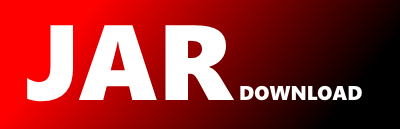
com.amazonaws.services.iot.model.OutgoingCertificate Maven / Gradle / Ivy
Show all versions of aws-java-sdk-iot Show documentation
/*
* Copyright 2011-2016 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.iot.model;
import java.io.Serializable;
/**
*
* A certificate that has been transfered but not yet accepted.
*
*/
public class OutgoingCertificate implements Serializable, Cloneable {
/**
*
* The certificate ARN.
*
*/
private String certificateArn;
/**
*
* The certificate ID.
*
*/
private String certificateId;
/**
*
* The AWS account to which the transfer was made.
*
*/
private String transferredTo;
/**
*
* The date the transfer was initiated.
*
*/
private java.util.Date transferDate;
/**
*
* The transfer message.
*
*/
private String transferMessage;
/**
*
* The certificate creation date.
*
*/
private java.util.Date creationDate;
/**
*
* The certificate ARN.
*
*
* @param certificateArn
* The certificate ARN.
*/
public void setCertificateArn(String certificateArn) {
this.certificateArn = certificateArn;
}
/**
*
* The certificate ARN.
*
*
* @return The certificate ARN.
*/
public String getCertificateArn() {
return this.certificateArn;
}
/**
*
* The certificate ARN.
*
*
* @param certificateArn
* The certificate ARN.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public OutgoingCertificate withCertificateArn(String certificateArn) {
setCertificateArn(certificateArn);
return this;
}
/**
*
* The certificate ID.
*
*
* @param certificateId
* The certificate ID.
*/
public void setCertificateId(String certificateId) {
this.certificateId = certificateId;
}
/**
*
* The certificate ID.
*
*
* @return The certificate ID.
*/
public String getCertificateId() {
return this.certificateId;
}
/**
*
* The certificate ID.
*
*
* @param certificateId
* The certificate ID.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public OutgoingCertificate withCertificateId(String certificateId) {
setCertificateId(certificateId);
return this;
}
/**
*
* The AWS account to which the transfer was made.
*
*
* @param transferredTo
* The AWS account to which the transfer was made.
*/
public void setTransferredTo(String transferredTo) {
this.transferredTo = transferredTo;
}
/**
*
* The AWS account to which the transfer was made.
*
*
* @return The AWS account to which the transfer was made.
*/
public String getTransferredTo() {
return this.transferredTo;
}
/**
*
* The AWS account to which the transfer was made.
*
*
* @param transferredTo
* The AWS account to which the transfer was made.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public OutgoingCertificate withTransferredTo(String transferredTo) {
setTransferredTo(transferredTo);
return this;
}
/**
*
* The date the transfer was initiated.
*
*
* @param transferDate
* The date the transfer was initiated.
*/
public void setTransferDate(java.util.Date transferDate) {
this.transferDate = transferDate;
}
/**
*
* The date the transfer was initiated.
*
*
* @return The date the transfer was initiated.
*/
public java.util.Date getTransferDate() {
return this.transferDate;
}
/**
*
* The date the transfer was initiated.
*
*
* @param transferDate
* The date the transfer was initiated.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public OutgoingCertificate withTransferDate(java.util.Date transferDate) {
setTransferDate(transferDate);
return this;
}
/**
*
* The transfer message.
*
*
* @param transferMessage
* The transfer message.
*/
public void setTransferMessage(String transferMessage) {
this.transferMessage = transferMessage;
}
/**
*
* The transfer message.
*
*
* @return The transfer message.
*/
public String getTransferMessage() {
return this.transferMessage;
}
/**
*
* The transfer message.
*
*
* @param transferMessage
* The transfer message.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public OutgoingCertificate withTransferMessage(String transferMessage) {
setTransferMessage(transferMessage);
return this;
}
/**
*
* The certificate creation date.
*
*
* @param creationDate
* The certificate creation date.
*/
public void setCreationDate(java.util.Date creationDate) {
this.creationDate = creationDate;
}
/**
*
* The certificate creation date.
*
*
* @return The certificate creation date.
*/
public java.util.Date getCreationDate() {
return this.creationDate;
}
/**
*
* The certificate creation date.
*
*
* @param creationDate
* The certificate creation date.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public OutgoingCertificate withCreationDate(java.util.Date creationDate) {
setCreationDate(creationDate);
return this;
}
/**
* Returns a string representation of this object; useful for testing and debugging.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getCertificateArn() != null)
sb.append("CertificateArn: " + getCertificateArn() + ",");
if (getCertificateId() != null)
sb.append("CertificateId: " + getCertificateId() + ",");
if (getTransferredTo() != null)
sb.append("TransferredTo: " + getTransferredTo() + ",");
if (getTransferDate() != null)
sb.append("TransferDate: " + getTransferDate() + ",");
if (getTransferMessage() != null)
sb.append("TransferMessage: " + getTransferMessage() + ",");
if (getCreationDate() != null)
sb.append("CreationDate: " + getCreationDate());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof OutgoingCertificate == false)
return false;
OutgoingCertificate other = (OutgoingCertificate) obj;
if (other.getCertificateArn() == null ^ this.getCertificateArn() == null)
return false;
if (other.getCertificateArn() != null && other.getCertificateArn().equals(this.getCertificateArn()) == false)
return false;
if (other.getCertificateId() == null ^ this.getCertificateId() == null)
return false;
if (other.getCertificateId() != null && other.getCertificateId().equals(this.getCertificateId()) == false)
return false;
if (other.getTransferredTo() == null ^ this.getTransferredTo() == null)
return false;
if (other.getTransferredTo() != null && other.getTransferredTo().equals(this.getTransferredTo()) == false)
return false;
if (other.getTransferDate() == null ^ this.getTransferDate() == null)
return false;
if (other.getTransferDate() != null && other.getTransferDate().equals(this.getTransferDate()) == false)
return false;
if (other.getTransferMessage() == null ^ this.getTransferMessage() == null)
return false;
if (other.getTransferMessage() != null && other.getTransferMessage().equals(this.getTransferMessage()) == false)
return false;
if (other.getCreationDate() == null ^ this.getCreationDate() == null)
return false;
if (other.getCreationDate() != null && other.getCreationDate().equals(this.getCreationDate()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getCertificateArn() == null) ? 0 : getCertificateArn().hashCode());
hashCode = prime * hashCode + ((getCertificateId() == null) ? 0 : getCertificateId().hashCode());
hashCode = prime * hashCode + ((getTransferredTo() == null) ? 0 : getTransferredTo().hashCode());
hashCode = prime * hashCode + ((getTransferDate() == null) ? 0 : getTransferDate().hashCode());
hashCode = prime * hashCode + ((getTransferMessage() == null) ? 0 : getTransferMessage().hashCode());
hashCode = prime * hashCode + ((getCreationDate() == null) ? 0 : getCreationDate().hashCode());
return hashCode;
}
@Override
public OutgoingCertificate clone() {
try {
return (OutgoingCertificate) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
}