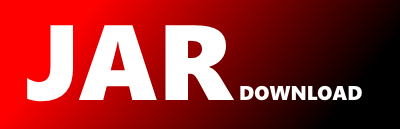
com.amazonaws.services.iotevents.model.Action Maven / Gradle / Ivy
Show all versions of aws-java-sdk-iotevents Show documentation
/*
* Copyright 2015-2020 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.iotevents.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* An action to be performed when the condition
is TRUE.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class Action implements Serializable, Cloneable, StructuredPojo {
/**
*
* Sets a variable to a specified value.
*
*/
private SetVariableAction setVariable;
/**
*
* Sends an Amazon SNS message.
*
*/
private SNSTopicPublishAction sns;
/**
*
* Publishes an MQTT message with the given topic to the AWS IoT message broker.
*
*/
private IotTopicPublishAction iotTopicPublish;
/**
*
* Information needed to set the timer.
*
*/
private SetTimerAction setTimer;
/**
*
* Information needed to clear the timer.
*
*/
private ClearTimerAction clearTimer;
/**
*
* Information needed to reset the timer.
*
*/
private ResetTimerAction resetTimer;
/**
*
* Calls a Lambda function, passing in information about the detector model instance and the event that triggered
* the action.
*
*/
private LambdaAction lambda;
/**
*
* Sends AWS IoT Events input, which passes information about the detector model instance and the event that
* triggered the action.
*
*/
private IotEventsAction iotEvents;
/**
*
* Sends information about the detector model instance and the event that triggered the action to an Amazon SQS
* queue.
*
*/
private SqsAction sqs;
/**
*
* Sends information about the detector model instance and the event that triggered the action to an Amazon Kinesis
* Data Firehose delivery stream.
*
*/
private FirehoseAction firehose;
/**
*
* Writes to the DynamoDB table that you created. The default action payload contains all attribute-value pairs that
* have the information about the detector model instance and the event that triggered the action. You can also
* customize the payload.
* One column of the DynamoDB table receives all attribute-value pairs in the payload that you specify. For more
* information, see Actions in
* AWS IoT Events Developer Guide.
*
*/
private DynamoDBAction dynamoDB;
/**
*
* Writes to the DynamoDB table that you created. The default action payload contains all attribute-value pairs that
* have the information about the detector model instance and the event that triggered the action. You can also
* customize the payload. A
* separate column of the DynamoDB table receives one attribute-value pair in the payload that you specify. For more
* information, see Actions in
* AWS IoT Events Developer Guide.
*
*/
private DynamoDBv2Action dynamoDBv2;
/**
*
* Sends information about the detector model instance and the event that triggered the action to an asset property
* in AWS IoT SiteWise .
*
*/
private IotSiteWiseAction iotSiteWise;
/**
*
* Sets a variable to a specified value.
*
*
* @param setVariable
* Sets a variable to a specified value.
*/
public void setSetVariable(SetVariableAction setVariable) {
this.setVariable = setVariable;
}
/**
*
* Sets a variable to a specified value.
*
*
* @return Sets a variable to a specified value.
*/
public SetVariableAction getSetVariable() {
return this.setVariable;
}
/**
*
* Sets a variable to a specified value.
*
*
* @param setVariable
* Sets a variable to a specified value.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Action withSetVariable(SetVariableAction setVariable) {
setSetVariable(setVariable);
return this;
}
/**
*
* Sends an Amazon SNS message.
*
*
* @param sns
* Sends an Amazon SNS message.
*/
public void setSns(SNSTopicPublishAction sns) {
this.sns = sns;
}
/**
*
* Sends an Amazon SNS message.
*
*
* @return Sends an Amazon SNS message.
*/
public SNSTopicPublishAction getSns() {
return this.sns;
}
/**
*
* Sends an Amazon SNS message.
*
*
* @param sns
* Sends an Amazon SNS message.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Action withSns(SNSTopicPublishAction sns) {
setSns(sns);
return this;
}
/**
*
* Publishes an MQTT message with the given topic to the AWS IoT message broker.
*
*
* @param iotTopicPublish
* Publishes an MQTT message with the given topic to the AWS IoT message broker.
*/
public void setIotTopicPublish(IotTopicPublishAction iotTopicPublish) {
this.iotTopicPublish = iotTopicPublish;
}
/**
*
* Publishes an MQTT message with the given topic to the AWS IoT message broker.
