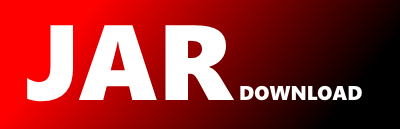
com.amazonaws.services.iottwinmaker.AWSIoTTwinMaker Maven / Gradle / Ivy
Show all versions of aws-java-sdk-iottwinmaker Show documentation
/*
* Copyright 2017-2022 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.iottwinmaker;
import javax.annotation.Generated;
import com.amazonaws.*;
import com.amazonaws.regions.*;
import com.amazonaws.services.iottwinmaker.model.*;
/**
* Interface for accessing AWS IoT TwinMaker.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.iottwinmaker.AbstractAWSIoTTwinMaker} instead.
*
*
*
*
* TwinMaker is in public preview and is subject to change.
*
*
*
* IoT TwinMaker is a service that enables you to build operational digital twins of physical systems. IoT TwinMaker
* overlays measurements and analysis from real-world sensors, cameras, and enterprise applications so you can create
* data visualizations to monitor your physical factory, building, or industrial plant. You can use this real-world data
* to monitor operations and diagnose and repair errors.
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AWSIoTTwinMaker {
/**
* The region metadata service name for computing region endpoints. You can use this value to retrieve metadata
* (such as supported regions) of the service.
*
* @see RegionUtils#getRegionsForService(String)
*/
String ENDPOINT_PREFIX = "iottwinmaker";
/**
*
* Sets values for multiple time series properties.
*
*
* @param batchPutPropertyValuesRequest
* @return Result of the BatchPutPropertyValues operation returned by the service.
* @throws InternalServerException
* An unexpected error has occurred.
* @throws ResourceNotFoundException
* The resource wasn't found.
* @throws ThrottlingException
* The rate exceeds the limit.
* @throws ValidationException
* Failed
* @sample AWSIoTTwinMaker.BatchPutPropertyValues
* @see AWS API Documentation
*/
BatchPutPropertyValuesResult batchPutPropertyValues(BatchPutPropertyValuesRequest batchPutPropertyValuesRequest);
/**
*
* Creates a component type.
*
*
*
* TwinMaker is in public preview and is subject to change.
*
*
*
* @param createComponentTypeRequest
* @return Result of the CreateComponentType operation returned by the service.
* @throws InternalServerException
* An unexpected error has occurred.
* @throws AccessDeniedException
* Access is denied.
* @throws ThrottlingException
* The rate exceeds the limit.
* @throws ValidationException
* Failed
* @throws ConflictException
* A conflict occurred.
* @throws ServiceQuotaExceededException
* The service quota was exceeded.
* @sample AWSIoTTwinMaker.CreateComponentType
* @see AWS API Documentation
*/
CreateComponentTypeResult createComponentType(CreateComponentTypeRequest createComponentTypeRequest);
/**
*
* Creates an entity.
*
*
* @param createEntityRequest
* @return Result of the CreateEntity operation returned by the service.
* @throws InternalServerException
* An unexpected error has occurred.
* @throws AccessDeniedException
* Access is denied.
* @throws ThrottlingException
* The rate exceeds the limit.
* @throws ValidationException
* Failed
* @throws ConflictException
* A conflict occurred.
* @throws ServiceQuotaExceededException
* The service quota was exceeded.
* @sample AWSIoTTwinMaker.CreateEntity
* @see AWS API
* Documentation
*/
CreateEntityResult createEntity(CreateEntityRequest createEntityRequest);
/**
*
* Creates a scene.
*
*
* @param createSceneRequest
* @return Result of the CreateScene operation returned by the service.
* @throws InternalServerException
* An unexpected error has occurred.
* @throws AccessDeniedException
* Access is denied.
* @throws ThrottlingException
* The rate exceeds the limit.
* @throws ValidationException
* Failed
* @throws ConflictException
* A conflict occurred.
* @throws ServiceQuotaExceededException
* The service quota was exceeded.
* @sample AWSIoTTwinMaker.CreateScene
* @see AWS API
* Documentation
*/
CreateSceneResult createScene(CreateSceneRequest createSceneRequest);
/**
*
* Creates a workplace.
*
*
* @param createWorkspaceRequest
* @return Result of the CreateWorkspace operation returned by the service.
* @throws InternalServerException
* An unexpected error has occurred.
* @throws AccessDeniedException
* Access is denied.
* @throws ThrottlingException
* The rate exceeds the limit.
* @throws ValidationException
* Failed
* @throws ConflictException
* A conflict occurred.
* @throws ServiceQuotaExceededException
* The service quota was exceeded.
* @sample AWSIoTTwinMaker.CreateWorkspace
* @see AWS
* API Documentation
*/
CreateWorkspaceResult createWorkspace(CreateWorkspaceRequest createWorkspaceRequest);
/**
*
* Deletes a component type.
