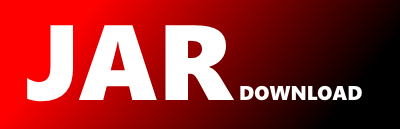
com.amazonaws.services.iotanalytics.model.PipelineActivity Maven / Gradle / Ivy
Show all versions of aws-java-sdk-iotanalytics Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.iotanalytics.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* An activity that performs a transformation on a message.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class PipelineActivity implements Serializable, Cloneable, StructuredPojo {
/**
*
* Determines the source of the messages to be processed.
*
*/
private ChannelActivity channel;
/**
*
* Runs a Lambda function to modify the message.
*
*/
private LambdaActivity lambda;
/**
*
* Specifies where to store the processed message data.
*
*/
private DatastoreActivity datastore;
/**
*
* Adds other attributes based on existing attributes in the message.
*
*/
private AddAttributesActivity addAttributes;
/**
*
* Removes attributes from a message.
*
*/
private RemoveAttributesActivity removeAttributes;
/**
*
* Used to create a new message using only the specified attributes from the original message.
*
*/
private SelectAttributesActivity selectAttributes;
/**
*
* Filters a message based on its attributes.
*
*/
private FilterActivity filter;
/**
*
* Computes an arithmetic expression using the message's attributes and adds it to the message.
*
*/
private MathActivity math;
/**
*
* Adds data from the IoT device registry to your message.
*
*/
private DeviceRegistryEnrichActivity deviceRegistryEnrich;
/**
*
* Adds information from the IoT Device Shadow service to a message.
*
*/
private DeviceShadowEnrichActivity deviceShadowEnrich;
/**
*
* Determines the source of the messages to be processed.
*
*
* @param channel
* Determines the source of the messages to be processed.
*/
public void setChannel(ChannelActivity channel) {
this.channel = channel;
}
/**
*
* Determines the source of the messages to be processed.
*
*
* @return Determines the source of the messages to be processed.
*/
public ChannelActivity getChannel() {
return this.channel;
}
/**
*
* Determines the source of the messages to be processed.
*
*
* @param channel
* Determines the source of the messages to be processed.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PipelineActivity withChannel(ChannelActivity channel) {
setChannel(channel);
return this;
}
/**
*
* Runs a Lambda function to modify the message.
*
*
* @param lambda
* Runs a Lambda function to modify the message.
*/
public void setLambda(LambdaActivity lambda) {
this.lambda = lambda;
}
/**
*
* Runs a Lambda function to modify the message.
*
*
* @return Runs a Lambda function to modify the message.
*/
public LambdaActivity getLambda() {
return this.lambda;
}
/**
*
* Runs a Lambda function to modify the message.
*
*
* @param lambda
* Runs a Lambda function to modify the message.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PipelineActivity withLambda(LambdaActivity lambda) {
setLambda(lambda);
return this;
}
/**
*
* Specifies where to store the processed message data.
*
*
* @param datastore
* Specifies where to store the processed message data.
*/
public void setDatastore(DatastoreActivity datastore) {
this.datastore = datastore;
}
/**
*
* Specifies where to store the processed message data.
*
*
* @return Specifies where to store the processed message data.
*/
public DatastoreActivity getDatastore() {
return this.datastore;
}
/**
*
* Specifies where to store the processed message data.
*
*
* @param datastore
* Specifies where to store the processed message data.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PipelineActivity withDatastore(DatastoreActivity datastore) {
setDatastore(datastore);
return this;
}
/**
*
* Adds other attributes based on existing attributes in the message.
*
*
* @param addAttributes
* Adds other attributes based on existing attributes in the message.
*/
public void setAddAttributes(AddAttributesActivity addAttributes) {
this.addAttributes = addAttributes;
}
/**
*
* Adds other attributes based on existing attributes in the message.
*
*
* @return Adds other attributes based on existing attributes in the message.
*/
public AddAttributesActivity getAddAttributes() {
return this.addAttributes;
}
/**
*
* Adds other attributes based on existing attributes in the message.
*
*
* @param addAttributes
* Adds other attributes based on existing attributes in the message.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PipelineActivity withAddAttributes(AddAttributesActivity addAttributes) {
setAddAttributes(addAttributes);
return this;
}
/**
*
* Removes attributes from a message.
*
*
* @param removeAttributes
* Removes attributes from a message.
*/
public void setRemoveAttributes(RemoveAttributesActivity removeAttributes) {
this.removeAttributes = removeAttributes;
}
/**
*
* Removes attributes from a message.
