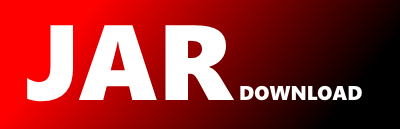
com.amazonaws.services.iot1clickprojects.AWSIoT1ClickProjectsAsync Maven / Gradle / Ivy
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.iot1clickprojects;
import javax.annotation.Generated;
import com.amazonaws.services.iot1clickprojects.model.*;
/**
* Interface for accessing AWS IoT 1-Click Projects asynchronously. Each asynchronous method will return a Java Future
* object representing the asynchronous operation; overloads which accept an {@code AsyncHandler} can be used to receive
* notification when an asynchronous operation completes.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.iot1clickprojects.AbstractAWSIoT1ClickProjectsAsync} instead.
*
*
*
* The AWS IoT 1-Click Projects API Reference
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AWSIoT1ClickProjectsAsync extends AWSIoT1ClickProjects {
/**
*
* Associates a physical device with a placement.
*
*
* @param associateDeviceWithPlacementRequest
* @return A Java Future containing the result of the AssociateDeviceWithPlacement operation returned by the
* service.
* @sample AWSIoT1ClickProjectsAsync.AssociateDeviceWithPlacement
* @see AWS API Documentation
*/
java.util.concurrent.Future associateDeviceWithPlacementAsync(
AssociateDeviceWithPlacementRequest associateDeviceWithPlacementRequest);
/**
*
* Associates a physical device with a placement.
*
*
* @param associateDeviceWithPlacementRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AssociateDeviceWithPlacement operation returned by the
* service.
* @sample AWSIoT1ClickProjectsAsyncHandler.AssociateDeviceWithPlacement
* @see AWS API Documentation
*/
java.util.concurrent.Future associateDeviceWithPlacementAsync(
AssociateDeviceWithPlacementRequest associateDeviceWithPlacementRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates an empty placement.
*
*
* @param createPlacementRequest
* @return A Java Future containing the result of the CreatePlacement operation returned by the service.
* @sample AWSIoT1ClickProjectsAsync.CreatePlacement
* @see AWS API Documentation
*/
java.util.concurrent.Future createPlacementAsync(CreatePlacementRequest createPlacementRequest);
/**
*
* Creates an empty placement.
*
*
* @param createPlacementRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreatePlacement operation returned by the service.
* @sample AWSIoT1ClickProjectsAsyncHandler.CreatePlacement
* @see AWS API Documentation
*/
java.util.concurrent.Future createPlacementAsync(CreatePlacementRequest createPlacementRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates an empty project with a placement template. A project contains zero or more placements that adhere to the
* placement template defined in the project.
*
*
* @param createProjectRequest
* @return A Java Future containing the result of the CreateProject operation returned by the service.
* @sample AWSIoT1ClickProjectsAsync.CreateProject
* @see AWS API Documentation
*/
java.util.concurrent.Future createProjectAsync(CreateProjectRequest createProjectRequest);
/**
*
* Creates an empty project with a placement template. A project contains zero or more placements that adhere to the
* placement template defined in the project.
*
*
* @param createProjectRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateProject operation returned by the service.
* @sample AWSIoT1ClickProjectsAsyncHandler.CreateProject
* @see AWS API Documentation
*/
java.util.concurrent.Future createProjectAsync(CreateProjectRequest createProjectRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a placement. To delete a placement, it must not have any devices associated with it.
*
*
*
* When you delete a placement, all associated data becomes irretrievable.
*
*
*
* @param deletePlacementRequest
* @return A Java Future containing the result of the DeletePlacement operation returned by the service.
* @sample AWSIoT1ClickProjectsAsync.DeletePlacement
* @see AWS API Documentation
*/
java.util.concurrent.Future deletePlacementAsync(DeletePlacementRequest deletePlacementRequest);
/**
*
* Deletes a placement. To delete a placement, it must not have any devices associated with it.
*
*
*
* When you delete a placement, all associated data becomes irretrievable.
*
*
*
* @param deletePlacementRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeletePlacement operation returned by the service.
* @sample AWSIoT1ClickProjectsAsyncHandler.DeletePlacement
* @see AWS API Documentation
*/
java.util.concurrent.Future deletePlacementAsync(DeletePlacementRequest deletePlacementRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a project. To delete a project, it must not have any placements associated with it.
*
*
*
* When you delete a project, all associated data becomes irretrievable.
*
*
*
* @param deleteProjectRequest
* @return A Java Future containing the result of the DeleteProject operation returned by the service.
