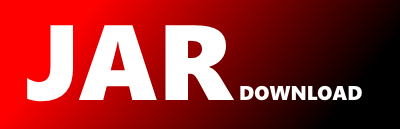
com.amazonaws.services.iotanalytics.AWSIoTAnalyticsAsync Maven / Gradle / Ivy
Show all versions of aws-java-sdk-iotanalytics Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.iotanalytics;
import javax.annotation.Generated;
import com.amazonaws.services.iotanalytics.model.*;
/**
* Interface for accessing AWS IoT Analytics asynchronously. Each asynchronous method will return a Java Future object
* representing the asynchronous operation; overloads which accept an {@code AsyncHandler} can be used to receive
* notification when an asynchronous operation completes.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.iotanalytics.AbstractAWSIoTAnalyticsAsync} instead.
*
*
*
* IoT Analytics allows you to collect large amounts of device data, process messages, and store them. You can then
* query the data and run sophisticated analytics on it. IoT Analytics enables advanced data exploration through
* integration with Jupyter Notebooks and data visualization through integration with Amazon QuickSight.
*
*
* Traditional analytics and business intelligence tools are designed to process structured data. IoT data often comes
* from devices that record noisy processes (such as temperature, motion, or sound). As a result the data from these
* devices can have significant gaps, corrupted messages, and false readings that must be cleaned up before analysis can
* occur. Also, IoT data is often only meaningful in the context of other data from external sources.
*
*
* IoT Analytics automates the steps required to analyze data from IoT devices. IoT Analytics filters, transforms, and
* enriches IoT data before storing it in a time-series data store for analysis. You can set up the service to collect
* only the data you need from your devices, apply mathematical transforms to process the data, and enrich the data with
* device-specific metadata such as device type and location before storing it. Then, you can analyze your data by
* running queries using the built-in SQL query engine, or perform more complex analytics and machine learning
* inference. IoT Analytics includes pre-built models for common IoT use cases so you can answer questions like which
* devices are about to fail or which customers are at risk of abandoning their wearable devices.
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AWSIoTAnalyticsAsync extends AWSIoTAnalytics {
/**
*
* Sends messages to a channel.
*
*
* @param batchPutMessageRequest
* @return A Java Future containing the result of the BatchPutMessage operation returned by the service.
* @sample AWSIoTAnalyticsAsync.BatchPutMessage
* @see AWS
* API Documentation
*/
java.util.concurrent.Future batchPutMessageAsync(BatchPutMessageRequest batchPutMessageRequest);
/**
*
* Sends messages to a channel.
*
*
* @param batchPutMessageRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the BatchPutMessage operation returned by the service.
* @sample AWSIoTAnalyticsAsyncHandler.BatchPutMessage
* @see AWS
* API Documentation
*/
java.util.concurrent.Future batchPutMessageAsync(BatchPutMessageRequest batchPutMessageRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Cancels the reprocessing of data through the pipeline.
*
*
* @param cancelPipelineReprocessingRequest
* @return A Java Future containing the result of the CancelPipelineReprocessing operation returned by the service.
* @sample AWSIoTAnalyticsAsync.CancelPipelineReprocessing
* @see AWS API Documentation
*/
java.util.concurrent.Future cancelPipelineReprocessingAsync(
CancelPipelineReprocessingRequest cancelPipelineReprocessingRequest);
/**
*
* Cancels the reprocessing of data through the pipeline.
*
*
* @param cancelPipelineReprocessingRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CancelPipelineReprocessing operation returned by the service.
* @sample AWSIoTAnalyticsAsyncHandler.CancelPipelineReprocessing
* @see AWS API Documentation
*/
java.util.concurrent.Future cancelPipelineReprocessingAsync(
CancelPipelineReprocessingRequest cancelPipelineReprocessingRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Used to create a channel. A channel collects data from an MQTT topic and archives the raw, unprocessed messages
* before publishing the data to a pipeline.
*
*
* @param createChannelRequest
* @return A Java Future containing the result of the CreateChannel operation returned by the service.
* @sample AWSIoTAnalyticsAsync.CreateChannel
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createChannelAsync(CreateChannelRequest createChannelRequest);
/**
*
* Used to create a channel. A channel collects data from an MQTT topic and archives the raw, unprocessed messages
* before publishing the data to a pipeline.
*
*
* @param createChannelRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateChannel operation returned by the service.
