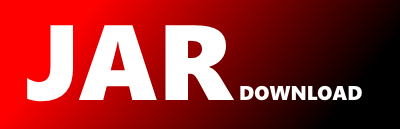
com.amazonaws.services.iotthingsgraph.AWSIoTThingsGraph Maven / Gradle / Ivy
Show all versions of aws-java-sdk-iotthingsgraph Show documentation
/*
* Copyright 2018-2023 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.iotthingsgraph;
import javax.annotation.Generated;
import com.amazonaws.*;
import com.amazonaws.regions.*;
import com.amazonaws.services.iotthingsgraph.model.*;
/**
* Interface for accessing AWS IoT Things Graph.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.iotthingsgraph.AbstractAWSIoTThingsGraph} instead.
*
*
* AWS IoT Things Graph
*
* AWS IoT Things Graph provides an integrated set of tools that enable developers to connect devices and services that
* use different standards, such as units of measure and communication protocols. AWS IoT Things Graph makes it possible
* to build IoT applications with little to no code by connecting devices and services and defining how they interact at
* an abstract level.
*
*
* For more information about how AWS IoT Things Graph works, see the User Guide.
*
*
* The AWS IoT Things Graph service is discontinued.
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AWSIoTThingsGraph {
/**
* The region metadata service name for computing region endpoints. You can use this value to retrieve metadata
* (such as supported regions) of the service.
*
* @see RegionUtils#getRegionsForService(String)
*/
String ENDPOINT_PREFIX = "iotthingsgraph";
/**
*
* Associates a device with a concrete thing that is in the user's registry.
*
*
* A thing can be associated with only one device at a time. If you associate a thing with a new device id, its
* previous association will be removed.
*
*
* @param associateEntityToThingRequest
* @return Result of the AssociateEntityToThing operation returned by the service.
* @throws InvalidRequestException
* @throws ResourceNotFoundException
* @throws InternalFailureException
* @throws ThrottlingException
* @sample AWSIoTThingsGraph.AssociateEntityToThing
* @see AWS API Documentation
*/
@Deprecated
AssociateEntityToThingResult associateEntityToThing(AssociateEntityToThingRequest associateEntityToThingRequest);
/**
*
* Creates a workflow template. Workflows can be created only in the user's namespace. (The public namespace
* contains only entities.) The workflow can contain only entities in the specified namespace. The workflow is
* validated against the entities in the latest version of the user's namespace unless another namespace version is
* specified in the request.
*
*
* @param createFlowTemplateRequest
* @return Result of the CreateFlowTemplate operation returned by the service.
* @throws InvalidRequestException
* @throws ResourceAlreadyExistsException
* @throws ThrottlingException
* @throws LimitExceededException
* @throws InternalFailureException
* @sample AWSIoTThingsGraph.CreateFlowTemplate
* @see AWS API Documentation
*/
@Deprecated
CreateFlowTemplateResult createFlowTemplate(CreateFlowTemplateRequest createFlowTemplateRequest);
/**
*
* Creates a system instance.
*
*
* This action validates the system instance, prepares the deployment-related resources. For Greengrass deployments,
* it updates the Greengrass group that is specified by the greengrassGroupName
parameter. It also adds
* a file to the S3 bucket specified by the s3BucketName
parameter. You need to call
* DeploySystemInstance
after running this action.
*
*
* For Greengrass deployments, since this action modifies and adds resources to a Greengrass group and an S3 bucket
* on the caller's behalf, the calling identity must have write permissions to both the specified Greengrass group
* and S3 bucket. Otherwise, the call will fail with an authorization error.
*
*
* For cloud deployments, this action requires a flowActionsRoleArn
value. This is an IAM role that has
* permissions to access AWS services, such as AWS Lambda and AWS IoT, that the flow uses when it executes.
*
*
* If the definition document doesn't specify a version of the user's namespace, the latest version will be used by
* default.
*
*
* @param createSystemInstanceRequest
* @return Result of the CreateSystemInstance operation returned by the service.
* @throws InvalidRequestException
* @throws ResourceAlreadyExistsException
* @throws ThrottlingException
* @throws InternalFailureException
* @throws LimitExceededException
* @sample AWSIoTThingsGraph.CreateSystemInstance
* @see AWS API Documentation
*/
@Deprecated
CreateSystemInstanceResult createSystemInstance(CreateSystemInstanceRequest createSystemInstanceRequest);
/**
*
* Creates a system. The system is validated against the entities in the latest version of the user's namespace
* unless another namespace version is specified in the request.
