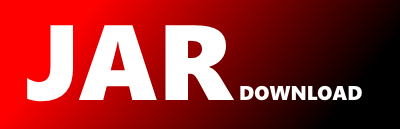
com.amazonaws.services.iotanalytics.model.CreateDatasetRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-iotanalytics Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.iotanalytics.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class CreateDatasetRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* The name of the dataset.
*
*/
private String datasetName;
/**
*
* A list of actions that create the dataset contents.
*
*/
private java.util.List actions;
/**
*
* A list of triggers. A trigger causes dataset contents to be populated at a specified time interval or when
* another dataset's contents are created. The list of triggers can be empty or contain up to five
* DataSetTrigger
objects.
*
*/
private java.util.List triggers;
/**
*
* When dataset contents are created, they are delivered to destinations specified here.
*
*/
private java.util.List contentDeliveryRules;
/**
*
* Optional. How long, in days, versions of dataset contents are kept for the dataset. If not specified or set to
* null
, versions of dataset contents are retained for at most 90 days. The number of versions of
* dataset contents retained is determined by the versioningConfiguration
parameter. For more
* information, see Keeping Multiple Versions of IoT Analytics datasets in the IoT Analytics User Guide.
*
*/
private RetentionPeriod retentionPeriod;
/**
*
* Optional. How many versions of dataset contents are kept. If not specified or set to null, only the latest
* version plus the latest succeeded version (if they are different) are kept for the time period specified by the
* retentionPeriod
parameter. For more information, see Keeping Multiple Versions of IoT Analytics datasets in the IoT Analytics User Guide.
*
*/
private VersioningConfiguration versioningConfiguration;
/**
*
* Metadata which can be used to manage the dataset.
*
*/
private java.util.List tags;
/**
*
* A list of data rules that send notifications to CloudWatch, when data arrives late. To specify
* lateDataRules
, the dataset must use a DeltaTimer filter.
*
*/
private java.util.List lateDataRules;
/**
*
* The name of the dataset.
*
*
* @param datasetName
* The name of the dataset.
*/
public void setDatasetName(String datasetName) {
this.datasetName = datasetName;
}
/**
*
* The name of the dataset.
*
*
* @return The name of the dataset.
*/
public String getDatasetName() {
return this.datasetName;
}
/**
*
* The name of the dataset.
*
*
* @param datasetName
* The name of the dataset.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateDatasetRequest withDatasetName(String datasetName) {
setDatasetName(datasetName);
return this;
}
/**
*
* A list of actions that create the dataset contents.
*
*
* @return A list of actions that create the dataset contents.
*/
public java.util.List getActions() {
return actions;
}
/**
*
* A list of actions that create the dataset contents.
*
*
* @param actions
* A list of actions that create the dataset contents.
*/
public void setActions(java.util.Collection actions) {
if (actions == null) {
this.actions = null;
return;
}
this.actions = new java.util.ArrayList(actions);
}
/**
*
* A list of actions that create the dataset contents.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setActions(java.util.Collection)} or {@link #withActions(java.util.Collection)} if you want to override
* the existing values.
*
*
* @param actions
* A list of actions that create the dataset contents.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateDatasetRequest withActions(DatasetAction... actions) {
if (this.actions == null) {
setActions(new java.util.ArrayList(actions.length));
}
for (DatasetAction ele : actions) {
this.actions.add(ele);
}
return this;
}
/**
*
* A list of actions that create the dataset contents.
*
*
* @param actions
* A list of actions that create the dataset contents.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateDatasetRequest withActions(java.util.Collection actions) {
setActions(actions);
return this;
}
/**
*
* A list of triggers. A trigger causes dataset contents to be populated at a specified time interval or when
* another dataset's contents are created. The list of triggers can be empty or contain up to five
* DataSetTrigger
objects.
*
*
* @return A list of triggers. A trigger causes dataset contents to be populated at a specified time interval or
* when another dataset's contents are created. The list of triggers can be empty or contain up to five
* DataSetTrigger
objects.
