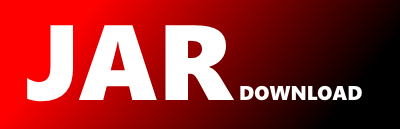
com.amazonaws.services.iot1clickprojects.AWSIoT1ClickProjects Maven / Gradle / Ivy
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.iot1clickprojects;
import javax.annotation.Generated;
import com.amazonaws.*;
import com.amazonaws.regions.*;
import com.amazonaws.services.iot1clickprojects.model.*;
/**
* Interface for accessing AWS IoT 1-Click Projects.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.iot1clickprojects.AbstractAWSIoT1ClickProjects} instead.
*
*
*
* The AWS IoT 1-Click Projects API Reference
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AWSIoT1ClickProjects {
/**
* The region metadata service name for computing region endpoints. You can use this value to retrieve metadata
* (such as supported regions) of the service.
*
* @see RegionUtils#getRegionsForService(String)
*/
String ENDPOINT_PREFIX = "projects.iot1click";
/**
*
* Associates a physical device with a placement.
*
*
* @param associateDeviceWithPlacementRequest
* @return Result of the AssociateDeviceWithPlacement operation returned by the service.
* @throws InternalFailureException
* @throws InvalidRequestException
* @throws ResourceConflictException
* @throws ResourceNotFoundException
* @sample AWSIoT1ClickProjects.AssociateDeviceWithPlacement
* @see AWS API Documentation
*/
AssociateDeviceWithPlacementResult associateDeviceWithPlacement(AssociateDeviceWithPlacementRequest associateDeviceWithPlacementRequest);
/**
*
* Creates an empty placement.
*
*
* @param createPlacementRequest
* @return Result of the CreatePlacement operation returned by the service.
* @throws InternalFailureException
* @throws InvalidRequestException
* @throws ResourceConflictException
* @throws ResourceNotFoundException
* @sample AWSIoT1ClickProjects.CreatePlacement
* @see AWS API Documentation
*/
CreatePlacementResult createPlacement(CreatePlacementRequest createPlacementRequest);
/**
*
* Creates an empty project with a placement template. A project contains zero or more placements that adhere to the
* placement template defined in the project.
*
*
* @param createProjectRequest
* @return Result of the CreateProject operation returned by the service.
* @throws InternalFailureException
* @throws InvalidRequestException
* @throws ResourceConflictException
* @sample AWSIoT1ClickProjects.CreateProject
* @see AWS API Documentation
*/
CreateProjectResult createProject(CreateProjectRequest createProjectRequest);
/**
*
* Deletes a placement. To delete a placement, it must not have any devices associated with it.
*
*
*
* When you delete a placement, all associated data becomes irretrievable.
*
*
*
* @param deletePlacementRequest
* @return Result of the DeletePlacement operation returned by the service.
* @throws InternalFailureException
* @throws InvalidRequestException
* @throws ResourceNotFoundException
* @throws TooManyRequestsException
* @sample AWSIoT1ClickProjects.DeletePlacement
* @see AWS API Documentation
*/
DeletePlacementResult deletePlacement(DeletePlacementRequest deletePlacementRequest);
/**
*
* Deletes a project. To delete a project, it must not have any placements associated with it.
*
*
*
* When you delete a project, all associated data becomes irretrievable.
*
*
*
* @param deleteProjectRequest
* @return Result of the DeleteProject operation returned by the service.
* @throws InternalFailureException
* @throws InvalidRequestException
* @throws ResourceNotFoundException
* @throws TooManyRequestsException
* @sample AWSIoT1ClickProjects.DeleteProject
* @see AWS API Documentation
*/
DeleteProjectResult deleteProject(DeleteProjectRequest deleteProjectRequest);
/**
*
* Describes a placement in a project.
*
*
* @param describePlacementRequest
* @return Result of the DescribePlacement operation returned by the service.
* @throws InternalFailureException
* @throws InvalidRequestException
* @throws ResourceNotFoundException
* @sample AWSIoT1ClickProjects.DescribePlacement
* @see AWS API Documentation
*/
DescribePlacementResult describePlacement(DescribePlacementRequest describePlacementRequest);
/**
*
* Returns an object describing a project.
*
*
* @param describeProjectRequest
* @return Result of the DescribeProject operation returned by the service.
* @throws InternalFailureException
* @throws InvalidRequestException
* @throws ResourceNotFoundException
* @sample AWSIoT1ClickProjects.DescribeProject
* @see AWS API Documentation
*/
DescribeProjectResult describeProject(DescribeProjectRequest describeProjectRequest);
/**
*
* Removes a physical device from a placement.
*
*
* @param disassociateDeviceFromPlacementRequest
* @return Result of the DisassociateDeviceFromPlacement operation returned by the service.
* @throws InternalFailureException
* @throws InvalidRequestException
* @throws ResourceNotFoundException
* @throws TooManyRequestsException
* @sample AWSIoT1ClickProjects.DisassociateDeviceFromPlacement
* @see AWS API Documentation
*/
DisassociateDeviceFromPlacementResult disassociateDeviceFromPlacement(DisassociateDeviceFromPlacementRequest disassociateDeviceFromPlacementRequest);
/**
*
* Returns an object enumerating the devices in a placement.
