanceProfile
*/
java.util.concurrent.Future deleteInstanceProfileAsync(
DeleteInstanceProfileRequest deleteInstanceProfileRequest);
/**
*
* Deletes the specified instance profile. The instance profile must not
* have an associated role.
*
*
*
* Make sure you do not have any Amazon EC2 instances running with the
* instance profile you are about to delete. Deleting a role or instance
* profile that is associated with a running instance will break any
* applications running on the instance.
*
*
*
* For more information about instance profiles, go to About Instance Profiles.
*
*
* @param deleteInstanceProfileRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteInstanceProfile
* operation returned by the service.
* @sample AmazonIdentityManagementAsyncHandler.DeleteInstanceProfile
*/
java.util.concurrent.Future deleteInstanceProfileAsync(
DeleteInstanceProfileRequest deleteInstanceProfileRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the password for the specified IAM user, which terminates the
* user's ability to access AWS services through the AWS Management Console.
*
*
*
* Deleting a user's password does not prevent a user from accessing AWS
* through the command line interface or the API. To prevent all user access
* you must also either make any access keys inactive or delete them. For
* more information about making keys inactive or deleting them, see
* UpdateAccessKey and DeleteAccessKey.
*
*
*
* @param deleteLoginProfileRequest
* @return A Java Future containing the result of the DeleteLoginProfile
* operation returned by the service.
* @sample AmazonIdentityManagementAsync.DeleteLoginProfile
*/
java.util.concurrent.Future deleteLoginProfileAsync(
DeleteLoginProfileRequest deleteLoginProfileRequest);
/**
*
* Deletes the password for the specified IAM user, which terminates the
* user's ability to access AWS services through the AWS Management Console.
*
*
*
* Deleting a user's password does not prevent a user from accessing AWS
* through the command line interface or the API. To prevent all user access
* you must also either make any access keys inactive or delete them. For
* more information about making keys inactive or deleting them, see
* UpdateAccessKey and DeleteAccessKey.
*
*
*
* @param deleteLoginProfileRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteLoginProfile
* operation returned by the service.
* @sample AmazonIdentityManagementAsyncHandler.DeleteLoginProfile
*/
java.util.concurrent.Future deleteLoginProfileAsync(
DeleteLoginProfileRequest deleteLoginProfileRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes an OpenID Connect identity provider (IdP) resource object in IAM.
*
*
* Deleting an IAM OIDC provider resource does not update any roles that
* reference the provider as a principal in their trust policies. Any
* attempt to assume a role that references a deleted provider fails.
*
*
* This action is idempotent; it does not fail or return an error if you
* call the action for a provider that does not exist.
*
*
* @param deleteOpenIDConnectProviderRequest
* @return A Java Future containing the result of the
* DeleteOpenIDConnectProvider operation returned by the service.
* @sample AmazonIdentityManagementAsync.DeleteOpenIDConnectProvider
*/
java.util.concurrent.Future deleteOpenIDConnectProviderAsync(
DeleteOpenIDConnectProviderRequest deleteOpenIDConnectProviderRequest);
/**
*
* Deletes an OpenID Connect identity provider (IdP) resource object in IAM.
*
*
* Deleting an IAM OIDC provider resource does not update any roles that
* reference the provider as a principal in their trust policies. Any
* attempt to assume a role that references a deleted provider fails.
*
*
* This action is idempotent; it does not fail or return an error if you
* call the action for a provider that does not exist.
*
*
* @param deleteOpenIDConnectProviderRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the
* DeleteOpenIDConnectProvider operation returned by the service.
* @sample AmazonIdentityManagementAsyncHandler.DeleteOpenIDConnectProvider
*/
java.util.concurrent.Future deleteOpenIDConnectProviderAsync(
DeleteOpenIDConnectProviderRequest deleteOpenIDConnectProviderRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the specified managed policy.
*
*
* Before you can delete a managed policy, you must first detach the policy
* from all users, groups, and roles that it is attached to, and you must
* delete all of the policy's versions. The following steps describe the
* process for deleting a managed policy:
*
*
* -
*
* Detach the policy from all users, groups, and roles that the policy is
* attached to, using the DetachUserPolicy, DetachGroupPolicy,
* or DetachRolePolicy APIs. To list all the users, groups, and roles
* that a policy is attached to, use ListEntitiesForPolicy.
*
*
* -
*
* Delete all versions of the policy using DeletePolicyVersion. To
* list the policy's versions, use ListPolicyVersions. You cannot use
* DeletePolicyVersion to delete the version that is marked as the
* default version. You delete the policy's default version in the next step
* of the process.
*
*
* -
*
* Delete the policy (this automatically deletes the policy's default
* version) using this API.
*
*
*
*
* For information about managed policies, see Managed Policies and Inline Policies in the IAM User Guide.
*
*
* @param deletePolicyRequest
* @return A Java Future containing the result of the DeletePolicy operation
* returned by the service.
* @sample AmazonIdentityManagementAsync.DeletePolicy
*/
java.util.concurrent.Future deletePolicyAsync(
DeletePolicyRequest deletePolicyRequest);
/**
*
* Deletes the specified managed policy.
*
*
* Before you can delete a managed policy, you must first detach the policy
* from all users, groups, and roles that it is attached to, and you must
* delete all of the policy's versions. The following steps describe the
* process for deleting a managed policy:
*
*
* -
*
* Detach the policy from all users, groups, and roles that the policy is
* attached to, using the DetachUserPolicy, DetachGroupPolicy,
* or DetachRolePolicy APIs. To list all the users, groups, and roles
* that a policy is attached to, use ListEntitiesForPolicy.
*
*
* -
*
* Delete all versions of the policy using DeletePolicyVersion. To
* list the policy's versions, use ListPolicyVersions. You cannot use
* DeletePolicyVersion to delete the version that is marked as the
* default version. You delete the policy's default version in the next step
* of the process.
*
*
* -
*
* Delete the policy (this automatically deletes the policy's default
* version) using this API.
*
*
*
*
* For information about managed policies, see Managed Policies and Inline Policies in the IAM User Guide.
*
*
* @param deletePolicyRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeletePolicy operation
* returned by the service.
* @sample AmazonIdentityManagementAsyncHandler.DeletePolicy
*/
java.util.concurrent.Future deletePolicyAsync(
DeletePolicyRequest deletePolicyRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the specified version from the specified managed policy.
*
*
* You cannot delete the default version from a policy using this API. To
* delete the default version from a policy, use DeletePolicy. To
* find out which version of a policy is marked as the default version, use
* ListPolicyVersions.
*
*
* For information about versions for managed policies, see Versioning for Managed Policies in the IAM User Guide.
*
*
* @param deletePolicyVersionRequest
* @return A Java Future containing the result of the DeletePolicyVersion
* operation returned by the service.
* @sample AmazonIdentityManagementAsync.DeletePolicyVersion
*/
java.util.concurrent.Future deletePolicyVersionAsync(
DeletePolicyVersionRequest deletePolicyVersionRequest);
/**
*
* Deletes the specified version from the specified managed policy.
*
*
* You cannot delete the default version from a policy using this API. To
* delete the default version from a policy, use DeletePolicy. To
* find out which version of a policy is marked as the default version, use
* ListPolicyVersions.
*
*
* For information about versions for managed policies, see Versioning for Managed Policies in the IAM User Guide.
*
*
* @param deletePolicyVersionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeletePolicyVersion
* operation returned by the service.
* @sample AmazonIdentityManagementAsyncHandler.DeletePolicyVersion
*/
java.util.concurrent.Future deletePolicyVersionAsync(
DeletePolicyVersionRequest deletePolicyVersionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the specified role. The role must not have any policies attached.
* For more information about roles, go to Working with Roles.
*
*
*
* Make sure you do not have any Amazon EC2 instances running with the role
* you are about to delete. Deleting a role or instance profile that is
* associated with a running instance will break any applications running on
* the instance.
*
*
*
* @param deleteRoleRequest
* @return A Java Future containing the result of the DeleteRole operation
* returned by the service.
* @sample AmazonIdentityManagementAsync.DeleteRole
*/
java.util.concurrent.Future deleteRoleAsync(
DeleteRoleRequest deleteRoleRequest);
/**
*
* Deletes the specified role. The role must not have any policies attached.
* For more information about roles, go to Working with Roles.
*
*
*
* Make sure you do not have any Amazon EC2 instances running with the role
* you are about to delete. Deleting a role or instance profile that is
* associated with a running instance will break any applications running on
* the instance.