*/
public java.util.List getTriggers() {
return triggers;
}
/**
*
* A list of triggers. A trigger causes dataset contents to be populated at a specified time interval or when
* another dataset's contents are created. The list of triggers can be empty or contain up to five
* DataSetTrigger
objects.
*
*
* @param triggers
* A list of triggers. A trigger causes dataset contents to be populated at a specified time interval or when
* another dataset's contents are created. The list of triggers can be empty or contain up to five
* DataSetTrigger
objects.
*/
public void setTriggers(java.util.Collection triggers) {
if (triggers == null) {
this.triggers = null;
return;
}
this.triggers = new java.util.ArrayList(triggers);
}
/**
*
* A list of triggers. A trigger causes dataset contents to be populated at a specified time interval or when
* another dataset's contents are created. The list of triggers can be empty or contain up to five
* DataSetTrigger
objects.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setTriggers(java.util.Collection)} or {@link #withTriggers(java.util.Collection)} if you want to override
* the existing values.
*
*
* @param triggers
* A list of triggers. A trigger causes dataset contents to be populated at a specified time interval or when
* another dataset's contents are created. The list of triggers can be empty or contain up to five
* DataSetTrigger
objects.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateDatasetRequest withTriggers(DatasetTrigger... triggers) {
if (this.triggers == null) {
setTriggers(new java.util.ArrayList(triggers.length));
}
for (DatasetTrigger ele : triggers) {
this.triggers.add(ele);
}
return this;
}
/**
*
* A list of triggers. A trigger causes dataset contents to be populated at a specified time interval or when
* another dataset's contents are created. The list of triggers can be empty or contain up to five
* DataSetTrigger
objects.
*
*
* @param triggers
* A list of triggers. A trigger causes dataset contents to be populated at a specified time interval or when
* another dataset's contents are created. The list of triggers can be empty or contain up to five
* DataSetTrigger
objects.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateDatasetRequest withTriggers(java.util.Collection triggers) {
setTriggers(triggers);
return this;
}
/**
*
* When dataset contents are created, they are delivered to destinations specified here.
*
*
* @return When dataset contents are created, they are delivered to destinations specified here.
*/
public java.util.List getContentDeliveryRules() {
return contentDeliveryRules;
}
/**
*
* When dataset contents are created, they are delivered to destinations specified here.
*
*
* @param contentDeliveryRules
* When dataset contents are created, they are delivered to destinations specified here.
*/
public void setContentDeliveryRules(java.util.Collection contentDeliveryRules) {
if (contentDeliveryRules == null) {
this.contentDeliveryRules = null;
return;
}
this.contentDeliveryRules = new java.util.ArrayList(contentDeliveryRules);
}
/**
*
* When dataset contents are created, they are delivered to destinations specified here.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setContentDeliveryRules(java.util.Collection)} or {@link #withContentDeliveryRules(java.util.Collection)}
* if you want to override the existing values.
*
*
* @param contentDeliveryRules
* When dataset contents are created, they are delivered to destinations specified here.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateDatasetRequest withContentDeliveryRules(DatasetContentDeliveryRule... contentDeliveryRules) {
if (this.contentDeliveryRules == null) {
setContentDeliveryRules(new java.util.ArrayList(contentDeliveryRules.length));
}
for (DatasetContentDeliveryRule ele : contentDeliveryRules) {
this.contentDeliveryRules.add(ele);
}
return this;
}
/**
*
* When dataset contents are created, they are delivered to destinations specified here.
*
*
* @param contentDeliveryRules
* When dataset contents are created, they are delivered to destinations specified here.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateDatasetRequest withContentDeliveryRules(java.util.Collection contentDeliveryRules) {
setContentDeliveryRules(contentDeliveryRules);
return this;
}
/**
*
* Optional. How long, in days, versions of dataset contents are kept for the dataset. If not specified or set to
* null
, versions of dataset contents are retained for at most 90 days. The number of versions of
* dataset contents retained is determined by the versioningConfiguration
parameter. For more
* information, see Keeping Multiple Versions of IoT Analytics datasets in the IoT Analytics User Guide.
