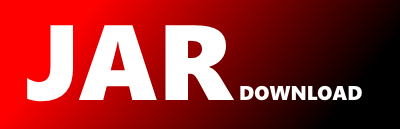
com.amazonaws.services.inspector2.model.CodeVulnerabilityDetails Maven / Gradle / Ivy
Show all versions of aws-java-sdk-inspector2 Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.inspector2.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* Contains information on the code vulnerability identified in your Lambda function.
*
*
* @see AWS API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class CodeVulnerabilityDetails implements Serializable, Cloneable, StructuredPojo {
/**
*
* The Common Weakness Enumeration (CWE) item associated with the detected vulnerability.
*
*/
private java.util.List cwes;
/**
*
* The ID for the Amazon CodeGuru detector associated with the finding. For more information on detectors see Amazon CodeGuru Detector Library.
*
*/
private String detectorId;
/**
*
* The name of the detector used to identify the code vulnerability. For more information on detectors see CodeGuru Detector Library.
*
*/
private String detectorName;
/**
*
* The detector tag associated with the vulnerability. Detector tags group related vulnerabilities by common themes
* or tactics. For a list of available tags by programming language, see Java tags, or Python tags.
*
*/
private java.util.List detectorTags;
/**
*
* Contains information on where the code vulnerability is located in your code.
*
*/
private CodeFilePath filePath;
/**
*
* A URL containing supporting documentation about the code vulnerability detected.
*
*/
private java.util.List referenceUrls;
/**
*
* The identifier for a rule that was used to detect the code vulnerability.
*
*/
private String ruleId;
/**
*
* The Amazon Resource Name (ARN) of the Lambda layer that the code vulnerability was detected in.
*
*/
private String sourceLambdaLayerArn;
/**
*
* The Common Weakness Enumeration (CWE) item associated with the detected vulnerability.
*
*
* @return The Common Weakness Enumeration (CWE) item associated with the detected vulnerability.
*/
public java.util.List getCwes() {
return cwes;
}
/**
*
* The Common Weakness Enumeration (CWE) item associated with the detected vulnerability.
*
*
* @param cwes
* The Common Weakness Enumeration (CWE) item associated with the detected vulnerability.
*/
public void setCwes(java.util.Collection cwes) {
if (cwes == null) {
this.cwes = null;
return;
}
this.cwes = new java.util.ArrayList(cwes);
}
/**
*
* The Common Weakness Enumeration (CWE) item associated with the detected vulnerability.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setCwes(java.util.Collection)} or {@link #withCwes(java.util.Collection)} if you want to override the
* existing values.
*
*
* @param cwes
* The Common Weakness Enumeration (CWE) item associated with the detected vulnerability.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CodeVulnerabilityDetails withCwes(String... cwes) {
if (this.cwes == null) {
setCwes(new java.util.ArrayList(cwes.length));
}
for (String ele : cwes) {
this.cwes.add(ele);
}
return this;
}
/**
*
* The Common Weakness Enumeration (CWE) item associated with the detected vulnerability.
*
*
* @param cwes
* The Common Weakness Enumeration (CWE) item associated with the detected vulnerability.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CodeVulnerabilityDetails withCwes(java.util.Collection cwes) {
setCwes(cwes);
return this;
}
/**
*
* The ID for the Amazon CodeGuru detector associated with the finding. For more information on detectors see Amazon CodeGuru Detector Library.
*
*
* @param detectorId
* The ID for the Amazon CodeGuru detector associated with the finding. For more information on detectors see
* Amazon CodeGuru Detector Library.
*/
public void setDetectorId(String detectorId) {
this.detectorId = detectorId;
}
/**
*
* The ID for the Amazon CodeGuru detector associated with the finding. For more information on detectors see Amazon CodeGuru Detector Library.
*
*
* @return The ID for the Amazon CodeGuru detector associated with the finding. For more information on detectors
* see Amazon CodeGuru Detector Library.
