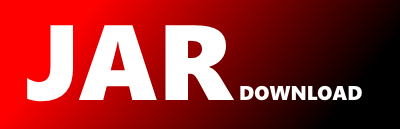
com.amazonaws.services.iotthingsgraph.AWSIoTThingsGraphAsync Maven / Gradle / Ivy
Show all versions of aws-java-sdk-iotthingsgraph Show documentation
/*
* Copyright 2015-2020 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.iotthingsgraph;
import javax.annotation.Generated;
import com.amazonaws.services.iotthingsgraph.model.*;
/**
* Interface for accessing AWS IoT Things Graph asynchronously. Each asynchronous method will return a Java Future
* object representing the asynchronous operation; overloads which accept an {@code AsyncHandler} can be used to receive
* notification when an asynchronous operation completes.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.iotthingsgraph.AbstractAWSIoTThingsGraphAsync} instead.
*
*
* AWS IoT Things Graph
*
* AWS IoT Things Graph provides an integrated set of tools that enable developers to connect devices and services that
* use different standards, such as units of measure and communication protocols. AWS IoT Things Graph makes it possible
* to build IoT applications with little to no code by connecting devices and services and defining how they interact at
* an abstract level.
*
*
* For more information about how AWS IoT Things Graph works, see the User Guide.
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AWSIoTThingsGraphAsync extends AWSIoTThingsGraph {
/**
*
* Associates a device with a concrete thing that is in the user's registry.
*
*
* A thing can be associated with only one device at a time. If you associate a thing with a new device id, its
* previous association will be removed.
*
*
* @param associateEntityToThingRequest
* @return A Java Future containing the result of the AssociateEntityToThing operation returned by the service.
* @sample AWSIoTThingsGraphAsync.AssociateEntityToThing
* @see AWS API Documentation
*/
java.util.concurrent.Future associateEntityToThingAsync(AssociateEntityToThingRequest associateEntityToThingRequest);
/**
*
* Associates a device with a concrete thing that is in the user's registry.
*
*
* A thing can be associated with only one device at a time. If you associate a thing with a new device id, its
* previous association will be removed.
*
*
* @param associateEntityToThingRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AssociateEntityToThing operation returned by the service.
* @sample AWSIoTThingsGraphAsyncHandler.AssociateEntityToThing
* @see AWS API Documentation
*/
java.util.concurrent.Future associateEntityToThingAsync(AssociateEntityToThingRequest associateEntityToThingRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a workflow template. Workflows can be created only in the user's namespace. (The public namespace
* contains only entities.) The workflow can contain only entities in the specified namespace. The workflow is
* validated against the entities in the latest version of the user's namespace unless another namespace version is
* specified in the request.
*
*
* @param createFlowTemplateRequest
* @return A Java Future containing the result of the CreateFlowTemplate operation returned by the service.
* @sample AWSIoTThingsGraphAsync.CreateFlowTemplate
* @see AWS API Documentation
*/
java.util.concurrent.Future createFlowTemplateAsync(CreateFlowTemplateRequest createFlowTemplateRequest);
/**
*
* Creates a workflow template. Workflows can be created only in the user's namespace. (The public namespace
* contains only entities.) The workflow can contain only entities in the specified namespace. The workflow is
* validated against the entities in the latest version of the user's namespace unless another namespace version is
* specified in the request.
*
*
* @param createFlowTemplateRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateFlowTemplate operation returned by the service.
* @sample AWSIoTThingsGraphAsyncHandler.CreateFlowTemplate
* @see AWS API Documentation
*/
java.util.concurrent.Future createFlowTemplateAsync(CreateFlowTemplateRequest createFlowTemplateRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a system instance.
*
*
* This action validates the system instance, prepares the deployment-related resources. For Greengrass deployments,
* it updates the Greengrass group that is specified by the greengrassGroupName
parameter. It also adds
* a file to the S3 bucket specified by the s3BucketName
parameter. You need to call
* DeploySystemInstance
after running this action.
*
*
* For Greengrass deployments, since this action modifies and adds resources to a Greengrass group and an S3 bucket
* on the caller's behalf, the calling identity must have write permissions to both the specified Greengrass group
* and S3 bucket. Otherwise, the call will fail with an authorization error.
*
*
* For cloud deployments, this action requires a flowActionsRoleArn
value. This is an IAM role that has
* permissions to access AWS services, such as AWS Lambda and AWS IoT, that the flow uses when it executes.
*
*
* If the definition document doesn't specify a version of the user's namespace, the latest version will be used by
* default.
*
*
* @param createSystemInstanceRequest
* @return A Java Future containing the result of the CreateSystemInstance operation returned by the service.
* @sample AWSIoTThingsGraphAsync.CreateSystemInstance
* @see AWS API Documentation
*/
java.util.concurrent.Future createSystemInstanceAsync(CreateSystemInstanceRequest createSystemInstanceRequest);
/**
*
* Creates a system instance.