*
*
*
* @param deleteRoleRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteRole operation
* returned by the service.
* @sample AmazonIdentityManagementAsyncHandler.DeleteRole
*/
java.util.concurrent.Future deleteRoleAsync(
DeleteRoleRequest deleteRoleRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the specified inline policy that is embedded in the specified IAM
* role.
*
*
* A role can also have managed policies attached to it. To detach a managed
* policy from a role, use DetachRolePolicy. For more information
* about policies, refer to Managed Policies and Inline Policies in the IAM User Guide.
*
*
* @param deleteRolePolicyRequest
* @return A Java Future containing the result of the DeleteRolePolicy
* operation returned by the service.
* @sample AmazonIdentityManagementAsync.DeleteRolePolicy
*/
java.util.concurrent.Future deleteRolePolicyAsync(
DeleteRolePolicyRequest deleteRolePolicyRequest);
/**
*
* Deletes the specified inline policy that is embedded in the specified IAM
* role.
*
*
* A role can also have managed policies attached to it. To detach a managed
* policy from a role, use DetachRolePolicy. For more information
* about policies, refer to Managed Policies and Inline Policies in the IAM User Guide.
*
*
* @param deleteRolePolicyRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteRolePolicy
* operation returned by the service.
* @sample AmazonIdentityManagementAsyncHandler.DeleteRolePolicy
*/
java.util.concurrent.Future deleteRolePolicyAsync(
DeleteRolePolicyRequest deleteRolePolicyRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a SAML provider resource in IAM.
*
*
* Deleting the provider resource from IAM does not update any roles that
* reference the SAML provider resource's ARN as a principal in their trust
* policies. Any attempt to assume a role that references a non-existent
* provider resource ARN fails.
*
*
*
* This operation requires Signature Version 4.
*
*
*
* @param deleteSAMLProviderRequest
* @return A Java Future containing the result of the DeleteSAMLProvider
* operation returned by the service.
* @sample AmazonIdentityManagementAsync.DeleteSAMLProvider
*/
java.util.concurrent.Future deleteSAMLProviderAsync(
DeleteSAMLProviderRequest deleteSAMLProviderRequest);
/**
*
* Deletes a SAML provider resource in IAM.
*
*
* Deleting the provider resource from IAM does not update any roles that
* reference the SAML provider resource's ARN as a principal in their trust
* policies. Any attempt to assume a role that references a non-existent
* provider resource ARN fails.
*
*
*
* This operation requires Signature Version 4.
*
*
*
* @param deleteSAMLProviderRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteSAMLProvider
* operation returned by the service.
* @sample AmazonIdentityManagementAsyncHandler.DeleteSAMLProvider
*/
java.util.concurrent.Future deleteSAMLProviderAsync(
DeleteSAMLProviderRequest deleteSAMLProviderRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the specified SSH public key.
*
*
* The SSH public key deleted by this action is used only for authenticating
* the associated IAM user to an AWS CodeCommit repository. For more
* information about using SSH keys to authenticate to an AWS CodeCommit
* repository, see Set up AWS CodeCommit for SSH Connections in the AWS CodeCommit
* User Guide.
*
*
* @param deleteSSHPublicKeyRequest
* @return A Java Future containing the result of the DeleteSSHPublicKey
* operation returned by the service.
* @sample AmazonIdentityManagementAsync.DeleteSSHPublicKey
*/
java.util.concurrent.Future deleteSSHPublicKeyAsync(
DeleteSSHPublicKeyRequest deleteSSHPublicKeyRequest);
/**
*
* Deletes the specified SSH public key.
*
*
* The SSH public key deleted by this action is used only for authenticating
* the associated IAM user to an AWS CodeCommit repository. For more
* information about using SSH keys to authenticate to an AWS CodeCommit
* repository, see Set up AWS CodeCommit for SSH Connections in the AWS CodeCommit
* User Guide.
*
*
* @param deleteSSHPublicKeyRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteSSHPublicKey
* operation returned by the service.
* @sample AmazonIdentityManagementAsyncHandler.DeleteSSHPublicKey
*/
java.util.concurrent.Future deleteSSHPublicKeyAsync(
DeleteSSHPublicKeyRequest deleteSSHPublicKeyRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the specified server certificate.
*
*
* For more information about working with server certificates, including a
* list of AWS services that can use the server certificates that you manage
* with IAM, go to Working with Server Certificates in the IAM User Guide.
*
*
*
* If you are using a server certificate with Elastic Load Balancing,
* deleting the certificate could have implications for your application. If
* Elastic Load Balancing doesn't detect the deletion of bound certificates,
* it may continue to use the certificates. This could cause Elastic Load
* Balancing to stop accepting traffic. We recommend that you remove the
* reference to the certificate from Elastic Load Balancing before using
* this command to delete the certificate. For more information, go to DeleteLoadBalancerListeners in the Elastic Load Balancing API
* Reference.
*
*
*
* @param deleteServerCertificateRequest
* @return A Java Future containing the result of the
* DeleteServerCertificate operation returned by the service.
* @sample AmazonIdentityManagementAsync.DeleteServerCertificate
*/
java.util.concurrent.Future deleteServerCertificateAsync(
DeleteServerCertificateRequest deleteServerCertificateRequest);
/**
*
* Deletes the specified server certificate.
*
*
* For more information about working with server certificates, including a
* list of AWS services that can use the server certificates that you manage
* with IAM, go to Working with Server Certificates in the IAM User Guide.
*
*
*
* If you are using a server certificate with Elastic Load Balancing,
* deleting the certificate could have implications for your application. If
* Elastic Load Balancing doesn't detect the deletion of bound certificates,
* it may continue to use the certificates. This could cause Elastic Load
* Balancing to stop accepting traffic. We recommend that you remove the
* reference to the certificate from Elastic Load Balancing before using
* this command to delete the certificate. For more information, go to DeleteLoadBalancerListeners in the Elastic Load Balancing API
* Reference.
*
*
*
* @param deleteServerCertificateRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the
* DeleteServerCertificate operation returned by the service.
* @sample AmazonIdentityManagementAsyncHandler.DeleteServerCertificate
*/
java.util.concurrent.Future deleteServerCertificateAsync(
DeleteServerCertificateRequest deleteServerCertificateRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a signing certificate associated with the specified IAM user.
*
*
* If you do not specify a user name, IAM determines the user name
* implicitly based on the AWS access key ID signing the request. Because
* this action works for access keys under the AWS account, you can use this
* action to manage root credentials even if the AWS account has no
* associated IAM users.
*
*
* @param deleteSigningCertificateRequest
* @return A Java Future containing the result of the
* DeleteSigningCertificate operation returned by the service.
* @sample AmazonIdentityManagementAsync.DeleteSigningCertificate
*/
java.util.concurrent.Future deleteSigningCertificateAsync(
DeleteSigningCertificateRequest deleteSigningCertificateRequest);
/**
*
* Deletes a signing certificate associated with the specified IAM user.
*
*
* If you do not specify a user name, IAM determines the user name
* implicitly based on the AWS access key ID signing the request. Because
* this action works for access keys under the AWS account, you can use this
* action to manage root credentials even if the AWS account has no
* associated IAM users.
*
*
* @param deleteSigningCertificateRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the
* DeleteSigningCertificate operation returned by the service.
* @sample AmazonIdentityManagementAsyncHandler.DeleteSigningCertificate
*/
java.util.concurrent.Future deleteSigningCertificateAsync(
DeleteSigningCertificateRequest deleteSigningCertificateRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the specified IAM user. The user must not belong to any groups or
* have any access keys, signing certificates, or attached policies.
*
*
* @param deleteUserRequest
* @return A Java Future containing the result of the DeleteUser operation
* returned by the service.
* @sample AmazonIdentityManagementAsync.DeleteUser
*/
java.util.concurrent.Future deleteUserAsync(
DeleteUserRequest deleteUserRequest);
/**
*
* Deletes the specified IAM user. The user must not belong to any groups or
* have any access keys, signing certificates, or attached policies.
*
*
* @param deleteUserRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteUser operation
* returned by the service.
* @sample AmazonIdentityManagementAsyncHandler.DeleteUser
*/
java.util.concurrent.Future deleteUserAsync(
DeleteUserRequest deleteUserRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the specified inline policy that is embedded in the specified IAM
* user.
