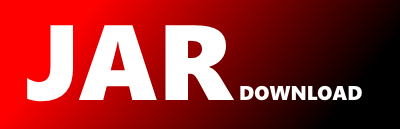
com.amazonaws.services.iotdeviceadvisor.AWSIoTDeviceAdvisorAsync Maven / Gradle / Ivy
Show all versions of aws-java-sdk-iotdeviceadvisor Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.iotdeviceadvisor;
import javax.annotation.Generated;
import com.amazonaws.services.iotdeviceadvisor.model.*;
/**
* Interface for accessing AWSIoTDeviceAdvisor asynchronously. Each asynchronous method will return a Java Future object
* representing the asynchronous operation; overloads which accept an {@code AsyncHandler} can be used to receive
* notification when an asynchronous operation completes.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.iotdeviceadvisor.AbstractAWSIoTDeviceAdvisorAsync} instead.
*
*
*
* Amazon Web Services IoT Core Device Advisor is a cloud-based, fully managed test capability for validating IoT
* devices during device software development. Device Advisor provides pre-built tests that you can use to validate IoT
* devices for reliable and secure connectivity with Amazon Web Services IoT Core before deploying devices to
* production. By using Device Advisor, you can confirm that your devices can connect to Amazon Web Services IoT Core,
* follow security best practices and, if applicable, receive software updates from IoT Device Management. You can also
* download signed qualification reports to submit to the Amazon Web Services Partner Network to get your device
* qualified for the Amazon Web Services Partner Device Catalog without the need to send your device in and wait for it
* to be tested.
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AWSIoTDeviceAdvisorAsync extends AWSIoTDeviceAdvisor {
/**
*
* Creates a Device Advisor test suite.
*
*
* Requires permission to access the CreateSuiteDefinition action.
*
*
* @param createSuiteDefinitionRequest
* @return A Java Future containing the result of the CreateSuiteDefinition operation returned by the service.
* @sample AWSIoTDeviceAdvisorAsync.CreateSuiteDefinition
* @see AWS API Documentation
*/
java.util.concurrent.Future createSuiteDefinitionAsync(CreateSuiteDefinitionRequest createSuiteDefinitionRequest);
/**
*
* Creates a Device Advisor test suite.
*
*
* Requires permission to access the CreateSuiteDefinition action.
*
*
* @param createSuiteDefinitionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateSuiteDefinition operation returned by the service.
* @sample AWSIoTDeviceAdvisorAsyncHandler.CreateSuiteDefinition
* @see AWS API Documentation
*/
java.util.concurrent.Future createSuiteDefinitionAsync(CreateSuiteDefinitionRequest createSuiteDefinitionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a Device Advisor test suite.
*
*
* Requires permission to access the DeleteSuiteDefinition action.
*
*
* @param deleteSuiteDefinitionRequest
* @return A Java Future containing the result of the DeleteSuiteDefinition operation returned by the service.
* @sample AWSIoTDeviceAdvisorAsync.DeleteSuiteDefinition
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteSuiteDefinitionAsync(DeleteSuiteDefinitionRequest deleteSuiteDefinitionRequest);
/**
*
* Deletes a Device Advisor test suite.
*
*
* Requires permission to access the DeleteSuiteDefinition action.
*
*
* @param deleteSuiteDefinitionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteSuiteDefinition operation returned by the service.
* @sample AWSIoTDeviceAdvisorAsyncHandler.DeleteSuiteDefinition
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteSuiteDefinitionAsync(DeleteSuiteDefinitionRequest deleteSuiteDefinitionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets information about an Device Advisor endpoint.
*
*
* @param getEndpointRequest
* @return A Java Future containing the result of the GetEndpoint operation returned by the service.
* @sample AWSIoTDeviceAdvisorAsync.GetEndpoint
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getEndpointAsync(GetEndpointRequest getEndpointRequest);
/**
*
* Gets information about an Device Advisor endpoint.
*
*
* @param getEndpointRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetEndpoint operation returned by the service.
* @sample AWSIoTDeviceAdvisorAsyncHandler.GetEndpoint
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getEndpointAsync(GetEndpointRequest getEndpointRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets information about a Device Advisor test suite.
*
*
* Requires permission to access the GetSuiteDefinition action.
*
*
* @param getSuiteDefinitionRequest
* @return A Java Future containing the result of the GetSuiteDefinition operation returned by the service.
* @sample AWSIoTDeviceAdvisorAsync.GetSuiteDefinition
* @see AWS API Documentation
*/
java.util.concurrent.Future getSuiteDefinitionAsync(GetSuiteDefinitionRequest getSuiteDefinitionRequest);
/**
*
* Gets information about a Device Advisor test suite.
