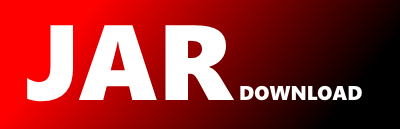
com.amazonaws.services.iotthingsgraph.model.FlowExecutionSummary Maven / Gradle / Ivy
Show all versions of aws-java-sdk-iotthingsgraph Show documentation
/*
* Copyright 2018-2023 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.iotthingsgraph.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* An object that contains summary information about a flow execution.
*
*
* @see AWS API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class FlowExecutionSummary implements Serializable, Cloneable, StructuredPojo {
/**
*
* The ID of the flow execution.
*
*/
private String flowExecutionId;
/**
*
* The current status of the flow execution.
*
*/
private String status;
/**
*
* The ID of the system instance that contains the flow.
*
*/
private String systemInstanceId;
/**
*
* The ID of the flow.
*
*/
private String flowTemplateId;
/**
*
* The date and time when the flow execution summary was created.
*
*/
private java.util.Date createdAt;
/**
*
* The date and time when the flow execution summary was last updated.
*
*/
private java.util.Date updatedAt;
/**
*
* The ID of the flow execution.
*
*
* @param flowExecutionId
* The ID of the flow execution.
*/
public void setFlowExecutionId(String flowExecutionId) {
this.flowExecutionId = flowExecutionId;
}
/**
*
* The ID of the flow execution.
*
*
* @return The ID of the flow execution.
*/
public String getFlowExecutionId() {
return this.flowExecutionId;
}
/**
*
* The ID of the flow execution.
*
*
* @param flowExecutionId
* The ID of the flow execution.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public FlowExecutionSummary withFlowExecutionId(String flowExecutionId) {
setFlowExecutionId(flowExecutionId);
return this;
}
/**
*
* The current status of the flow execution.
*
*
* @param status
* The current status of the flow execution.
* @see FlowExecutionStatus
*/
public void setStatus(String status) {
this.status = status;
}
/**
*
* The current status of the flow execution.
*
*
* @return The current status of the flow execution.
* @see FlowExecutionStatus
*/
public String getStatus() {
return this.status;
}
/**
*
* The current status of the flow execution.
*
*
* @param status
* The current status of the flow execution.
* @return Returns a reference to this object so that method calls can be chained together.
* @see FlowExecutionStatus
*/
public FlowExecutionSummary withStatus(String status) {
setStatus(status);
return this;
}
/**
*
* The current status of the flow execution.
*
*
* @param status
* The current status of the flow execution.
* @return Returns a reference to this object so that method calls can be chained together.
* @see FlowExecutionStatus
*/
public FlowExecutionSummary withStatus(FlowExecutionStatus status) {
this.status = status.toString();
return this;
}
/**
*
* The ID of the system instance that contains the flow.
*
*
* @param systemInstanceId
* The ID of the system instance that contains the flow.
*/
public void setSystemInstanceId(String systemInstanceId) {
this.systemInstanceId = systemInstanceId;
}
/**
*
* The ID of the system instance that contains the flow.
*
*
* @return The ID of the system instance that contains the flow.
*/
public String getSystemInstanceId() {
return this.systemInstanceId;
}
/**
*
* The ID of the system instance that contains the flow.
*
*
* @param systemInstanceId
* The ID of the system instance that contains the flow.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public FlowExecutionSummary withSystemInstanceId(String systemInstanceId) {
setSystemInstanceId(systemInstanceId);
return this;
}
/**
*
* The ID of the flow.
*
*
* @param flowTemplateId
* The ID of the flow.
*/
public void setFlowTemplateId(String flowTemplateId) {
this.flowTemplateId = flowTemplateId;
}
/**
*
* The ID of the flow.
*
*
* @return The ID of the flow.
*/
public String getFlowTemplateId() {
return this.flowTemplateId;
}
/**
*
* The ID of the flow.
*
*
* @param flowTemplateId
* The ID of the flow.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public FlowExecutionSummary withFlowTemplateId(String flowTemplateId) {
setFlowTemplateId(flowTemplateId);
return this;
}
/**
*
* The date and time when the flow execution summary was created.
*
*
* @param createdAt
* The date and time when the flow execution summary was created.