*
*
* @param deleteComponentTypeRequest
* @return Result of the DeleteComponentType operation returned by the service.
* @throws InternalServerException
* An unexpected error has occurred.
* @throws AccessDeniedException
* Access is denied.
* @throws ResourceNotFoundException
* The resource wasn't found.
* @throws ThrottlingException
* The rate exceeds the limit.
* @throws ValidationException
* Failed
* @sample AWSIoTTwinMaker.DeleteComponentType
* @see AWS API Documentation
*/
DeleteComponentTypeResult deleteComponentType(DeleteComponentTypeRequest deleteComponentTypeRequest);
/**
*
* Deletes an entity.
*
*
* @param deleteEntityRequest
* @return Result of the DeleteEntity operation returned by the service.
* @throws InternalServerException
* An unexpected error has occurred.
* @throws ResourceNotFoundException
* The resource wasn't found.
* @throws ThrottlingException
* The rate exceeds the limit.
* @throws ValidationException
* Failed
* @throws ServiceQuotaExceededException
* The service quota was exceeded.
* @sample AWSIoTTwinMaker.DeleteEntity
* @see AWS API
* Documentation
*/
DeleteEntityResult deleteEntity(DeleteEntityRequest deleteEntityRequest);
/**
*
* Deletes a scene.
*
*
* @param deleteSceneRequest
* @return Result of the DeleteScene operation returned by the service.
* @throws InternalServerException
* An unexpected error has occurred.
* @throws AccessDeniedException
* Access is denied.
* @throws ResourceNotFoundException
* The resource wasn't found.
* @throws ThrottlingException
* The rate exceeds the limit.
* @throws ValidationException
* Failed
* @sample AWSIoTTwinMaker.DeleteScene
* @see AWS API
* Documentation
*/
DeleteSceneResult deleteScene(DeleteSceneRequest deleteSceneRequest);
/**
*
* Deletes a workspace.
*
*
* @param deleteWorkspaceRequest
* @return Result of the DeleteWorkspace operation returned by the service.
* @throws InternalServerException
* An unexpected error has occurred.
* @throws AccessDeniedException
* Access is denied.
* @throws ResourceNotFoundException
* The resource wasn't found.
* @throws ThrottlingException
* The rate exceeds the limit.
* @throws ValidationException
* Failed
* @sample AWSIoTTwinMaker.DeleteWorkspace
* @see AWS
* API Documentation
*/
DeleteWorkspaceResult deleteWorkspace(DeleteWorkspaceRequest deleteWorkspaceRequest);
/**
*
* Retrieves information about a component type.
*
*
* @param getComponentTypeRequest
* @return Result of the GetComponentType operation returned by the service.
* @throws InternalServerException
* An unexpected error has occurred.
* @throws AccessDeniedException
* Access is denied.
* @throws ResourceNotFoundException
* The resource wasn't found.
* @throws ThrottlingException
* The rate exceeds the limit.
* @sample AWSIoTTwinMaker.GetComponentType
* @see AWS
* API Documentation
*/
GetComponentTypeResult getComponentType(GetComponentTypeRequest getComponentTypeRequest);
/**
*
* Retrieves information about an entity.
*
*
* @param getEntityRequest
* @return Result of the GetEntity operation returned by the service.
* @throws InternalServerException
* An unexpected error has occurred.
* @throws ResourceNotFoundException
* The resource wasn't found.
* @throws ThrottlingException
* The rate exceeds the limit.
* @throws ValidationException
* Failed
* @throws ServiceQuotaExceededException
* The service quota was exceeded.
* @sample AWSIoTTwinMaker.GetEntity
* @see AWS API
* Documentation
*/
GetEntityResult getEntity(GetEntityRequest getEntityRequest);
/**
*
* Gets the property values for a component, component type, entity, or workspace.
*
*
* You must specify a value for either componentName
, componentTypeId
,
* entityId
, or workspaceId
.
*
*
* @param getPropertyValueRequest
* @return Result of the GetPropertyValue operation returned by the service.
* @throws InternalServerException
* An unexpected error has occurred.
* @throws ConnectorFailureException
* The connector failed.
* @throws AccessDeniedException
* Access is denied.
* @throws ResourceNotFoundException
* The resource wasn't found.
* @throws ThrottlingException
* The rate exceeds the limit.
* @throws ValidationException
* Failed
* @throws ConnectorTimeoutException
* The connector timed out.
* @sample AWSIoTTwinMaker.GetPropertyValue
* @see AWS
* API Documentation
*/
GetPropertyValueResult getPropertyValue(GetPropertyValueRequest getPropertyValueRequest);
/**
*
* Retrieves information about the history of a time series property value for a component, component type, entity,
* or workspace.