*
*
* A user can also have managed policies attached to it. To detach a managed
* policy from a user, use DetachUserPolicy. For more information
* about policies, refer to Managed Policies and Inline Policies in the IAM User Guide.
*
*
* @param deleteUserPolicyRequest
* @return A Java Future containing the result of the DeleteUserPolicy
* operation returned by the service.
* @sample AmazonIdentityManagementAsync.DeleteUserPolicy
*/
java.util.concurrent.Future deleteUserPolicyAsync(
DeleteUserPolicyRequest deleteUserPolicyRequest);
/**
*
* Deletes the specified inline policy that is embedded in the specified IAM
* user.
*
*
* A user can also have managed policies attached to it. To detach a managed
* policy from a user, use DetachUserPolicy. For more information
* about policies, refer to Managed Policies and Inline Policies in the IAM User Guide.
*
*
* @param deleteUserPolicyRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteUserPolicy
* operation returned by the service.
* @sample AmazonIdentityManagementAsyncHandler.DeleteUserPolicy
*/
java.util.concurrent.Future deleteUserPolicyAsync(
DeleteUserPolicyRequest deleteUserPolicyRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a virtual MFA device.
*
*
*
* You must deactivate a user's virtual MFA device before you can delete it.
* For information about deactivating MFA devices, see
* DeactivateMFADevice.
*
*
*
* @param deleteVirtualMFADeviceRequest
* @return A Java Future containing the result of the DeleteVirtualMFADevice
* operation returned by the service.
* @sample AmazonIdentityManagementAsync.DeleteVirtualMFADevice
*/
java.util.concurrent.Future deleteVirtualMFADeviceAsync(
DeleteVirtualMFADeviceRequest deleteVirtualMFADeviceRequest);
/**
*
* Deletes a virtual MFA device.
*
*
*
* You must deactivate a user's virtual MFA device before you can delete it.
* For information about deactivating MFA devices, see
* DeactivateMFADevice.
*
*
*
* @param deleteVirtualMFADeviceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteVirtualMFADevice
* operation returned by the service.
* @sample AmazonIdentityManagementAsyncHandler.DeleteVirtualMFADevice
*/
java.util.concurrent.Future deleteVirtualMFADeviceAsync(
DeleteVirtualMFADeviceRequest deleteVirtualMFADeviceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Removes the specified managed policy from the specified IAM group.
*
*
* A group can also have inline policies embedded with it. To delete an
* inline policy, use the DeleteGroupPolicy API. For information
* about policies, see Managed Policies and Inline Policies in the IAM User Guide.
*
*
* @param detachGroupPolicyRequest
* @return A Java Future containing the result of the DetachGroupPolicy
* operation returned by the service.
* @sample AmazonIdentityManagementAsync.DetachGroupPolicy
*/
java.util.concurrent.Future detachGroupPolicyAsync(
DetachGroupPolicyRequest detachGroupPolicyRequest);
/**
*
* Removes the specified managed policy from the specified IAM group.
*
*
* A group can also have inline policies embedded with it. To delete an
* inline policy, use the DeleteGroupPolicy API. For information
* about policies, see Managed Policies and Inline Policies in the IAM User Guide.
*
*
* @param detachGroupPolicyRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DetachGroupPolicy
* operation returned by the service.
* @sample AmazonIdentityManagementAsyncHandler.DetachGroupPolicy
*/
java.util.concurrent.Future detachGroupPolicyAsync(
DetachGroupPolicyRequest detachGroupPolicyRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Removes the specified managed policy from the specified role.
*
*
* A role can also have inline policies embedded with it. To delete an
* inline policy, use the DeleteRolePolicy API. For information about
* policies, see Managed Policies and Inline Policies in the IAM User Guide.
*
*
* @param detachRolePolicyRequest
* @return A Java Future containing the result of the DetachRolePolicy
* operation returned by the service.
* @sample AmazonIdentityManagementAsync.DetachRolePolicy
*/
java.util.concurrent.Future detachRolePolicyAsync(
DetachRolePolicyRequest detachRolePolicyRequest);
/**
*
* Removes the specified managed policy from the specified role.
*
*
* A role can also have inline policies embedded with it. To delete an
* inline policy, use the DeleteRolePolicy API. For information about
* policies, see Managed Policies and Inline Policies in the IAM User Guide.
*
*
* @param detachRolePolicyRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DetachRolePolicy
* operation returned by the service.
* @sample AmazonIdentityManagementAsyncHandler.DetachRolePolicy
*/
java.util.concurrent.Future detachRolePolicyAsync(
DetachRolePolicyRequest detachRolePolicyRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Removes the specified managed policy from the specified user.
*
*
* A user can also have inline policies embedded with it. To delete an
* inline policy, use the DeleteUserPolicy API. For information about
* policies, see Managed Policies and Inline Policies in the IAM User Guide.
*
*
* @param detachUserPolicyRequest
* @return A Java Future containing the result of the DetachUserPolicy
* operation returned by the service.
* @sample AmazonIdentityManagementAsync.DetachUserPolicy
*/
java.util.concurrent.Future detachUserPolicyAsync(
DetachUserPolicyRequest detachUserPolicyRequest);
/**
*
* Removes the specified managed policy from the specified user.
*
*
* A user can also have inline policies embedded with it. To delete an
* inline policy, use the DeleteUserPolicy API. For information about
* policies, see Managed Policies and Inline Policies in the IAM User Guide.
*
*
* @param detachUserPolicyRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DetachUserPolicy
* operation returned by the service.
* @sample AmazonIdentityManagementAsyncHandler.DetachUserPolicy
*/
java.util.concurrent.Future detachUserPolicyAsync(
DetachUserPolicyRequest detachUserPolicyRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Enables the specified MFA device and associates it with the specified IAM
* user. When enabled, the MFA device is required for every subsequent login
* by the IAM user associated with the device.
*
*
* @param enableMFADeviceRequest
* @return A Java Future containing the result of the EnableMFADevice
* operation returned by the service.
* @sample AmazonIdentityManagementAsync.EnableMFADevice
*/
java.util.concurrent.Future enableMFADeviceAsync(
EnableMFADeviceRequest enableMFADeviceRequest);
/**
*
* Enables the specified MFA device and associates it with the specified IAM
* user. When enabled, the MFA device is required for every subsequent login
* by the IAM user associated with the device.
*
*
* @param enableMFADeviceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the EnableMFADevice
* operation returned by the service.
* @sample AmazonIdentityManagementAsyncHandler.EnableMFADevice
*/
java.util.concurrent.Future enableMFADeviceAsync(
EnableMFADeviceRequest enableMFADeviceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Generates a credential report for the AWS account. For more information
* about the credential report, see Getting Credential Reports in the IAM User Guide.
*
*
* @param generateCredentialReportRequest
* @return A Java Future containing the result of the
* GenerateCredentialReport operation returned by the service.
* @sample AmazonIdentityManagementAsync.GenerateCredentialReport
*/
java.util.concurrent.Future generateCredentialReportAsync(
GenerateCredentialReportRequest generateCredentialReportRequest);
/**
*
* Generates a credential report for the AWS account. For more information
* about the credential report, see Getting Credential Reports in the IAM User Guide.
*
*
* @param generateCredentialReportRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the
* GenerateCredentialReport operation returned by the service.
* @sample AmazonIdentityManagementAsyncHandler.GenerateCredentialReport
*/
java.util.concurrent.Future generateCredentialReportAsync(
GenerateCredentialReportRequest generateCredentialReportRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Simplified method form for invoking the GenerateCredentialReport
* operation.
*
* @see #generateCredentialReportAsync(GenerateCredentialReportRequest)
*/
java.util.concurrent.Future generateCredentialReportAsync();
/**
* Simplified method form for invoking the GenerateCredentialReport
* operation with an AsyncHandler.
*
* @see #generateCredentialReportAsync(GenerateCredentialReportRequest,
* com.amazonaws.handlers.AsyncHandler)
*/
java.util.concurrent.Future generateCredentialReportAsync(
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves information about when the specified access key was last used.
* The information includes the date and time of last use, along with the
* AWS service and region that were specified in the last request made with
* that key.
*
*
* @param getAccessKeyLastUsedRequest
* @return A Java Future containing the result of the GetAccessKeyLastUsed
* operation returned by the service.
* @sample AmazonIdentityManagementAsync.GetAccessKeyLastUsed
*/
java.util.concurrent.Future getAccessKeyLastUsedAsync(
GetAccessKeyLastUsedRequest getAccessKeyLastUsedRequest);
/**
*
* Retrieves information about when the specified access key was last used.