*/
public void setCreatedAt(java.util.Date createdAt) {
this.createdAt = createdAt;
}
/**
*
* The date and time when the flow execution summary was created.
*
*
* @return The date and time when the flow execution summary was created.
*/
public java.util.Date getCreatedAt() {
return this.createdAt;
}
/**
*
* The date and time when the flow execution summary was created.
*
*
* @param createdAt
* The date and time when the flow execution summary was created.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public FlowExecutionSummary withCreatedAt(java.util.Date createdAt) {
setCreatedAt(createdAt);
return this;
}
/**
*
* The date and time when the flow execution summary was last updated.
*
*
* @param updatedAt
* The date and time when the flow execution summary was last updated.
*/
public void setUpdatedAt(java.util.Date updatedAt) {
this.updatedAt = updatedAt;
}
/**
*
* The date and time when the flow execution summary was last updated.
*
*
* @return The date and time when the flow execution summary was last updated.
*/
public java.util.Date getUpdatedAt() {
return this.updatedAt;
}
/**
*
* The date and time when the flow execution summary was last updated.
*
*
* @param updatedAt
* The date and time when the flow execution summary was last updated.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public FlowExecutionSummary withUpdatedAt(java.util.Date updatedAt) {
setUpdatedAt(updatedAt);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getFlowExecutionId() != null)
sb.append("FlowExecutionId: ").append(getFlowExecutionId()).append(",");
if (getStatus() != null)
sb.append("Status: ").append(getStatus()).append(",");
if (getSystemInstanceId() != null)
sb.append("SystemInstanceId: ").append(getSystemInstanceId()).append(",");
if (getFlowTemplateId() != null)
sb.append("FlowTemplateId: ").append(getFlowTemplateId()).append(",");
if (getCreatedAt() != null)
sb.append("CreatedAt: ").append(getCreatedAt()).append(",");
if (getUpdatedAt() != null)
sb.append("UpdatedAt: ").append(getUpdatedAt());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof FlowExecutionSummary == false)
return false;
FlowExecutionSummary other = (FlowExecutionSummary) obj;
if (other.getFlowExecutionId() == null ^ this.getFlowExecutionId() == null)
return false;
if (other.getFlowExecutionId() != null && other.getFlowExecutionId().equals(this.getFlowExecutionId()) == false)
return false;
if (other.getStatus() == null ^ this.getStatus() == null)
return false;
if (other.getStatus() != null && other.getStatus().equals(this.getStatus()) == false)
return false;
if (other.getSystemInstanceId() == null ^ this.getSystemInstanceId() == null)
return false;
if (other.getSystemInstanceId() != null && other.getSystemInstanceId().equals(this.getSystemInstanceId()) == false)
return false;
if (other.getFlowTemplateId() == null ^ this.getFlowTemplateId() == null)
return false;
if (other.getFlowTemplateId() != null && other.getFlowTemplateId().equals(this.getFlowTemplateId()) == false)
return false;
if (other.getCreatedAt() == null ^ this.getCreatedAt() == null)
return false;
if (other.getCreatedAt() != null && other.getCreatedAt().equals(this.getCreatedAt()) == false)
return false;
if (other.getUpdatedAt() == null ^ this.getUpdatedAt() == null)
return false;
if (other.getUpdatedAt() != null && other.getUpdatedAt().equals(this.getUpdatedAt()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getFlowExecutionId() == null) ? 0 : getFlowExecutionId().hashCode());
hashCode = prime * hashCode + ((getStatus() == null) ? 0 : getStatus().hashCode());
hashCode = prime * hashCode + ((getSystemInstanceId() == null) ? 0 : getSystemInstanceId().hashCode());
hashCode = prime * hashCode + ((getFlowTemplateId() == null) ? 0 : getFlowTemplateId().hashCode());
hashCode = prime * hashCode + ((getCreatedAt() == null) ? 0 : getCreatedAt().hashCode());
hashCode = prime * hashCode + ((getUpdatedAt() == null) ? 0 : getUpdatedAt().hashCode());
return hashCode;
}
@Override
public FlowExecutionSummary clone() {
try {
return (FlowExecutionSummary) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.iotthingsgraph.model.transform.FlowExecutionSummaryMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}