*
*
* This action validates the system instance, prepares the deployment-related resources. For Greengrass deployments,
* it updates the Greengrass group that is specified by the greengrassGroupName
parameter. It also adds
* a file to the S3 bucket specified by the s3BucketName
parameter. You need to call
* DeploySystemInstance
after running this action.
*
*
* For Greengrass deployments, since this action modifies and adds resources to a Greengrass group and an S3 bucket
* on the caller's behalf, the calling identity must have write permissions to both the specified Greengrass group
* and S3 bucket. Otherwise, the call will fail with an authorization error.
*
*
* For cloud deployments, this action requires a flowActionsRoleArn
value. This is an IAM role that has
* permissions to access AWS services, such as AWS Lambda and AWS IoT, that the flow uses when it executes.
*
*
* If the definition document doesn't specify a version of the user's namespace, the latest version will be used by
* default.
*
*
* @param createSystemInstanceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateSystemInstance operation returned by the service.
* @sample AWSIoTThingsGraphAsyncHandler.CreateSystemInstance
* @see AWS API Documentation
*/
java.util.concurrent.Future createSystemInstanceAsync(CreateSystemInstanceRequest createSystemInstanceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a system. The system is validated against the entities in the latest version of the user's namespace
* unless another namespace version is specified in the request.
*
*
* @param createSystemTemplateRequest
* @return A Java Future containing the result of the CreateSystemTemplate operation returned by the service.
* @sample AWSIoTThingsGraphAsync.CreateSystemTemplate
* @see AWS API Documentation
*/
java.util.concurrent.Future createSystemTemplateAsync(CreateSystemTemplateRequest createSystemTemplateRequest);
/**
*
* Creates a system. The system is validated against the entities in the latest version of the user's namespace
* unless another namespace version is specified in the request.
*
*
* @param createSystemTemplateRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateSystemTemplate operation returned by the service.
* @sample AWSIoTThingsGraphAsyncHandler.CreateSystemTemplate
* @see AWS API Documentation
*/
java.util.concurrent.Future createSystemTemplateAsync(CreateSystemTemplateRequest createSystemTemplateRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a workflow. Any new system or deployment that contains this workflow will fail to update or deploy.
* Existing deployments that contain the workflow will continue to run (since they use a snapshot of the workflow
* taken at the time of deployment).
*
*
* @param deleteFlowTemplateRequest
* @return A Java Future containing the result of the DeleteFlowTemplate operation returned by the service.
* @sample AWSIoTThingsGraphAsync.DeleteFlowTemplate
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteFlowTemplateAsync(DeleteFlowTemplateRequest deleteFlowTemplateRequest);
/**
*
* Deletes a workflow. Any new system or deployment that contains this workflow will fail to update or deploy.
* Existing deployments that contain the workflow will continue to run (since they use a snapshot of the workflow
* taken at the time of deployment).
*
*
* @param deleteFlowTemplateRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteFlowTemplate operation returned by the service.
* @sample AWSIoTThingsGraphAsyncHandler.DeleteFlowTemplate
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteFlowTemplateAsync(DeleteFlowTemplateRequest deleteFlowTemplateRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the specified namespace. This action deletes all of the entities in the namespace. Delete the systems and
* flows that use entities in the namespace before performing this action.
*
*
* @param deleteNamespaceRequest
* @return A Java Future containing the result of the DeleteNamespace operation returned by the service.
* @sample AWSIoTThingsGraphAsync.DeleteNamespace
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteNamespaceAsync(DeleteNamespaceRequest deleteNamespaceRequest);
/**
*
* Deletes the specified namespace. This action deletes all of the entities in the namespace. Delete the systems and
* flows that use entities in the namespace before performing this action.
*
*
* @param deleteNamespaceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteNamespace operation returned by the service.
* @sample AWSIoTThingsGraphAsyncHandler.DeleteNamespace
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteNamespaceAsync(DeleteNamespaceRequest deleteNamespaceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a system instance. Only system instances that have never been deployed, or that have been undeployed can
* be deleted.
*
*
* Users can create a new system instance that has the same ID as a deleted system instance.
*
*
* @param deleteSystemInstanceRequest
* @return A Java Future containing the result of the DeleteSystemInstance operation returned by the service.
* @sample AWSIoTThingsGraphAsync.DeleteSystemInstance
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteSystemInstanceAsync(DeleteSystemInstanceRequest deleteSystemInstanceRequest);
/**
*
* Deletes a system instance. Only system instances that have never been deployed, or that have been undeployed can
* be deleted.
*
*
* Users can create a new system instance that has the same ID as a deleted system instance.
*
*
* @param deleteSystemInstanceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteSystemInstance operation returned by the service.