* @sample AWSIoTAnalyticsAsyncHandler.CreateChannel
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createChannelAsync(CreateChannelRequest createChannelRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Used to create a dataset. A dataset stores data retrieved from a data store by applying a
* queryAction
(a SQL query) or a containerAction
(executing a containerized application).
* This operation creates the skeleton of a dataset. The dataset can be populated manually by calling
* CreateDatasetContent
or automatically according to a trigger you specify.
*
*
* @param createDatasetRequest
* @return A Java Future containing the result of the CreateDataset operation returned by the service.
* @sample AWSIoTAnalyticsAsync.CreateDataset
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createDatasetAsync(CreateDatasetRequest createDatasetRequest);
/**
*
* Used to create a dataset. A dataset stores data retrieved from a data store by applying a
* queryAction
(a SQL query) or a containerAction
(executing a containerized application).
* This operation creates the skeleton of a dataset. The dataset can be populated manually by calling
* CreateDatasetContent
or automatically according to a trigger you specify.
*
*
* @param createDatasetRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateDataset operation returned by the service.
* @sample AWSIoTAnalyticsAsyncHandler.CreateDataset
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createDatasetAsync(CreateDatasetRequest createDatasetRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates the content of a dataset by applying a queryAction
(a SQL query) or a
* containerAction
(executing a containerized application).
*
*
* @param createDatasetContentRequest
* @return A Java Future containing the result of the CreateDatasetContent operation returned by the service.
* @sample AWSIoTAnalyticsAsync.CreateDatasetContent
* @see AWS API Documentation
*/
java.util.concurrent.Future createDatasetContentAsync(CreateDatasetContentRequest createDatasetContentRequest);
/**
*
* Creates the content of a dataset by applying a queryAction
(a SQL query) or a
* containerAction
(executing a containerized application).
*
*
* @param createDatasetContentRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateDatasetContent operation returned by the service.
* @sample AWSIoTAnalyticsAsyncHandler.CreateDatasetContent
* @see AWS API Documentation
*/
java.util.concurrent.Future createDatasetContentAsync(CreateDatasetContentRequest createDatasetContentRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a data store, which is a repository for messages.
*
*
* @param createDatastoreRequest
* @return A Java Future containing the result of the CreateDatastore operation returned by the service.
* @sample AWSIoTAnalyticsAsync.CreateDatastore
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createDatastoreAsync(CreateDatastoreRequest createDatastoreRequest);
/**
*
* Creates a data store, which is a repository for messages.
*
*
* @param createDatastoreRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateDatastore operation returned by the service.
* @sample AWSIoTAnalyticsAsyncHandler.CreateDatastore
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createDatastoreAsync(CreateDatastoreRequest createDatastoreRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a pipeline. A pipeline consumes messages from a channel and allows you to process the messages before
* storing them in a data store. You must specify both a channel
and a datastore
activity
* and, optionally, as many as 23 additional activities in the pipelineActivities
array.
*
*
* @param createPipelineRequest
* @return A Java Future containing the result of the CreatePipeline operation returned by the service.
* @sample AWSIoTAnalyticsAsync.CreatePipeline
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createPipelineAsync(CreatePipelineRequest createPipelineRequest);
/**
*
* Creates a pipeline. A pipeline consumes messages from a channel and allows you to process the messages before
* storing them in a data store. You must specify both a channel
and a datastore
activity
* and, optionally, as many as 23 additional activities in the pipelineActivities
array.
*
*
* @param createPipelineRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreatePipeline operation returned by the service.
* @sample AWSIoTAnalyticsAsyncHandler.CreatePipeline
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createPipelineAsync(CreatePipelineRequest createPipelineRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the specified channel.
*
*
* @param deleteChannelRequest
* @return A Java Future containing the result of the DeleteChannel operation returned by the service.
* @sample AWSIoTAnalyticsAsync.DeleteChannel
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteChannelAsync(DeleteChannelRequest deleteChannelRequest);
/**
*
* Deletes the specified channel.
*
*
* @param deleteChannelRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteChannel operation returned by the service.
* @sample AWSIoTAnalyticsAsyncHandler.DeleteChannel
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteChannelAsync(DeleteChannelRequest deleteChannelRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the specified dataset.
*
*
* You do not have to delete the content of the dataset before you perform this operation.
*
*
* @param deleteDatasetRequest
* @return A Java Future containing the result of the DeleteDataset operation returned by the service.
