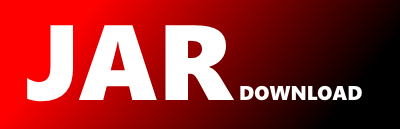
com.amazonaws.services.iottwinmaker.model.DataValue Maven / Gradle / Ivy
Show all versions of aws-java-sdk-iottwinmaker Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.iottwinmaker.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* An object that specifies a value for a property.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class DataValue implements Serializable, Cloneable, StructuredPojo {
/**
*
* A Boolean value.
*
*/
private Boolean booleanValue;
/**
*
* A double value.
*
*/
private Double doubleValue;
/**
*
* An integer value.
*
*/
private Integer integerValue;
/**
*
* A long value.
*
*/
private Long longValue;
/**
*
* A string value.
*
*/
private String stringValue;
/**
*
* A list of multiple values.
*
*/
private java.util.List listValue;
/**
*
* An object that maps strings to multiple DataValue
objects.
*
*/
private java.util.Map mapValue;
/**
*
* A value that relates a component to another component.
*
*/
private RelationshipValue relationshipValue;
/**
*
* An expression that produces the value.
*
*/
private String expression;
/**
*
* A Boolean value.
*
*
* @param booleanValue
* A Boolean value.
*/
public void setBooleanValue(Boolean booleanValue) {
this.booleanValue = booleanValue;
}
/**
*
* A Boolean value.
*
*
* @return A Boolean value.
*/
public Boolean getBooleanValue() {
return this.booleanValue;
}
/**
*
* A Boolean value.
*
*
* @param booleanValue
* A Boolean value.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DataValue withBooleanValue(Boolean booleanValue) {
setBooleanValue(booleanValue);
return this;
}
/**
*
* A Boolean value.
*
*
* @return A Boolean value.
*/
public Boolean isBooleanValue() {
return this.booleanValue;
}
/**
*
* A double value.
*
*
* @param doubleValue
* A double value.
*/
public void setDoubleValue(Double doubleValue) {
this.doubleValue = doubleValue;
}
/**
*
* A double value.
*
*
* @return A double value.
*/
public Double getDoubleValue() {
return this.doubleValue;
}
/**
*
* A double value.
*
*
* @param doubleValue
* A double value.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DataValue withDoubleValue(Double doubleValue) {
setDoubleValue(doubleValue);
return this;
}
/**
*
* An integer value.
*
*
* @param integerValue
* An integer value.
*/
public void setIntegerValue(Integer integerValue) {
this.integerValue = integerValue;
}
/**
*
* An integer value.
*
*
* @return An integer value.
*/
public Integer getIntegerValue() {
return this.integerValue;
}
/**
*
* An integer value.
*
*
* @param integerValue
* An integer value.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DataValue withIntegerValue(Integer integerValue) {
setIntegerValue(integerValue);
return this;
}
/**
*
* A long value.
*
*
* @param longValue
* A long value.
*/
public void setLongValue(Long longValue) {
this.longValue = longValue;
}
/**
*
* A long value.
*
*
* @return A long value.
*/
public Long getLongValue() {
return this.longValue;
}
/**
*
* A long value.
*
*
* @param longValue
* A long value.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DataValue withLongValue(Long longValue) {
setLongValue(longValue);
return this;
}
/**
*
* A string value.
*
*
* @param stringValue
* A string value.
*/
public void setStringValue(String stringValue) {
this.stringValue = stringValue;
}
/**
*
* A string value.
*
*
* @return A string value.
*/
public String getStringValue() {
return this.stringValue;
}
/**
*
* A string value.
*
*
* @param stringValue
* A string value.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DataValue withStringValue(String stringValue) {
setStringValue(stringValue);
return this;
}
/**
*
* A list of multiple values.
*
*
* @return A list of multiple values.
*/
public java.util.List getListValue() {
return listValue;
}
/**
*
* A list of multiple values.
*
*
* @param listValue
* A list of multiple values.
*/
public void setListValue(java.util.Collection listValue) {
if (listValue == null) {
this.listValue = null;
return;
}
this.listValue = new java.util.ArrayList(listValue);
}
/**
*
* A list of multiple values.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setListValue(java.util.Collection)} or {@link #withListValue(java.util.Collection)} if you want to
* override the existing values.
*
*
* @param listValue
* A list of multiple values.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DataValue withListValue(DataValue... listValue) {
if (this.listValue == null) {
setListValue(new java.util.ArrayList(listValue.length));
}
for (DataValue ele : listValue) {
this.listValue.add(ele);
}
return this;
}
/**
*
* A list of multiple values.
*
*
* @param listValue
* A list of multiple values.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DataValue withListValue(java.util.Collection listValue) {
setListValue(listValue);
return this;
}
/**
*
* An object that maps strings to multiple DataValue
objects.
*
*
* @return An object that maps strings to multiple DataValue
objects.
*/
public java.util.Map getMapValue() {
return mapValue;
}
/**
*
* An object that maps strings to multiple DataValue
objects.
*
*
* @param mapValue
* An object that maps strings to multiple DataValue
objects.
*/
public void setMapValue(java.util.Map mapValue) {
this.mapValue = mapValue;
}
/**
*
* An object that maps strings to multiple DataValue
objects.
*
*
* @param mapValue
* An object that maps strings to multiple DataValue
objects.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DataValue withMapValue(java.util.Map mapValue) {
setMapValue(mapValue);
return this;
}
/**
* Add a single MapValue entry
*
* @see DataValue#withMapValue
* @returns a reference to this object so that method calls can be chained together.