* The information includes the date and time of last use, along with the
* AWS service and region that were specified in the last request made with
* that key.
*
*
* @param getAccessKeyLastUsedRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetAccessKeyLastUsed
* operation returned by the service.
* @sample AmazonIdentityManagementAsyncHandler.GetAccessKeyLastUsed
*/
java.util.concurrent.Future getAccessKeyLastUsedAsync(
GetAccessKeyLastUsedRequest getAccessKeyLastUsedRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves information about all IAM users, groups, roles, and policies in
* your AWS account, including their relationships to one another. Use this
* API to obtain a snapshot of the configuration of IAM permissions (users,
* groups, roles, and policies) in your account.
*
*
* You can optionally filter the results using the Filter
* parameter. You can paginate the results using the MaxItems
* and Marker
parameters.
*
*
* @param getAccountAuthorizationDetailsRequest
* @return A Java Future containing the result of the
* GetAccountAuthorizationDetails operation returned by the service.
* @sample AmazonIdentityManagementAsync.GetAccountAuthorizationDetails
*/
java.util.concurrent.Future getAccountAuthorizationDetailsAsync(
GetAccountAuthorizationDetailsRequest getAccountAuthorizationDetailsRequest);
/**
*
* Retrieves information about all IAM users, groups, roles, and policies in
* your AWS account, including their relationships to one another. Use this
* API to obtain a snapshot of the configuration of IAM permissions (users,
* groups, roles, and policies) in your account.
*
*
* You can optionally filter the results using the Filter
* parameter. You can paginate the results using the MaxItems
* and Marker
parameters.
*
*
* @param getAccountAuthorizationDetailsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the
* GetAccountAuthorizationDetails operation returned by the service.
* @sample
* AmazonIdentityManagementAsyncHandler.GetAccountAuthorizationDetails
*/
java.util.concurrent.Future getAccountAuthorizationDetailsAsync(
GetAccountAuthorizationDetailsRequest getAccountAuthorizationDetailsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Simplified method form for invoking the GetAccountAuthorizationDetails
* operation.
*
* @see #getAccountAuthorizationDetailsAsync(GetAccountAuthorizationDetailsRequest)
*/
java.util.concurrent.Future getAccountAuthorizationDetailsAsync();
/**
* Simplified method form for invoking the GetAccountAuthorizationDetails
* operation with an AsyncHandler.
*
* @see #getAccountAuthorizationDetailsAsync(GetAccountAuthorizationDetailsRequest,
* com.amazonaws.handlers.AsyncHandler)
*/
java.util.concurrent.Future getAccountAuthorizationDetailsAsync(
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves the password policy for the AWS account. For more information
* about using a password policy, go to Managing an IAM Password Policy.
*
*
* @param getAccountPasswordPolicyRequest
* @return A Java Future containing the result of the
* GetAccountPasswordPolicy operation returned by the service.
* @sample AmazonIdentityManagementAsync.GetAccountPasswordPolicy
*/
java.util.concurrent.Future getAccountPasswordPolicyAsync(
GetAccountPasswordPolicyRequest getAccountPasswordPolicyRequest);
/**
*
* Retrieves the password policy for the AWS account. For more information
* about using a password policy, go to Managing an IAM Password Policy.
*
*
* @param getAccountPasswordPolicyRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the
* GetAccountPasswordPolicy operation returned by the service.
* @sample AmazonIdentityManagementAsyncHandler.GetAccountPasswordPolicy
*/
java.util.concurrent.Future getAccountPasswordPolicyAsync(
GetAccountPasswordPolicyRequest getAccountPasswordPolicyRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Simplified method form for invoking the GetAccountPasswordPolicy
* operation.
*
* @see #getAccountPasswordPolicyAsync(GetAccountPasswordPolicyRequest)
*/
java.util.concurrent.Future getAccountPasswordPolicyAsync();
/**
* Simplified method form for invoking the GetAccountPasswordPolicy
* operation with an AsyncHandler.
*
* @see #getAccountPasswordPolicyAsync(GetAccountPasswordPolicyRequest,
* com.amazonaws.handlers.AsyncHandler)
*/
java.util.concurrent.Future getAccountPasswordPolicyAsync(
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves information about IAM entity usage and IAM quotas in the AWS
* account.
*
*
* For information about limitations on IAM entities, see Limitations on IAM Entities in the IAM User Guide.
*
*
* @param getAccountSummaryRequest
* @return A Java Future containing the result of the GetAccountSummary
* operation returned by the service.
* @sample AmazonIdentityManagementAsync.GetAccountSummary
*/
java.util.concurrent.Future getAccountSummaryAsync(
GetAccountSummaryRequest getAccountSummaryRequest);
/**
*
* Retrieves information about IAM entity usage and IAM quotas in the AWS
* account.
*
*
* For information about limitations on IAM entities, see Limitations on IAM Entities in the IAM User Guide.
*
*
* @param getAccountSummaryRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetAccountSummary
* operation returned by the service.
* @sample AmazonIdentityManagementAsyncHandler.GetAccountSummary
*/
java.util.concurrent.Future getAccountSummaryAsync(
GetAccountSummaryRequest getAccountSummaryRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Simplified method form for invoking the GetAccountSummary operation.
*
* @see #getAccountSummaryAsync(GetAccountSummaryRequest)
*/
java.util.concurrent.Future getAccountSummaryAsync();
/**
* Simplified method form for invoking the GetAccountSummary operation with
* an AsyncHandler.
*
* @see #getAccountSummaryAsync(GetAccountSummaryRequest,
* com.amazonaws.handlers.AsyncHandler)
*/
java.util.concurrent.Future getAccountSummaryAsync(
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets a list of all of the context keys referenced in the input policies.
* The policies are supplied as a list of one or more strings. To get the
* context keys from policies associated with an IAM user, group, or role,
* use GetContextKeysForPrincipalPolicy.
*
*
* Context keys are variables maintained by AWS and its services that
* provide details about the context of an API query request, and can be
* evaluated by testing against a value specified in an IAM policy. Use
* GetContextKeysForCustomPolicy to understand what key names and values you
* must supply when you call SimulateCustomPolicy. Note that all
* parameters are shown in unencoded form here for clarity, but must be URL
* encoded to be included as a part of a real HTML request.
*
*
* @param getContextKeysForCustomPolicyRequest
* @return A Java Future containing the result of the
* GetContextKeysForCustomPolicy operation returned by the service.
* @sample AmazonIdentityManagementAsync.GetContextKeysForCustomPolicy
*/
java.util.concurrent.Future getContextKeysForCustomPolicyAsync(
GetContextKeysForCustomPolicyRequest getContextKeysForCustomPolicyRequest);
/**
*
* Gets a list of all of the context keys referenced in the input policies.
* The policies are supplied as a list of one or more strings. To get the
* context keys from policies associated with an IAM user, group, or role,
* use GetContextKeysForPrincipalPolicy.
*
*
* Context keys are variables maintained by AWS and its services that
* provide details about the context of an API query request, and can be
* evaluated by testing against a value specified in an IAM policy. Use
* GetContextKeysForCustomPolicy to understand what key names and values you
* must supply when you call SimulateCustomPolicy. Note that all
* parameters are shown in unencoded form here for clarity, but must be URL
* encoded to be included as a part of a real HTML request.
*
*
* @param getContextKeysForCustomPolicyRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the
* GetContextKeysForCustomPolicy operation returned by the service.
* @sample
* AmazonIdentityManagementAsyncHandler.GetContextKeysForCustomPolicy
*/
java.util.concurrent.Future getContextKeysForCustomPolicyAsync(
GetContextKeysForCustomPolicyRequest getContextKeysForCustomPolicyRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets a list of all of the context keys referenced in all of the IAM
* policies attached to the specified IAM entity. The entity can be an IAM
* user, group, or role. If you specify a user, then the request also
* includes all of the policies attached to groups that the user is a member
* of.
*
*
* You can optionally include a list of one or more additional policies,
* specified as strings. If you want to include only a list of
* policies by string, use GetContextKeysForCustomPolicy instead.
*
*
* Note: This API discloses information about the permissions granted
* to other users. If you do not want users to see other user's permissions,
* then consider allowing them to use GetContextKeysForCustomPolicy
* instead.