* @sample AWSIoTThingsGraphAsyncHandler.DeleteSystemInstance
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteSystemInstanceAsync(DeleteSystemInstanceRequest deleteSystemInstanceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a system. New deployments can't contain the system after its deletion. Existing deployments that contain
* the system will continue to work because they use a snapshot of the system that is taken when it is deployed.
*
*
* @param deleteSystemTemplateRequest
* @return A Java Future containing the result of the DeleteSystemTemplate operation returned by the service.
* @sample AWSIoTThingsGraphAsync.DeleteSystemTemplate
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteSystemTemplateAsync(DeleteSystemTemplateRequest deleteSystemTemplateRequest);
/**
*
* Deletes a system. New deployments can't contain the system after its deletion. Existing deployments that contain
* the system will continue to work because they use a snapshot of the system that is taken when it is deployed.
*
*
* @param deleteSystemTemplateRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteSystemTemplate operation returned by the service.
* @sample AWSIoTThingsGraphAsyncHandler.DeleteSystemTemplate
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteSystemTemplateAsync(DeleteSystemTemplateRequest deleteSystemTemplateRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Greengrass and Cloud Deployments
*
*
* Deploys the system instance to the target specified in CreateSystemInstance
.
*
*
* Greengrass Deployments
*
*
* If the system or any workflows and entities have been updated before this action is called, then the deployment
* will create a new Amazon Simple Storage Service resource file and then deploy it.
*
*
* Since this action creates a Greengrass deployment on the caller's behalf, the calling identity must have write
* permissions to the specified Greengrass group. Otherwise, the call will fail with an authorization error.
*
*
* For information about the artifacts that get added to your Greengrass core device when you use this API, see AWS IoT Things Graph and AWS IoT
* Greengrass.
*
*
* @param deploySystemInstanceRequest
* @return A Java Future containing the result of the DeploySystemInstance operation returned by the service.
* @sample AWSIoTThingsGraphAsync.DeploySystemInstance
* @see AWS API Documentation
*/
java.util.concurrent.Future deploySystemInstanceAsync(DeploySystemInstanceRequest deploySystemInstanceRequest);
/**
*
* Greengrass and Cloud Deployments
*
*
* Deploys the system instance to the target specified in CreateSystemInstance
.
*
*
* Greengrass Deployments
*
*
* If the system or any workflows and entities have been updated before this action is called, then the deployment
* will create a new Amazon Simple Storage Service resource file and then deploy it.
*
*
* Since this action creates a Greengrass deployment on the caller's behalf, the calling identity must have write
* permissions to the specified Greengrass group. Otherwise, the call will fail with an authorization error.
*
*
* For information about the artifacts that get added to your Greengrass core device when you use this API, see AWS IoT Things Graph and AWS IoT
* Greengrass.
*
*
* @param deploySystemInstanceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeploySystemInstance operation returned by the service.
* @sample AWSIoTThingsGraphAsyncHandler.DeploySystemInstance
* @see AWS API Documentation
*/
java.util.concurrent.Future deploySystemInstanceAsync(DeploySystemInstanceRequest deploySystemInstanceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deprecates the specified workflow. This action marks the workflow for deletion. Deprecated flows can't be
* deployed, but existing deployments will continue to run.
*
*
* @param deprecateFlowTemplateRequest
* @return A Java Future containing the result of the DeprecateFlowTemplate operation returned by the service.
* @sample AWSIoTThingsGraphAsync.DeprecateFlowTemplate
* @see AWS API Documentation
*/
java.util.concurrent.Future deprecateFlowTemplateAsync(DeprecateFlowTemplateRequest deprecateFlowTemplateRequest);
/**
*
* Deprecates the specified workflow. This action marks the workflow for deletion. Deprecated flows can't be
* deployed, but existing deployments will continue to run.
*
*
* @param deprecateFlowTemplateRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeprecateFlowTemplate operation returned by the service.
* @sample AWSIoTThingsGraphAsyncHandler.DeprecateFlowTemplate
* @see AWS API Documentation
*/
java.util.concurrent.Future deprecateFlowTemplateAsync(DeprecateFlowTemplateRequest deprecateFlowTemplateRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deprecates the specified system.
*
*
* @param deprecateSystemTemplateRequest
* @return A Java Future containing the result of the DeprecateSystemTemplate operation returned by the service.
* @sample AWSIoTThingsGraphAsync.DeprecateSystemTemplate
* @see AWS API Documentation
*/
java.util.concurrent.Future deprecateSystemTemplateAsync(DeprecateSystemTemplateRequest deprecateSystemTemplateRequest);
/**
*
* Deprecates the specified system.
*
*
* @param deprecateSystemTemplateRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeprecateSystemTemplate operation returned by the service.
