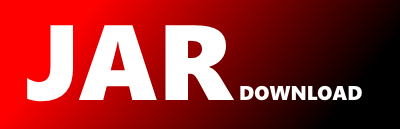
com.amazonaws.services.identitymanagement.model.ResourceSpecificResult Maven / Gradle / Ivy
Show all versions of aws-java-sdk-iam Show documentation
/*
* Copyright 2010-2016 Amazon.com, Inc. or its affiliates. All Rights
* Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License").
* You may not use this file except in compliance with the License.
* A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed
* on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either
* express or implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
package com.amazonaws.services.identitymanagement.model;
import java.io.Serializable;
/**
*
* Contains the result of the simulation of a single API action call on a single
* resource.
*
*
* This data type is used by a member of the EvaluationResult data type.
*
*/
public class ResourceSpecificResult implements Serializable, Cloneable {
/**
*
* The name of the simulated resource, in Amazon Resource Name (ARN) format.
*
*/
private String evalResourceName;
/**
*
* The result of the simulation of the simulated API action on the resource
* specified in EvalResourceName
.
*
*/
private String evalResourceDecision;
/**
*
* A list of the statements in the input policies that determine the result
* for this part of the simulation. Remember that even if multiple
* statements allow the action on the resource, if any statement
* denies that action, then the explicit deny overrides any allow, and the
* deny statement is the only entry included in the result.
*
*/
private com.amazonaws.internal.SdkInternalList matchedStatements;
/**
*
* A list of context keys that are required by the included input policies
* but that were not provided by one of the input parameters. This list is
* used when a list of ARNs is included in the ResourceArns
* parameter instead of "*". If you do not specify individual resources, by
* setting ResourceArns
to "*" or by not including the
* ResourceArns
parameter, then any missing context values are
* instead included under the EvaluationResults
section. To
* discover the context keys used by a set of policies, you can call
* GetContextKeysForCustomPolicy or
* GetContextKeysForPrincipalPolicy.
*
*/
private com.amazonaws.internal.SdkInternalList missingContextValues;
/**
*
* Additional details about the results of the evaluation decision. When
* there are both IAM policies and resource policies, this parameter
* explains how each set of policies contributes to the final evaluation
* decision. When simulating cross-account access to a resource, both the
* resource-based policy and the caller's IAM policy must grant access.
*
*/
private com.amazonaws.internal.SdkInternalMap evalDecisionDetails;
/**
*
* The name of the simulated resource, in Amazon Resource Name (ARN) format.
*
*
* @param evalResourceName
* The name of the simulated resource, in Amazon Resource Name (ARN)
* format.
*/
public void setEvalResourceName(String evalResourceName) {
this.evalResourceName = evalResourceName;
}
/**
*
* The name of the simulated resource, in Amazon Resource Name (ARN) format.
*
*
* @return The name of the simulated resource, in Amazon Resource Name (ARN)
* format.
*/
public String getEvalResourceName() {
return this.evalResourceName;
}
/**
*
* The name of the simulated resource, in Amazon Resource Name (ARN) format.
*
*
* @param evalResourceName
* The name of the simulated resource, in Amazon Resource Name (ARN)
* format.
* @return Returns a reference to this object so that method calls can be
* chained together.
*/
public ResourceSpecificResult withEvalResourceName(String evalResourceName) {
setEvalResourceName(evalResourceName);
return this;
}
/**
*
* The result of the simulation of the simulated API action on the resource
* specified in EvalResourceName
.
*
*
* @param evalResourceDecision
* The result of the simulation of the simulated API action on the
* resource specified in EvalResourceName
.
* @see PolicyEvaluationDecisionType
*/
public void setEvalResourceDecision(String evalResourceDecision) {
this.evalResourceDecision = evalResourceDecision;
}
/**
*
* The result of the simulation of the simulated API action on the resource
* specified in EvalResourceName
.
*
*
* @return The result of the simulation of the simulated API action on the
* resource specified in EvalResourceName
.