*
*
* @return Removes attributes from a message.
*/
public RemoveAttributesActivity getRemoveAttributes() {
return this.removeAttributes;
}
/**
*
* Removes attributes from a message.
*
*
* @param removeAttributes
* Removes attributes from a message.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PipelineActivity withRemoveAttributes(RemoveAttributesActivity removeAttributes) {
setRemoveAttributes(removeAttributes);
return this;
}
/**
*
* Used to create a new message using only the specified attributes from the original message.
*
*
* @param selectAttributes
* Used to create a new message using only the specified attributes from the original message.
*/
public void setSelectAttributes(SelectAttributesActivity selectAttributes) {
this.selectAttributes = selectAttributes;
}
/**
*
* Used to create a new message using only the specified attributes from the original message.
*
*
* @return Used to create a new message using only the specified attributes from the original message.
*/
public SelectAttributesActivity getSelectAttributes() {
return this.selectAttributes;
}
/**
*
* Used to create a new message using only the specified attributes from the original message.
*
*
* @param selectAttributes
* Used to create a new message using only the specified attributes from the original message.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PipelineActivity withSelectAttributes(SelectAttributesActivity selectAttributes) {
setSelectAttributes(selectAttributes);
return this;
}
/**
*
* Filters a message based on its attributes.
*
*
* @param filter
* Filters a message based on its attributes.
*/
public void setFilter(FilterActivity filter) {
this.filter = filter;
}
/**
*
* Filters a message based on its attributes.
*
*
* @return Filters a message based on its attributes.
*/
public FilterActivity getFilter() {
return this.filter;
}
/**
*
* Filters a message based on its attributes.
*
*
* @param filter
* Filters a message based on its attributes.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PipelineActivity withFilter(FilterActivity filter) {
setFilter(filter);
return this;
}
/**
*
* Computes an arithmetic expression using the message's attributes and adds it to the message.
*
*
* @param math
* Computes an arithmetic expression using the message's attributes and adds it to the message.
*/
public void setMath(MathActivity math) {
this.math = math;
}
/**
*
* Computes an arithmetic expression using the message's attributes and adds it to the message.
*
*
* @return Computes an arithmetic expression using the message's attributes and adds it to the message.
*/
public MathActivity getMath() {
return this.math;
}
/**
*
* Computes an arithmetic expression using the message's attributes and adds it to the message.
*
*
* @param math
* Computes an arithmetic expression using the message's attributes and adds it to the message.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PipelineActivity withMath(MathActivity math) {
setMath(math);
return this;
}
/**
*
* Adds data from the IoT device registry to your message.
*
*
* @param deviceRegistryEnrich
* Adds data from the IoT device registry to your message.
*/
public void setDeviceRegistryEnrich(DeviceRegistryEnrichActivity deviceRegistryEnrich) {
this.deviceRegistryEnrich = deviceRegistryEnrich;
}
/**
*
* Adds data from the IoT device registry to your message.
*
*
* @return Adds data from the IoT device registry to your message.
*/
public DeviceRegistryEnrichActivity getDeviceRegistryEnrich() {
return this.deviceRegistryEnrich;
}
/**
*
* Adds data from the IoT device registry to your message.
*
*
* @param deviceRegistryEnrich
* Adds data from the IoT device registry to your message.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PipelineActivity withDeviceRegistryEnrich(DeviceRegistryEnrichActivity deviceRegistryEnrich) {
setDeviceRegistryEnrich(deviceRegistryEnrich);
return this;
}
/**
*
* Adds information from the IoT Device Shadow service to a message.
*
*
* @param deviceShadowEnrich
* Adds information from the IoT Device Shadow service to a message.
*/
public void setDeviceShadowEnrich(DeviceShadowEnrichActivity deviceShadowEnrich) {
this.deviceShadowEnrich = deviceShadowEnrich;
}
/**
*
* Adds information from the IoT Device Shadow service to a message.
*
*
* @return Adds information from the IoT Device Shadow service to a message.
*/
public DeviceShadowEnrichActivity getDeviceShadowEnrich() {
return this.deviceShadowEnrich;
}
/**
*
* Adds information from the IoT Device Shadow service to a message.