*
*
* @param retentionPeriod
* Optional. How long, in days, versions of dataset contents are kept for the dataset. If not specified or
* set to null
, versions of dataset contents are retained for at most 90 days. The number of
* versions of dataset contents retained is determined by the versioningConfiguration
parameter.
* For more information, see Keeping Multiple Versions of IoT Analytics datasets in the IoT Analytics User Guide.
*/
public void setRetentionPeriod(RetentionPeriod retentionPeriod) {
this.retentionPeriod = retentionPeriod;
}
/**
*
* Optional. How long, in days, versions of dataset contents are kept for the dataset. If not specified or set to
* null
, versions of dataset contents are retained for at most 90 days. The number of versions of
* dataset contents retained is determined by the versioningConfiguration
parameter. For more
* information, see Keeping Multiple Versions of IoT Analytics datasets in the IoT Analytics User Guide.
*
*
* @return Optional. How long, in days, versions of dataset contents are kept for the dataset. If not specified or
* set to null
, versions of dataset contents are retained for at most 90 days. The number of
* versions of dataset contents retained is determined by the versioningConfiguration
* parameter. For more information, see Keeping Multiple Versions of IoT Analytics datasets in the IoT Analytics User Guide.
*/
public RetentionPeriod getRetentionPeriod() {
return this.retentionPeriod;
}
/**
*
* Optional. How long, in days, versions of dataset contents are kept for the dataset. If not specified or set to
* null
, versions of dataset contents are retained for at most 90 days. The number of versions of
* dataset contents retained is determined by the versioningConfiguration
parameter. For more
* information, see Keeping Multiple Versions of IoT Analytics datasets in the IoT Analytics User Guide.
*
*
* @param retentionPeriod
* Optional. How long, in days, versions of dataset contents are kept for the dataset. If not specified or
* set to null
, versions of dataset contents are retained for at most 90 days. The number of
* versions of dataset contents retained is determined by the versioningConfiguration
parameter.
* For more information, see Keeping Multiple Versions of IoT Analytics datasets in the IoT Analytics User Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateDatasetRequest withRetentionPeriod(RetentionPeriod retentionPeriod) {
setRetentionPeriod(retentionPeriod);
return this;
}
/**
*
* Optional. How many versions of dataset contents are kept. If not specified or set to null, only the latest
* version plus the latest succeeded version (if they are different) are kept for the time period specified by the
* retentionPeriod
parameter. For more information, see Keeping Multiple Versions of IoT Analytics datasets in the IoT Analytics User Guide.
*
*
* @param versioningConfiguration
* Optional. How many versions of dataset contents are kept. If not specified or set to null, only the latest
* version plus the latest succeeded version (if they are different) are kept for the time period specified
* by the retentionPeriod
parameter. For more information, see Keeping Multiple Versions of IoT Analytics datasets in the IoT Analytics User Guide.
*/
public void setVersioningConfiguration(VersioningConfiguration versioningConfiguration) {
this.versioningConfiguration = versioningConfiguration;
}
/**
*
* Optional. How many versions of dataset contents are kept. If not specified or set to null, only the latest
* version plus the latest succeeded version (if they are different) are kept for the time period specified by the
* retentionPeriod
parameter. For more information, see Keeping Multiple Versions of IoT Analytics datasets in the IoT Analytics User Guide.
*
*
* @return Optional. How many versions of dataset contents are kept. If not specified or set to null, only the
* latest version plus the latest succeeded version (if they are different) are kept for the time period
* specified by the retentionPeriod
parameter. For more information, see Keeping Multiple Versions of IoT Analytics datasets in the IoT Analytics User Guide.