* @sample AWSIoTThingsGraphAsyncHandler.DeprecateSystemTemplate
* @see AWS API Documentation
*/
java.util.concurrent.Future deprecateSystemTemplateAsync(DeprecateSystemTemplateRequest deprecateSystemTemplateRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets the latest version of the user's namespace and the public version that it is tracking.
*
*
* @param describeNamespaceRequest
* @return A Java Future containing the result of the DescribeNamespace operation returned by the service.
* @sample AWSIoTThingsGraphAsync.DescribeNamespace
* @see AWS API Documentation
*/
java.util.concurrent.Future describeNamespaceAsync(DescribeNamespaceRequest describeNamespaceRequest);
/**
*
* Gets the latest version of the user's namespace and the public version that it is tracking.
*
*
* @param describeNamespaceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeNamespace operation returned by the service.
* @sample AWSIoTThingsGraphAsyncHandler.DescribeNamespace
* @see AWS API Documentation
*/
java.util.concurrent.Future describeNamespaceAsync(DescribeNamespaceRequest describeNamespaceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Dissociates a device entity from a concrete thing. The action takes only the type of the entity that you need to
* dissociate because only one entity of a particular type can be associated with a thing.
*
*
* @param dissociateEntityFromThingRequest
* @return A Java Future containing the result of the DissociateEntityFromThing operation returned by the service.
* @sample AWSIoTThingsGraphAsync.DissociateEntityFromThing
* @see AWS API Documentation
*/
java.util.concurrent.Future dissociateEntityFromThingAsync(
DissociateEntityFromThingRequest dissociateEntityFromThingRequest);
/**
*
* Dissociates a device entity from a concrete thing. The action takes only the type of the entity that you need to
* dissociate because only one entity of a particular type can be associated with a thing.
*
*
* @param dissociateEntityFromThingRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DissociateEntityFromThing operation returned by the service.
* @sample AWSIoTThingsGraphAsyncHandler.DissociateEntityFromThing
* @see AWS API Documentation
*/
java.util.concurrent.Future dissociateEntityFromThingAsync(
DissociateEntityFromThingRequest dissociateEntityFromThingRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets definitions of the specified entities. Uses the latest version of the user's namespace by default. This API
* returns the following TDM entities.
*
*
* -
*
* Properties
*
*
* -
*
* States
*
*
* -
*
* Events
*
*
* -
*
* Actions
*
*
* -
*
* Capabilities
*
*
* -
*
* Mappings
*
*
* -
*
* Devices
*
*
* -
*
* Device Models
*
*
* -
*
* Services
*
*
*
*
* This action doesn't return definitions for systems, flows, and deployments.
*
*
* @param getEntitiesRequest
* @return A Java Future containing the result of the GetEntities operation returned by the service.
* @sample AWSIoTThingsGraphAsync.GetEntities
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getEntitiesAsync(GetEntitiesRequest getEntitiesRequest);
/**
*
* Gets definitions of the specified entities. Uses the latest version of the user's namespace by default. This API
* returns the following TDM entities.
*
*
* -
*
* Properties
*
*
* -
*
* States
*
*
* -
*
* Events
*
*
* -
*
* Actions
*
*
* -
*
* Capabilities
*
*
* -
*
* Mappings
*
*
* -
*
* Devices
*
*
* -
*
* Device Models
*
*
* -
*
* Services
*
*
*
*
* This action doesn't return definitions for systems, flows, and deployments.
*
*
* @param getEntitiesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetEntities operation returned by the service.
* @sample AWSIoTThingsGraphAsyncHandler.GetEntities
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getEntitiesAsync(GetEntitiesRequest getEntitiesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets the latest version of the DefinitionDocument
and FlowTemplateSummary
for the
* specified workflow.
*
*
* @param getFlowTemplateRequest
* @return A Java Future containing the result of the GetFlowTemplate operation returned by the service.
* @sample AWSIoTThingsGraphAsync.GetFlowTemplate
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getFlowTemplateAsync(GetFlowTemplateRequest getFlowTemplateRequest);
/**
*
* Gets the latest version of the DefinitionDocument
and FlowTemplateSummary
for the
* specified workflow.
*
*
* @param getFlowTemplateRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetFlowTemplate operation returned by the service.
* @sample AWSIoTThingsGraphAsyncHandler.GetFlowTemplate
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getFlowTemplateAsync(GetFlowTemplateRequest getFlowTemplateRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets revisions of the specified workflow. Only the last 100 revisions are stored. If the workflow has been
* deprecated, this action will return revisions that occurred before the deprecation. This action won't work for
* workflows that have been deleted.
*
*
* @param getFlowTemplateRevisionsRequest
* @return A Java Future containing the result of the GetFlowTemplateRevisions operation returned by the service.