*
*
* Requires permission to access the GetSuiteDefinition action.
*
*
* @param getSuiteDefinitionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetSuiteDefinition operation returned by the service.
* @sample AWSIoTDeviceAdvisorAsyncHandler.GetSuiteDefinition
* @see AWS API Documentation
*/
java.util.concurrent.Future getSuiteDefinitionAsync(GetSuiteDefinitionRequest getSuiteDefinitionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets information about a Device Advisor test suite run.
*
*
* Requires permission to access the GetSuiteRun action.
*
*
* @param getSuiteRunRequest
* @return A Java Future containing the result of the GetSuiteRun operation returned by the service.
* @sample AWSIoTDeviceAdvisorAsync.GetSuiteRun
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getSuiteRunAsync(GetSuiteRunRequest getSuiteRunRequest);
/**
*
* Gets information about a Device Advisor test suite run.
*
*
* Requires permission to access the GetSuiteRun action.
*
*
* @param getSuiteRunRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetSuiteRun operation returned by the service.
* @sample AWSIoTDeviceAdvisorAsyncHandler.GetSuiteRun
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getSuiteRunAsync(GetSuiteRunRequest getSuiteRunRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets a report download link for a successful Device Advisor qualifying test suite run.
*
*
* Requires permission to access the GetSuiteRunReport action.
*
*
* @param getSuiteRunReportRequest
* @return A Java Future containing the result of the GetSuiteRunReport operation returned by the service.
* @sample AWSIoTDeviceAdvisorAsync.GetSuiteRunReport
* @see AWS API Documentation
*/
java.util.concurrent.Future getSuiteRunReportAsync(GetSuiteRunReportRequest getSuiteRunReportRequest);
/**
*
* Gets a report download link for a successful Device Advisor qualifying test suite run.
*
*
* Requires permission to access the GetSuiteRunReport action.
*
*
* @param getSuiteRunReportRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetSuiteRunReport operation returned by the service.
* @sample AWSIoTDeviceAdvisorAsyncHandler.GetSuiteRunReport
* @see AWS API Documentation
*/
java.util.concurrent.Future getSuiteRunReportAsync(GetSuiteRunReportRequest getSuiteRunReportRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists the Device Advisor test suites you have created.
*
*
* Requires permission to access the ListSuiteDefinitions action.
*
*
* @param listSuiteDefinitionsRequest
* @return A Java Future containing the result of the ListSuiteDefinitions operation returned by the service.
* @sample AWSIoTDeviceAdvisorAsync.ListSuiteDefinitions
* @see AWS API Documentation
*/
java.util.concurrent.Future listSuiteDefinitionsAsync(ListSuiteDefinitionsRequest listSuiteDefinitionsRequest);
/**
*
* Lists the Device Advisor test suites you have created.
*
*
* Requires permission to access the ListSuiteDefinitions action.
*
*
* @param listSuiteDefinitionsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListSuiteDefinitions operation returned by the service.
* @sample AWSIoTDeviceAdvisorAsyncHandler.ListSuiteDefinitions
* @see AWS API Documentation
*/
java.util.concurrent.Future listSuiteDefinitionsAsync(ListSuiteDefinitionsRequest listSuiteDefinitionsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists runs of the specified Device Advisor test suite. You can list all runs of the test suite, or the runs of a
* specific version of the test suite.
*
*
* Requires permission to access the ListSuiteRuns action.
*
*
* @param listSuiteRunsRequest
* @return A Java Future containing the result of the ListSuiteRuns operation returned by the service.
* @sample AWSIoTDeviceAdvisorAsync.ListSuiteRuns
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listSuiteRunsAsync(ListSuiteRunsRequest listSuiteRunsRequest);
/**
*
* Lists runs of the specified Device Advisor test suite. You can list all runs of the test suite, or the runs of a
* specific version of the test suite.
*
*
* Requires permission to access the ListSuiteRuns action.
*
*
* @param listSuiteRunsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListSuiteRuns operation returned by the service.
* @sample AWSIoTDeviceAdvisorAsyncHandler.ListSuiteRuns
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listSuiteRunsAsync(ListSuiteRunsRequest listSuiteRunsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists the tags attached to an IoT Device Advisor resource.
*
*
* Requires permission to access the ListTagsForResource action.
*
*
* @param listTagsForResourceRequest
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* @sample AWSIoTDeviceAdvisorAsync.ListTagsForResource
* @see AWS API Documentation
*/
java.util.concurrent.Future listTagsForResourceAsync(ListTagsForResourceRequest listTagsForResourceRequest);
/**
*
* Lists the tags attached to an IoT Device Advisor resource.
*
*
* Requires permission to access the ListTagsForResource action.