*/
public VersioningConfiguration getVersioningConfiguration() {
return this.versioningConfiguration;
}
/**
*
* Optional. How many versions of dataset contents are kept. If not specified or set to null, only the latest
* version plus the latest succeeded version (if they are different) are kept for the time period specified by the
* retentionPeriod
parameter. For more information, see Keeping Multiple Versions of IoT Analytics datasets in the IoT Analytics User Guide.
*
*
* @param versioningConfiguration
* Optional. How many versions of dataset contents are kept. If not specified or set to null, only the latest
* version plus the latest succeeded version (if they are different) are kept for the time period specified
* by the retentionPeriod
parameter. For more information, see Keeping Multiple Versions of IoT Analytics datasets in the IoT Analytics User Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateDatasetRequest withVersioningConfiguration(VersioningConfiguration versioningConfiguration) {
setVersioningConfiguration(versioningConfiguration);
return this;
}
/**
*
* Metadata which can be used to manage the dataset.
*
*
* @return Metadata which can be used to manage the dataset.
*/
public java.util.List getTags() {
return tags;
}
/**
*
* Metadata which can be used to manage the dataset.
*
*
* @param tags
* Metadata which can be used to manage the dataset.
*/
public void setTags(java.util.Collection tags) {
if (tags == null) {
this.tags = null;
return;
}
this.tags = new java.util.ArrayList(tags);
}
/**
*
* Metadata which can be used to manage the dataset.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setTags(java.util.Collection)} or {@link #withTags(java.util.Collection)} if you want to override the
* existing values.
*
*
* @param tags
* Metadata which can be used to manage the dataset.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateDatasetRequest withTags(Tag... tags) {
if (this.tags == null) {
setTags(new java.util.ArrayList(tags.length));
}
for (Tag ele : tags) {
this.tags.add(ele);
}
return this;
}
/**
*
* Metadata which can be used to manage the dataset.
*
*
* @param tags
* Metadata which can be used to manage the dataset.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateDatasetRequest withTags(java.util.Collection tags) {
setTags(tags);
return this;
}
/**
*
* A list of data rules that send notifications to CloudWatch, when data arrives late. To specify
* lateDataRules
, the dataset must use a DeltaTimer filter.
*
*
* @return A list of data rules that send notifications to CloudWatch, when data arrives late. To specify
* lateDataRules
, the dataset must use a DeltaTimer
* filter.
*/
public java.util.List getLateDataRules() {
return lateDataRules;
}
/**
*
* A list of data rules that send notifications to CloudWatch, when data arrives late. To specify
* lateDataRules
, the dataset must use a DeltaTimer filter.
*
*
* @param lateDataRules
* A list of data rules that send notifications to CloudWatch, when data arrives late. To specify
* lateDataRules
, the dataset must use a DeltaTimer
* filter.
*/
public void setLateDataRules(java.util.Collection lateDataRules) {
if (lateDataRules == null) {
this.lateDataRules = null;
return;
}
this.lateDataRules = new java.util.ArrayList(lateDataRules);
}
/**
*
* A list of data rules that send notifications to CloudWatch, when data arrives late. To specify
* lateDataRules
, the dataset must use a DeltaTimer filter.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setLateDataRules(java.util.Collection)} or {@link #withLateDataRules(java.util.Collection)} if you want
* to override the existing values.
*
*
* @param lateDataRules
* A list of data rules that send notifications to CloudWatch, when data arrives late. To specify
* lateDataRules
, the dataset must use a DeltaTimer
* filter.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateDatasetRequest withLateDataRules(LateDataRule... lateDataRules) {
if (this.lateDataRules == null) {
setLateDataRules(new java.util.ArrayList(lateDataRules.length));
}
for (LateDataRule ele : lateDataRules) {
this.lateDataRules.add(ele);
}
return this;
}
/**
*
* A list of data rules that send notifications to CloudWatch, when data arrives late. To specify
* lateDataRules
, the dataset must use a DeltaTimer filter.