*
*
* @return Publishes an MQTT message with the given topic to the AWS IoT message broker.
*/
public IotTopicPublishAction getIotTopicPublish() {
return this.iotTopicPublish;
}
/**
*
* Publishes an MQTT message with the given topic to the AWS IoT message broker.
*
*
* @param iotTopicPublish
* Publishes an MQTT message with the given topic to the AWS IoT message broker.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Action withIotTopicPublish(IotTopicPublishAction iotTopicPublish) {
setIotTopicPublish(iotTopicPublish);
return this;
}
/**
*
* Information needed to set the timer.
*
*
* @param setTimer
* Information needed to set the timer.
*/
public void setSetTimer(SetTimerAction setTimer) {
this.setTimer = setTimer;
}
/**
*
* Information needed to set the timer.
*
*
* @return Information needed to set the timer.
*/
public SetTimerAction getSetTimer() {
return this.setTimer;
}
/**
*
* Information needed to set the timer.
*
*
* @param setTimer
* Information needed to set the timer.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Action withSetTimer(SetTimerAction setTimer) {
setSetTimer(setTimer);
return this;
}
/**
*
* Information needed to clear the timer.
*
*
* @param clearTimer
* Information needed to clear the timer.
*/
public void setClearTimer(ClearTimerAction clearTimer) {
this.clearTimer = clearTimer;
}
/**
*
* Information needed to clear the timer.
*
*
* @return Information needed to clear the timer.
*/
public ClearTimerAction getClearTimer() {
return this.clearTimer;
}
/**
*
* Information needed to clear the timer.
*
*
* @param clearTimer
* Information needed to clear the timer.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Action withClearTimer(ClearTimerAction clearTimer) {
setClearTimer(clearTimer);
return this;
}
/**
*
* Information needed to reset the timer.
*
*
* @param resetTimer
* Information needed to reset the timer.
*/
public void setResetTimer(ResetTimerAction resetTimer) {
this.resetTimer = resetTimer;
}
/**
*
* Information needed to reset the timer.
*
*
* @return Information needed to reset the timer.
*/
public ResetTimerAction getResetTimer() {
return this.resetTimer;
}
/**
*
* Information needed to reset the timer.
*
*
* @param resetTimer
* Information needed to reset the timer.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Action withResetTimer(ResetTimerAction resetTimer) {
setResetTimer(resetTimer);
return this;
}
/**
*
* Calls a Lambda function, passing in information about the detector model instance and the event that triggered
* the action.
*
*
* @param lambda
* Calls a Lambda function, passing in information about the detector model instance and the event that
* triggered the action.
*/
public void setLambda(LambdaAction lambda) {
this.lambda = lambda;
}
/**
*
* Calls a Lambda function, passing in information about the detector model instance and the event that triggered
* the action.
*
*
* @return Calls a Lambda function, passing in information about the detector model instance and the event that
* triggered the action.
*/
public LambdaAction getLambda() {
return this.lambda;
}
/**
*
* Calls a Lambda function, passing in information about the detector model instance and the event that triggered
* the action.
*
*
* @param lambda
* Calls a Lambda function, passing in information about the detector model instance and the event that
* triggered the action.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Action withLambda(LambdaAction lambda) {
setLambda(lambda);
return this;
}
/**
*
* Sends AWS IoT Events input, which passes information about the detector model instance and the event that
* triggered the action.
*
*
* @param iotEvents
* Sends AWS IoT Events input, which passes information about the detector model instance and the event that
* triggered the action.
*/
public void setIotEvents(IotEventsAction iotEvents) {
this.iotEvents = iotEvents;
}
/**
*
* Sends AWS IoT Events input, which passes information about the detector model instance and the event that
* triggered the action.
*
*
* @return Sends AWS IoT Events input, which passes information about the detector model instance and the event that
* triggered the action.
*/
public IotEventsAction getIotEvents() {
return this.iotEvents;
}
/**
*
* Sends AWS IoT Events input, which passes information about the detector model instance and the event that
* triggered the action.
*
*
* @param iotEvents
* Sends AWS IoT Events input, which passes information about the detector model instance and the event that
* triggered the action.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Action withIotEvents(IotEventsAction iotEvents) {
setIotEvents(iotEvents);
return this;
}
/**
*
* Sends information about the detector model instance and the event that triggered the action to an Amazon SQS
* queue.
*
*
* @param sqs
* Sends information about the detector model instance and the event that triggered the action to an Amazon
* SQS queue.