* @see PolicyEvaluationDecisionType
*/
public String getEvalResourceDecision() {
return this.evalResourceDecision;
}
/**
*
* The result of the simulation of the simulated API action on the resource
* specified in EvalResourceName
.
*
*
* @param evalResourceDecision
* The result of the simulation of the simulated API action on the
* resource specified in EvalResourceName
.
* @return Returns a reference to this object so that method calls can be
* chained together.
* @see PolicyEvaluationDecisionType
*/
public ResourceSpecificResult withEvalResourceDecision(
String evalResourceDecision) {
setEvalResourceDecision(evalResourceDecision);
return this;
}
/**
*
* The result of the simulation of the simulated API action on the resource
* specified in EvalResourceName
.
*
*
* @param evalResourceDecision
* The result of the simulation of the simulated API action on the
* resource specified in EvalResourceName
.
* @see PolicyEvaluationDecisionType
*/
public void setEvalResourceDecision(
PolicyEvaluationDecisionType evalResourceDecision) {
this.evalResourceDecision = evalResourceDecision.toString();
}
/**
*
* The result of the simulation of the simulated API action on the resource
* specified in EvalResourceName
.
*
*
* @param evalResourceDecision
* The result of the simulation of the simulated API action on the
* resource specified in EvalResourceName
.
* @return Returns a reference to this object so that method calls can be
* chained together.
* @see PolicyEvaluationDecisionType
*/
public ResourceSpecificResult withEvalResourceDecision(
PolicyEvaluationDecisionType evalResourceDecision) {
setEvalResourceDecision(evalResourceDecision);
return this;
}
/**
*
* A list of the statements in the input policies that determine the result
* for this part of the simulation. Remember that even if multiple
* statements allow the action on the resource, if any statement
* denies that action, then the explicit deny overrides any allow, and the
* deny statement is the only entry included in the result.
*
*
* @return A list of the statements in the input policies that determine the
* result for this part of the simulation. Remember that even if
* multiple statements allow the action on the resource, if
* any statement denies that action, then the explicit deny
* overrides any allow, and the deny statement is the only entry
* included in the result.
*/
public java.util.List getMatchedStatements() {
if (matchedStatements == null) {
matchedStatements = new com.amazonaws.internal.SdkInternalList();
}
return matchedStatements;
}
/**
*
* A list of the statements in the input policies that determine the result
* for this part of the simulation. Remember that even if multiple
* statements allow the action on the resource, if any statement
* denies that action, then the explicit deny overrides any allow, and the
* deny statement is the only entry included in the result.
*
*
* @param matchedStatements
* A list of the statements in the input policies that determine the
* result for this part of the simulation. Remember that even if
* multiple statements allow the action on the resource, if
* any statement denies that action, then the explicit deny
* overrides any allow, and the deny statement is the only entry
* included in the result.
*/
public void setMatchedStatements(
java.util.Collection matchedStatements) {
if (matchedStatements == null) {
this.matchedStatements = null;
return;
}
this.matchedStatements = new com.amazonaws.internal.SdkInternalList(
matchedStatements);
}
/**
*
* A list of the statements in the input policies that determine the result
* for this part of the simulation. Remember that even if multiple
* statements allow the action on the resource, if any statement
* denies that action, then the explicit deny overrides any allow, and the
* deny statement is the only entry included in the result.
*
*
* NOTE: This method appends the values to the existing list (if
* any). Use {@link #setMatchedStatements(java.util.Collection)} or
* {@link #withMatchedStatements(java.util.Collection)} if you want to
* override the existing values.
*
*
* @param matchedStatements
* A list of the statements in the input policies that determine the
* result for this part of the simulation. Remember that even if
* multiple statements allow the action on the resource, if
* any statement denies that action, then the explicit deny
* overrides any allow, and the deny statement is the only entry
* included in the result.
* @return Returns a reference to this object so that method calls can be
* chained together.
*/
public ResourceSpecificResult withMatchedStatements(
Statement... matchedStatements) {
if (this.matchedStatements == null) {
setMatchedStatements(new com.amazonaws.internal.SdkInternalList(
matchedStatements.length));
}
for (Statement ele : matchedStatements) {
this.matchedStatements.add(ele);
}
return this;
}
/**
*
* A list of the statements in the input policies that determine the result
* for this part of the simulation. Remember that even if multiple
* statements allow the action on the resource, if any statement
* denies that action, then the explicit deny overrides any allow, and the
* deny statement is the only entry included in the result.
