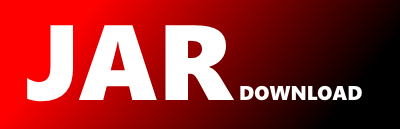
com.amazonaws.services.iotdeviceadvisor.AWSIoTDeviceAdvisor Maven / Gradle / Ivy
Show all versions of aws-java-sdk-iotdeviceadvisor Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.iotdeviceadvisor;
import javax.annotation.Generated;
import com.amazonaws.*;
import com.amazonaws.regions.*;
import com.amazonaws.services.iotdeviceadvisor.model.*;
/**
* Interface for accessing AWSIoTDeviceAdvisor.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.iotdeviceadvisor.AbstractAWSIoTDeviceAdvisor} instead.
*
*
*
* Amazon Web Services IoT Core Device Advisor is a cloud-based, fully managed test capability for validating IoT
* devices during device software development. Device Advisor provides pre-built tests that you can use to validate IoT
* devices for reliable and secure connectivity with Amazon Web Services IoT Core before deploying devices to
* production. By using Device Advisor, you can confirm that your devices can connect to Amazon Web Services IoT Core,
* follow security best practices and, if applicable, receive software updates from IoT Device Management. You can also
* download signed qualification reports to submit to the Amazon Web Services Partner Network to get your device
* qualified for the Amazon Web Services Partner Device Catalog without the need to send your device in and wait for it
* to be tested.
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AWSIoTDeviceAdvisor {
/**
* The region metadata service name for computing region endpoints. You can use this value to retrieve metadata
* (such as supported regions) of the service.
*
* @see RegionUtils#getRegionsForService(String)
*/
String ENDPOINT_PREFIX = "api.iotdeviceadvisor";
/**
*
* Creates a Device Advisor test suite.
*
*
* Requires permission to access the CreateSuiteDefinition action.
*
*
* @param createSuiteDefinitionRequest
* @return Result of the CreateSuiteDefinition operation returned by the service.
* @throws ValidationException
* Sends a validation exception.
* @throws InternalServerException
* Sends an Internal Failure exception.
* @sample AWSIoTDeviceAdvisor.CreateSuiteDefinition
* @see AWS API Documentation
*/
CreateSuiteDefinitionResult createSuiteDefinition(CreateSuiteDefinitionRequest createSuiteDefinitionRequest);
/**
*
* Deletes a Device Advisor test suite.
*
*
* Requires permission to access the DeleteSuiteDefinition action.
*
*
* @param deleteSuiteDefinitionRequest
* @return Result of the DeleteSuiteDefinition operation returned by the service.
* @throws ValidationException
* Sends a validation exception.
* @throws InternalServerException
* Sends an Internal Failure exception.
* @sample AWSIoTDeviceAdvisor.DeleteSuiteDefinition
* @see AWS API Documentation
*/
DeleteSuiteDefinitionResult deleteSuiteDefinition(DeleteSuiteDefinitionRequest deleteSuiteDefinitionRequest);
/**
*
* Gets information about an Device Advisor endpoint.
*
*
* @param getEndpointRequest
* @return Result of the GetEndpoint operation returned by the service.
* @throws ValidationException
* Sends a validation exception.
* @throws InternalServerException
* Sends an Internal Failure exception.
* @throws ResourceNotFoundException
* Sends a Resource Not Found exception.
* @sample AWSIoTDeviceAdvisor.GetEndpoint
* @see AWS
* API Documentation
*/
GetEndpointResult getEndpoint(GetEndpointRequest getEndpointRequest);
/**
*
* Gets information about a Device Advisor test suite.
*
*
* Requires permission to access the GetSuiteDefinition action.
*
*
* @param getSuiteDefinitionRequest
* @return Result of the GetSuiteDefinition operation returned by the service.
* @throws ValidationException
* Sends a validation exception.
* @throws InternalServerException
* Sends an Internal Failure exception.
* @throws ResourceNotFoundException
* Sends a Resource Not Found exception.
* @sample AWSIoTDeviceAdvisor.GetSuiteDefinition
* @see AWS API Documentation
*/
GetSuiteDefinitionResult getSuiteDefinition(GetSuiteDefinitionRequest getSuiteDefinitionRequest);
/**
*
* Gets information about a Device Advisor test suite run.
*
*
* Requires permission to access the GetSuiteRun action.
*
*
* @param getSuiteRunRequest
* @return Result of the GetSuiteRun operation returned by the service.
* @throws ValidationException
* Sends a validation exception.
* @throws InternalServerException
* Sends an Internal Failure exception.
* @throws ResourceNotFoundException
* Sends a Resource Not Found exception.
* @sample AWSIoTDeviceAdvisor.GetSuiteRun
* @see AWS
* API Documentation
*/
GetSuiteRunResult getSuiteRun(GetSuiteRunRequest getSuiteRunRequest);
/**
*
* Gets a report download link for a successful Device Advisor qualifying test suite run.
*
*
* Requires permission to access the GetSuiteRunReport action.
*
*
* @param getSuiteRunReportRequest
* @return Result of the GetSuiteRunReport operation returned by the service.
* @throws ValidationException
* Sends a validation exception.
* @throws InternalServerException
* Sends an Internal Failure exception.
* @throws ResourceNotFoundException
* Sends a Resource Not Found exception.
* @sample AWSIoTDeviceAdvisor.GetSuiteRunReport
* @see AWS API Documentation
*/
GetSuiteRunReportResult getSuiteRunReport(GetSuiteRunReportRequest getSuiteRunReportRequest);
/**
*
* Lists the Device Advisor test suites you have created.
*
*
* Requires permission to access the ListSuiteDefinitions action.