*/
public String getDetectorId() {
return this.detectorId;
}
/**
*
* The ID for the Amazon CodeGuru detector associated with the finding. For more information on detectors see Amazon CodeGuru Detector Library.
*
*
* @param detectorId
* The ID for the Amazon CodeGuru detector associated with the finding. For more information on detectors see
* Amazon CodeGuru Detector Library.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CodeVulnerabilityDetails withDetectorId(String detectorId) {
setDetectorId(detectorId);
return this;
}
/**
*
* The name of the detector used to identify the code vulnerability. For more information on detectors see CodeGuru Detector Library.
*
*
* @param detectorName
* The name of the detector used to identify the code vulnerability. For more information on detectors see CodeGuru Detector Library.
*/
public void setDetectorName(String detectorName) {
this.detectorName = detectorName;
}
/**
*
* The name of the detector used to identify the code vulnerability. For more information on detectors see CodeGuru Detector Library.
*
*
* @return The name of the detector used to identify the code vulnerability. For more information on detectors see
* CodeGuru Detector Library.
*/
public String getDetectorName() {
return this.detectorName;
}
/**
*
* The name of the detector used to identify the code vulnerability. For more information on detectors see CodeGuru Detector Library.
*
*
* @param detectorName
* The name of the detector used to identify the code vulnerability. For more information on detectors see CodeGuru Detector Library.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CodeVulnerabilityDetails withDetectorName(String detectorName) {
setDetectorName(detectorName);
return this;
}
/**
*
* The detector tag associated with the vulnerability. Detector tags group related vulnerabilities by common themes
* or tactics. For a list of available tags by programming language, see Java tags, or Python tags.
*
*
* @return The detector tag associated with the vulnerability. Detector tags group related vulnerabilities by common
* themes or tactics. For a list of available tags by programming language, see Java tags, or Python tags.
*/
public java.util.List getDetectorTags() {
return detectorTags;
}
/**
*
* The detector tag associated with the vulnerability. Detector tags group related vulnerabilities by common themes
* or tactics. For a list of available tags by programming language, see Java tags, or Python tags.
*
*
* @param detectorTags
* The detector tag associated with the vulnerability. Detector tags group related vulnerabilities by common
* themes or tactics. For a list of available tags by programming language, see Java tags, or Python tags.
*/
public void setDetectorTags(java.util.Collection detectorTags) {
if (detectorTags == null) {
this.detectorTags = null;
return;
}
this.detectorTags = new java.util.ArrayList(detectorTags);
}
/**
*
* The detector tag associated with the vulnerability. Detector tags group related vulnerabilities by common themes
* or tactics. For a list of available tags by programming language, see Java tags, or Python tags.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setDetectorTags(java.util.Collection)} or {@link #withDetectorTags(java.util.Collection)} if you want to
* override the existing values.
*
*
* @param detectorTags
* The detector tag associated with the vulnerability. Detector tags group related vulnerabilities by common
* themes or tactics. For a list of available tags by programming language, see Java tags, or Python tags.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CodeVulnerabilityDetails withDetectorTags(String... detectorTags) {
if (this.detectorTags == null) {
setDetectorTags(new java.util.ArrayList(detectorTags.length));
}
for (String ele : detectorTags) {
this.detectorTags.add(ele);
}
return this;
}
/**
*
* The detector tag associated with the vulnerability. Detector tags group related vulnerabilities by common themes
* or tactics. For a list of available tags by programming language, see Java tags, or Python tags.
*
*
* @param detectorTags
* The detector tag associated with the vulnerability. Detector tags group related vulnerabilities by common
* themes or tactics. For a list of available tags by programming language, see Java tags, or Python tags.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CodeVulnerabilityDetails withDetectorTags(java.util.Collection detectorTags) {
setDetectorTags(detectorTags);
return this;
}
/**
*
* Contains information on where the code vulnerability is located in your code.