* @sample AWSIoT1ClickProjectsAsync.DeleteProject
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteProjectAsync(DeleteProjectRequest deleteProjectRequest);
/**
*
* Deletes a project. To delete a project, it must not have any placements associated with it.
*
*
*
* When you delete a project, all associated data becomes irretrievable.
*
*
*
* @param deleteProjectRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteProject operation returned by the service.
* @sample AWSIoT1ClickProjectsAsyncHandler.DeleteProject
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteProjectAsync(DeleteProjectRequest deleteProjectRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Describes a placement in a project.
*
*
* @param describePlacementRequest
* @return A Java Future containing the result of the DescribePlacement operation returned by the service.
* @sample AWSIoT1ClickProjectsAsync.DescribePlacement
* @see AWS API Documentation
*/
java.util.concurrent.Future describePlacementAsync(DescribePlacementRequest describePlacementRequest);
/**
*
* Describes a placement in a project.
*
*
* @param describePlacementRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribePlacement operation returned by the service.
* @sample AWSIoT1ClickProjectsAsyncHandler.DescribePlacement
* @see AWS API Documentation
*/
java.util.concurrent.Future describePlacementAsync(DescribePlacementRequest describePlacementRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns an object describing a project.
*
*
* @param describeProjectRequest
* @return A Java Future containing the result of the DescribeProject operation returned by the service.
* @sample AWSIoT1ClickProjectsAsync.DescribeProject
* @see AWS API Documentation
*/
java.util.concurrent.Future describeProjectAsync(DescribeProjectRequest describeProjectRequest);
/**
*
* Returns an object describing a project.
*
*
* @param describeProjectRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeProject operation returned by the service.
* @sample AWSIoT1ClickProjectsAsyncHandler.DescribeProject
* @see AWS API Documentation
*/
java.util.concurrent.Future describeProjectAsync(DescribeProjectRequest describeProjectRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Removes a physical device from a placement.
*
*
* @param disassociateDeviceFromPlacementRequest
* @return A Java Future containing the result of the DisassociateDeviceFromPlacement operation returned by the
* service.
* @sample AWSIoT1ClickProjectsAsync.DisassociateDeviceFromPlacement
* @see AWS API Documentation
*/
java.util.concurrent.Future disassociateDeviceFromPlacementAsync(
DisassociateDeviceFromPlacementRequest disassociateDeviceFromPlacementRequest);
/**
*
* Removes a physical device from a placement.
*
*
* @param disassociateDeviceFromPlacementRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DisassociateDeviceFromPlacement operation returned by the
* service.
* @sample AWSIoT1ClickProjectsAsyncHandler.DisassociateDeviceFromPlacement
* @see AWS API Documentation
*/
java.util.concurrent.Future disassociateDeviceFromPlacementAsync(
DisassociateDeviceFromPlacementRequest disassociateDeviceFromPlacementRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns an object enumerating the devices in a placement.
*
*
* @param getDevicesInPlacementRequest
* @return A Java Future containing the result of the GetDevicesInPlacement operation returned by the service.
* @sample AWSIoT1ClickProjectsAsync.GetDevicesInPlacement
* @see AWS API Documentation
*/
java.util.concurrent.Future getDevicesInPlacementAsync(GetDevicesInPlacementRequest getDevicesInPlacementRequest);
/**
*
* Returns an object enumerating the devices in a placement.
*
*
* @param getDevicesInPlacementRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetDevicesInPlacement operation returned by the service.
* @sample AWSIoT1ClickProjectsAsyncHandler.GetDevicesInPlacement
* @see AWS API Documentation
*/
java.util.concurrent.Future getDevicesInPlacementAsync(GetDevicesInPlacementRequest getDevicesInPlacementRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists the placement(s) of a project.
*
*
* @param listPlacementsRequest
* @return A Java Future containing the result of the ListPlacements operation returned by the service.
* @sample AWSIoT1ClickProjectsAsync.ListPlacements
* @see AWS API Documentation
*/
java.util.concurrent.Future listPlacementsAsync(ListPlacementsRequest listPlacementsRequest);
/**
*
* Lists the placement(s) of a project.
*
*
* @param listPlacementsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListPlacements operation returned by the service.
* @sample AWSIoT1ClickProjectsAsyncHandler.ListPlacements
* @see AWS API Documentation
*/
java.util.concurrent.Future listPlacementsAsync(ListPlacementsRequest listPlacementsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists the AWS IoT 1-Click project(s) associated with your AWS account and region.
*
*
* @param listProjectsRequest
* @return A Java Future containing the result of the ListProjects operation returned by the service.
