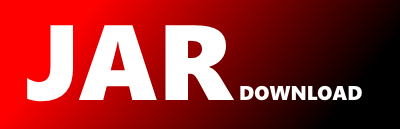
com.amazonaws.services.iotevents.AWSIoTEvents Maven / Gradle / Ivy
Show all versions of aws-java-sdk-iotevents Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.iotevents;
import javax.annotation.Generated;
import com.amazonaws.*;
import com.amazonaws.regions.*;
import com.amazonaws.services.iotevents.model.*;
/**
* Interface for accessing AWS IoT Events.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.iotevents.AbstractAWSIoTEvents} instead.
*
*
*
* AWS IoT Events monitors your equipment or device fleets for failures or changes in operation, and triggers actions
* when such events occur. You can use AWS IoT Events API operations to create, read, update, and delete inputs and
* detector models, and to list their versions.
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AWSIoTEvents {
/**
* The region metadata service name for computing region endpoints. You can use this value to retrieve metadata
* (such as supported regions) of the service.
*
* @see RegionUtils#getRegionsForService(String)
*/
String ENDPOINT_PREFIX = "iotevents";
/**
*
* Creates an alarm model to monitor an AWS IoT Events input attribute. You can use the alarm to get notified when
* the value is outside a specified range. For more information, see Create an alarm model
* in the AWS IoT Events Developer Guide.
*
*
* @param createAlarmModelRequest
* @return Result of the CreateAlarmModel operation returned by the service.
* @throws InvalidRequestException
* The request was invalid.
* @throws ResourceInUseException
* The resource is in use.
* @throws ResourceAlreadyExistsException
* The resource already exists.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ThrottlingException
* The request could not be completed due to throttling.
* @throws InternalFailureException
* An internal failure occurred.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @sample AWSIoTEvents.CreateAlarmModel
* @see AWS API
* Documentation
*/
CreateAlarmModelResult createAlarmModel(CreateAlarmModelRequest createAlarmModelRequest);
/**
*
* Creates a detector model.
*
*
* @param createDetectorModelRequest
* @return Result of the CreateDetectorModel operation returned by the service.
* @throws InvalidRequestException
* The request was invalid.
* @throws ResourceInUseException
* The resource is in use.
* @throws ResourceAlreadyExistsException
* The resource already exists.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ThrottlingException
* The request could not be completed due to throttling.
* @throws InternalFailureException
* An internal failure occurred.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @sample AWSIoTEvents.CreateDetectorModel
* @see AWS
* API Documentation
*/
CreateDetectorModelResult createDetectorModel(CreateDetectorModelRequest createDetectorModelRequest);
/**
*
* Creates an input.
*
*
* @param createInputRequest
* @return Result of the CreateInput operation returned by the service.
* @throws InvalidRequestException
* The request was invalid.
* @throws ThrottlingException
* The request could not be completed due to throttling.
* @throws InternalFailureException
* An internal failure occurred.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ResourceAlreadyExistsException
* The resource already exists.
* @sample AWSIoTEvents.CreateInput
* @see AWS API
* Documentation
*/
CreateInputResult createInput(CreateInputRequest createInputRequest);
/**
*
* Deletes an alarm model. Any alarm instances that were created based on this alarm model are also deleted. This
* action can't be undone.
*
*
* @param deleteAlarmModelRequest
* @return Result of the DeleteAlarmModel operation returned by the service.
* @throws InvalidRequestException
* The request was invalid.
* @throws ResourceInUseException
* The resource is in use.
* @throws ResourceNotFoundException
* The resource was not found.
* @throws ThrottlingException
* The request could not be completed due to throttling.
* @throws InternalFailureException
* An internal failure occurred.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @sample AWSIoTEvents.DeleteAlarmModel
* @see AWS API
* Documentation
*/
DeleteAlarmModelResult deleteAlarmModel(DeleteAlarmModelRequest deleteAlarmModelRequest);
/**
*
* Deletes a detector model. Any active instances of the detector model are also deleted.