*
*
* @param listSuiteDefinitionsRequest
* @return Result of the ListSuiteDefinitions operation returned by the service.
* @throws ValidationException
* Sends a validation exception.
* @throws InternalServerException
* Sends an Internal Failure exception.
* @sample AWSIoTDeviceAdvisor.ListSuiteDefinitions
* @see AWS API Documentation
*/
ListSuiteDefinitionsResult listSuiteDefinitions(ListSuiteDefinitionsRequest listSuiteDefinitionsRequest);
/**
*
* Lists runs of the specified Device Advisor test suite. You can list all runs of the test suite, or the runs of a
* specific version of the test suite.
*
*
* Requires permission to access the ListSuiteRuns action.
*
*
* @param listSuiteRunsRequest
* @return Result of the ListSuiteRuns operation returned by the service.
* @throws ValidationException
* Sends a validation exception.
* @throws InternalServerException
* Sends an Internal Failure exception.
* @sample AWSIoTDeviceAdvisor.ListSuiteRuns
* @see AWS
* API Documentation
*/
ListSuiteRunsResult listSuiteRuns(ListSuiteRunsRequest listSuiteRunsRequest);
/**
*
* Lists the tags attached to an IoT Device Advisor resource.
*
*
* Requires permission to access the ListTagsForResource action.
*
*
* @param listTagsForResourceRequest
* @return Result of the ListTagsForResource operation returned by the service.
* @throws InternalServerException
* Sends an Internal Failure exception.
* @throws ValidationException
* Sends a validation exception.
* @throws ResourceNotFoundException
* Sends a Resource Not Found exception.
* @sample AWSIoTDeviceAdvisor.ListTagsForResource
* @see AWS API Documentation
*/
ListTagsForResourceResult listTagsForResource(ListTagsForResourceRequest listTagsForResourceRequest);
/**
*
* Starts a Device Advisor test suite run.
*
*
* Requires permission to access the StartSuiteRun action.
*
*
* @param startSuiteRunRequest
* @return Result of the StartSuiteRun operation returned by the service.
* @throws ValidationException
* Sends a validation exception.
* @throws InternalServerException
* Sends an Internal Failure exception.
* @throws ConflictException
* Sends a Conflict Exception.
* @sample AWSIoTDeviceAdvisor.StartSuiteRun
* @see AWS
* API Documentation
*/
StartSuiteRunResult startSuiteRun(StartSuiteRunRequest startSuiteRunRequest);
/**
*
* Stops a Device Advisor test suite run that is currently running.
*
*
* Requires permission to access the StopSuiteRun action.
*
*
* @param stopSuiteRunRequest
* @return Result of the StopSuiteRun operation returned by the service.
* @throws ValidationException
* Sends a validation exception.
* @throws ResourceNotFoundException
* Sends a Resource Not Found exception.
* @throws InternalServerException
* Sends an Internal Failure exception.
* @sample AWSIoTDeviceAdvisor.StopSuiteRun
* @see AWS
* API Documentation
*/
StopSuiteRunResult stopSuiteRun(StopSuiteRunRequest stopSuiteRunRequest);
/**
*
* Adds to and modifies existing tags of an IoT Device Advisor resource.
*
*
* Requires permission to access the TagResource action.
*
*
* @param tagResourceRequest
* @return Result of the TagResource operation returned by the service.
* @throws InternalServerException
* Sends an Internal Failure exception.
* @throws ValidationException
* Sends a validation exception.
* @throws ResourceNotFoundException
* Sends a Resource Not Found exception.
* @sample AWSIoTDeviceAdvisor.TagResource
* @see AWS
* API Documentation
*/
TagResourceResult tagResource(TagResourceRequest tagResourceRequest);
/**
*
* Removes tags from an IoT Device Advisor resource.
*
*
* Requires permission to access the UntagResource action.
*
*
* @param untagResourceRequest
* @return Result of the UntagResource operation returned by the service.
* @throws InternalServerException
* Sends an Internal Failure exception.
* @throws ValidationException
* Sends a validation exception.
* @throws ResourceNotFoundException
* Sends a Resource Not Found exception.
* @sample AWSIoTDeviceAdvisor.UntagResource
* @see AWS
* API Documentation
*/
UntagResourceResult untagResource(UntagResourceRequest untagResourceRequest);
/**
*
* Updates a Device Advisor test suite.
*
*
* Requires permission to access the UpdateSuiteDefinition action.
*
*
* @param updateSuiteDefinitionRequest
* @return Result of the UpdateSuiteDefinition operation returned by the service.
* @throws ValidationException
* Sends a validation exception.
* @throws InternalServerException
* Sends an Internal Failure exception.
* @sample AWSIoTDeviceAdvisor.UpdateSuiteDefinition
* @see AWS API Documentation
*/
UpdateSuiteDefinitionResult updateSuiteDefinition(UpdateSuiteDefinitionRequest updateSuiteDefinitionRequest);
/**
* Shuts down this client object, releasing any resources that might be held open. This is an optional method, and
* callers are not expected to call it, but can if they want to explicitly release any open resources. Once a client
* has been shutdown, it should not be used to make any more requests.
*/
void shutdown();
/**
* Returns additional metadata for a previously executed successful request, typically used for debugging issues
* where a service isn't acting as expected. This data isn't considered part of the result data returned by an
* operation, so it's available through this separate, diagnostic interface.
*
* Response metadata is only cached for a limited period of time, so if you need to access this extra diagnostic
* information for an executed request, you should use this method to retrieve it as soon as possible after
* executing a request.
*
* @param request
* The originally executed request.
*
* @return The response metadata for the specified request, or null if none is available.
*/
ResponseMetadata getCachedResponseMetadata(AmazonWebServiceRequest request);
}