*
*
* @param getDevicesInPlacementRequest
* @return Result of the GetDevicesInPlacement operation returned by the service.
* @throws InternalFailureException
* @throws InvalidRequestException
* @throws ResourceNotFoundException
* @sample AWSIoT1ClickProjects.GetDevicesInPlacement
* @see AWS API Documentation
*/
GetDevicesInPlacementResult getDevicesInPlacement(GetDevicesInPlacementRequest getDevicesInPlacementRequest);
/**
*
* Lists the placement(s) of a project.
*
*
* @param listPlacementsRequest
* @return Result of the ListPlacements operation returned by the service.
* @throws InternalFailureException
* @throws InvalidRequestException
* @throws ResourceNotFoundException
* @sample AWSIoT1ClickProjects.ListPlacements
* @see AWS API Documentation
*/
ListPlacementsResult listPlacements(ListPlacementsRequest listPlacementsRequest);
/**
*
* Lists the AWS IoT 1-Click project(s) associated with your AWS account and region.
*
*
* @param listProjectsRequest
* @return Result of the ListProjects operation returned by the service.
* @throws InternalFailureException
* @throws InvalidRequestException
* @sample AWSIoT1ClickProjects.ListProjects
* @see AWS API Documentation
*/
ListProjectsResult listProjects(ListProjectsRequest listProjectsRequest);
/**
*
* Lists the tags (metadata key/value pairs) which you have assigned to the resource.
*
*
* @param listTagsForResourceRequest
* @return Result of the ListTagsForResource operation returned by the service.
* @throws InternalFailureException
* @throws InvalidRequestException
* @throws ResourceNotFoundException
* @sample AWSIoT1ClickProjects.ListTagsForResource
* @see AWS API Documentation
*/
ListTagsForResourceResult listTagsForResource(ListTagsForResourceRequest listTagsForResourceRequest);
/**
*
* Creates or modifies tags for a resource. Tags are key/value pairs (metadata) that can be used to manage a
* resource. For more information, see AWS Tagging Strategies.
*
*
* @param tagResourceRequest
* @return Result of the TagResource operation returned by the service.
* @throws InternalFailureException
* @throws InvalidRequestException
* @throws ResourceNotFoundException
* @sample AWSIoT1ClickProjects.TagResource
* @see AWS
* API Documentation
*/
TagResourceResult tagResource(TagResourceRequest tagResourceRequest);
/**
*
* Removes one or more tags (metadata key/value pairs) from a resource.
*
*
* @param untagResourceRequest
* @return Result of the UntagResource operation returned by the service.
* @throws InternalFailureException
* @throws InvalidRequestException
* @throws ResourceNotFoundException
* @sample AWSIoT1ClickProjects.UntagResource
* @see AWS API Documentation
*/
UntagResourceResult untagResource(UntagResourceRequest untagResourceRequest);
/**
*
* Updates a placement with the given attributes. To clear an attribute, pass an empty value (i.e., "").
*
*
* @param updatePlacementRequest
* @return Result of the UpdatePlacement operation returned by the service.
* @throws InternalFailureException
* @throws InvalidRequestException
* @throws ResourceNotFoundException
* @throws TooManyRequestsException
* @sample AWSIoT1ClickProjects.UpdatePlacement
* @see AWS API Documentation
*/
UpdatePlacementResult updatePlacement(UpdatePlacementRequest updatePlacementRequest);
/**
*
* Updates a project associated with your AWS account and region. With the exception of device template names, you
* can pass just the values that need to be updated because the update request will change only the values that are
* provided. To clear a value, pass the empty string (i.e., ""
).
*
*
* @param updateProjectRequest
* @return Result of the UpdateProject operation returned by the service.
* @throws InternalFailureException
* @throws InvalidRequestException
* @throws ResourceNotFoundException
* @throws TooManyRequestsException
* @sample AWSIoT1ClickProjects.UpdateProject
* @see AWS API Documentation
*/
UpdateProjectResult updateProject(UpdateProjectRequest updateProjectRequest);
/**
* Shuts down this client object, releasing any resources that might be held open. This is an optional method, and
* callers are not expected to call it, but can if they want to explicitly release any open resources. Once a client
* has been shutdown, it should not be used to make any more requests.
*/
void shutdown();
/**
* Returns additional metadata for a previously executed successful request, typically used for debugging issues
* where a service isn't acting as expected. This data isn't considered part of the result data returned by an
* operation, so it's available through this separate, diagnostic interface.
*
* Response metadata is only cached for a limited period of time, so if you need to access this extra diagnostic
* information for an executed request, you should use this method to retrieve it as soon as possible after
* executing a request.
*
* @param request
* The originally executed request.
*
* @return The response metadata for the specified request, or null if none is available.
*/
ResponseMetadata getCachedResponseMetadata(AmazonWebServiceRequest request);
}