*
*
* You must specify a value for workspaceId
. For entity-specific queries, specify values for
* componentName
and entityId
. For cross-entity quries, specify a value for
* componentTypeId
.
*
*
* @param getPropertyValueHistoryRequest
* @return Result of the GetPropertyValueHistory operation returned by the service.
* @throws InternalServerException
* An unexpected error has occurred.
* @throws ConnectorFailureException
* The connector failed.
* @throws AccessDeniedException
* Access is denied.
* @throws ResourceNotFoundException
* The resource wasn't found.
* @throws ThrottlingException
* The rate exceeds the limit.
* @throws ValidationException
* Failed
* @throws ConnectorTimeoutException
* The connector timed out.
* @sample AWSIoTTwinMaker.GetPropertyValueHistory
* @see AWS API Documentation
*/
GetPropertyValueHistoryResult getPropertyValueHistory(GetPropertyValueHistoryRequest getPropertyValueHistoryRequest);
/**
*
* Retrieves information about a scene.
*
*
* @param getSceneRequest
* @return Result of the GetScene operation returned by the service.
* @throws InternalServerException
* An unexpected error has occurred.
* @throws AccessDeniedException
* Access is denied.
* @throws ResourceNotFoundException
* The resource wasn't found.
* @throws ThrottlingException
* The rate exceeds the limit.
* @throws ValidationException
* Failed
* @sample AWSIoTTwinMaker.GetScene
* @see AWS API
* Documentation
*/
GetSceneResult getScene(GetSceneRequest getSceneRequest);
/**
*
* Retrieves information about a workspace.
*
*
* @param getWorkspaceRequest
* @return Result of the GetWorkspace operation returned by the service.
* @throws InternalServerException
* An unexpected error has occurred.
* @throws ResourceNotFoundException
* The resource wasn't found.
* @throws ThrottlingException
* The rate exceeds the limit.
* @throws ValidationException
* Failed
* @throws ServiceQuotaExceededException
* The service quota was exceeded.
* @sample AWSIoTTwinMaker.GetWorkspace
* @see AWS API
* Documentation
*/
GetWorkspaceResult getWorkspace(GetWorkspaceRequest getWorkspaceRequest);
/**
*
* Lists all component types in a workspace.
*
*
* @param listComponentTypesRequest
* @return Result of the ListComponentTypes operation returned by the service.
* @throws InternalServerException
* An unexpected error has occurred.
* @throws AccessDeniedException
* Access is denied.
* @throws ThrottlingException
* The rate exceeds the limit.
* @throws ValidationException
* Failed
* @sample AWSIoTTwinMaker.ListComponentTypes
* @see AWS API Documentation
*/
ListComponentTypesResult listComponentTypes(ListComponentTypesRequest listComponentTypesRequest);
/**
*
* Lists all entities in a workspace.
*
*
* @param listEntitiesRequest
* @return Result of the ListEntities operation returned by the service.
* @throws InternalServerException
* An unexpected error has occurred.
* @throws ThrottlingException
* The rate exceeds the limit.
* @throws ValidationException
* Failed
* @throws ServiceQuotaExceededException
* The service quota was exceeded.
* @sample AWSIoTTwinMaker.ListEntities
* @see AWS API
* Documentation
*/
ListEntitiesResult listEntities(ListEntitiesRequest listEntitiesRequest);
/**
*
* Lists all scenes in a workspace.
*
*
* @param listScenesRequest
* @return Result of the ListScenes operation returned by the service.
* @throws InternalServerException
* An unexpected error has occurred.
* @throws AccessDeniedException
* Access is denied.
* @throws ThrottlingException
* The rate exceeds the limit.
* @throws ValidationException
* Failed
* @sample AWSIoTTwinMaker.ListScenes
* @see AWS API
* Documentation
*/
ListScenesResult listScenes(ListScenesRequest listScenesRequest);
/**
*
* Lists all tags associated with a resource.
*
*
* @param listTagsForResourceRequest
* @return Result of the ListTagsForResource operation returned by the service.
* @throws AccessDeniedException
* Access is denied.
* @throws ResourceNotFoundException
* The resource wasn't found.
* @sample AWSIoTTwinMaker.ListTagsForResource
* @see AWS API Documentation
*/
ListTagsForResourceResult listTagsForResource(ListTagsForResourceRequest listTagsForResourceRequest);
/**
*
* Retrieves information about workspaces in the current account.
*
*
* @param listWorkspacesRequest
* @return Result of the ListWorkspaces operation returned by the service.
* @throws InternalServerException
* An unexpected error has occurred.
* @throws ThrottlingException
* The rate exceeds the limit.
* @throws ValidationException
* Failed
* @throws ServiceQuotaExceededException
* The service quota was exceeded.