*
*
* Context keys are variables maintained by AWS and its services that
* provide details about the context of an API query request, and can be
* evaluated by testing against a value in an IAM policy. Use
* GetContextKeysForPrincipalPolicy to understand what key names and
* values you must supply when you call SimulatePrincipalPolicy.
*
*
* @param getContextKeysForPrincipalPolicyRequest
* @return A Java Future containing the result of the
* GetContextKeysForPrincipalPolicy operation returned by the
* service.
* @sample AmazonIdentityManagementAsync.GetContextKeysForPrincipalPolicy
*/
java.util.concurrent.Future getContextKeysForPrincipalPolicyAsync(
GetContextKeysForPrincipalPolicyRequest getContextKeysForPrincipalPolicyRequest);
/**
*
* Gets a list of all of the context keys referenced in all of the IAM
* policies attached to the specified IAM entity. The entity can be an IAM
* user, group, or role. If you specify a user, then the request also
* includes all of the policies attached to groups that the user is a member
* of.
*
*
* You can optionally include a list of one or more additional policies,
* specified as strings. If you want to include only a list of
* policies by string, use GetContextKeysForCustomPolicy instead.
*
*
* Note: This API discloses information about the permissions granted
* to other users. If you do not want users to see other user's permissions,
* then consider allowing them to use GetContextKeysForCustomPolicy
* instead.
*
*
* Context keys are variables maintained by AWS and its services that
* provide details about the context of an API query request, and can be
* evaluated by testing against a value in an IAM policy. Use
* GetContextKeysForPrincipalPolicy to understand what key names and
* values you must supply when you call SimulatePrincipalPolicy.
*
*
* @param getContextKeysForPrincipalPolicyRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the
* GetContextKeysForPrincipalPolicy operation returned by the
* service.
* @sample
* AmazonIdentityManagementAsyncHandler.GetContextKeysForPrincipalPolicy
*/
java.util.concurrent.Future getContextKeysForPrincipalPolicyAsync(
GetContextKeysForPrincipalPolicyRequest getContextKeysForPrincipalPolicyRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves a credential report for the AWS account. For more information
* about the credential report, see Getting Credential Reports in the IAM User Guide.
*
*
* @param getCredentialReportRequest
* @return A Java Future containing the result of the GetCredentialReport
* operation returned by the service.
* @sample AmazonIdentityManagementAsync.GetCredentialReport
*/
java.util.concurrent.Future getCredentialReportAsync(
GetCredentialReportRequest getCredentialReportRequest);
/**
*
* Retrieves a credential report for the AWS account. For more information
* about the credential report, see Getting Credential Reports in the IAM User Guide.
*
*
* @param getCredentialReportRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetCredentialReport
* operation returned by the service.
* @sample AmazonIdentityManagementAsyncHandler.GetCredentialReport
*/
java.util.concurrent.Future getCredentialReportAsync(
GetCredentialReportRequest getCredentialReportRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Simplified method form for invoking the GetCredentialReport operation.
*
* @see #getCredentialReportAsync(GetCredentialReportRequest)
*/
java.util.concurrent.Future getCredentialReportAsync();
/**
* Simplified method form for invoking the GetCredentialReport operation
* with an AsyncHandler.
*
* @see #getCredentialReportAsync(GetCredentialReportRequest,
* com.amazonaws.handlers.AsyncHandler)
*/
java.util.concurrent.Future getCredentialReportAsync(
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of IAM users that are in the specified IAM group. You can
* paginate the results using the MaxItems
and
* Marker
parameters.
*
*
* @param getGroupRequest
* @return A Java Future containing the result of the GetGroup operation
* returned by the service.
* @sample AmazonIdentityManagementAsync.GetGroup
*/
java.util.concurrent.Future getGroupAsync(
GetGroupRequest getGroupRequest);
/**
*
* Returns a list of IAM users that are in the specified IAM group. You can
* paginate the results using the MaxItems
and
* Marker
parameters.
*
*
* @param getGroupRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetGroup operation
* returned by the service.
* @sample AmazonIdentityManagementAsyncHandler.GetGroup
*/
java.util.concurrent.Future getGroupAsync(
GetGroupRequest getGroupRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves the specified inline policy document that is embedded in the
* specified IAM group.
*
*
* An IAM group can also have managed policies attached to it. To retrieve a
* managed policy document that is attached to a group, use GetPolicy
* to determine the policy's default version, then use
* GetPolicyVersion to retrieve the policy document.
*
*
* For more information about policies, see Managed Policies and Inline Policies in the IAM User Guide.
*
*
* @param getGroupPolicyRequest
* @return A Java Future containing the result of the GetGroupPolicy
* operation returned by the service.
* @sample AmazonIdentityManagementAsync.GetGroupPolicy
*/
java.util.concurrent.Future getGroupPolicyAsync(
GetGroupPolicyRequest getGroupPolicyRequest);
/**
*
* Retrieves the specified inline policy document that is embedded in the
* specified IAM group.
*
*
* An IAM group can also have managed policies attached to it. To retrieve a
* managed policy document that is attached to a group, use GetPolicy
* to determine the policy's default version, then use
* GetPolicyVersion to retrieve the policy document.
*
*
* For more information about policies, see Managed Policies and Inline Policies in the IAM User Guide.
*
*
* @param getGroupPolicyRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetGroupPolicy
* operation returned by the service.
* @sample AmazonIdentityManagementAsyncHandler.GetGroupPolicy
*/
java.util.concurrent.Future getGroupPolicyAsync(
GetGroupPolicyRequest getGroupPolicyRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves information about the specified instance profile, including the
* instance profile's path, GUID, ARN, and role. For more information about
* instance profiles, see About Instance Profiles in the IAM User Guide.
*
*
* @param getInstanceProfileRequest
* @return A Java Future containing the result of the GetInstanceProfile
* operation returned by the service.
* @sample AmazonIdentityManagementAsync.GetInstanceProfile
*/
java.util.concurrent.Future getInstanceProfileAsync(
GetInstanceProfileRequest getInstanceProfileRequest);
/**
*
* Retrieves information about the specified instance profile, including the
* instance profile's path, GUID, ARN, and role. For more information about
* instance profiles, see About Instance Profiles in the IAM User Guide.
*
*
* @param getInstanceProfileRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetInstanceProfile
* operation returned by the service.
* @sample AmazonIdentityManagementAsyncHandler.GetInstanceProfile
*/
java.util.concurrent.Future getInstanceProfileAsync(
GetInstanceProfileRequest getInstanceProfileRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves the user name and password-creation date for the specified IAM
* user. If the user has not been assigned a password, the action returns a
* 404 (NoSuchEntity
) error.
*
*
* @param getLoginProfileRequest
* @return A Java Future containing the result of the GetLoginProfile
* operation returned by the service.
* @sample AmazonIdentityManagementAsync.GetLoginProfile
*/
java.util.concurrent.Future getLoginProfileAsync(
GetLoginProfileRequest getLoginProfileRequest);
/**
*
* Retrieves the user name and password-creation date for the specified IAM
* user. If the user has not been assigned a password, the action returns a
* 404 (NoSuchEntity
) error.
*
*
* @param getLoginProfileRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetLoginProfile
* operation returned by the service.
* @sample AmazonIdentityManagementAsyncHandler.GetLoginProfile
*/
java.util.concurrent.Future getLoginProfileAsync(
GetLoginProfileRequest getLoginProfileRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns information about the specified OpenID Connect (OIDC) provider
* resource object in IAM.
*
*
* @param getOpenIDConnectProviderRequest
* @return A Java Future containing the result of the
* GetOpenIDConnectProvider operation returned by the service.
* @sample AmazonIdentityManagementAsync.GetOpenIDConnectProvider
*/
java.util.concurrent.Future getOpenIDConnectProviderAsync(
GetOpenIDConnectProviderRequest getOpenIDConnectProviderRequest);
/**
*
* Returns information about the specified OpenID Connect (OIDC) provider
* resource object in IAM.
*
*
* @param getOpenIDConnectProviderRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the
* GetOpenIDConnectProvider operation returned by the service.
* @sample AmazonIdentityManagementAsyncHandler.GetOpenIDConnectProvider
*/
java.util.concurrent.Future getOpenIDConnectProviderAsync(
GetOpenIDConnectProviderRequest getOpenIDConnectProviderRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves information about the specified managed policy, including the
* policy's default version and the total number of IAM users, groups, and
* roles to which the policy is attached. To retrieve the list of the
* specific users, groups, and roles that the policy is attached to, use the
* ListEntitiesForPolicy API. This API returns metadata about the
* policy. To retrieve the actual policy document for a specific version of
* the policy, use GetPolicyVersion.