* @sample AWSIoTThingsGraphAsync.GetFlowTemplateRevisions
* @see AWS API Documentation
*/
java.util.concurrent.Future getFlowTemplateRevisionsAsync(GetFlowTemplateRevisionsRequest getFlowTemplateRevisionsRequest);
/**
*
* Gets revisions of the specified workflow. Only the last 100 revisions are stored. If the workflow has been
* deprecated, this action will return revisions that occurred before the deprecation. This action won't work for
* workflows that have been deleted.
*
*
* @param getFlowTemplateRevisionsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetFlowTemplateRevisions operation returned by the service.
* @sample AWSIoTThingsGraphAsyncHandler.GetFlowTemplateRevisions
* @see AWS API Documentation
*/
java.util.concurrent.Future getFlowTemplateRevisionsAsync(GetFlowTemplateRevisionsRequest getFlowTemplateRevisionsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets the status of a namespace deletion task.
*
*
* @param getNamespaceDeletionStatusRequest
* @return A Java Future containing the result of the GetNamespaceDeletionStatus operation returned by the service.
* @sample AWSIoTThingsGraphAsync.GetNamespaceDeletionStatus
* @see AWS API Documentation
*/
java.util.concurrent.Future getNamespaceDeletionStatusAsync(
GetNamespaceDeletionStatusRequest getNamespaceDeletionStatusRequest);
/**
*
* Gets the status of a namespace deletion task.
*
*
* @param getNamespaceDeletionStatusRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetNamespaceDeletionStatus operation returned by the service.
* @sample AWSIoTThingsGraphAsyncHandler.GetNamespaceDeletionStatus
* @see AWS API Documentation
*/
java.util.concurrent.Future getNamespaceDeletionStatusAsync(
GetNamespaceDeletionStatusRequest getNamespaceDeletionStatusRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets a system instance.
*
*
* @param getSystemInstanceRequest
* @return A Java Future containing the result of the GetSystemInstance operation returned by the service.
* @sample AWSIoTThingsGraphAsync.GetSystemInstance
* @see AWS API Documentation
*/
java.util.concurrent.Future getSystemInstanceAsync(GetSystemInstanceRequest getSystemInstanceRequest);
/**
*
* Gets a system instance.
*
*
* @param getSystemInstanceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetSystemInstance operation returned by the service.
* @sample AWSIoTThingsGraphAsyncHandler.GetSystemInstance
* @see AWS API Documentation
*/
java.util.concurrent.Future getSystemInstanceAsync(GetSystemInstanceRequest getSystemInstanceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets a system.
*
*
* @param getSystemTemplateRequest
* @return A Java Future containing the result of the GetSystemTemplate operation returned by the service.
* @sample AWSIoTThingsGraphAsync.GetSystemTemplate
* @see AWS API Documentation
*/
java.util.concurrent.Future getSystemTemplateAsync(GetSystemTemplateRequest getSystemTemplateRequest);
/**
*
* Gets a system.
*
*
* @param getSystemTemplateRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetSystemTemplate operation returned by the service.
* @sample AWSIoTThingsGraphAsyncHandler.GetSystemTemplate
* @see AWS API Documentation
*/
java.util.concurrent.Future getSystemTemplateAsync(GetSystemTemplateRequest getSystemTemplateRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets revisions made to the specified system template. Only the previous 100 revisions are stored. If the system
* has been deprecated, this action will return the revisions that occurred before its deprecation. This action
* won't work with systems that have been deleted.
*
*
* @param getSystemTemplateRevisionsRequest
* @return A Java Future containing the result of the GetSystemTemplateRevisions operation returned by the service.
* @sample AWSIoTThingsGraphAsync.GetSystemTemplateRevisions
* @see AWS API Documentation
*/
java.util.concurrent.Future getSystemTemplateRevisionsAsync(
GetSystemTemplateRevisionsRequest getSystemTemplateRevisionsRequest);
/**
*
* Gets revisions made to the specified system template. Only the previous 100 revisions are stored. If the system
* has been deprecated, this action will return the revisions that occurred before its deprecation. This action
* won't work with systems that have been deleted.
*
*
* @param getSystemTemplateRevisionsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetSystemTemplateRevisions operation returned by the service.
* @sample AWSIoTThingsGraphAsyncHandler.GetSystemTemplateRevisions
* @see AWS API Documentation
*/
java.util.concurrent.Future getSystemTemplateRevisionsAsync(
GetSystemTemplateRevisionsRequest getSystemTemplateRevisionsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets the status of the specified upload.
*
*
* @param getUploadStatusRequest
* @return A Java Future containing the result of the GetUploadStatus operation returned by the service.
* @sample AWSIoTThingsGraphAsync.GetUploadStatus
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getUploadStatusAsync(GetUploadStatusRequest getUploadStatusRequest);
/**
*
* Gets the status of the specified upload.
*
*
* @param getUploadStatusRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetUploadStatus operation returned by the service.
* @sample AWSIoTThingsGraphAsyncHandler.GetUploadStatus
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getUploadStatusAsync(GetUploadStatusRequest getUploadStatusRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of objects that contain information about events in a flow execution.