*
*
* @param deviceShadowEnrich
* Adds information from the IoT Device Shadow service to a message.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PipelineActivity withDeviceShadowEnrich(DeviceShadowEnrichActivity deviceShadowEnrich) {
setDeviceShadowEnrich(deviceShadowEnrich);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getChannel() != null)
sb.append("Channel: ").append(getChannel()).append(",");
if (getLambda() != null)
sb.append("Lambda: ").append(getLambda()).append(",");
if (getDatastore() != null)
sb.append("Datastore: ").append(getDatastore()).append(",");
if (getAddAttributes() != null)
sb.append("AddAttributes: ").append(getAddAttributes()).append(",");
if (getRemoveAttributes() != null)
sb.append("RemoveAttributes: ").append(getRemoveAttributes()).append(",");
if (getSelectAttributes() != null)
sb.append("SelectAttributes: ").append(getSelectAttributes()).append(",");
if (getFilter() != null)
sb.append("Filter: ").append(getFilter()).append(",");
if (getMath() != null)
sb.append("Math: ").append(getMath()).append(",");
if (getDeviceRegistryEnrich() != null)
sb.append("DeviceRegistryEnrich: ").append(getDeviceRegistryEnrich()).append(",");
if (getDeviceShadowEnrich() != null)
sb.append("DeviceShadowEnrich: ").append(getDeviceShadowEnrich());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof PipelineActivity == false)
return false;
PipelineActivity other = (PipelineActivity) obj;
if (other.getChannel() == null ^ this.getChannel() == null)
return false;
if (other.getChannel() != null && other.getChannel().equals(this.getChannel()) == false)
return false;
if (other.getLambda() == null ^ this.getLambda() == null)
return false;
if (other.getLambda() != null && other.getLambda().equals(this.getLambda()) == false)
return false;
if (other.getDatastore() == null ^ this.getDatastore() == null)
return false;
if (other.getDatastore() != null && other.getDatastore().equals(this.getDatastore()) == false)
return false;
if (other.getAddAttributes() == null ^ this.getAddAttributes() == null)
return false;
if (other.getAddAttributes() != null && other.getAddAttributes().equals(this.getAddAttributes()) == false)
return false;
if (other.getRemoveAttributes() == null ^ this.getRemoveAttributes() == null)
return false;
if (other.getRemoveAttributes() != null && other.getRemoveAttributes().equals(this.getRemoveAttributes()) == false)
return false;
if (other.getSelectAttributes() == null ^ this.getSelectAttributes() == null)
return false;
if (other.getSelectAttributes() != null && other.getSelectAttributes().equals(this.getSelectAttributes()) == false)
return false;
if (other.getFilter() == null ^ this.getFilter() == null)
return false;
if (other.getFilter() != null && other.getFilter().equals(this.getFilter()) == false)
return false;
if (other.getMath() == null ^ this.getMath() == null)
return false;
if (other.getMath() != null && other.getMath().equals(this.getMath()) == false)
return false;
if (other.getDeviceRegistryEnrich() == null ^ this.getDeviceRegistryEnrich() == null)
return false;
if (other.getDeviceRegistryEnrich() != null && other.getDeviceRegistryEnrich().equals(this.getDeviceRegistryEnrich()) == false)
return false;
if (other.getDeviceShadowEnrich() == null ^ this.getDeviceShadowEnrich() == null)
return false;
if (other.getDeviceShadowEnrich() != null && other.getDeviceShadowEnrich().equals(this.getDeviceShadowEnrich()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getChannel() == null) ? 0 : getChannel().hashCode());
hashCode = prime * hashCode + ((getLambda() == null) ? 0 : getLambda().hashCode());
hashCode = prime * hashCode + ((getDatastore() == null) ? 0 : getDatastore().hashCode());
hashCode = prime * hashCode + ((getAddAttributes() == null) ? 0 : getAddAttributes().hashCode());
hashCode = prime * hashCode + ((getRemoveAttributes() == null) ? 0 : getRemoveAttributes().hashCode());
hashCode = prime * hashCode + ((getSelectAttributes() == null) ? 0 : getSelectAttributes().hashCode());
hashCode = prime * hashCode + ((getFilter() == null) ? 0 : getFilter().hashCode());
hashCode = prime * hashCode + ((getMath() == null) ? 0 : getMath().hashCode());
hashCode = prime * hashCode + ((getDeviceRegistryEnrich() == null) ? 0 : getDeviceRegistryEnrich().hashCode());
hashCode = prime * hashCode + ((getDeviceShadowEnrich() == null) ? 0 : getDeviceShadowEnrich().hashCode());
return hashCode;
}
@Override
public PipelineActivity clone() {
try {
return (PipelineActivity) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.iotanalytics.model.transform.PipelineActivityMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}