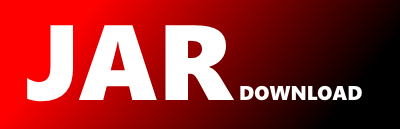
com.amazonaws.services.iotdata.AWSIotDataAsync Maven / Gradle / Ivy
Show all versions of aws-java-sdk-iot Show documentation
/*
* Copyright 2011-2016 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.iotdata;
import com.amazonaws.services.iotdata.model.*;
/**
* Interface for accessing AWS IoT Data Plane asynchronously. Each asynchronous method will return a Java Future object
* representing the asynchronous operation; overloads which accept an {@code AsyncHandler} can be used to receive
* notification when an asynchronous operation completes.
*
* AWS IoT
*
* AWS IoT-Data enables secure, bi-directional communication between Internet-connected things (such as sensors,
* actuators, embedded devices, or smart appliances) and the AWS cloud. It implements a broker for applications and
* things to publish messages over HTTP (Publish) and retrieve, update, and delete thing shadows. A thing shadow is a
* persistent representation of your things and their state in the AWS cloud.
*
*/
public interface AWSIotDataAsync extends AWSIotData {
/**
*
* Deletes the thing shadow for the specified thing.
*
*
* For more information, see DeleteThingShadow in
* the AWS IoT Developer Guide.
*
*
* @param deleteThingShadowRequest
* The input for the DeleteThingShadow operation.
* @return A Java Future containing the result of the DeleteThingShadow operation returned by the service.
* @sample AWSIotDataAsync.DeleteThingShadow
*/
java.util.concurrent.Future deleteThingShadowAsync(DeleteThingShadowRequest deleteThingShadowRequest);
/**
*
* Deletes the thing shadow for the specified thing.
*
*
* For more information, see DeleteThingShadow in
* the AWS IoT Developer Guide.
*
*
* @param deleteThingShadowRequest
* The input for the DeleteThingShadow operation.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteThingShadow operation returned by the service.
* @sample AWSIotDataAsyncHandler.DeleteThingShadow
*/
java.util.concurrent.Future deleteThingShadowAsync(DeleteThingShadowRequest deleteThingShadowRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets the thing shadow for the specified thing.
*
*
* For more information, see GetThingShadow in the
* AWS IoT Developer Guide.
*
*
* @param getThingShadowRequest
* The input for the GetThingShadow operation.
* @return A Java Future containing the result of the GetThingShadow operation returned by the service.
* @sample AWSIotDataAsync.GetThingShadow
*/
java.util.concurrent.Future getThingShadowAsync(GetThingShadowRequest getThingShadowRequest);
/**
*
* Gets the thing shadow for the specified thing.
*
*
* For more information, see GetThingShadow in the
* AWS IoT Developer Guide.
*
*
* @param getThingShadowRequest
* The input for the GetThingShadow operation.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetThingShadow operation returned by the service.
* @sample AWSIotDataAsyncHandler.GetThingShadow
*/
java.util.concurrent.Future getThingShadowAsync(GetThingShadowRequest getThingShadowRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Publishes state information.
*
*
* For more information, see HTTP
* Protocol in the AWS IoT Developer Guide.
*
*
* @param publishRequest
* The input for the Publish operation.
* @return A Java Future containing the result of the Publish operation returned by the service.
* @sample AWSIotDataAsync.Publish
*/
java.util.concurrent.Future publishAsync(PublishRequest publishRequest);
/**
*
* Publishes state information.
*
*
* For more information, see HTTP
* Protocol in the AWS IoT Developer Guide.
*
*
* @param publishRequest
* The input for the Publish operation.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the Publish operation returned by the service.
* @sample AWSIotDataAsyncHandler.Publish
*/
java.util.concurrent.Future publishAsync(PublishRequest publishRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates the thing shadow for the specified thing.
*
*
* For more information, see UpdateThingShadow in
* the AWS IoT Developer Guide.
*
*
* @param updateThingShadowRequest
* The input for the UpdateThingShadow operation.
* @return A Java Future containing the result of the UpdateThingShadow operation returned by the service.
* @sample AWSIotDataAsync.UpdateThingShadow
*/
java.util.concurrent.Future updateThingShadowAsync(UpdateThingShadowRequest updateThingShadowRequest);
/**
*
* Updates the thing shadow for the specified thing.
*
*
* For more information, see UpdateThingShadow in
* the AWS IoT Developer Guide.
*
*
* @param updateThingShadowRequest
* The input for the UpdateThingShadow operation.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateThingShadow operation returned by the service.
* @sample AWSIotDataAsyncHandler.UpdateThingShadow
*/
java.util.concurrent.Future updateThingShadowAsync(UpdateThingShadowRequest updateThingShadowRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
}