*
*
* @param filePath
* Contains information on where the code vulnerability is located in your code.
*/
public void setFilePath(CodeFilePath filePath) {
this.filePath = filePath;
}
/**
*
* Contains information on where the code vulnerability is located in your code.
*
*
* @return Contains information on where the code vulnerability is located in your code.
*/
public CodeFilePath getFilePath() {
return this.filePath;
}
/**
*
* Contains information on where the code vulnerability is located in your code.
*
*
* @param filePath
* Contains information on where the code vulnerability is located in your code.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CodeVulnerabilityDetails withFilePath(CodeFilePath filePath) {
setFilePath(filePath);
return this;
}
/**
*
* A URL containing supporting documentation about the code vulnerability detected.
*
*
* @return A URL containing supporting documentation about the code vulnerability detected.
*/
public java.util.List getReferenceUrls() {
return referenceUrls;
}
/**
*
* A URL containing supporting documentation about the code vulnerability detected.
*
*
* @param referenceUrls
* A URL containing supporting documentation about the code vulnerability detected.
*/
public void setReferenceUrls(java.util.Collection referenceUrls) {
if (referenceUrls == null) {
this.referenceUrls = null;
return;
}
this.referenceUrls = new java.util.ArrayList(referenceUrls);
}
/**
*
* A URL containing supporting documentation about the code vulnerability detected.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setReferenceUrls(java.util.Collection)} or {@link #withReferenceUrls(java.util.Collection)} if you want
* to override the existing values.
*
*
* @param referenceUrls
* A URL containing supporting documentation about the code vulnerability detected.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CodeVulnerabilityDetails withReferenceUrls(String... referenceUrls) {
if (this.referenceUrls == null) {
setReferenceUrls(new java.util.ArrayList(referenceUrls.length));
}
for (String ele : referenceUrls) {
this.referenceUrls.add(ele);
}
return this;
}
/**
*
* A URL containing supporting documentation about the code vulnerability detected.
*
*
* @param referenceUrls
* A URL containing supporting documentation about the code vulnerability detected.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CodeVulnerabilityDetails withReferenceUrls(java.util.Collection referenceUrls) {
setReferenceUrls(referenceUrls);
return this;
}
/**
*
* The identifier for a rule that was used to detect the code vulnerability.
*
*
* @param ruleId
* The identifier for a rule that was used to detect the code vulnerability.
*/
public void setRuleId(String ruleId) {
this.ruleId = ruleId;
}
/**
*
* The identifier for a rule that was used to detect the code vulnerability.
*
*
* @return The identifier for a rule that was used to detect the code vulnerability.
*/
public String getRuleId() {
return this.ruleId;
}
/**
*
* The identifier for a rule that was used to detect the code vulnerability.
*
*
* @param ruleId
* The identifier for a rule that was used to detect the code vulnerability.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CodeVulnerabilityDetails withRuleId(String ruleId) {
setRuleId(ruleId);
return this;
}
/**
*
* The Amazon Resource Name (ARN) of the Lambda layer that the code vulnerability was detected in.
*
*
* @param sourceLambdaLayerArn
* The Amazon Resource Name (ARN) of the Lambda layer that the code vulnerability was detected in.
*/
public void setSourceLambdaLayerArn(String sourceLambdaLayerArn) {
this.sourceLambdaLayerArn = sourceLambdaLayerArn;
}
/**
*
* The Amazon Resource Name (ARN) of the Lambda layer that the code vulnerability was detected in.
*
*
* @return The Amazon Resource Name (ARN) of the Lambda layer that the code vulnerability was detected in.
*/
public String getSourceLambdaLayerArn() {
return this.sourceLambdaLayerArn;
}
/**
*
* The Amazon Resource Name (ARN) of the Lambda layer that the code vulnerability was detected in.