*
*
* @param listTagsForResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* @sample AWSIoTDeviceAdvisorAsyncHandler.ListTagsForResource
* @see AWS API Documentation
*/
java.util.concurrent.Future listTagsForResourceAsync(ListTagsForResourceRequest listTagsForResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Starts a Device Advisor test suite run.
*
*
* Requires permission to access the StartSuiteRun action.
*
*
* @param startSuiteRunRequest
* @return A Java Future containing the result of the StartSuiteRun operation returned by the service.
* @sample AWSIoTDeviceAdvisorAsync.StartSuiteRun
* @see AWS
* API Documentation
*/
java.util.concurrent.Future startSuiteRunAsync(StartSuiteRunRequest startSuiteRunRequest);
/**
*
* Starts a Device Advisor test suite run.
*
*
* Requires permission to access the StartSuiteRun action.
*
*
* @param startSuiteRunRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the StartSuiteRun operation returned by the service.
* @sample AWSIoTDeviceAdvisorAsyncHandler.StartSuiteRun
* @see AWS
* API Documentation
*/
java.util.concurrent.Future startSuiteRunAsync(StartSuiteRunRequest startSuiteRunRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Stops a Device Advisor test suite run that is currently running.
*
*
* Requires permission to access the StopSuiteRun action.
*
*
* @param stopSuiteRunRequest
* @return A Java Future containing the result of the StopSuiteRun operation returned by the service.
* @sample AWSIoTDeviceAdvisorAsync.StopSuiteRun
* @see AWS
* API Documentation
*/
java.util.concurrent.Future stopSuiteRunAsync(StopSuiteRunRequest stopSuiteRunRequest);
/**
*
* Stops a Device Advisor test suite run that is currently running.
*
*
* Requires permission to access the StopSuiteRun action.
*
*
* @param stopSuiteRunRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the StopSuiteRun operation returned by the service.
* @sample AWSIoTDeviceAdvisorAsyncHandler.StopSuiteRun
* @see AWS
* API Documentation
*/
java.util.concurrent.Future stopSuiteRunAsync(StopSuiteRunRequest stopSuiteRunRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Adds to and modifies existing tags of an IoT Device Advisor resource.
*
*
* Requires permission to access the TagResource action.
*
*
* @param tagResourceRequest
* @return A Java Future containing the result of the TagResource operation returned by the service.
* @sample AWSIoTDeviceAdvisorAsync.TagResource
* @see AWS
* API Documentation
*/
java.util.concurrent.Future tagResourceAsync(TagResourceRequest tagResourceRequest);
/**
*
* Adds to and modifies existing tags of an IoT Device Advisor resource.
*
*
* Requires permission to access the TagResource action.
*
*
* @param tagResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the TagResource operation returned by the service.
* @sample AWSIoTDeviceAdvisorAsyncHandler.TagResource
* @see AWS
* API Documentation
*/
java.util.concurrent.Future tagResourceAsync(TagResourceRequest tagResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Removes tags from an IoT Device Advisor resource.
*
*
* Requires permission to access the UntagResource action.
*
*
* @param untagResourceRequest
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* @sample AWSIoTDeviceAdvisorAsync.UntagResource
* @see AWS
* API Documentation
*/
java.util.concurrent.Future untagResourceAsync(UntagResourceRequest untagResourceRequest);
/**
*
* Removes tags from an IoT Device Advisor resource.
*
*
* Requires permission to access the UntagResource action.
*
*
* @param untagResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* @sample AWSIoTDeviceAdvisorAsyncHandler.UntagResource
* @see AWS
* API Documentation
*/
java.util.concurrent.Future untagResourceAsync(UntagResourceRequest untagResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates a Device Advisor test suite.
*
*
* Requires permission to access the UpdateSuiteDefinition action.
*
*
* @param updateSuiteDefinitionRequest
* @return A Java Future containing the result of the UpdateSuiteDefinition operation returned by the service.
* @sample AWSIoTDeviceAdvisorAsync.UpdateSuiteDefinition
* @see AWS API Documentation
*/
java.util.concurrent.Future updateSuiteDefinitionAsync(UpdateSuiteDefinitionRequest updateSuiteDefinitionRequest);
/**
*
* Updates a Device Advisor test suite.
*
*
* Requires permission to access the UpdateSuiteDefinition action.
*
*
* @param updateSuiteDefinitionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateSuiteDefinition operation returned by the service.
* @sample AWSIoTDeviceAdvisorAsyncHandler.UpdateSuiteDefinition
* @see AWS API Documentation
*/
java.util.concurrent.Future updateSuiteDefinitionAsync(UpdateSuiteDefinitionRequest updateSuiteDefinitionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
}