*
*
* @param createSystemTemplateRequest
* @return Result of the CreateSystemTemplate operation returned by the service.
* @throws InvalidRequestException
* @throws ResourceAlreadyExistsException
* @throws ThrottlingException
* @throws InternalFailureException
* @sample AWSIoTThingsGraph.CreateSystemTemplate
* @see AWS API Documentation
*/
@Deprecated
CreateSystemTemplateResult createSystemTemplate(CreateSystemTemplateRequest createSystemTemplateRequest);
/**
*
* Deletes a workflow. Any new system or deployment that contains this workflow will fail to update or deploy.
* Existing deployments that contain the workflow will continue to run (since they use a snapshot of the workflow
* taken at the time of deployment).
*
*
* @param deleteFlowTemplateRequest
* @return Result of the DeleteFlowTemplate operation returned by the service.
* @throws InvalidRequestException
* @throws ThrottlingException
* @throws InternalFailureException
* @throws ResourceInUseException
* @sample AWSIoTThingsGraph.DeleteFlowTemplate
* @see AWS API Documentation
*/
@Deprecated
DeleteFlowTemplateResult deleteFlowTemplate(DeleteFlowTemplateRequest deleteFlowTemplateRequest);
/**
*
* Deletes the specified namespace. This action deletes all of the entities in the namespace. Delete the systems and
* flows that use entities in the namespace before performing this action. This action takes no request parameters.
*
*
* @param deleteNamespaceRequest
* @return Result of the DeleteNamespace operation returned by the service.
* @throws InternalFailureException
* @throws ThrottlingException
* @sample AWSIoTThingsGraph.DeleteNamespace
* @see AWS
* API Documentation
*/
@Deprecated
DeleteNamespaceResult deleteNamespace(DeleteNamespaceRequest deleteNamespaceRequest);
/**
*
* Deletes a system instance. Only system instances that have never been deployed, or that have been undeployed can
* be deleted.
*
*
* Users can create a new system instance that has the same ID as a deleted system instance.
*
*
* @param deleteSystemInstanceRequest
* @return Result of the DeleteSystemInstance operation returned by the service.
* @throws InvalidRequestException
* @throws ThrottlingException
* @throws InternalFailureException
* @throws ResourceInUseException
* @sample AWSIoTThingsGraph.DeleteSystemInstance
* @see AWS API Documentation
*/
@Deprecated
DeleteSystemInstanceResult deleteSystemInstance(DeleteSystemInstanceRequest deleteSystemInstanceRequest);
/**
*
* Deletes a system. New deployments can't contain the system after its deletion. Existing deployments that contain
* the system will continue to work because they use a snapshot of the system that is taken when it is deployed.
*
*
* @param deleteSystemTemplateRequest
* @return Result of the DeleteSystemTemplate operation returned by the service.
* @throws InvalidRequestException
* @throws ThrottlingException
* @throws InternalFailureException
* @throws ResourceInUseException
* @sample AWSIoTThingsGraph.DeleteSystemTemplate
* @see AWS API Documentation
*/
@Deprecated
DeleteSystemTemplateResult deleteSystemTemplate(DeleteSystemTemplateRequest deleteSystemTemplateRequest);
/**
*
* Greengrass and Cloud Deployments
*
*
* Deploys the system instance to the target specified in CreateSystemInstance
.
*
*
* Greengrass Deployments
*
*
* If the system or any workflows and entities have been updated before this action is called, then the deployment
* will create a new Amazon Simple Storage Service resource file and then deploy it.
*
*
* Since this action creates a Greengrass deployment on the caller's behalf, the calling identity must have write
* permissions to the specified Greengrass group. Otherwise, the call will fail with an authorization error.
*
*
* For information about the artifacts that get added to your Greengrass core device when you use this API, see AWS IoT Things Graph and AWS IoT
* Greengrass.
*
*
* @param deploySystemInstanceRequest
* @return Result of the DeploySystemInstance operation returned by the service.
* @throws ResourceNotFoundException
* @throws InvalidRequestException
* @throws ThrottlingException
* @throws InternalFailureException
* @throws ResourceInUseException
* @sample AWSIoTThingsGraph.DeploySystemInstance
* @see AWS API Documentation
*/
@Deprecated
DeploySystemInstanceResult deploySystemInstance(DeploySystemInstanceRequest deploySystemInstanceRequest);
/**
*
* Deprecates the specified workflow. This action marks the workflow for deletion. Deprecated flows can't be
* deployed, but existing deployments will continue to run.