*/
public DataValue addMapValueEntry(String key, DataValue value) {
if (null == this.mapValue) {
this.mapValue = new java.util.HashMap();
}
if (this.mapValue.containsKey(key))
throw new IllegalArgumentException("Duplicated keys (" + key.toString() + ") are provided.");
this.mapValue.put(key, value);
return this;
}
/**
* Removes all the entries added into MapValue.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DataValue clearMapValueEntries() {
this.mapValue = null;
return this;
}
/**
*
* A value that relates a component to another component.
*
*
* @param relationshipValue
* A value that relates a component to another component.
*/
public void setRelationshipValue(RelationshipValue relationshipValue) {
this.relationshipValue = relationshipValue;
}
/**
*
* A value that relates a component to another component.
*
*
* @return A value that relates a component to another component.
*/
public RelationshipValue getRelationshipValue() {
return this.relationshipValue;
}
/**
*
* A value that relates a component to another component.
*
*
* @param relationshipValue
* A value that relates a component to another component.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DataValue withRelationshipValue(RelationshipValue relationshipValue) {
setRelationshipValue(relationshipValue);
return this;
}
/**
*
* An expression that produces the value.
*
*
* @param expression
* An expression that produces the value.
*/
public void setExpression(String expression) {
this.expression = expression;
}
/**
*
* An expression that produces the value.
*
*
* @return An expression that produces the value.
*/
public String getExpression() {
return this.expression;
}
/**
*
* An expression that produces the value.
*
*
* @param expression
* An expression that produces the value.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DataValue withExpression(String expression) {
setExpression(expression);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getBooleanValue() != null)
sb.append("BooleanValue: ").append(getBooleanValue()).append(",");
if (getDoubleValue() != null)
sb.append("DoubleValue: ").append(getDoubleValue()).append(",");
if (getIntegerValue() != null)
sb.append("IntegerValue: ").append(getIntegerValue()).append(",");
if (getLongValue() != null)
sb.append("LongValue: ").append(getLongValue()).append(",");
if (getStringValue() != null)
sb.append("StringValue: ").append(getStringValue()).append(",");
if (getListValue() != null)
sb.append("ListValue: ").append(getListValue()).append(",");
if (getMapValue() != null)
sb.append("MapValue: ").append(getMapValue()).append(",");
if (getRelationshipValue() != null)
sb.append("RelationshipValue: ").append(getRelationshipValue()).append(",");
if (getExpression() != null)
sb.append("Expression: ").append(getExpression());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof DataValue == false)
return false;
DataValue other = (DataValue) obj;
if (other.getBooleanValue() == null ^ this.getBooleanValue() == null)
return false;
if (other.getBooleanValue() != null && other.getBooleanValue().equals(this.getBooleanValue()) == false)
return false;
if (other.getDoubleValue() == null ^ this.getDoubleValue() == null)
return false;
if (other.getDoubleValue() != null && other.getDoubleValue().equals(this.getDoubleValue()) == false)
return false;
if (other.getIntegerValue() == null ^ this.getIntegerValue() == null)
return false;
if (other.getIntegerValue() != null && other.getIntegerValue().equals(this.getIntegerValue()) == false)
return false;
if (other.getLongValue() == null ^ this.getLongValue() == null)
return false;
if (other.getLongValue() != null && other.getLongValue().equals(this.getLongValue()) == false)
return false;
if (other.getStringValue() == null ^ this.getStringValue() == null)
return false;
if (other.getStringValue() != null && other.getStringValue().equals(this.getStringValue()) == false)
return false;
if (other.getListValue() == null ^ this.getListValue() == null)
return false;
if (other.getListValue() != null && other.getListValue().equals(this.getListValue()) == false)
return false;
if (other.getMapValue() == null ^ this.getMapValue() == null)
return false;
if (other.getMapValue() != null && other.getMapValue().equals(this.getMapValue()) == false)
return false;
if (other.getRelationshipValue() == null ^ this.getRelationshipValue() == null)
return false;
if (other.getRelationshipValue() != null && other.getRelationshipValue().equals(this.getRelationshipValue()) == false)
return false;
if (other.getExpression() == null ^ this.getExpression() == null)
return false;
if (other.getExpression() != null && other.getExpression().equals(this.getExpression()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getBooleanValue() == null) ? 0 : getBooleanValue().hashCode());
hashCode = prime * hashCode + ((getDoubleValue() == null) ? 0 : getDoubleValue().hashCode());
hashCode = prime * hashCode + ((getIntegerValue() == null) ? 0 : getIntegerValue().hashCode());
hashCode = prime * hashCode + ((getLongValue() == null) ? 0 : getLongValue().hashCode());
hashCode = prime * hashCode + ((getStringValue() == null) ? 0 : getStringValue().hashCode());
hashCode = prime * hashCode + ((getListValue() == null) ? 0 : getListValue().hashCode());
hashCode = prime * hashCode + ((getMapValue() == null) ? 0 : getMapValue().hashCode());
hashCode = prime * hashCode + ((getRelationshipValue() == null) ? 0 : getRelationshipValue().hashCode());
hashCode = prime * hashCode + ((getExpression() == null) ? 0 : getExpression().hashCode());
return hashCode;
}
@Override
public DataValue clone() {
try {
return (DataValue) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.iottwinmaker.model.transform.DataValueMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}