*
*
* @param deleteDetectorModelRequest
* @return Result of the DeleteDetectorModel operation returned by the service.
* @throws InvalidRequestException
* The request was invalid.
* @throws ResourceInUseException
* The resource is in use.
* @throws ResourceNotFoundException
* The resource was not found.
* @throws ThrottlingException
* The request could not be completed due to throttling.
* @throws InternalFailureException
* An internal failure occurred.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @sample AWSIoTEvents.DeleteDetectorModel
* @see AWS
* API Documentation
*/
DeleteDetectorModelResult deleteDetectorModel(DeleteDetectorModelRequest deleteDetectorModelRequest);
/**
*
* Deletes an input.
*
*
* @param deleteInputRequest
* @return Result of the DeleteInput operation returned by the service.
* @throws InvalidRequestException
* The request was invalid.
* @throws ResourceNotFoundException
* The resource was not found.
* @throws ThrottlingException
* The request could not be completed due to throttling.
* @throws InternalFailureException
* An internal failure occurred.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ResourceInUseException
* The resource is in use.
* @sample AWSIoTEvents.DeleteInput
* @see AWS API
* Documentation
*/
DeleteInputResult deleteInput(DeleteInputRequest deleteInputRequest);
/**
*
* Retrieves information about an alarm model. If you don't specify a value for the alarmModelVersion
* parameter, the latest version is returned.
*
*
* @param describeAlarmModelRequest
* @return Result of the DescribeAlarmModel operation returned by the service.
* @throws InvalidRequestException
* The request was invalid.
* @throws ResourceNotFoundException
* The resource was not found.
* @throws ThrottlingException
* The request could not be completed due to throttling.
* @throws InternalFailureException
* An internal failure occurred.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @sample AWSIoTEvents.DescribeAlarmModel
* @see AWS
* API Documentation
*/
DescribeAlarmModelResult describeAlarmModel(DescribeAlarmModelRequest describeAlarmModelRequest);
/**
*
* Describes a detector model. If the version
parameter is not specified, information about the latest
* version is returned.
*
*
* @param describeDetectorModelRequest
* @return Result of the DescribeDetectorModel operation returned by the service.
* @throws InvalidRequestException
* The request was invalid.
* @throws ResourceNotFoundException
* The resource was not found.
* @throws ThrottlingException
* The request could not be completed due to throttling.
* @throws InternalFailureException
* An internal failure occurred.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @sample AWSIoTEvents.DescribeDetectorModel
* @see AWS API Documentation
*/
DescribeDetectorModelResult describeDetectorModel(DescribeDetectorModelRequest describeDetectorModelRequest);
/**
*
* Retrieves runtime information about a detector model analysis.
*
*
*
* After AWS IoT Events starts analyzing your detector model, you have up to 24 hours to retrieve the analysis
* results.
*
*
*
* @param describeDetectorModelAnalysisRequest
* @return Result of the DescribeDetectorModelAnalysis operation returned by the service.
* @throws InvalidRequestException
* The request was invalid.
* @throws ResourceNotFoundException
* The resource was not found.
* @throws ThrottlingException
* The request could not be completed due to throttling.
* @throws InternalFailureException
* An internal failure occurred.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @sample AWSIoTEvents.DescribeDetectorModelAnalysis
* @see AWS API Documentation
*/
DescribeDetectorModelAnalysisResult describeDetectorModelAnalysis(DescribeDetectorModelAnalysisRequest describeDetectorModelAnalysisRequest);
/**
*
* Describes an input.
*
*
* @param describeInputRequest
* @return Result of the DescribeInput operation returned by the service.
* @throws InvalidRequestException
* The request was invalid.
* @throws ResourceNotFoundException
* The resource was not found.
* @throws ThrottlingException
* The request could not be completed due to throttling.
* @throws InternalFailureException
* An internal failure occurred.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @sample AWSIoTEvents.DescribeInput
* @see AWS API
* Documentation
*/
DescribeInputResult describeInput(DescribeInputRequest describeInputRequest);
/**
*
* Retrieves the current settings of the AWS IoT Events logging options.