*
*
* @param matchedStatements
* A list of the statements in the input policies that determine the
* result for this part of the simulation. Remember that even if
* multiple statements allow the action on the resource, if
* any statement denies that action, then the explicit deny
* overrides any allow, and the deny statement is the only entry
* included in the result.
* @return Returns a reference to this object so that method calls can be
* chained together.
*/
public ResourceSpecificResult withMatchedStatements(
java.util.Collection matchedStatements) {
setMatchedStatements(matchedStatements);
return this;
}
/**
*
* A list of context keys that are required by the included input policies
* but that were not provided by one of the input parameters. This list is
* used when a list of ARNs is included in the ResourceArns
* parameter instead of "*". If you do not specify individual resources, by
* setting ResourceArns
to "*" or by not including the
* ResourceArns
parameter, then any missing context values are
* instead included under the EvaluationResults
section. To
* discover the context keys used by a set of policies, you can call
* GetContextKeysForCustomPolicy or
* GetContextKeysForPrincipalPolicy.
*
*
* @return A list of context keys that are required by the included input
* policies but that were not provided by one of the input
* parameters. This list is used when a list of ARNs is included in
* the ResourceArns
parameter instead of "*". If you do
* not specify individual resources, by setting
* ResourceArns
to "*" or by not including the
* ResourceArns
parameter, then any missing context
* values are instead included under the
* EvaluationResults
section. To discover the context
* keys used by a set of policies, you can call
* GetContextKeysForCustomPolicy or
* GetContextKeysForPrincipalPolicy.
*/
public java.util.List getMissingContextValues() {
if (missingContextValues == null) {
missingContextValues = new com.amazonaws.internal.SdkInternalList();
}
return missingContextValues;
}
/**
*
* A list of context keys that are required by the included input policies
* but that were not provided by one of the input parameters. This list is
* used when a list of ARNs is included in the ResourceArns
* parameter instead of "*". If you do not specify individual resources, by
* setting ResourceArns
to "*" or by not including the
* ResourceArns
parameter, then any missing context values are
* instead included under the EvaluationResults
section. To
* discover the context keys used by a set of policies, you can call
* GetContextKeysForCustomPolicy or
* GetContextKeysForPrincipalPolicy.
*
*
* @param missingContextValues
* A list of context keys that are required by the included input
* policies but that were not provided by one of the input
* parameters. This list is used when a list of ARNs is included in
* the ResourceArns
parameter instead of "*". If you do
* not specify individual resources, by setting
* ResourceArns
to "*" or by not including the
* ResourceArns
parameter, then any missing context
* values are instead included under the
* EvaluationResults
section. To discover the context
* keys used by a set of policies, you can call
* GetContextKeysForCustomPolicy or
* GetContextKeysForPrincipalPolicy.
*/
public void setMissingContextValues(
java.util.Collection missingContextValues) {
if (missingContextValues == null) {
this.missingContextValues = null;
return;
}
this.missingContextValues = new com.amazonaws.internal.SdkInternalList(
missingContextValues);
}
/**
*
* A list of context keys that are required by the included input policies
* but that were not provided by one of the input parameters. This list is
* used when a list of ARNs is included in the ResourceArns
* parameter instead of "*". If you do not specify individual resources, by
* setting ResourceArns
to "*" or by not including the
* ResourceArns
parameter, then any missing context values are
* instead included under the EvaluationResults
section. To
* discover the context keys used by a set of policies, you can call
* GetContextKeysForCustomPolicy or
* GetContextKeysForPrincipalPolicy.
*
*
* NOTE: This method appends the values to the existing list (if
* any). Use {@link #setMissingContextValues(java.util.Collection)} or
* {@link #withMissingContextValues(java.util.Collection)} if you want to
* override the existing values.