*/
public void setSqs(SqsAction sqs) {
this.sqs = sqs;
}
/**
*
* Sends information about the detector model instance and the event that triggered the action to an Amazon SQS
* queue.
*
*
* @return Sends information about the detector model instance and the event that triggered the action to an Amazon
* SQS queue.
*/
public SqsAction getSqs() {
return this.sqs;
}
/**
*
* Sends information about the detector model instance and the event that triggered the action to an Amazon SQS
* queue.
*
*
* @param sqs
* Sends information about the detector model instance and the event that triggered the action to an Amazon
* SQS queue.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Action withSqs(SqsAction sqs) {
setSqs(sqs);
return this;
}
/**
*
* Sends information about the detector model instance and the event that triggered the action to an Amazon Kinesis
* Data Firehose delivery stream.
*
*
* @param firehose
* Sends information about the detector model instance and the event that triggered the action to an Amazon
* Kinesis Data Firehose delivery stream.
*/
public void setFirehose(FirehoseAction firehose) {
this.firehose = firehose;
}
/**
*
* Sends information about the detector model instance and the event that triggered the action to an Amazon Kinesis
* Data Firehose delivery stream.
*
*
* @return Sends information about the detector model instance and the event that triggered the action to an Amazon
* Kinesis Data Firehose delivery stream.
*/
public FirehoseAction getFirehose() {
return this.firehose;
}
/**
*
* Sends information about the detector model instance and the event that triggered the action to an Amazon Kinesis
* Data Firehose delivery stream.
*
*
* @param firehose
* Sends information about the detector model instance and the event that triggered the action to an Amazon
* Kinesis Data Firehose delivery stream.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Action withFirehose(FirehoseAction firehose) {
setFirehose(firehose);
return this;
}
/**
*
* Writes to the DynamoDB table that you created. The default action payload contains all attribute-value pairs that
* have the information about the detector model instance and the event that triggered the action. You can also
* customize the payload.
* One column of the DynamoDB table receives all attribute-value pairs in the payload that you specify. For more
* information, see Actions in
* AWS IoT Events Developer Guide.
*
*
* @param dynamoDB
* Writes to the DynamoDB table that you created. The default action payload contains all attribute-value
* pairs that have the information about the detector model instance and the event that triggered the action.
* You can also customize the payload. One column
* of the DynamoDB table receives all attribute-value pairs in the payload that you specify. For more
* information, see Actions in AWS IoT Events Developer Guide.
*/
public void setDynamoDB(DynamoDBAction dynamoDB) {
this.dynamoDB = dynamoDB;
}
/**
*
* Writes to the DynamoDB table that you created. The default action payload contains all attribute-value pairs that
* have the information about the detector model instance and the event that triggered the action. You can also
* customize the payload.
* One column of the DynamoDB table receives all attribute-value pairs in the payload that you specify. For more
* information, see Actions in
* AWS IoT Events Developer Guide.
*
*
* @return Writes to the DynamoDB table that you created. The default action payload contains all attribute-value
* pairs that have the information about the detector model instance and the event that triggered the
* action. You can also customize the payload. One column
* of the DynamoDB table receives all attribute-value pairs in the payload that you specify. For more
* information, see Actions in AWS IoT Events Developer Guide.
*/
public DynamoDBAction getDynamoDB() {
return this.dynamoDB;
}
/**
*
* Writes to the DynamoDB table that you created. The default action payload contains all attribute-value pairs that
* have the information about the detector model instance and the event that triggered the action. You can also
* customize the payload.
* One column of the DynamoDB table receives all attribute-value pairs in the payload that you specify. For more
* information, see Actions in
* AWS IoT Events Developer Guide.
*
*
* @param dynamoDB
* Writes to the DynamoDB table that you created. The default action payload contains all attribute-value
* pairs that have the information about the detector model instance and the event that triggered the action.
* You can also customize the payload. One column
* of the DynamoDB table receives all attribute-value pairs in the payload that you specify. For more
* information, see Actions in AWS IoT Events Developer Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Action withDynamoDB(DynamoDBAction dynamoDB) {
setDynamoDB(dynamoDB);
return this;
}
/**
*
* Writes to the DynamoDB table that you created. The default action payload contains all attribute-value pairs that
* have the information about the detector model instance and the event that triggered the action. You can also
* customize the payload. A
* separate column of the DynamoDB table receives one attribute-value pair in the payload that you specify. For more
* information, see Actions in
* AWS IoT Events Developer Guide.
