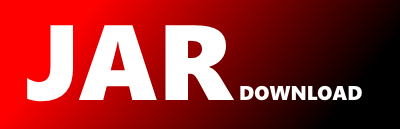
com.amazonaws.services.ioteventsdata.AWSIoTEventsDataAsync Maven / Gradle / Ivy
Show all versions of aws-java-sdk-ioteventsdata Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.ioteventsdata;
import javax.annotation.Generated;
import com.amazonaws.services.ioteventsdata.model.*;
/**
* Interface for accessing AWS IoT Events Data asynchronously. Each asynchronous method will return a Java Future object
* representing the asynchronous operation; overloads which accept an {@code AsyncHandler} can be used to receive
* notification when an asynchronous operation completes.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.ioteventsdata.AbstractAWSIoTEventsDataAsync} instead.
*
*
*
* IoT Events monitors your equipment or device fleets for failures or changes in operation, and triggers actions when
* such events occur. You can use IoT Events Data API commands to send inputs to detectors, list detectors, and view or
* update a detector's status.
*
*
* For more information, see What is IoT Events? in
* the IoT Events Developer Guide.
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AWSIoTEventsDataAsync extends AWSIoTEventsData {
/**
*
* Acknowledges one or more alarms. The alarms change to the ACKNOWLEDGED
state after you acknowledge
* them.
*
*
* @param batchAcknowledgeAlarmRequest
* @return A Java Future containing the result of the BatchAcknowledgeAlarm operation returned by the service.
* @sample AWSIoTEventsDataAsync.BatchAcknowledgeAlarm
* @see AWS API Documentation
*/
java.util.concurrent.Future batchAcknowledgeAlarmAsync(BatchAcknowledgeAlarmRequest batchAcknowledgeAlarmRequest);
/**
*
* Acknowledges one or more alarms. The alarms change to the ACKNOWLEDGED
state after you acknowledge
* them.
*
*
* @param batchAcknowledgeAlarmRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the BatchAcknowledgeAlarm operation returned by the service.
* @sample AWSIoTEventsDataAsyncHandler.BatchAcknowledgeAlarm
* @see AWS API Documentation
*/
java.util.concurrent.Future batchAcknowledgeAlarmAsync(BatchAcknowledgeAlarmRequest batchAcknowledgeAlarmRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes one or more detectors that were created. When a detector is deleted, its state will be cleared and the
* detector will be removed from the list of detectors. The deleted detector will no longer appear if referenced in
* the
* ListDetectors API call.
*
*
* @param batchDeleteDetectorRequest
* @return A Java Future containing the result of the BatchDeleteDetector operation returned by the service.
* @sample AWSIoTEventsDataAsync.BatchDeleteDetector
* @see AWS API Documentation
*/
java.util.concurrent.Future batchDeleteDetectorAsync(BatchDeleteDetectorRequest batchDeleteDetectorRequest);
/**
*
* Deletes one or more detectors that were created. When a detector is deleted, its state will be cleared and the
* detector will be removed from the list of detectors. The deleted detector will no longer appear if referenced in
* the
* ListDetectors API call.
*
*
* @param batchDeleteDetectorRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the BatchDeleteDetector operation returned by the service.
* @sample AWSIoTEventsDataAsyncHandler.BatchDeleteDetector
* @see AWS API Documentation
*/
java.util.concurrent.Future batchDeleteDetectorAsync(BatchDeleteDetectorRequest batchDeleteDetectorRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Disables one or more alarms. The alarms change to the DISABLED
state after you disable them.
*
*
* @param batchDisableAlarmRequest
* @return A Java Future containing the result of the BatchDisableAlarm operation returned by the service.
* @sample AWSIoTEventsDataAsync.BatchDisableAlarm
* @see AWS API Documentation
*/
java.util.concurrent.Future batchDisableAlarmAsync(BatchDisableAlarmRequest batchDisableAlarmRequest);
/**
*
* Disables one or more alarms. The alarms change to the DISABLED
state after you disable them.