*
*
* @param missingContextValues
* A list of context keys that are required by the included input
* policies but that were not provided by one of the input
* parameters. This list is used when a list of ARNs is included in
* the ResourceArns
parameter instead of "*". If you do
* not specify individual resources, by setting
* ResourceArns
to "*" or by not including the
* ResourceArns
parameter, then any missing context
* values are instead included under the
* EvaluationResults
section. To discover the context
* keys used by a set of policies, you can call
* GetContextKeysForCustomPolicy or
* GetContextKeysForPrincipalPolicy.
* @return Returns a reference to this object so that method calls can be
* chained together.
*/
public ResourceSpecificResult withMissingContextValues(
String... missingContextValues) {
if (this.missingContextValues == null) {
setMissingContextValues(new com.amazonaws.internal.SdkInternalList(
missingContextValues.length));
}
for (String ele : missingContextValues) {
this.missingContextValues.add(ele);
}
return this;
}
/**
*
* A list of context keys that are required by the included input policies
* but that were not provided by one of the input parameters. This list is
* used when a list of ARNs is included in the ResourceArns
* parameter instead of "*". If you do not specify individual resources, by
* setting ResourceArns
to "*" or by not including the
* ResourceArns
parameter, then any missing context values are
* instead included under the EvaluationResults
section. To
* discover the context keys used by a set of policies, you can call
* GetContextKeysForCustomPolicy or
* GetContextKeysForPrincipalPolicy.
*
*
* @param missingContextValues
* A list of context keys that are required by the included input
* policies but that were not provided by one of the input
* parameters. This list is used when a list of ARNs is included in
* the ResourceArns
parameter instead of "*". If you do
* not specify individual resources, by setting
* ResourceArns
to "*" or by not including the
* ResourceArns
parameter, then any missing context
* values are instead included under the
* EvaluationResults
section. To discover the context
* keys used by a set of policies, you can call
* GetContextKeysForCustomPolicy or
* GetContextKeysForPrincipalPolicy.
* @return Returns a reference to this object so that method calls can be
* chained together.
*/
public ResourceSpecificResult withMissingContextValues(
java.util.Collection missingContextValues) {
setMissingContextValues(missingContextValues);
return this;
}
/**
*
* Additional details about the results of the evaluation decision. When
* there are both IAM policies and resource policies, this parameter
* explains how each set of policies contributes to the final evaluation
* decision. When simulating cross-account access to a resource, both the
* resource-based policy and the caller's IAM policy must grant access.
*
*
* @return Additional details about the results of the evaluation decision.
* When there are both IAM policies and resource policies, this
* parameter explains how each set of policies contributes to the
* final evaluation decision. When simulating cross-account access
* to a resource, both the resource-based policy and the caller's
* IAM policy must grant access.
*/
public java.util.Map getEvalDecisionDetails() {
if (evalDecisionDetails == null) {
evalDecisionDetails = new com.amazonaws.internal.SdkInternalMap();
}
return evalDecisionDetails;
}
/**
*
* Additional details about the results of the evaluation decision. When
* there are both IAM policies and resource policies, this parameter
* explains how each set of policies contributes to the final evaluation
* decision. When simulating cross-account access to a resource, both the
* resource-based policy and the caller's IAM policy must grant access.
*
*
* @param evalDecisionDetails
* Additional details about the results of the evaluation decision.
* When there are both IAM policies and resource policies, this
* parameter explains how each set of policies contributes to the
* final evaluation decision. When simulating cross-account access to
* a resource, both the resource-based policy and the caller's IAM
* policy must grant access.
*/
public void setEvalDecisionDetails(
java.util.Map evalDecisionDetails) {
this.evalDecisionDetails = evalDecisionDetails == null ? null
: new com.amazonaws.internal.SdkInternalMap(
evalDecisionDetails);
}
/**
*
* Additional details about the results of the evaluation decision. When
* there are both IAM policies and resource policies, this parameter
* explains how each set of policies contributes to the final evaluation
* decision. When simulating cross-account access to a resource, both the
* resource-based policy and the caller's IAM policy must grant access.