*
*
* @param dynamoDBv2
* Writes to the DynamoDB table that you created. The default action payload contains all attribute-value
* pairs that have the information about the detector model instance and the event that triggered the action.
* You can also customize the payload. A separate
* column of the DynamoDB table receives one attribute-value pair in the payload that you specify. For more
* information, see Actions in AWS IoT Events Developer Guide.
*/
public void setDynamoDBv2(DynamoDBv2Action dynamoDBv2) {
this.dynamoDBv2 = dynamoDBv2;
}
/**
*
* Writes to the DynamoDB table that you created. The default action payload contains all attribute-value pairs that
* have the information about the detector model instance and the event that triggered the action. You can also
* customize the payload. A
* separate column of the DynamoDB table receives one attribute-value pair in the payload that you specify. For more
* information, see Actions in
* AWS IoT Events Developer Guide.
*
*
* @return Writes to the DynamoDB table that you created. The default action payload contains all attribute-value
* pairs that have the information about the detector model instance and the event that triggered the
* action. You can also customize the payload. A separate
* column of the DynamoDB table receives one attribute-value pair in the payload that you specify. For more
* information, see Actions in AWS IoT Events Developer Guide.
*/
public DynamoDBv2Action getDynamoDBv2() {
return this.dynamoDBv2;
}
/**
*
* Writes to the DynamoDB table that you created. The default action payload contains all attribute-value pairs that
* have the information about the detector model instance and the event that triggered the action. You can also
* customize the payload. A
* separate column of the DynamoDB table receives one attribute-value pair in the payload that you specify. For more
* information, see Actions in
* AWS IoT Events Developer Guide.
*
*
* @param dynamoDBv2
* Writes to the DynamoDB table that you created. The default action payload contains all attribute-value
* pairs that have the information about the detector model instance and the event that triggered the action.
* You can also customize the payload. A separate
* column of the DynamoDB table receives one attribute-value pair in the payload that you specify. For more
* information, see Actions in AWS IoT Events Developer Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Action withDynamoDBv2(DynamoDBv2Action dynamoDBv2) {
setDynamoDBv2(dynamoDBv2);
return this;
}
/**
*
* Sends information about the detector model instance and the event that triggered the action to an asset property
* in AWS IoT SiteWise .
*
*
* @param iotSiteWise
* Sends information about the detector model instance and the event that triggered the action to an asset
* property in AWS IoT SiteWise .
*/
public void setIotSiteWise(IotSiteWiseAction iotSiteWise) {
this.iotSiteWise = iotSiteWise;
}
/**
*
* Sends information about the detector model instance and the event that triggered the action to an asset property
* in AWS IoT SiteWise .
*
*
* @return Sends information about the detector model instance and the event that triggered the action to an asset
* property in AWS IoT SiteWise .
*/
public IotSiteWiseAction getIotSiteWise() {
return this.iotSiteWise;
}
/**
*
* Sends information about the detector model instance and the event that triggered the action to an asset property
* in AWS IoT SiteWise .
*
*
* @param iotSiteWise
* Sends information about the detector model instance and the event that triggered the action to an asset
* property in AWS IoT SiteWise .