* @sample AWSIoT1ClickProjectsAsync.ListProjects
* @see AWS API Documentation
*/
java.util.concurrent.Future listProjectsAsync(ListProjectsRequest listProjectsRequest);
/**
*
* Lists the AWS IoT 1-Click project(s) associated with your AWS account and region.
*
*
* @param listProjectsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListProjects operation returned by the service.
* @sample AWSIoT1ClickProjectsAsyncHandler.ListProjects
* @see AWS API Documentation
*/
java.util.concurrent.Future listProjectsAsync(ListProjectsRequest listProjectsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists the tags (metadata key/value pairs) which you have assigned to the resource.
*
*
* @param listTagsForResourceRequest
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* @sample AWSIoT1ClickProjectsAsync.ListTagsForResource
* @see AWS API Documentation
*/
java.util.concurrent.Future listTagsForResourceAsync(ListTagsForResourceRequest listTagsForResourceRequest);
/**
*
* Lists the tags (metadata key/value pairs) which you have assigned to the resource.
*
*
* @param listTagsForResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* @sample AWSIoT1ClickProjectsAsyncHandler.ListTagsForResource
* @see AWS API Documentation
*/
java.util.concurrent.Future listTagsForResourceAsync(ListTagsForResourceRequest listTagsForResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates or modifies tags for a resource. Tags are key/value pairs (metadata) that can be used to manage a
* resource. For more information, see AWS Tagging Strategies.
*
*
* @param tagResourceRequest
* @return A Java Future containing the result of the TagResource operation returned by the service.
* @sample AWSIoT1ClickProjectsAsync.TagResource
* @see AWS
* API Documentation
*/
java.util.concurrent.Future tagResourceAsync(TagResourceRequest tagResourceRequest);
/**
*
* Creates or modifies tags for a resource. Tags are key/value pairs (metadata) that can be used to manage a
* resource. For more information, see AWS Tagging Strategies.
*
*
* @param tagResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the TagResource operation returned by the service.
* @sample AWSIoT1ClickProjectsAsyncHandler.TagResource
* @see AWS
* API Documentation
*/
java.util.concurrent.Future tagResourceAsync(TagResourceRequest tagResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Removes one or more tags (metadata key/value pairs) from a resource.
*
*
* @param untagResourceRequest
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* @sample AWSIoT1ClickProjectsAsync.UntagResource
* @see AWS API Documentation
*/
java.util.concurrent.Future untagResourceAsync(UntagResourceRequest untagResourceRequest);
/**
*
* Removes one or more tags (metadata key/value pairs) from a resource.
*
*
* @param untagResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* @sample AWSIoT1ClickProjectsAsyncHandler.UntagResource
* @see AWS API Documentation
*/
java.util.concurrent.Future untagResourceAsync(UntagResourceRequest untagResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates a placement with the given attributes. To clear an attribute, pass an empty value (i.e., "").
*
*
* @param updatePlacementRequest
* @return A Java Future containing the result of the UpdatePlacement operation returned by the service.
* @sample AWSIoT1ClickProjectsAsync.UpdatePlacement
* @see AWS API Documentation
*/
java.util.concurrent.Future updatePlacementAsync(UpdatePlacementRequest updatePlacementRequest);
/**
*
* Updates a placement with the given attributes. To clear an attribute, pass an empty value (i.e., "").
*
*
* @param updatePlacementRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdatePlacement operation returned by the service.
* @sample AWSIoT1ClickProjectsAsyncHandler.UpdatePlacement
* @see AWS API Documentation
*/
java.util.concurrent.Future updatePlacementAsync(UpdatePlacementRequest updatePlacementRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates a project associated with your AWS account and region. With the exception of device template names, you
* can pass just the values that need to be updated because the update request will change only the values that are
* provided. To clear a value, pass the empty string (i.e., ""
).
*
*
* @param updateProjectRequest
* @return A Java Future containing the result of the UpdateProject operation returned by the service.
* @sample AWSIoT1ClickProjectsAsync.UpdateProject
* @see AWS API Documentation
*/
java.util.concurrent.Future updateProjectAsync(UpdateProjectRequest updateProjectRequest);
/**
*
* Updates a project associated with your AWS account and region. With the exception of device template names, you
* can pass just the values that need to be updated because the update request will change only the values that are
* provided. To clear a value, pass the empty string (i.e., ""
).
*
*
* @param updateProjectRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateProject operation returned by the service.
* @sample AWSIoT1ClickProjectsAsyncHandler.UpdateProject
* @see AWS API Documentation
*/
java.util.concurrent.Future updateProjectAsync(UpdateProjectRequest updateProjectRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
}