*
*
* @param listFlowExecutionMessagesRequest
* @return A Java Future containing the result of the ListFlowExecutionMessages operation returned by the service.
* @sample AWSIoTThingsGraphAsync.ListFlowExecutionMessages
* @see AWS API Documentation
*/
java.util.concurrent.Future listFlowExecutionMessagesAsync(
ListFlowExecutionMessagesRequest listFlowExecutionMessagesRequest);
/**
*
* Returns a list of objects that contain information about events in a flow execution.
*
*
* @param listFlowExecutionMessagesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListFlowExecutionMessages operation returned by the service.
* @sample AWSIoTThingsGraphAsyncHandler.ListFlowExecutionMessages
* @see AWS API Documentation
*/
java.util.concurrent.Future listFlowExecutionMessagesAsync(
ListFlowExecutionMessagesRequest listFlowExecutionMessagesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists all tags on an AWS IoT Things Graph resource.
*
*
* @param listTagsForResourceRequest
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* @sample AWSIoTThingsGraphAsync.ListTagsForResource
* @see AWS API Documentation
*/
java.util.concurrent.Future listTagsForResourceAsync(ListTagsForResourceRequest listTagsForResourceRequest);
/**
*
* Lists all tags on an AWS IoT Things Graph resource.
*
*
* @param listTagsForResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* @sample AWSIoTThingsGraphAsyncHandler.ListTagsForResource
* @see AWS API Documentation
*/
java.util.concurrent.Future listTagsForResourceAsync(ListTagsForResourceRequest listTagsForResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Searches for entities of the specified type. You can search for entities in your namespace and the public
* namespace that you're tracking.
*
*
* @param searchEntitiesRequest
* @return A Java Future containing the result of the SearchEntities operation returned by the service.
* @sample AWSIoTThingsGraphAsync.SearchEntities
* @see AWS
* API Documentation
*/
java.util.concurrent.Future searchEntitiesAsync(SearchEntitiesRequest searchEntitiesRequest);
/**
*
* Searches for entities of the specified type. You can search for entities in your namespace and the public
* namespace that you're tracking.
*
*
* @param searchEntitiesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the SearchEntities operation returned by the service.
* @sample AWSIoTThingsGraphAsyncHandler.SearchEntities
* @see AWS
* API Documentation
*/
java.util.concurrent.Future searchEntitiesAsync(SearchEntitiesRequest searchEntitiesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Searches for AWS IoT Things Graph workflow execution instances.
*
*
* @param searchFlowExecutionsRequest
* @return A Java Future containing the result of the SearchFlowExecutions operation returned by the service.
* @sample AWSIoTThingsGraphAsync.SearchFlowExecutions
* @see AWS API Documentation
*/
java.util.concurrent.Future searchFlowExecutionsAsync(SearchFlowExecutionsRequest searchFlowExecutionsRequest);
/**
*
* Searches for AWS IoT Things Graph workflow execution instances.
*
*
* @param searchFlowExecutionsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the SearchFlowExecutions operation returned by the service.
* @sample AWSIoTThingsGraphAsyncHandler.SearchFlowExecutions
* @see AWS API Documentation
*/
java.util.concurrent.Future searchFlowExecutionsAsync(SearchFlowExecutionsRequest searchFlowExecutionsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Searches for summary information about workflows.
*
*
* @param searchFlowTemplatesRequest
* @return A Java Future containing the result of the SearchFlowTemplates operation returned by the service.
* @sample AWSIoTThingsGraphAsync.SearchFlowTemplates
* @see AWS API Documentation
*/
java.util.concurrent.Future searchFlowTemplatesAsync(SearchFlowTemplatesRequest searchFlowTemplatesRequest);
/**
*
* Searches for summary information about workflows.
*
*
* @param searchFlowTemplatesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the SearchFlowTemplates operation returned by the service.
* @sample AWSIoTThingsGraphAsyncHandler.SearchFlowTemplates
* @see AWS API Documentation
*/
java.util.concurrent.Future searchFlowTemplatesAsync(SearchFlowTemplatesRequest searchFlowTemplatesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Searches for system instances in the user's account.
*
*
* @param searchSystemInstancesRequest
* @return A Java Future containing the result of the SearchSystemInstances operation returned by the service.
* @sample AWSIoTThingsGraphAsync.SearchSystemInstances
* @see AWS API Documentation
*/
java.util.concurrent.Future searchSystemInstancesAsync(SearchSystemInstancesRequest searchSystemInstancesRequest);
/**
*
* Searches for system instances in the user's account.
*
*
* @param searchSystemInstancesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the SearchSystemInstances operation returned by the service.