*
*
* @param evalDecisionDetails
* Additional details about the results of the evaluation decision.
* When there are both IAM policies and resource policies, this
* parameter explains how each set of policies contributes to the
* final evaluation decision. When simulating cross-account access to
* a resource, both the resource-based policy and the caller's IAM
* policy must grant access.
* @return Returns a reference to this object so that method calls can be
* chained together.
*/
public ResourceSpecificResult withEvalDecisionDetails(
java.util.Map evalDecisionDetails) {
setEvalDecisionDetails(evalDecisionDetails);
return this;
}
public ResourceSpecificResult addEvalDecisionDetailsEntry(String key,
String value) {
if (null == this.evalDecisionDetails) {
this.evalDecisionDetails = new com.amazonaws.internal.SdkInternalMap();
}
if (this.evalDecisionDetails.containsKey(key))
throw new IllegalArgumentException("Duplicated keys ("
+ key.toString() + ") are provided.");
this.evalDecisionDetails.put(key, value);
return this;
}
/**
* Removes all the entries added into EvalDecisionDetails. <p> Returns a
* reference to this object so that method calls can be chained together.
*/
public ResourceSpecificResult clearEvalDecisionDetailsEntries() {
this.evalDecisionDetails = null;
return this;
}
/**
* Returns a string representation of this object; useful for testing and
* debugging.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getEvalResourceName() != null)
sb.append("EvalResourceName: " + getEvalResourceName() + ",");
if (getEvalResourceDecision() != null)
sb.append("EvalResourceDecision: " + getEvalResourceDecision()
+ ",");
if (getMatchedStatements() != null)
sb.append("MatchedStatements: " + getMatchedStatements() + ",");
if (getMissingContextValues() != null)
sb.append("MissingContextValues: " + getMissingContextValues()
+ ",");
if (getEvalDecisionDetails() != null)
sb.append("EvalDecisionDetails: " + getEvalDecisionDetails());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof ResourceSpecificResult == false)
return false;
ResourceSpecificResult other = (ResourceSpecificResult) obj;
if (other.getEvalResourceName() == null
^ this.getEvalResourceName() == null)
return false;
if (other.getEvalResourceName() != null
&& other.getEvalResourceName().equals(
this.getEvalResourceName()) == false)
return false;
if (other.getEvalResourceDecision() == null
^ this.getEvalResourceDecision() == null)
return false;
if (other.getEvalResourceDecision() != null
&& other.getEvalResourceDecision().equals(
this.getEvalResourceDecision()) == false)
return false;
if (other.getMatchedStatements() == null
^ this.getMatchedStatements() == null)
return false;
if (other.getMatchedStatements() != null
&& other.getMatchedStatements().equals(
this.getMatchedStatements()) == false)
return false;
if (other.getMissingContextValues() == null
^ this.getMissingContextValues() == null)
return false;
if (other.getMissingContextValues() != null
&& other.getMissingContextValues().equals(
this.getMissingContextValues()) == false)
return false;
if (other.getEvalDecisionDetails() == null
^ this.getEvalDecisionDetails() == null)
return false;
if (other.getEvalDecisionDetails() != null
&& other.getEvalDecisionDetails().equals(
this.getEvalDecisionDetails()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime
* hashCode
+ ((getEvalResourceName() == null) ? 0 : getEvalResourceName()
.hashCode());
hashCode = prime
* hashCode
+ ((getEvalResourceDecision() == null) ? 0
: getEvalResourceDecision().hashCode());
hashCode = prime
* hashCode
+ ((getMatchedStatements() == null) ? 0
: getMatchedStatements().hashCode());
hashCode = prime
* hashCode
+ ((getMissingContextValues() == null) ? 0
: getMissingContextValues().hashCode());
hashCode = prime
* hashCode
+ ((getEvalDecisionDetails() == null) ? 0
: getEvalDecisionDetails().hashCode());
return hashCode;
}
@Override
public ResourceSpecificResult clone() {
try {
return (ResourceSpecificResult) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException(
"Got a CloneNotSupportedException from Object.clone() "
+ "even though we're Cloneable!", e);
}
}
}