* @sample AWSIoTAnalyticsAsync.DeleteDataset
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteDatasetAsync(DeleteDatasetRequest deleteDatasetRequest);
/**
*
* Deletes the specified dataset.
*
*
* You do not have to delete the content of the dataset before you perform this operation.
*
*
* @param deleteDatasetRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteDataset operation returned by the service.
* @sample AWSIoTAnalyticsAsyncHandler.DeleteDataset
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteDatasetAsync(DeleteDatasetRequest deleteDatasetRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the content of the specified dataset.
*
*
* @param deleteDatasetContentRequest
* @return A Java Future containing the result of the DeleteDatasetContent operation returned by the service.
* @sample AWSIoTAnalyticsAsync.DeleteDatasetContent
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteDatasetContentAsync(DeleteDatasetContentRequest deleteDatasetContentRequest);
/**
*
* Deletes the content of the specified dataset.
*
*
* @param deleteDatasetContentRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteDatasetContent operation returned by the service.
* @sample AWSIoTAnalyticsAsyncHandler.DeleteDatasetContent
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteDatasetContentAsync(DeleteDatasetContentRequest deleteDatasetContentRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the specified data store.
*
*
* @param deleteDatastoreRequest
* @return A Java Future containing the result of the DeleteDatastore operation returned by the service.
* @sample AWSIoTAnalyticsAsync.DeleteDatastore
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteDatastoreAsync(DeleteDatastoreRequest deleteDatastoreRequest);
/**
*
* Deletes the specified data store.
*
*
* @param deleteDatastoreRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteDatastore operation returned by the service.
* @sample AWSIoTAnalyticsAsyncHandler.DeleteDatastore
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteDatastoreAsync(DeleteDatastoreRequest deleteDatastoreRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the specified pipeline.
*
*
* @param deletePipelineRequest
* @return A Java Future containing the result of the DeletePipeline operation returned by the service.
* @sample AWSIoTAnalyticsAsync.DeletePipeline
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deletePipelineAsync(DeletePipelineRequest deletePipelineRequest);
/**
*
* Deletes the specified pipeline.
*
*
* @param deletePipelineRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeletePipeline operation returned by the service.
* @sample AWSIoTAnalyticsAsyncHandler.DeletePipeline
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deletePipelineAsync(DeletePipelineRequest deletePipelineRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves information about a channel.
*
*
* @param describeChannelRequest
* @return A Java Future containing the result of the DescribeChannel operation returned by the service.
* @sample AWSIoTAnalyticsAsync.DescribeChannel
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeChannelAsync(DescribeChannelRequest describeChannelRequest);
/**
*
* Retrieves information about a channel.
*
*
* @param describeChannelRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeChannel operation returned by the service.
* @sample AWSIoTAnalyticsAsyncHandler.DescribeChannel
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeChannelAsync(DescribeChannelRequest describeChannelRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves information about a dataset.
*
*
* @param describeDatasetRequest
* @return A Java Future containing the result of the DescribeDataset operation returned by the service.
* @sample AWSIoTAnalyticsAsync.DescribeDataset
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeDatasetAsync(DescribeDatasetRequest describeDatasetRequest);
/**
*
* Retrieves information about a dataset.
*
*
* @param describeDatasetRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeDataset operation returned by the service.
* @sample AWSIoTAnalyticsAsyncHandler.DescribeDataset
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeDatasetAsync(DescribeDatasetRequest describeDatasetRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves information about a data store.
*
*
* @param describeDatastoreRequest
* @return A Java Future containing the result of the DescribeDatastore operation returned by the service.
* @sample AWSIoTAnalyticsAsync.DescribeDatastore
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeDatastoreAsync(DescribeDatastoreRequest describeDatastoreRequest);
/**
*
* Retrieves information about a data store.
*
*
* @param describeDatastoreRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeDatastore operation returned by the service.
* @sample AWSIoTAnalyticsAsyncHandler.DescribeDatastore
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeDatastoreAsync(DescribeDatastoreRequest describeDatastoreRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves the current settings of the IoT Analytics logging options.
*
*
* @param describeLoggingOptionsRequest
* @return A Java Future containing the result of the DescribeLoggingOptions operation returned by the service.
* @sample AWSIoTAnalyticsAsync.DescribeLoggingOptions
* @see AWS API Documentation
*/
java.util.concurrent.Future describeLoggingOptionsAsync(DescribeLoggingOptionsRequest describeLoggingOptionsRequest);
/**
*
* Retrieves the current settings of the IoT Analytics logging options.