*
*
* @param deprecateFlowTemplateRequest
* @return Result of the DeprecateFlowTemplate operation returned by the service.
* @throws InvalidRequestException
* @throws ThrottlingException
* @throws InternalFailureException
* @throws ResourceNotFoundException
* @sample AWSIoTThingsGraph.DeprecateFlowTemplate
* @see AWS API Documentation
*/
@Deprecated
DeprecateFlowTemplateResult deprecateFlowTemplate(DeprecateFlowTemplateRequest deprecateFlowTemplateRequest);
/**
*
* Deprecates the specified system.
*
*
* @param deprecateSystemTemplateRequest
* @return Result of the DeprecateSystemTemplate operation returned by the service.
* @throws InvalidRequestException
* @throws ThrottlingException
* @throws InternalFailureException
* @throws ResourceNotFoundException
* @sample AWSIoTThingsGraph.DeprecateSystemTemplate
* @see AWS API Documentation
*/
@Deprecated
DeprecateSystemTemplateResult deprecateSystemTemplate(DeprecateSystemTemplateRequest deprecateSystemTemplateRequest);
/**
*
* Gets the latest version of the user's namespace and the public version that it is tracking.
*
*
* @param describeNamespaceRequest
* @return Result of the DescribeNamespace operation returned by the service.
* @throws ResourceNotFoundException
* @throws InvalidRequestException
* @throws InternalFailureException
* @throws ThrottlingException
* @sample AWSIoTThingsGraph.DescribeNamespace
* @see AWS API Documentation
*/
@Deprecated
DescribeNamespaceResult describeNamespace(DescribeNamespaceRequest describeNamespaceRequest);
/**
*
* Dissociates a device entity from a concrete thing. The action takes only the type of the entity that you need to
* dissociate because only one entity of a particular type can be associated with a thing.
*
*
* @param dissociateEntityFromThingRequest
* @return Result of the DissociateEntityFromThing operation returned by the service.
* @throws InvalidRequestException
* @throws ResourceNotFoundException
* @throws InternalFailureException
* @throws ThrottlingException
* @sample AWSIoTThingsGraph.DissociateEntityFromThing
* @see AWS API Documentation
*/
@Deprecated
DissociateEntityFromThingResult dissociateEntityFromThing(DissociateEntityFromThingRequest dissociateEntityFromThingRequest);
/**
*
* Gets definitions of the specified entities. Uses the latest version of the user's namespace by default. This API
* returns the following TDM entities.
*
*
* -
*
* Properties
*
*
* -
*
* States
*
*
* -
*
* Events
*
*
* -
*
* Actions
*
*
* -
*
* Capabilities
*
*
* -
*
* Mappings
*
*
* -
*
* Devices
*
*
* -
*
* Device Models
*
*
* -
*
* Services
*
*
*
*
* This action doesn't return definitions for systems, flows, and deployments.
*
*
* @param getEntitiesRequest
* @return Result of the GetEntities operation returned by the service.
* @throws InvalidRequestException
* @throws ResourceNotFoundException
* @throws ThrottlingException
* @throws InternalFailureException
* @sample AWSIoTThingsGraph.GetEntities
* @see AWS API
* Documentation
*/
@Deprecated
GetEntitiesResult getEntities(GetEntitiesRequest getEntitiesRequest);
/**
*
* Gets the latest version of the DefinitionDocument
and FlowTemplateSummary
for the
* specified workflow.
*
*
* @param getFlowTemplateRequest
* @return Result of the GetFlowTemplate operation returned by the service.
* @throws InvalidRequestException
* @throws ThrottlingException
* @throws InternalFailureException
* @throws ResourceNotFoundException
* @sample AWSIoTThingsGraph.GetFlowTemplate
* @see AWS
* API Documentation
*/
@Deprecated
GetFlowTemplateResult getFlowTemplate(GetFlowTemplateRequest getFlowTemplateRequest);
/**
*
* Gets revisions of the specified workflow. Only the last 100 revisions are stored. If the workflow has been
* deprecated, this action will return revisions that occurred before the deprecation. This action won't work for
* workflows that have been deleted.
*
*
* @param getFlowTemplateRevisionsRequest
* @return Result of the GetFlowTemplateRevisions operation returned by the service.