*
*
* @param describeLoggingOptionsRequest
* @return Result of the DescribeLoggingOptions operation returned by the service.
* @throws InvalidRequestException
* The request was invalid.
* @throws ThrottlingException
* The request could not be completed due to throttling.
* @throws InternalFailureException
* An internal failure occurred.
* @throws ResourceNotFoundException
* The resource was not found.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws UnsupportedOperationException
* The requested operation is not supported.
* @sample AWSIoTEvents.DescribeLoggingOptions
* @see AWS API Documentation
*/
DescribeLoggingOptionsResult describeLoggingOptions(DescribeLoggingOptionsRequest describeLoggingOptionsRequest);
/**
*
* Retrieves one or more analysis results of the detector model.
*
*
*
* After AWS IoT Events starts analyzing your detector model, you have up to 24 hours to retrieve the analysis
* results.
*
*
*
* @param getDetectorModelAnalysisResultsRequest
* @return Result of the GetDetectorModelAnalysisResults operation returned by the service.
* @throws InvalidRequestException
* The request was invalid.
* @throws ResourceNotFoundException
* The resource was not found.
* @throws ThrottlingException
* The request could not be completed due to throttling.
* @throws InternalFailureException
* An internal failure occurred.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @sample AWSIoTEvents.GetDetectorModelAnalysisResults
* @see AWS API Documentation
*/
GetDetectorModelAnalysisResultsResult getDetectorModelAnalysisResults(GetDetectorModelAnalysisResultsRequest getDetectorModelAnalysisResultsRequest);
/**
*
* Lists all the versions of an alarm model. The operation returns only the metadata associated with each alarm
* model version.
*
*
* @param listAlarmModelVersionsRequest
* @return Result of the ListAlarmModelVersions operation returned by the service.
* @throws InvalidRequestException
* The request was invalid.
* @throws ResourceNotFoundException
* The resource was not found.
* @throws ThrottlingException
* The request could not be completed due to throttling.
* @throws InternalFailureException
* An internal failure occurred.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @sample AWSIoTEvents.ListAlarmModelVersions
* @see AWS API Documentation
*/
ListAlarmModelVersionsResult listAlarmModelVersions(ListAlarmModelVersionsRequest listAlarmModelVersionsRequest);
/**
*
* Lists the alarm models that you created. The operation returns only the metadata associated with each alarm
* model.
*
*
* @param listAlarmModelsRequest
* @return Result of the ListAlarmModels operation returned by the service.
* @throws InvalidRequestException
* The request was invalid.
* @throws ThrottlingException
* The request could not be completed due to throttling.
* @throws InternalFailureException
* An internal failure occurred.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @sample AWSIoTEvents.ListAlarmModels
* @see AWS API
* Documentation
*/
ListAlarmModelsResult listAlarmModels(ListAlarmModelsRequest listAlarmModelsRequest);
/**
*
* Lists all the versions of a detector model. Only the metadata associated with each detector model version is
* returned.
*
*
* @param listDetectorModelVersionsRequest
* @return Result of the ListDetectorModelVersions operation returned by the service.
* @throws InvalidRequestException
* The request was invalid.
* @throws ResourceNotFoundException
* The resource was not found.
* @throws ThrottlingException
* The request could not be completed due to throttling.
* @throws InternalFailureException
* An internal failure occurred.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @sample AWSIoTEvents.ListDetectorModelVersions
* @see AWS API Documentation
*/
ListDetectorModelVersionsResult listDetectorModelVersions(ListDetectorModelVersionsRequest listDetectorModelVersionsRequest);
/**
*
* Lists the detector models you have created. Only the metadata associated with each detector model is returned.
*
*
* @param listDetectorModelsRequest
* @return Result of the ListDetectorModels operation returned by the service.
* @throws InvalidRequestException
* The request was invalid.
* @throws ThrottlingException
* The request could not be completed due to throttling.