*
*
* @param describeLoggingOptionsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeLoggingOptions operation returned by the service.
* @sample AWSIoTAnalyticsAsyncHandler.DescribeLoggingOptions
* @see AWS API Documentation
*/
java.util.concurrent.Future describeLoggingOptionsAsync(DescribeLoggingOptionsRequest describeLoggingOptionsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves information about a pipeline.
*
*
* @param describePipelineRequest
* @return A Java Future containing the result of the DescribePipeline operation returned by the service.
* @sample AWSIoTAnalyticsAsync.DescribePipeline
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describePipelineAsync(DescribePipelineRequest describePipelineRequest);
/**
*
* Retrieves information about a pipeline.
*
*
* @param describePipelineRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribePipeline operation returned by the service.
* @sample AWSIoTAnalyticsAsyncHandler.DescribePipeline
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describePipelineAsync(DescribePipelineRequest describePipelineRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves the contents of a dataset as presigned URIs.
*
*
* @param getDatasetContentRequest
* @return A Java Future containing the result of the GetDatasetContent operation returned by the service.
* @sample AWSIoTAnalyticsAsync.GetDatasetContent
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getDatasetContentAsync(GetDatasetContentRequest getDatasetContentRequest);
/**
*
* Retrieves the contents of a dataset as presigned URIs.
*
*
* @param getDatasetContentRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetDatasetContent operation returned by the service.
* @sample AWSIoTAnalyticsAsyncHandler.GetDatasetContent
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getDatasetContentAsync(GetDatasetContentRequest getDatasetContentRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves a list of channels.
*
*
* @param listChannelsRequest
* @return A Java Future containing the result of the ListChannels operation returned by the service.
* @sample AWSIoTAnalyticsAsync.ListChannels
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listChannelsAsync(ListChannelsRequest listChannelsRequest);
/**
*
* Retrieves a list of channels.
*
*
* @param listChannelsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListChannels operation returned by the service.
* @sample AWSIoTAnalyticsAsyncHandler.ListChannels
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listChannelsAsync(ListChannelsRequest listChannelsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists information about dataset contents that have been created.
*
*
* @param listDatasetContentsRequest
* @return A Java Future containing the result of the ListDatasetContents operation returned by the service.
* @sample AWSIoTAnalyticsAsync.ListDatasetContents
* @see AWS API Documentation
*/
java.util.concurrent.Future listDatasetContentsAsync(ListDatasetContentsRequest listDatasetContentsRequest);
/**
*
* Lists information about dataset contents that have been created.
*
*
* @param listDatasetContentsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListDatasetContents operation returned by the service.
* @sample AWSIoTAnalyticsAsyncHandler.ListDatasetContents
* @see AWS API Documentation
*/
java.util.concurrent.Future listDatasetContentsAsync(ListDatasetContentsRequest listDatasetContentsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves information about datasets.
*
*
* @param listDatasetsRequest
* @return A Java Future containing the result of the ListDatasets operation returned by the service.
* @sample AWSIoTAnalyticsAsync.ListDatasets
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listDatasetsAsync(ListDatasetsRequest listDatasetsRequest);
/**
*
* Retrieves information about datasets.
*
*
* @param listDatasetsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListDatasets operation returned by the service.
* @sample AWSIoTAnalyticsAsyncHandler.ListDatasets
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listDatasetsAsync(ListDatasetsRequest listDatasetsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves a list of data stores.
*
*
* @param listDatastoresRequest
* @return A Java Future containing the result of the ListDatastores operation returned by the service.
* @sample AWSIoTAnalyticsAsync.ListDatastores
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listDatastoresAsync(ListDatastoresRequest listDatastoresRequest);
/**
*
* Retrieves a list of data stores.
*
*
* @param listDatastoresRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListDatastores operation returned by the service.
* @sample AWSIoTAnalyticsAsyncHandler.ListDatastores
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listDatastoresAsync(ListDatastoresRequest listDatastoresRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves a list of pipelines.
*
*
* @param listPipelinesRequest
* @return A Java Future containing the result of the ListPipelines operation returned by the service.
* @sample AWSIoTAnalyticsAsync.ListPipelines
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listPipelinesAsync(ListPipelinesRequest listPipelinesRequest);
/**
*
* Retrieves a list of pipelines.