* @sample AWSIoTThingsGraphAsyncHandler.SearchSystemInstances
* @see AWS API Documentation
*/
java.util.concurrent.Future searchSystemInstancesAsync(SearchSystemInstancesRequest searchSystemInstancesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Searches for summary information about systems in the user's account. You can filter by the ID of a workflow to
* return only systems that use the specified workflow.
*
*
* @param searchSystemTemplatesRequest
* @return A Java Future containing the result of the SearchSystemTemplates operation returned by the service.
* @sample AWSIoTThingsGraphAsync.SearchSystemTemplates
* @see AWS API Documentation
*/
java.util.concurrent.Future searchSystemTemplatesAsync(SearchSystemTemplatesRequest searchSystemTemplatesRequest);
/**
*
* Searches for summary information about systems in the user's account. You can filter by the ID of a workflow to
* return only systems that use the specified workflow.
*
*
* @param searchSystemTemplatesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the SearchSystemTemplates operation returned by the service.
* @sample AWSIoTThingsGraphAsyncHandler.SearchSystemTemplates
* @see AWS API Documentation
*/
java.util.concurrent.Future searchSystemTemplatesAsync(SearchSystemTemplatesRequest searchSystemTemplatesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Searches for things associated with the specified entity. You can search by both device and device model.
*
*
* For example, if two different devices, camera1 and camera2, implement the camera device model, the user can
* associate thing1 to camera1 and thing2 to camera2. SearchThings(camera2)
will return only thing2,
* but SearchThings(camera)
will return both thing1 and thing2.
*
*
* This action searches for exact matches and doesn't perform partial text matching.
*
*
* @param searchThingsRequest
* @return A Java Future containing the result of the SearchThings operation returned by the service.
* @sample AWSIoTThingsGraphAsync.SearchThings
* @see AWS
* API Documentation
*/
java.util.concurrent.Future searchThingsAsync(SearchThingsRequest searchThingsRequest);
/**
*
* Searches for things associated with the specified entity. You can search by both device and device model.
*
*
* For example, if two different devices, camera1 and camera2, implement the camera device model, the user can
* associate thing1 to camera1 and thing2 to camera2. SearchThings(camera2)
will return only thing2,
* but SearchThings(camera)
will return both thing1 and thing2.
*
*
* This action searches for exact matches and doesn't perform partial text matching.
*
*
* @param searchThingsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the SearchThings operation returned by the service.
* @sample AWSIoTThingsGraphAsyncHandler.SearchThings
* @see AWS
* API Documentation
*/
java.util.concurrent.Future searchThingsAsync(SearchThingsRequest searchThingsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a tag for the specified resource.
*
*
* @param tagResourceRequest
* @return A Java Future containing the result of the TagResource operation returned by the service.
* @sample AWSIoTThingsGraphAsync.TagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future tagResourceAsync(TagResourceRequest tagResourceRequest);
/**
*
* Creates a tag for the specified resource.
*
*
* @param tagResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the TagResource operation returned by the service.
* @sample AWSIoTThingsGraphAsyncHandler.TagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future tagResourceAsync(TagResourceRequest tagResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Removes a system instance from its target (Cloud or Greengrass).
*
*
* @param undeploySystemInstanceRequest
* @return A Java Future containing the result of the UndeploySystemInstance operation returned by the service.
* @sample AWSIoTThingsGraphAsync.UndeploySystemInstance
* @see AWS API Documentation
*/
java.util.concurrent.Future undeploySystemInstanceAsync(UndeploySystemInstanceRequest undeploySystemInstanceRequest);
/**
*
* Removes a system instance from its target (Cloud or Greengrass).
*
*
* @param undeploySystemInstanceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UndeploySystemInstance operation returned by the service.
* @sample AWSIoTThingsGraphAsyncHandler.UndeploySystemInstance
* @see AWS API Documentation
*/
java.util.concurrent.Future undeploySystemInstanceAsync(UndeploySystemInstanceRequest undeploySystemInstanceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Removes a tag from the specified resource.
*
*
* @param untagResourceRequest
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* @sample AWSIoTThingsGraphAsync.UntagResource
* @see AWS
* API Documentation
*/
java.util.concurrent.Future untagResourceAsync(UntagResourceRequest untagResourceRequest);
/**
*
* Removes a tag from the specified resource.
*
*
* @param untagResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* @sample AWSIoTThingsGraphAsyncHandler.UntagResource
* @see AWS
* API Documentation
*/
java.util.concurrent.Future untagResourceAsync(UntagResourceRequest untagResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates the specified workflow. All deployed systems and system instances that use the workflow will see the
* changes in the flow when it is redeployed. If you don't want this behavior, copy the workflow (creating a new
* workflow with a different ID), and update the copy. The workflow can contain only entities in the specified
* namespace.
*
*
* @param updateFlowTemplateRequest
* @return A Java Future containing the result of the UpdateFlowTemplate operation returned by the service.