* @throws InternalFailureException
* An internal failure occurred.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @sample AWSIoTEvents.ListDetectorModels
* @see AWS
* API Documentation
*/
ListDetectorModelsResult listDetectorModels(ListDetectorModelsRequest listDetectorModelsRequest);
/**
*
* Lists one or more input routings.
*
*
* @param listInputRoutingsRequest
* @return Result of the ListInputRoutings operation returned by the service.
* @throws InvalidRequestException
* The request was invalid.
* @throws ThrottlingException
* The request could not be completed due to throttling.
* @throws InternalFailureException
* An internal failure occurred.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ResourceNotFoundException
* The resource was not found.
* @sample AWSIoTEvents.ListInputRoutings
* @see AWS
* API Documentation
*/
ListInputRoutingsResult listInputRoutings(ListInputRoutingsRequest listInputRoutingsRequest);
/**
*
* Lists the inputs you have created.
*
*
* @param listInputsRequest
* @return Result of the ListInputs operation returned by the service.
* @throws InvalidRequestException
* The request was invalid.
* @throws ThrottlingException
* The request could not be completed due to throttling.
* @throws InternalFailureException
* An internal failure occurred.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @sample AWSIoTEvents.ListInputs
* @see AWS API
* Documentation
*/
ListInputsResult listInputs(ListInputsRequest listInputsRequest);
/**
*
* Lists the tags (metadata) you have assigned to the resource.
*
*
* @param listTagsForResourceRequest
* @return Result of the ListTagsForResource operation returned by the service.
* @throws InvalidRequestException
* The request was invalid.
* @throws ResourceNotFoundException
* The resource was not found.
* @throws ResourceInUseException
* The resource is in use.
* @throws ThrottlingException
* The request could not be completed due to throttling.
* @throws InternalFailureException
* An internal failure occurred.
* @sample AWSIoTEvents.ListTagsForResource
* @see AWS
* API Documentation
*/
ListTagsForResourceResult listTagsForResource(ListTagsForResourceRequest listTagsForResourceRequest);
/**
*
* Sets or updates the AWS IoT Events logging options.
*
*
* If you update the value of any loggingOptions
field, it takes up to one minute for the change to
* take effect. If you change the policy attached to the role you specified in the roleArn
field (for
* example, to correct an invalid policy), it takes up to five minutes for that change to take effect.
*
*
* @param putLoggingOptionsRequest
* @return Result of the PutLoggingOptions operation returned by the service.
* @throws InvalidRequestException
* The request was invalid.
* @throws ThrottlingException
* The request could not be completed due to throttling.
* @throws InternalFailureException
* An internal failure occurred.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws UnsupportedOperationException
* The requested operation is not supported.
* @throws ResourceInUseException
* The resource is in use.
* @sample AWSIoTEvents.PutLoggingOptions
* @see AWS
* API Documentation
*/
PutLoggingOptionsResult putLoggingOptions(PutLoggingOptionsRequest putLoggingOptionsRequest);
/**
*
* Performs an analysis of your detector model. For more information, see Troubleshooting a
* detector model in the AWS IoT Events Developer Guide.
*
*
* @param startDetectorModelAnalysisRequest
* @return Result of the StartDetectorModelAnalysis operation returned by the service.
* @throws InvalidRequestException
* The request was invalid.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ThrottlingException
* The request could not be completed due to throttling.
* @throws InternalFailureException
* An internal failure occurred.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @sample AWSIoTEvents.StartDetectorModelAnalysis
* @see AWS API Documentation
*/
StartDetectorModelAnalysisResult startDetectorModelAnalysis(StartDetectorModelAnalysisRequest startDetectorModelAnalysisRequest);
/**
*
* Adds to or modifies the tags of the given resource. Tags are metadata that can be used to manage a resource.
*
*
* @param tagResourceRequest
* @return Result of the TagResource operation returned by the service.
* @throws InvalidRequestException
* The request was invalid.
* @throws ResourceNotFoundException
* The resource was not found.