*
*
* @param listPipelinesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListPipelines operation returned by the service.
* @sample AWSIoTAnalyticsAsyncHandler.ListPipelines
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listPipelinesAsync(ListPipelinesRequest listPipelinesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists the tags (metadata) that you have assigned to the resource.
*
*
* @param listTagsForResourceRequest
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* @sample AWSIoTAnalyticsAsync.ListTagsForResource
* @see AWS API Documentation
*/
java.util.concurrent.Future listTagsForResourceAsync(ListTagsForResourceRequest listTagsForResourceRequest);
/**
*
* Lists the tags (metadata) that you have assigned to the resource.
*
*
* @param listTagsForResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* @sample AWSIoTAnalyticsAsyncHandler.ListTagsForResource
* @see AWS API Documentation
*/
java.util.concurrent.Future listTagsForResourceAsync(ListTagsForResourceRequest listTagsForResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Sets or updates the IoT Analytics logging options.
*
*
* If you update the value of any loggingOptions
field, it takes up to one minute for the change to
* take effect. Also, if you change the policy attached to the role you specified in the roleArn
field
* (for example, to correct an invalid policy), it takes up to five minutes for that change to take effect.
*
*
* @param putLoggingOptionsRequest
* @return A Java Future containing the result of the PutLoggingOptions operation returned by the service.
* @sample AWSIoTAnalyticsAsync.PutLoggingOptions
* @see AWS
* API Documentation
*/
java.util.concurrent.Future putLoggingOptionsAsync(PutLoggingOptionsRequest putLoggingOptionsRequest);
/**
*
* Sets or updates the IoT Analytics logging options.
*
*
* If you update the value of any loggingOptions
field, it takes up to one minute for the change to
* take effect. Also, if you change the policy attached to the role you specified in the roleArn
field
* (for example, to correct an invalid policy), it takes up to five minutes for that change to take effect.
*
*
* @param putLoggingOptionsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the PutLoggingOptions operation returned by the service.
* @sample AWSIoTAnalyticsAsyncHandler.PutLoggingOptions
* @see AWS
* API Documentation
*/
java.util.concurrent.Future putLoggingOptionsAsync(PutLoggingOptionsRequest putLoggingOptionsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Simulates the results of running a pipeline activity on a message payload.
*
*
* @param runPipelineActivityRequest
* @return A Java Future containing the result of the RunPipelineActivity operation returned by the service.
* @sample AWSIoTAnalyticsAsync.RunPipelineActivity
* @see AWS API Documentation
*/
java.util.concurrent.Future runPipelineActivityAsync(RunPipelineActivityRequest runPipelineActivityRequest);
/**
*
* Simulates the results of running a pipeline activity on a message payload.
*
*
* @param runPipelineActivityRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the RunPipelineActivity operation returned by the service.
* @sample AWSIoTAnalyticsAsyncHandler.RunPipelineActivity
* @see AWS API Documentation
*/
java.util.concurrent.Future runPipelineActivityAsync(RunPipelineActivityRequest runPipelineActivityRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves a sample of messages from the specified channel ingested during the specified timeframe. Up to 10
* messages can be retrieved.
*
*
* @param sampleChannelDataRequest
* @return A Java Future containing the result of the SampleChannelData operation returned by the service.
* @sample AWSIoTAnalyticsAsync.SampleChannelData
* @see AWS
* API Documentation
*/
java.util.concurrent.Future sampleChannelDataAsync(SampleChannelDataRequest sampleChannelDataRequest);
/**
*
* Retrieves a sample of messages from the specified channel ingested during the specified timeframe. Up to 10
* messages can be retrieved.
*
*
* @param sampleChannelDataRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the SampleChannelData operation returned by the service.
* @sample AWSIoTAnalyticsAsyncHandler.SampleChannelData
* @see AWS
* API Documentation
*/
java.util.concurrent.Future sampleChannelDataAsync(SampleChannelDataRequest sampleChannelDataRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Starts the reprocessing of raw message data through the pipeline.
*
*
* @param startPipelineReprocessingRequest
* @return A Java Future containing the result of the StartPipelineReprocessing operation returned by the service.
* @sample AWSIoTAnalyticsAsync.StartPipelineReprocessing
* @see AWS API Documentation
*/
java.util.concurrent.Future startPipelineReprocessingAsync(
StartPipelineReprocessingRequest startPipelineReprocessingRequest);
/**
*
* Starts the reprocessing of raw message data through the pipeline.