*
*
* @param lateDataRules
* A list of data rules that send notifications to CloudWatch, when data arrives late. To specify
* lateDataRules
, the dataset must use a DeltaTimer
* filter.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateDatasetRequest withLateDataRules(java.util.Collection lateDataRules) {
setLateDataRules(lateDataRules);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getDatasetName() != null)
sb.append("DatasetName: ").append(getDatasetName()).append(",");
if (getActions() != null)
sb.append("Actions: ").append(getActions()).append(",");
if (getTriggers() != null)
sb.append("Triggers: ").append(getTriggers()).append(",");
if (getContentDeliveryRules() != null)
sb.append("ContentDeliveryRules: ").append(getContentDeliveryRules()).append(",");
if (getRetentionPeriod() != null)
sb.append("RetentionPeriod: ").append(getRetentionPeriod()).append(",");
if (getVersioningConfiguration() != null)
sb.append("VersioningConfiguration: ").append(getVersioningConfiguration()).append(",");
if (getTags() != null)
sb.append("Tags: ").append(getTags()).append(",");
if (getLateDataRules() != null)
sb.append("LateDataRules: ").append(getLateDataRules());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof CreateDatasetRequest == false)
return false;
CreateDatasetRequest other = (CreateDatasetRequest) obj;
if (other.getDatasetName() == null ^ this.getDatasetName() == null)
return false;
if (other.getDatasetName() != null && other.getDatasetName().equals(this.getDatasetName()) == false)
return false;
if (other.getActions() == null ^ this.getActions() == null)
return false;
if (other.getActions() != null && other.getActions().equals(this.getActions()) == false)
return false;
if (other.getTriggers() == null ^ this.getTriggers() == null)
return false;
if (other.getTriggers() != null && other.getTriggers().equals(this.getTriggers()) == false)
return false;
if (other.getContentDeliveryRules() == null ^ this.getContentDeliveryRules() == null)
return false;
if (other.getContentDeliveryRules() != null && other.getContentDeliveryRules().equals(this.getContentDeliveryRules()) == false)
return false;
if (other.getRetentionPeriod() == null ^ this.getRetentionPeriod() == null)
return false;
if (other.getRetentionPeriod() != null && other.getRetentionPeriod().equals(this.getRetentionPeriod()) == false)
return false;
if (other.getVersioningConfiguration() == null ^ this.getVersioningConfiguration() == null)
return false;
if (other.getVersioningConfiguration() != null && other.getVersioningConfiguration().equals(this.getVersioningConfiguration()) == false)
return false;
if (other.getTags() == null ^ this.getTags() == null)
return false;
if (other.getTags() != null && other.getTags().equals(this.getTags()) == false)
return false;
if (other.getLateDataRules() == null ^ this.getLateDataRules() == null)
return false;
if (other.getLateDataRules() != null && other.getLateDataRules().equals(this.getLateDataRules()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getDatasetName() == null) ? 0 : getDatasetName().hashCode());
hashCode = prime * hashCode + ((getActions() == null) ? 0 : getActions().hashCode());
hashCode = prime * hashCode + ((getTriggers() == null) ? 0 : getTriggers().hashCode());
hashCode = prime * hashCode + ((getContentDeliveryRules() == null) ? 0 : getContentDeliveryRules().hashCode());
hashCode = prime * hashCode + ((getRetentionPeriod() == null) ? 0 : getRetentionPeriod().hashCode());
hashCode = prime * hashCode + ((getVersioningConfiguration() == null) ? 0 : getVersioningConfiguration().hashCode());
hashCode = prime * hashCode + ((getTags() == null) ? 0 : getTags().hashCode());
hashCode = prime * hashCode + ((getLateDataRules() == null) ? 0 : getLateDataRules().hashCode());
return hashCode;
}
@Override
public CreateDatasetRequest clone() {
return (CreateDatasetRequest) super.clone();
}
}