* @throws ResourceInUseException
* The resource is in use.
* @throws ThrottlingException
* The request could not be completed due to throttling.
* @throws LimitExceededException
* A limit was exceeded.
* @throws InternalFailureException
* An internal failure occurred.
* @sample AWSIoTEvents.TagResource
* @see AWS API
* Documentation
*/
TagResourceResult tagResource(TagResourceRequest tagResourceRequest);
/**
*
* Removes the given tags (metadata) from the resource.
*
*
* @param untagResourceRequest
* @return Result of the UntagResource operation returned by the service.
* @throws InvalidRequestException
* The request was invalid.
* @throws ResourceNotFoundException
* The resource was not found.
* @throws ResourceInUseException
* The resource is in use.
* @throws ThrottlingException
* The request could not be completed due to throttling.
* @throws InternalFailureException
* An internal failure occurred.
* @sample AWSIoTEvents.UntagResource
* @see AWS API
* Documentation
*/
UntagResourceResult untagResource(UntagResourceRequest untagResourceRequest);
/**
*
* Updates an alarm model. Any alarms that were created based on the previous version are deleted and then created
* again as new data arrives.
*
*
* @param updateAlarmModelRequest
* @return Result of the UpdateAlarmModel operation returned by the service.
* @throws InvalidRequestException
* The request was invalid.
* @throws ResourceInUseException
* The resource is in use.
* @throws ResourceNotFoundException
* The resource was not found.
* @throws ThrottlingException
* The request could not be completed due to throttling.
* @throws InternalFailureException
* An internal failure occurred.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @sample AWSIoTEvents.UpdateAlarmModel
* @see AWS API
* Documentation
*/
UpdateAlarmModelResult updateAlarmModel(UpdateAlarmModelRequest updateAlarmModelRequest);
/**
*
* Updates a detector model. Detectors (instances) spawned by the previous version are deleted and then re-created
* as new inputs arrive.
*
*
* @param updateDetectorModelRequest
* @return Result of the UpdateDetectorModel operation returned by the service.
* @throws InvalidRequestException
* The request was invalid.
* @throws ResourceInUseException
* The resource is in use.
* @throws ResourceNotFoundException
* The resource was not found.
* @throws ThrottlingException
* The request could not be completed due to throttling.
* @throws InternalFailureException
* An internal failure occurred.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @sample AWSIoTEvents.UpdateDetectorModel
* @see AWS
* API Documentation
*/
UpdateDetectorModelResult updateDetectorModel(UpdateDetectorModelRequest updateDetectorModelRequest);
/**
*
* Updates an input.
*
*
* @param updateInputRequest
* @return Result of the UpdateInput operation returned by the service.
* @throws InvalidRequestException
* The request was invalid.
* @throws ThrottlingException
* The request could not be completed due to throttling.
* @throws ResourceNotFoundException
* The resource was not found.
* @throws InternalFailureException
* An internal failure occurred.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ResourceInUseException
* The resource is in use.
* @sample AWSIoTEvents.UpdateInput
* @see AWS API
* Documentation
*/
UpdateInputResult updateInput(UpdateInputRequest updateInputRequest);
/**
* Shuts down this client object, releasing any resources that might be held open. This is an optional method, and
* callers are not expected to call it, but can if they want to explicitly release any open resources. Once a client
* has been shutdown, it should not be used to make any more requests.
*/
void shutdown();
/**
* Returns additional metadata for a previously executed successful request, typically used for debugging issues
* where a service isn't acting as expected. This data isn't considered part of the result data returned by an
* operation, so it's available through this separate, diagnostic interface.
*
* Response metadata is only cached for a limited period of time, so if you need to access this extra diagnostic
* information for an executed request, you should use this method to retrieve it as soon as possible after
* executing a request.
*
* @param request
* The originally executed request.
*
* @return The response metadata for the specified request, or null if none is available.
*/
ResponseMetadata getCachedResponseMetadata(AmazonWebServiceRequest request);
}