* @throws InvalidRequestException
* @throws ThrottlingException
* @throws InternalFailureException
* @throws ResourceNotFoundException
* @sample AWSIoTThingsGraph.GetFlowTemplateRevisions
* @see AWS API Documentation
*/
@Deprecated
GetFlowTemplateRevisionsResult getFlowTemplateRevisions(GetFlowTemplateRevisionsRequest getFlowTemplateRevisionsRequest);
/**
*
* Gets the status of a namespace deletion task.
*
*
* @param getNamespaceDeletionStatusRequest
* @return Result of the GetNamespaceDeletionStatus operation returned by the service.
* @throws InvalidRequestException
* @throws InternalFailureException
* @throws ThrottlingException
* @sample AWSIoTThingsGraph.GetNamespaceDeletionStatus
* @see AWS API Documentation
*/
@Deprecated
GetNamespaceDeletionStatusResult getNamespaceDeletionStatus(GetNamespaceDeletionStatusRequest getNamespaceDeletionStatusRequest);
/**
*
* Gets a system instance.
*
*
* @param getSystemInstanceRequest
* @return Result of the GetSystemInstance operation returned by the service.
* @throws InvalidRequestException
* @throws ThrottlingException
* @throws InternalFailureException
* @throws ResourceNotFoundException
* @sample AWSIoTThingsGraph.GetSystemInstance
* @see AWS API Documentation
*/
@Deprecated
GetSystemInstanceResult getSystemInstance(GetSystemInstanceRequest getSystemInstanceRequest);
/**
*
* Gets a system.
*
*
* @param getSystemTemplateRequest
* @return Result of the GetSystemTemplate operation returned by the service.
* @throws InvalidRequestException
* @throws ThrottlingException
* @throws InternalFailureException
* @throws ResourceNotFoundException
* @sample AWSIoTThingsGraph.GetSystemTemplate
* @see AWS API Documentation
*/
@Deprecated
GetSystemTemplateResult getSystemTemplate(GetSystemTemplateRequest getSystemTemplateRequest);
/**
*
* Gets revisions made to the specified system template. Only the previous 100 revisions are stored. If the system
* has been deprecated, this action will return the revisions that occurred before its deprecation. This action
* won't work with systems that have been deleted.
*
*
* @param getSystemTemplateRevisionsRequest
* @return Result of the GetSystemTemplateRevisions operation returned by the service.
* @throws InvalidRequestException
* @throws ThrottlingException
* @throws InternalFailureException
* @throws ResourceNotFoundException
* @sample AWSIoTThingsGraph.GetSystemTemplateRevisions
* @see AWS API Documentation
*/
@Deprecated
GetSystemTemplateRevisionsResult getSystemTemplateRevisions(GetSystemTemplateRevisionsRequest getSystemTemplateRevisionsRequest);
/**
*
* Gets the status of the specified upload.
*
*
* @param getUploadStatusRequest
* @return Result of the GetUploadStatus operation returned by the service.
* @throws InvalidRequestException
* @throws ResourceNotFoundException
* @throws InternalFailureException
* @throws ThrottlingException
* @sample AWSIoTThingsGraph.GetUploadStatus
* @see AWS
* API Documentation
*/
@Deprecated
GetUploadStatusResult getUploadStatus(GetUploadStatusRequest getUploadStatusRequest);
/**
*
* Returns a list of objects that contain information about events in a flow execution.
*
*
* @param listFlowExecutionMessagesRequest
* @return Result of the ListFlowExecutionMessages operation returned by the service.
* @throws InvalidRequestException
* @throws ThrottlingException
* @throws InternalFailureException
* @throws ResourceNotFoundException
* @sample AWSIoTThingsGraph.ListFlowExecutionMessages
* @see AWS API Documentation
*/
@Deprecated
ListFlowExecutionMessagesResult listFlowExecutionMessages(ListFlowExecutionMessagesRequest listFlowExecutionMessagesRequest);
/**
*
* Lists all tags on an AWS IoT Things Graph resource.
*
*
* @param listTagsForResourceRequest
* @return Result of the ListTagsForResource operation returned by the service.
* @throws InvalidRequestException
* @throws ResourceAlreadyExistsException
* @throws ThrottlingException
* @throws InternalFailureException
* @sample AWSIoTThingsGraph.ListTagsForResource
* @see AWS API Documentation
*/
@Deprecated
ListTagsForResourceResult listTagsForResource(ListTagsForResourceRequest listTagsForResourceRequest);
/**
*
* Searches for entities of the specified type. You can search for entities in your namespace and the public
* namespace that you're tracking.