*
*
* This API retrieves information about managed policies. To retrieve
* information about an inline policy that is embedded with an IAM user,
* group, or role, use the GetUserPolicy, GetGroupPolicy, or
* GetRolePolicy API.
*
*
* For more information about policies, see Managed Policies and Inline Policies in the IAM User Guide.
*
*
* @param getPolicyRequest
* @return A Java Future containing the result of the GetPolicy operation
* returned by the service.
* @sample AmazonIdentityManagementAsync.GetPolicy
*/
java.util.concurrent.Future getPolicyAsync(
GetPolicyRequest getPolicyRequest);
/**
*
* Retrieves information about the specified managed policy, including the
* policy's default version and the total number of IAM users, groups, and
* roles to which the policy is attached. To retrieve the list of the
* specific users, groups, and roles that the policy is attached to, use the
* ListEntitiesForPolicy API. This API returns metadata about the
* policy. To retrieve the actual policy document for a specific version of
* the policy, use GetPolicyVersion.
*
*
* This API retrieves information about managed policies. To retrieve
* information about an inline policy that is embedded with an IAM user,
* group, or role, use the GetUserPolicy, GetGroupPolicy, or
* GetRolePolicy API.
*
*
* For more information about policies, see Managed Policies and Inline Policies in the IAM User Guide.
*
*
* @param getPolicyRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetPolicy operation
* returned by the service.
* @sample AmazonIdentityManagementAsyncHandler.GetPolicy
*/
java.util.concurrent.Future getPolicyAsync(
GetPolicyRequest getPolicyRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves information about the specified version of the specified
* managed policy, including the policy document.
*
*
* To list the available versions for a policy, use
* ListPolicyVersions.
*
*
* This API retrieves information about managed policies. To retrieve
* information about an inline policy that is embedded in a user, group, or
* role, use the GetUserPolicy, GetGroupPolicy, or
* GetRolePolicy API.
*
*
* For more information about the types of policies, see Managed Policies and Inline Policies in the IAM User Guide.
*
*
* For more information about managed policy versions, see Versioning for Managed Policies in the IAM User Guide.
*
*
* @param getPolicyVersionRequest
* @return A Java Future containing the result of the GetPolicyVersion
* operation returned by the service.
* @sample AmazonIdentityManagementAsync.GetPolicyVersion
*/
java.util.concurrent.Future getPolicyVersionAsync(
GetPolicyVersionRequest getPolicyVersionRequest);
/**
*
* Retrieves information about the specified version of the specified
* managed policy, including the policy document.
*
*
* To list the available versions for a policy, use
* ListPolicyVersions.
*
*
* This API retrieves information about managed policies. To retrieve
* information about an inline policy that is embedded in a user, group, or
* role, use the GetUserPolicy, GetGroupPolicy, or
* GetRolePolicy API.
*
*
* For more information about the types of policies, see Managed Policies and Inline Policies in the IAM User Guide.
*
*
* For more information about managed policy versions, see Versioning for Managed Policies in the IAM User Guide.
*
*
* @param getPolicyVersionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetPolicyVersion
* operation returned by the service.
* @sample AmazonIdentityManagementAsyncHandler.GetPolicyVersion
*/
java.util.concurrent.Future getPolicyVersionAsync(
GetPolicyVersionRequest getPolicyVersionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves information about the specified role, including the role's
* path, GUID, ARN, and the role's trust policy that grants permission to
* assume the role. For more information about roles, see Working with Roles.
*
*
* @param getRoleRequest
* @return A Java Future containing the result of the GetRole operation
* returned by the service.
* @sample AmazonIdentityManagementAsync.GetRole
*/
java.util.concurrent.Future getRoleAsync(
GetRoleRequest getRoleRequest);
/**
*
* Retrieves information about the specified role, including the role's
* path, GUID, ARN, and the role's trust policy that grants permission to
* assume the role. For more information about roles, see Working with Roles.
*
*
* @param getRoleRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetRole operation
* returned by the service.
* @sample AmazonIdentityManagementAsyncHandler.GetRole
*/
java.util.concurrent.Future getRoleAsync(
GetRoleRequest getRoleRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves the specified inline policy document that is embedded with the
* specified IAM role.
*
*
* An IAM role can also have managed policies attached to it. To retrieve a
* managed policy document that is attached to a role, use GetPolicy
* to determine the policy's default version, then use
* GetPolicyVersion to retrieve the policy document.
*
*
* For more information about policies, see Managed Policies and Inline Policies in the IAM User Guide.
*
*
* For more information about roles, see Using Roles to Delegate Permissions and Federate Identities.
*
*
* @param getRolePolicyRequest
* @return A Java Future containing the result of the GetRolePolicy
* operation returned by the service.
* @sample AmazonIdentityManagementAsync.GetRolePolicy
*/
java.util.concurrent.Future getRolePolicyAsync(
GetRolePolicyRequest getRolePolicyRequest);
/**
*
* Retrieves the specified inline policy document that is embedded with the
* specified IAM role.
*
*
* An IAM role can also have managed policies attached to it. To retrieve a
* managed policy document that is attached to a role, use GetPolicy
* to determine the policy's default version, then use
* GetPolicyVersion to retrieve the policy document.
*
*
* For more information about policies, see Managed Policies and Inline Policies in the IAM User Guide.
*
*
* For more information about roles, see Using Roles to Delegate Permissions and Federate Identities.
*
*
* @param getRolePolicyRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetRolePolicy
* operation returned by the service.
* @sample AmazonIdentityManagementAsyncHandler.GetRolePolicy
*/
java.util.concurrent.Future getRolePolicyAsync(
GetRolePolicyRequest getRolePolicyRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns the SAML provider metadocument that was uploaded when the IAM
* SAML provider resource object was created or updated.
*
*
*
* This operation requires Signature Version 4.
*
*
*
* @param getSAMLProviderRequest
* @return A Java Future containing the result of the GetSAMLProvider
* operation returned by the service.
* @sample AmazonIdentityManagementAsync.GetSAMLProvider
*/
java.util.concurrent.Future getSAMLProviderAsync(
GetSAMLProviderRequest getSAMLProviderRequest);
/**
*
* Returns the SAML provider metadocument that was uploaded when the IAM
* SAML provider resource object was created or updated.
*
*
*
* This operation requires Signature Version 4.
*
*
*
* @param getSAMLProviderRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetSAMLProvider
* operation returned by the service.
* @sample AmazonIdentityManagementAsyncHandler.GetSAMLProvider
*/
java.util.concurrent.Future getSAMLProviderAsync(
GetSAMLProviderRequest getSAMLProviderRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves the specified SSH public key, including metadata about the key.
*
*
* The SSH public key retrieved by this action is used only for
* authenticating the associated IAM user to an AWS CodeCommit repository.
* For more information about using SSH keys to authenticate to an AWS
* CodeCommit repository, see Set up AWS CodeCommit for SSH Connections in the AWS CodeCommit
* User Guide.
*
*
* @param getSSHPublicKeyRequest
* @return A Java Future containing the result of the GetSSHPublicKey
* operation returned by the service.
* @sample AmazonIdentityManagementAsync.GetSSHPublicKey
*/
java.util.concurrent.Future getSSHPublicKeyAsync(
GetSSHPublicKeyRequest getSSHPublicKeyRequest);
/**
*
* Retrieves the specified SSH public key, including metadata about the key.
*
*
* The SSH public key retrieved by this action is used only for
* authenticating the associated IAM user to an AWS CodeCommit repository.
* For more information about using SSH keys to authenticate to an AWS
* CodeCommit repository, see Set up AWS CodeCommit for SSH Connections in the AWS CodeCommit
* User Guide.
*
*
* @param getSSHPublicKeyRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetSSHPublicKey
* operation returned by the service.
* @sample AmazonIdentityManagementAsyncHandler.GetSSHPublicKey
*/
java.util.concurrent.Future getSSHPublicKeyAsync(
GetSSHPublicKeyRequest getSSHPublicKeyRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves information about the specified server certificate stored in
* IAM.
*
*
* For more information about working with server certificates, including a
* list of AWS services that can use the server certificates that you manage
* with IAM, go to Working with Server Certificates in the IAM User Guide.