*
*
* @param startPipelineReprocessingRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the StartPipelineReprocessing operation returned by the service.
* @sample AWSIoTAnalyticsAsyncHandler.StartPipelineReprocessing
* @see AWS API Documentation
*/
java.util.concurrent.Future startPipelineReprocessingAsync(
StartPipelineReprocessingRequest startPipelineReprocessingRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Adds to or modifies the tags of the given resource. Tags are metadata that can be used to manage a resource.
*
*
* @param tagResourceRequest
* @return A Java Future containing the result of the TagResource operation returned by the service.
* @sample AWSIoTAnalyticsAsync.TagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future tagResourceAsync(TagResourceRequest tagResourceRequest);
/**
*
* Adds to or modifies the tags of the given resource. Tags are metadata that can be used to manage a resource.
*
*
* @param tagResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the TagResource operation returned by the service.
* @sample AWSIoTAnalyticsAsyncHandler.TagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future tagResourceAsync(TagResourceRequest tagResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Removes the given tags (metadata) from the resource.
*
*
* @param untagResourceRequest
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* @sample AWSIoTAnalyticsAsync.UntagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future untagResourceAsync(UntagResourceRequest untagResourceRequest);
/**
*
* Removes the given tags (metadata) from the resource.
*
*
* @param untagResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* @sample AWSIoTAnalyticsAsyncHandler.UntagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future untagResourceAsync(UntagResourceRequest untagResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Used to update the settings of a channel.
*
*
* @param updateChannelRequest
* @return A Java Future containing the result of the UpdateChannel operation returned by the service.
* @sample AWSIoTAnalyticsAsync.UpdateChannel
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateChannelAsync(UpdateChannelRequest updateChannelRequest);
/**
*
* Used to update the settings of a channel.
*
*
* @param updateChannelRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateChannel operation returned by the service.
* @sample AWSIoTAnalyticsAsyncHandler.UpdateChannel
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateChannelAsync(UpdateChannelRequest updateChannelRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates the settings of a dataset.
*
*
* @param updateDatasetRequest
* @return A Java Future containing the result of the UpdateDataset operation returned by the service.
* @sample AWSIoTAnalyticsAsync.UpdateDataset
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateDatasetAsync(UpdateDatasetRequest updateDatasetRequest);
/**
*
* Updates the settings of a dataset.
*
*
* @param updateDatasetRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateDataset operation returned by the service.
* @sample AWSIoTAnalyticsAsyncHandler.UpdateDataset
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateDatasetAsync(UpdateDatasetRequest updateDatasetRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Used to update the settings of a data store.
*
*
* @param updateDatastoreRequest
* @return A Java Future containing the result of the UpdateDatastore operation returned by the service.
* @sample AWSIoTAnalyticsAsync.UpdateDatastore
* @see AWS
* API Documentation
*/
java.util.concurrent.Future updateDatastoreAsync(UpdateDatastoreRequest updateDatastoreRequest);
/**
*
* Used to update the settings of a data store.
*
*
* @param updateDatastoreRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateDatastore operation returned by the service.
* @sample AWSIoTAnalyticsAsyncHandler.UpdateDatastore
* @see AWS
* API Documentation
*/
java.util.concurrent.Future updateDatastoreAsync(UpdateDatastoreRequest updateDatastoreRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates the settings of a pipeline. You must specify both a channel
and a datastore
* activity and, optionally, as many as 23 additional activities in the pipelineActivities
array.
*
*
* @param updatePipelineRequest
* @return A Java Future containing the result of the UpdatePipeline operation returned by the service.
* @sample AWSIoTAnalyticsAsync.UpdatePipeline
* @see AWS
* API Documentation
*/
java.util.concurrent.Future updatePipelineAsync(UpdatePipelineRequest updatePipelineRequest);
/**
*
* Updates the settings of a pipeline. You must specify both a channel
and a datastore
* activity and, optionally, as many as 23 additional activities in the pipelineActivities
array.
*
*
* @param updatePipelineRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdatePipeline operation returned by the service.
* @sample AWSIoTAnalyticsAsyncHandler.UpdatePipeline
* @see AWS
* API Documentation
*/
java.util.concurrent.Future updatePipelineAsync(UpdatePipelineRequest updatePipelineRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
}