*
*
* @param searchEntitiesRequest
* @return Result of the SearchEntities operation returned by the service.
* @throws InvalidRequestException
* @throws InternalFailureException
* @throws ThrottlingException
* @sample AWSIoTThingsGraph.SearchEntities
* @see AWS
* API Documentation
*/
@Deprecated
SearchEntitiesResult searchEntities(SearchEntitiesRequest searchEntitiesRequest);
/**
*
* Searches for AWS IoT Things Graph workflow execution instances.
*
*
* @param searchFlowExecutionsRequest
* @return Result of the SearchFlowExecutions operation returned by the service.
* @throws InvalidRequestException
* @throws ThrottlingException
* @throws InternalFailureException
* @throws ResourceNotFoundException
* @sample AWSIoTThingsGraph.SearchFlowExecutions
* @see AWS API Documentation
*/
@Deprecated
SearchFlowExecutionsResult searchFlowExecutions(SearchFlowExecutionsRequest searchFlowExecutionsRequest);
/**
*
* Searches for summary information about workflows.
*
*
* @param searchFlowTemplatesRequest
* @return Result of the SearchFlowTemplates operation returned by the service.
* @throws InvalidRequestException
* @throws ThrottlingException
* @throws InternalFailureException
* @sample AWSIoTThingsGraph.SearchFlowTemplates
* @see AWS API Documentation
*/
@Deprecated
SearchFlowTemplatesResult searchFlowTemplates(SearchFlowTemplatesRequest searchFlowTemplatesRequest);
/**
*
* Searches for system instances in the user's account.
*
*
* @param searchSystemInstancesRequest
* @return Result of the SearchSystemInstances operation returned by the service.
* @throws InvalidRequestException
* @throws ThrottlingException
* @throws InternalFailureException
* @sample AWSIoTThingsGraph.SearchSystemInstances
* @see AWS API Documentation
*/
@Deprecated
SearchSystemInstancesResult searchSystemInstances(SearchSystemInstancesRequest searchSystemInstancesRequest);
/**
*
* Searches for summary information about systems in the user's account. You can filter by the ID of a workflow to
* return only systems that use the specified workflow.
*
*
* @param searchSystemTemplatesRequest
* @return Result of the SearchSystemTemplates operation returned by the service.
* @throws InvalidRequestException
* @throws ThrottlingException
* @throws InternalFailureException
* @sample AWSIoTThingsGraph.SearchSystemTemplates
* @see AWS API Documentation
*/
@Deprecated
SearchSystemTemplatesResult searchSystemTemplates(SearchSystemTemplatesRequest searchSystemTemplatesRequest);
/**
*
* Searches for things associated with the specified entity. You can search by both device and device model.
*
*
* For example, if two different devices, camera1 and camera2, implement the camera device model, the user can
* associate thing1 to camera1 and thing2 to camera2. SearchThings(camera2)
will return only thing2,
* but SearchThings(camera)
will return both thing1 and thing2.
*
*
* This action searches for exact matches and doesn't perform partial text matching.
*
*
* @param searchThingsRequest
* @return Result of the SearchThings operation returned by the service.
* @throws InvalidRequestException
* @throws ResourceNotFoundException
* @throws InternalFailureException
* @throws ThrottlingException
* @sample AWSIoTThingsGraph.SearchThings
* @see AWS
* API Documentation
*/
@Deprecated
SearchThingsResult searchThings(SearchThingsRequest searchThingsRequest);
/**
*
* Creates a tag for the specified resource.
*
*
* @param tagResourceRequest
* @return Result of the TagResource operation returned by the service.
* @throws InvalidRequestException
* @throws ResourceAlreadyExistsException
* @throws ThrottlingException
* @throws InternalFailureException
* @sample AWSIoTThingsGraph.TagResource
* @see AWS API
* Documentation
*/
@Deprecated
TagResourceResult tagResource(TagResourceRequest tagResourceRequest);
/**
*
* Removes a system instance from its target (Cloud or Greengrass).
*
*
* @param undeploySystemInstanceRequest
* @return Result of the UndeploySystemInstance operation returned by the service.
* @throws InvalidRequestException
* @throws ThrottlingException
* @throws InternalFailureException
* @throws ResourceNotFoundException
* @throws ResourceInUseException
* @sample AWSIoTThingsGraph.UndeploySystemInstance
* @see AWS API Documentation
*/
@Deprecated
UndeploySystemInstanceResult undeploySystemInstance(UndeploySystemInstanceRequest undeploySystemInstanceRequest);
/**
*
* Removes a tag from the specified resource.