*
*
* @param getServerCertificateRequest
* @return A Java Future containing the result of the GetServerCertificate
* operation returned by the service.
* @sample AmazonIdentityManagementAsync.GetServerCertificate
*/
java.util.concurrent.Future getServerCertificateAsync(
GetServerCertificateRequest getServerCertificateRequest);
/**
*
* Retrieves information about the specified server certificate stored in
* IAM.
*
*
* For more information about working with server certificates, including a
* list of AWS services that can use the server certificates that you manage
* with IAM, go to Working with Server Certificates in the IAM User Guide.
*
*
* @param getServerCertificateRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetServerCertificate
* operation returned by the service.
* @sample AmazonIdentityManagementAsyncHandler.GetServerCertificate
*/
java.util.concurrent.Future getServerCertificateAsync(
GetServerCertificateRequest getServerCertificateRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves information about the specified IAM user, including the user's
* creation date, path, unique ID, and ARN.
*
*
* If you do not specify a user name, IAM determines the user name
* implicitly based on the AWS access key ID used to sign the request to
* this API.
*
*
* @param getUserRequest
* @return A Java Future containing the result of the GetUser operation
* returned by the service.
* @sample AmazonIdentityManagementAsync.GetUser
*/
java.util.concurrent.Future getUserAsync(
GetUserRequest getUserRequest);
/**
*
* Retrieves information about the specified IAM user, including the user's
* creation date, path, unique ID, and ARN.
*
*
* If you do not specify a user name, IAM determines the user name
* implicitly based on the AWS access key ID used to sign the request to
* this API.
*
*
* @param getUserRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetUser operation
* returned by the service.
* @sample AmazonIdentityManagementAsyncHandler.GetUser
*/
java.util.concurrent.Future getUserAsync(
GetUserRequest getUserRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Simplified method form for invoking the GetUser operation.
*
* @see #getUserAsync(GetUserRequest)
*/
java.util.concurrent.Future getUserAsync();
/**
* Simplified method form for invoking the GetUser operation with an
* AsyncHandler.
*
* @see #getUserAsync(GetUserRequest, com.amazonaws.handlers.AsyncHandler)
*/
java.util.concurrent.Future getUserAsync(
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves the specified inline policy document that is embedded in the
* specified IAM user.
*
*
* An IAM user can also have managed policies attached to it. To retrieve a
* managed policy document that is attached to a user, use GetPolicy
* to determine the policy's default version, then use
* GetPolicyVersion to retrieve the policy document.
*
*
* For more information about policies, see Managed Policies and Inline Policies in the IAM User Guide.
*
*
* @param getUserPolicyRequest
* @return A Java Future containing the result of the GetUserPolicy
* operation returned by the service.
* @sample AmazonIdentityManagementAsync.GetUserPolicy
*/
java.util.concurrent.Future getUserPolicyAsync(
GetUserPolicyRequest getUserPolicyRequest);
/**
*
* Retrieves the specified inline policy document that is embedded in the
* specified IAM user.
*
*
* An IAM user can also have managed policies attached to it. To retrieve a
* managed policy document that is attached to a user, use GetPolicy
* to determine the policy's default version, then use
* GetPolicyVersion to retrieve the policy document.
*
*
* For more information about policies, see Managed Policies and Inline Policies in the IAM User Guide.
*
*
* @param getUserPolicyRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetUserPolicy
* operation returned by the service.
* @sample AmazonIdentityManagementAsyncHandler.GetUserPolicy
*/
java.util.concurrent.Future getUserPolicyAsync(
GetUserPolicyRequest getUserPolicyRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns information about the access key IDs associated with the
* specified IAM user. If there are none, the action returns an empty list.
*
*
* Although each user is limited to a small number of keys, you can still
* paginate the results using the MaxItems
and
* Marker
parameters.
*
*
* If the UserName
field is not specified, the UserName is
* determined implicitly based on the AWS access key ID used to sign the
* request. Because this action works for access keys under the AWS account,
* you can use this action to manage root credentials even if the AWS
* account has no associated users.
*
*
*
* To ensure the security of your AWS account, the secret access key is
* accessible only during key and user creation.
*
*
*
* @param listAccessKeysRequest
* @return A Java Future containing the result of the ListAccessKeys
* operation returned by the service.
* @sample AmazonIdentityManagementAsync.ListAccessKeys
*/
java.util.concurrent.Future listAccessKeysAsync(
ListAccessKeysRequest listAccessKeysRequest);
/**
*
* Returns information about the access key IDs associated with the
* specified IAM user. If there are none, the action returns an empty list.
*
*
* Although each user is limited to a small number of keys, you can still
* paginate the results using the MaxItems
and
* Marker
parameters.
*
*
* If the UserName
field is not specified, the UserName is
* determined implicitly based on the AWS access key ID used to sign the
* request. Because this action works for access keys under the AWS account,
* you can use this action to manage root credentials even if the AWS
* account has no associated users.
*
*
*
* To ensure the security of your AWS account, the secret access key is
* accessible only during key and user creation.
*
*
*
* @param listAccessKeysRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListAccessKeys
* operation returned by the service.
* @sample AmazonIdentityManagementAsyncHandler.ListAccessKeys
*/
java.util.concurrent.Future listAccessKeysAsync(
ListAccessKeysRequest listAccessKeysRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Simplified method form for invoking the ListAccessKeys operation.
*
* @see #listAccessKeysAsync(ListAccessKeysRequest)
*/
java.util.concurrent.Future listAccessKeysAsync();
/**
* Simplified method form for invoking the ListAccessKeys operation with an
* AsyncHandler.
*
* @see #listAccessKeysAsync(ListAccessKeysRequest,
* com.amazonaws.handlers.AsyncHandler)
*/
java.util.concurrent.Future listAccessKeysAsync(
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists the account alias associated with the AWS account (Note: you can
* have only one). For information about using an AWS account alias, see
* Using an Alias for Your AWS Account ID in the IAM User Guide.
*
*
* @param listAccountAliasesRequest
* @return A Java Future containing the result of the ListAccountAliases
* operation returned by the service.
* @sample AmazonIdentityManagementAsync.ListAccountAliases
*/
java.util.concurrent.Future listAccountAliasesAsync(
ListAccountAliasesRequest listAccountAliasesRequest);
/**
*
* Lists the account alias associated with the AWS account (Note: you can
* have only one). For information about using an AWS account alias, see
* Using an Alias for Your AWS Account ID in the IAM User Guide.
*
*
* @param listAccountAliasesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListAccountAliases
* operation returned by the service.
* @sample AmazonIdentityManagementAsyncHandler.ListAccountAliases
*/
java.util.concurrent.Future listAccountAliasesAsync(
ListAccountAliasesRequest listAccountAliasesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Simplified method form for invoking the ListAccountAliases operation.
*
* @see #listAccountAliasesAsync(ListAccountAliasesRequest)
*/
java.util.concurrent.Future listAccountAliasesAsync();
/**
* Simplified method form for invoking the ListAccountAliases operation with
* an AsyncHandler.
*
* @see #listAccountAliasesAsync(ListAccountAliasesRequest,
* com.amazonaws.handlers.AsyncHandler)
*/
java.util.concurrent.Future listAccountAliasesAsync(
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists all managed policies that are attached to the specified IAM group.
*
*
* An IAM group can also have inline policies embedded with it. To list the
* inline policies for a group, use the ListGroupPolicies API. For
* information about policies, see Managed Policies and Inline Policies in the IAM User Guide.
*
*
* You can paginate the results using the MaxItems
and
* Marker
parameters. You can use the PathPrefix
* parameter to limit the list of policies to only those matching the
* specified path prefix. If there are no policies attached to the specified
* group (or none that match the specified path prefix), the action returns
* an empty list.
*
*
* @param listAttachedGroupPoliciesRequest
* @return A Java Future containing the result of the
* ListAttachedGroupPolicies operation returned by the service.
* @sample AmazonIdentityManagementAsync.ListAttachedGroupPolicies
*/
java.util.concurrent.Future listAttachedGroupPoliciesAsync(
ListAttachedGroupPoliciesRequest listAttachedGroupPoliciesRequest);
/**
*
* Lists all managed policies that are attached to the specified IAM group.
*
*
* An IAM group can also have inline policies embedded with it. To list the
* inline policies for a group, use the ListGroupPolicies API. For
* information about policies, see Managed Policies and Inline Policies in the IAM User Guide.