*
*
* @param batchDisableAlarmRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the BatchDisableAlarm operation returned by the service.
* @sample AWSIoTEventsDataAsyncHandler.BatchDisableAlarm
* @see AWS API Documentation
*/
java.util.concurrent.Future batchDisableAlarmAsync(BatchDisableAlarmRequest batchDisableAlarmRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Enables one or more alarms. The alarms change to the NORMAL
state after you enable them.
*
*
* @param batchEnableAlarmRequest
* @return A Java Future containing the result of the BatchEnableAlarm operation returned by the service.
* @sample AWSIoTEventsDataAsync.BatchEnableAlarm
* @see AWS API Documentation
*/
java.util.concurrent.Future batchEnableAlarmAsync(BatchEnableAlarmRequest batchEnableAlarmRequest);
/**
*
* Enables one or more alarms. The alarms change to the NORMAL
state after you enable them.
*
*
* @param batchEnableAlarmRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the BatchEnableAlarm operation returned by the service.
* @sample AWSIoTEventsDataAsyncHandler.BatchEnableAlarm
* @see AWS API Documentation
*/
java.util.concurrent.Future batchEnableAlarmAsync(BatchEnableAlarmRequest batchEnableAlarmRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Sends a set of messages to the IoT Events system. Each message payload is transformed into the input you specify
* ("inputName"
) and ingested into any detectors that monitor that input. If multiple messages are
* sent, the order in which the messages are processed isn't guaranteed. To guarantee ordering, you must send
* messages one at a time and wait for a successful response.
*
*
* @param batchPutMessageRequest
* @return A Java Future containing the result of the BatchPutMessage operation returned by the service.
* @sample AWSIoTEventsDataAsync.BatchPutMessage
* @see AWS
* API Documentation
*/
java.util.concurrent.Future batchPutMessageAsync(BatchPutMessageRequest batchPutMessageRequest);
/**
*
* Sends a set of messages to the IoT Events system. Each message payload is transformed into the input you specify
* ("inputName"
) and ingested into any detectors that monitor that input. If multiple messages are
* sent, the order in which the messages are processed isn't guaranteed. To guarantee ordering, you must send
* messages one at a time and wait for a successful response.
*
*
* @param batchPutMessageRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the BatchPutMessage operation returned by the service.
* @sample AWSIoTEventsDataAsyncHandler.BatchPutMessage
* @see AWS
* API Documentation
*/
java.util.concurrent.Future batchPutMessageAsync(BatchPutMessageRequest batchPutMessageRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Resets one or more alarms. The alarms return to the NORMAL
state after you reset them.
*
*
* @param batchResetAlarmRequest
* @return A Java Future containing the result of the BatchResetAlarm operation returned by the service.
* @sample AWSIoTEventsDataAsync.BatchResetAlarm
* @see AWS
* API Documentation
*/
java.util.concurrent.Future batchResetAlarmAsync(BatchResetAlarmRequest batchResetAlarmRequest);
/**
*
* Resets one or more alarms. The alarms return to the NORMAL
state after you reset them.
*
*
* @param batchResetAlarmRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the BatchResetAlarm operation returned by the service.
* @sample AWSIoTEventsDataAsyncHandler.BatchResetAlarm
* @see AWS
* API Documentation
*/
java.util.concurrent.Future batchResetAlarmAsync(BatchResetAlarmRequest batchResetAlarmRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Changes one or more alarms to the snooze mode. The alarms change to the SNOOZE_DISABLED
state after
* you set them to the snooze mode.
*
*
* @param batchSnoozeAlarmRequest
* @return A Java Future containing the result of the BatchSnoozeAlarm operation returned by the service.
* @sample AWSIoTEventsDataAsync.BatchSnoozeAlarm
* @see AWS API Documentation
*/
java.util.concurrent.Future batchSnoozeAlarmAsync(BatchSnoozeAlarmRequest batchSnoozeAlarmRequest);
/**
*
* Changes one or more alarms to the snooze mode. The alarms change to the SNOOZE_DISABLED
state after
* you set them to the snooze mode.