*
*
* @param untagResourceRequest
* @return Result of the UntagResource operation returned by the service.
* @throws InvalidRequestException
* @throws ResourceAlreadyExistsException
* @throws ThrottlingException
* @throws InternalFailureException
* @sample AWSIoTThingsGraph.UntagResource
* @see AWS
* API Documentation
*/
@Deprecated
UntagResourceResult untagResource(UntagResourceRequest untagResourceRequest);
/**
*
* Updates the specified workflow. All deployed systems and system instances that use the workflow will see the
* changes in the flow when it is redeployed. If you don't want this behavior, copy the workflow (creating a new
* workflow with a different ID), and update the copy. The workflow can contain only entities in the specified
* namespace.
*
*
* @param updateFlowTemplateRequest
* @return Result of the UpdateFlowTemplate operation returned by the service.
* @throws InvalidRequestException
* @throws ResourceNotFoundException
* @throws ThrottlingException
* @throws InternalFailureException
* @sample AWSIoTThingsGraph.UpdateFlowTemplate
* @see AWS API Documentation
*/
@Deprecated
UpdateFlowTemplateResult updateFlowTemplate(UpdateFlowTemplateRequest updateFlowTemplateRequest);
/**
*
* Updates the specified system. You don't need to run this action after updating a workflow. Any deployment that
* uses the system will see the changes in the system when it is redeployed.
*
*
* @param updateSystemTemplateRequest
* @return Result of the UpdateSystemTemplate operation returned by the service.
* @throws InvalidRequestException
* @throws ResourceNotFoundException
* @throws ThrottlingException
* @throws InternalFailureException
* @sample AWSIoTThingsGraph.UpdateSystemTemplate
* @see AWS API Documentation
*/
@Deprecated
UpdateSystemTemplateResult updateSystemTemplate(UpdateSystemTemplateRequest updateSystemTemplateRequest);
/**
*
* Asynchronously uploads one or more entity definitions to the user's namespace. The document
* parameter is required if syncWithPublicNamespace
and deleteExistingEntites
are false.
* If the syncWithPublicNamespace
parameter is set to true
, the user's namespace will
* synchronize with the latest version of the public namespace. If deprecateExistingEntities
is set to
* true, all entities in the latest version will be deleted before the new DefinitionDocument
is
* uploaded.
*
*
* When a user uploads entity definitions for the first time, the service creates a new namespace for the user. The
* new namespace tracks the public namespace. Currently users can have only one namespace. The namespace version
* increments whenever a user uploads entity definitions that are backwards-incompatible and whenever a user sets
* the syncWithPublicNamespace
parameter or the deprecateExistingEntities
parameter to
* true
.
*
*
* The IDs for all of the entities should be in URN format. Each entity must be in the user's namespace. Users can't
* create entities in the public namespace, but entity definitions can refer to entities in the public namespace.
*
*
* Valid entities are Device
, DeviceModel
, Service
, Capability
,
* State
, Action
, Event
, Property
, Mapping
,
* Enum
.
*
*
* @param uploadEntityDefinitionsRequest
* @return Result of the UploadEntityDefinitions operation returned by the service.
* @throws InvalidRequestException
* @throws InternalFailureException
* @throws ThrottlingException
* @sample AWSIoTThingsGraph.UploadEntityDefinitions
* @see AWS API Documentation
*/
@Deprecated
UploadEntityDefinitionsResult uploadEntityDefinitions(UploadEntityDefinitionsRequest uploadEntityDefinitionsRequest);
/**
* Shuts down this client object, releasing any resources that might be held open. This is an optional method, and
* callers are not expected to call it, but can if they want to explicitly release any open resources. Once a client
* has been shutdown, it should not be used to make any more requests.
*/
void shutdown();
/**
* Returns additional metadata for a previously executed successful request, typically used for debugging issues
* where a service isn't acting as expected. This data isn't considered part of the result data returned by an
* operation, so it's available through this separate, diagnostic interface.
*
* Response metadata is only cached for a limited period of time, so if you need to access this extra diagnostic
* information for an executed request, you should use this method to retrieve it as soon as possible after
* executing a request.
*
* @param request
* The originally executed request.
*
* @return The response metadata for the specified request, or null if none is available.
*/
ResponseMetadata getCachedResponseMetadata(AmazonWebServiceRequest request);
}