*
*
* @param sourceLambdaLayerArn
* The Amazon Resource Name (ARN) of the Lambda layer that the code vulnerability was detected in.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CodeVulnerabilityDetails withSourceLambdaLayerArn(String sourceLambdaLayerArn) {
setSourceLambdaLayerArn(sourceLambdaLayerArn);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getCwes() != null)
sb.append("Cwes: ").append(getCwes()).append(",");
if (getDetectorId() != null)
sb.append("DetectorId: ").append(getDetectorId()).append(",");
if (getDetectorName() != null)
sb.append("DetectorName: ").append(getDetectorName()).append(",");
if (getDetectorTags() != null)
sb.append("DetectorTags: ").append(getDetectorTags()).append(",");
if (getFilePath() != null)
sb.append("FilePath: ").append(getFilePath()).append(",");
if (getReferenceUrls() != null)
sb.append("ReferenceUrls: ").append(getReferenceUrls()).append(",");
if (getRuleId() != null)
sb.append("RuleId: ").append(getRuleId()).append(",");
if (getSourceLambdaLayerArn() != null)
sb.append("SourceLambdaLayerArn: ").append(getSourceLambdaLayerArn());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof CodeVulnerabilityDetails == false)
return false;
CodeVulnerabilityDetails other = (CodeVulnerabilityDetails) obj;
if (other.getCwes() == null ^ this.getCwes() == null)
return false;
if (other.getCwes() != null && other.getCwes().equals(this.getCwes()) == false)
return false;
if (other.getDetectorId() == null ^ this.getDetectorId() == null)
return false;
if (other.getDetectorId() != null && other.getDetectorId().equals(this.getDetectorId()) == false)
return false;
if (other.getDetectorName() == null ^ this.getDetectorName() == null)
return false;
if (other.getDetectorName() != null && other.getDetectorName().equals(this.getDetectorName()) == false)
return false;
if (other.getDetectorTags() == null ^ this.getDetectorTags() == null)
return false;
if (other.getDetectorTags() != null && other.getDetectorTags().equals(this.getDetectorTags()) == false)
return false;
if (other.getFilePath() == null ^ this.getFilePath() == null)
return false;
if (other.getFilePath() != null && other.getFilePath().equals(this.getFilePath()) == false)
return false;
if (other.getReferenceUrls() == null ^ this.getReferenceUrls() == null)
return false;
if (other.getReferenceUrls() != null && other.getReferenceUrls().equals(this.getReferenceUrls()) == false)
return false;
if (other.getRuleId() == null ^ this.getRuleId() == null)
return false;
if (other.getRuleId() != null && other.getRuleId().equals(this.getRuleId()) == false)
return false;
if (other.getSourceLambdaLayerArn() == null ^ this.getSourceLambdaLayerArn() == null)
return false;
if (other.getSourceLambdaLayerArn() != null && other.getSourceLambdaLayerArn().equals(this.getSourceLambdaLayerArn()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getCwes() == null) ? 0 : getCwes().hashCode());
hashCode = prime * hashCode + ((getDetectorId() == null) ? 0 : getDetectorId().hashCode());
hashCode = prime * hashCode + ((getDetectorName() == null) ? 0 : getDetectorName().hashCode());
hashCode = prime * hashCode + ((getDetectorTags() == null) ? 0 : getDetectorTags().hashCode());
hashCode = prime * hashCode + ((getFilePath() == null) ? 0 : getFilePath().hashCode());
hashCode = prime * hashCode + ((getReferenceUrls() == null) ? 0 : getReferenceUrls().hashCode());
hashCode = prime * hashCode + ((getRuleId() == null) ? 0 : getRuleId().hashCode());
hashCode = prime * hashCode + ((getSourceLambdaLayerArn() == null) ? 0 : getSourceLambdaLayerArn().hashCode());
return hashCode;
}
@Override
public CodeVulnerabilityDetails clone() {
try {
return (CodeVulnerabilityDetails) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.inspector2.model.transform.CodeVulnerabilityDetailsMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}