*
*
* @param batchSnoozeAlarmRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the BatchSnoozeAlarm operation returned by the service.
* @sample AWSIoTEventsDataAsyncHandler.BatchSnoozeAlarm
* @see AWS API Documentation
*/
java.util.concurrent.Future batchSnoozeAlarmAsync(BatchSnoozeAlarmRequest batchSnoozeAlarmRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates the state, variable values, and timer settings of one or more detectors (instances) of a specified
* detector model.
*
*
* @param batchUpdateDetectorRequest
* @return A Java Future containing the result of the BatchUpdateDetector operation returned by the service.
* @sample AWSIoTEventsDataAsync.BatchUpdateDetector
* @see AWS API Documentation
*/
java.util.concurrent.Future batchUpdateDetectorAsync(BatchUpdateDetectorRequest batchUpdateDetectorRequest);
/**
*
* Updates the state, variable values, and timer settings of one or more detectors (instances) of a specified
* detector model.
*
*
* @param batchUpdateDetectorRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the BatchUpdateDetector operation returned by the service.
* @sample AWSIoTEventsDataAsyncHandler.BatchUpdateDetector
* @see AWS API Documentation
*/
java.util.concurrent.Future batchUpdateDetectorAsync(BatchUpdateDetectorRequest batchUpdateDetectorRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves information about an alarm.
*
*
* @param describeAlarmRequest
* @return A Java Future containing the result of the DescribeAlarm operation returned by the service.
* @sample AWSIoTEventsDataAsync.DescribeAlarm
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeAlarmAsync(DescribeAlarmRequest describeAlarmRequest);
/**
*
* Retrieves information about an alarm.
*
*
* @param describeAlarmRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeAlarm operation returned by the service.
* @sample AWSIoTEventsDataAsyncHandler.DescribeAlarm
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeAlarmAsync(DescribeAlarmRequest describeAlarmRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns information about the specified detector (instance).
*
*
* @param describeDetectorRequest
* @return A Java Future containing the result of the DescribeDetector operation returned by the service.
* @sample AWSIoTEventsDataAsync.DescribeDetector
* @see AWS API Documentation
*/
java.util.concurrent.Future describeDetectorAsync(DescribeDetectorRequest describeDetectorRequest);
/**
*
* Returns information about the specified detector (instance).
*
*
* @param describeDetectorRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeDetector operation returned by the service.
* @sample AWSIoTEventsDataAsyncHandler.DescribeDetector
* @see AWS API Documentation
*/
java.util.concurrent.Future describeDetectorAsync(DescribeDetectorRequest describeDetectorRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists one or more alarms. The operation returns only the metadata associated with each alarm.
*
*
* @param listAlarmsRequest
* @return A Java Future containing the result of the ListAlarms operation returned by the service.
* @sample AWSIoTEventsDataAsync.ListAlarms
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listAlarmsAsync(ListAlarmsRequest listAlarmsRequest);
/**
*
* Lists one or more alarms. The operation returns only the metadata associated with each alarm.
*
*
* @param listAlarmsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListAlarms operation returned by the service.
* @sample AWSIoTEventsDataAsyncHandler.ListAlarms
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listAlarmsAsync(ListAlarmsRequest listAlarmsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists detectors (the instances of a detector model).
*
*
* @param listDetectorsRequest
* @return A Java Future containing the result of the ListDetectors operation returned by the service.
* @sample AWSIoTEventsDataAsync.ListDetectors
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listDetectorsAsync(ListDetectorsRequest listDetectorsRequest);
/**
*
* Lists detectors (the instances of a detector model).
*
*
* @param listDetectorsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListDetectors operation returned by the service.
* @sample AWSIoTEventsDataAsyncHandler.ListDetectors
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listDetectorsAsync(ListDetectorsRequest listDetectorsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
}