* @sample AWSIoTTwinMaker.ListWorkspaces
* @see AWS
* API Documentation
*/
ListWorkspacesResult listWorkspaces(ListWorkspacesRequest listWorkspacesRequest);
/**
*
* Adds tags to a resource.
*
*
* @param tagResourceRequest
* @return Result of the TagResource operation returned by the service.
* @throws TooManyTagsException
* The number of tags exceeds the limit.
* @throws AccessDeniedException
* Access is denied.
* @throws ResourceNotFoundException
* The resource wasn't found.
* @sample AWSIoTTwinMaker.TagResource
* @see AWS API
* Documentation
*/
TagResourceResult tagResource(TagResourceRequest tagResourceRequest);
/**
*
* Removes tags from a resource.
*
*
* @param untagResourceRequest
* @return Result of the UntagResource operation returned by the service.
* @throws AccessDeniedException
* Access is denied.
* @throws ResourceNotFoundException
* The resource wasn't found.
* @sample AWSIoTTwinMaker.UntagResource
* @see AWS API
* Documentation
*/
UntagResourceResult untagResource(UntagResourceRequest untagResourceRequest);
/**
*
* Updates information in a component type.
*
*
* @param updateComponentTypeRequest
* @return Result of the UpdateComponentType operation returned by the service.
* @throws InternalServerException
* An unexpected error has occurred.
* @throws AccessDeniedException
* Access is denied.
* @throws ResourceNotFoundException
* The resource wasn't found.
* @throws ThrottlingException
* The rate exceeds the limit.
* @throws ValidationException
* Failed
* @throws ServiceQuotaExceededException
* The service quota was exceeded.
* @sample AWSIoTTwinMaker.UpdateComponentType
* @see AWS API Documentation
*/
UpdateComponentTypeResult updateComponentType(UpdateComponentTypeRequest updateComponentTypeRequest);
/**
*
* Updates an entity.
*
*
* @param updateEntityRequest
* @return Result of the UpdateEntity operation returned by the service.
* @throws InternalServerException
* An unexpected error has occurred.
* @throws AccessDeniedException
* Access is denied.
* @throws ResourceNotFoundException
* The resource wasn't found.
* @throws ThrottlingException
* The rate exceeds the limit.
* @throws ValidationException
* Failed
* @throws ConflictException
* A conflict occurred.
* @throws ServiceQuotaExceededException
* The service quota was exceeded.
* @sample AWSIoTTwinMaker.UpdateEntity
* @see AWS API
* Documentation
*/
UpdateEntityResult updateEntity(UpdateEntityRequest updateEntityRequest);
/**
*
* Updates a scene.
*
*
* @param updateSceneRequest
* @return Result of the UpdateScene operation returned by the service.
* @throws InternalServerException
* An unexpected error has occurred.
* @throws AccessDeniedException
* Access is denied.
* @throws ResourceNotFoundException
* The resource wasn't found.
* @throws ThrottlingException
* The rate exceeds the limit.
* @throws ValidationException
* Failed
* @sample AWSIoTTwinMaker.UpdateScene
* @see AWS API
* Documentation
*/
UpdateSceneResult updateScene(UpdateSceneRequest updateSceneRequest);
/**
*
* Updates a workspace.
*
*
* @param updateWorkspaceRequest
* @return Result of the UpdateWorkspace operation returned by the service.
* @throws InternalServerException
* An unexpected error has occurred.
* @throws AccessDeniedException
* Access is denied.
* @throws ResourceNotFoundException
* The resource wasn't found.
* @throws ThrottlingException
* The rate exceeds the limit.
* @throws ValidationException
* Failed
* @throws ServiceQuotaExceededException
* The service quota was exceeded.
* @sample AWSIoTTwinMaker.UpdateWorkspace
* @see AWS
* API Documentation
*/
UpdateWorkspaceResult updateWorkspace(UpdateWorkspaceRequest updateWorkspaceRequest);
/**
* Shuts down this client object, releasing any resources that might be held open. This is an optional method, and
* callers are not expected to call it, but can if they want to explicitly release any open resources. Once a client
* has been shutdown, it should not be used to make any more requests.
*/
void shutdown();
/**
* Returns additional metadata for a previously executed successful request, typically used for debugging issues
* where a service isn't acting as expected. This data isn't considered part of the result data returned by an
* operation, so it's available through this separate, diagnostic interface.
*
* Response metadata is only cached for a limited period of time, so if you need to access this extra diagnostic
* information for an executed request, you should use this method to retrieve it as soon as possible after
* executing a request.
*
* @param request
* The originally executed request.
*
* @return The response metadata for the specified request, or null if none is available.
*/
ResponseMetadata getCachedResponseMetadata(AmazonWebServiceRequest request);
}