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Action withIotSiteWise(IotSiteWiseAction iotSiteWise) {
setIotSiteWise(iotSiteWise);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getSetVariable() != null)
sb.append("SetVariable: ").append(getSetVariable()).append(",");
if (getSns() != null)
sb.append("Sns: ").append(getSns()).append(",");
if (getIotTopicPublish() != null)
sb.append("IotTopicPublish: ").append(getIotTopicPublish()).append(",");
if (getSetTimer() != null)
sb.append("SetTimer: ").append(getSetTimer()).append(",");
if (getClearTimer() != null)
sb.append("ClearTimer: ").append(getClearTimer()).append(",");
if (getResetTimer() != null)
sb.append("ResetTimer: ").append(getResetTimer()).append(",");
if (getLambda() != null)
sb.append("Lambda: ").append(getLambda()).append(",");
if (getIotEvents() != null)
sb.append("IotEvents: ").append(getIotEvents()).append(",");
if (getSqs() != null)
sb.append("Sqs: ").append(getSqs()).append(",");
if (getFirehose() != null)
sb.append("Firehose: ").append(getFirehose()).append(",");
if (getDynamoDB() != null)
sb.append("DynamoDB: ").append(getDynamoDB()).append(",");
if (getDynamoDBv2() != null)
sb.append("DynamoDBv2: ").append(getDynamoDBv2()).append(",");
if (getIotSiteWise() != null)
sb.append("IotSiteWise: ").append(getIotSiteWise());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof Action == false)
return false;
Action other = (Action) obj;
if (other.getSetVariable() == null ^ this.getSetVariable() == null)
return false;
if (other.getSetVariable() != null && other.getSetVariable().equals(this.getSetVariable()) == false)
return false;
if (other.getSns() == null ^ this.getSns() == null)
return false;
if (other.getSns() != null && other.getSns().equals(this.getSns()) == false)
return false;
if (other.getIotTopicPublish() == null ^ this.getIotTopicPublish() == null)
return false;
if (other.getIotTopicPublish() != null && other.getIotTopicPublish().equals(this.getIotTopicPublish()) == false)
return false;
if (other.getSetTimer() == null ^ this.getSetTimer() == null)
return false;
if (other.getSetTimer() != null && other.getSetTimer().equals(this.getSetTimer()) == false)
return false;
if (other.getClearTimer() == null ^ this.getClearTimer() == null)
return false;
if (other.getClearTimer() != null && other.getClearTimer().equals(this.getClearTimer()) == false)
return false;
if (other.getResetTimer() == null ^ this.getResetTimer() == null)
return false;
if (other.getResetTimer() != null && other.getResetTimer().equals(this.getResetTimer()) == false)
return false;
if (other.getLambda() == null ^ this.getLambda() == null)
return false;
if (other.getLambda() != null && other.getLambda().equals(this.getLambda()) == false)
return false;
if (other.getIotEvents() == null ^ this.getIotEvents() == null)
return false;
if (other.getIotEvents() != null && other.getIotEvents().equals(this.getIotEvents()) == false)
return false;
if (other.getSqs() == null ^ this.getSqs() == null)
return false;
if (other.getSqs() != null && other.getSqs().equals(this.getSqs()) == false)
return false;
if (other.getFirehose() == null ^ this.getFirehose() == null)
return false;
if (other.getFirehose() != null && other.getFirehose().equals(this.getFirehose()) == false)
return false;
if (other.getDynamoDB() == null ^ this.getDynamoDB() == null)
return false;
if (other.getDynamoDB() != null && other.getDynamoDB().equals(this.getDynamoDB()) == false)
return false;
if (other.getDynamoDBv2() == null ^ this.getDynamoDBv2() == null)
return false;
if (other.getDynamoDBv2() != null && other.getDynamoDBv2().equals(this.getDynamoDBv2()) == false)
return false;
if (other.getIotSiteWise() == null ^ this.getIotSiteWise() == null)
return false;
if (other.getIotSiteWise() != null && other.getIotSiteWise().equals(this.getIotSiteWise()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getSetVariable() == null) ? 0 : getSetVariable().hashCode());
hashCode = prime * hashCode + ((getSns() == null) ? 0 : getSns().hashCode());
hashCode = prime * hashCode + ((getIotTopicPublish() == null) ? 0 : getIotTopicPublish().hashCode());
hashCode = prime * hashCode + ((getSetTimer() == null) ? 0 : getSetTimer().hashCode());
hashCode = prime * hashCode + ((getClearTimer() == null) ? 0 : getClearTimer().hashCode());
hashCode = prime * hashCode + ((getResetTimer() == null) ? 0 : getResetTimer().hashCode());
hashCode = prime * hashCode + ((getLambda() == null) ? 0 : getLambda().hashCode());
hashCode = prime * hashCode + ((getIotEvents() == null) ? 0 : getIotEvents().hashCode());
hashCode = prime * hashCode + ((getSqs() == null) ? 0 : getSqs().hashCode());
hashCode = prime * hashCode + ((getFirehose() == null) ? 0 : getFirehose().hashCode());
hashCode = prime * hashCode + ((getDynamoDB() == null) ? 0 : getDynamoDB().hashCode());
hashCode = prime * hashCode + ((getDynamoDBv2() == null) ? 0 : getDynamoDBv2().hashCode());
hashCode = prime * hashCode + ((getIotSiteWise() == null) ? 0 : getIotSiteWise().hashCode());
return hashCode;
}
@Override
public Action clone() {
try {
return (Action) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.iotevents.model.transform.ActionMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}