* @sample AWSIoTThingsGraphAsync.UpdateFlowTemplate
* @see AWS API Documentation
*/
java.util.concurrent.Future updateFlowTemplateAsync(UpdateFlowTemplateRequest updateFlowTemplateRequest);
/**
*
* Updates the specified workflow. All deployed systems and system instances that use the workflow will see the
* changes in the flow when it is redeployed. If you don't want this behavior, copy the workflow (creating a new
* workflow with a different ID), and update the copy. The workflow can contain only entities in the specified
* namespace.
*
*
* @param updateFlowTemplateRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateFlowTemplate operation returned by the service.
* @sample AWSIoTThingsGraphAsyncHandler.UpdateFlowTemplate
* @see AWS API Documentation
*/
java.util.concurrent.Future updateFlowTemplateAsync(UpdateFlowTemplateRequest updateFlowTemplateRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates the specified system. You don't need to run this action after updating a workflow. Any deployment that
* uses the system will see the changes in the system when it is redeployed.
*
*
* @param updateSystemTemplateRequest
* @return A Java Future containing the result of the UpdateSystemTemplate operation returned by the service.
* @sample AWSIoTThingsGraphAsync.UpdateSystemTemplate
* @see AWS API Documentation
*/
java.util.concurrent.Future updateSystemTemplateAsync(UpdateSystemTemplateRequest updateSystemTemplateRequest);
/**
*
* Updates the specified system. You don't need to run this action after updating a workflow. Any deployment that
* uses the system will see the changes in the system when it is redeployed.
*
*
* @param updateSystemTemplateRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateSystemTemplate operation returned by the service.
* @sample AWSIoTThingsGraphAsyncHandler.UpdateSystemTemplate
* @see AWS API Documentation
*/
java.util.concurrent.Future updateSystemTemplateAsync(UpdateSystemTemplateRequest updateSystemTemplateRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Asynchronously uploads one or more entity definitions to the user's namespace. The document
* parameter is required if syncWithPublicNamespace
and deleteExistingEntites
are false.
* If the syncWithPublicNamespace
parameter is set to true
, the user's namespace will
* synchronize with the latest version of the public namespace. If deprecateExistingEntities
is set to
* true, all entities in the latest version will be deleted before the new DefinitionDocument
is
* uploaded.
*
*
* When a user uploads entity definitions for the first time, the service creates a new namespace for the user. The
* new namespace tracks the public namespace. Currently users can have only one namespace. The namespace version
* increments whenever a user uploads entity definitions that are backwards-incompatible and whenever a user sets
* the syncWithPublicNamespace
parameter or the deprecateExistingEntities
parameter to
* true
.
*
*
* The IDs for all of the entities should be in URN format. Each entity must be in the user's namespace. Users can't
* create entities in the public namespace, but entity definitions can refer to entities in the public namespace.
*
*
* Valid entities are Device
, DeviceModel
, Service
, Capability
,
* State
, Action
, Event
, Property
, Mapping
,
* Enum
.
*
*
* @param uploadEntityDefinitionsRequest
* @return A Java Future containing the result of the UploadEntityDefinitions operation returned by the service.
* @sample AWSIoTThingsGraphAsync.UploadEntityDefinitions
* @see AWS API Documentation
*/
java.util.concurrent.Future uploadEntityDefinitionsAsync(UploadEntityDefinitionsRequest uploadEntityDefinitionsRequest);
/**
*
* Asynchronously uploads one or more entity definitions to the user's namespace. The document
* parameter is required if syncWithPublicNamespace
and deleteExistingEntites
are false.
* If the syncWithPublicNamespace
parameter is set to true
, the user's namespace will
* synchronize with the latest version of the public namespace. If deprecateExistingEntities
is set to
* true, all entities in the latest version will be deleted before the new DefinitionDocument
is
* uploaded.
*
*
* When a user uploads entity definitions for the first time, the service creates a new namespace for the user. The
* new namespace tracks the public namespace. Currently users can have only one namespace. The namespace version
* increments whenever a user uploads entity definitions that are backwards-incompatible and whenever a user sets
* the syncWithPublicNamespace
parameter or the deprecateExistingEntities
parameter to
* true
.
*
*
* The IDs for all of the entities should be in URN format. Each entity must be in the user's namespace. Users can't
* create entities in the public namespace, but entity definitions can refer to entities in the public namespace.
*
*
* Valid entities are Device
, DeviceModel
, Service
, Capability
,
* State
, Action
, Event
, Property
, Mapping
,
* Enum
.
*
*
* @param uploadEntityDefinitionsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UploadEntityDefinitions operation returned by the service.
* @sample AWSIoTThingsGraphAsyncHandler.UploadEntityDefinitions
* @see AWS API Documentation
*/
java.util.concurrent.Future uploadEntityDefinitionsAsync(UploadEntityDefinitionsRequest uploadEntityDefinitionsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
}