*
*
* You can paginate the results using the MaxItems
and
* Marker
parameters. You can use the PathPrefix
* parameter to limit the list of policies to only those matching the
* specified path prefix. If there are no policies attached to the specified
* group (or none that match the specified path prefix), the action returns
* an empty list.
*
*
* @param listAttachedGroupPoliciesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the
* ListAttachedGroupPolicies operation returned by the service.
* @sample AmazonIdentityManagementAsyncHandler.ListAttachedGroupPolicies
*/
java.util.concurrent.Future listAttachedGroupPoliciesAsync(
ListAttachedGroupPoliciesRequest listAttachedGroupPoliciesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists all managed policies that are attached to the specified IAM role.
*
*
* An IAM role can also have inline policies embedded with it. To list the
* inline policies for a role, use the ListRolePolicies API. For
* information about policies, see Managed Policies and Inline Policies in the IAM User Guide.
*
*
* You can paginate the results using the MaxItems
and
* Marker
parameters. You can use the PathPrefix
* parameter to limit the list of policies to only those matching the
* specified path prefix. If there are no policies attached to the specified
* role (or none that match the specified path prefix), the action returns
* an empty list.
*
*
* @param listAttachedRolePoliciesRequest
* @return A Java Future containing the result of the
* ListAttachedRolePolicies operation returned by the service.
* @sample AmazonIdentityManagementAsync.ListAttachedRolePolicies
*/
java.util.concurrent.Future listAttachedRolePoliciesAsync(
ListAttachedRolePoliciesRequest listAttachedRolePoliciesRequest);
/**
*
* Lists all managed policies that are attached to the specified IAM role.
*
*
* An IAM role can also have inline policies embedded with it. To list the
* inline policies for a role, use the ListRolePolicies API. For
* information about policies, see Managed Policies and Inline Policies in the IAM User Guide.
*
*
* You can paginate the results using the MaxItems
and
* Marker
parameters. You can use the PathPrefix
* parameter to limit the list of policies to only those matching the
* specified path prefix. If there are no policies attached to the specified
* role (or none that match the specified path prefix), the action returns
* an empty list.
*
*
* @param listAttachedRolePoliciesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the
* ListAttachedRolePolicies operation returned by the service.
* @sample AmazonIdentityManagementAsyncHandler.ListAttachedRolePolicies
*/
java.util.concurrent.Future listAttachedRolePoliciesAsync(
ListAttachedRolePoliciesRequest listAttachedRolePoliciesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists all managed policies that are attached to the specified IAM user.
*
*
* An IAM user can also have inline policies embedded with it. To list the
* inline policies for a user, use the ListUserPolicies API. For
* information about policies, see Managed Policies and Inline Policies in the IAM User Guide.
*
*
* You can paginate the results using the MaxItems
and
* Marker
parameters. You can use the PathPrefix
* parameter to limit the list of policies to only those matching the
* specified path prefix. If there are no policies attached to the specified
* group (or none that match the specified path prefix), the action returns
* an empty list.
*
*
* @param listAttachedUserPoliciesRequest
* @return A Java Future containing the result of the
* ListAttachedUserPolicies operation returned by the service.
* @sample AmazonIdentityManagementAsync.ListAttachedUserPolicies
*/
java.util.concurrent.Future listAttachedUserPoliciesAsync(
ListAttachedUserPoliciesRequest listAttachedUserPoliciesRequest);
/**
*
* Lists all managed policies that are attached to the specified IAM user.
*
*
* An IAM user can also have inline policies embedded with it. To list the
* inline policies for a user, use the ListUserPolicies API. For
* information about policies, see Managed Policies and Inline Policies in the IAM User Guide.
*
*
* You can paginate the results using the MaxItems
and
* Marker
parameters. You can use the PathPrefix
* parameter to limit the list of policies to only those matching the
* specified path prefix. If there are no policies attached to the specified
* group (or none that match the specified path prefix), the action returns
* an empty list.
*
*
* @param listAttachedUserPoliciesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the
* ListAttachedUserPolicies operation returned by the service.
* @sample AmazonIdentityManagementAsyncHandler.ListAttachedUserPolicies
*/
java.util.concurrent.Future listAttachedUserPoliciesAsync(
ListAttachedUserPoliciesRequest listAttachedUserPoliciesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists all IAM users, groups, and roles that the specified managed policy
* is attached to.
*
*
* You can use the optional EntityFilter
parameter to limit the
* results to a particular type of entity (users, groups, or roles). For
* example, to list only the roles that are attached to the specified
* policy, set EntityFilter
to Role
.
*
*
* You can paginate the results using the MaxItems
and
* Marker
parameters.
*
*
* @param listEntitiesForPolicyRequest
* @return A Java Future containing the result of the ListEntitiesForPolicy
* operation returned by the service.
* @sample AmazonIdentityManagementAsync.ListEntitiesForPolicy
*/
java.util.concurrent.Future listEntitiesForPolicyAsync(
ListEntitiesForPolicyRequest listEntitiesForPolicyRequest);
/**
*
* Lists all IAM users, groups, and roles that the specified managed policy
* is attached to.
*
*
* You can use the optional EntityFilter
parameter to limit the
* results to a particular type of entity (users, groups, or roles). For
* example, to list only the roles that are attached to the specified
* policy, set EntityFilter
to Role
.
*
*
* You can paginate the results using the MaxItems
and
* Marker
parameters.
*
*
* @param listEntitiesForPolicyRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListEntitiesForPolicy
* operation returned by the service.
* @sample AmazonIdentityManagementAsyncHandler.ListEntitiesForPolicy
*/
java.util.concurrent.Future listEntitiesForPolicyAsync(
ListEntitiesForPolicyRequest listEntitiesForPolicyRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists the names of the inline policies that are embedded in the specified
* IAM group.
*
*
* An IAM group can also have managed policies attached to it. To list the
* managed policies that are attached to a group, use
* ListAttachedGroupPolicies. For more information about policies,
* see Managed Policies and Inline Policies in the IAM User Guide.
*
*
* You can paginate the results using the MaxItems
and
* Marker
parameters. If there are no inline policies embedded
* with the specified group, the action returns an empty list.
*
*
* @param listGroupPoliciesRequest
* @return A Java Future containing the result of the ListGroupPolicies
* operation returned by the service.
* @sample AmazonIdentityManagementAsync.ListGroupPolicies
*/
java.util.concurrent.Future listGroupPoliciesAsync(
ListGroupPoliciesRequest listGroupPoliciesRequest);
/**
*
* Lists the names of the inline policies that are embedded in the specified
* IAM group.
*
*
* An IAM group can also have managed policies attached to it. To list the
* managed policies that are attached to a group, use
* ListAttachedGroupPolicies. For more information about policies,
* see Managed Policies and Inline Policies in the IAM User Guide.
*
*
* You can paginate the results using the MaxItems
and
* Marker
parameters. If there are no inline policies embedded
* with the specified group, the action returns an empty list.
*
*
* @param listGroupPoliciesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListGroupPolicies
* operation returned by the service.
* @sample AmazonIdentityManagementAsyncHandler.ListGroupPolicies
*/
java.util.concurrent.Future listGroupPoliciesAsync(
ListGroupPoliciesRequest listGroupPoliciesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists the IAM groups that have the specified path prefix.
*
*
* You can paginate the results using the MaxItems
and
* Marker
parameters.
*
*
* @param listGroupsRequest
* @return A Java Future containing the result of the ListGroups operation
* returned by the service.
* @sample AmazonIdentityManagementAsync.ListGroups
*/
java.util.concurrent.Future listGroupsAsync(
ListGroupsRequest listGroupsRequest);
/**
*
* Lists the IAM groups that have the specified path prefix.
*
*
* You can paginate the results using the MaxItems
and
* Marker
parameters.
*
*
* @param listGroupsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListGroups operation
* returned by the service.
* @sample AmazonIdentityManagementAsyncHandler.ListGroups
*/
java.util.concurrent.Future listGroupsAsync(
ListGroupsRequest listGroupsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Simplified method form for invoking the ListGroups operation.
*
* @see #listGroupsAsync(ListGroupsRequest)
*/
java.util.concurrent.Future listGroupsAsync();
/**
* Simplified method form for invoking the ListGroups operation with an
* AsyncHandler.
*
* @see #listGroupsAsync(ListGroupsRequest,
* com.amazonaws.handlers.AsyncHandler)
*/
java.util.concurrent.Future